一、力扣
1、字符串相加
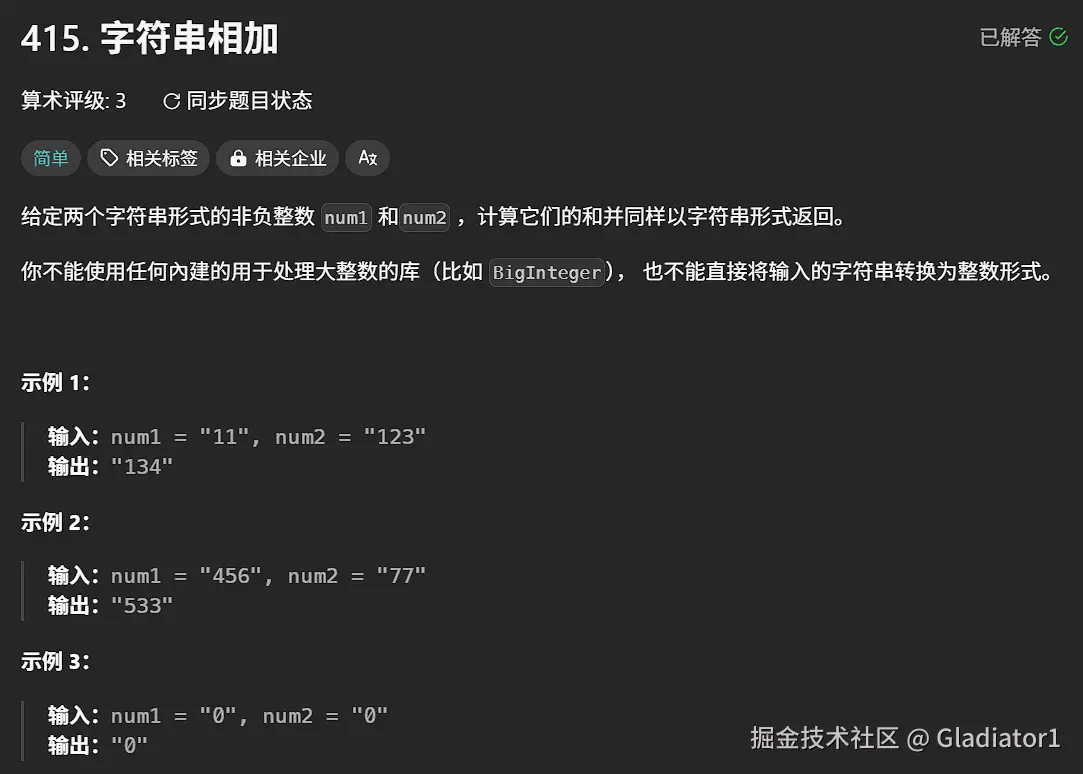
java
class Solution {
public String addStrings(String num1, String num2) {
StringBuilder res = new StringBuilder();
int i = num1.length() - 1, j = num2.length() - 1, carry = 0;
while(i >= 0 || j >= 0){
int n1 = i >= 0 ? num1.charAt(i) - '0' : 0;
int n2 = j >= 0 ? num2.charAt(j) - '0' : 0;
int tmp = n1 + n2 + carry;
carry = tmp / 10;
res.append(tmp % 10);
i--; j--;
}
if(carry == 1) res.append(1);
return res.reverse().toString();
}
}
2、重排链表
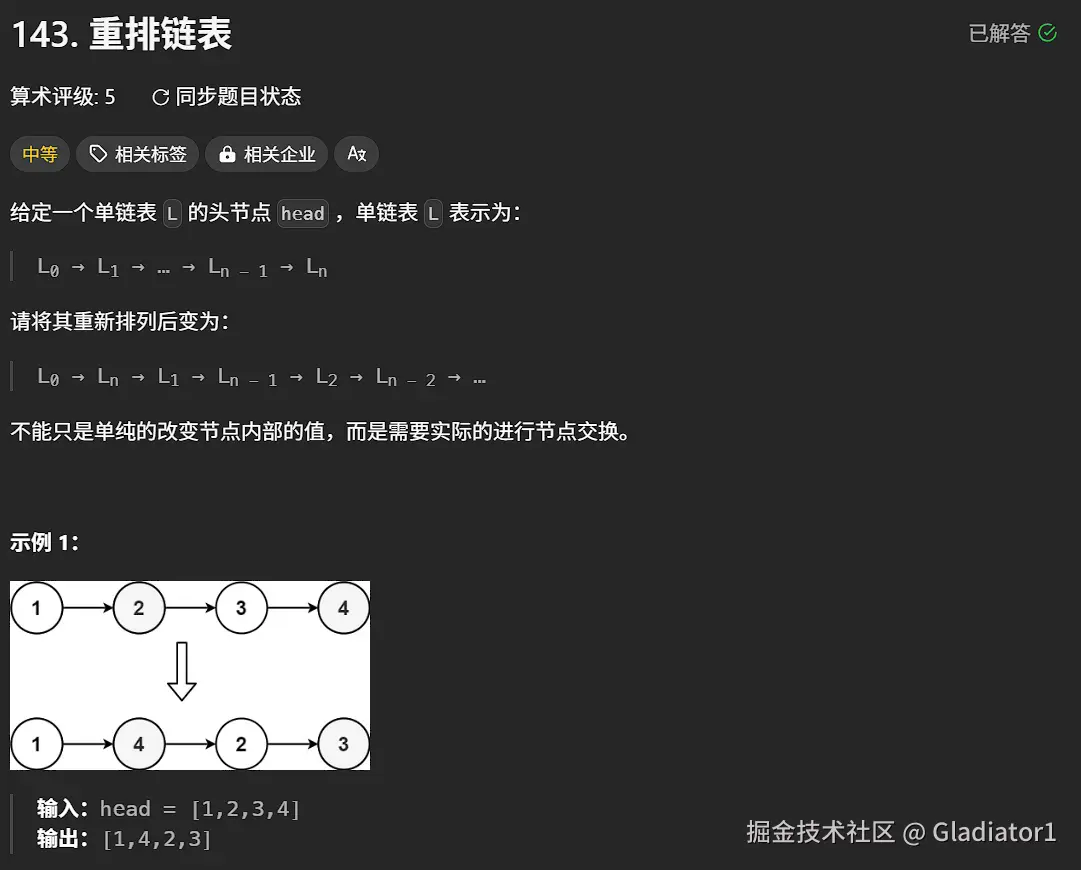
java
class Solution {
public void reorderList(ListNode head) {
ListNode sec=findmid(head);
ListNode head2=reverse(sec);
ListNode cur=head;
while(head2!=null){
ListNode head2next=head2.next;
head2.next=null;
ListNode cop=cur.next;
cur.next=head2;
head2.next=cop;
cur=cop;
head2=head2next;
