1. HTTP协议
1.1 基本介绍
- HTTP协议又称超文本传输协议,属于应用层协议,在传输层使用TCP协议。
- HTTP协议属是无状态的,对事务处理没有记忆能力,如果需要保存状态需要引用其他技术,如Cookie。
- HTTP协议属是无连接的,每次连接只处理一个请求。因为早期带宽和计算资源有限,这么做是为了加快传输速度,后来通过Connection:Keep-Alive实现了长连接。
- http1.1废弃了Keep-Alive,默认支持长连接。
1.2 http requst(请求报文)
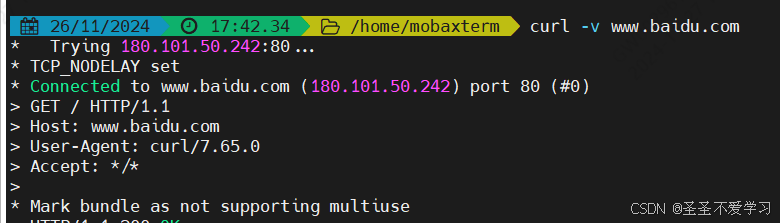
(1)请求行:GET / HTTP/1.1
- GET:请求方法。
- /:URL(请求路径)
- 协议版本:HTTP/1.1
(2)请求头
- Host: www.baidu.com:告诉服务器请求的目标地址。
- User-Agent: curl/7.65.0:表明请求是由curl工具发起的,版本是7.65.0。
- Accept: */*:表示客户端可以接受任何类型的响应内容。
如果是POST请求,那么请求头中的内容更多。如:
- Accept-Language: en-US,en:客户端可接受的语言。
- **
Accept-Encoding: gzip, deflate, br:
**客户端能够处理的压缩格式。- Authorization: Bearer token_value:如果需要认证,此处包含认证(证书)信息。
- Cache-Control: no-cache:指定请求和响应遵循的缓存机制。no-cache表示无缓存机制。
- 等等其他内容。
(3)空行
就Accept: */*下面那个空行。
(4)请求正文
注意:GET请求,是没有请求正文的。举例一个POST请求:
name=John+Doe&age=30,这就是POST请求的请求主正文。
1.3 请求方法介绍
|----------|----------------------------------------|
| 请求方法 | 含义 |
| GET | 请求获取Request-URI所标识的资源,如下载一张图片。 |
| POST | 向服务器提交数据进行处理,比如提交表单或者上传文件。数据通常放在请求正文中。 |
| HEAD | 类似于GET,但是返回的响应中没有具体的内容。主要用于获取响应头部。 |
| PUT | 上传文件或提交资源到服务器,通常指定了资源的URI。 |
| DELETE | 请求服务器删除指定的页面。 |
| OPTIONS | 查看服务端的性能。 |
| TRACE | 类似于链路追踪,可以看到请求经过了哪些节点以及耗时等。 |
| PATCH | 类似PUT,但它可以只对资源的一部分进行更新,资源不存在时则创建。 |
[ ]
注意:
- 实际工作中,服务端对各种请求方法的处理方式可能不是按照我们标准的协议来的,比如服务端收到的是PUT请求,但执行的是删除操作,具体还要看开发者怎么定义。
- 大多数浏览器只支持GET和POST。
1.4 http response(响应报文)
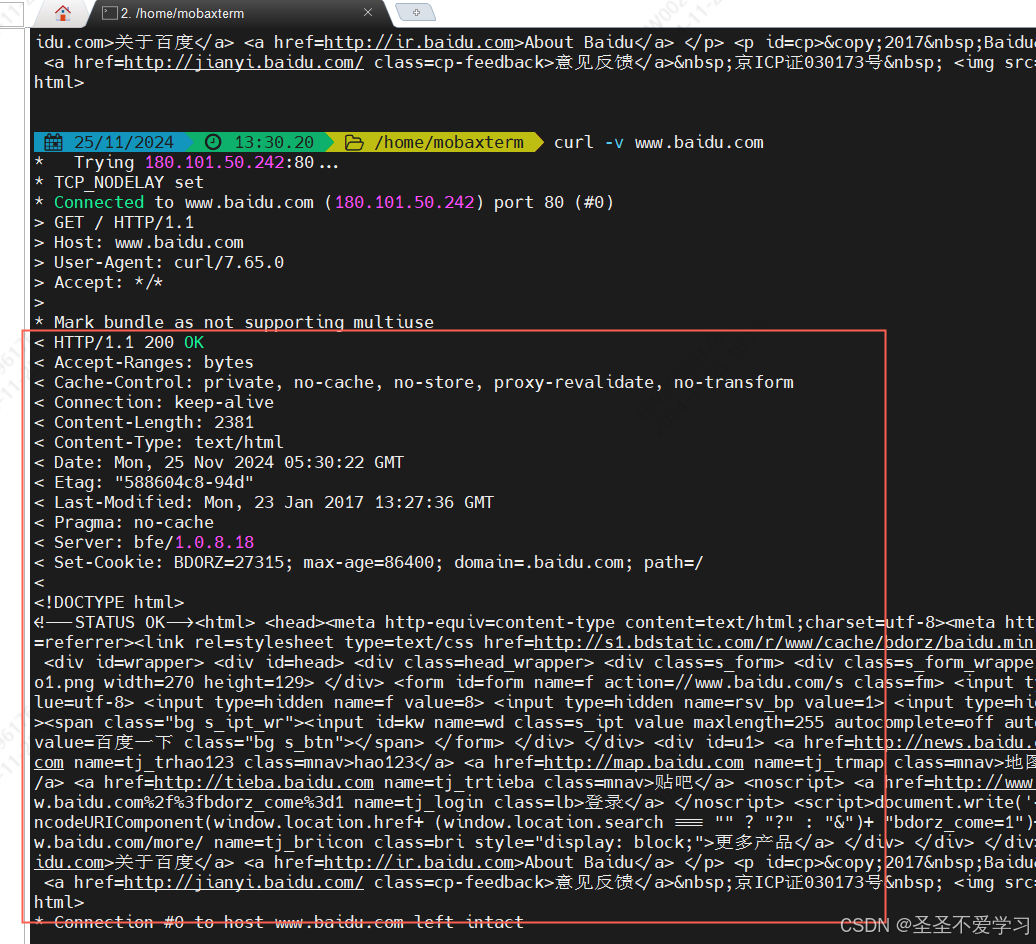
(1)响应状态行:HTTP/1.1 200 OK
- HTTP/1.1:表示请求协议版本。
- 200:表示请求状态码。
- OK:表示状态消息。
(2)响应头
- Accept-Ranges: bytes:表示服务器能够接受以字节为单位的范围请求。
- Cache-Control:指示响应不能被缓存。
- Connection: keep-alive:表示这个TCP连接在发送完响应后不会关闭,可以被重用。
- Content-Length: 2381:响应体的长度是2381字节。
- Content-Type: text/html:响应体的类型是HTML。
- Date:响应生成的日期和时间。
- Etag:资源的一个特定版本的标识。
- Last-Modified:资源最后被修改的日期和时间。
- Pragma: no-cache:一个指令,要求请求和响应遵循HTTP/1.0的缓存机制,不要缓存。
- Server: bfe/1.0.8.18:服务器使用的软件信息。
- Set-Cookie:服务器设置了一个名为BDOZ的cookie,有效期为86400秒,域名为.baidu.com。
(3)空行
(4)响应报文主体
- <!DOCTYPE html> ... </html>
1.5 URL和URI
1.5.1 基本介绍
- URI :统一资源标识符(Uniform Resource Identifier),是一个用于标识资源的字符串。它提供了一种方式,通过该方式可以唯一地标识互联网上的资源,但没有提供访问方式。
- URL :统一资源定位符(Uniform Resource Locator),是URI的一种,用于定位资源。它不仅标识资源,还提供了如何通过互联网访问该资源的具体信息。
1.5.2 示例
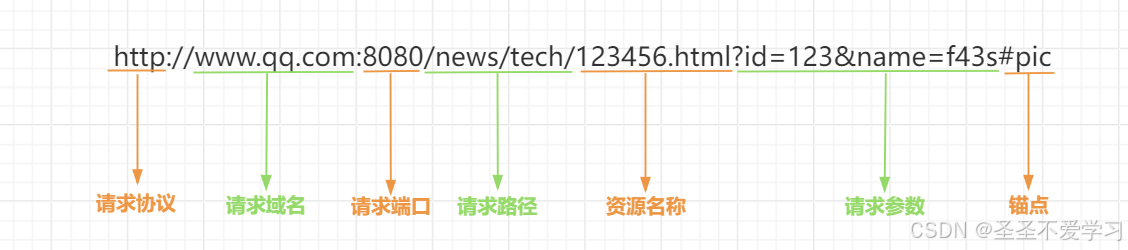
2. go语言http标准库
