代码仓库:https://gitee.com/linguanzhong/share_harmonyos 鸿蒙api:12
引用的harmony-utils地址:OpenHarmony三方库中心仓
1.拷贝文件到缓存目录
import { FileUtil, ObjectUtil } from '@pura/harmony-utils'
import { common } from '@kit.AbilityKit'
import { BusinessError } from '@kit.BasicServicesKit'
import { BaseDialog } from './dialog/BaseDialog'
import fs from '@ohos.file.fs'
//拷贝文件到缓存目录
static copyFileToCache(mkdir: string, originPath: string, success: (cachePath: string, originalPath?: string) => void,
fail: (err: BusinessError) => void,
isShowLoading: boolean = true) {
if (isShowLoading) {
BaseDialog.showLoading()
}
fs.open(originPath, fs.OpenMode.READ_ONLY).then((file: fs.File) => {
let path = FileUtil.getCacheDirPath(mkdir) + '/' + file.name
let aaa = fs.accessSync(path)
if (aaa) {
if (isShowLoading) {
BaseDialog.hintLoading()
}
success(path, originPath)
fs.closeSync(file);
} else {
fs.copyFile(file.fd, path).then(() => {
if (isShowLoading) {
BaseDialog.hintLoading()
}
success(path, originPath)
fs.closeSync(file);
}).catch((err: BusinessError) => {
if (isShowLoading) {
BaseDialog.hintLoading()
}
fail(err)
})
}
}).catch((err: BusinessError) => {
if (isShowLoading) {
BaseDialog.hintLoading()
}
fail(err)
})
}
文件选择
//文件选择
static setFilePicker(context: Context,
documentSelectOptions: picker.DocumentSelectOptions,
successCallBack: (uris: Array<string>) => void,
errorCallBack: (err: BusinessError) => void) {
const documentViewPicker = new picker.DocumentViewPicker(context);
documentViewPicker.select(documentSelectOptions).then((documentSelectResult: Array<string>) => {
successCallBack(documentSelectResult)
}).catch((err: BusinessError) => {
errorCallBack(err)
})
}
使用:
BaseText({
text: "文件选择",
fontColor: $r('app.color.color_DD2942')
}).margin({
top: 10
}).onClick(() => {
let options = new picker.DocumentSelectOptions()
options.maxSelectNumber = 1
options.authMode = true
BaseUtil.setFilePicker(getContext(), options, (uris) => {
BaseLog.i("uris:" + uris.toString())
if (uris.length > 0) {
uris.forEach(item => {
BaseUtil.copyFileToCache("file", item, (path) => {
BaseLog.i("path:" + path) // /data/storage/el2/base/haps/entry/cache/file/欢迎使用华为文件管理.pdf
}, (error) => {
BaseLog.i("error11:" + JSON.stringify(error))
})
})
}
}, (error) => {
BaseDialog.showToast("调用文件选择失败:" + error.message)
})
})
图片或视频选择
申请权限:
"requestPermissions": [
{
"name": "ohos.permission.READ_IMAGEVIDEO",
"reason": "$string:permission_READ_IMAGEVIDEO",
"usedScene": {
"abilities": [
]
}
},
{
"name": "ohos.permission.WRITE_IMAGEVIDEO",
"reason": "$string:permission_READ_IMAGEVIDEO",
"usedScene": {
"abilities": [
]
}
}
]
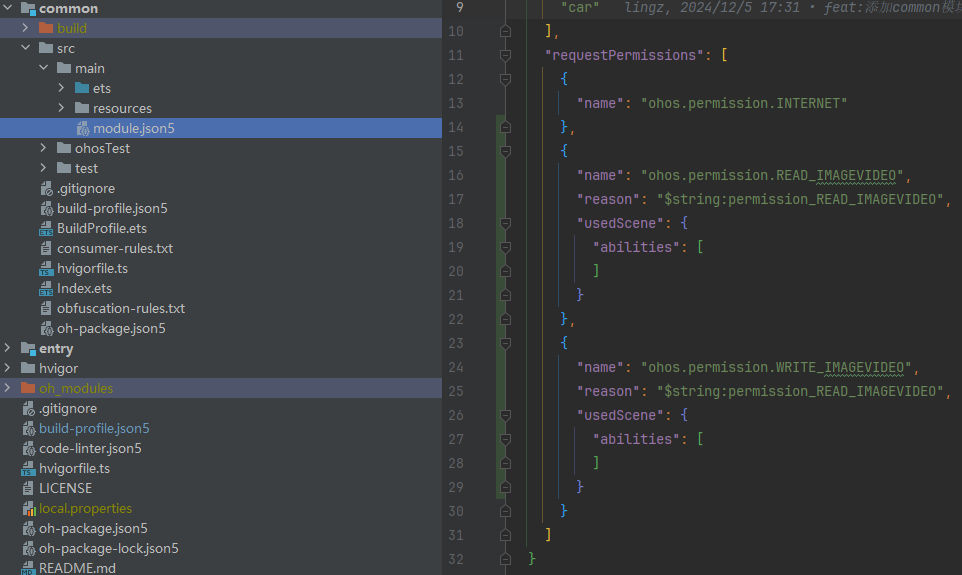
申请权限之后登录签名
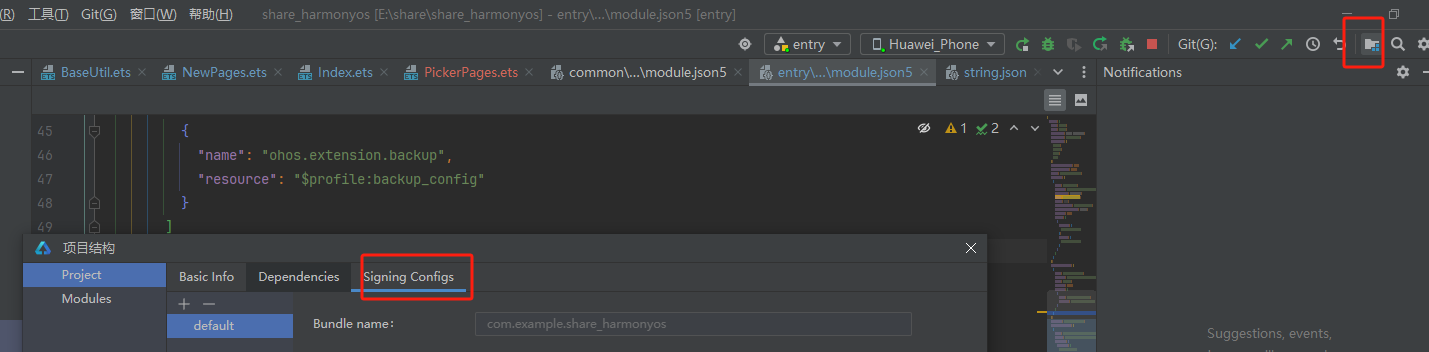
//type:0图片 1:视频
getPermission(type: number) {
let ps: Permissions[] = ['ohos.permission.READ_IMAGEVIDEO'];
PermissionUtil.requestPermissions(ps).then((result) => {
if (result) {
this.photoSelect(type)
} else {
BaseDialog.showToast("权限申请失败")
}
})
}
//type:0图片 1:视频
photoSelect(type: number) {
let options: photoAccessHelper.PhotoSelectOptions = {
