项目场景:
获取手机上手指滑动的距离超过一定距离 来操作一些逻辑
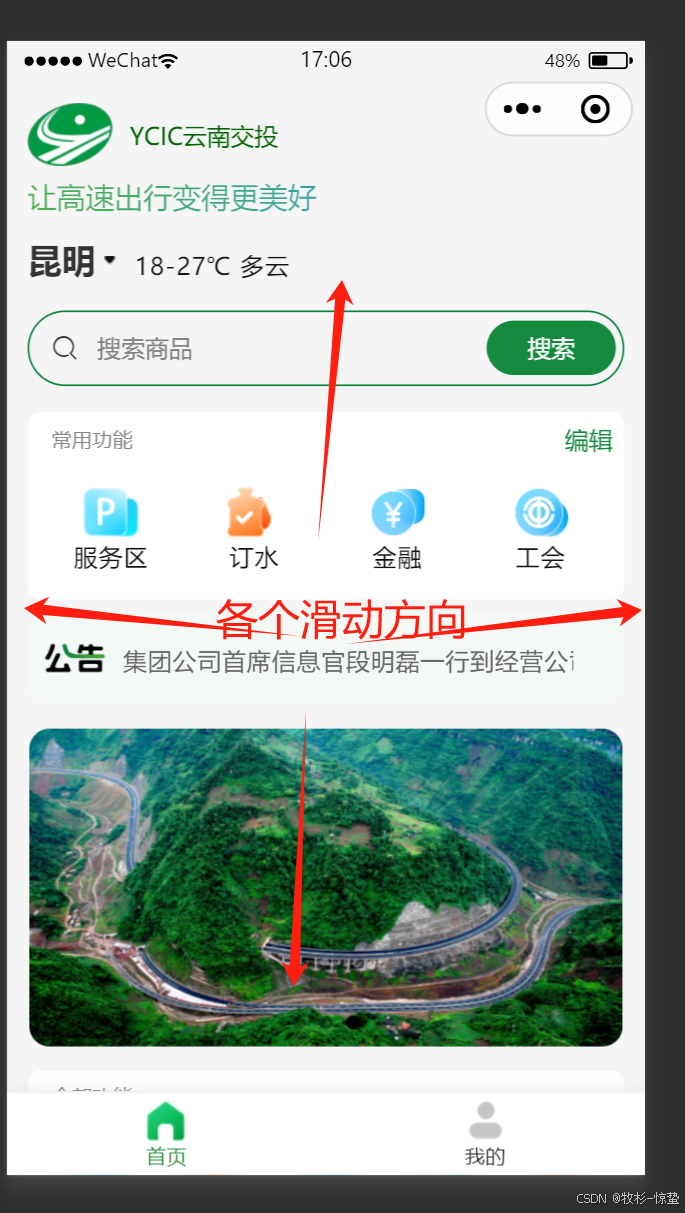
解决方案:
在uniapp中,可以通过监听触摸事件来判断滑动的方向。常用的触摸事件包括
touchstart
,touchmove
, 和touchend
。通过这些事件的参数,可以计算出用户的滑动起点和终点,进而判断滑动方向
touchStart
方法记录了触摸开始时的坐标,touchMove
方法在触摸移动时更新了坐标,touchEnd
方法则在触摸结束时计算出滑动的方向。getSwipeDirection
方法用于根据起点和终点的坐标判断出水平或垂直方向的滑动,如果水平方向的位移大于阈值,则判定为水平滑动;如果垂直方向的位移大于阈值,则判定为垂直滑动。
html
<template>
<view @touchstart="touchStart" @touchmove="touchMove" @touchend="touchEnd">
<!-- 滑动区域内容 -->
<view
v-for="(item,index) in 20"
:key="index"
style="border:1rpx solid #ccc;height:100rpx">
测试11111
</view>
</view>
</template>
<script>
export default {
data() {
return {
startX: 0, // 触摸开始的X坐标
startY: 0, // 触摸开始的Y坐标
endX: 0, // 触摸结束的X坐标
endY: 0, // 触摸结束的Y坐标
};
},
methods: {
touchStart(event) {
this.startX = event.touches[0].pageX;
this.startY = event.touches[0].pageY;
},
touchMove(event) {
// 移动时更新结束坐标
this.endX = event.touches[0].pageX;
this.endY = event.touches[0].pageY;
},
touchEnd(event) {
let direction = this.getSwipeDirection(
this.startX, this.startY, this.endX, this.endY
);
console.log('Swipe direction: ' + direction);
},
getSwipeDirection(startX, startY, endX, endY) {
const threshold = 30; // 设定一个阈值,用于判断是否是滑动
const deltaX = endX - startX;
const deltaY = endY - startY;
// 如果水平方向的位移大于垂直方向,且水平方向的位移大于阈值,则认为是水平滑动
if (Math.abs(deltaX) > Math.abs(deltaY) && Math.abs(deltaX) > threshold) {
return deltaX > 0 ? 'right' : 'left';
}
// 如果垂直方向的位移大于水平方向,且垂直方向的位移大于阈值,则认为是垂直滑动
else if (Math.abs(deltaY) > Math.abs(deltaX) && Math.abs(deltaY) > threshold) {
return deltaY > 0 ? 'down' : 'up';
}
// 其他情况视为无效滑动
return 'none';
},
},
};
</script>