在go中实现http编程,主要是使用go包中的net/http。
net:net包为网络I/O提供了一个可移植的接口,包括TCP/IP、UDP、域名解析和Unix域套接字。
- http:http 包提供了 HTTP 客户端和服务器实现。
- 其余协议参考官网。
2.1 GET请求
2.1.1 http服务端
2.1.1.1 编辑代码
Go
package main
import (
"fmt"
"log"
"net/http"
)
// w http.ResponseWriter, r *http.Request: 为固定写法
func BoyHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hello Boy")
}
func GirlHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hello Girl")
}
func main() {
// 定义路由
// 请求"/boy"路径,就调用BoyHandler函数
http.HandleFunc("/boy", BoyHandler)
http.HandleFunc("/gir", GirlHandler)
// 服务启动后的端口号
err := http.ListenAndServe(":5656", nil) // 注意:成功启动的话,就会一直阻塞(没有输出)。
if err != nil {
log.Panic(err)
}
}
2.1.1.2 浏览器请求服务端
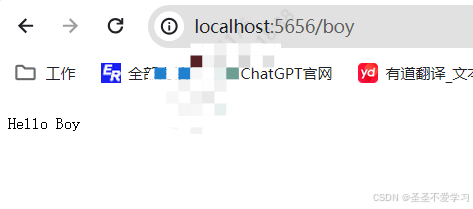
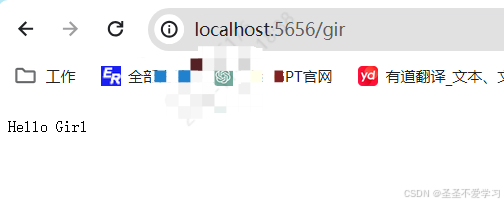
2.1.2 http客户端
2.1.2.1 编辑代码并发起GET请求
Go
package main
import (
"io"
"log"
"net/http"
"os"
)
func get() {
// 定义一个GET请求
r, err := http.Get("http://localhost:5656/gir")
if err != nil {
log.Panic(err)
}
defer r.Body.Close() // 用完关闭,否则会协程泄露
// 因为Body方法没有办法直接打印出来,所以把它复制到标准输出。
io.Copy(os.Stdout, r.Body)
}
func main() {
get()
}
============调试结果============
Hello Girl
2.1.3 增加其他http信息
2.1.3.1 服务端
Go
package main
import (
"fmt"
"log"
"net/http"
)
// w http.ResponseWriter, r *http.Request: 为固定写法
func BoyHandler(w http.ResponseWriter, r *http.Request) {
// 显示请求头
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
fmt.Fprint(w, "Hello Boy\n")
}
func GirlHandler(w http.ResponseWriter, r *http.Request) {
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
fmt.Fprint(w, "Hello Girl\n")
}
func main() {
http.HandleFunc("/boy", BoyHandler)
http.HandleFunc("/gir", GirlHandler)
err := http.ListenAndServe(":5656", nil)
if err != nil {
log.Panic(err)
}
}
2.1.3.2 客户端
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
)
func get() {
r, err := http.Get("http://localhost:5656/boy")
if err != nil {
log.Panic(err)
}
defer r.Body.Close()
io.Copy(os.Stdout, r.Body)
// 显示响应头
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
// 显示请求协议
fmt.Printf("Proto:%v\n", r.Proto)
fmt.Printf("Close: %v\n", r.Close)
// 显示请求内容长度
fmt.Printf("ContentLength: %v\n", r.ContentLength)
// 请求协议主版本号
fmt.Printf("ProtoMajor: %+v\n", r.ProtoMajor)
// 请求协议次版本号
