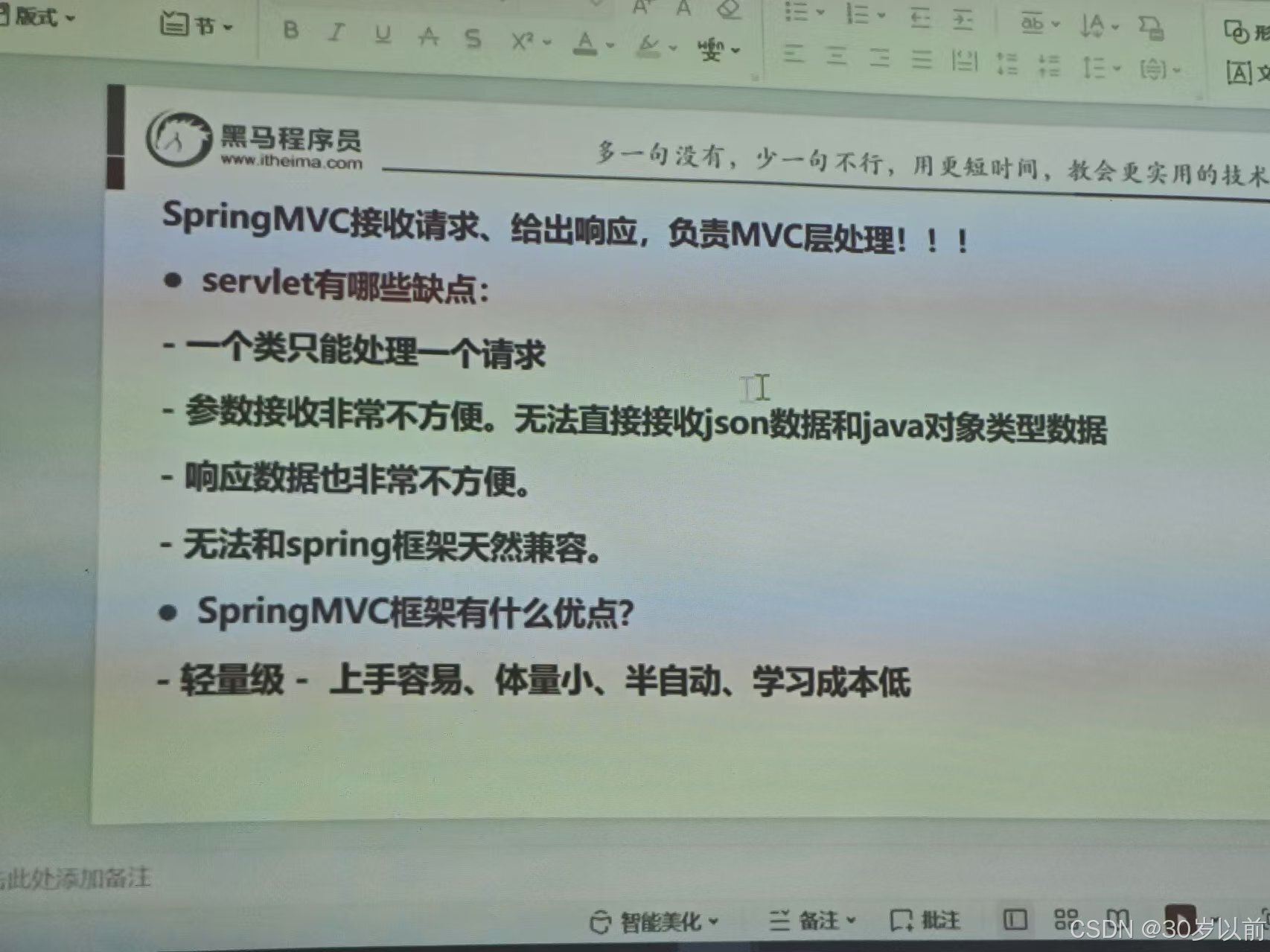
mvc思想
前端-controller - service - mapper - 数据库
view - controller - model
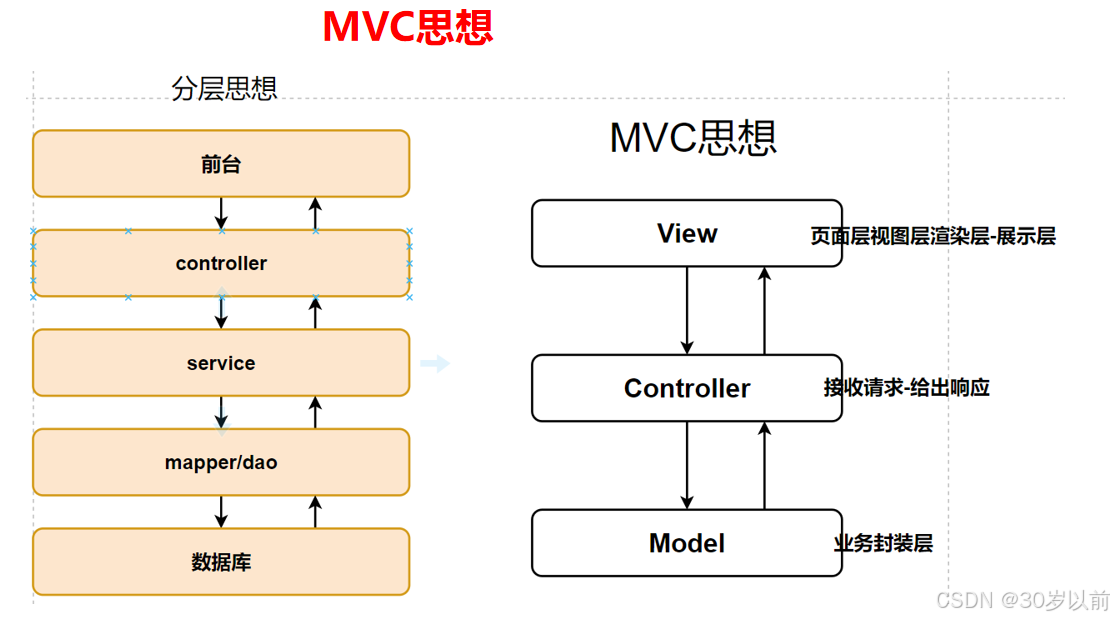
创建Springmvc入门案例
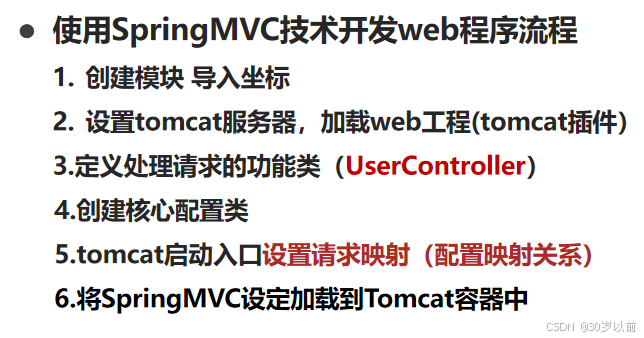
1.创建模块,导入坐标
<!-- 因为是tomcat方式所以打包方式用war包 -->
<packaging>war</packaging>
<!-- 集成依赖 -->
<dependencies>
<!-- servlet -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<!-- servlet包在tomcat中冲突 给他划定依赖范围-->
<scope>provided</scope>
</dependency>
