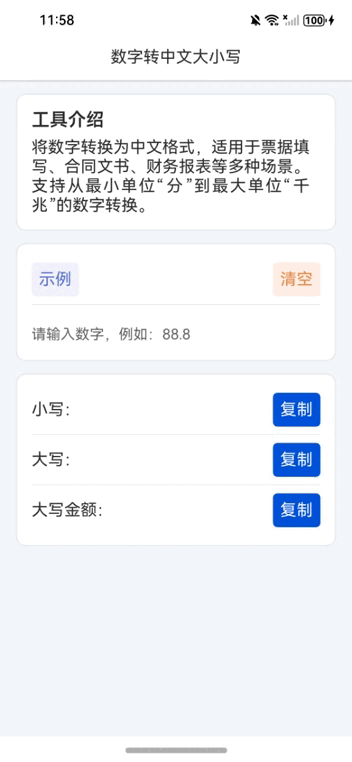
【引言】
本应用的主要功能是将用户输入的数字转换为中文的小写、大写及大写金额形式。用户可以在输入框中输入任意数字,点击"示例"按钮可以快速填充预设的数字,点击"清空"按钮则会清除当前输入。转换结果显示在下方的结果区域,每个结果旁边都有一个"复制"按钮,方便用户将结果复制到剪贴板。
【环境准备】
• 操作系统:Windows 10
• 开发工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
• 目标设备:华为Mate60 Pro
• 开发语言:ArkTS
• 框架:ArkUI
• API版本:API 12
• 三方库:chinese-number-format(数字转中文)、chinese-finance-number(将数字转换成财务用的中文大写数字)
ohpm install @nutpi/chinese-finance-number
ohpm install @nutpi/chinese-number-format
【功能实现】
• 输入监听:通过 @Watch 装饰器监听输入框的变化,一旦输入发生变化,即调用 inputChanged 方法更新转换结果。
• 转换逻辑:利用 @nutpi/chinese-number-format 和 @nutpi/chinese-finance-number 库提供的方法完成数字到中文的各种转换。
• 复制功能:使用 pasteboard 模块将结果显示的中文文本复制到剪贴板,通过 promptAction.showToast 提示用户复制成功。
【完整代码】
// 导入必要的模块
import { promptAction } from '@kit.ArkUI'; // 用于显示提示信息
import { pasteboard } from '@kit.BasicServicesKit'; // 用于处理剪贴板操作
import { toChineseNumber } from '@nutpi/chinese-finance-number'; // 将数字转换为中文大写金额
import {
toChineseWithUnits, // 将数字转换为带单位的中文
toUpperCase, // 将中文小写转换为大写
} from '@nutpi/chinese-number-format';
@Entry // 标记此组件为入口点
@Component // 定义一个组件
struct NumberToChineseConverter {
@State private exampleNumber: number = 88.8; // 示例数字
@State private textColor: string = "#2e2e2e"; // 文本颜色
@State private lineColor: string = "#d5d5d5"; // 分割线颜色
@State private basePadding: number = 30; // 基础内边距
@State private chineseLowercase: string = ""; // 转换后的小写中文
@State private chineseUppercase: string = ""; // 转换后的中文大写
@State private chineseUppercaseAmount: string = ""; // 转换后的中文大写金额
@State @Watch('inputChanged') private inputText: string = ""; // 监听输入文本变化
// 当输入文本改变时触发的方法
inputChanged() {
this.chineseLowercase = toChineseWithUnits(Number(this.inputText), 'zh-CN'); // 转换为小写中文并带上单位
this.chineseUppercase = toUpperCase(this.chineseLowercase, 'zh-CN'); // 将小写中文转换为大写
this.chineseUppercaseAmount = toChineseNumber(Number(this.inputText)); // 转换为大写金额
}
// 复制文本到剪贴板的方法
private copyToClipboard(text: string): void {
const pasteboardData = pasteboard.createData(pasteboard.MIMETYPE_TEXT_PLAIN, text); // 创建剪贴板数据
const systemPasteboard = pasteboard.getSystemPasteboard(); // 获取系统剪贴板
systemPasteboard.setData(pasteboardData); // 设置剪贴板数据
promptAction.showToast({ message: '已复制' }); // 显示复制成功的提示
}
// 构建用户界面的方法
build() {
Column() { // 主列容器
// 页面标题
Text('数字转中文大小写')
.fontColor(this.textColor) // 设置字体颜色
.fontSize(18) // 设置字体大小
.width('100%') // 设置宽度
.height(50) // 设置高度
.textAlign(TextAlign.Center) // 文本居中对齐
.backgroundColor(Color.White) // 设置背景颜色
.shadow({ // 添加阴影效果
radius: 2, // 阴影半径
color: this.lineColor, // 阴影颜色
offsetX: 0, // X轴偏移量
offsetY: 5 // Y轴偏移量
});
Scroll() { // 滚动视图
Column() { // 内部列容器
// 工具介绍部分
Column() {
Text('工具介绍').fontSize(20).fontWeight(600).fontColor(this.textColor); // 设置介绍文字样式
Text('将数字转换为中文格式,适用于票据填写、合同文书、财务报表等多种场景。支持从最小单位“分”到最大单位“千兆”的数字转换。')
.textAlign(TextAlign.JUSTIFY)
.fontSize(18).fontColor(this.textColor).margin({ top: `${this.basePadding / 2}lpx` }); // 设置介绍详情文字样式
}
.alignItems(HorizontalAlign.Start) // 对齐方式
.width('650lpx') // 设置宽度
.padding(`${this.basePadding}lpx`) // 设置内边距
.margin({ top: `${this.basePadding}lpx` }) // 设置外边距
.borderRadius(10) // 设置圆角
.backgroundColor(Color.White) // 设置背景颜色
.shadow({ // 添加阴影效果
radius: 10, // 阴影半径
color: this.lineColor, // 阴影颜色
offsetX: 0, // X轴偏移量
offsetY: 0 // Y轴偏移量
});
// 输入区
Column() {
Row() { // 行容器
Text('示例')
.fontColor("#5871ce") // 设置字体颜色
.fontSize(18) // 设置字体大小
.padding(`${this.basePadding / 2}lpx`) // 设置内边距
.backgroundColor("#f2f1fd") // 设置背景颜色
.borderRadius(5) // 设置圆角
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 设置点击效果
.onClick(() => { // 点击事件
