22 ArcGIS Pro 放置处理程序
22.1 添加控件
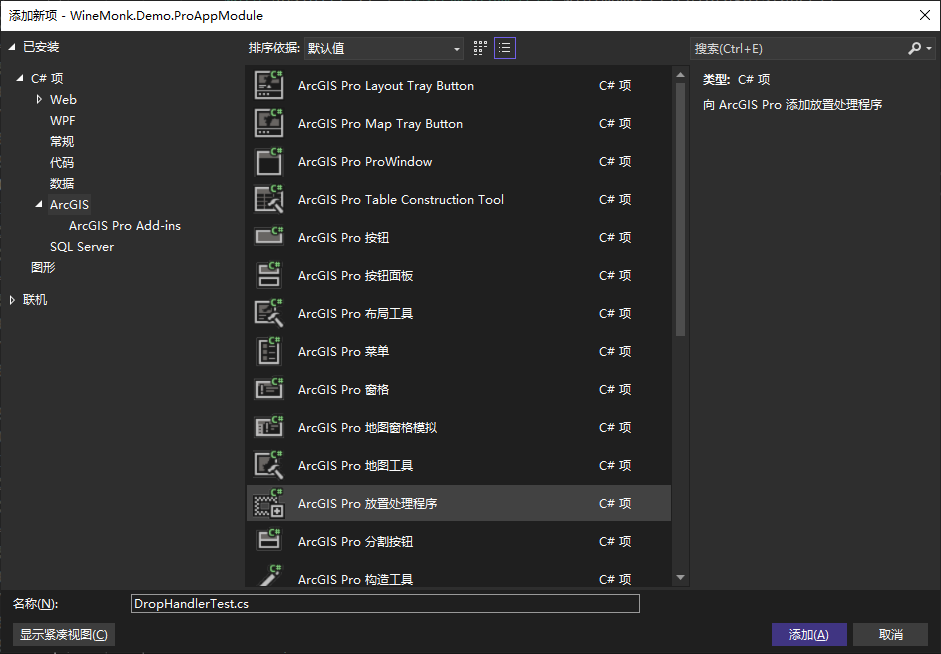
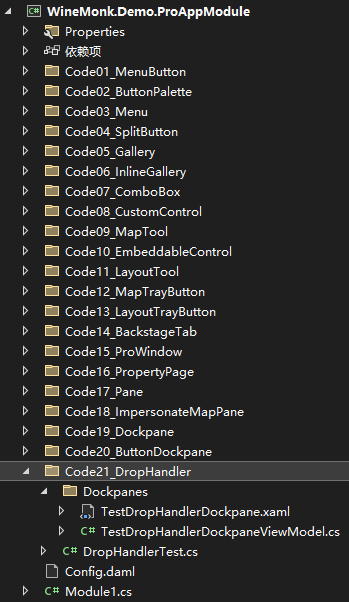
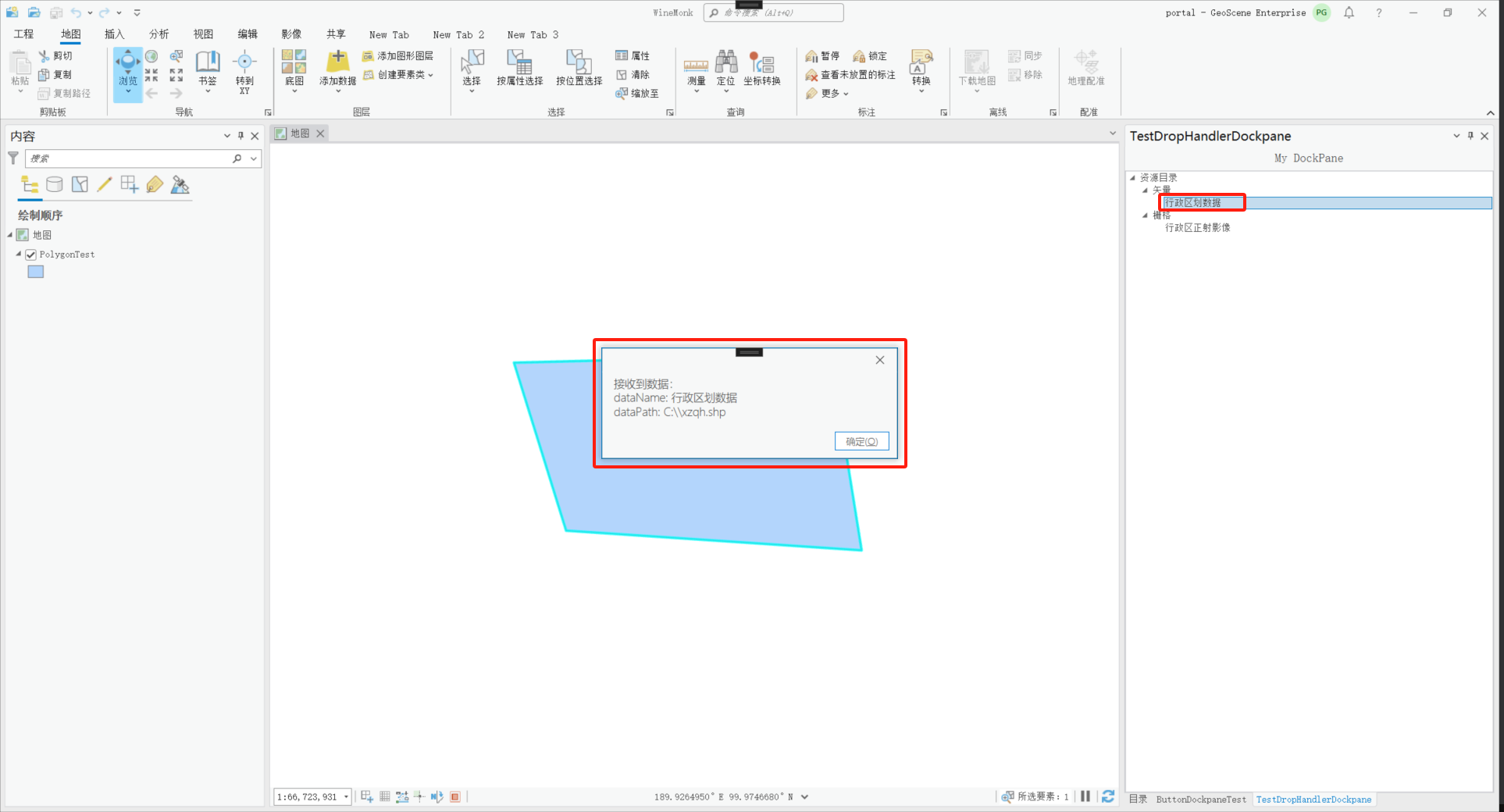
22.2 Code
放置处理程序可以实现文件拖动放置、TreeVIew、ListBox等控件拖动放置功能,此处新建一个停靠窗并添加一个TreeVIew,实现节点拖动事件;
TestDropHandlerDockpane.xaml
xaml
<UserControl
x:Class="WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes.TestDropHandlerDockpaneView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:extensions="clr-namespace:ArcGIS.Desktop.Extensions;assembly=ArcGIS.Desktop.Extensions"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:ui="clr-namespace:WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes"
d:DataContext="{Binding Path=ui.TestDropHandlerDockpaneViewModel}"
d:DesignHeight="300"
d:DesignWidth="300"
mc:Ignorable="d">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<extensions:DesignOnlyResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<DockPanel
Grid.Row="0"
Height="30"
KeyboardNavigation.TabNavigation="Local"
LastChildFill="true">
<TextBlock Style="{DynamicResource Esri_TextBlockDockPaneHeader}" Text="{Binding Heading}">
<TextBlock.ToolTip>
<WrapPanel MaxWidth="300" Orientation="Vertical">
<TextBlock Text="{Binding Heading}" TextWrapping="Wrap" />
</WrapPanel>
