一、组件之间的传值
-
组件可以由内部的Data提供数据,也可以由父组件通过prop的方式传值。
-
兄弟组件之间可以通过Vuex等统一数据源提供数据共享
第一种
Movie.vue
<template>
<div>
<h1>我才不要和你做朋友</h1>
</div>
</template>
App.vue
<template>
<div id="app">
<Movie></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
export default {
name: 'App',
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
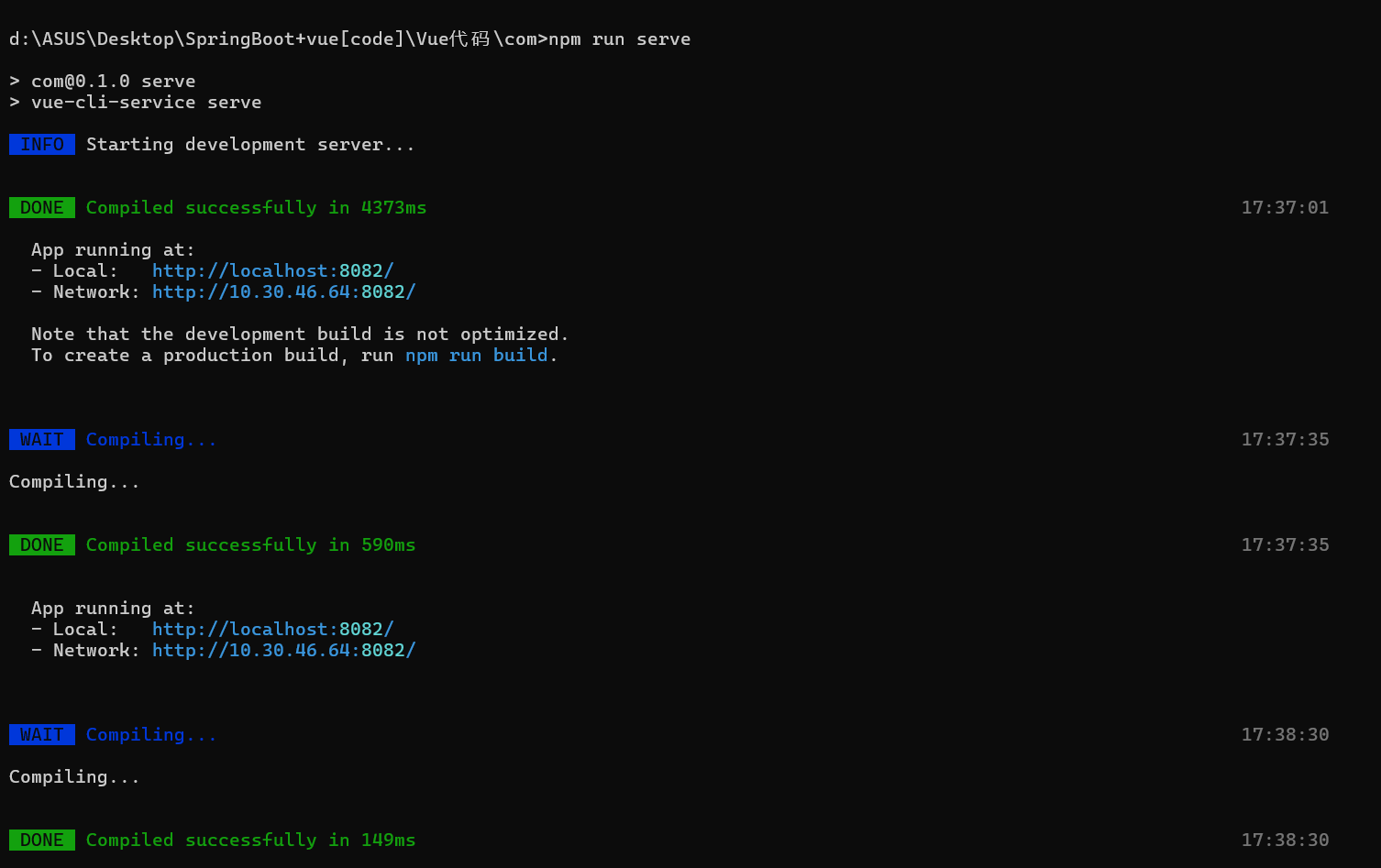
第二种
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
</div>
</template>
<script>
export default {
name: "Movie",
data:function(){
return{
title:"我才不要跟你做朋友"
}
}
}
</script>
App.vue
<template>
<div id="app">
<Movie></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
Movie.name //
export default {
name: 'App',
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
第三种
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
</div>
</template>
<script>
export default {
name: "Movie",
props:["title"],
data:function(){
return{
}
}
}
</script>
