前言
最近领导交代了一个需求,就是有些许客户不单单满足平台告警日志外发到邮箱、短信的形式,还要以
消息聊天的形式外发给企业微信
。
具体操作
1、注册企业微信。
2、登录企业微信,找到应用管理,创建应用。
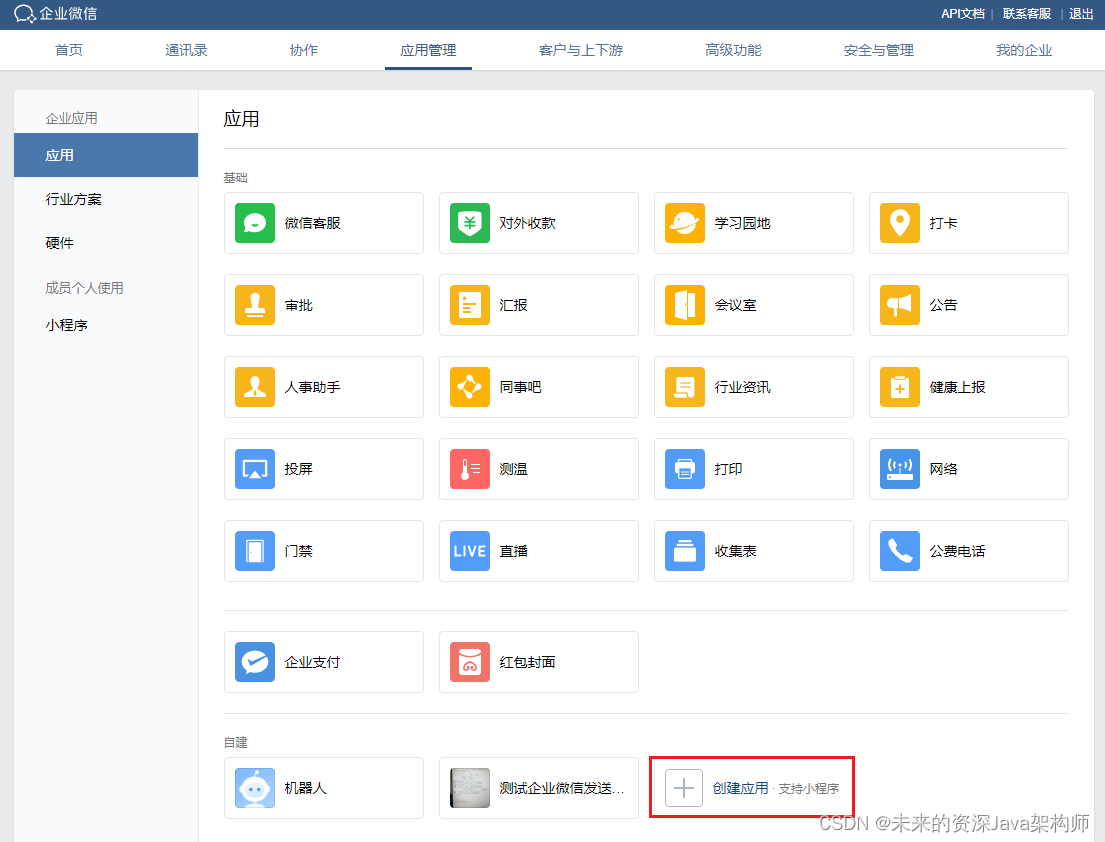
3、创建完之后需要记录以下图片中两个值的信息。
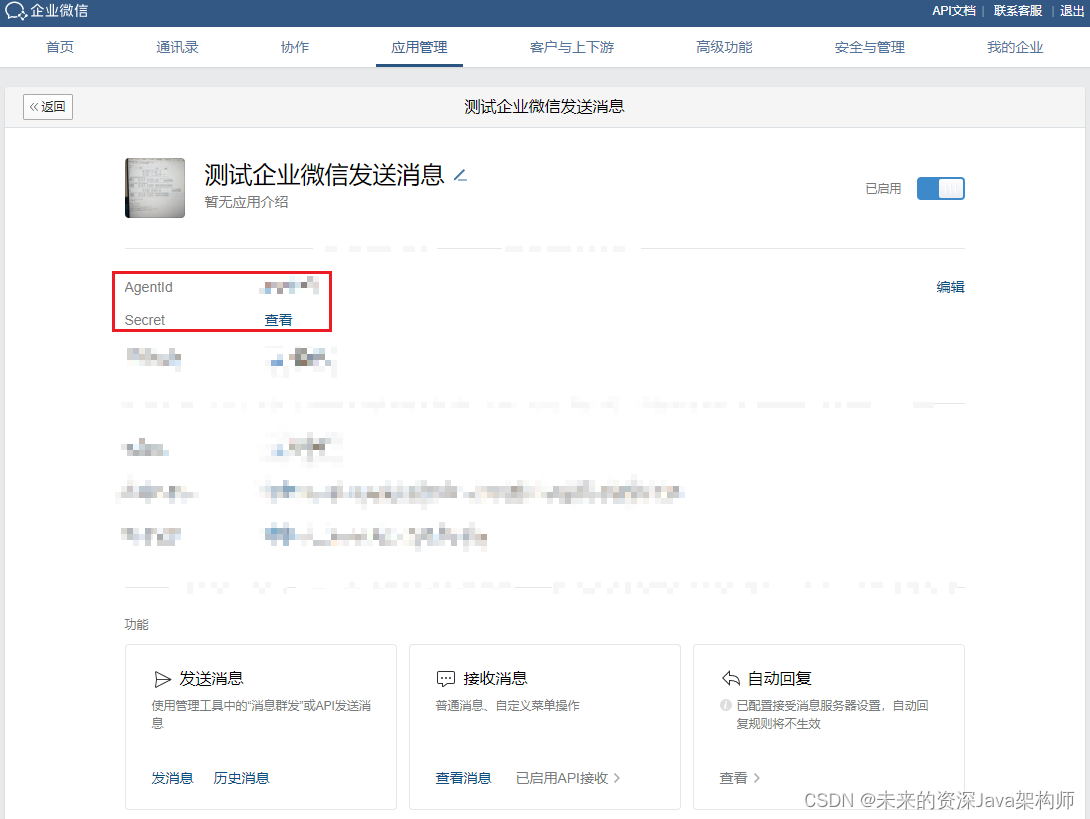
4、然后记录下本企业内任意帐号的名称。
5、再记录下本企业的id。
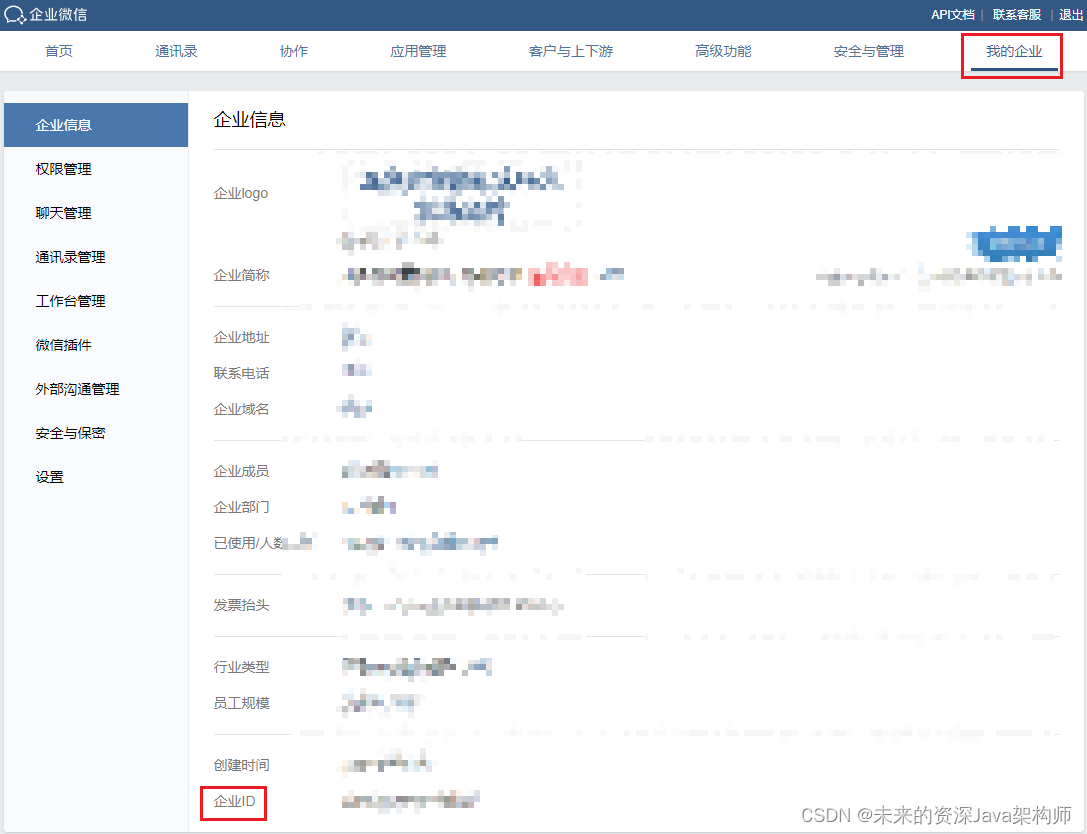
6、写一个接口,先获取token再发送消息。
java
@RestController
@RequestMapping("/weChat")
public class WeChatController {
private static final String CORP_ID = "xxx"; //企业id
private static final String CORP_SECRET = "xxx"; //企业的Secret
private static final String TO_USER = "xxx"; //账户名称
private static final String AGENT_ID = "xxx"; //企业的AgentId
private static final String ACCESS_TOKEN_URL = "https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=" + CORP_ID + "&corpsecret=" + CORP_SECRET;