}
}
public ListNode findmid(ListNode head){
ListNode fast=head;
ListNode slow=head;
while(fast!=null&&fast.next!=null){
fast=fast.next.next;
slow=slow.next;
}
ListNode res=slow.next;
slow.next=null;
return res;
}
public ListNode reverse(ListNode head){
ListNode pre=null;
while(head!=null){
ListNode nextHead=head.next;
head.next=pre;
pre=head;
head=nextHead;
}
return pre;
}
}
3、相交链表
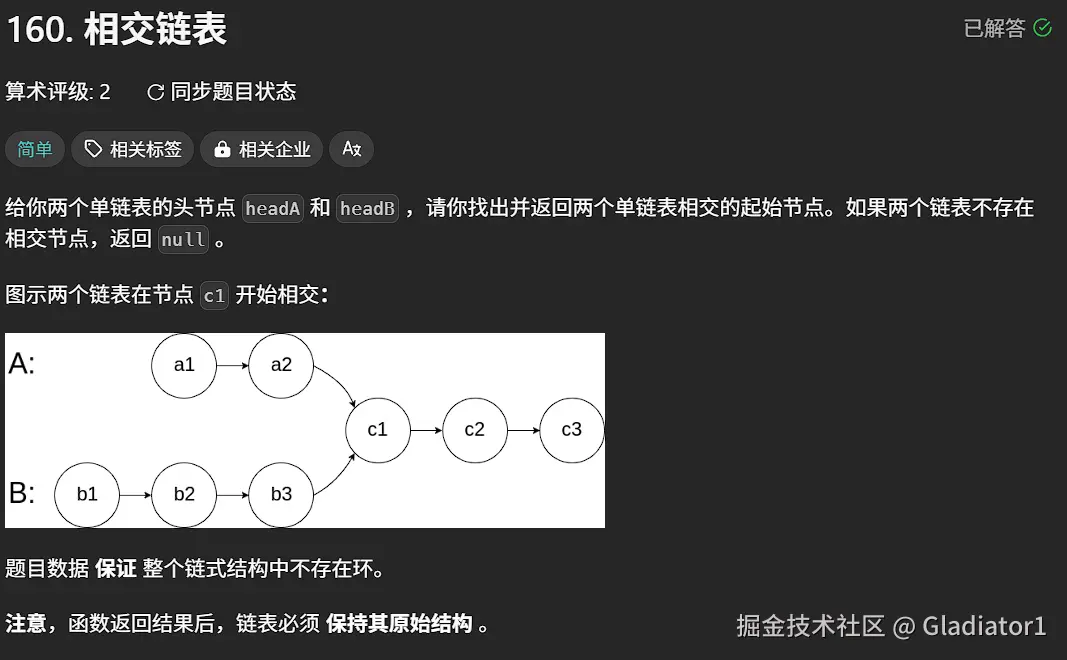
java
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
ListNode temp1=headA,temp2=headB;
while(temp1!=temp2){
temp1=(temp1==null?headB:temp1.next);
temp2=(temp2==null?headA:temp2.next);
}
return temp1;
}
}
二、语雀-场景题
1、如何实现敏感词过滤
1. 字符串匹配
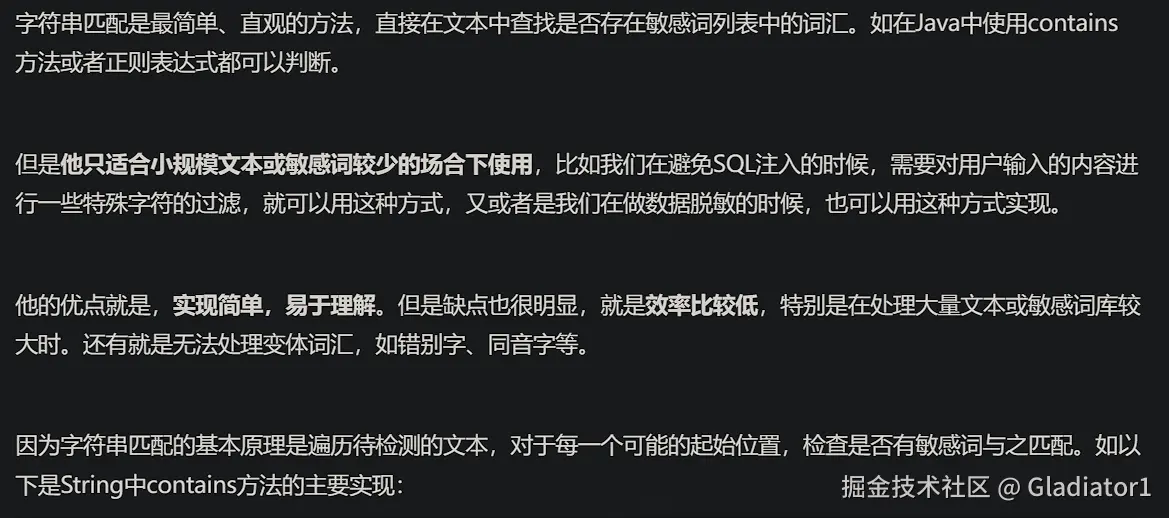
2. 前缀树
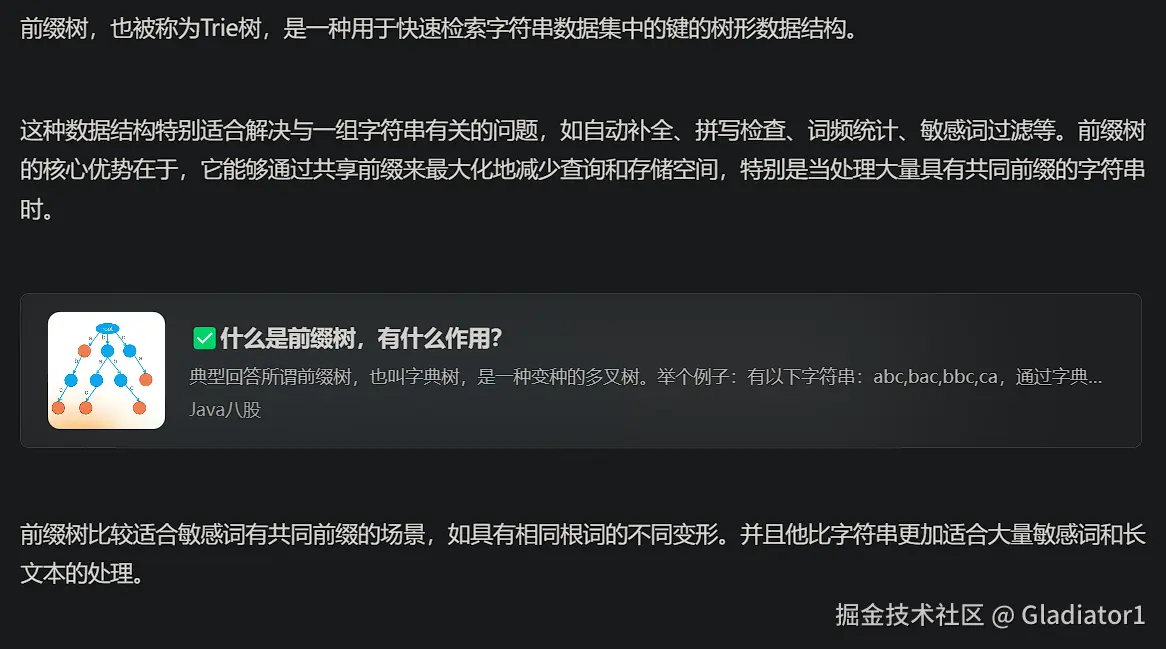
3. DFA算法
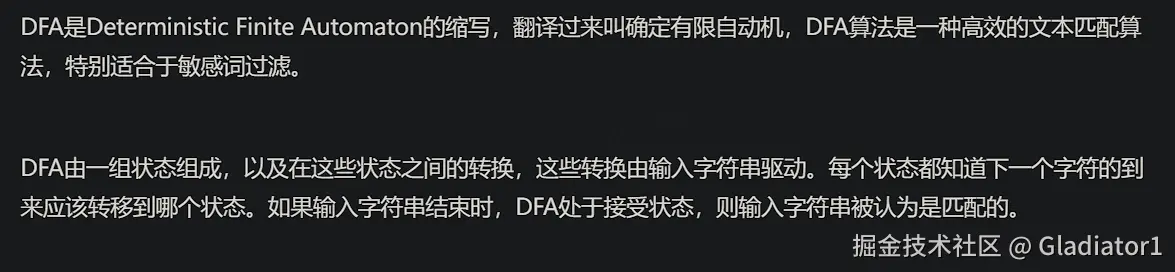
2、为啥不要在事务中做外部调用?
