15 ArcGIS Pro 后台选项卡
15.1 添加控件
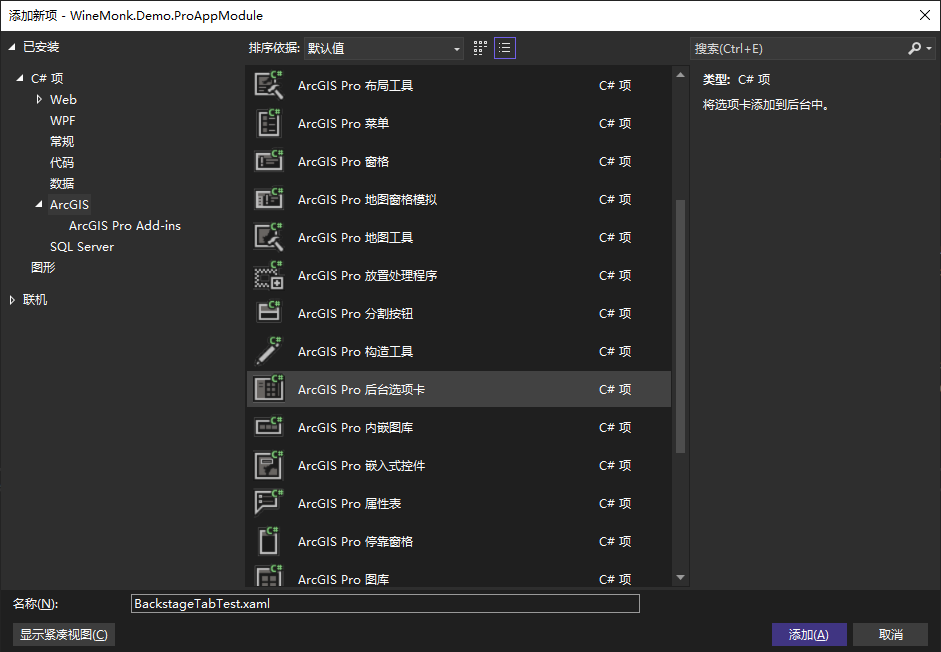
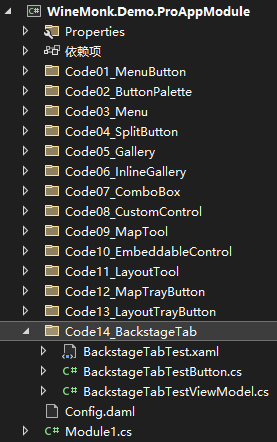
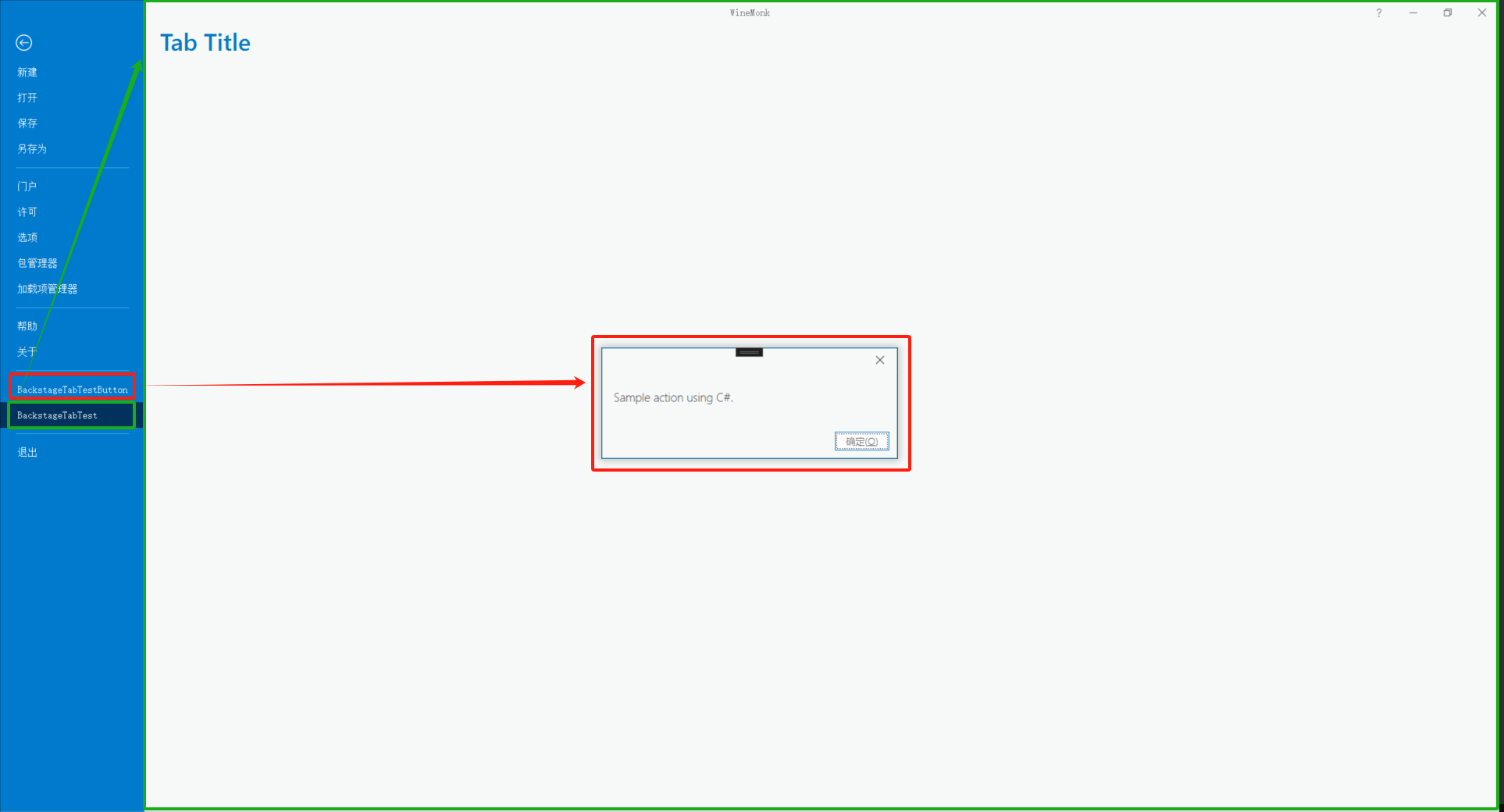
15.2 Code
15.2.1 选项卡按钮
BackstageTabTestButton.cs
csharp
using ArcGIS.Desktop.Framework.Contracts;
using ArcGIS.Desktop.Framework.Dialogs;
namespace WineMonk.Demo.ProAppModule.Code14_BackstageTab
{
internal class BackstageTabTestButton : Button
{
protected override void OnClick()
{
MessageBox.Show("Sample action using C#.");
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1"> <controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code14_BackstageTab_BackstageTabTest_Button" caption="BackstageTabTestButton" className="WineMonk.Demo.ProAppModule.Code14_BackstageTab.BackstageTabTestButton" loadOnClick="true">
<tooltip heading="BackstageTab Button Heading">BackstageTab button tool tip text.<disabledText /></tooltip>
</button>
</controls>
</insertModule>
</modules>
<backstage>
<insertButton refID="WineMonk_Demo_ProAppModule_Code14_BackstageTab_BackstageTabTest_Button" insert="before" placeWith="esri_core_exitApplicationButton" separator="true" />
</backstage>
15.2.2 选项卡页
BackstageTabTest.xaml
xaml
<UserControl x:Class="WineMonk.Demo.ProAppModule.Code14_BackstageTab.BackstageTabTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code14_BackstageTab"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{Binding Path=ui.BackstageTabTestViewModel}">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid Margin="20,0">
<StackPanel>
<TextBlock Style="{DynamicResource Esri_TextBlockBackStageTitle}" Text="{Binding TabHeading}" />
<!-- design content for the tab here -->
</StackPanel>
</Grid>
</UserControl>
BackstageTabTestViewModel.cs
csharp
using ArcGIS.Desktop.Framework.Contracts;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code14_BackstageTab
{
internal class BackstageTabTestViewModel : BackstageTab
{
/// <summary>
/// Called when the backstage tab is selected.
/// </summary>
protected override Task InitializeAsync()
{
return base.InitializeAsync();
}
/// <summary>
/// Called when the backstage tab is unselected.