private static final String SEND_MESSAGE_URL = "https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token=";
@GetMapping("/sendMessage")
public String sendMessage() {
String accessToken = getAccessToken();
if (accessToken != null) {
sendTextMessage(accessToken, "Hello, WeChat Work! This is a test message from Java.");
return "Message has send success.";
} else {
return "Failed to get access token.";
}
}
private String getAccessToken() {
try {
URL url = new URL(ACCESS_TOKEN_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Content-Type", "application/json");
connection.setDoOutput(true);
BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), "UTF-8"));
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
br.close();
JSONObject jsonObject = new JSONObject(response.toString());
return jsonObject.getStr("access_token");
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
private void sendTextMessage(String accessToken, String content) {
try {
URL url = new URL(SEND_MESSAGE_URL + accessToken);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setDoOutput(true);
JSONObject json = new JSONObject();
json.put("touser", TO_USER);
json.put("msgtype", "text");
json.put("agentid", AGENT_ID);
JSONObject text = new JSONObject();
text.put("content", content);
json.put("text", text);
String jsonString = json.toString();
byte[] outputInBytes = jsonString.getBytes("UTF-8");
connection.getOutputStream().write(outputInBytes, 0, outputInBytes.length);
BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), "UTF-8"));
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
br.close();
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
7、调用接口。
但是报错了,原因是本机ip不是可信ip,需要在企业微信上配置可信ip。
java
{
"errcode": 60020,
573 "errmsg": "not allow to access from your ip, hint: [1607593647887697837], from ip: xx.xx.xxx.xxx, more info at https://open.work.weixin.qq.com/devtool/query?e=60020"
}
8、配置可信ip。
不过配完了之后还是报60020错误,原因还是本企业为第三方服务商,还需要再配置个企业可信ip的白名单。
9、配置第三方服务商的企业可信ip白名单,在运营管理=》自己刚刚创建的应用=》最下方。
- 点击配置,发现里面置灰不可编辑,只有两种方式,设置可信域名或设置接收消息服务URL,两个都点开一脸懵逼。
- 后者还好,还有说明文档,
点击帮助
,跳转到帮助文档中,发现需要自己提供一个接口供企业微信调用,调用成功之后才能使API接收消息成功,进而开启企业微信可信IP白名单的填写权限。