</TextBlock.ToolTip>
</TextBlock>
</DockPanel>
<TreeView
Grid.Row="1"
AllowDrop="False"
MouseMove="TreeView_MouseMove"
PreviewMouseLeftButtonDown="TreeView_PreviewMouseLeftButtonDown">
<TreeViewItem AllowDrop="False" Header="资源目录">
<TreeViewItem AllowDrop="False" Header="矢量">
<TreeViewItem
AllowDrop="True"
Header="行政区划数据"
Tag="C:\\xzqh.shp" />
</TreeViewItem>
<TreeViewItem AllowDrop="False" Header="栅格">
<TreeViewItem
AllowDrop="True"
Header="行政区正射影像"
Tag="C:\\xzq.tif" />
</TreeViewItem>
</TreeViewItem>
</TreeView>
</Grid>
</UserControl>
TestDropHandlerDockpaneViewModel.cs
csharp
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Framework.Contracts;
namespace WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes
{
internal class TestDropHandlerDockpaneViewModel : DockPane
{
private const string _dockPaneID = "WineMonk_Demo_ProAppModule_Code21_DropHandler_Dockpanes_TestDropHandlerDockpane";
protected TestDropHandlerDockpaneViewModel() { }
/// <summary>
/// Show the DockPane.
/// </summary>
internal static void Show()
{
DockPane pane = FrameworkApplication.DockPaneManager.Find(_dockPaneID);
if (pane == null)
return;
pane.Activate();
}
/// <summary>
/// Text shown near the top of the DockPane.
/// </summary>