/// </summary>
protected override Task UninitializeAsync()
{
return base.UninitializeAsync();
}
private string _tabHeading = "Tab Title";
public string TabHeading
{
get
{
return _tabHeading;
}
set
{
SetProperty(ref _tabHeading, value, () => TabHeading);
}
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
</insertModule>
</modules>
<backstage>
<insertTab id="WineMonk_Demo_ProAppModule_Code14_BackstageTab_BackstageTabTest" caption="BackstageTabTest" className="WineMonk.Demo.ProAppModule.Code14_BackstageTab.BackstageTabTestViewModel" insert="before" placeWith="esri_core_exitApplicationButton">
<content className="WineMonk.Demo.ProAppModule.Code14_BackstageTab.BackstageTabTestView" />
</insertTab>
</backstage>
16 ArcGIS Pro 窗体
16.1 添加控件
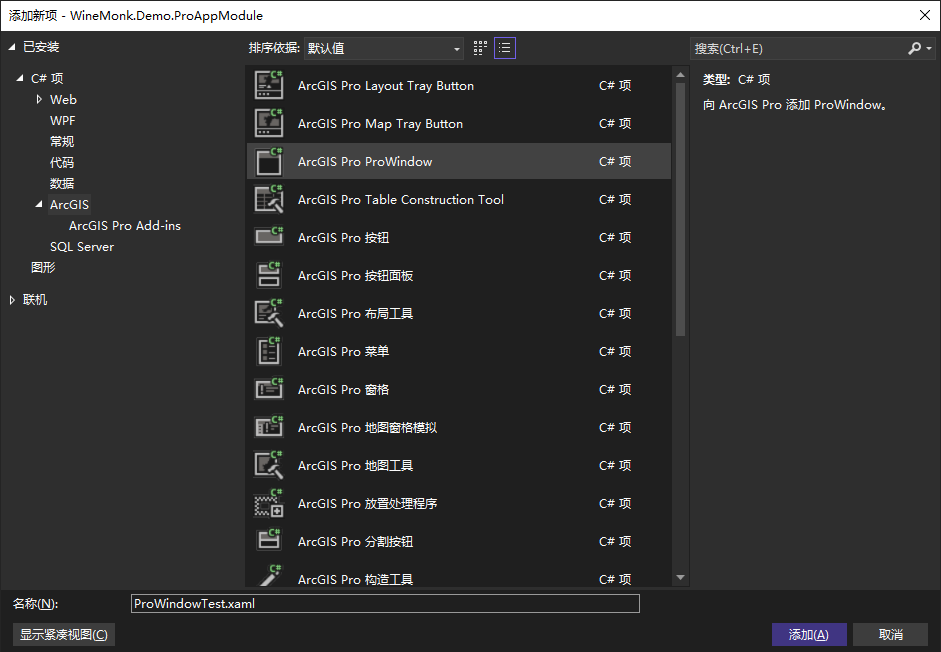
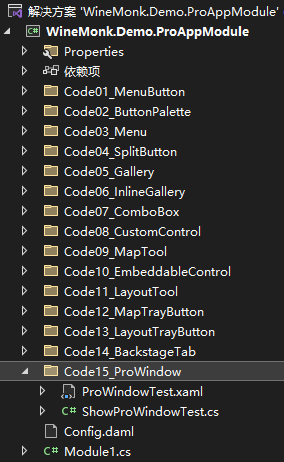
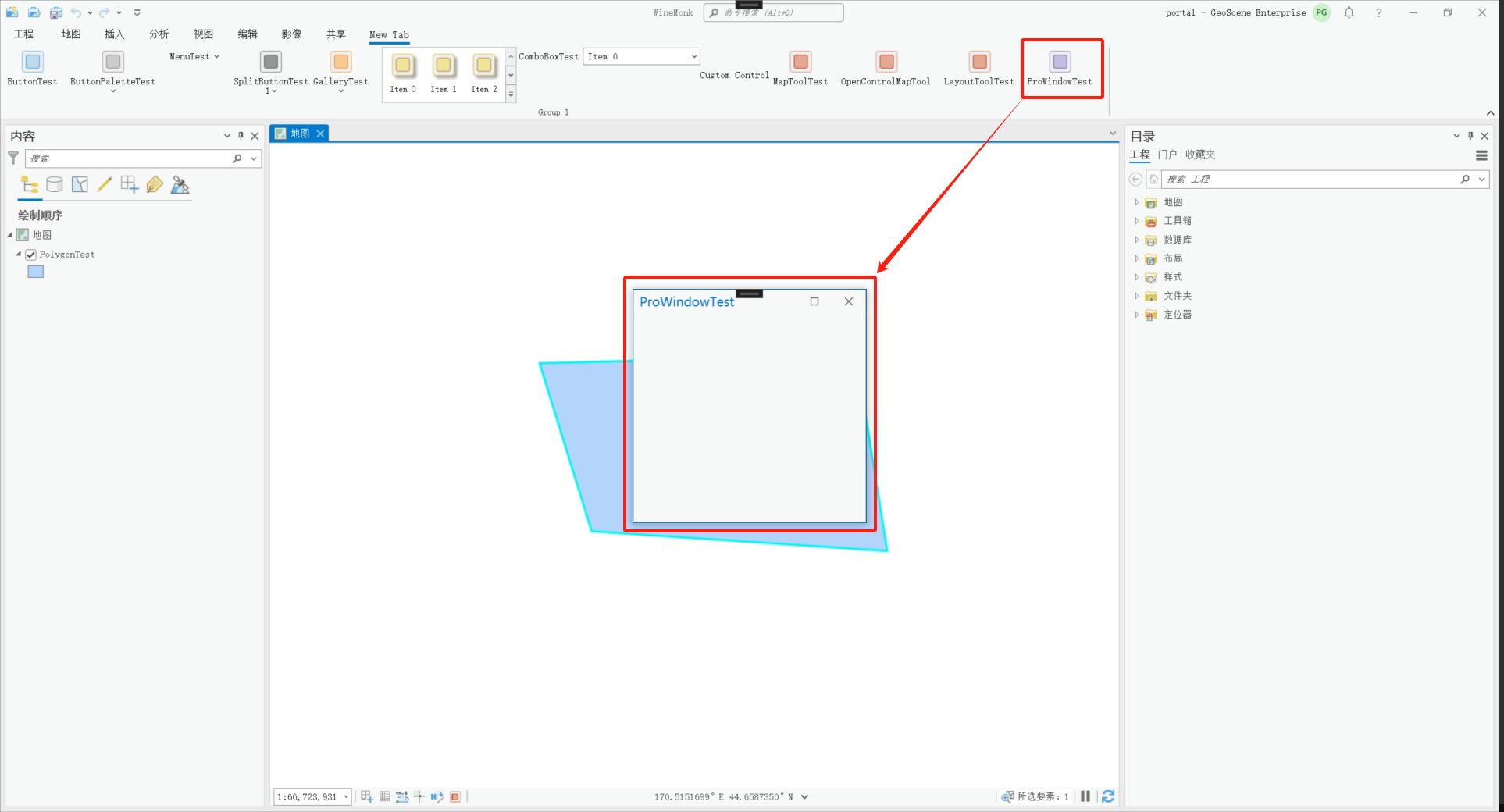
16.2 Code
ShowProWindowTest.cs
csharp
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
namespace WineMonk.Demo.ProAppModule.Code15_ProWindow
{
internal class ShowProWindowTest : Button
{
private ProWindowTest _prowindowtest = null;
protected override void OnClick()
{
//already open?
