一、实验目的
- 学习面向对象程序设计的方法。
- 学习建立对象数组的方法。
- 学习在数组中存储和处理对象。
二、实验内容 、 过程及结果
**10.7 (Game: ATM machine) Use the Account class created in Programming Exer
cise 9.7 to simulate an ATM machine. Create ten accounts in an array with id 0,1,.1.9, and initial balance $100. The system prompts the user to enter an id. If the id is entered incorrectly, ask the user to enter a correct id. Once an id is accepted, the main menu is displayed as shown in the sample run. You can enter a choice 1 for viewing the current balance, 2 for withdrawing money, 3 for depositing money, and 4 for exiting the main menu. Once you exit, the system will prompt for an id again. Thus, once the system starts, it will not stop.
**10.7 (游戏:ATM机) 使用编程练习9.7中创建的Account类来模拟ATM机。在数组中创建十个账户,ID为0、1、2、3、9,初始余额为100美元。系统提示用户输入ID。如果ID输入错误,请用户输入正确的ID。一旦接受ID,就会显示与样本运行中所示相同的主菜单。您可以输入1查看当前余额,输入2提取现金,输入3存入现金,输入4退出主菜单。一旦退出,系统将再次提示输入ID。因此,一旦系统启动,就不会停止。
运行代码如下 :
java
package chapter10;
import java.util.Date;
import java.util.Scanner;
class Code_07 {
public static void main(String[] args) {
Account[] accounts = new Account[10];
for (int i = 0; i < 10; i++)
accounts[i] = new Account(1, 100);
System.out.print("Enter an id:");
Scanner input = new Scanner(System.in);
int id = input.nextInt();
while (id < 0 || id > 9) {
System.out.print("The if is nonExistent,please input again:");
id = input.nextInt();
}
mainMenu();
int choice = input.nextInt();
boolean judge = choice == 1 || choice == 2 || choice == 3;
while (judge) {
switch (choice) {
case 1:
System.out.println("The balance is "+accounts[id].getBalance());
break;
case 2:
System.out.print("Enter an amount to withdraw: ");
double withdraw = input.nextDouble();
accounts[id].withdraw(withdraw);
break;
case 3:
System.out.print("Enter an amount to deposit:");
double deposit=input.nextDouble();
accounts[id].deposit(deposit);
break;
}
mainMenu();
choice=input.nextInt();
judge = choice == 1 || choice == 2 || choice == 3;
}
Code_07.main(args);
}
public static void mainMenu(){
System.out.println("Main menu");
System.out.println("1: check balance ");
System.out.println("2: withdraw ");
System.out.println("3: deposit ");
System.out.println("4: exit ");
System.out.print("Enter a choice: ");
}
}
class Account {
public Account() {
dateCreated = new Date();
}
public Account(int id,double balance){
this.id = id;
this.balance = balance;
dateCreated = new Date();
}
private int id;
private double balance;
private double annualInterestRate;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
public double getAnnualInterestRate() {
return annualInterestRate;
}
public void setAnnualInterestRate(double annualInterestRate) {
this.annualInterestRate = annualInterestRate;
}
private Date dateCreated=new Date();
public void Account() {
}
public double getMonthlyInterest() {
return balance*(annualInterestRate/100/12);
}
public void withdraw(double reduce) {
balance-=reduce;
}
public void deposit(double increase) {
balance+=increase;
}
public Date getDateCreated() {
return dateCreated;
}
}
运行结果
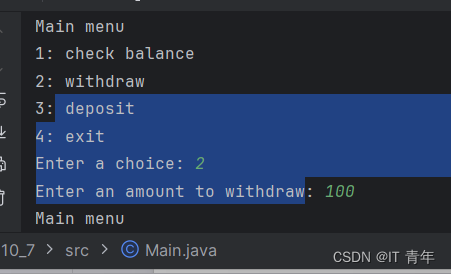
10.14(The MyDate class) Design a class named MyDate. The class contains:
The data fields year, month, and day that represent a date. month is
O-based, i.e., 0 is for January.
1Anoarg constructor that creates a MyDate object for the current date.■ A constructor that constructs a MyDate object with a specified elapsed time since midnight, January 1, 1970, in millise--conds.
A constructor that constructs a MyDate object with the specified year,
month, and day.
■ Three getter methods for the data fields year, month, and day, respectively.■ A method named setDate(long elapsedTime) that sets a new date for the object using the elapsed time.
Draw the UML diagram for the class and then implement the class. Write a test program that creates two MyDate objects (using new MyDate() and new MyDate(34355555133101L)) and displays their------year,monthandday.(Hint: The first two constructors will extract the year, month, and day from the elapsed time. For example, if the elapsed time is 561555550000 miliseconds, the year is 1987, the month is 9, and the day is 18. You may use the GregorianCalendar class discussed in Programming.Exercise 9.5 to simplify coding.)