private string _heading = "My DockPane";
public string Heading
{
get { return _heading; }
set
{
SetProperty(ref _heading, value, () => Heading);
}
}
}
/// <summary>
/// Button implementation to show the DockPane.
/// </summary>
internal class TestDropHandlerDockpane_ShowButton : Button
{
protected override void OnClick()
{
TestDropHandlerDockpaneViewModel.Show();
}
}
}
DropHandlerTest.cs
cs
using ArcGIS.Desktop.Framework.Contracts;
using ArcGIS.Desktop.Framework.DragDrop;
using System;
using System.Windows;
namespace WineMonk.Demo.ProAppModule.Code21_DropHandler
{
internal class DropHandlerTest : DropHandlerBase
{
public override void OnDragOver(DropInfo dropInfo)
{
//default is to accept our data types
dropInfo.Effects = DragDropEffects.All;
}
public override void OnDrop(DropInfo dropInfo)
{
//eg, if you are accessing a dropped file
//string filePath = dropInfo.Items[0].Data.ToString();
if (dropInfo.Items == null || dropInfo.Items.Count < 1)
{
return;
}
DropDataItem dropDataItem = dropInfo.Items[0];
object data = dropDataItem.Data;
if (data == null)
{
return;
}
if (data is DataObject dataObject)
{
string[] formats = dataObject.GetFormats();
if (formats == null || formats.Length < 1)
{
return;
}
string msg = string.Empty;