if (_prowindowtest != null)
return;
_prowindowtest = new ProWindowTest();
_prowindowtest.Owner = FrameworkApplication.Current.MainWindow;
_prowindowtest.Closed += (o, e) => { _prowindowtest = null; };
_prowindowtest.Show();
//uncomment for modal
//_prowindowtest.ShowDialog();
}
}
}
ProWindowTest.xaml
xaml
<controls:ProWindow x:Class="WineMonk.Demo.ProAppModule.Code15_ProWindow.ProWindowTest"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:controls="clr-namespace:ArcGIS.Desktop.Framework.Controls;assembly=ArcGIS.Desktop.Framework"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
mc:Ignorable="d"
Title="ProWindowTest" Height="300" Width="300"
WindowStartupLocation="CenterOwner"
>
<controls:ProWindow.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</controls:ProWindow.Resources>
<Grid>
</Grid>
</controls:ProWindow>
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code15_ProWindow_ProWindowTest" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code15_ProWindow_ProWindowTest" caption="ProWindowTest" className="WineMonk.Demo.ProAppModule.Code15_ProWindow.ShowProWindowTest" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple32.png">
<tooltip heading="Tooltip Heading">Tooltip text<disabledText /></tooltip>
</button>
</controls>
</insertModule>
</modules>
17 ArcGIS Pro 属性表
17.1 添加控件
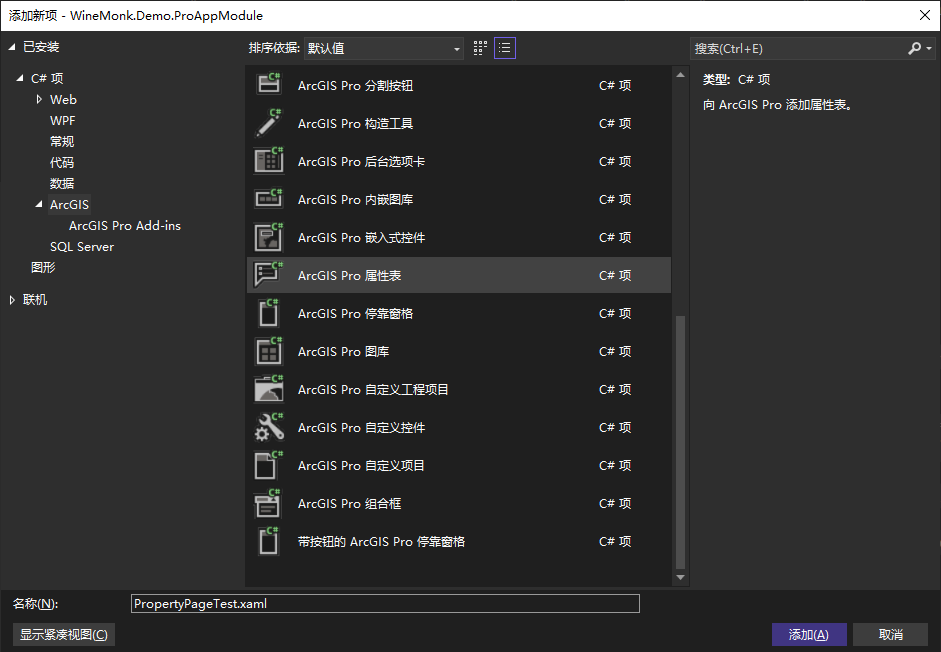
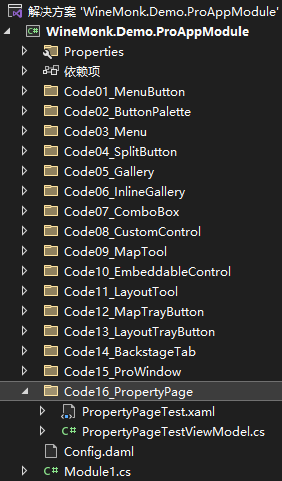
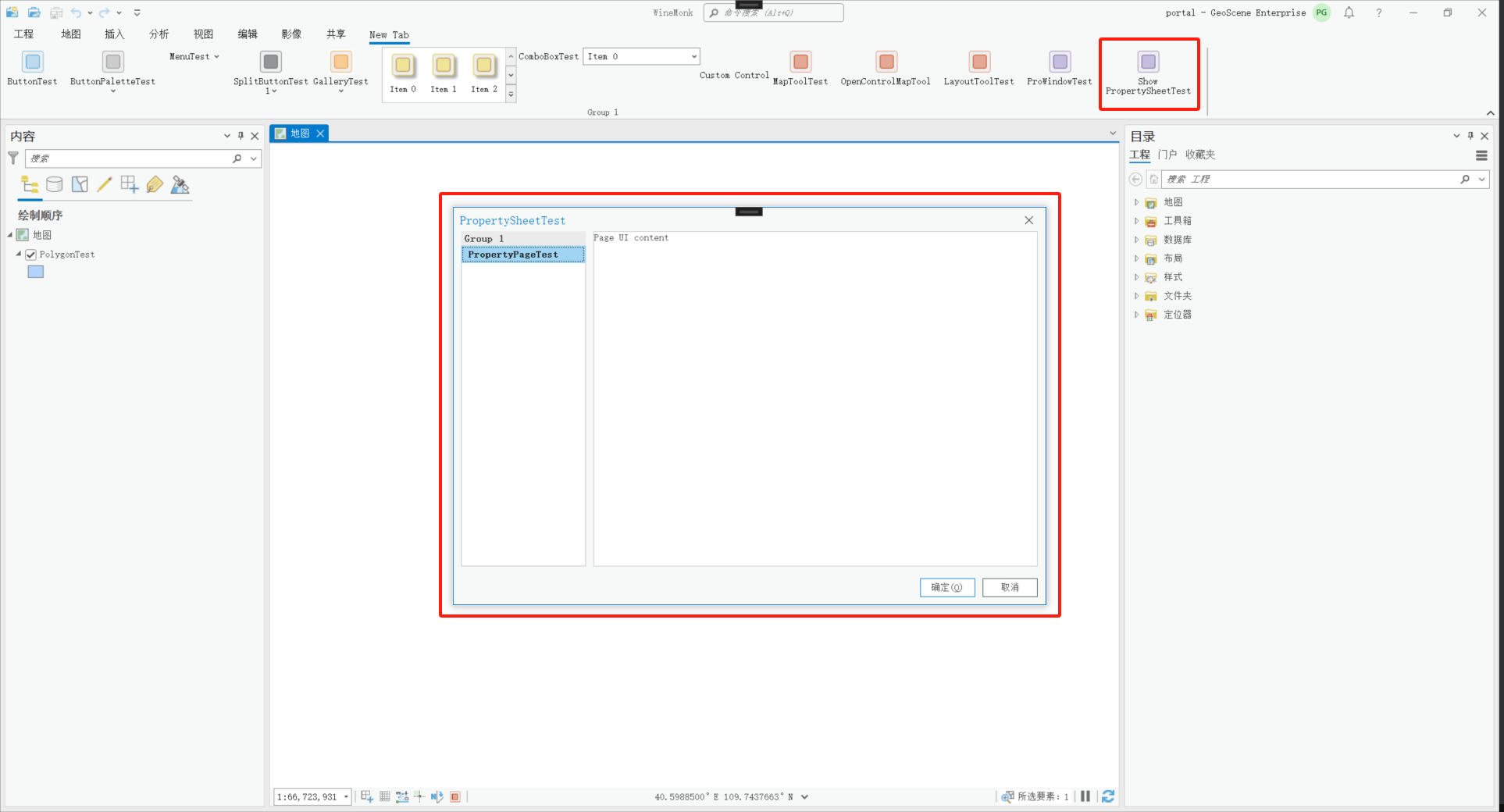
17.2 Code
PropertyPageTest.xaml
xaml
<UserControl
x:Class="WineMonk.Demo.ProAppModule.Code16_PropertyPage.PropertyPageTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code16_PropertyPage"
d:DataContext="{Binding Path=ui.PropertyPageTestViewModel}"
d:DesignHeight="300"
d:DesignWidth="300"
mc:Ignorable="d">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<!-- Replace text block below with your UI components. -->
<TextBlock Style="{DynamicResource Esri_TextBlockRegular}" Text="{Binding DataUIContent}" />
</Grid>
</UserControl>
PropertyPageTestViewModel.cs
csharp
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
using System;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code16_PropertyPage
{
internal class PropertyPageTestViewModel : Page
{
/// <summary>
/// Invoked when the OK or apply button on the property sheet has been clicked.
/// </summary>
/// <returns>A task that represents the work queued to execute in the ThreadPool.</returns>
/// <remarks>This function is only called if the page has set its IsModified flag to true.</remarks>
protected override Task CommitAsync()
{
return Task.FromResult(0);
}
/// <summary>
/// Called when the page loads because it has become visible.
/// </summary>
/// <returns>A task that represents the work queued to execute in the ThreadPool.</returns>
protected override Task InitializeAsync()
{
return Task.FromResult(true);
}
/// <summary>
/// Called when the page is destroyed.