App.vue
<template>
<div id="app">
<Movie title="我才不要跟你做朋友"></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
Movie.name
export default {
name: 'App',
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
显示效果(前三种一样):
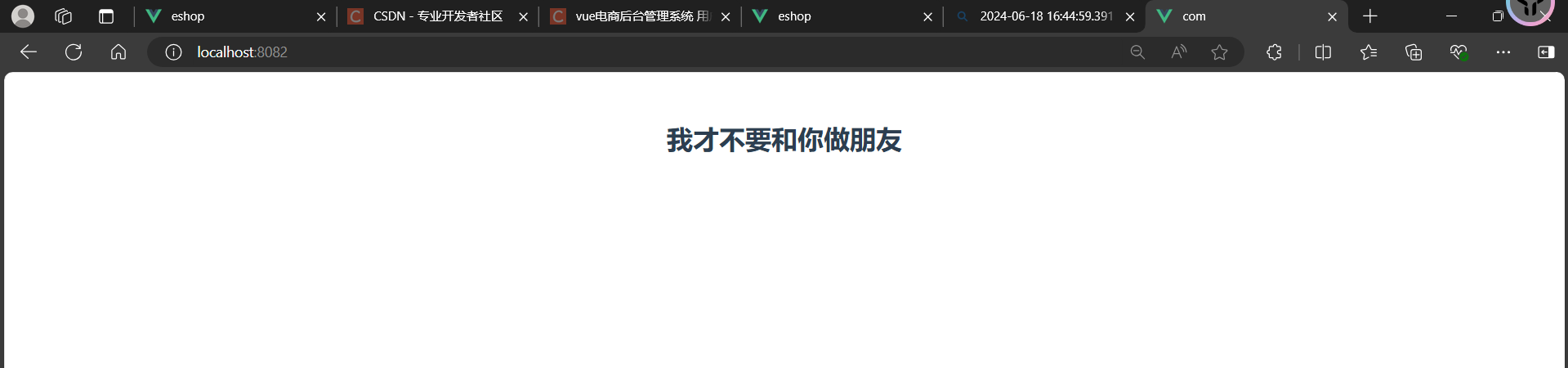
第四种:
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
<span>{{rating}}</span>
</div>
</template>
<script>
export default {
name: "Movie",
props:["title","rating"],
data:function(){
return{
}
}
}
</script>
App.vue
<template>
<div id="app">
<Movie title="我才不要跟你做朋友" rating="6.8"></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
Movie.name
export default {
name: 'App',
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
显示效果:

第五种(关于组件的传值):
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
<span>{{rating}}</span>
</div>
</template>
<script>
export default {
name: "Movie",
props:["title","rating"],
data:function(){
return{
}
}
}
</script>