foreach (string f in formats)
{
object subData = dataObject.GetData(f);
msg += $"{Environment.NewLine}{f}: {subData}";
}
ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show($"接收到数据:{msg}");
dropInfo.Handled = true;
}
//set to true if you handled the drop
//dropInfo.Handled = true;
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<groups>
<!-- comment this out if you have no controls on the Addin tab to avoid an empty group -->
<!-- 如果您没有插件选项卡上的控件,请将其注释掉,以避免出现空组 -->
<group id="WineMonk_Demo_ProAppModule_Group3" caption="Group 3" appearsOnAddInTab="false">
<button refID="WineMonk_Demo_ProAppModule_Code21_DropHandler_Dockpanes_TestDropHandlerDockpane_ShowButton" size="large" />
</group>
</groups>
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<button id="WineMonk_Demo_ProAppModule_Code21_DropHandler_Dockpanes_TestDropHandlerDockpane_ShowButton" caption="Show TestDropHandlerDockpane" className="WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes.TestDropHandlerDockpane_ShowButton" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonPurple32.png">
<tooltip heading="Show Dockpane">Show Dockpane<disabledText /></tooltip>
</button>
</controls>
<dockPanes>
<dockPane id="WineMonk_Demo_ProAppModule_Code21_DropHandler_Dockpanes_TestDropHandlerDockpane" caption="TestDropHandlerDockpane" className="WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes.TestDropHandlerDockpaneViewModel" dock="group" dockWith="esri_core_projectDockPane">
<content className="WineMonk.Demo.ProAppModule.Code21_DropHandler.Dockpanes.TestDropHandlerDockpaneView" />
</dockPane>
</dockPanes>
</insertModule>
</modules>
<dropHandlers>
<!--specific file extensions can be set like so: dataTypes=".XLS|.xls"-->
<insertHandler id="WineMonk_Demo_ProAppModule_Code21_DropHandler_DropHandlerTest" className="WineMonk.Demo.ProAppModule.Code21_DropHandler.DropHandlerTest" dataTypes="*" />
</dropHandlers>
23 ArcGIS Pro 构造工具
23.1 添加控件
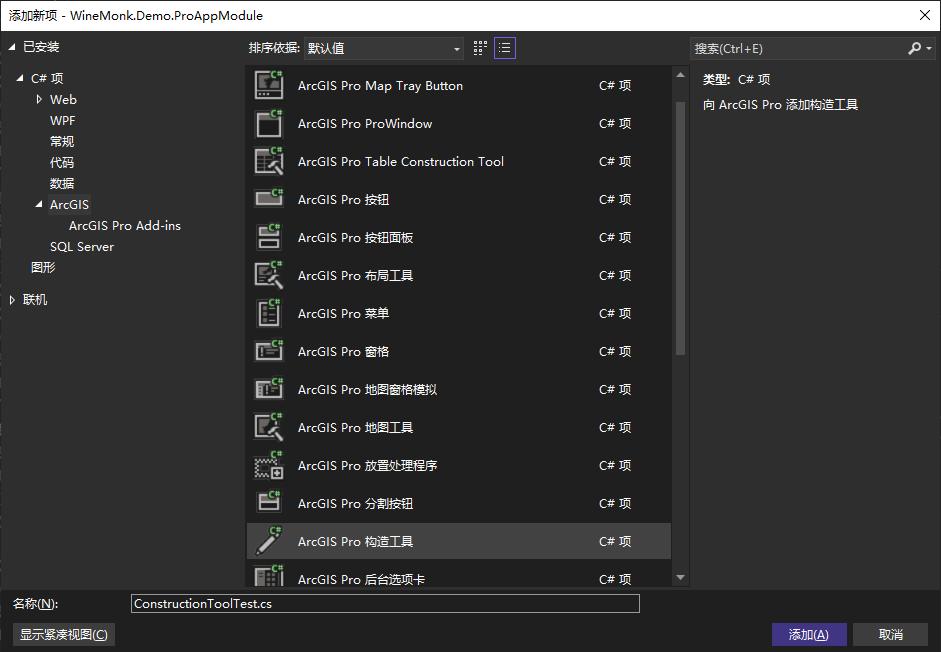
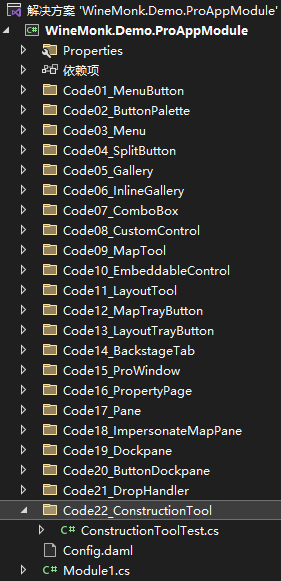
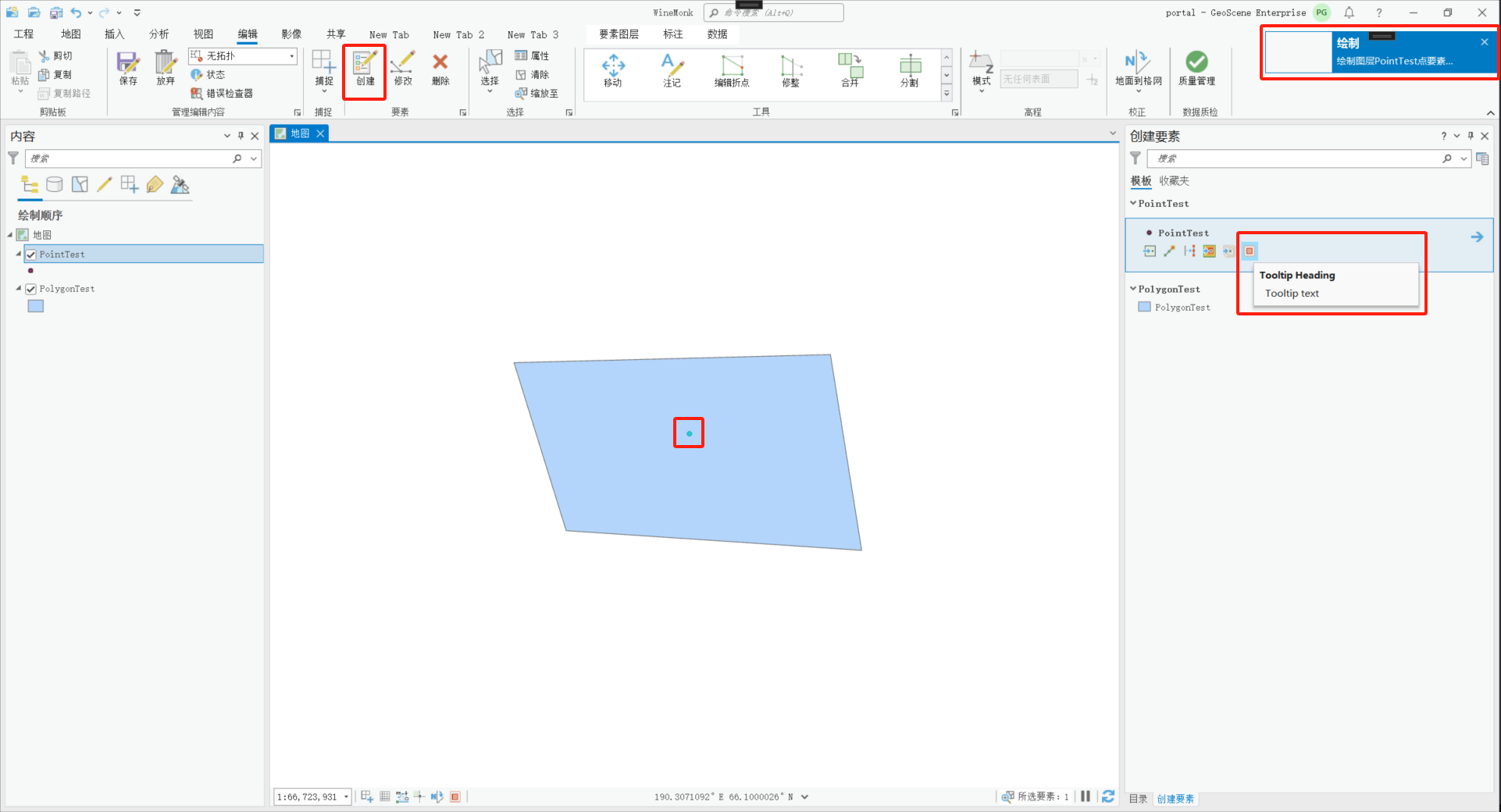
23.2 Code
ConstructionToolTest.cs
csharp
using ArcGIS.Core.Geometry;
using ArcGIS.Desktop.Editing;
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Mapping;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code22_ConstructionTool
{
internal class ConstructionToolTest : MapTool
{
public ConstructionToolTest()
{
IsSketchTool = true;
UseSnapping = true;
// Select the type of construction tool you wish to implement.