/// </summary>
protected override void Uninitialize()
{
}
/// <summary>
/// Text shown inside the page hosted by the property sheet
/// </summary>
public string DataUIContent
{
get
{
return base.Data[0] as string;
}
set
{
SetProperty(ref base.Data[0], value, () => DataUIContent);
}
}
}
/// <summary>
/// Button implementation to show the property sheet.
/// </summary>
internal class PropertyPageTest_ShowButton : Button
{
protected override void OnClick()
{
// collect data to be passed to the page(s) of the property sheet
Object[] data = new object[] { "Page UI content" };
if (!PropertySheet.IsVisible)
PropertySheet.ShowDialog("WineMonk_Demo_ProAppModule_Code16_PropertySheet_PropertySheetTest", "WineMonk_Demo_ProAppModule_Code16_PropertyPage_PropertyPageTest", data);
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code16_PropertySheet_PropertySheetTest_ShowPropertySheet" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code16_PropertySheet_PropertySheetTest_ShowPropertySheet" caption="Show PropertySheetTest" className="WineMonk.Demo.ProAppModule.Code16_PropertyPage.PropertyPageTest_ShowButton" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple32.png">
<tooltip heading="Show Property Sheet">Show Property Sheet<disabledText /></tooltip>
</button>
</controls>
</insertModule>
</modules>
<propertySheets>
<insertSheet id="WineMonk_Demo_ProAppModule_Code16_PropertySheet_PropertySheetTest" caption="PropertySheetTest" hasGroups="true">
<page id="WineMonk_Demo_ProAppModule_Code16_PropertyPage_PropertyPageTest" caption="PropertyPageTest" className="WineMonk.Demo.ProAppModule.Code16_PropertyPage.PropertyPageTestViewModel" group="Group 1">
<content className="WineMonk.Demo.ProAppModule.Code16_PropertyPage.PropertyPageTestView" />
</page>
</insertSheet>
</propertySheets>
18 ArcGIS Pro 窗格
18.1 添加控件
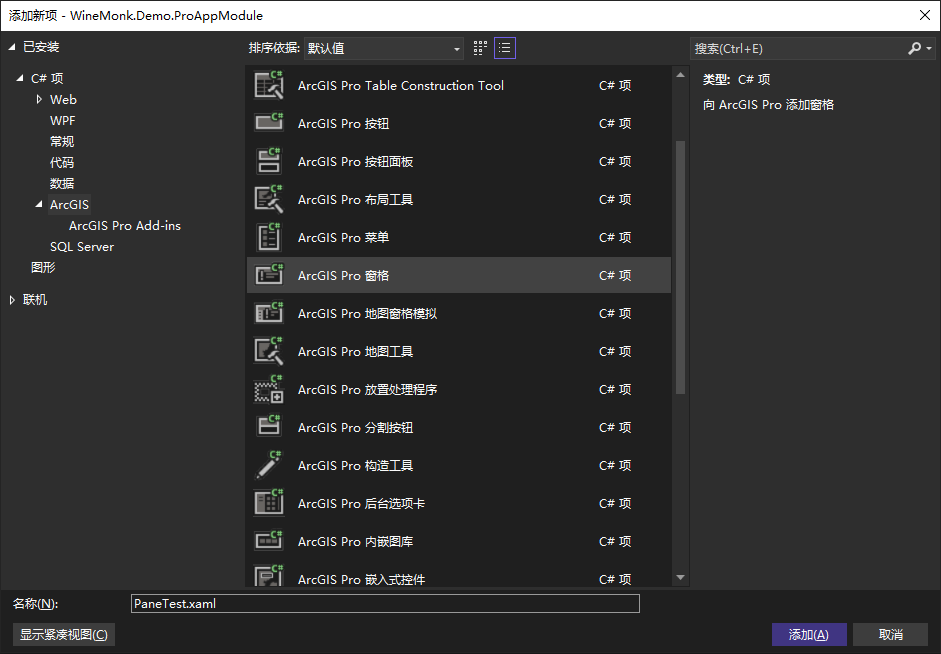
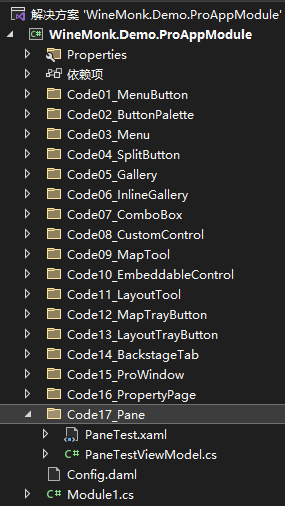
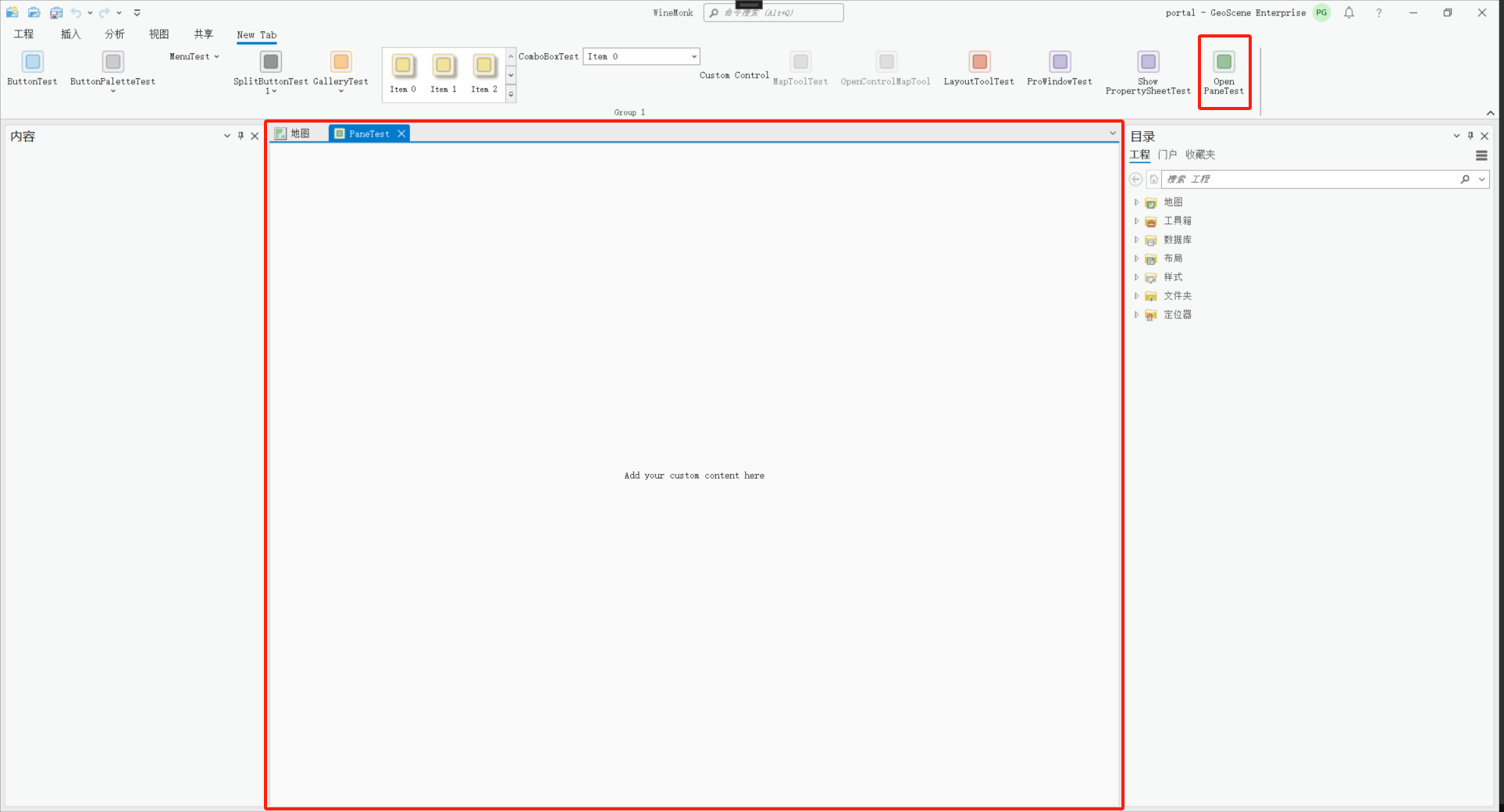
18.2 Code
PaneTest.xaml
xaml
<UserControl x:Class="WineMonk.Demo.ProAppModule.Code17_Pane.PaneTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code17_Pane"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{Binding Path=ui.PaneTestViewModel}">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<TextBlock Text="Add your custom content here" VerticalAlignment="Center" HorizontalAlignment="Center"></TextBlock>
</Grid>
</UserControl>
PaneTestViewModel.cs
csharp
using ArcGIS.Core.CIM;
using ArcGIS.Desktop.Core;
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code17_Pane
{
internal class PaneTestViewModel : ViewStatePane
{
private const string _viewPaneID = "WineMonk_Demo_ProAppModule_Code17_Pane_PaneTest";
/// <summary>
/// Consume the passed in CIMView. Call the base constructor to wire up the CIMView.