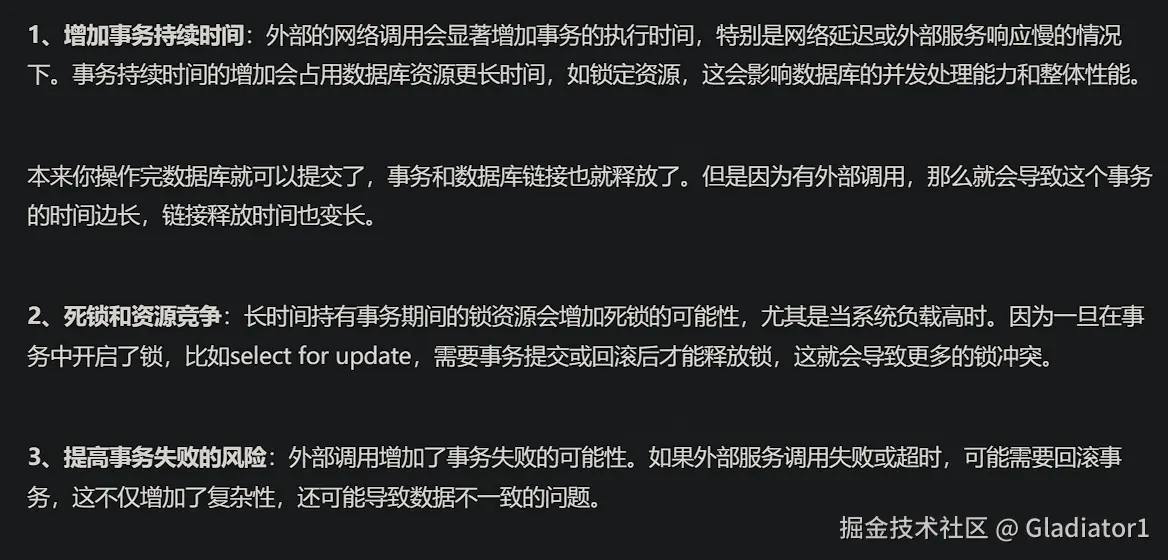
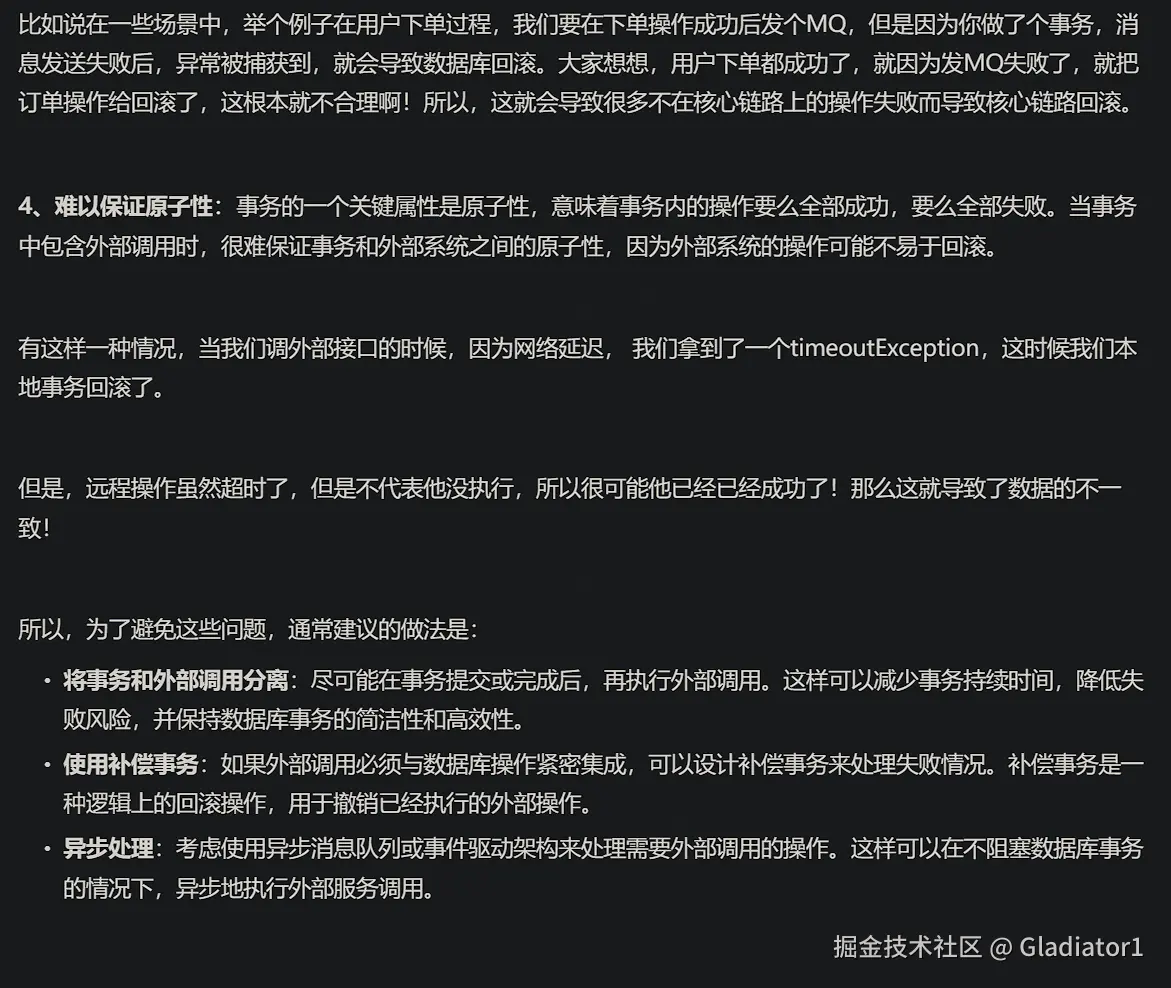
3、如何实现一个抢红包功能
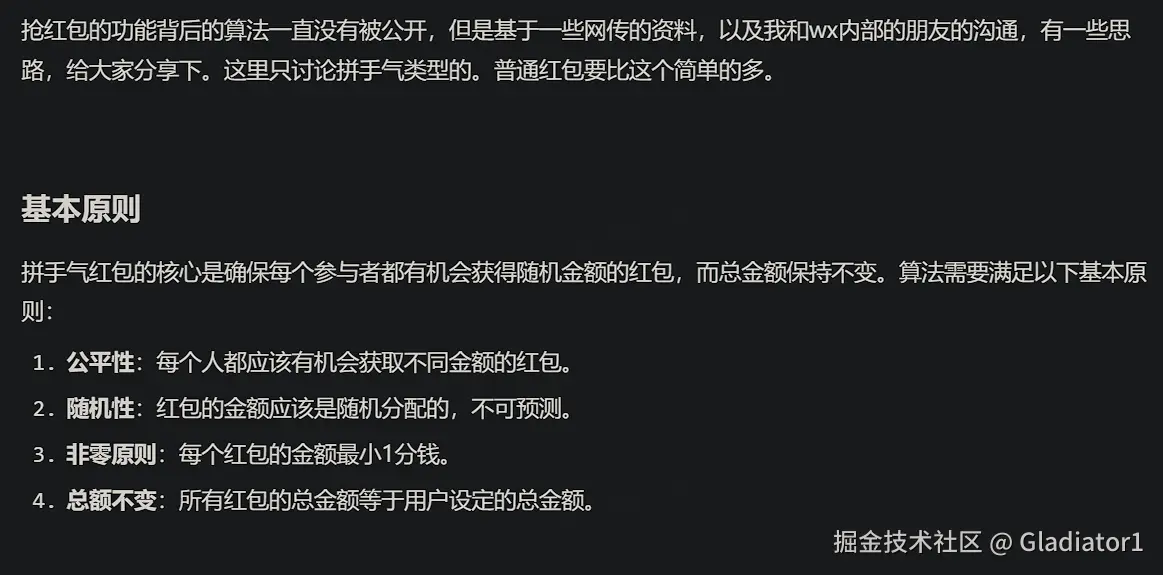
1. 二倍均值
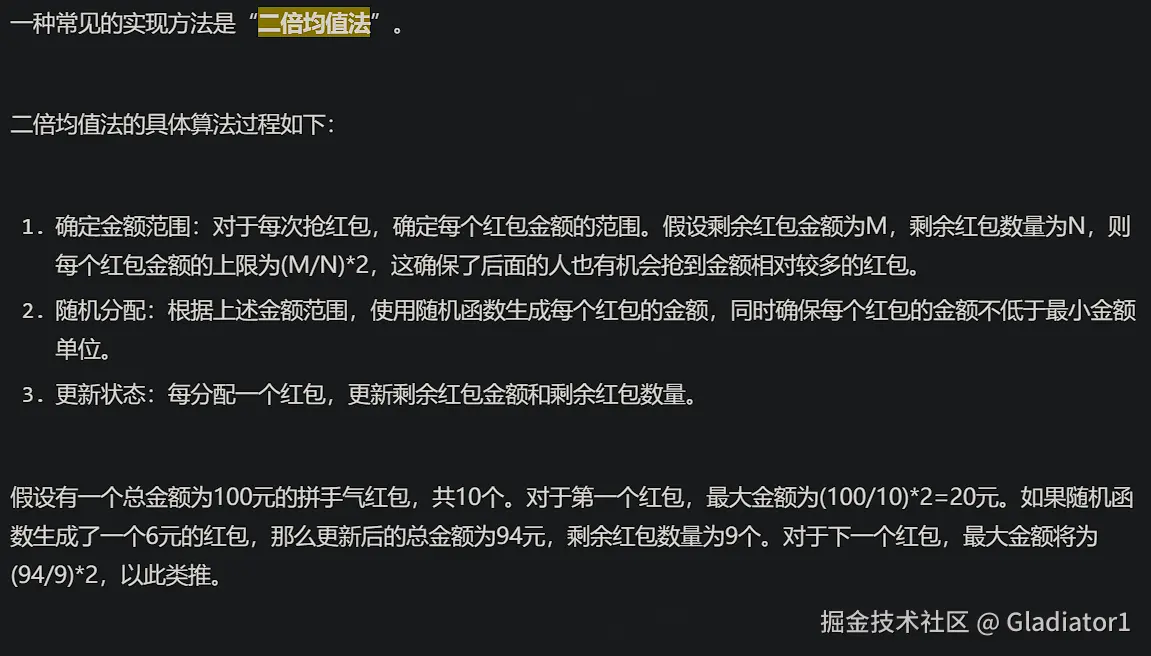
2. 高并发
