文章目录
本博客相关参考文档 :
- WebAPIs 参考文档 : https://developer.mozilla.org/zh-CN/docs/Web/API
- getElementById 函数参考文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/Document/getElementById
- Element 对象参考文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/Element
- getElementsByTagName 文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/Document/getElementsByTagName
- HTMLCollection 文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/HTMLCollection
- getElementsByClassName 文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/Document/getElementsByClassName
- querySelector 函数文档 : https://developer.mozilla.org/zh-CN/docs/Web/API/Document/querySelector
- 【CSS】CSS 总结 ① ( CSS 引入方式 | CSS 选择器 | 基础选择器 | 复合选择器 ) ★
- querySelectorAll 函数 : https://developer.mozilla.org/zh-CN/docs/Web/API/Document/querySelectorAll
- NodeList 对象 : https://developer.mozilla.org/zh-CN/docs/Web/API/NodeList
一、获取特殊元素
HTML 网页的基本结构如下 :
html
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
</body>
</html>
在 HTML 标签结构中 ,
- html 标签是最顶层的标签 , 所有的元素都在 html 标签内部 ,
- body 标签是显示部分内容的 顶层标签 ;
通过 JavaScript 和 DOM 操作 可以获取上述两个 html 和 body 特殊标签 元素 ;
1、获取 html 元素
通过 document.documentElement 属性 , 可以获取文档中的 html 元素 , 该元素是 HTML 网页文档的最顶层元素 ;
代码示例 :
javascript
const htmlElement = document.documentElement;
console.log(htmlElement); // 输出整个 <html> 元素的 DOM 对象
2、获取 body 元素
使用 document.body 属性 , 可以获取 body 元素 ;
代码示例 :
javascript
const bodyElement = document.body;
console.log(bodyElement); // 输出整个 <body> 元素的 DOM 对象
3、完整代码示例
在下面的代码中 , 通过 document.body 获取 body 元素 , 将背景颜色设置为黄色 ;
通过 document.documentElement 获取 html 元素 , 将该标签下的所有字体大小设置为 30 像素 ;
代码示例 :
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<!-- 设置 meta 视口标签 -->
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no,maximum-scale=1.0,minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>JavaScript</title>
<style></style>
</head>
<body>
<div class='box'>Hello</div>
<div class='box'>Hello</div>
<div>Hello</div>
<div>Hello2</div>
<div id="nav">
<div>Hello2</div>
<div class='box'>Hello2</div>
<div class='box'>Hello2</div>
</div>
<script>
// 注意 : HTML 文档加载顺序是从上到下加载
// 这里要先加载标签 , 然后加载 JavaScript 脚本
// 设置 <body> 元素的背景颜色
document.body.style.backgroundColor = 'yellow';
// 设置 <html> 元素的字体大小
document.documentElement.style.fontSize = '30px';
// 获取 <body> 元素的子节点数量
const bodyChildrenCount = document.body.children.length;
console.log(`Body 元素的子节点数量: ${bodyChildrenCount}`);
</script>
</body>
</html>
执行结果 :
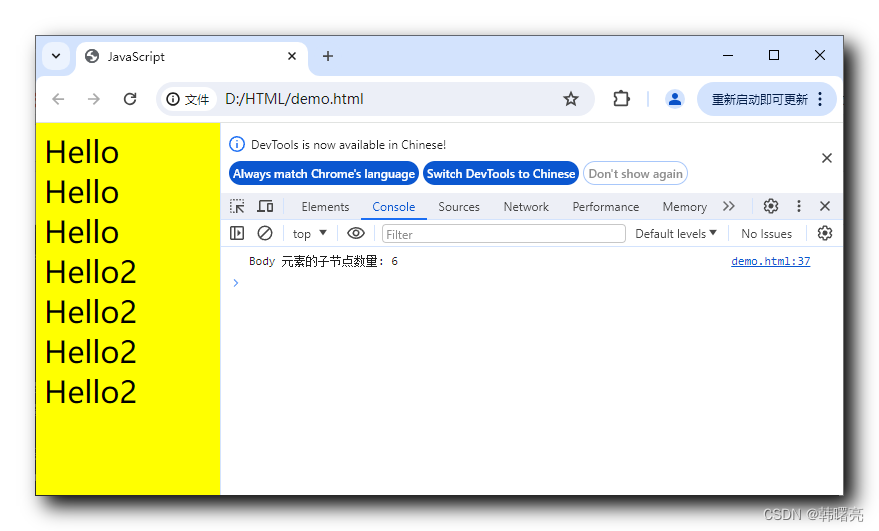