/// </summary>
public PaneTestViewModel(CIMView view)
: base(view) { }
/// <summary>
/// Create a new instance of the pane.
/// </summary>
internal static PaneTestViewModel Create()
{
var view = new CIMGenericView();
view.ViewType = _viewPaneID;
return FrameworkApplication.Panes.Create(_viewPaneID, new object[] { view }) as PaneTestViewModel;
}
#region Pane Overrides
/// <summary>
/// Must be overridden in child classes used to persist the state of the view to the CIM.
/// </summary>
public override CIMView ViewState
{
get
{
_cimView.InstanceID = (int)InstanceID;
return _cimView;
}
}
/// <summary>
/// Called when the pane is initialized.
/// </summary>
protected async override Task InitializeAsync()
{
await base.InitializeAsync();
}
/// <summary>
/// Called when the pane is uninitialized.
/// </summary>
protected async override Task UninitializeAsync()
{
await base.UninitializeAsync();
}
#endregion Pane Overrides
}
/// <summary>
/// Button implementation to create a new instance of the pane and activate it.
/// </summary>
internal class PaneTest_OpenButton : Button
{
protected override void OnClick()
{
PaneTestViewModel.Create();
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code17_Pane_PaneTest_OpenButton" size="large" />
</group>
</groups>
<panes>
<pane id="WineMonk_Demo_ProAppModule_Code17_Pane_PaneTest" caption="PaneTest" className="WineMonk.Demo.ProAppModule.Code17_Pane.PaneTestViewModel" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonGreen16.png" defaultTab="esri_mapping_homeTab" defaultTool="esri_mapping_navigateTool">
<content className="WineMonk.Demo.ProAppModule.Code17_Pane.PaneTestView" />
</pane>
</panes>
</insertModule>
</modules>
19 ArcGIS Pro 地图窗格模拟
19.1 添加控件
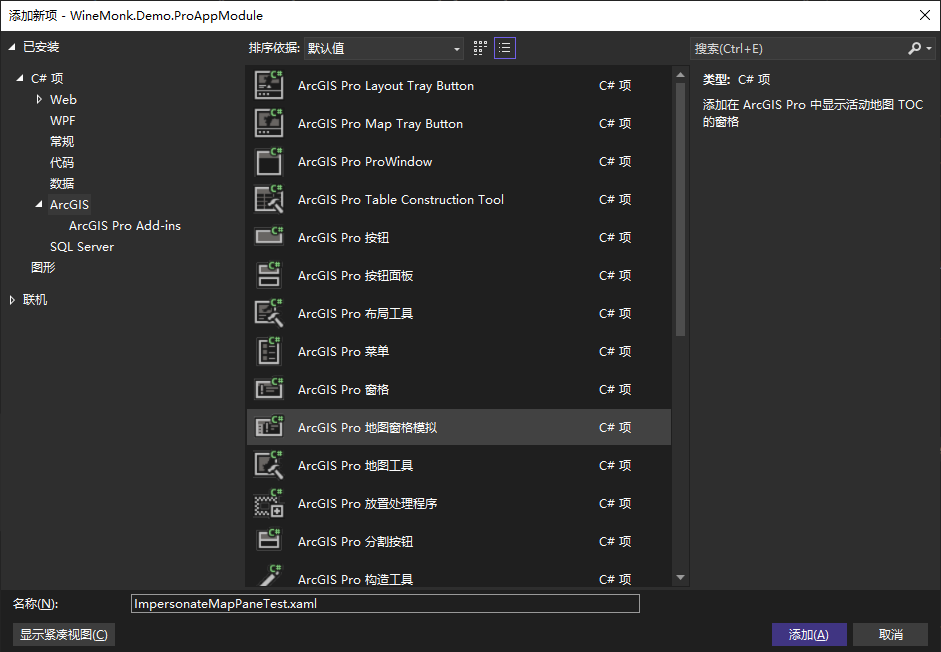
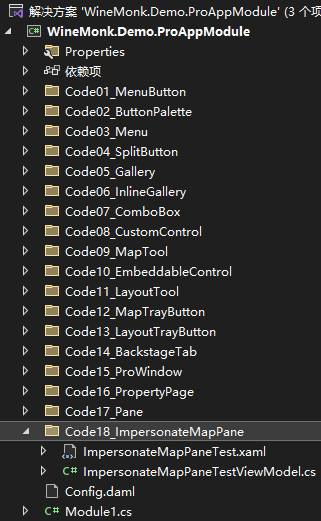
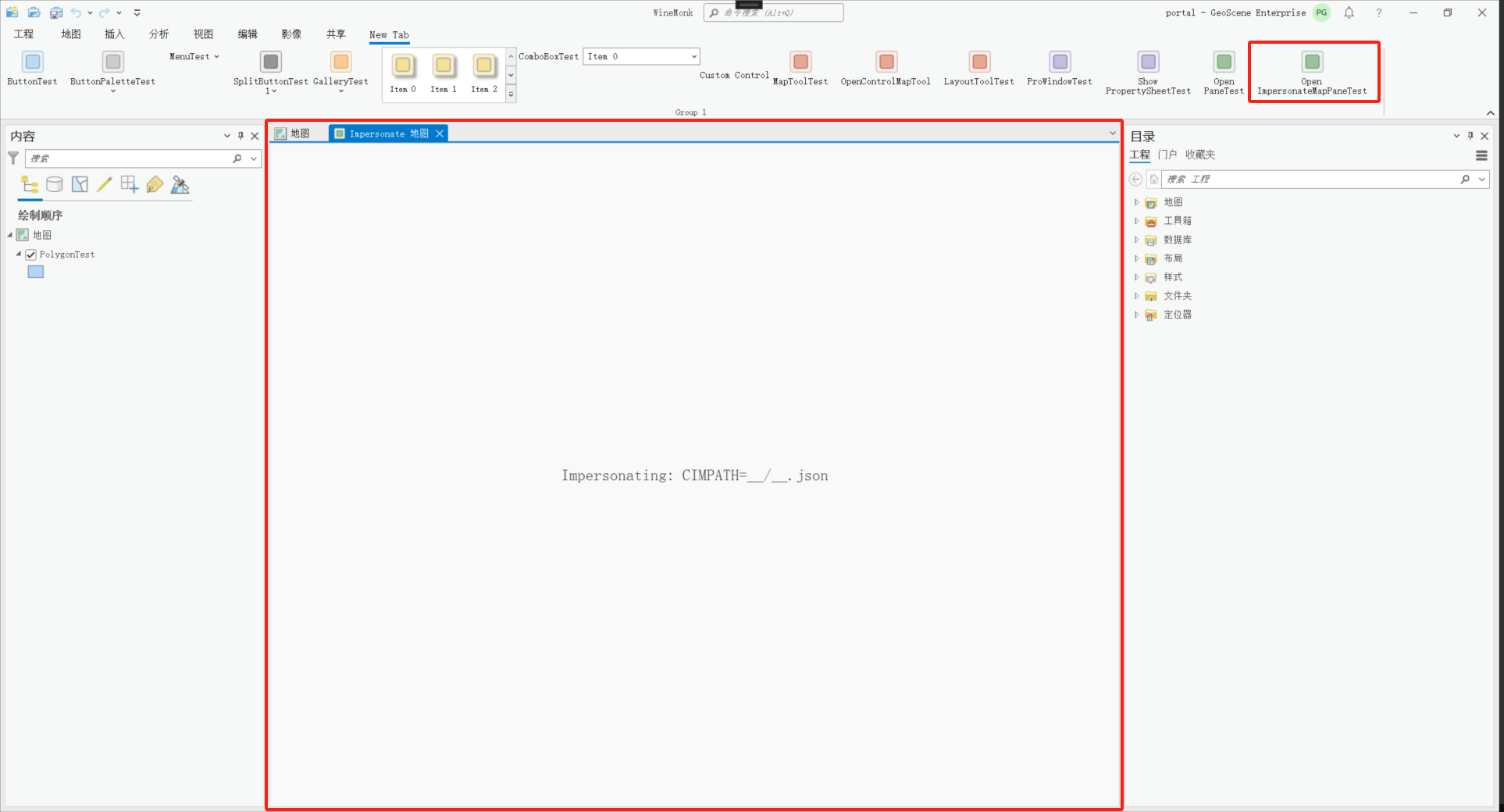
19.2 Code
ImpersonateMapPaneTest.xaml
xaml
<UserControl x:Class="WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane.ImpersonateMapPaneTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{Binding Path=ui.ImpersonateMapPaneTestViewModel}">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<StackPanel Orientation="Horizontal" HorizontalAlignment="Center" VerticalAlignment="Center">
<TextBlock Text="Impersonating:" Style="{DynamicResource Esri_TextBlockRegular}" FontSize="20" Margin="0,0,10,0"></TextBlock>
<TextBlock Text="{Binding MapURI, Mode=OneWay}" Style="{DynamicResource Esri_TextBlockRegular}" FontSize="20"></TextBlock>
</StackPanel>
</Grid>
</UserControl>
ImpersonateMapPaneTestViewModel.cs
csharp
using ArcGIS.Core.CIM;
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
using ArcGIS.Desktop.Mapping;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane
{
internal class ImpersonateMapPaneTestViewModel : TOCMapPaneProviderPane
{
private const string _viewPaneID = "WineMonk_Demo_ProAppModule_Code18_ImpersonateMapPane_ImpersonateMapPaneTest";
/// <summary>
/// Consume the passed in CIMView. Call the base constructor to wire up the CIMView.