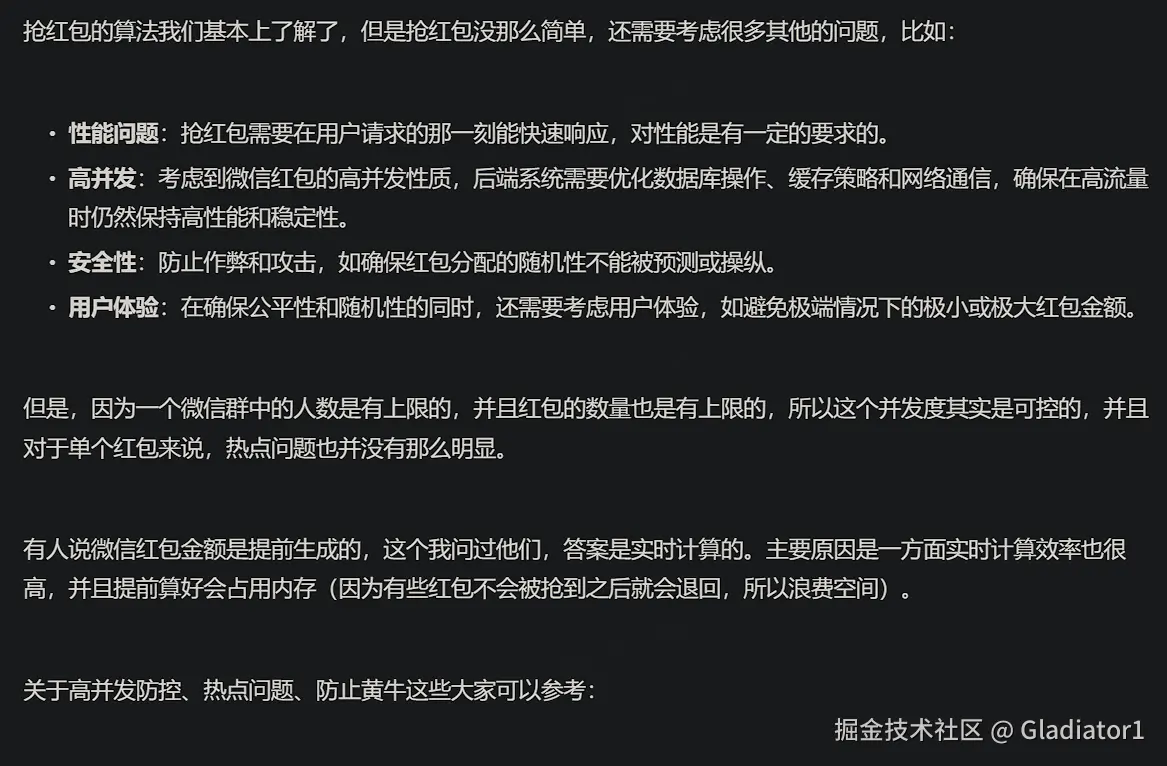
4、使用分布式锁时,分布式锁加在事务外面还是里面,有什么区别?
✅使用分布式锁时,分布式锁加在事务外面还是里面,有什么区别?
1. 锁的粒度大于事务粒度
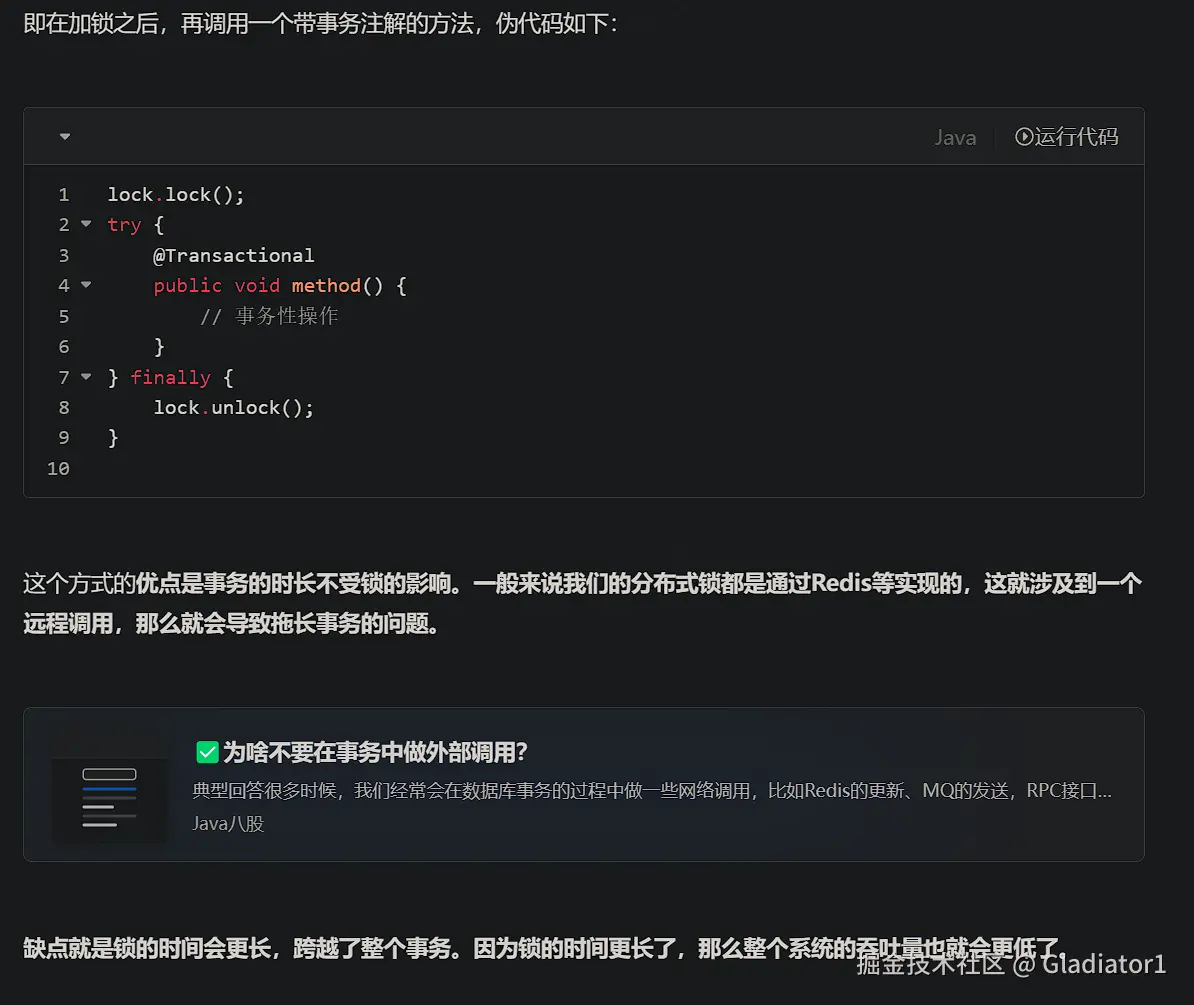
2. 事务的粒度大于锁粒度
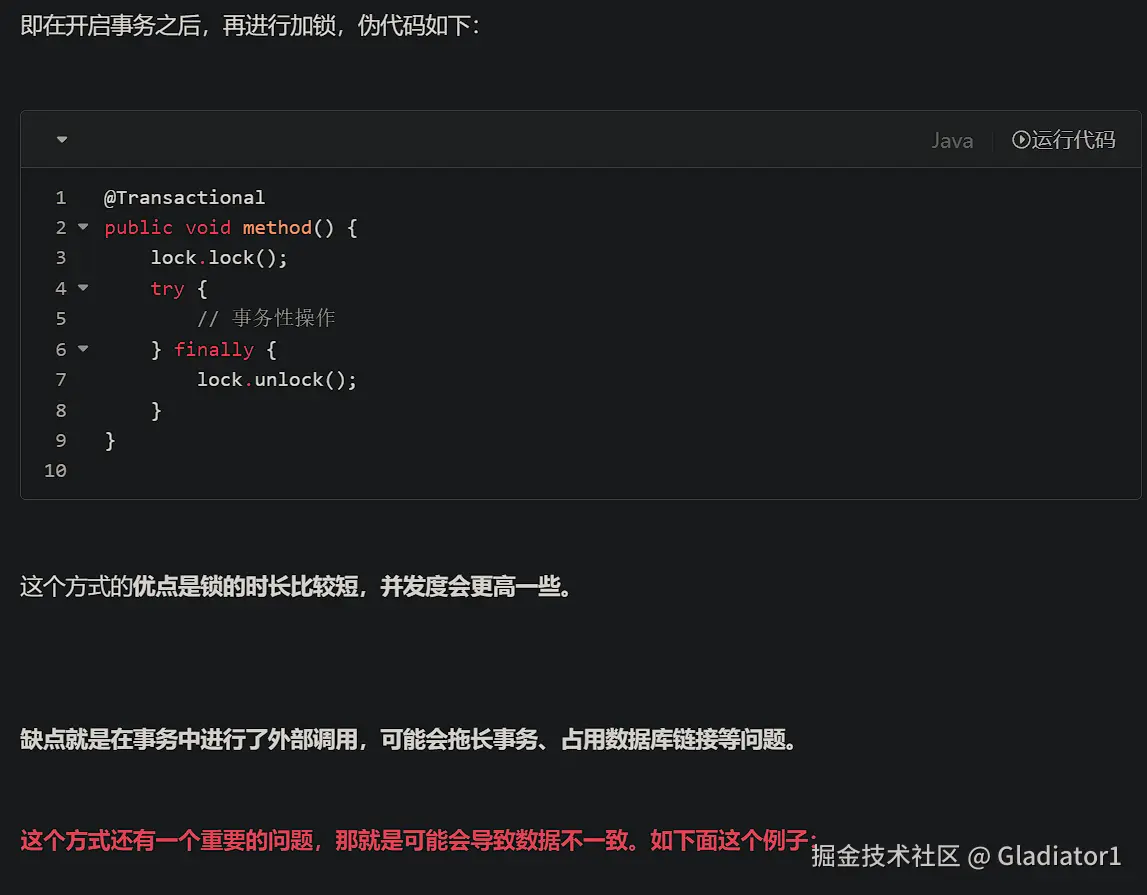