10、下载源码,放在项目中,再自行写一个接口
,使用企业微信调用,源码地址:https://developer.work.weixin.qq.com/devtool/introduce?id=10128
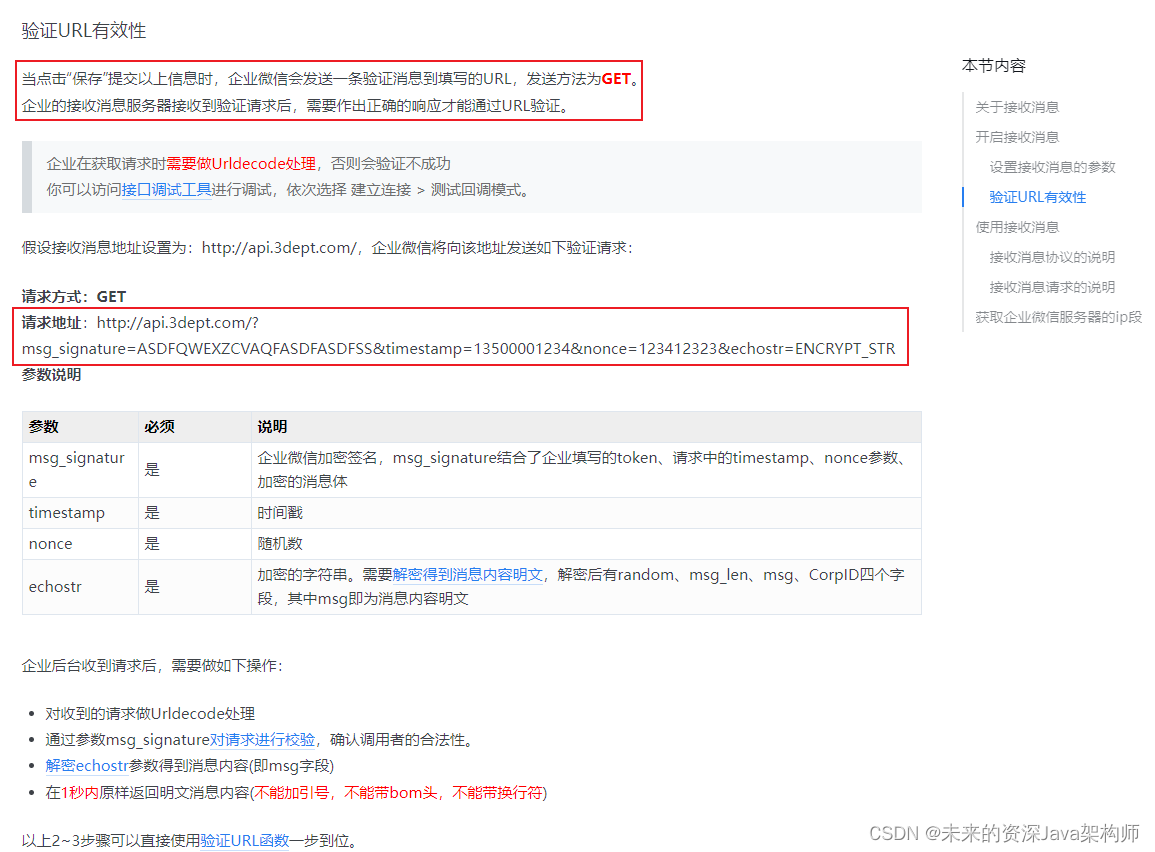
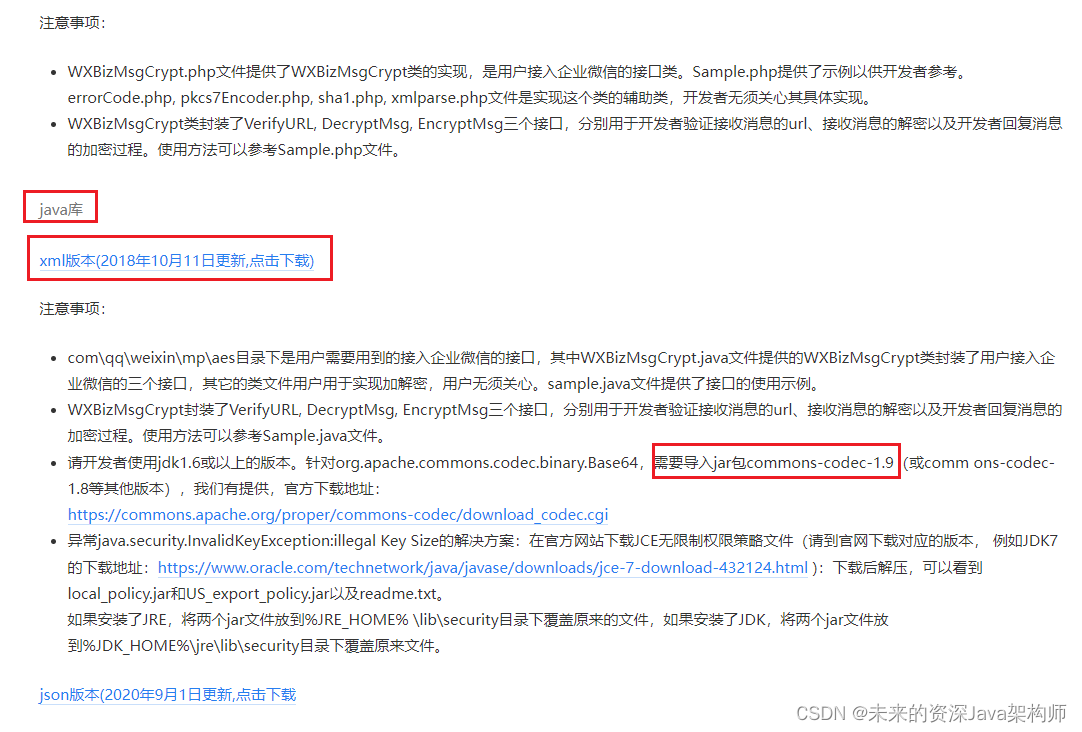
11、供企业微信调用的Java接口。
java
@RestController
@RequestMapping("/weChat")
public class WeChatController {
private static final String CORP_ID = "xxx"; //企业id
private static final String token = "xxx"; //就是第9步里面第二种方案中自动生成的Token
private static final String encodingAESKey = "xxx"; //就是第9步里面第二种方案中自动生成的EncodingAESKey
@GetMapping("/receiveMessage")
public String receiveMsg(String msg_signature, String timestamp, String nonce, String echostr) throws UnsupportedEncodingException {
echostr = this.handleEchoStr(echostr);
String res = null;
try {