<!-- spring-mvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.10.RELEASE</version>
</dependency>
<!-- 版本很容易出现问题 -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<!-- 配置插件 -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<configuration>
<!-- 端口号 -->
<port>8081</port>
<!-- 项目虚拟路径 -->
<path>/ssm</path>
<!-- get请求参数乱码 -->
<uriEncoding>utf8</uriEncoding>
</configuration>
</plugin>
</plugins>
</build>
下载插件,maven help 还有tomcat
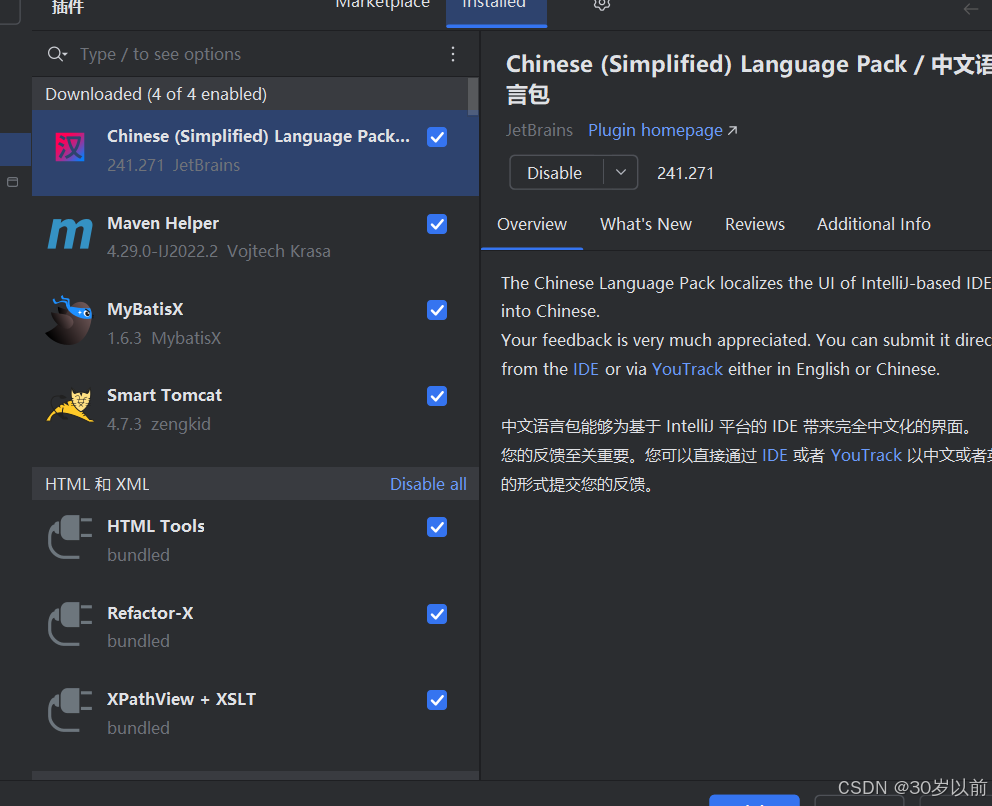
2.新建包和类
