目录
Vue代码编写风格
- 选项式API --> vue2
javascript
<script>
export default {
data() {
return {
count: 0
}
},
methods: {
increment() {
this.count++
}
},
mounted() {
console.log(`The initial count is ${this.count}.`)
}
}
</script>
<template>
<button @click="increment">Count is: {{ count }}</button>
</template>
- 组合式API --> vue3
javascript
<script setup>
import { ref, onMounted} from 'vue'
const count = ref(0)
function increment() {
count.value++
}
onMounted(() => {
console.log(`The initial count is ${count.value}.`)
})
</script>
<template>
<button @click="increment">Count is: {{ count }}</button>
</template>
前提条件
- 安装15.0或更高版本的Node.js
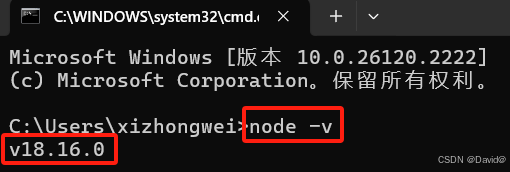
创建Vue项目
- cd进入你的项目所在文件夹
bash
npm init vue@latest
- 这个指令将会安装并执行
create-vue
,它是Vue官方的项目脚手架工具。你将会看到一些诸如TypeScript和测试支持之类的可选功能提示。
bash
√ project name: ... <your-project-name>
√ Add TypeScript? ... No / Yes
√ Add JSX Support? ... No / Yes