// Make sure that the tool is correctly registered with the correct component category type in the daml
SketchType = SketchGeometryType.Point;
// SketchType = SketchGeometryType.Line;
// SketchType = SketchGeometryType.Polygon;
//Gets or sets whether the sketch is for creating a feature and should use the CurrentTemplate.
UsesCurrentTemplate = true;
//Gets or sets whether the tool supports firing sketch events when the map sketch changes.
//Default value is false.
FireSketchEvents = true;
}
/// <summary>
/// Called when the sketch finishes. This is where we will create the sketch operation and then execute it.
/// </summary>
/// <param name="geometry">The geometry created by the sketch.</param>
/// <returns>A Task returning a Boolean indicating if the sketch complete event was successfully handled.</returns>
protected override Task<bool> OnSketchCompleteAsync(Geometry geometry)
{
if (CurrentTemplate == null || geometry == null)
return Task.FromResult(false);
// Create an edit operation
var createOperation = new EditOperation();
createOperation.Name = string.Format("Create {0}", CurrentTemplate.Layer.Name);
createOperation.SelectNewFeatures = true;
// Queue feature creation
createOperation.Create(CurrentTemplate, geometry);
// Execute the operation
Notification notification = new Notification();
notification.Title = "绘制";
notification.Message = $"绘制图层{CurrentTemplate.Layer.Name}点要素...";
FrameworkApplication.AddNotification(notification);
return createOperation.ExecuteAsync();
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<tool id="WineMonk_Demo_ProAppModule_Code22_ConstructionTool_ConstructionToolTest" categoryRefID="esri_editing_construction_point" caption="ConstructionToolTest" className="WineMonk.Demo.ProAppModule.Code22_ConstructionTool.ConstructionToolTest" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonRed16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonRed32.png">