/// </summary>
public ImpersonateMapPaneTestViewModel(CIMView view)
: base(view)
{
//Set to true to change docking behavior
_dockUnderMapView = false;
}
/// <summary>
/// Create a new instance of the pane.
/// </summary>
internal static ImpersonateMapPaneTestViewModel Create(MapView mapView)
{
var view = new CIMGenericView();
view.ViewType = _viewPaneID;
view.ViewProperties = new Dictionary<string, object>();
view.ViewProperties["MAPURI"] = mapView.Map.URI;
var newPane = FrameworkApplication.Panes.Create(_viewPaneID, new object[] { view }) as ImpersonateMapPaneTestViewModel;
newPane.Caption = $"Impersonate {mapView.Map.Name}";
return newPane;
}
#region Pane Overrides
/// <summary>
/// Must be overridden in child classes used to persist the state of the view to the CIM.
/// </summary>
/// <remarks>View state is called on each project save</remarks>
public override CIMView ViewState
{
get
{
_cimView.InstanceID = (int)InstanceID;
//Cache content in _cimView.ViewProperties
//((CIMGenericView)_cimView).ViewProperties["custom"] = "custom value";
//((CIMGenericView)_cimView).ViewProperties["custom2"] = "custom value2";
return _cimView;
}
}
/// <summary>
/// Called when the pane is initialized.
/// </summary>
protected async override Task InitializeAsync()
{
var uri = ((CIMGenericView)_cimView).ViewProperties["MAPURI"] as string;
await this.SetMapURI(uri);
await base.InitializeAsync();
}
/// <summary>
/// Called when the pane is uninitialized.
/// </summary>
protected async override Task UninitializeAsync()
{
await base.UninitializeAsync();
}
#endregion Pane Overrides
}
/// <summary>
/// Button implementation to create a new instance of the pane and activate it.
/// </summary>
internal class ImpersonateMapPaneTest_OpenButton : Button
{
protected override void OnClick()
{
ImpersonateMapPaneTestViewModel.Create(MapView.Active);
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code18_ImpersonateMapPane_ImpersonateMapPaneTest_OpenButton" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code18_ImpersonateMapPane_ImpersonateMapPaneTest_OpenButton" caption="Open ImpersonateMapPaneTest" className="WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane.ImpersonateMapPaneTest_OpenButton" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonGreen16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonGreen32.png" condition="esri_mapping_mapPane">
<tooltip heading="Open Pane">Open Pane<disabledText /></tooltip>
</button>
</controls>
<panes>
<pane id="WineMonk_Demo_ProAppModule_Code18_ImpersonateMapPane_ImpersonateMapPaneTest" caption="ImpersonateMapPaneTest" className="WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane.ImpersonateMapPaneTestViewModel" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonGreen16.png" defaultTab="esri_mapping_homeTab" defaultTool="esri_mapping_navigateTool">
<content className="WineMonk.Demo.ProAppModule.Code18_ImpersonateMapPane.ImpersonateMapPaneTestView" />
</pane>
</panes>
</insertModule>
</modules>
20 ArcGIS Pro 停靠窗格
20.1 添加控件
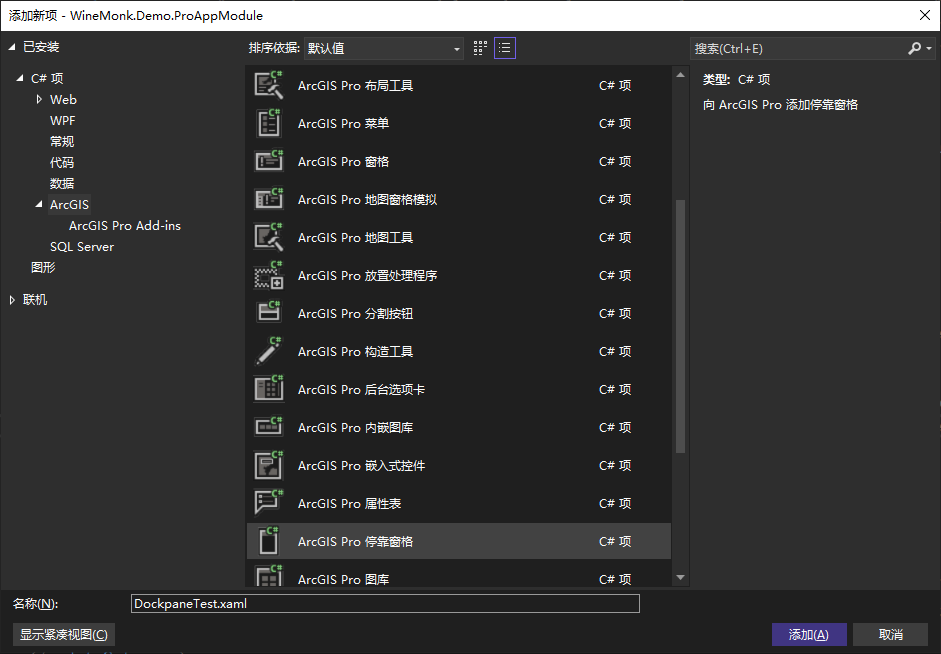
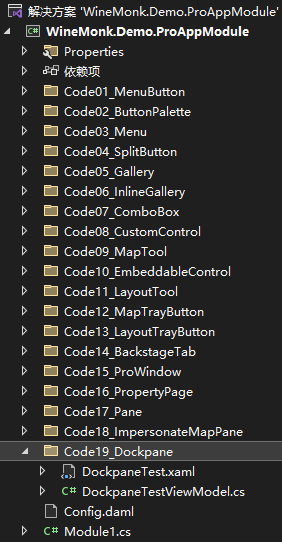
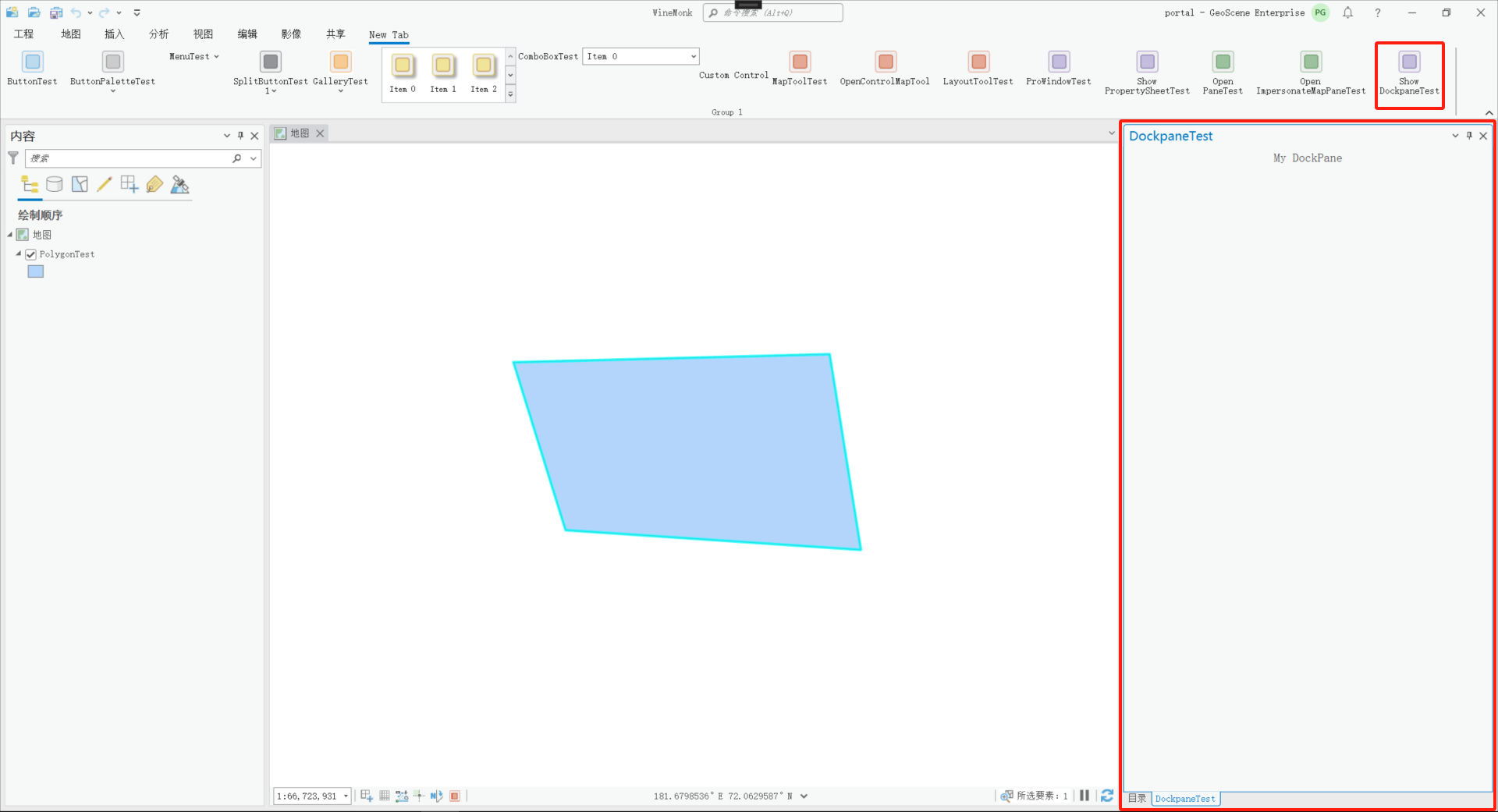
20.2 Code
DockpaneTest.xaml
xaml