fmt.Printf("ProtoMinor: %+v\n", r.ProtoMinor)
// 原始请求
fmt.Printf("Request: %+v\n", r.Request)
// 请求状态,含状态码和OK与否
fmt.Printf("Status: %+v\n", r.Status)
// 请求状态码
fmt.Printf("StatusCode: %+v\n", r.StatusCode)
// 请求方法
fmt.Printf("Request.Method: %+v\n", r.Request.Method)
// 请求地址
fmt.Printf("%+v\n", r.Request.URL)
// 还有很多内容,这里只展示部分。
}
func main() {
get()
}
2.1.4 请求测试
2.1.4.1 客户端
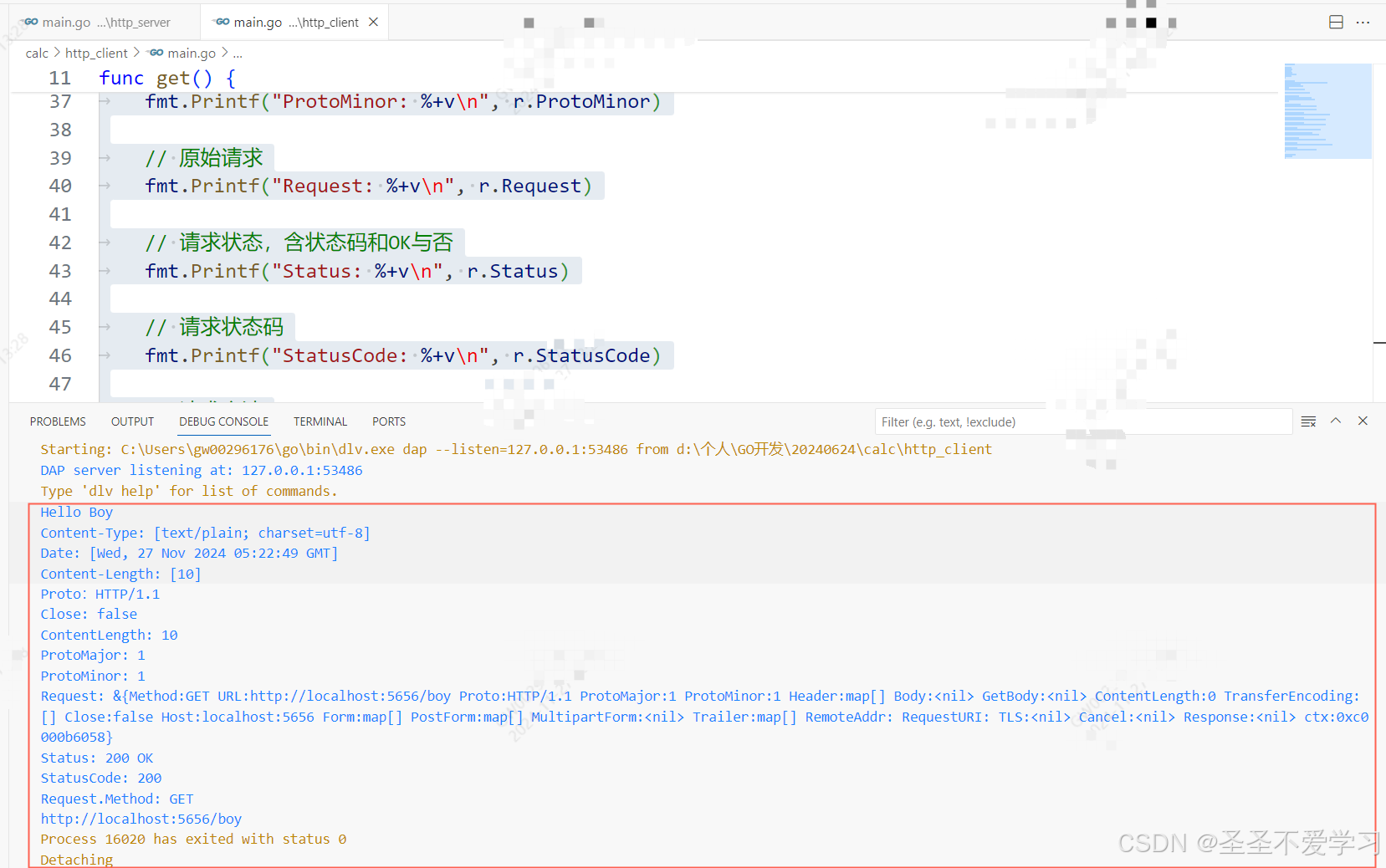
2.1.4.2 服务端
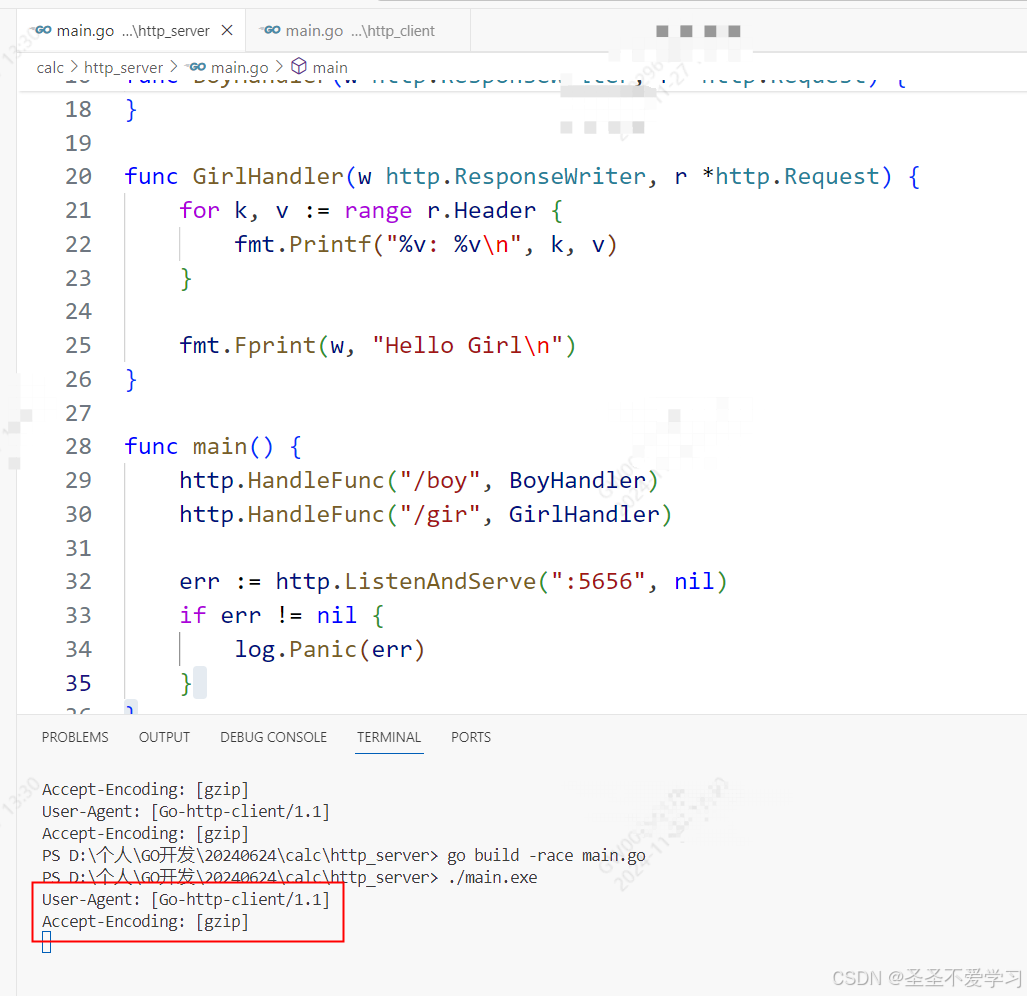
2.2 POST请求
2.2.1 服务端代码
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
)
func BoyHandler(w http.ResponseWriter, r *http.Request) {
// 关闭请求主体
defer r.Body.Close()
// 显示请求主体
io.Copy(os.Stdout, r.Body)
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
fmt.Fprint(w, "Hello Boy\n")
}
func GirlHandler(w http.ResponseWriter, r *http.Request) {
defer r.Body.Close()
// 显示请求主体
io.Copy(os.Stdout, r.Body)
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
fmt.Fprint(w, "Hello Girl\n")
}
func main() {
http.HandleFunc("/boy", BoyHandler)
http.HandleFunc("/gir", GirlHandler)
err := http.ListenAndServe(":5656", nil)
if err != nil {
log.Panic(err)
}
}
2.2.2 客户端代码
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
"strings"
)
func get() {
r, err := http.Get("http://localhost:5656/boy")
if err != nil {
log.Panic(err)
}
defer r.Body.Close()
io.Copy(os.Stdout, r.Body)
// 显示响应头
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
}
func post() {
// 请求服务端要发送的内容(请求主体)
r := strings.NewReader("hello server\n")
// 构建post请求
r2, err := http.Post("http://localhost:5656/boy", "text/plain", r)
if err != nil {
log.Panic(err)
}
defer r2.Body.Close()
io.Copy(os.Stdout, r2.Body)
for k, v := range r2.Header {
fmt.Printf("%v: %v\n", k, v)
}
}
func main() {
post()
}
2.2.3 请求测试
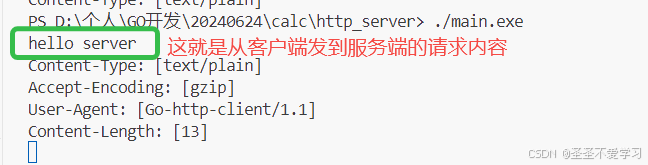
2.3 POST请求进阶版
使用函数:
- http.NewRequest(method string, url string, body io.Reader) (*http.Request, error)
参数:
- method string:请求的方法,比如 "GET"、"POST"、"PUT"、"DELETE" 等。
- url string:请求的 URL,可以是一个完整的 URL 字符串。
- body io.Reader:请求的主体(body),它是一个
io.Reader
接口,可以是nil
,表示没有请求体。返回值:
- *http.Request:一个新建的 HTTP 请求对象。
- error:如果在创建请求的过程中出现错误,这个错误对象会被返回,否则为
nil
。
2.3.1 服务端代码编辑
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
)