App.vue
<template>
<div id="app">
<Movie v-for="movie in movies" :key="movie.id" :title="movie.title" :rating="movie.rating"></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
Movie.name
export default {
name: 'App',
data:function(){
return{
movies:[
{id:1,title:"我才不要跟你做朋友1",rating:8.8},
{id:2,title:"我才不要跟你做朋友2",rating:8.9},
{id:3,title:"我才不要跟你做朋友3",rating:8.7},
]
}
},
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
显示效果:
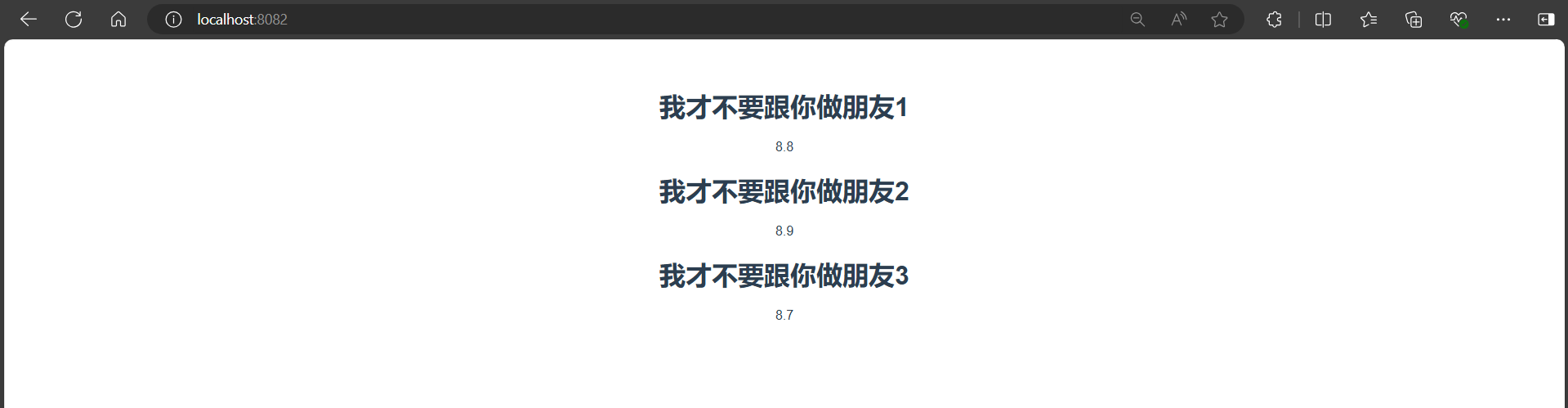
第六种(加按钮,弹窗):
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
<span>{{rating}}</span>
<button @click="fun">点击收藏</button>
</div>
</template>
<script>
export default {
name: "Movie",
props:["title","rating"],
data:function(){
return{
}
},
methods:{
fun(){
alert("收藏成功")
}
}
}
</script>
App.vue
<template>
<div id="app">
<Movie v-for="movie in movies" :key="movie.id" :title="movie.title" :rating="movie.rating"></Movie>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