<UserControl x:Class="WineMonk.Demo.ProAppModule.Code19_Dockpane.DockpaneTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code19_Dockpane"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{Binding Path=ui.DockpaneTestViewModel}">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<DockPanel Grid.Row="0" LastChildFill="true" KeyboardNavigation.TabNavigation="Local" Height="30">
<TextBlock Grid.Column="1" Text="{Binding Heading}" Style="{DynamicResource Esri_TextBlockDockPaneHeader}">
<TextBlock.ToolTip>
<WrapPanel Orientation="Vertical" MaxWidth="300">
<TextBlock Text="{Binding Heading}" TextWrapping="Wrap"/>
</WrapPanel>
</TextBlock.ToolTip>
</TextBlock>
</DockPanel>
</Grid>
</UserControl>
DockpaneTestViewModel.cs
csharp
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
namespace WineMonk.Demo.ProAppModule.Code19_Dockpane
{
internal class DockpaneTestViewModel : DockPane
{
private const string _dockPaneID = "WineMonk_Demo_ProAppModule_Code19_Dockpane_DockpaneTest";
protected DockpaneTestViewModel() { }
/// <summary>
/// Show the DockPane.
/// </summary>
internal static void Show()
{
DockPane pane = FrameworkApplication.DockPaneManager.Find(_dockPaneID);
if (pane == null)
return;
pane.Activate();
}
/// <summary>
/// Text shown near the top of the DockPane.
/// </summary>
private string _heading = "My DockPane";
public string Heading
{
get { return _heading; }
set
{
SetProperty(ref _heading, value, () => Heading);
}
}
}
/// <summary>
/// Button implementation to show the DockPane.
/// </summary>
internal class DockpaneTest_ShowButton : Button
{
protected override void OnClick()
{
DockpaneTestViewModel.Show();
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code19_Dockpane_DockpaneTest_ShowButton" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code19_Dockpane_DockpaneTest_ShowButton" caption="Show DockpaneTest" className="WineMonk.Demo.ProAppModule.Code19_Dockpane.DockpaneTest_ShowButton" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple32.png">
<tooltip heading="Show Dockpane">Show Dockpane<disabledText /></tooltip>
</button>
</controls>
<dockPanes>
<dockPane id="WineMonk_Demo_ProAppModule_Code19_Dockpane_DockpaneTest" caption="DockpaneTest" className="WineMonk.Demo.ProAppModule.Code19_Dockpane.DockpaneTestViewModel" dock="group" dockWith="esri_core_projectDockPane">
<content className="WineMonk.Demo.ProAppModule.Code19_Dockpane.DockpaneTestView" />
</dockPane>
</dockPanes>
</insertModule>
</modules>
21 ArcGIS Pro 带按钮的停靠窗格
21.1 添加控件
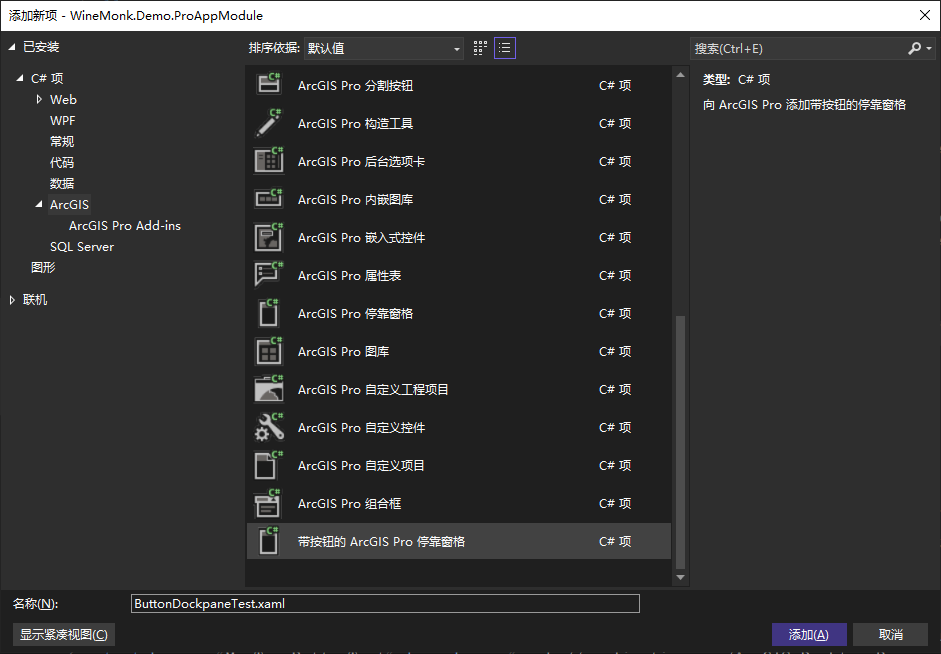
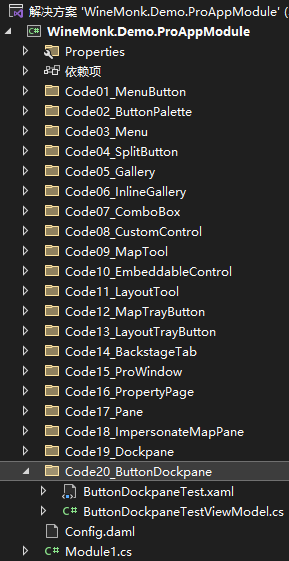
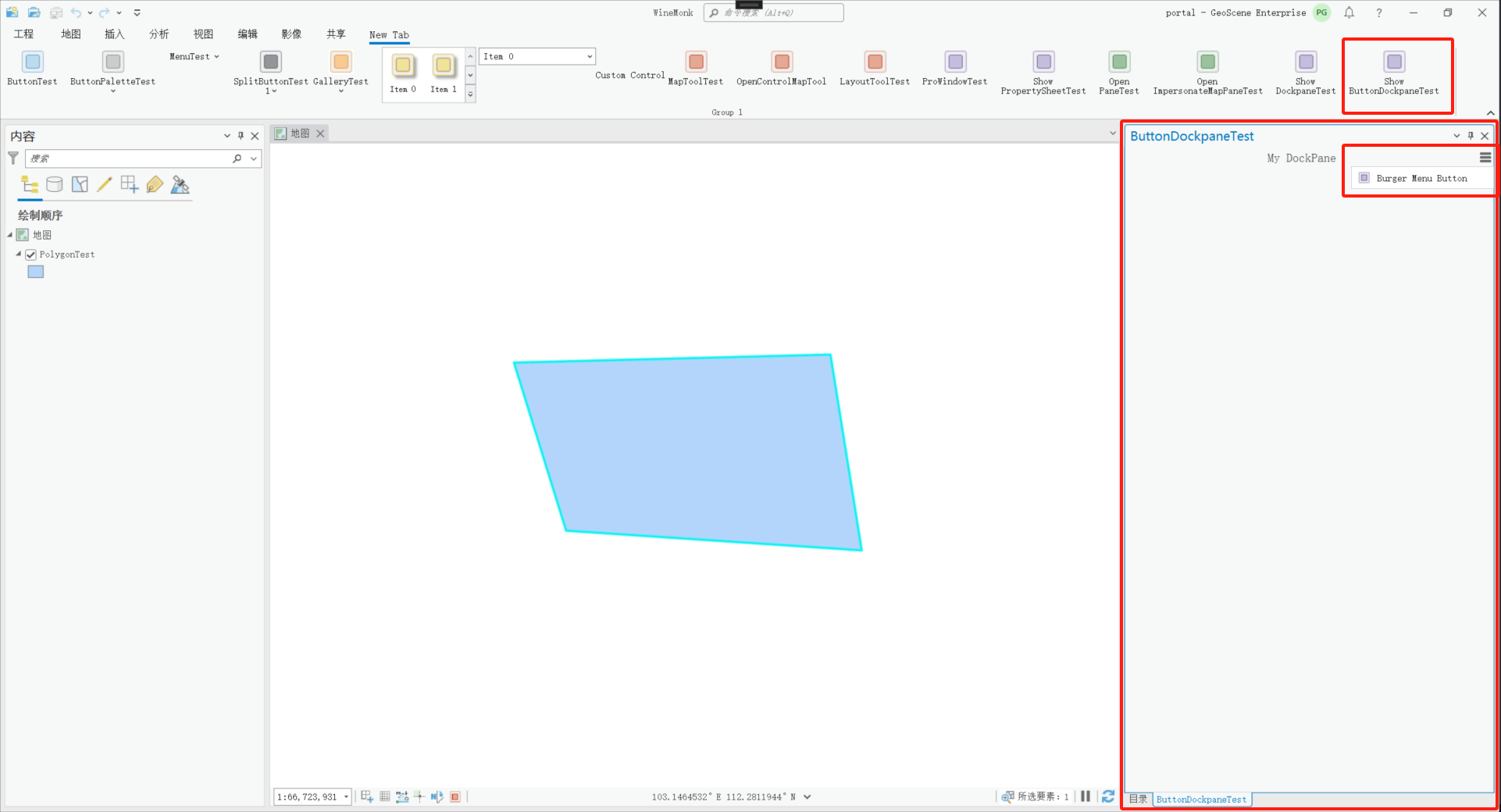
21.2 Code
ButtonDockpaneTest.xaml
xaml
<UserControl x:Class="WineMonk.Demo.ProAppModule.Code20_ButtonDockpane.ButtonDockpaneTestView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code20_ButtonDockpane"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
xmlns:controls="clr-namespace:ArcGIS.Desktop.Framework.Controls;assembly=ArcGIS.Desktop.Framework"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
d:DataContext="{Binding Path=ui.ButtonDockpaneTestViewModel}">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<DockPanel Grid.Row="0" LastChildFill="true" KeyboardNavigation.TabNavigation="Local" Height="30">
<controls:BurgerButton DockPanel.Dock="Right"
ToolTip="{Binding BurgerButtonTooltip}"