func BoyHandler(w http.ResponseWriter, r *http.Request) {
// 关闭请求主体
defer r.Body.Close()
// 显示请求主体
io.Copy(os.Stdout, r.Body)
// 显示请求头
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
// 显示Cookies
for _, cokkie := range r.Cookies() {
fmt.Printf("%v: %v\n", cokkie.Name, cokkie.Value)
}
fmt.Fprint(w, "Hello Boy\n")
}
func GirlHandler(w http.ResponseWriter, r *http.Request) {
defer r.Body.Close()
// 显示请求主体
io.Copy(os.Stdout, r.Body)
for k, v := range r.Header {
fmt.Printf("%v: %v\n", k, v)
}
fmt.Fprint(w, "Hello Girl\n")
}
func main() {
http.HandleFunc("/boy", BoyHandler)
http.HandleFunc("/gir", GirlHandler)
err := http.ListenAndServe(":5656", nil)
if err != nil {
log.Panic(err)
}
}
2.3.2 客户端代码编辑
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
"strings"
"time"
)
func complexHttpRequest() {
reader := strings.NewReader("hello server\n")
req, err := http.NewRequest("POST", "http://localhost:5656/boy", reader)
if err != nil {
log.Panic(err)
} else {
// 增加自定义请求头
req.Header.Add("User-Agent", "中国")
req.Header.Add("MyHeaderKey", "MyHeaderValue")
// 增加自定义Cokkie
req.AddCookie(&http.Cookie{
// 传给服务端的信息
Name: "auth",
Value: "passwd",
// 下面这3行信息,主要是作为记录,并不传给服务端
Path: "/",
Domain: "localhost",
Expires: time.Now().Add(time.Duration(time.Hour)),
})
client := &http.Client{
// Timeout: 100 * time.Microsecond, // 客户端等待服务端响应的超时时间为100毫秒
Timeout: 10 * time.Second, // 客户端等待服务端响应的超时时间为10秒
}
// 提交http请求
resp, err2 := client.Do(req)
if err2 != nil {
log.Panic(err2)
} else {
// 成功提交请求且拿到响应后,第一件事就是关闭请求
defer resp.Body.Close()
// 显示响应内容
io.Copy(os.Stdout, resp.Body)
for k, v := range resp.Header {
fmt.Printf("%s: %v\n", k, v)
}
fmt.Println(resp.Proto)
fmt.Println(resp.Status)
}
}
}
func main() {
complexHttpRequest()
}
2.3.3 请求测试
2.3.3.1 客户端请求
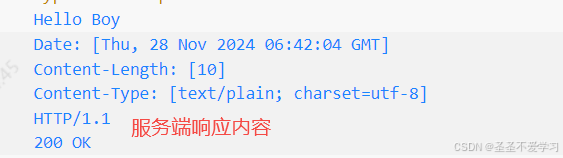
2.3.3.2 服务端
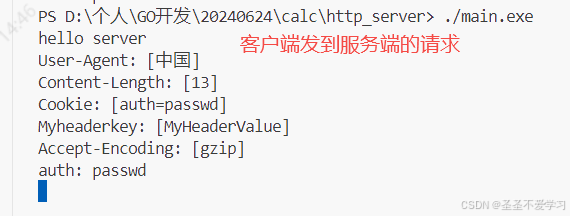
3. http router
3.1 基本介绍
http router 是 Go语言 中用于处理 HTTP请求 的组件。它能够识别 URL 和 HTTP方法(如GET、POST),并将请求分发到对应的处理函数。
- 下载:go get -u github.com/julienschmidt/httprouter
- Router实现了http.Handler接口。
- 为各种request method提供了便捷的路由方式。
- 支持restful请求方式。
- 支持ServerFiles访问静态文件(如html)。
- 可以自定义捕获panic的方法。
3.2 编辑服务端代码
Go
package main
import (
"fmt"
"io"
"net/http"
"os"
"github.com/julienschmidt/httprouter"
)