Movie.name
export default {
name: 'App',
data:function(){
return{
movies:[
{id:1,title:"我才不要跟你做朋友1",rating:8.8},
{id:2,title:"我才不要跟你做朋友2",rating:8.9},
{id:3,title:"我才不要跟你做朋友3",rating:8.7},
]
}
},
components: {
Movie
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
显示效果:
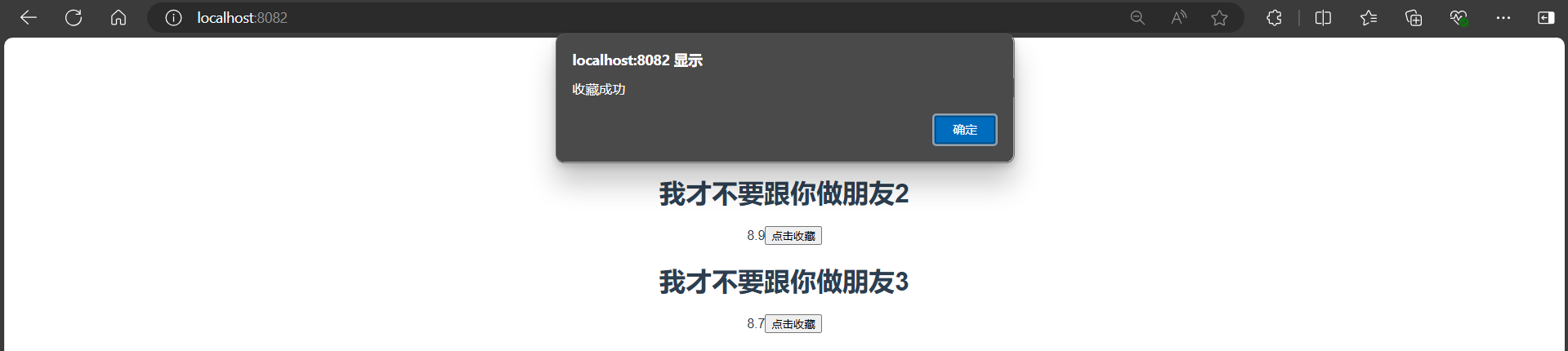
第七种(兄弟组件)
Movie.vue
<template>
<div>
<h1>{{title}}</h1>
<span>{{rating}}</span>
<button @click="fun">点击收藏</button>
</div>
</template>
<script>
export default {
name: "Movie",
props:["title","rating"],
data:function(){
return{
}
},
methods:{
fun(){
alert("收藏成功")
}
}
}
</script>
APP.vue
<template>
<div id="app">
<Movie v-for="movie in movies" :key="movie.id" :title="movie.title" :rating="movie.rating"></Movie>
<Hello></Hello>
</div>
</template>
<script>
import Movie from './components/Movie.vue'
import Hello from './components/Hello.vue'
Movie.name
export default {
