原生js实现的数字时间上下切换显示时间的效果,有参考相关设计,思路比较难,代码其实很简单
效果
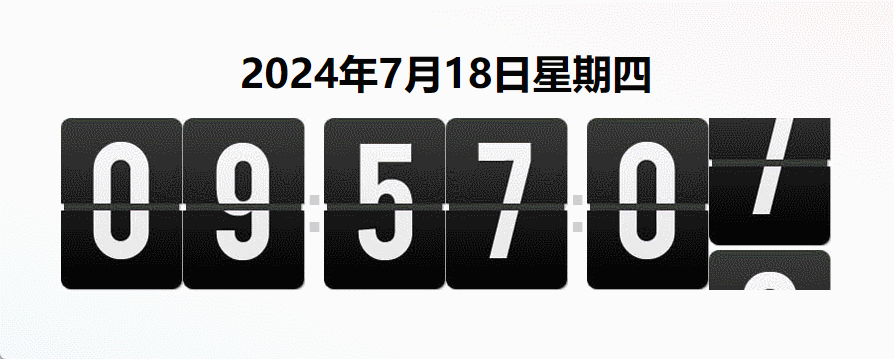
代码实现
- 必要的样式
css
<style>
* {
padding: 0;
margin: 0;
}
.content{
/* text-align: center; */
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
height: 100vh;
background: linear-gradient(202deg,#F3E7E9 0%,#FFFFFF 53%,#E3EEFF 100%);
}
#title{
margin:20px;
font-size: 40px;
}
ul {
list-style: none;
overflow: hidden;
}
li {
float: left;
}
li.time-box {
width: 122px;
height: 172px;
overflow: hidden;
position: relative;
}
li.colon{
animation: dotLignt 1s linear infinite alternate;
font-size: 80px;
height: 172px;
line-height: 172px;
font-family: 'Franklin Gothic Medium', 'Arial Narrow', Arial, sans-serif;
}
/*
闪烁东湖
*/
@keyframes dotLignt {
0% {
opacity: 1;
}
50% {
opacity: 50%;
}
100% {
opacity: 0;
}
}
</style>
- 静态页面
html
<div class="content">
<h2 id="title"></h2>
<p id="time"></p>
<ul>
<li id="h1" class="time-box">
<img class="time" src="./img/0.JPG" alt="" srcset="" />
</li>
<li id="h2" class="time-box">
<img class="time" src="./img/1.JPG" alt="" srcset="" />
</li>
<li class="colon">
<span>:</span>
</li>
<li id="fen1" class="time-box">
<img class="time" src="./img/2.JPG" alt="" srcset="" />
</li>
<li id="fen2" class="time-box">
<img class="time" src="./img/3.JPG" alt="" srcset="" />
</li>
<li class="colon">
<span>:</span>
</li>
<li id="miao1" class="time-box">
<img class="time" src="./img/4.JPG" alt="" srcset="" />
</li>
<li id="miao2" class="time-box up">
<img class="time" src="./img/5.JPG" alt="" srcset="" />
</li>
</ul>
</div>
- js核心代码
js
window.onload = function () {
const oBody = document.body
const oP = oBody.querySelector('#time')
const oTitle = document.querySelector('#title')
const oImg = document.querySelectorAll('img.time')
const oH1 = document.querySelector('#h1')
const oH2 = document.querySelector('#h2')
const oFen1 = document.querySelector('#fen1')
const oFen2 = document.querySelector('#fen2')
const oMiao1 = document.querySelector('#miao1')
const oMiao2 = document.querySelector('#miao2')
let isInit = true
fnTime()
function fnTime() {
const myTime = new Date()
const year = myTime.getFullYear()
const month = myTime.getMonth() + 1
const day = myTime.getDate()
const hour = myTime.getHours()
const minute = myTime.getMinutes()
const second = myTime.getSeconds()
//星期
let week = myTime.getDay()
switch (week) {
case 0:
week = '星期日'
break
case 1:
week = '星期一'
break
case 2:
week = '星期二'
break
case 3:
week = '星期三'
break
case 4:
week = '星期四'
break
case 5:
week = '星期五'
break
case 6:
week = '星期六'
break
default:
week = ''
}
const time = fnAddZero(hour) + fnAddZero(minute) + fnAddZero(second)
// oP.innerHTML = time
oTitle.innerHTML = year + '年' + month + '月' + day + '日' + week
//初始化加载一次,防止刷新的时候出现闪屏
if(isInit){
for (let i = 0; i < oImg.length; i++) {
oImg[i].src = `./img/${time.charAt(i)}.JPG`
}
}
isInit = false
return time
}
//辅助方法,补0
function fnAddZero(num) {
if (num < 10) {
return '0' + num
}
return '' + num
}
let ht1=0;
let h1 = 0
setInterval(function () {
let a = fnTime();
//获得当前的子结点
if(a[0] != h1){
h1 = a[0];
let childImg = oH1.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[0]+'.jpg';
oH1.appendChild(imgM);
let m2 = setInterval(function () {
ht1+=1;
oH1.scrollTop=ht1;
if (ht1>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
ht1=0;
},100)
}
},1)
}
},1000);
let ht2=0;
let h2 = 0
setInterval(function () {
let a = fnTime();
//获得当前的子结点
if(a[1] != h2){
h2 = a[1];
let childImg = oH2.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[1]+'.jpg';
oH2.appendChild(imgM);
let m2 = setInterval(function () {
ht2+=1;
oH2.scrollTop=ht2;
if (ht2>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
ht2=0;
},100)
}
},1)
}
},1000);
let ft1=0;
let f1 = 0
setInterval(function () {
let a = fnTime();
//获得当前的子结点
if(a[2] != f1){
f1 = a[2];
let childImg = oFen1.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[2]+'.jpg';
oFen1.appendChild(imgM);
let m2 = setInterval(function () {
ft1+=1;
// console.log(mt2);
oFen1.scrollTop=ft1;
if (ft1>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
ft1=0;
},100)
}
},1)
}
},1000);
let ft2=0;
let f2 = 0
setInterval(function () {
let a = fnTime();
//获得当前的子结点
if(a[3] != f2){
f2 = a[3];
let childImg = oFen2.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[3]+'.jpg';
oFen2.appendChild(imgM);
let m2 = setInterval(function () {
ft2+=1;
oFen2.scrollTop=ft2;
if (ft2>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
ft2=0;
},100)
}
},1)
}
},1000);
let mt1=0;
let m1 = 0
setInterval(function () {
let a = fnTime();
console.log("🚀 ~ a:", a)
//获得当前的子结点
if(a[4] != m1){
console.log("🚀 ~ m1:", m1)
console.log("🚀 ~ a[4]:", a[4])
m1 = a[4];
let childImg = oMiao1.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[4]+'.jpg';
oMiao1.appendChild(imgM);
let m2 = setInterval(function () {
mt1+=1;
// console.log(mt2);
oMiao1.scrollTop=mt1;
if (mt1>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
mt1=0;
},100)
}
},1)
}
},1000);
let mt2=0;
setInterval(function () {
let a = fnTime();
//获得当前的子结点
let childImg = oMiao2.getElementsByTagName('img')[0];
let imgM = document.createElement('img');
imgM.src='img/'+a[5]+'.jpg';
oMiao2.appendChild(imgM);
let m2 = setInterval(function () {
mt2+=1;
// console.log(mt2);
oMiao2.scrollTop=mt2;
if (mt2>=70){
clearInterval(m2);
setTimeout(function () {
childImg.remove();
mt2=0;
},100)
}
},1)
},1000);
}
这样就实现了我们的数字时间的切换效果