func handle(method string, w http.ResponseWriter, r *http.Request) {
fmt.Printf("request method: %v\n", r.Method)
fmt.Print("request boy: \n")
io.Copy(os.Stdout, r.Body)
// defer r.Body.Close()
// fmt.Fprint(w, "Hello boy")
// 或者(上下等价)
w.Write([]byte("Hello boy, your request method is " + method))
}
func get(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("GET", w, r)
}
func post(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("POST", w, r)
}
func main() {
// 定义一个router
router := httprouter.New()
router.GET("/", get)
router.POST("/", post)
// 服务启动后监听的端口号
http.ListenAndServe(":5656", router)
}
3.3 测试
3.3.1 GET方法测试
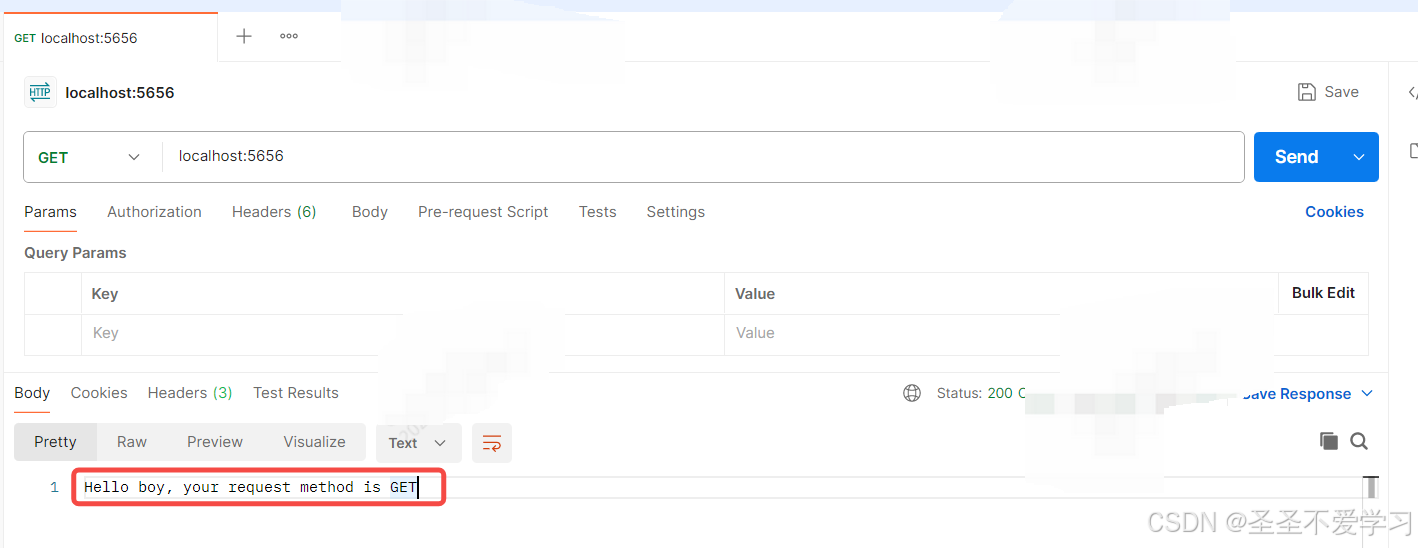
3.3.2 POST方法测试
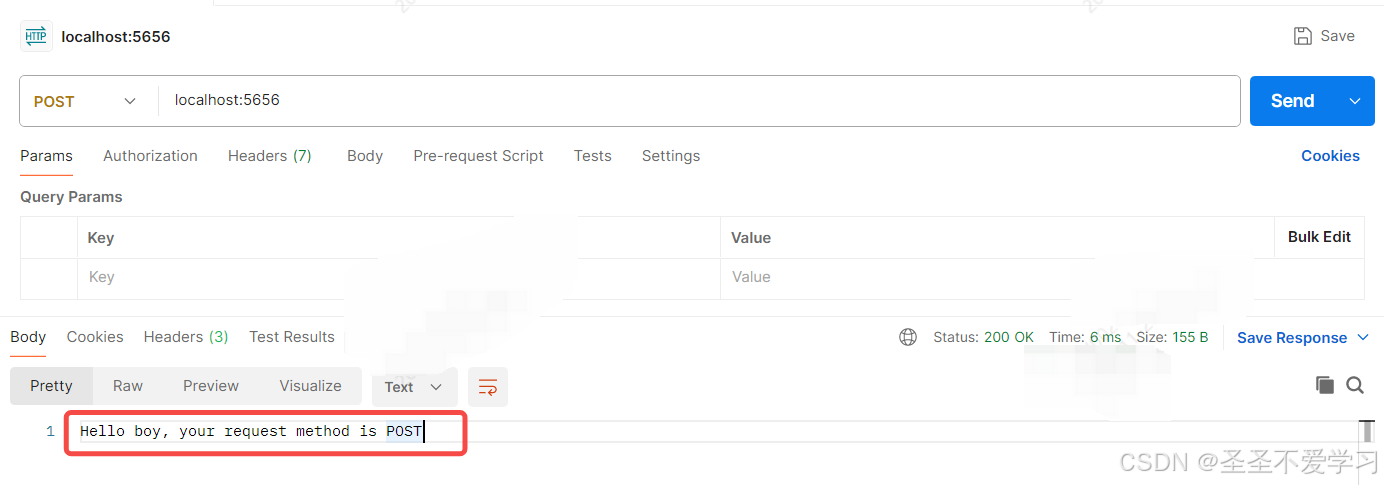
3.4 RESTful风格(POST传参)
3.4.1 服务端代码
Go
package main
import (
"fmt"
"io"
"net/http"
"os"
"github.com/julienschmidt/httprouter"
)
func handle(method string, w http.ResponseWriter, r *http.Request) {
fmt.Printf("request method: %v\n", r.Method)
// fmt.Print("request boy: \n")
io.Copy(os.Stdout, r.Body)
// defer r.Body.Close()
// fmt.Fprint(w, "Hello boy")
// 或者(上下等价)
w.Write([]byte("Hello boy, your request method is " + method))
}
func get(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("GET", w, r)
}
func post(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("POST", w, r)
}
func main() {
// 定义一个router
router := httprouter.New()
router.GET("/", get)
router.POST("/", post)
// /user:是我们请求的路径。
// :name:占位符,假定为请求时传递的用户名(参数)
// :type:占位符,假定为请求时传递的类型(参数)
// *addr:占位符,*表示可以匹配多级路径(参数)
router.POST("/user/:name/:type/*addr", func(w http.ResponseWriter, r *http.Request, p httprouter.Params) {
fmt.Printf("name: %v\ntype: %v\naddr: %v", p.ByName("name"), p.ByName("type"), p.ByName("addr"))
})
// 服务启动后监听的端口号
http.ListenAndServe(":5656", router)
}
3.4.2 客户端代码
Go
package main
import (
"fmt"
"io"
"log"
"net/http"
"os"
"strings"
"time"
)
func complexHttpRequest() {
reader := strings.NewReader("hello server\n")
req, err := http.NewRequest("POST", "http://localhost:5656/user/sanhua/vip/shahnghai/changning/GDAS", reader)
if err != nil {
log.Panic(err)
} else {
// 增加自定义请求头
req.Header.Add("User-Agent", "中国")
req.Header.Add("MyHeaderKey", "MyHeaderValue")
// 增加自定义Cokkie
