迭代器模式
迭代器模式属于行为型模式,用来遍历容器的一种模式。
图解
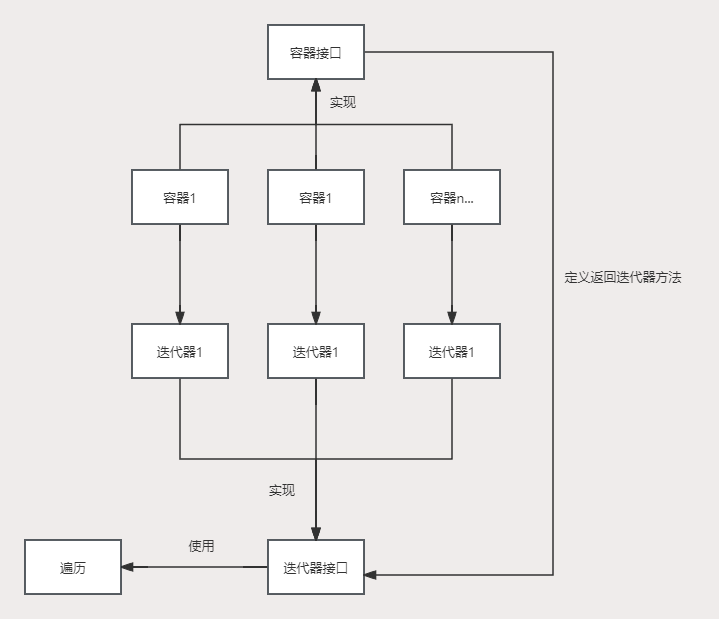
角色
- 迭代器接口:定义hasNext方法和next方法
- 具体迭代器:实现hasNext方法和next方法
- 容器接口:定义返回一个迭代器的接口
- 具体容器:实现返回迭代器接口
案例
该案例以简单实现ArrayList和LinkedList来演示迭代器模式。整体思想是,不管是什么容器,必须实现获取迭代器的方法,和定义一个迭代器来遍历对应的容器。
java
public interface Iterable_<E> {
Iterator_<E> iterator();
}
public interface Collection_<E> extends Iterable_<E>{
void add(E e);
int size();
}
public interface Iterator_<E> {
boolean hasNest();
E next();
}
ArrayList实现类
java
public class ArrayList_<E> implements Collection_<E>{
private static final int initSize = 10;
private Object [] objects = new Object[initSize];
private int index = 0;
@Override
public void add(E e) {
if(index >= objects.length){
Object[] newObjects = new Object[(int) (objects.length * 1.5)];
System.arraycopy(objects,0,newObjects,0,newObjects.length);
this.objects = newObjects;
}else {
objects[index] = e;
}
index++;
}
@Override
public int size() {
return index;
}
@Override
public Iterator_<E> iterator() {
return new ArrayIterator();
}
/** arrayList迭代器*/
private class ArrayIterator<E> implements Iterator_<E>{
int cursor;
@Override
public boolean hasNest() {
return cursor != index;
}
@Override
public E next() {
E e= (E) objects[cursor];
cursor ++;
return e;
}
}
}
linkedList实现类
java
public class LinkedList_<E> implements Collection_<E>{
private int size = 0;
private Node<E> lastNode = null;
private Node<E> handNode = null;
@Override
public void add(E e) {
Node<E> objectNode =new Node<>(e,null);
if(lastNode == null){
lastNode = objectNode;
handNode = objectNode;
}else {
lastNode.next = objectNode;
lastNode = objectNode;
}
this.size++;
}
@Override
public int size() {
return size;
}
@Override
public Iterator_<E> iterator() {
return new LinkedIterator();
}
private class LinkedIterator implements Iterator_<E>{
int cursor = 0;
Node<E> tempNode = null;
@Override
public boolean hasNest() {
return cursor != size;
}
@Override
public E next() {
cursor++;
if(cursor ==1){
tempNode = handNode;
return handNode.getValue();
}else {
return tempNode.next.getValue();
}
}
}
private class Node<E>{
private E value;
private Node<E> next;
public Node(E value, Node<E> next) {
this.value = value;
this.next = next;
}
public void setNext(Node<E> next) {
this.next = next;
}
public E getValue() {
return value;
}
}
}
Test类
java
package com.shaoby.designpatterns.Iterator;
public class Test {
public static void main(String[] args) {
Collection_<String> objectArrayList_ = new LinkedList_<>();
objectArrayList_.add("111");
objectArrayList_.add("222");
objectArrayList_.add("333");
Iterator_<String> iterator = objectArrayList_.iterator();
while (iterator.hasNest()){
System.out.println(iterator.next());
}
}
}