name: 'App',
data:function(){
return{
movies:[
{id:1,title:"我才不要跟你做朋友1",rating:8.8},
{id:2,title:"我才不要跟你做朋友2",rating:8.9},
{id:3,title:"我才不要跟你做朋友3",rating:8.7},
]
}
},
components: {
Movie,
Hello
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Hello.vue
<template>
<h3>hello</h3>
</template>
显示效果:
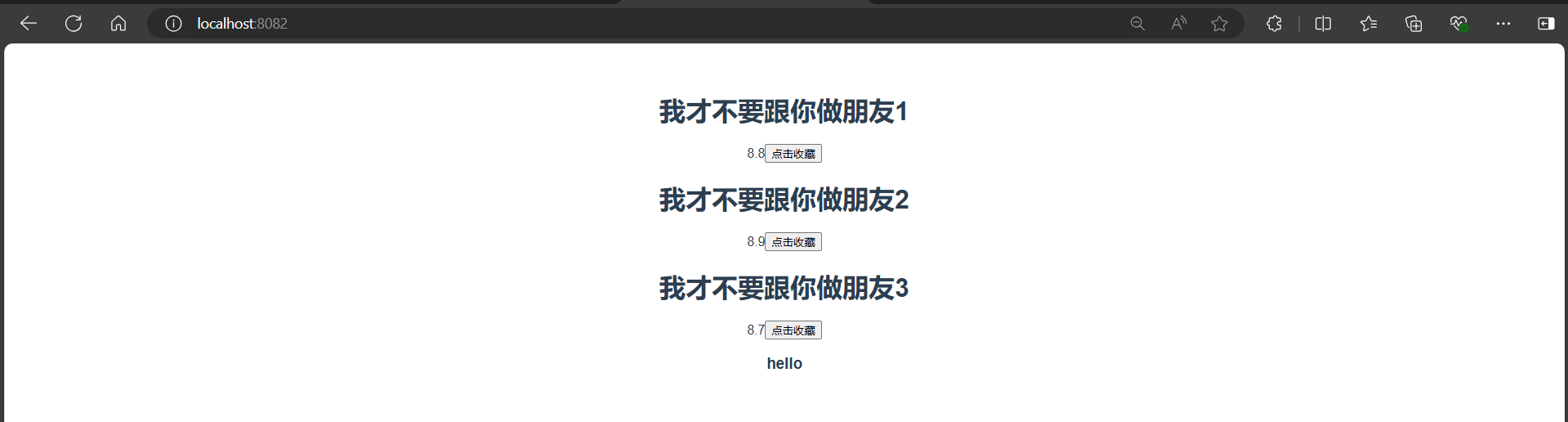
二、element-ui介绍
Element是国内饿了么公司提供的一套开源前端框架,简洁优雅,提供了Vue、React、Angular等多个版本。
-
文档地址:Element - The world's most popular Vue UI framework[提供很多现成的组件]
-
安装:npm i element-ui
下载好后,所以安装好的东西都会在node_mdules目录下
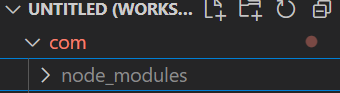
- 引入 Element(在main.js):
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
import App from './App.vue';
Vue.use(ElementUI);
new Vue({
el: '#app',
render: h => h(App)
});
三、组件的使用
main.js
import Vue from 'vue'
import App from './App.vue'
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.config.productionTip = false
Vue.use(ElementUI);