req.AddCookie(&http.Cookie{
// 传给服务端的信息
Name: "auth",
Value: "passwd",
// 下面这3行信息,主要是作为记录,并不传给服务端
Path: "/",
Domain: "localhost",
Expires: time.Now().Add(time.Duration(time.Hour)),
})
client := &http.Client{
// Timeout: 100 * time.Microsecond, // 客户端等待服务端响应的超时时间为100毫秒
Timeout: 10 * time.Second, // 客户端等待服务端响应的超时时间为10秒
}
// 提交http请求
resp, err2 := client.Do(req)
if err2 != nil {
log.Panic(err2)
} else {
// 成功提交请求且拿到响应后,第一件事就是关闭请求
defer resp.Body.Close()
// 显示响应内容
io.Copy(os.Stdout, resp.Body)
for k, v := range resp.Header {
fmt.Printf("%s: %v\n", k, v)
}
fmt.Println(resp.Proto)
fmt.Println(resp.Status)
}
}
}
func main() {
complexHttpRequest()
}
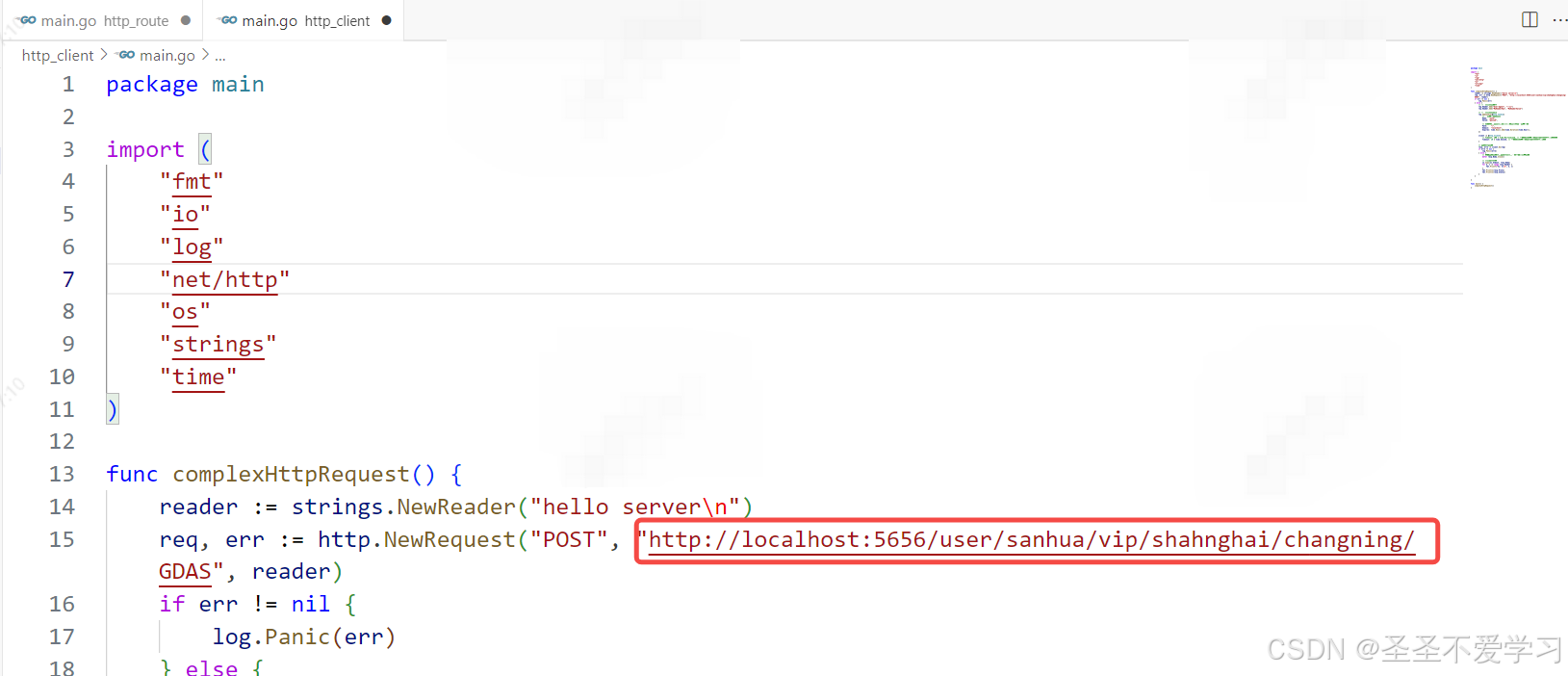
3.4.3 请求测试
3.4.3.1 客户端
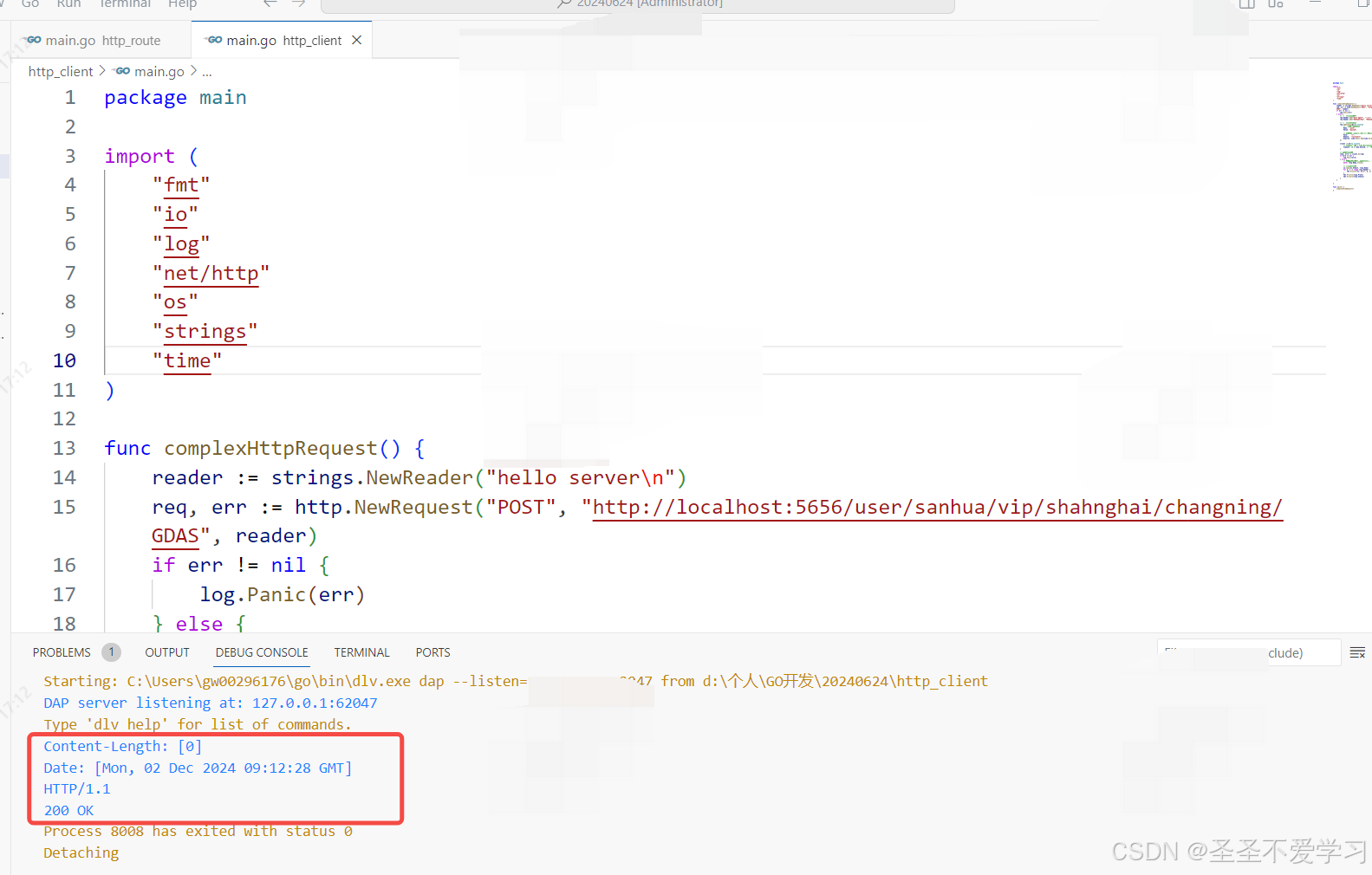
3.4.3.2 服务端
下图可以看到,服务端把客户端请求时的传参,提取出来了。
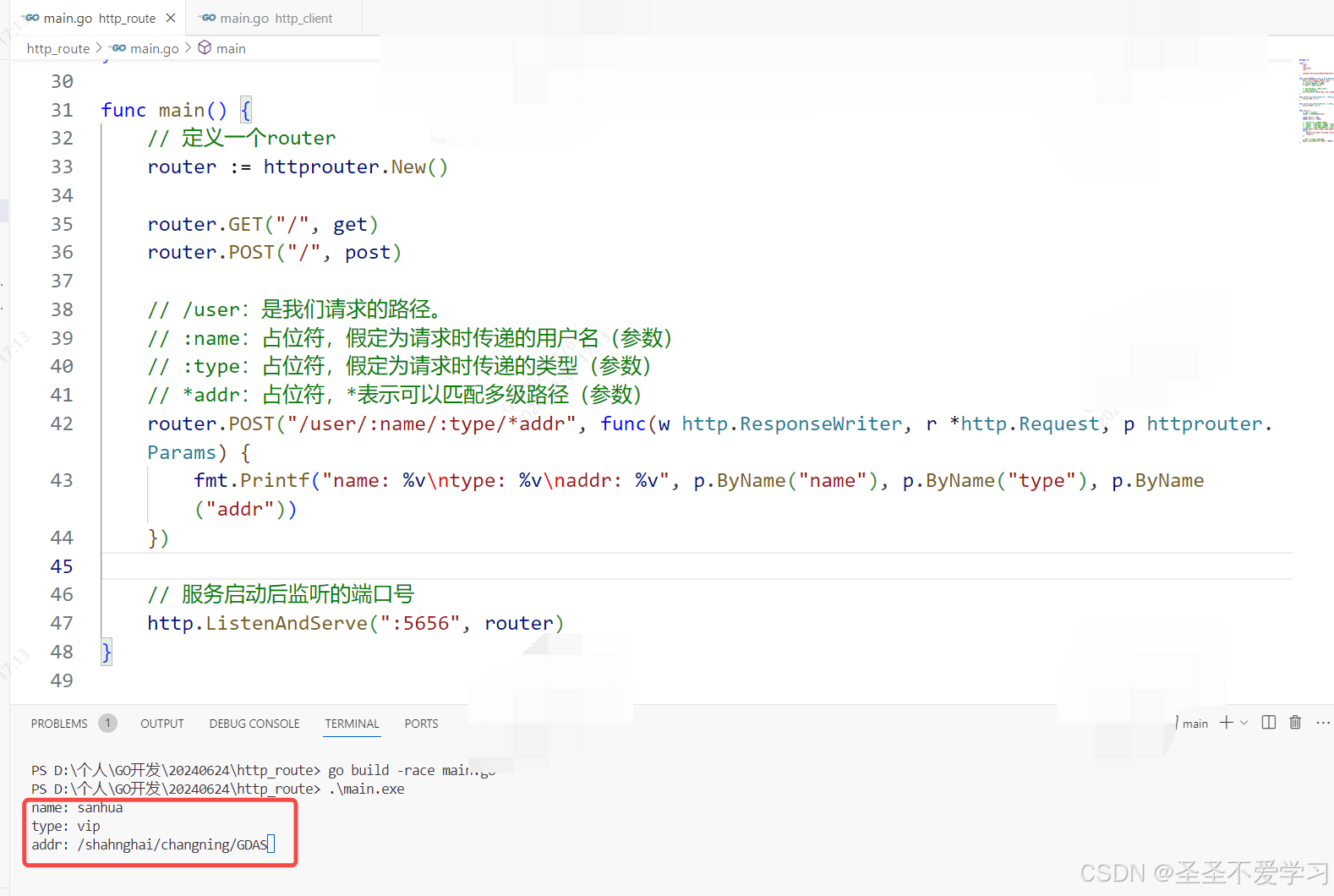
3.5 指定静态html文件
3.5.1 准备html文件
这是我在网上随便找的一个静态页面
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
* {
margin: 0;
padding: 0;
}
html {
height: 100%;
}
body {
height: 100%;
}
.container {
height: 100%;
background-image: linear-gradient(to right, #999999, #330867);
}
.login-wrapper {
background-color: bisque;
width: 358px;
height: 588px;
border-radius: 15px;
padding: 0 50px;
position: relative;
left: 50%;
top: 50%;
transform: translate(-50%,-50%);
}
.header {
font-size: 38px;
font-weight: bold;
text-align: center;
line-height: 200px;
}
.input-item {
display: block;
width: 100%;
margin-bottom: 20px;
border: 0;
padding: 10px;
border-bottom: 1px solid rgb(128,125,125);
font-size: 15px;
outline: none;
}
.input-item::placeholder {
text-transform: uppercase;
}
.btn {
text-align: center;
padding: 10px;
width: 100%;
margin-top: 40px;
background-image: linear-gradient(to right,#a6c1ee, #fbc2eb);
color: #fff;
}
.msg {
text-align: center;
line-height: 88px;
}
a {
text-decoration-line: none;
color: #abc1ee;
}
</style>
</head>
<body>
<div class="container">
<div class="login-wrapper">
<div class="header">Login</div>
<div class="form-wrapper">
<input type="text" name="username" placeholder="username" class="input-item">
<input type="password" name="password" placeholder="password" class="input-item">
<div class="btn">Login</div>
</div>
<div class="msg">
Don't have account?