isEditSupported: true,
isOriginalSupported: true,
maxSelectNumber: type == 0 ? 1 : 1,
isSearchSupported: true,
MIMEType: type == 0 ? photoAccessHelper.PhotoViewMIMETypes.IMAGE_TYPE : photoAccessHelper.PhotoViewMIMETypes.VIDEO_TYPE
}
PhotoHelper.select(options).then((uris: string[]) => {
if (uris.length > 0) {
uris.forEach(item => {
BaseUtil.copyFileToCache("image", item, (path) => {
if (type == 0) {
if (FileUtil.lstatSync(path).size >= 5 * 1024 * 1024) {
BaseDialog.showToast("只能上传大小为5M以下的图片~")
} else {
}
} else {
if (FileUtil.lstatSync(path).size >= 20 * 1024 * 1024) {
BaseDialog.showToast("只能上传大小为20M以下的视频~")
} else {
}
}
}, (error) => {
})
})
}
}).catch((err: BusinessError) => {
BaseDialog.showToast("调用相册失败:" + err.message)
})
}
使用:
BaseText({
text: "视频选择",
fontColor: $r('app.color.color_DD2942')
}).margin({
top: 10
}).onClick(() => {
this.getPermission(0)
})
保存到相册
//保存到相册
static async save(filePath: string, fileName: string, success: (path: string) => void, fail: (err: BusinessError) => void) {
BaseDialog.showLoading()
let photoHelper = photoAccessHelper.getPhotoAccessHelper(getContext());
let uri = fileUri.getUriFromPath(filePath)
let assetChangeRequest: photoAccessHelper.MediaAssetChangeRequest =
photoAccessHelper.MediaAssetChangeRequest.createImageAssetRequest(getContext(), uri);
photoHelper.applyChanges(assetChangeRequest).then((s) => {
BaseDialog.hintLoading()
success(filePath)
BaseDialog.hintLoading()
}).catch((err: BusinessError) => {
fail(err)
BaseDialog.hintLoading()
})
}
//拷贝到图库
static saveToAlbum(downLoadUrl: string, success: (path: string) => void, fail: (err: BusinessError) => void) {
let e: BusinessError = {
code: 0,
name: '',
message: ''
}
PermissionUtil.requestPermissions('ohos.permission.WRITE_IMAGEVIDEO').then((result) => {
if (result) {
let filePath = ""
let fileName = ""
let aa = downLoadUrl.split("/")
if (aa.length != 0) {
fileName = aa[aa.length-1]
//有些图片链接不是.jpg结尾
if (fileName.indexOf(".") == -1) {
fileName += ".jpg"
}
BaseLog.i("fileName:" + fileName)
filePath = BaseUtil.getImagePathByFileName(fileName)
}
BaseLog.i("filePath:" + filePath)
if (BaseUtil.isEmpty(filePath)) {
filePath = BaseUtil.getImagePath() + '/' + fileName
let baseRequestSet = new BaseRequestSet()
baseRequestSet.url = downLoadUrl
baseRequestSet.filePath = filePath
baseRequestSet.isLoading = true
new BaseHttp().downloadFile(baseRequestSet,
(msg) => {
BaseUtil.save(msg ?? "", fileName, success, fail)
},
(error) => {
e.message = error.message
e.code = Number(error.code)
fail(e)
},
() => {
},
(progress) => {
BaseLog.i("progress:" + progress.progress)
}
)
} else {
BaseUtil.save(filePath, fileName, success, fail)
}
} else {
e.message = "权限申请失败"
fail(e)
}
})
}
使用:
BaseText({
text: "下载保存到图库",
fontColor: $r('app.color.color_DD2942')
}).margin({
top: 10
}).onClick(() => {
BaseUtil.saveToAlbum("https://pics4.baidu.com/feed/314e251f95cad1c8dd6c162f5b8c5b07c83d5127.jpeg", (success) => {
BaseDialog.showToast("保存成功:" + success)
}, (error) => {
BaseDialog.showToast(error.message)
})
})