√ Add Vue Router for single Page Application development? ... No / Yes
√ Add Pinia for state management? ... No / Yes
√ Add Vitest for Unit testing? ... No / Yes
√ Add Cypress for both Unit and End-to-End testing? ... No / Yes
√ Add ESLint for code quality? ... No / Yes
√ Add Prettier for code formatting? ... No / Yes
scaffolding project in ./<your-project-name>...
Done.
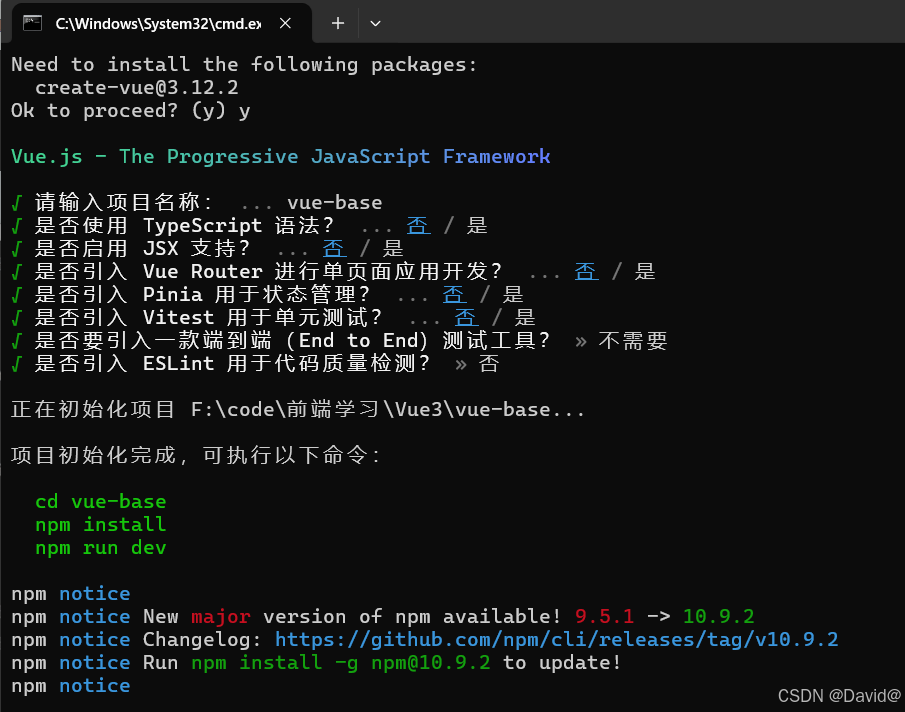
- 根据提示,继续运行,值得注意的是,npm可用cnpm,因为cnpm是npm的国内镜像,能加速下载
[cnpm 的安装与使用] https://developer.aliyun.com/article/1599824
bash
cd vue-base
npm install
npm run dev
- 浏览器打开这个网址,说明创建成功
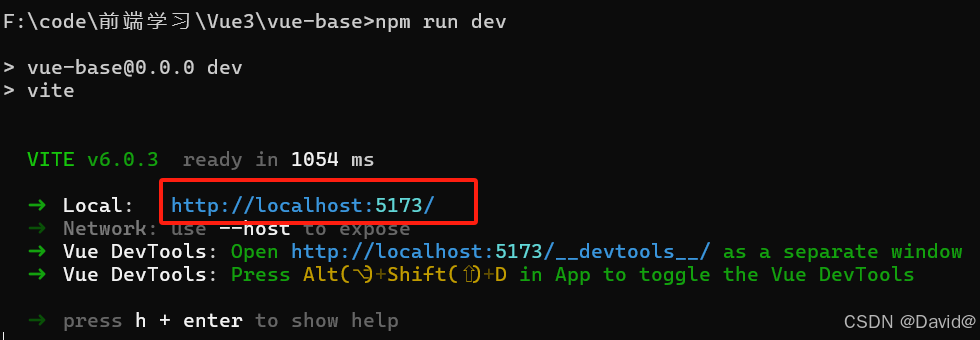
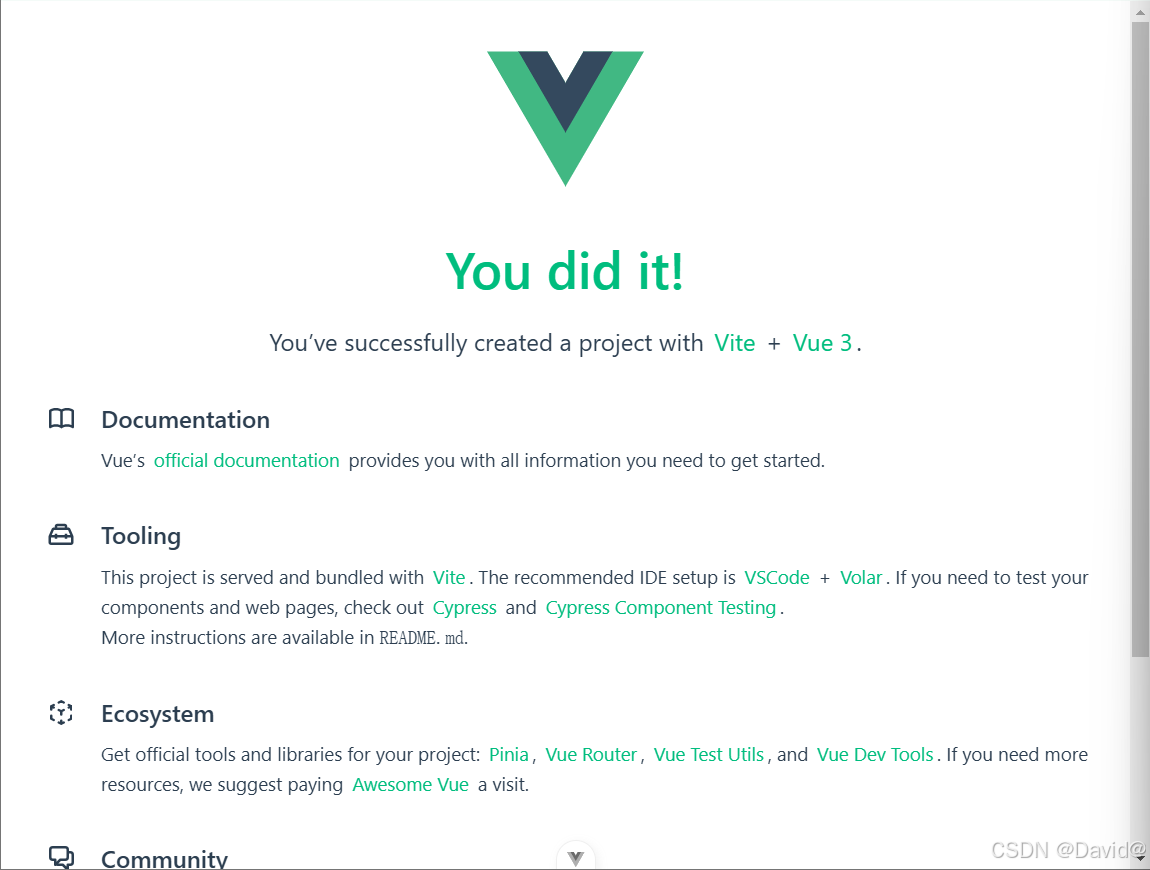
开发环境
推荐的IDE配置是Visual Studio Code
+ Volar
拓展
项目文件说明
bash
.vscode --- VSCode工具的配置文件夹
node-modules --- Vue项目的运行依赖文件夹
public --- 资源文件夹(浏览器图标)
src --- 源码文件夹
.gitignore --- git忽略文件
index.html --- 入口HTML文件
package.json --- 信息描述文件
README.md --- 注释文件
vite.config.js --- Vue配置文件