**10.14(MyDate类) 设计一个名为MyDate的类。该类包含以下内容: 表示日期的字段year、month和day。month是基于O的,即0表示一月。 一个无参构造函数,用于创建表示当前日期的MyDate对象。 一个构造函数,用于使用自午夜起的毫秒数创建一个指定的过去时间的MyDate对象。 一个构造函数,用于使用指定的年、月和日创建一个MyDate对象。 分别用于获取数据字段year、month和day的三个getter方法。 一个名为setDate(long elapsedTime)的方法,用于使用elapsedTime为对象设置新的日期。 绘制该类的UML图,然后实现该类。编写一个测试程序,创建两个MyDate对象(使用new MyDate()和new MyDate(34355555133101L)),并显示它们的年、月和日。(提示:前两个构造函数将从elapsedTime中提取年、月和日。例如,如果elapsedTime是561555550000毫秒,年份是1987,月份是9,日期是18。您可以使用Programming.Exercise 9.5中讨论的GregorianCalendar类简化编码。)
运行代码如下 :
java
package pack2;
import java.util.GregorianCalendar;
class MyDate {
private int year, month, day; //年、月、日
/**当前日期的无参构造方法*/
public MyDate() {
setDate(System.currentTimeMillis());
}
/**以流逝的毫秒数为时间的构造方法*/
public MyDate(long elapsedTime) {
setDate(elapsedTime);
}
/**带指定年、月、日的构造方法*/
public MyDate(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
/**使用流逝的时间设置新日期*/
public void setDate(long elapsedTime) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTimeInMillis(elapsedTime);
year = calendar.get(GregorianCalendar.YEAR);
month = calendar.get(GregorianCalendar.MONTH);
day = calendar.get(GregorianCalendar.DAY_OF_MONTH);
}
@Override /**返回年、
public int getMonth() {
return month;
}
public int getDay() {
return day;
}
//------------------------------------------------------------------------------------------------------------------------------------------------------------
public static void main(String[] args) {
MyDate date1 = new MyDate();
MyDate date2 = new MyDate(34355555133101L);
System.out.println("date1: \n" + date1);
System.out.println("\ndate2: \n" + date2);
date2.setDate(561555550000L);
System.out.println("\ndate2: \n" + date2);
}
}月、日的字符串*/
public String toString() {
return "Year: " + year + "\nMonth: " + month + "\nDay: " + day;
}
public int getYear() {
return year;
}
运行结果
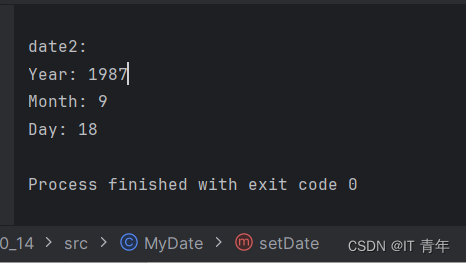
三、实验 结论
通过本次实验实践了ATM机知识和操作,趣味十足,得到了编程与数学,逻辑思维息息相关的感悟,要想写优秀的代码,头脑必须清醒,思维必须井井有条,敲写代码时必须心无旁骛。
结语
优秀是一种习惯
毅力里藏着帝王志气
!!!
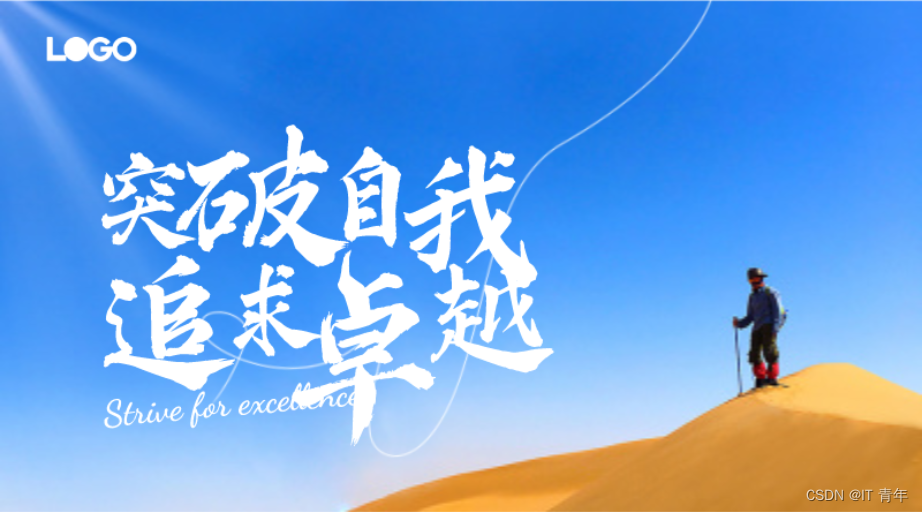