文章目录
简介
- Spring Cache是一个框架,实现了基于注解的缓存功能
- 底层可以使用EHCache、Caffeine、Redis实现缓存。
注解一般放在Controller的方法上,@CachePut 注解一般有两个参数,第一个时存储的名称,第二个时名称后边的key,使用SpEL动态的计算key。其余的注解也都是这两个参数。在用户端的查询操作需要使用@Cacheable,服务器端的增删改都使用@CacheEvict
引入依赖
xml
SpringCache
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
Redis
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
mysql
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
mybatis
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
application.yml配置
yml
server:
port: 8888
spring:
datasource:
druid:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/test?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&useSSL=false&allowPublicKeyRetrieval=true
username: root
password: 123456
redis:
host: localhost
port: 6379
password: 123456
database: 1
logging:
level:
com:
itheima:
mapper: debug
service: info
controller: info
常用注解
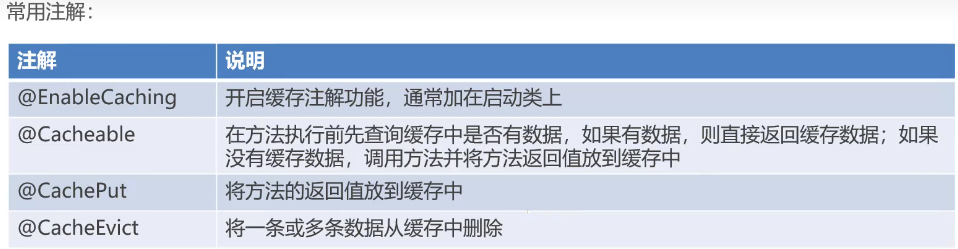
使用
1. 启动类添加注解
java
package com.itheima;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@Slf4j
@SpringBootApplication
-----------------------------------------
添加下面的注解
@EnableCaching
-----------------------------------------
public class CacheDemoApplication {
public static void main(String[] args) {
SpringApplication.run(CacheDemoApplication.class,args);
log.info("项目启动成功...");
}
}
使用方法上添加注解
java
package com.itheima.controller;
import com.itheima.entity.User;
import com.itheima.mapper.UserMapper;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/user")
@Slf4j
public class UserController {
@Autowired
private UserMapper userMapper;
/**
* 新增方法返回值放入缓存
*/
@PostMapping
//参数1,缓存名称,参数2,动态获取方法参数字段
//最总保存效果是 [参数1::参数2]。例如[userCache::1]
@CachePut(cacheNames = "userCache", key = "#user.id")
//也可以这样写,参数2不再是方法参数,而是方法的返回值
//@CachePut(cacheNames = "userCache",key = "#result.id")
//也可以这样写,p0代表第一个参数,p1代表第二个参数
//@CachePut(cacheNames = "userCache", key = "#p0.id")
public User save(@RequestBody User user) {
userMapper.insert(user);
return user;
}
//删除 userCache::id
@CacheEvict(cacheNames = "userCache", key = "#id")
@DeleteMapping
public void deleteById(Long id) {
userMapper.deleteById(id);
}
//删除 所有的 userCache::*
@CacheEvict(cacheNames = "userCache", allEntries = true)
@DeleteMapping("/delAll")
public void deleteAll() {
userMapper.deleteAll();
}
//cache生成 userCache::id
@Cacheable(cacheNames = "userCache", key = "#id")
@GetMapping
public User getById(Long id) {
User user = userMapper.getById(id);
return user;
}
}