3. 建议选择第一个
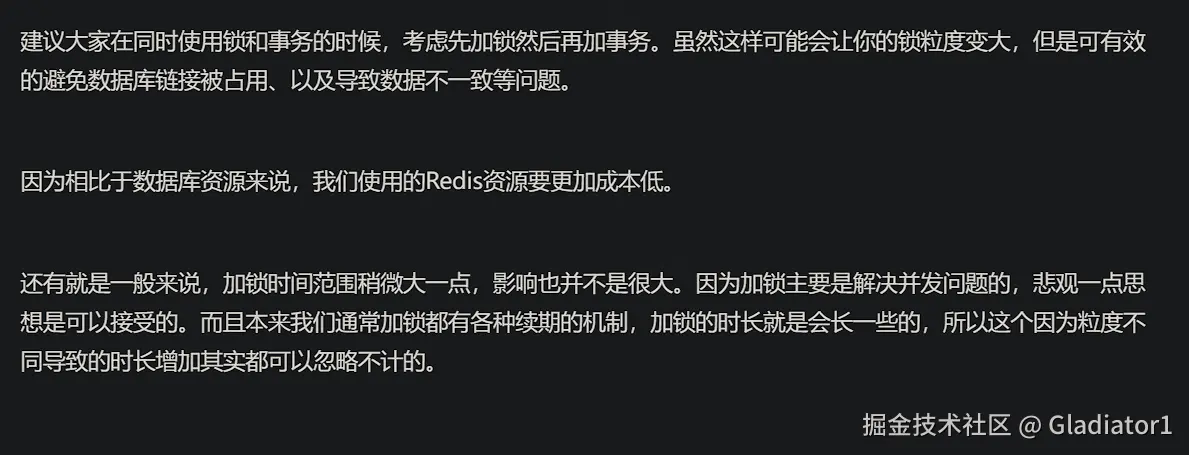
5、加分布式锁之后影响并发了怎么办?
1. 主要防止重复消费,消息重投
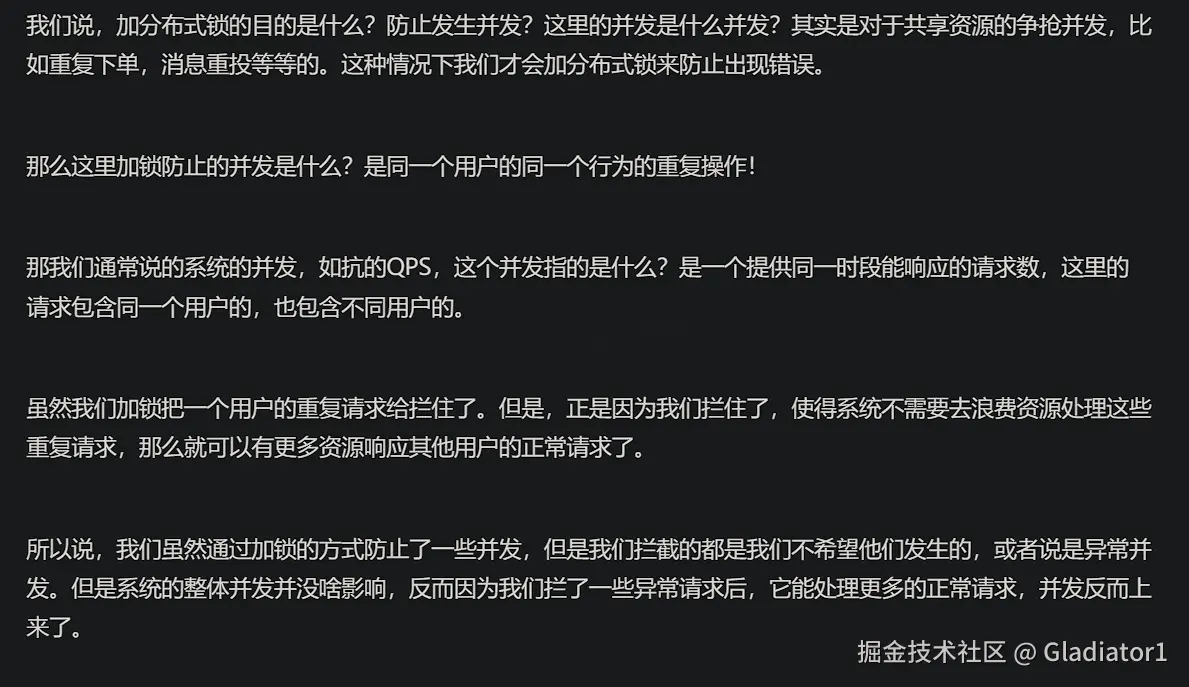
6、数据库乐观锁和悲观锁以及redis分布式锁的区别和使用场景?
✅数据库乐观锁和悲观锁以及redis分布式锁的区别和使用场景?
