.NET 9已经发布有一段时间了,近期整理一下.NET 9的新特性,今天重点分享.NET 9 JSON序列化方面的改进。
先引用官方的说明:
在 System.Text.Json 中,.NET 9 提供了用于序列化 JSON 的新选项和新的单一实例,可以更轻松地使用 Web 默认值进行序列化。
举个实际的例子,
缩进选项
JsonSerializerOptions 包括新的属性,可支持自定义写入 JSON 的缩进字符和缩进大小。
var options = new JsonSerializerOptions
{
WriteIndented = true,
IndentCharacter = '\t',
IndentSize = 2,
};
string json = JsonSerializer.Serialize(
new { Value = 1 },
options
);
Console.WriteLine(json);
实际的效果
//{
// "Value": 1
//}
大家不禁会问,这有什么用?
JsonSerializerOptions
新增了对自定义 JSON 缩进字符和缩进大小的支持。这项特性主要通过两个新属性实现:
IndentChars
: 指定用于缩进的字符(默认为空格" "
)。IndentSize
: 指定缩进的大小(默认为 2)。
这些属性使开发者能够更灵活地控制生成的 JSON 格式,适应不同的需求,比如:
-
日志格式优化 : 某些系统对日志的可读性有特定要求,需要用不同的缩进字符(例如制表符
\t
)或更大的缩进层次,以更容易定位层次结构。 -
前端需求匹配: 一些前端框架可能要求特定的 JSON 格式(例如 4 个空格的缩进),以便与前端代码风格保持一致。
-
节省空间: 在生成用于传输或存储的美化 JSON 时,调整缩进字符或大小可以帮助减少文件体积。
-
与外部工具集成: 某些外部工具或系统对 JSON 的缩进风格有特定要求,自定义这两项属性可以轻松实现兼容。
继续给一个示例代码
using System;
using System.Text.Json;
public class Program
{
public static void Main()
{
var data = new
{
Name = "Alice",
Age = 30,
Skills = new[] { "C#", "ASP.NET Core", "Blazor" }
};
// 默认缩进格式
var defaultOptions = new JsonSerializerOptions
{
WriteIndented = true
};
Console.WriteLine("默认格式:");
Console.WriteLine(JsonSerializer.Serialize(data, defaultOptions));
// 使用自定义缩进字符(制表符)和大小
var customOptions = new JsonSerializerOptions
{
WriteIndented = true,
IndentChars = "\t", // 使用制表符
IndentSize = 1 // 每层缩进 1 个制表符
};
Console.WriteLine("\n自定义制表符缩进:");
Console.WriteLine(JsonSerializer.Serialize(data, customOptions));
// 使用自定义空格缩进
var customSpaceOptions = new JsonSerializerOptions
{
WriteIndented = true,
IndentChars = " ", // 使用空格
IndentSize = 4 // 每层缩进 4 个空格
};
Console.WriteLine("\n自定义空格缩进:");
Console.WriteLine(JsonSerializer.Serialize(data, customSpaceOptions));
}
}
输出数据示例
- 默认格式
{
"Name": "Alice",
"Age": 30,
"Skills": [
"C#",
"ASP.NET Core",
"Blazor"
]
}
2.自定义制表符缩进
{
"Name": "Alice",
"Age": 30,
"Skills": [
"C#",
"ASP.NET Core",
"Blazor"
]
}
- 自定义空格缩进
{
"Name": "Alice",
"Age": 30,
"Skills": [
"C#",
"ASP.NET Core",
"Blazor"
]
}
这种灵活性让开发者能根据不同的上下文需求生成适合的 JSON 输出,既方便了可读性,也提升了兼容性。
然后,我们继续看新的单一实例
如果要使用 ASP.NET Core 用于 Web 应用的默认选项进行序列化,请使用新的 JsonSerializerOptions.Web 单一实例。
string webJson = JsonSerializer.Serialize(
new { SomeValue = 42 },
JsonSerializerOptions.Web // Defaults to camelCase naming policy.
