GraphQL中的使用Mutation查询,下面介绍详细操作,新建一个mutation.js文件。
1 定义schema
1.1 定义模型对象
(1)定义输入模型
input AccountInput {
name: String
age: Int
sex: String
department: String
}
(2)定义输出模型
type Account {
name: String
age: Int
sex: String
department: String
}
1.2 定义查询操作
定义了一个查询操作,返回所有用户数组
type Query {
accounts: [Account]
}
1.3 定义变更操作
type Mutation {
createAccount(input: AccountInput): Account
updateAccount(id: ID!, input: AccountInput): Account
}
2 实现处理器
2.1 定义查询处理函数
accounts() {
var arr = [];
for(const key in fakeDb) {
arr.push(fakeDb[key])
}
return arr;
},
2.2 定义变更处理函数
createAccount({ input }) {
// 相当于数据库的保存
fakeDb[input.name] = input;
// 返回保存结果
return fakeDb[input.name];
},
updateAccount({ id, input }) {
// 相当于数据库的更新
const updatedAccount = Object.assign({}, fakeDb[id], input);
fakeDb[id] = updatedAccount;
// 返回保存结果
return updatedAccount;
}
3 完整代码
const express = require('express');
const {buildSchema} = require('graphql');
const grapqlHTTP = require('express-graphql').graphqlHTTP;
// 定义schema,查询和类型, mutation
const schema = buildSchema(`
input AccountInput {
name: String
age: Int
sex: String
department: String
}
type Account {
name: String
age: Int
sex: String
department: String
}
type Mutation {
createAccount(input: AccountInput): Account
updateAccount(id: ID!, input: AccountInput): Account
}
type Query {
accounts: [Account]
}
`)
const fakeDb = {};
// 定义查询对应的处理器
const root = {
accounts() {
var arr = [];