<a href="#">Sign up</a>
</div>
</div>
</div>
</body>
</html>
3.5.2 服务端代码
Go
package main
import (
"fmt"
"io"
"net/http"
"os"
"github.com/julienschmidt/httprouter"
)
func handle(method string, w http.ResponseWriter, r *http.Request) {
fmt.Printf("request method: %v\n", r.Method)
// fmt.Print("request boy: \n")
io.Copy(os.Stdout, r.Body)
// defer r.Body.Close()
// fmt.Fprint(w, "Hello boy")
// 或者(上下等价)
w.Write([]byte("Hello boy, your request method is " + method))
}
func get(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("GET", w, r)
}
func post(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("POST", w, r)
}
func main() {
// 定义一个router
router := httprouter.New()
router.GET("/", get)
router.POST("/", post)
// 请求/file路径,服务端就会去static目录下读取a.html文件中的内容
router.ServeFiles("/file/*filepath", http.Dir("D:/个人/GO开发/20240624/static"))
// 服务启动后监听的端口号
http.ListenAndServe(":5656", router)
}
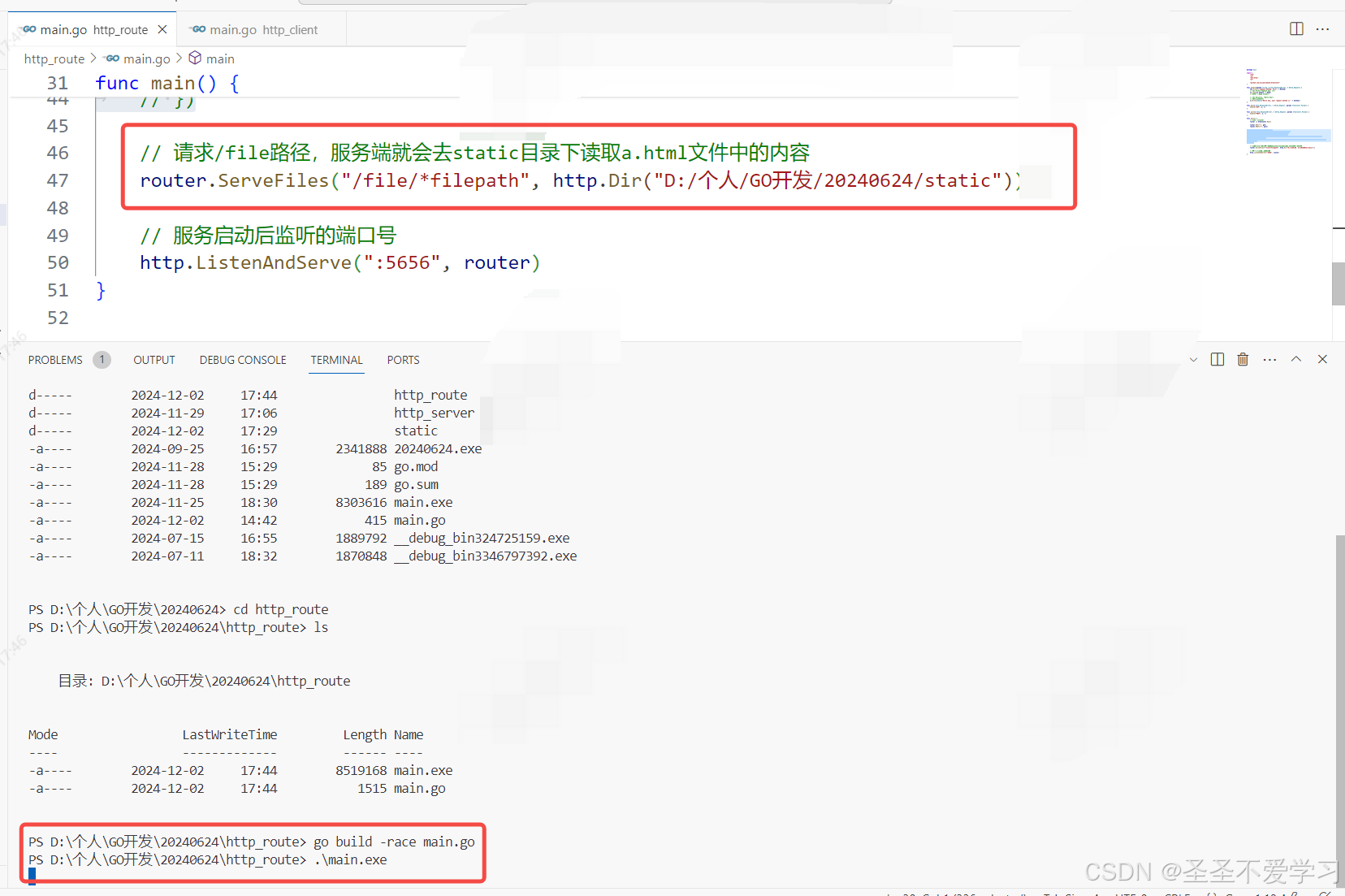
3.5.3 请求测试
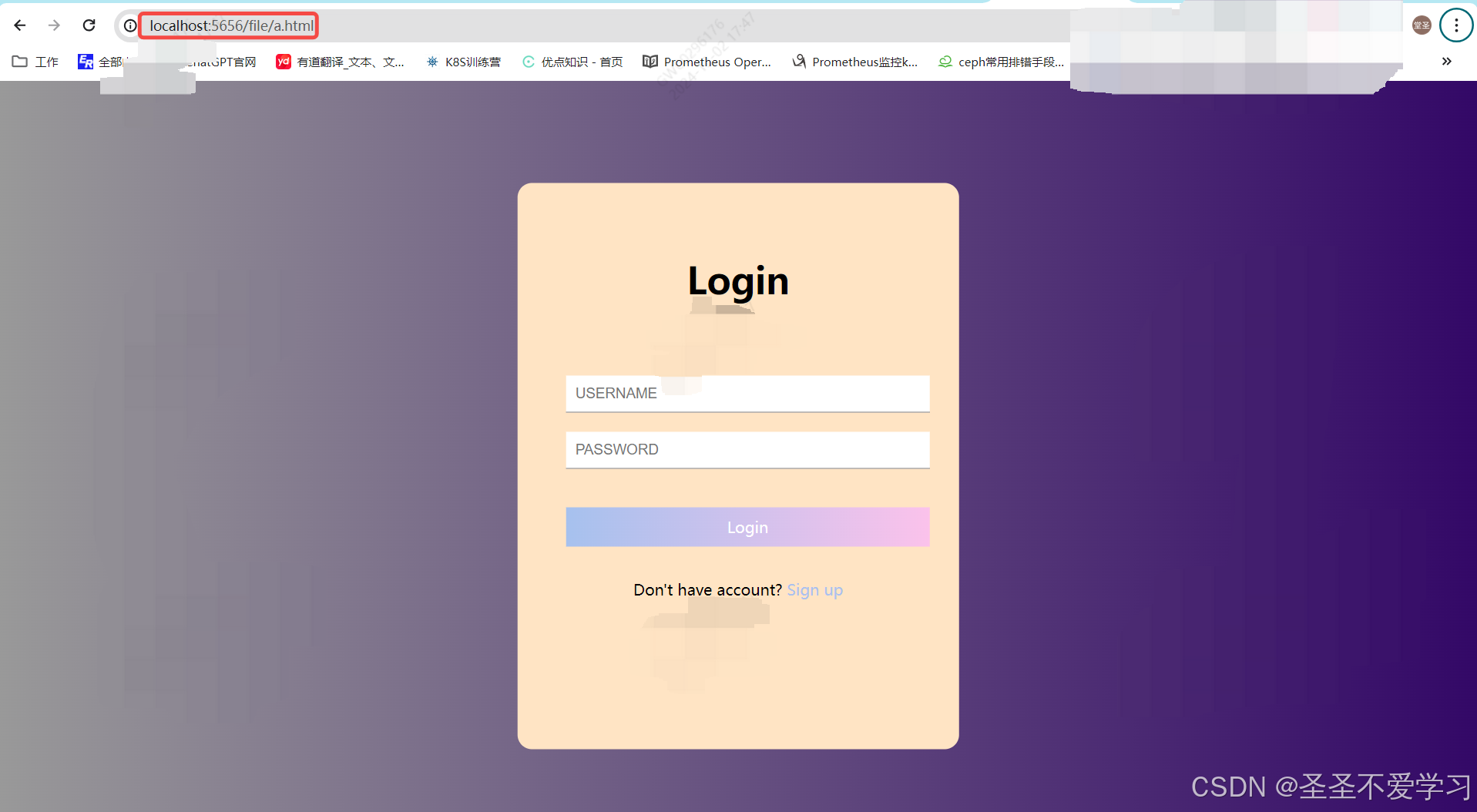
3.6 错误处理
在实际工作中,可能会有很多路由规则,在任何一处都有可能会报错,所以需要定义一个统一的告警处理。