<!-- note: use esri_editing_construction_polyline, esri_editing_construction_polygon for categoryRefID as needed -->
<!-- esri_editing_construction_annotation
esri_editing_construction_dimension
esri_editing_construction_knowledge_graph_entity
esri_editing_construction_knowledge_graph_relationship
esri_editing_construction_multipatch
esri_editing_construction_multipoint
esri_editing_construction_point
esri_editing_construction_polygon
esri_editing_construction_polyline
esri_editing_construction_table -->
<tooltip heading="Tooltip Heading">Tooltip text<disabledText /></tooltip>
<content guid="cdfdb0f2-c229-458e-bac8-5a638cd98bb3" />
</tool>
</controls>
</insertModule>
</modules>
24 ArcGIS Pro 表构造工具
24.1 添加控件
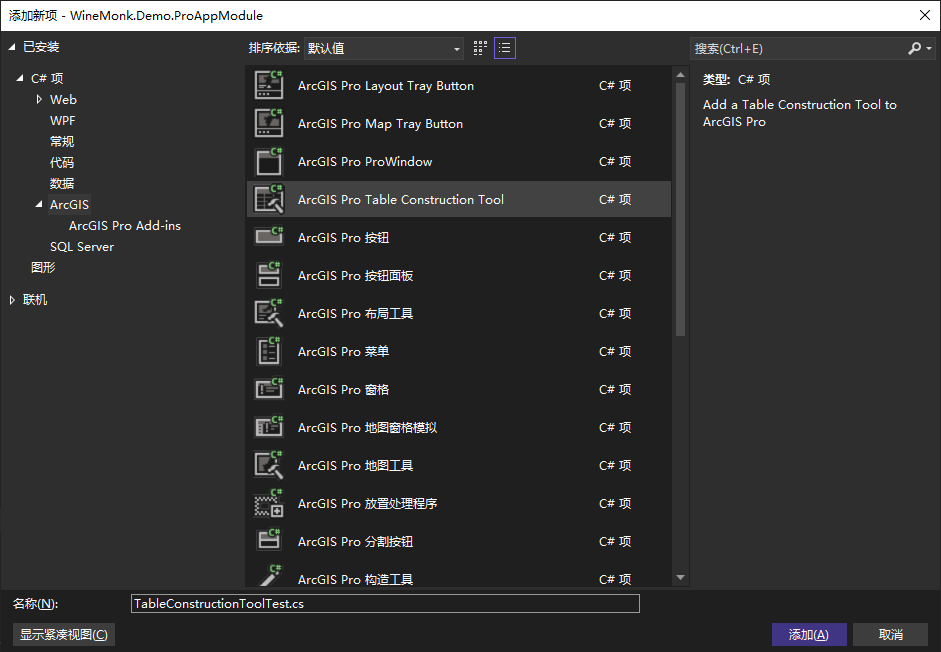
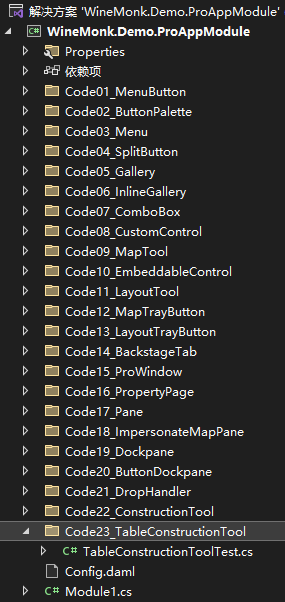
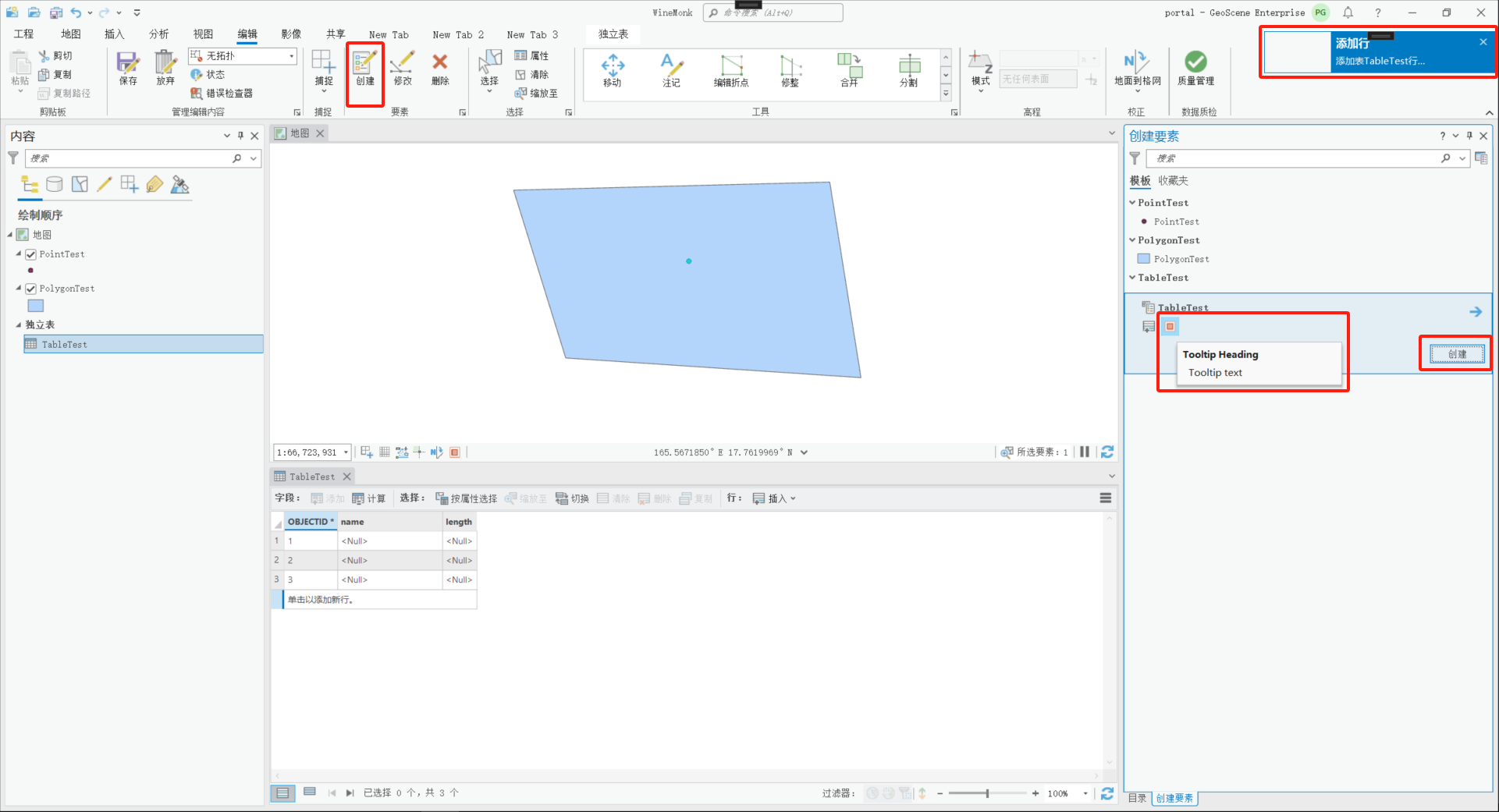
24.2 Code
TableConstructionToolTest.cs
csharp
using ArcGIS.Core.Geometry;
using ArcGIS.Desktop.Editing;
using ArcGIS.Desktop.Framework;
using ArcGIS.Desktop.Mapping;
using System.Threading.Tasks;
namespace WineMonk.Demo.ProAppModule.Code23_TableConstructionTool
{
internal class TableConstructionToolTest : MapTool
{
public TableConstructionToolTest()
{
IsSketchTool = false;
// set the SketchType to None
SketchType = SketchGeometryType.None;
//Gets or sets whether the sketch is for creating a feature and should use the CurrentTemplate.
UsesCurrentTemplate = true;
}
/// <summary>
/// Called when the "Create" button is clicked. This is where we will create the edit operation and then execute it.
/// </summary>
/// <param name="geometry">The geometry created by the sketch - will be null because SketchType = SketchGeometryType.None</param>