for(const key in fakeDb) {
arr.push(fakeDb[key])
}
return arr;
},
createAccount({ input }) {
// 相当于数据库的保存
fakeDb[input.name] = input;
// 返回保存结果
return fakeDb[input.name];
},
updateAccount({ id, input }) {
// 相当于数据库的更新
const updatedAccount = Object.assign({}, fakeDb[id], input);
fakeDb[id] = updatedAccount;
// 返回保存结果
return updatedAccount;
}
}
const app = express();
app.use('/graphql', grapqlHTTP({
schema: schema,
rootValue: root,
graphiql: true
}))
app.listen(3000);
4 实例测试
启动程序
node mutation.js
访问http://localhost:3000/graphql
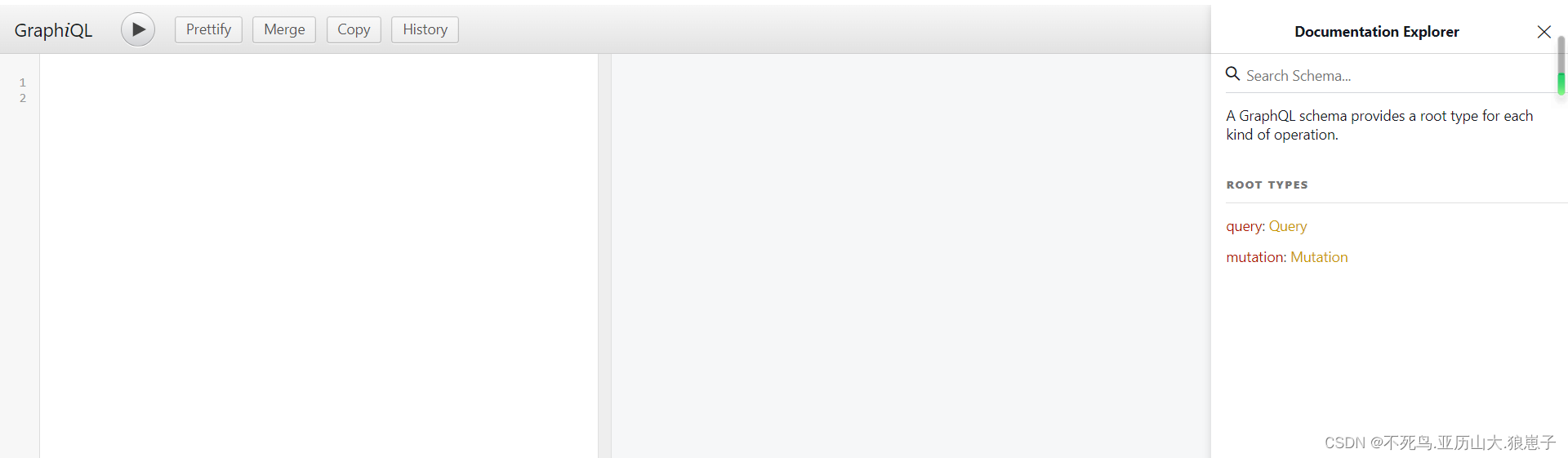
测试新增功能
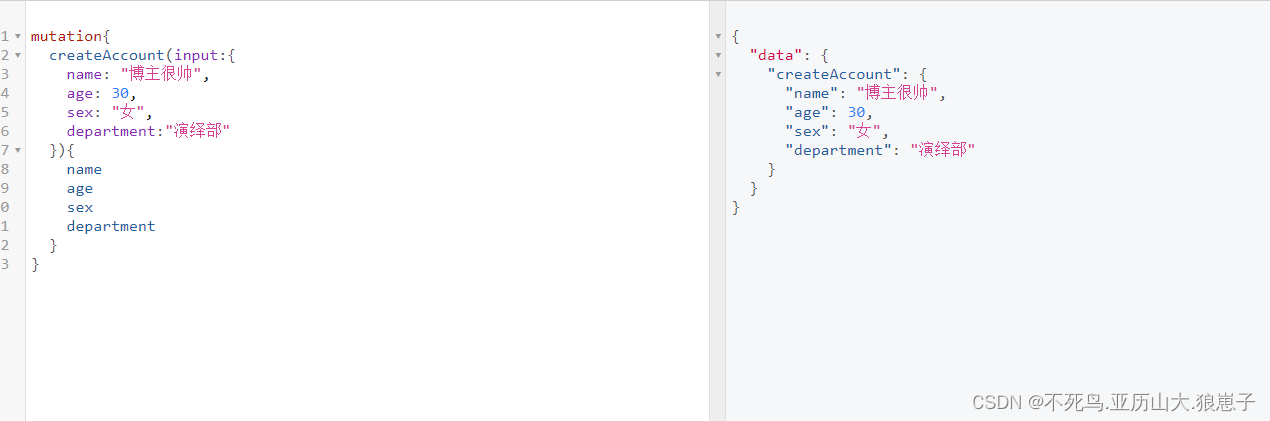
测试修改功能
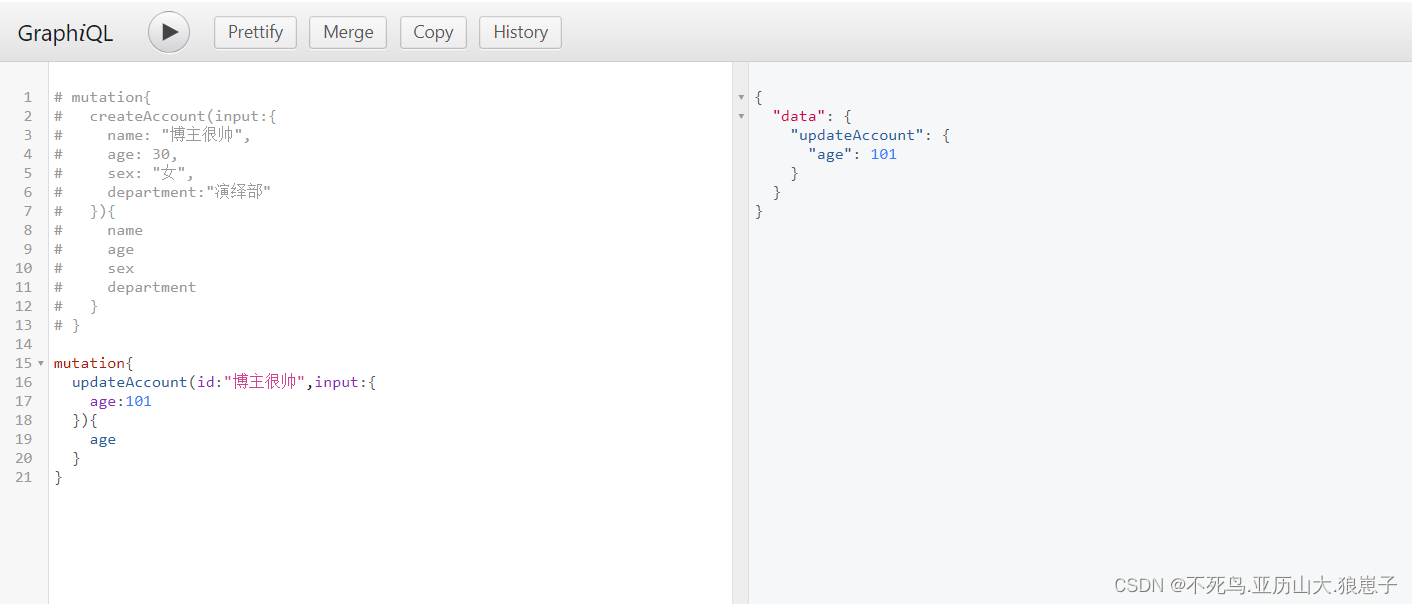
测试查询功能
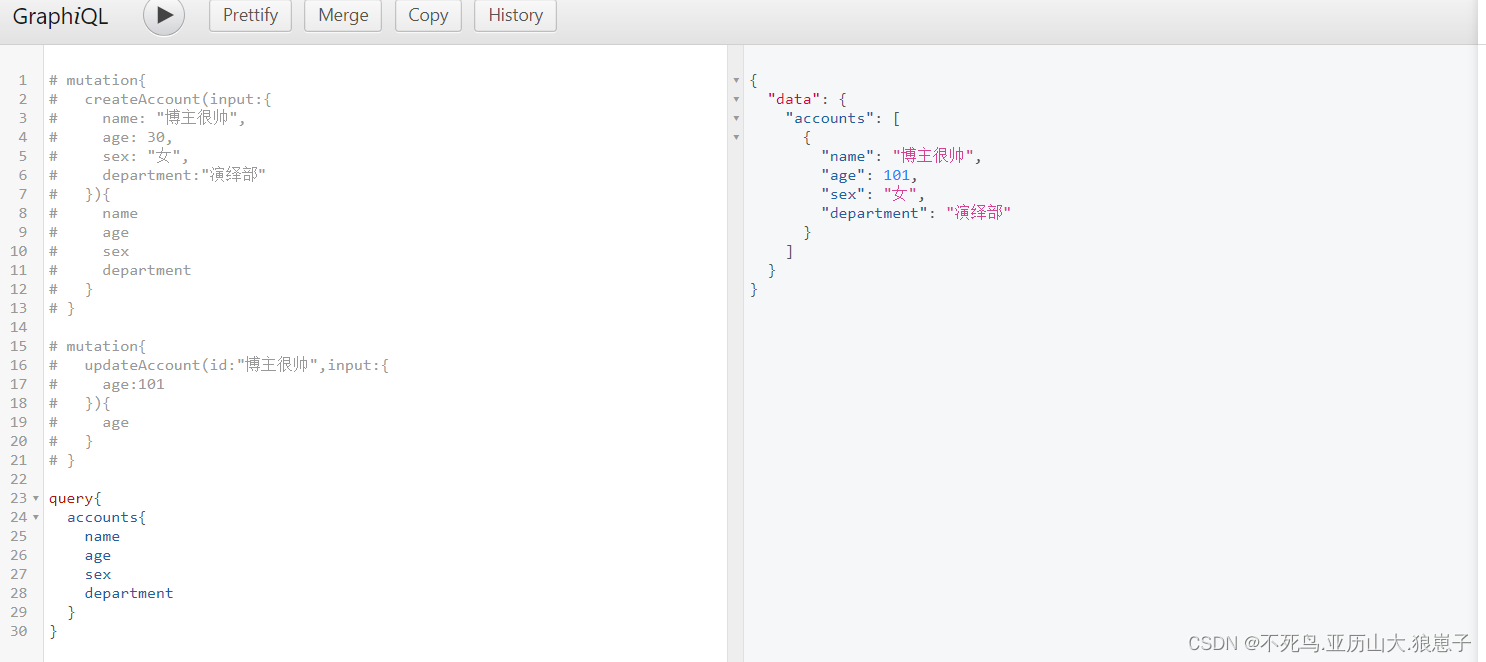