1. 悲观锁
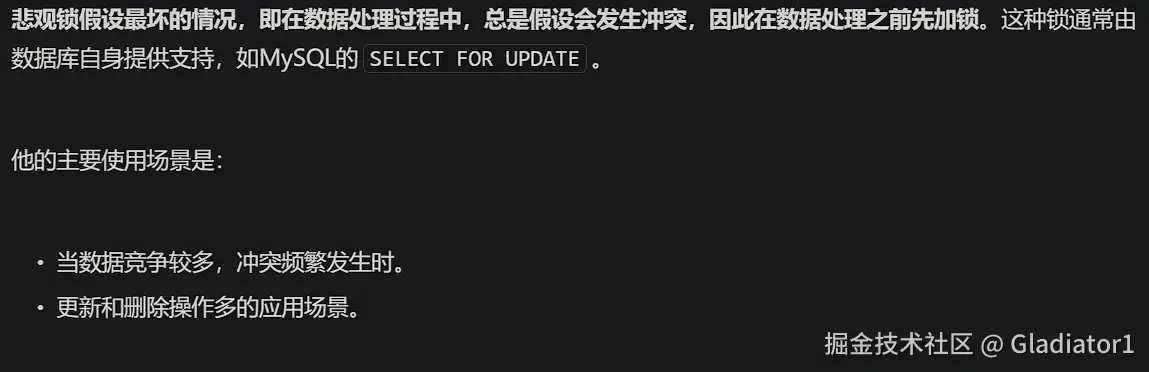
2. 乐观锁
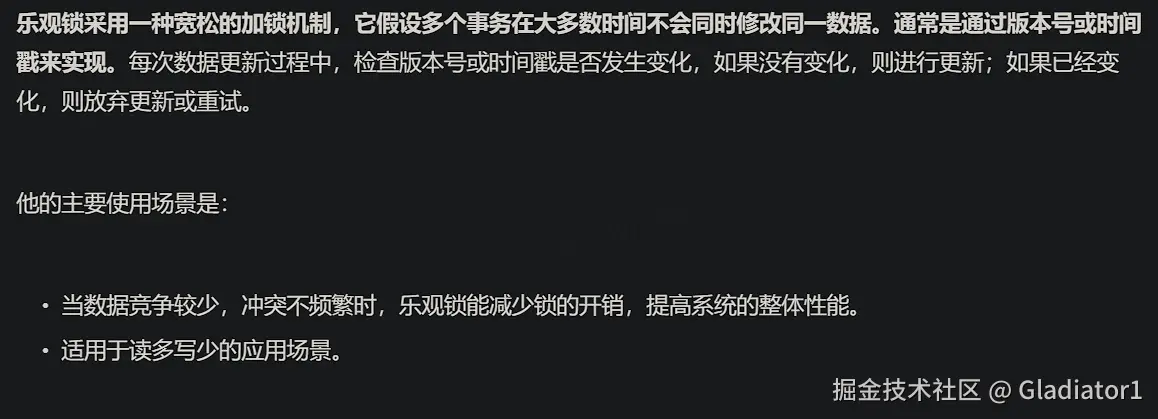
3. 乐观锁与悲观锁区别
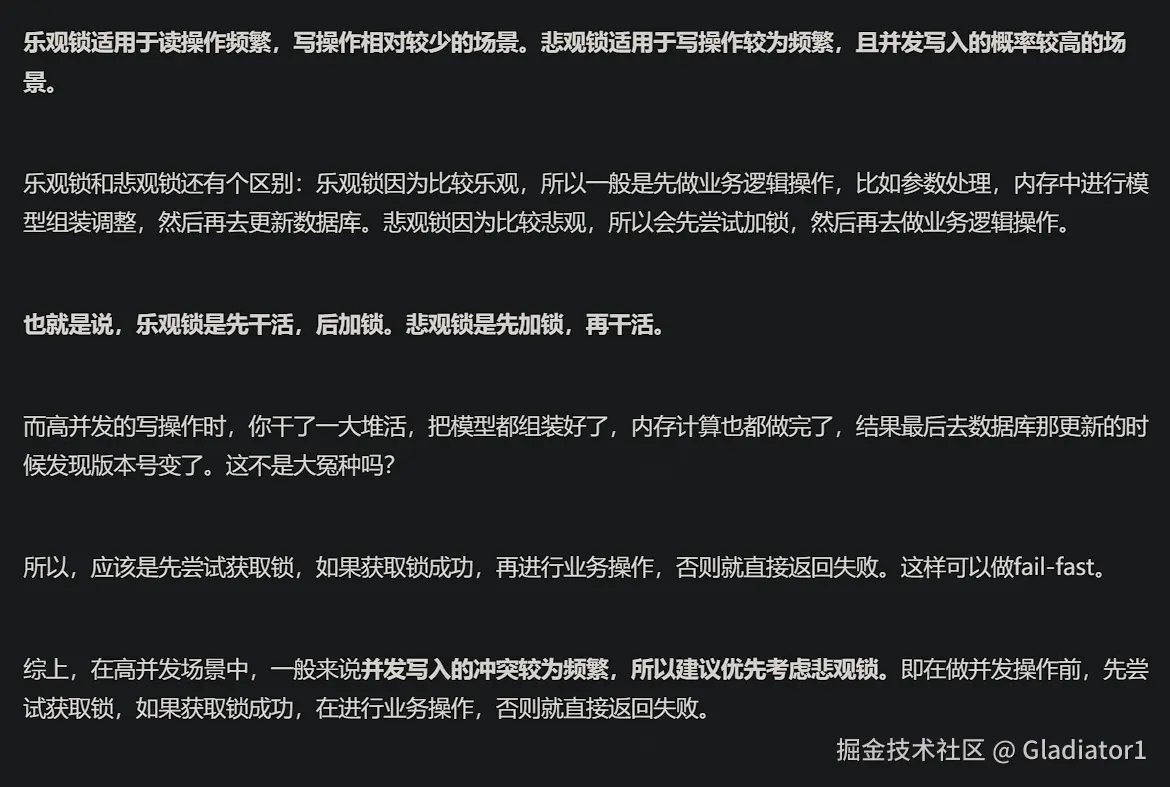
4. Redis分布式锁
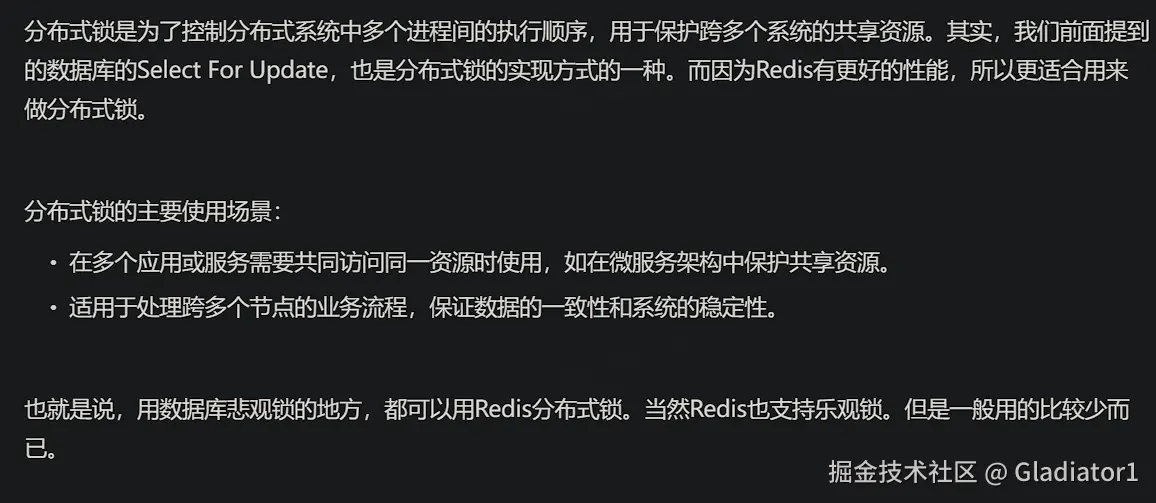
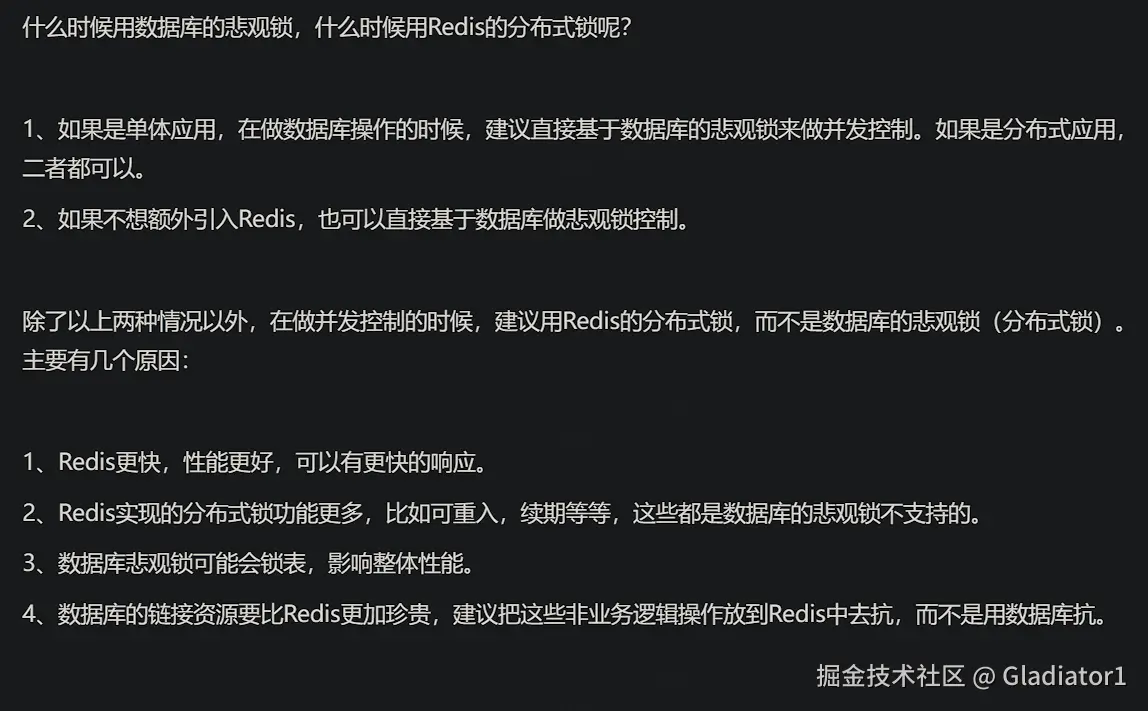
7、为什么不用分布式锁来实现秒杀?