res = this.getEchoStr(msg_signature, timestamp, nonce, echostr);
} catch (AesException e) {
throw new RuntimeException(e);
}
return res;
}
/**
* 处理echostr
*
* @param verifyEchoStr
* @return
* @throws UnsupportedEncodingException
*/
public static String handleEchoStr(String verifyEchoStr) throws UnsupportedEncodingException {
String enStr = URLEncoder.encode(verifyEchoStr, "UTF-8");
String reStr = enStr.replace("+", "%2B");
String echostr = URLDecoder.decode(reStr, "UTF-8");
return echostr;
}
/**
*
* @param msg_signature
* @param timestamp
* @param nonce
* @param echostr
* @return
* @throws AesException
*/
public static String getEchoStr(String msg_signature, String timestamp, String nonce, String echostr) throws AesException {
WXBizMsgCrypt wxcpt = new WXBizMsgCrypt(token, encodingAESKey, CORP_ID);
String sEchoStr = null; //需要返回的明文
try {
sEchoStr = wxcpt.VerifyURL(msg_signature, timestamp,
nonce, echostr);
System.out.println("verifyurl echostr: " + sEchoStr);
// 验证URL成功,将sEchoStr返回
// HttpUtils.SetResponse(sEchoStr);
} catch (Exception e) {
//验证URL失败,错误原因请查看异常
e.printStackTrace();
}
return sEchoStr;
}
}
12、写完之后运行项目,企业微信点击保存。
但是报错:
openapi回调地址请求不通过
。原因:项目必须放在公网上,以公网ip的形式供企业微信调用,所以此时你需要一台阿里云服务器,
正好我有
,哈哈。
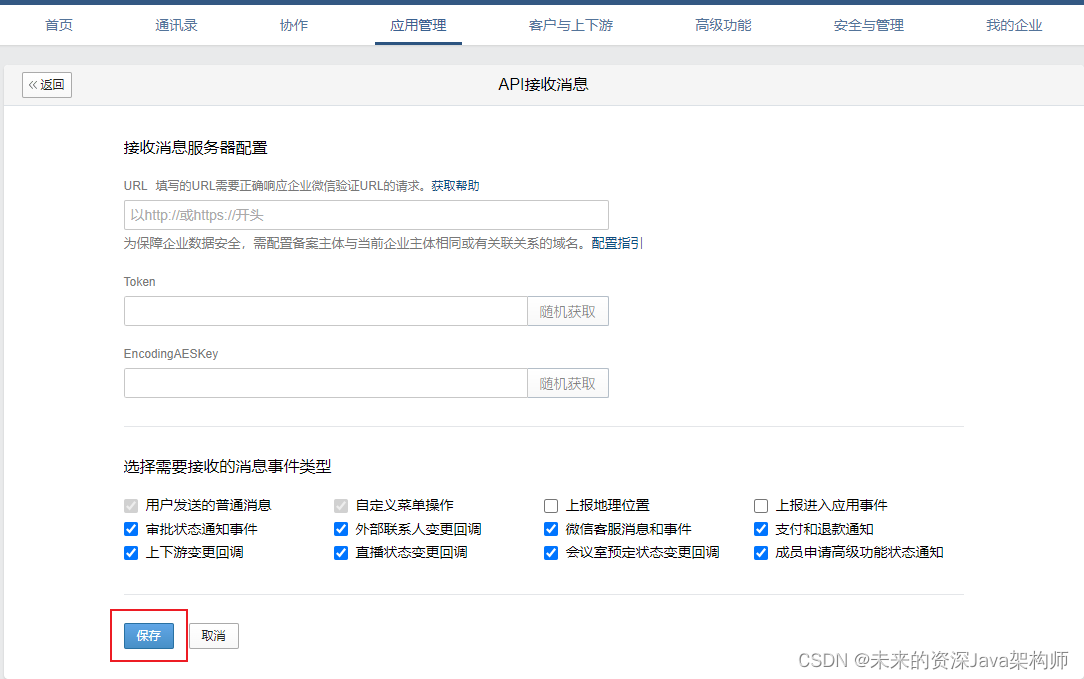
13、编写Dockerfile,将项目放在阿里云服务器上,生成镜像=》容器=》启动》企业微信点击保存即可成功。
14、在可信ip白名单中填写ip即可。
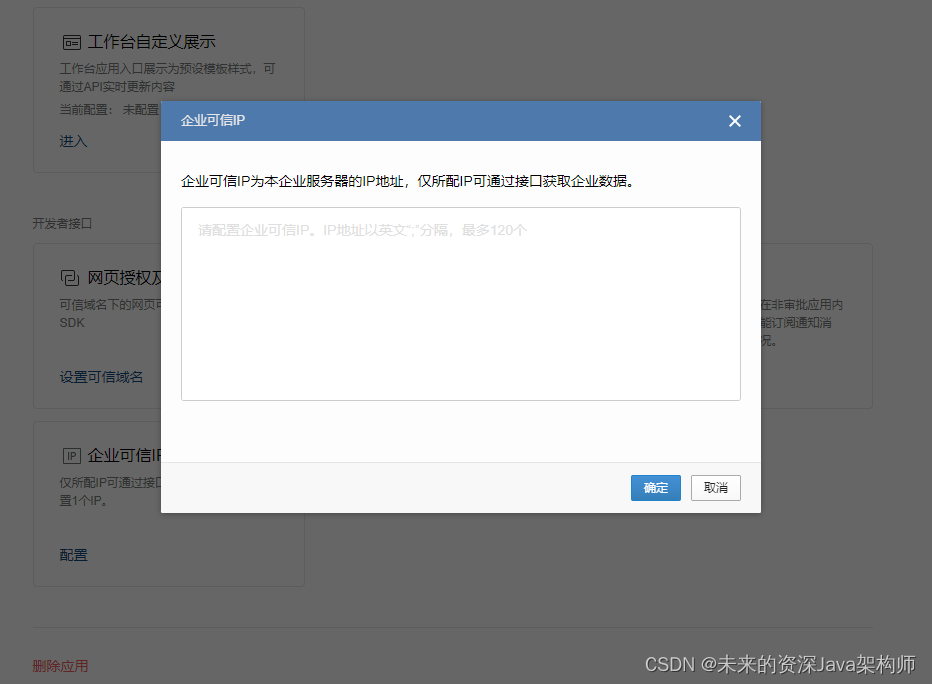
15、填写完,再调用第6步的接口即可。
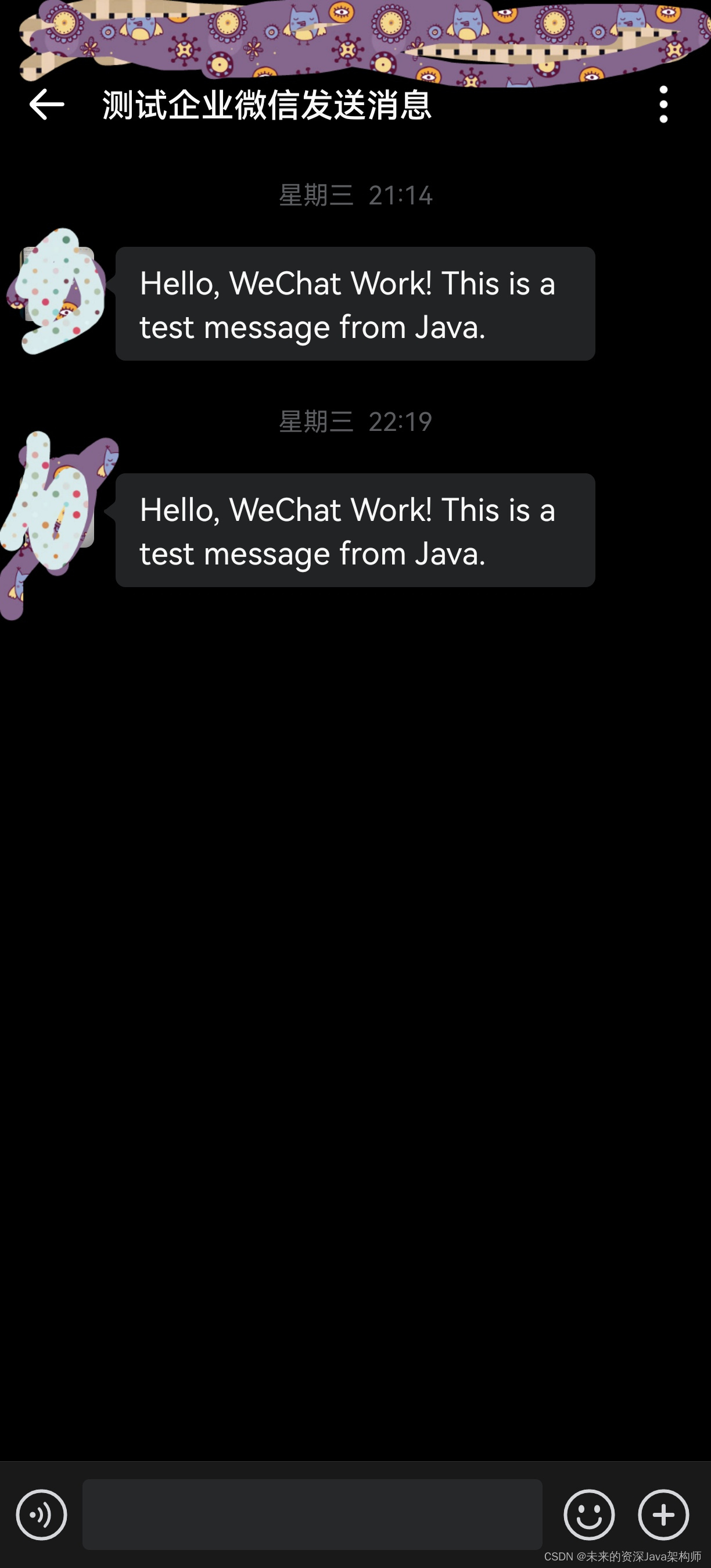
最终
亲测可用