);
Console.WriteLine(webJson);
// {"someValue":42}
其实就是给JsonSerializerOptions多了一个Web选项。官方的解释:
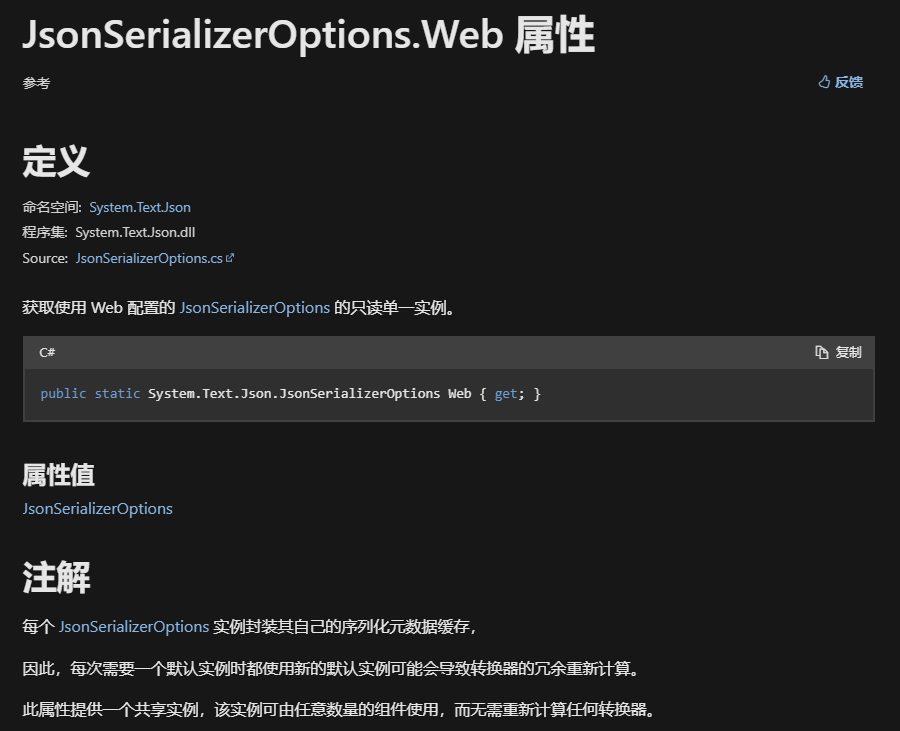
意会之后,整理了以下说明:
JsonSerializerOptions.Web
是一个新的单例实例,专为 Web 应用程序设计,提供了默认的 JSON 序列化选项。它的主要特点包括:
-
属性命名策略:将属性名称从 PascalCase 转换为 camelCase,以符合 JavaScript 的命名惯例。
-
忽略默认值 :在序列化过程中,忽略属性的默认值(如
null
、0
、false
),以减少传输的数据量。 -
数字处理:允许从字符串读取数字,以提高灵活性。
这些默认设置使得在 Web 环境中进行 JSON 序列化时,无需手动配置即可获得符合 Web 标准的输出。
实际应用场景:
-
Web API 开发 :在构建 RESTful API 时,使用
JsonSerializerOptions.Web
可以确保返回的 JSON 数据符合前端的预期格式,减少前后端数据格式不一致的问题。 -
前后端一致性:通过自动将属性名称转换为 camelCase,避免了前端在处理 JSON 数据时的额外转换工作,提高开发效率。
给个示例代码看看效果
using System.Text.Json;
public class Product
{
public int Id { get; set; }
public string Name { get; set; } = "Sample Product";
public decimal Price { get; set; } = 0.0m; // 默认值
public string? Description { get; set; } = null; // 可空类型
}
public class Program
{
public static void Main()
{
var product = new Product();
// 使用 JsonSerializerOptions.Web 进行序列化
string json = JsonSerializer.Serialize(product, JsonSerializerOptions.Web);
Console.WriteLine(json);
}
}
输出的JSON数据
{
"id": 0,
"name": "Sample Product"
}
-
属性命名策略 :
Id
被序列化为id
,Name
被序列化为name
,符合 camelCase 命名惯例。 -
忽略默认值 :
Price
属性的默认值为0.0
,Description
属性的默认值为null
,因此在序列化结果中被省略。
以上是对.NET 9中JSON序列化部分的研究、分享和介绍。
周国庆
2024/12/22