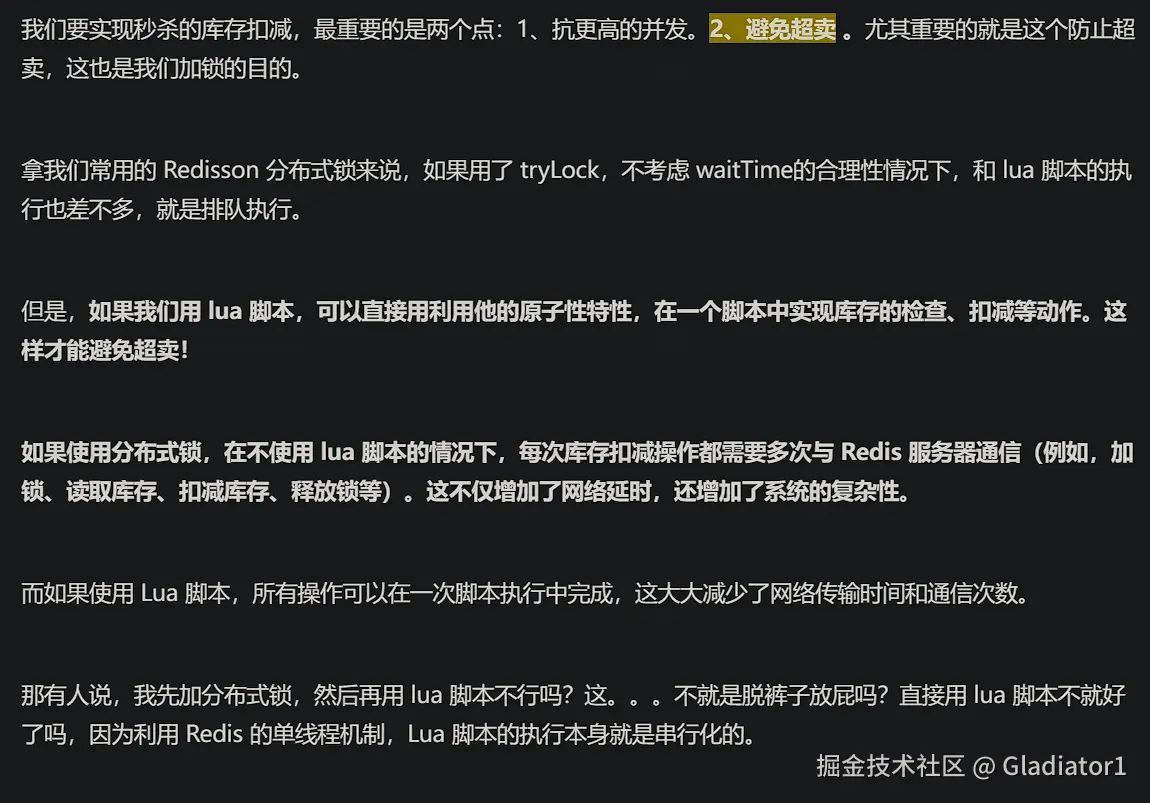
8、为什么不直接用原生的BlockinQueue做消息队列
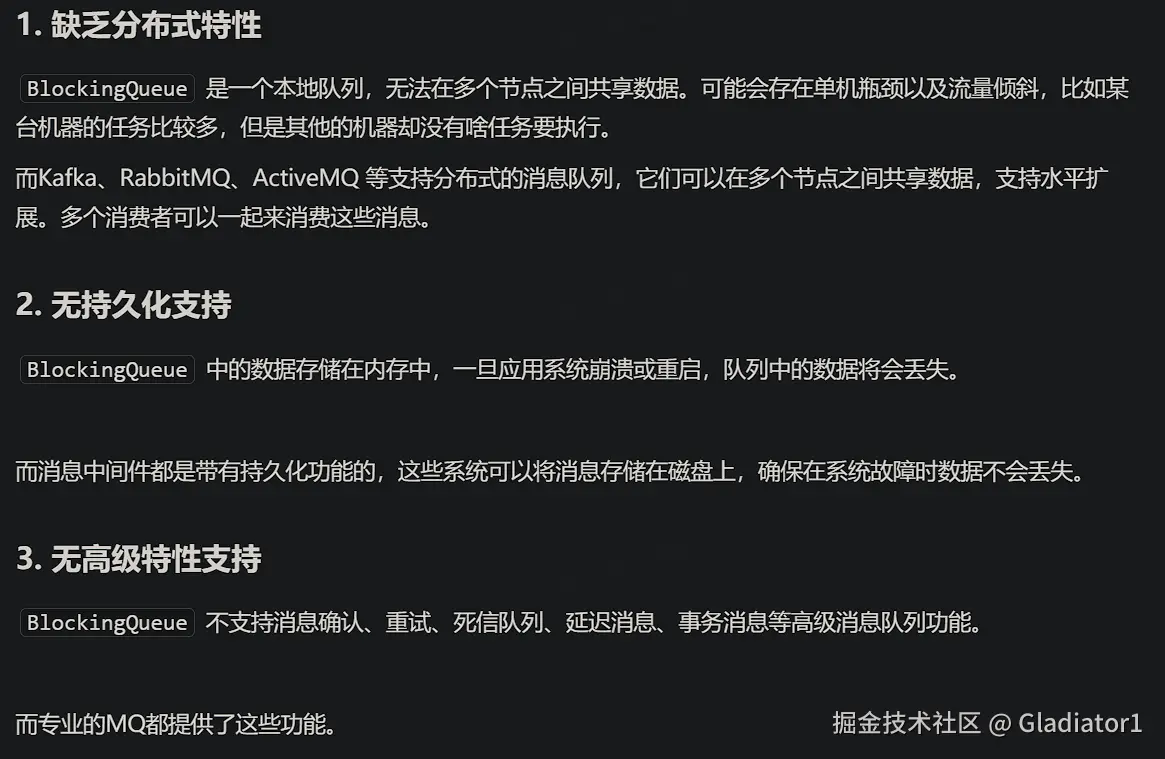
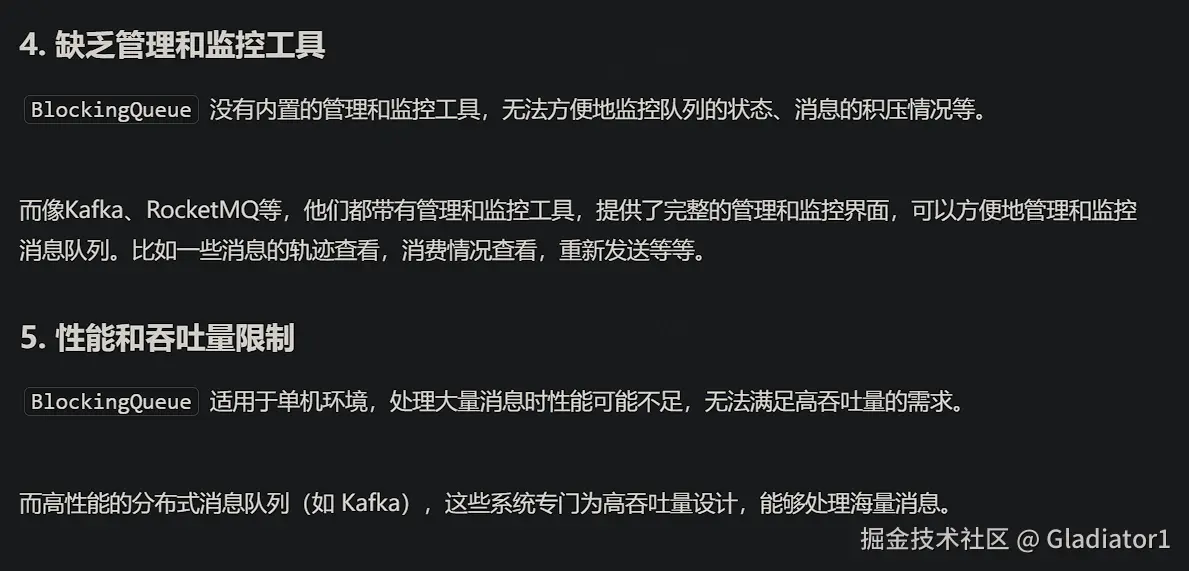
9、Spring Event和MQ有什么区别?各自适用场景是什么?
