二叉树从根节点出发的所有路径
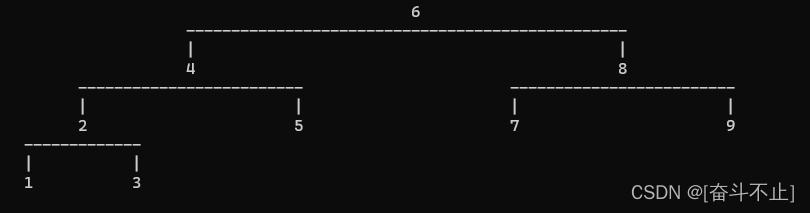
看上图中 二叉树结构
从根节点出发的所有路径 如下
6->4->2->1
6->4->2->3
6->4->5
6->8->7
6->8->9
逻辑思路:
按照先序遍历 加 回溯法 实现
代码如下
csharp
// 调用此方法,将根节点传递进来
public static IList<IList<int>> Path(BinNode<int> root)
{
// 存储所有路径,每一项为一个路径
List<IList<int>> resultList = new List<IList<int>>();
List<int> list = new List<int>();
Path(root, resultList, list);
// 遍历打印所有路径
foreach(var listTemp in resultList)
{
string msg = "";
foreach(var item in listTemp)
{
msg += "->" + item;
}
Console.WriteLine(msg);
}
return resultList;
}
public static void Path(BinNode<int> root, IList<IList<int>> resultList, List<int> list)
{
if (null == root)
{
return;
}
list.Add(root.Element);
// 如果 左子树和右子树 都不存在,则路径结束
if (null == root.LeftChild && null == root.RightChild)
{
List<int> newList = new List<int>(list);
// 将路径存储
resultList.Add(newList);
return;
}
if (root.LeftChild != null)
{
Path(root.LeftChild, resultList, list);
// 回溯
list.RemoveAt(list.Count - 1);
}
if (root.RightChild != null)
{
Path(root.RightChild, resultList, list);
// 回溯
list.RemoveAt(list.Count - 1);
}