PopupMenu="{Binding BurgerButtonMenu}"/>
<TextBlock Grid.Column="1" Text="{Binding Heading}" Style="{DynamicResource Esri_TextBlockDockPaneHeader}">
<TextBlock.ToolTip>
<WrapPanel Orientation="Vertical" MaxWidth="300">
<TextBlock Text="{Binding Heading}" TextWrapping="Wrap"/>
</WrapPanel>
</TextBlock.ToolTip>
</TextBlock>
</DockPanel>
</Grid>
</UserControl>
ButtonDockpaneTestViewModel.cs
csharp
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
namespace WineMonk.Demo.ProAppModule.Code20_ButtonDockpane
{
internal class ButtonDockpaneTestViewModel : DockPane
{
private const string _dockPaneID = "WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest";
private const string _menuID = "WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest_Menu";
protected ButtonDockpaneTestViewModel() { }
/// <summary>
/// Show the DockPane.
/// </summary>
internal static void Show()
{
DockPane pane = FrameworkApplication.DockPaneManager.Find(_dockPaneID);
if (pane == null)
return;
pane.Activate();
}
/// <summary>
/// Text shown near the top of the DockPane.
/// </summary>
private string _heading = "My DockPane";
public string Heading
{
get { return _heading; }
set
{
SetProperty(ref _heading, value, () => Heading);
}
}
#region Burger Button
/// <summary>
/// Tooltip shown when hovering over the burger button.
/// </summary>
public string BurgerButtonTooltip
{
get { return "Options"; }
}
/// <summary>
/// Menu shown when burger button is clicked.
/// </summary>
public System.Windows.Controls.ContextMenu BurgerButtonMenu
{
get { return FrameworkApplication.CreateContextMenu(_menuID); }
}
#endregion
}
/// <summary>
/// Button implementation to show the DockPane.
/// </summary>
internal class ButtonDockpaneTest_ShowButton : Button
{
protected override void OnClick()
{
ButtonDockpaneTestViewModel.Show();
}
}
/// <summary>
/// Button implementation for the button on the menu of the burger button.
/// </summary>
internal class ButtonDockpaneTest_MenuButton : Button
{
protected override void OnClick()
{
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group1" caption="Group 1" appearsOnAddInTab="false">
<!-- host controls within groups -->
<!-- 组内主机控件 -->
<button refID="WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest_ShowButton" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest_MenuButton" caption="Burger Menu Button" className="WineMonk.Demo.ProAppModule.Code20_ButtonDockpane.ButtonDockpaneTest_MenuButton" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple32.png">
<tooltip heading="Burger Menu Button">ToolTip<disabledText /></tooltip>
</button>
</controls>
<menus>
<menu id="WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest_Menu" caption="Options" contextMenu="true">
<button refID="WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest_MenuButton" />
</menu>
</menus>
<dockPanes>
<dockPane id="WineMonk_Demo_ProAppModule_Code20_ButtonDockpane_ButtonDockpaneTest" caption="ButtonDockpaneTest" className="WineMonk.Demo.ProAppModule.Code20_ButtonDockpane.ButtonDockpaneTestViewModel" dock="group" dockWith="esri_core_projectDockPane">
<content className="WineMonk.Demo.ProAppModule.Code20_ButtonDockpane.ButtonDockpaneTestView" />
</dockPane>
</dockPanes>
</insertModule>
</modules>