new Vue({
render: h => h(App),
}).$mount('#app')
Hello.vue
<template>
<div>
<el-table
:data="tableData"
style="width: 100%"
:row-class-name="tableRowClassName">
<el-table-column
prop="id"
label="编号"
width="180">
</el-table-column>
<el-table-column
prop="username"
label="姓名"
width="180">
</el-table-column>
<el-table-column
prop="birthday"
label="生日">
</el-table-column>
</el-table>
<el-date-picker
v-model="value1"
type="date"
placeholder="选择日期">
</el-date-picker>
<i class="fa fa-camera-retro"></i>
</div>
</template>
<script>
export default {
methods: {
tableRowClassName({row, rowIndex}) {
if (rowIndex === 1) {
return 'warning-row';
} else if (rowIndex === 3) {
return 'success-row';
}
return '';
}
},
created:function(){
this.$http.get("/user/findAll").then((response)=>{
this.tableData = response.data
})
},
data() {
return {
tableData: []
}
}
}
</script>
<style>
.el-table .warning-row {
background: oldlace;
}
.el-table .success-row {
background: #f0f9eb;
}
</style>
显示结果:
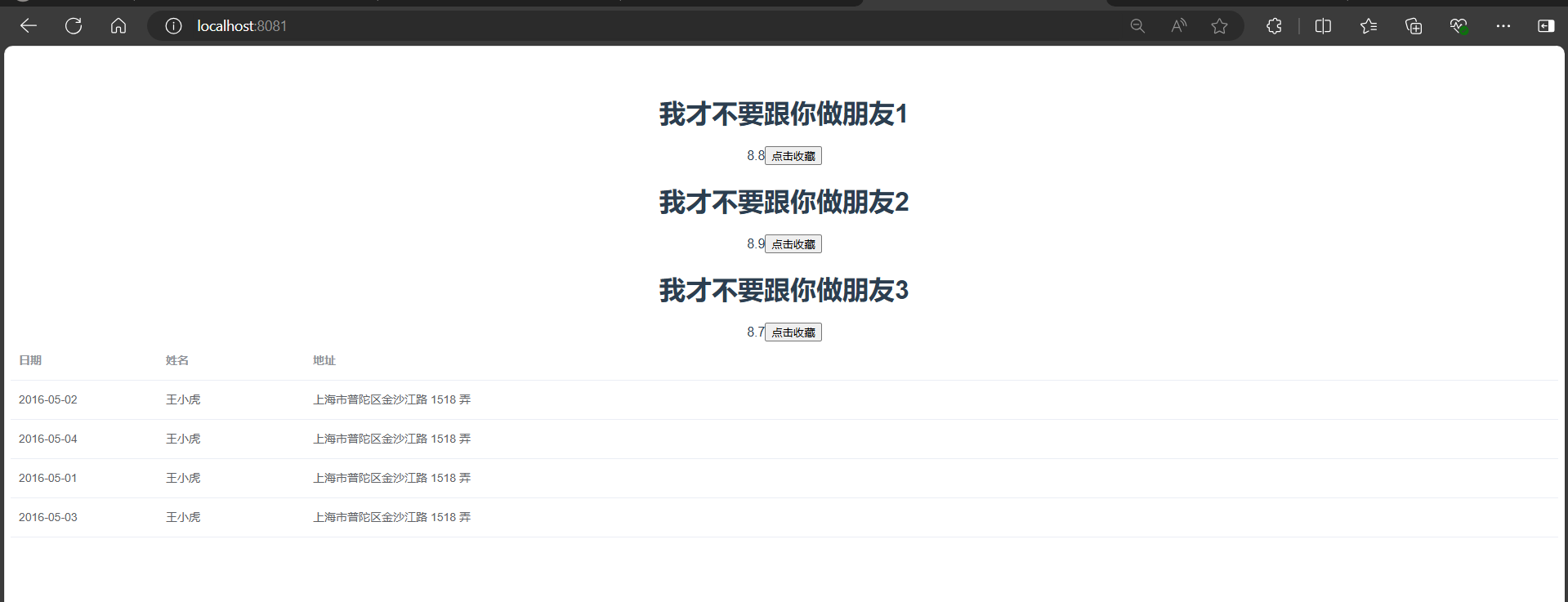
修改Hello.vue(隔行颜色不同)
<template>
<el-table
:data="tableData"
style="width: 100%"
:row-class-name="tableRowClassName">
<el-table-column
prop="date"
label="日期"
width="180">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
width="180">
</el-table-column>
<el-table-column
prop="address"
label="地址">
</el-table-column>
</el-table>
</template>
<script>
export default {
methods: {
tableRowClassName({row, rowIndex}) {
if (rowIndex === 1) {
return 'warning-row';
} else if (rowIndex === 3) {
return 'success-row';
}
return '';
}
},
data() {
return {
tableData: [{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄',
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄',
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}]
}
}
}
</script>
<style>
.el-table .warning-row {
background: oldlace;
}
.el-table .success-row {