/// <returns>A Task returning a Boolean indicating if the sketch complete event was successfully handled.</returns>
protected override Task<bool> OnSketchCompleteAsync(Geometry geometry)
{
if (CurrentTemplate == null)
return Task.FromResult(false);
// geometry will be null
// Create an edit operation
var createOperation = new EditOperation();
createOperation.Name = string.Format("Create {0}", CurrentTemplate.StandaloneTable.Name);
createOperation.SelectNewFeatures = false;
// determine the number of rows to add
var numRows = this.CurrentTemplateRows;
for (int idx = 0; idx < numRows; idx++)
// Queue feature creation
createOperation.Create(CurrentTemplate, null);
// Execute the operation
Notification notification = new Notification();
notification.Title = "添加行";
notification.Message = $"添加表{CurrentTemplate.MapMember.Name}行...";
FrameworkApplication.AddNotification(notification);
return createOperation.ExecuteAsync();
}
}
}
Config.daml
xml
<modules>
<insertModule id="WineMonk_Demo_ProAppModule_Module" className="Module1" autoLoad="false" caption="Module1">
<controls>
<!-- add your controls here -->
<!-- 在这里添加控件 -->
<tool id="WineMonk_Demo_ProAppModule_Code23_TableConstructionTool_TableConstructionToolTest" categoryRefID="esri_editing_construction_table" caption="TableConstructionToolTest" className="WineMonk.Demo.ProAppModule.Code23_TableConstructionTool.TableConstructionToolTest" loadOnClick="true" smallImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonRed16.png" largeImage="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericButtonRed32.png">
<tooltip heading="Tooltip Heading">Tooltip text<disabledText /></tooltip>
<content guid="a3d2b675-374a-44c1-be88-e0991cc7fe39" />
</tool>
</controls>
</insertModule>
</modules>