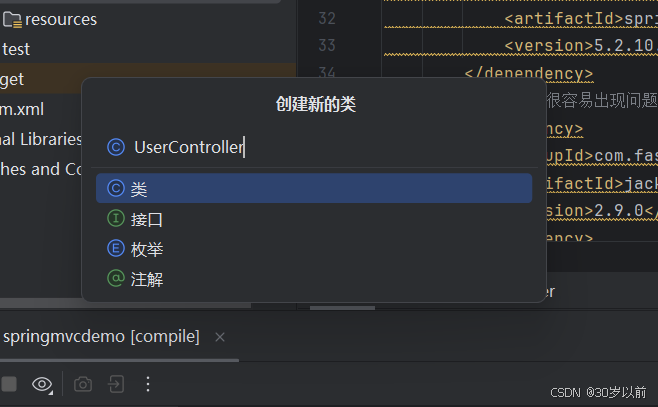
3.新建config配置类
SpringConfig
package com.heima.config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
//扫描包
@ComponentScan("com.heima")
//注明config配置类
@Configuration
public class SpringConfig {
}
SpringMvcConfig
package com.heima.config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
//写入注解
@Configuration
@ComponentScan("com.heima.controller")
public class SpringMvcConfig {
}
4.新建初始化启动类
package com.heima.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
//请求被spring容器管理
@Controller
//请求路径为user
@RequestMapping("/user")
//返回给前端
@ResponseBody
public class UserController {
//定义功能,写入注解
@RequestMapping(value = "/login",produces = "text/html;charset=utf-8")
public String login(HttpServletRequest req, HttpServletResponse res){
res.setContentType("text/html;charset=utf-8");
System.out.println("2022103718 张红");
return "张红 2022103752";
}
}
结果
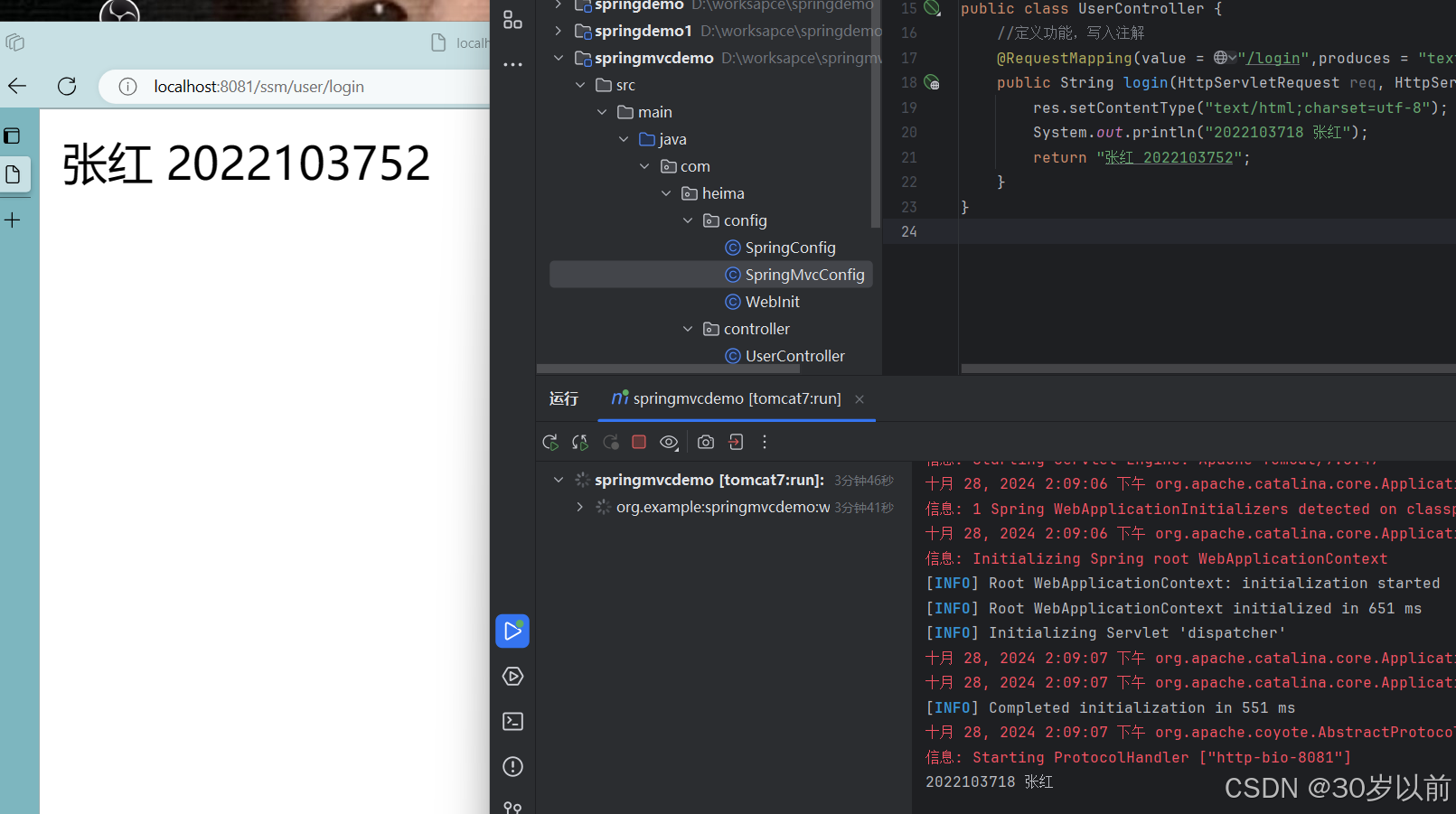