background: #f0f9eb;
}
</style>
测试结果:
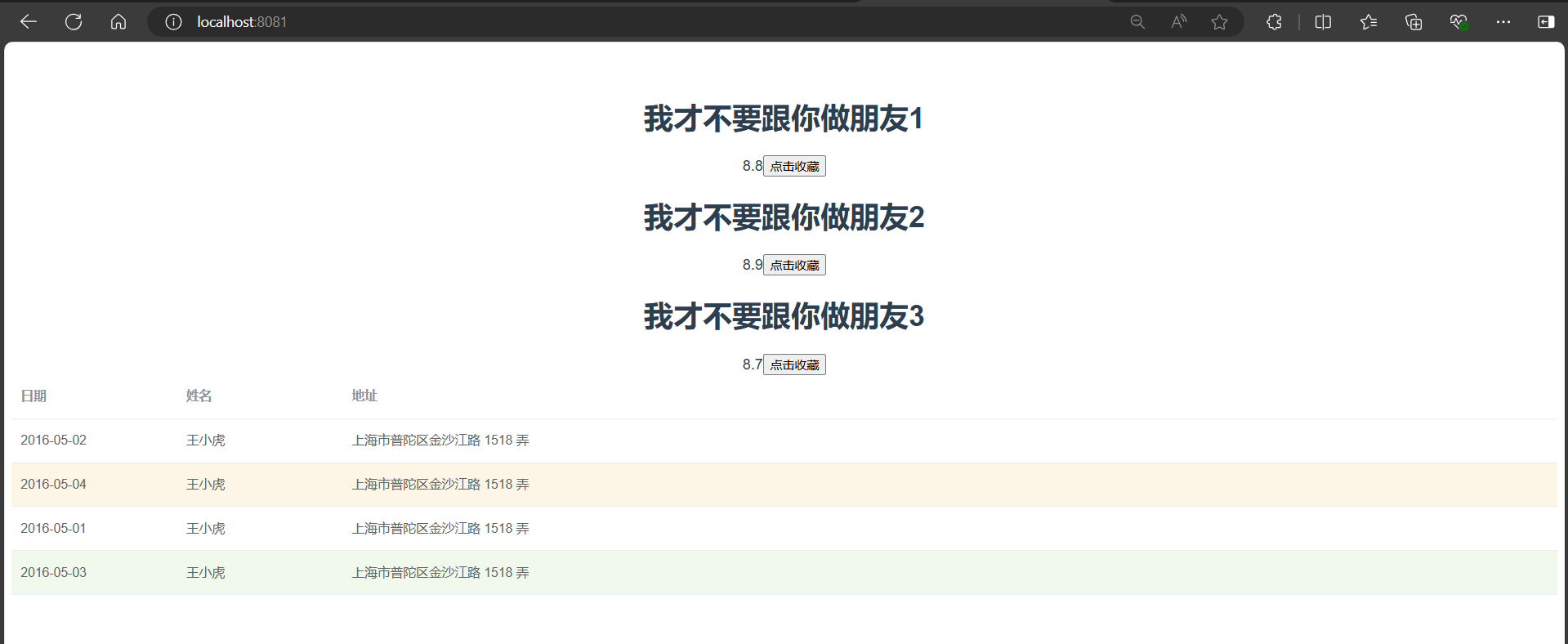
修改Hello.vue(添加日期选择器)
<template>
<div><el-table
:data="tableData"
style="width: 100%"
:row-class-name="tableRowClassName">
<el-table-column
prop="date"
label="日期"
width="180">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
width="180">
</el-table-column>
<el-table-column
prop="address"
label="地址">
</el-table-column>
</el-table>
<el-date-picker
v-model="value1"
type="date"
placeholder="选择日期">
</el-date-picker>
</div>
</template>
显示结果
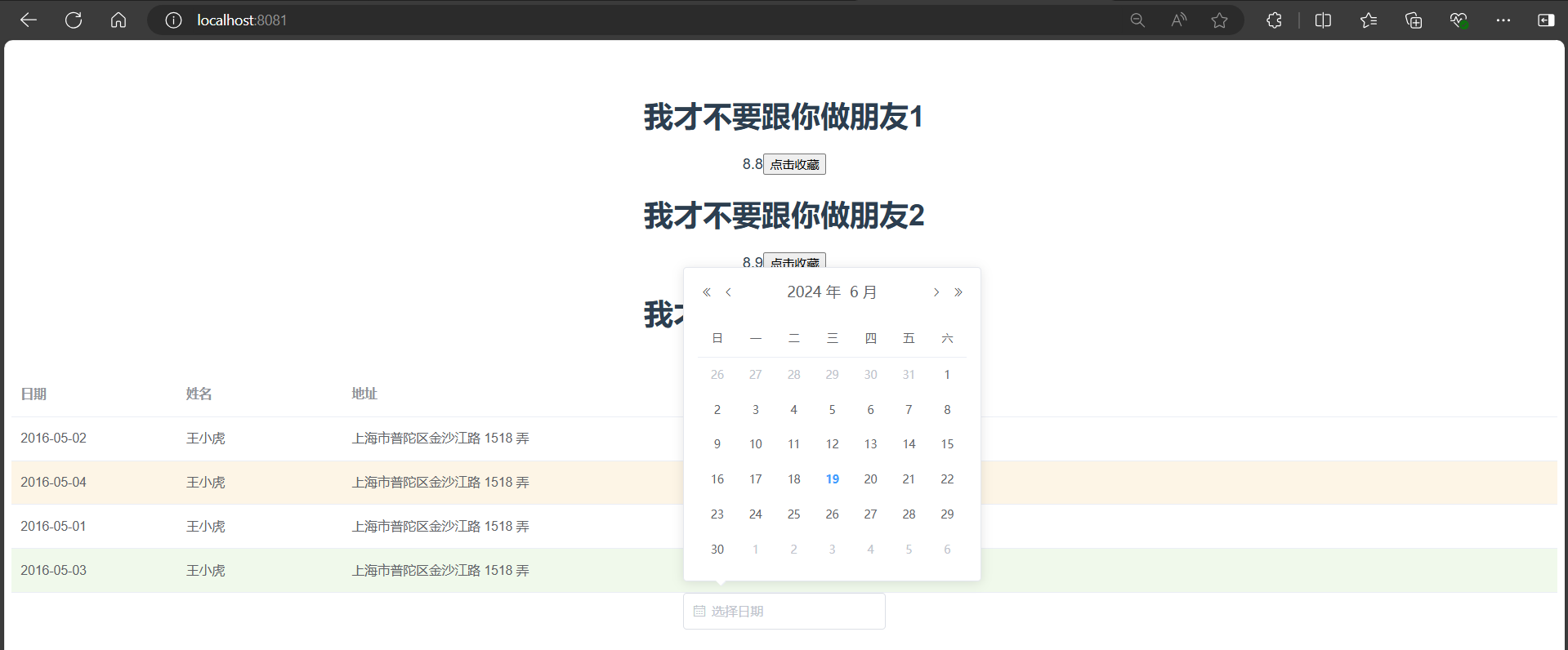
四、图标的使用
第三方图标库
由于Element UI提供的字体图符较少,一般会采用其他图表库,如著名的FontAwesome
Font Awesome提供了675个可缩放的矢量图标,可以使用CSS所提供的所有特性对它们进行更改,包括大小、颜色、阴影或者其他任何支持的效果。
安装:npm install font-awesome
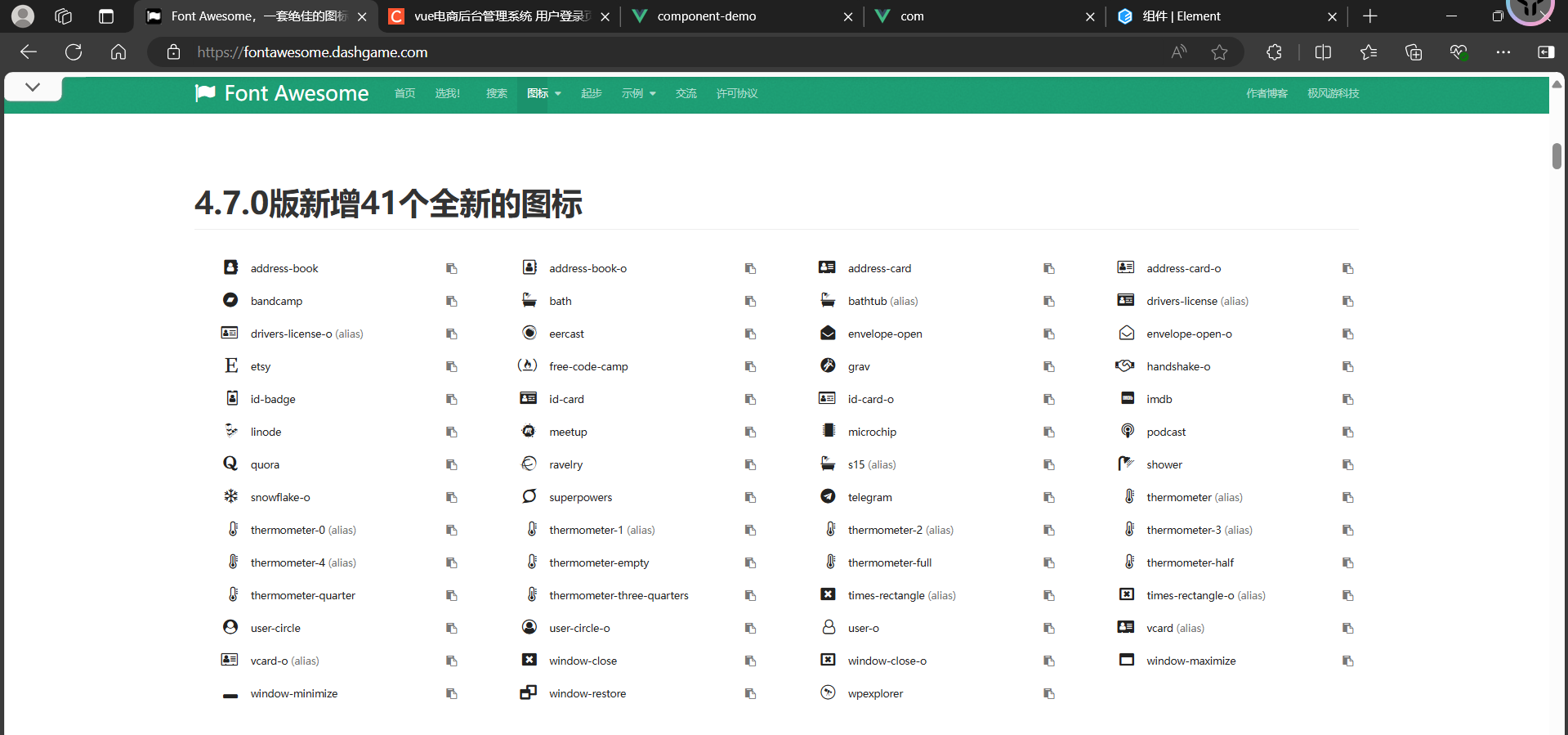
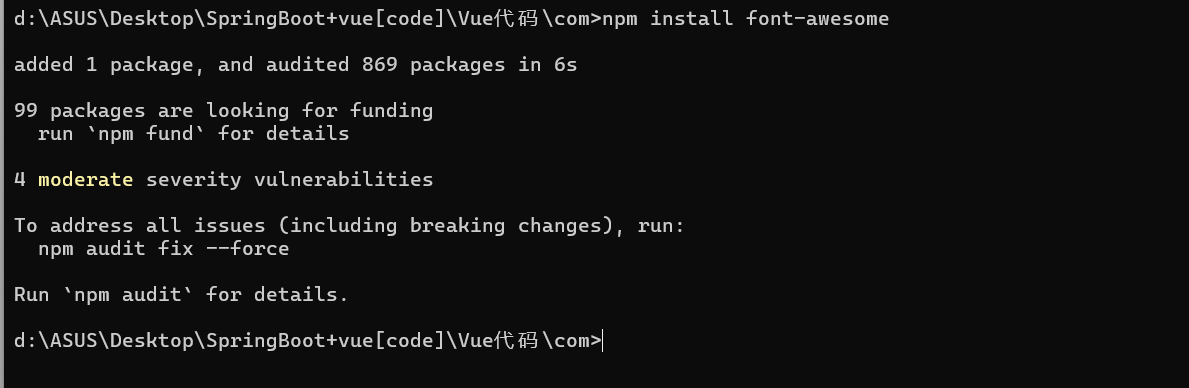
使用:import 'font-awesome/css/font-awesome.min.css
测试结果(添加图标):
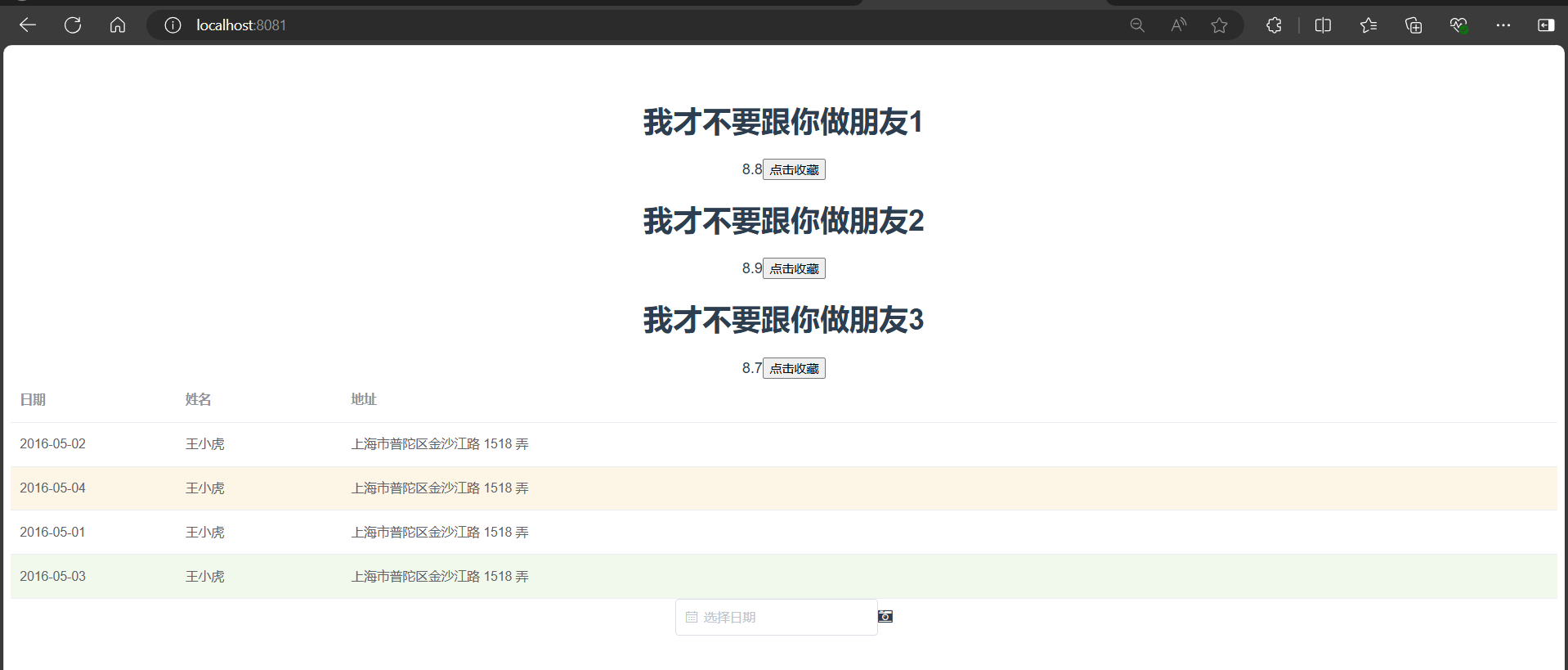