✅Spring Event和MQ有什么区别?各自适用场景是什么?
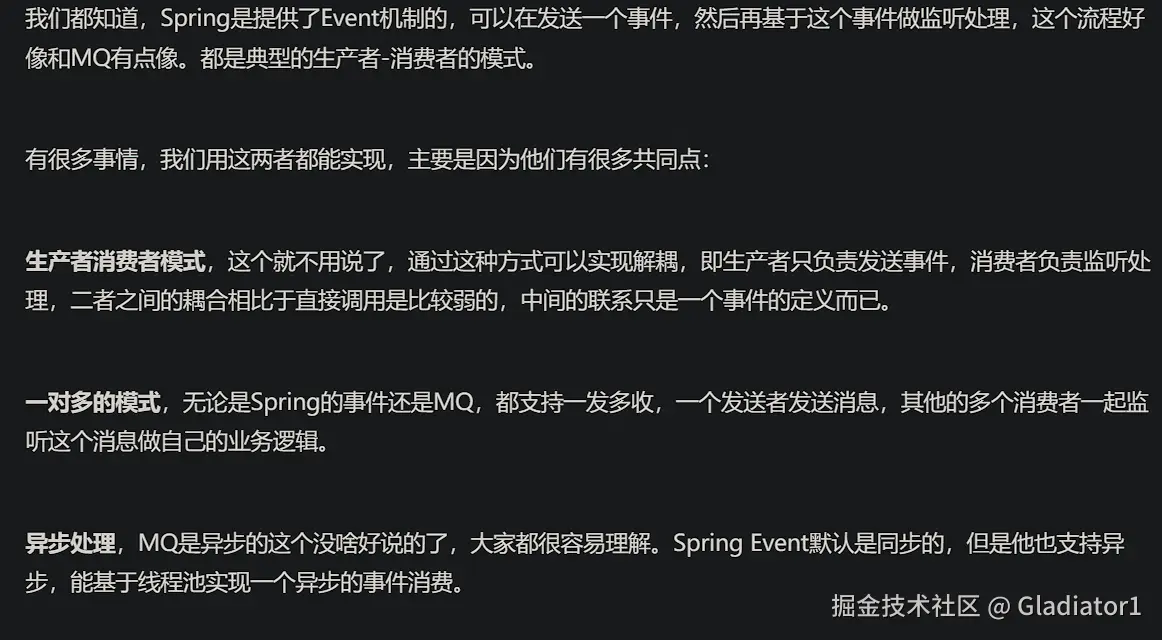
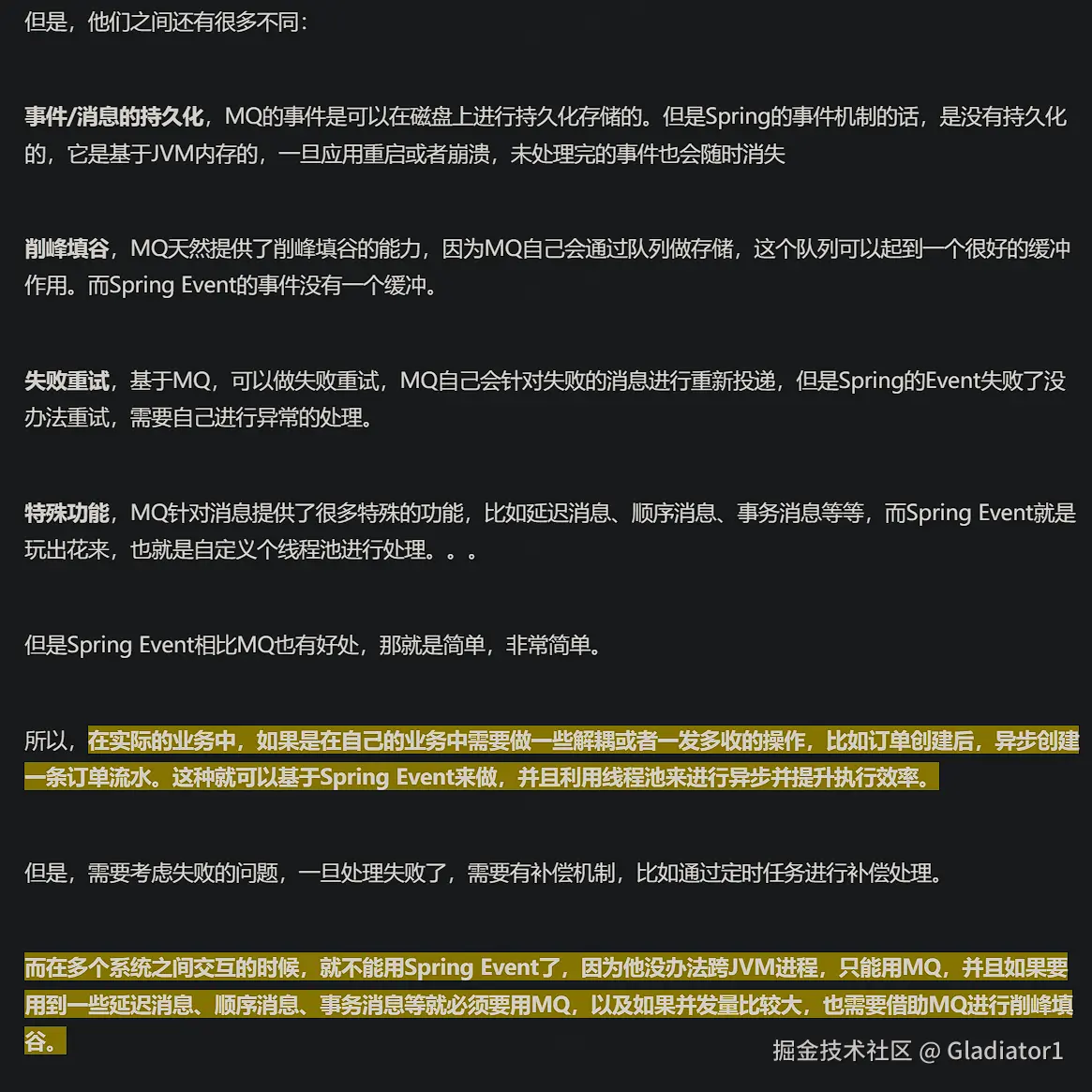