this.inputText = `${this.exampleNumber}`; // 设置输入框文本为示例数字
});
Blank(); // 占位符
Text('清空')
.fontColor("#e48742") // 设置字体颜色
.fontSize(18) // 设置字体大小
.padding(`${this.basePadding / 2}lpx`) // 设置内边距
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 设置点击效果
.backgroundColor("#ffefe6") // 设置背景颜色
.borderRadius(5) // 设置圆角
.onClick(() => { // 点击事件
this.inputText = ""; // 清空输入框
});
}.height(45) // 设置高度
.justifyContent(FlexAlign.SpaceBetween) // 子元素水平分布方式
.width('100%'); // 设置宽度
Divider().margin({ top: 5, bottom: 5 }); // 分割线
TextInput({ text: $$this.inputText, placeholder: `请输入数字,例如:${this.exampleNumber}` }) // 输入框
.width('100%') // 设置宽度
.fontSize(18) // 设置字体大小
.caretColor(this.textColor) // 设置光标颜色
.fontColor(this.textColor) // 设置字体颜色
.margin({ top: `${this.basePadding}lpx` }) // 设置外边距
.padding(0) // 设置内边距
.backgroundColor(Color.Transparent) // 设置背景颜色
.borderRadius(0) // 设置圆角
.type(InputType.NUMBER_DECIMAL); // 设置输入类型为数字
}
.alignItems(HorizontalAlign.Start) // 对齐方式
.width('650lpx') // 设置宽度
.padding(`${this.basePadding}lpx`) // 设置内边距
.margin({ top: `${this.basePadding}lpx` }) // 设置外边距
.borderRadius(10) // 设置圆角
.backgroundColor(Color.White) // 设置背景颜色
.shadow({ // 添加阴影效果
radius: 10, // 阴影半径
color: this.lineColor, // 阴影颜色
offsetX: 0, // X轴偏移量
offsetY: 0 // Y轴偏移量
});
// 结果区
Column() {
Row() {
Text(`小写:${this.chineseLowercase}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 显示小写结果
Text('复制')
.fontColor(Color.White) // 设置字体颜色
.fontSize(18) // 设置字体大小
.padding(`${this.basePadding / 2}lpx`) // 设置内边距
.backgroundColor("#0052d9") // 设置背景颜色
.borderRadius(5) // 设置圆角
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 设置点击效果
.onClick(() => { // 点击事件
this.copyToClipboard(this.chineseLowercase); // 复制小写结果到剪贴板
});
}.constraintSize({ minHeight: 45 }) // 最小高度
.justifyContent(FlexAlign.SpaceBetween) // 子元素水平分布方式
.width('100%'); // 设置宽度
Divider().margin({ top: 5, bottom: 5 }); // 分割线
Row() {
Text(`大写:${this.chineseUppercase}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 显示大写结果
Text('复制')
.fontColor(Color.White) // 设置字体颜色
.fontSize(18) // 设置字体大小
.padding(`${this.basePadding / 2}lpx`) // 设置内边距
.backgroundColor("#0052d9") // 设置背景颜色
.borderRadius(5) // 设置圆角
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 设置点击效果
.onClick(() => { // 点击事件
this.copyToClipboard(this.chineseUppercase); // 复制大写结果到剪贴板
});
}.constraintSize({ minHeight: 45 }) // 最小高度
.justifyContent(FlexAlign.SpaceBetween) // 子元素水平分布方式
.width('100%'); // 设置宽度
Divider().margin({ top: 5, bottom: 5 }); // 分割线
Row() {
Text(`大写金额:${this.chineseUppercaseAmount}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 显示大写金额结果
Text('复制')
.fontColor(Color.White) // 设置字体颜色
.fontSize(18) // 设置字体大小
.padding(`${this.basePadding / 2}lpx`) // 设置内边距
.backgroundColor("#0052d9") // 设置背景颜色
.borderRadius(5) // 设置圆角
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 设置点击效果
.onClick(() => { // 点击事件
this.copyToClipboard(this.chineseUppercaseAmount); // 复制大写金额结果到剪贴板
});
}.constraintSize({ minHeight: 45 }) // 最小高度
.justifyContent(FlexAlign.SpaceBetween) // 子元素水平分布方式
.width('100%'); // 设置宽度
}
.alignItems(HorizontalAlign.Start) // 对齐方式
.width('650lpx') // 设置宽度
.padding(`${this.basePadding}lpx`) // 设置内边距
.margin({ top: `${this.basePadding}lpx` }) // 设置外边距
.borderRadius(10) // 设置圆角
.backgroundColor(Color.White) // 设置背景颜色
.shadow({ // 添加阴影效果
radius: 10, // 阴影半径
color: this.lineColor, // 阴影颜色
offsetX: 0, // X轴偏移量
offsetY: 0 // Y轴偏移量
});
}
}.scrollBar(BarState.Off).clip(false); // 关闭滚动条,不允许裁剪
}
.height('100%') // 设置高度
.width('100%') // 设置宽度
.backgroundColor("#f4f8fb"); // 设置页面背景颜色
}
}