3.6.1 服务端代码
Go
package main
import (
"fmt"
"io"
"net/http"
"os"
"github.com/julienschmidt/httprouter"
)
func handle(method string, w http.ResponseWriter, r *http.Request) {
fmt.Printf("request method: %v\n", r.Method)
// fmt.Print("request boy: \n")
io.Copy(os.Stdout, r.Body)
// defer r.Body.Close()
// fmt.Fprint(w, "Hello boy")
// 或者(上下等价)
w.Write([]byte("Hello boy, your request method is " + method))
}
func get(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("GET", w, r)
// 手动定义一个数组超界的异常,触发panic
var arr []int
_ = arr[1]
}
func post(w http.ResponseWriter, r *http.Request, params httprouter.Params) {
handle("POST", w, r)
}
func main() {
// 定义一个router
router := httprouter.New()
// 错误处理
router.PanicHandler = func(w http.ResponseWriter, r *http.Request, i interface{}) {
fmt.Fprintf(w, "server panic %v", i)
}
router.GET("/", get)
router.POST("/", post)
// 服务启动后监听的端口号
http.ListenAndServe(":5656", router)
}
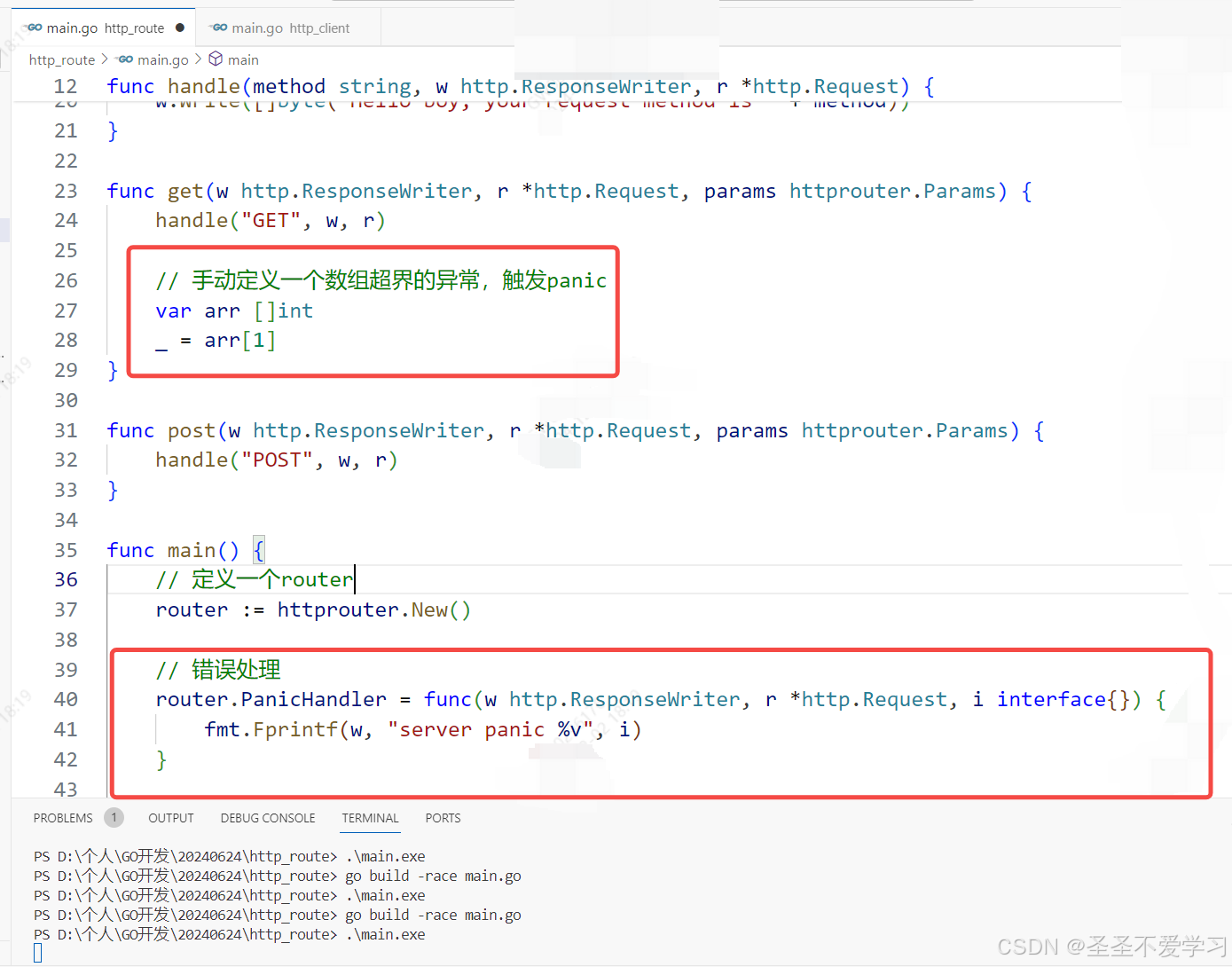
3.6.2 请求测试
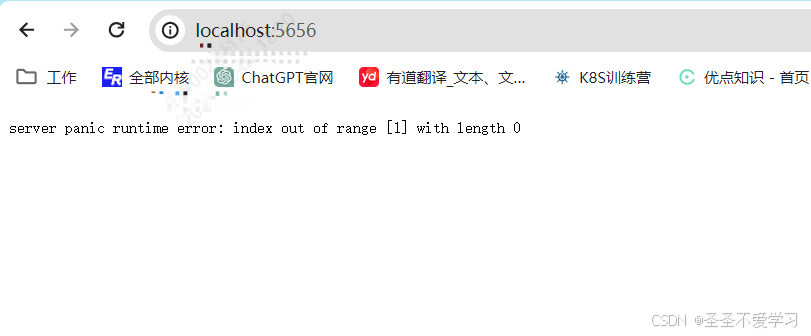