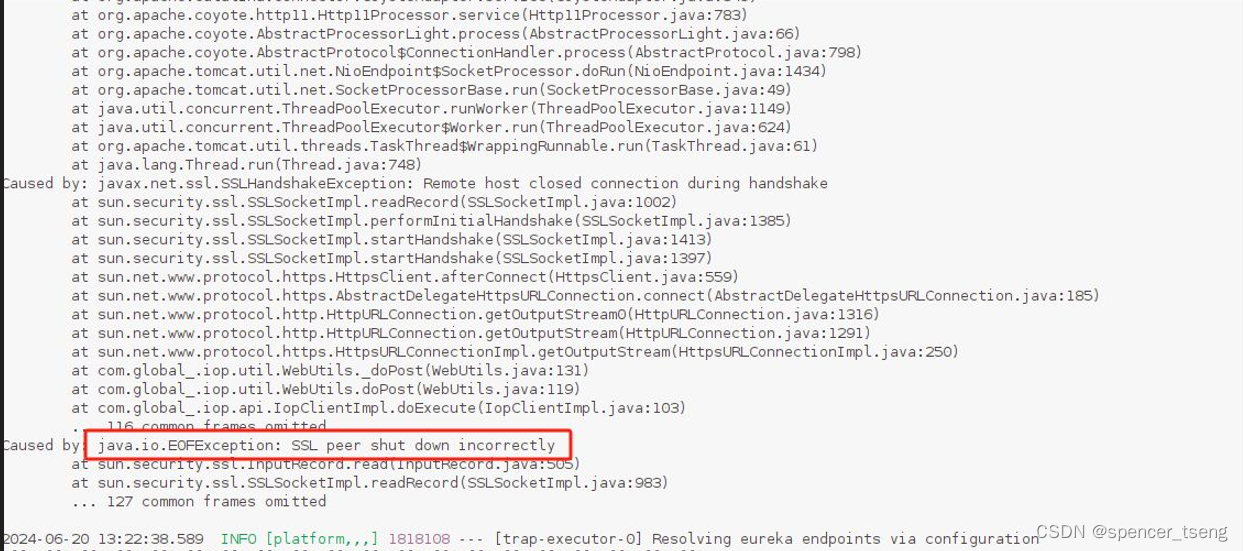
可能是因为 1)https设置 2)超时设置
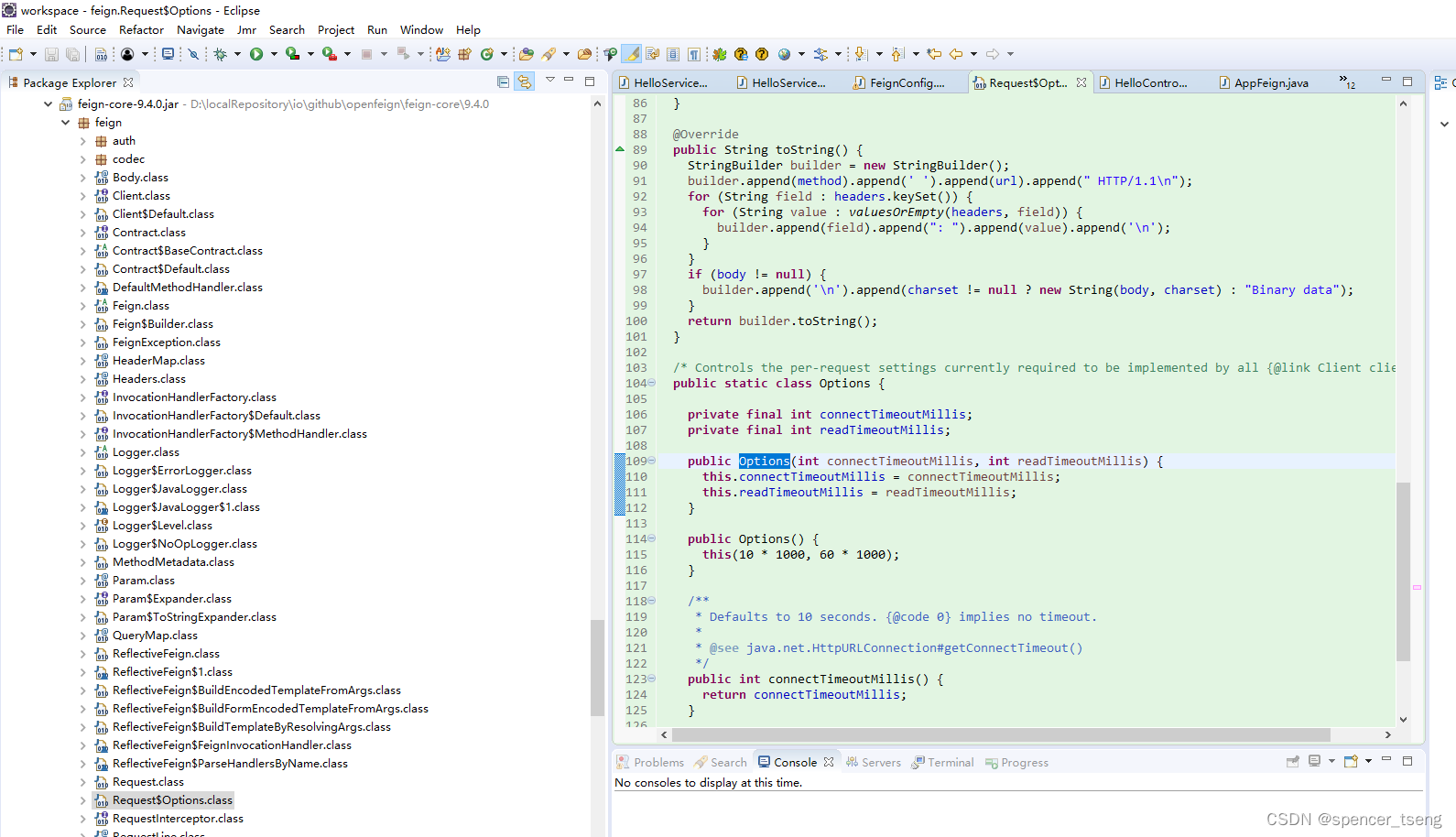
FeignConfig.java
package zwf.service;
import java.io.IOException;
import java.io.InputStream;
import java.security.KeyStore;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSocketFactory;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLContexts;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import feign.Client;
import feign.Feign;
import feign.Request;
import feign.Retryer;
@Configuration
public class FeignConfig
{
@Bean
public Request.Options feignOptions()
{
int connectTimeoutMillis = 5 * 1000;
int readTimeoutMillis = 5 * 1000;
//请求连接超时,请求读取超时
return new Request.Options(connectTimeoutMillis, readTimeoutMillis);
}
@Bean
public Retryer feignRetryer()
{
long period = 100;
long maxPeriod = 1000;
int maxAttempts = 5;//最多尝试次数
//
return new Retryer.Default(period, maxPeriod, maxAttempts);
}
@Bean
public Feign.Builder feignBuilder()
{
final Client trustSSLSockets = client();
return Feign.builder().client(trustSSLSockets);
}
public static SSLSocketFactory feignTrustingSSLSocketFactory = null;
@Bean
public Client client()
{
if (feignTrustingSSLSocketFactory == null)
{
try
{
feignTrustingSSLSocketFactory = getFeignTrustingSSLSocketFactory();
}
catch (Exception e)
{
e.printStackTrace();
}
}
Client client = new Client.Default(feignTrustingSSLSocketFactory, new NoopHostnameVerifier());
return client;
}
public static SSLSocketFactory getFeignTrustingSSLSocketFactory() throws Exception
{
String passwd = "zengwenfeng";
String keyStoreType = "zengwenfeng";
InputStream inputStream = null;
SSLContext sslContext = SSLContext.getInstance("SSL");
try
{
inputStream = FeignConfig.class.getResourceAsStream("d://zengwenfeng.p12");
KeyStore keyStore = KeyStore.getInstance(keyStoreType);
keyStore.load(inputStream, passwd.toCharArray());
sslContext = SSLContexts.custom().loadKeyMaterial(keyStore, passwd.toCharArray()).build();
}
catch (Exception e)
{
throw new RuntimeException(e);
}
finally
{
if (inputStream != null)
{
try
{
inputStream.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
return sslContext.getSocketFactory();
}
}