前言
如果在开发大型项目 同时为多人协作开发 那么 ESLint 在项目中极为重要 在使用 ESLint 的同时 也需要使用 Pretter插件 统一对代码进行格式化 二者相辅相成 缺一不可
1. 安装 VsCode 插件
在 VsCode 插件市场搜索安装 ESLint 和 Pretter
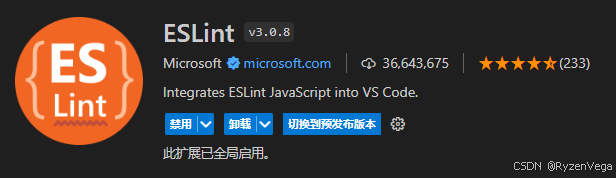
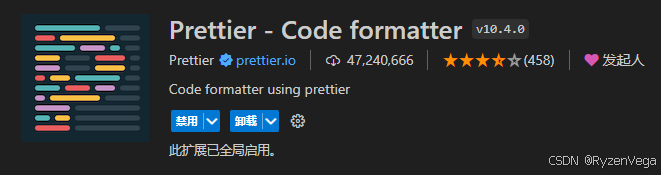
2. 安装依赖
这里直接在 package.json 内写入 之后使用 npm 等包管理器直接 install 安装即可
javascript
"devDependencies": {
"@vue/eslint-config-prettier": "^9.0.0",
"eslint": "^8.57.0",
"eslint-plugin-import": "^2.29.1",
"eslint-plugin-prettier": "^5.2.1",
"eslint-plugin-simple-import-sort": "^12.1.1",
"eslint-plugin-vue": "^9.23.0",
"eslint-plugin-vue-scoped-css": "^2.8.1",
"prettier": "^3.2.5",
"vite-plugin-eslint": "^1.8.1",
}
以上依赖包具体作用如下 感兴趣可以了解:
@vue/eslint-config-prettier
这是一个 ESLint 配置,专为 Vue 项目整合 Prettier 格式化而设计,确保 ESLint 规则不会与 Prettier 的格式化规则发生冲突。
eslint
ESLint 的核心库,用于识别和报告 JavaScript 代码中的模式匹配,帮助维护代码质量和风格一致性。
eslint-plugin-import
提供了一系列规则,用于检查 ES6+ 的 import/export 语法,帮助管理模块的导入和避免文件路径错误、拼写错误等问题。
eslint-plugin-prettier
将 Prettier 作为 ESLint 规则运行,可以在使用 ESLint 的同时应用 Prettier 的代码格式化功能。
eslint-plugin-simple-import-sort
用于自动排序 import 语句,以保持代码的整洁和一致性,减少合并时的冲突。
eslint-plugin-vue
官方 Vue.js 的 ESLint 插件,提供了适用于 Vue 文件的 ESLint 规则,帮助维护
.vue
文件中的 JavaScript 和模板代码的质量。prettier
一个流行的代码格式化工具,用于自动格式化代码,使其符合一致的风格。
vite-plugin-eslint
用于将 ESLint 集成到 Vite 构建流程中,使得在使用 Vite 开发 Vue 项目时,可以实时进行代码质量检查。
eslint-plugin-vue-scoped-css
这个插件专门用于检查Vue单文件组件中的scoped CSS,确保样式的作用域正确无误。
3. 配置 VsCode 规则
1. 在项目根目录创建 .vscode 文件夹
如果你的项目使用 VsCode 打开 那这个文件夹应该是自动生成的
2. 创建规则文件
在 .vscode 文件夹里创建 extensions.json 和 settings.json
此时 您的文件结构理应如此
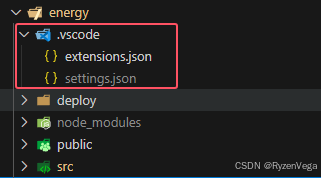
3. 编辑规则文件
extensions.json
主要作为实时检查和修正代码问题使用
需配合 VsCode 中 ESLint 扩展和 .eslintrc 配置文件使用
javascript
{
"recommendations": ["dbaeumer.vscode-eslint"]
}
settings.json
主要作为对指定文件进行格式化 从而符合预定标准
javascript
{
"editor.formatOnType": true, // 控制编辑器在键入一行后是否自动格式化该行
"editor.formatOnSave": true, // 在保存时格式化文档
"eslint.codeAction.showDocumentation": {
"enable": true // 显示相关规则的文档链接
},
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "explicit" // 保存文件时 应用所有 ESLint 的自动修复
},
"files.eol": "\n", // 设置文件的结束行字符为 Unix 风格的换行符
"editor.tabSize": 2, // 设置制表符大小为2个空格
"eslint.format.enable": true, // 这允许 ESLint 作为格式化工具
"eslint.validate": ["javascript", "javascriptreact", "vue"],
"[vue]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "dbaeumer.vscode-eslint"
},
"[javascriptreact]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "dbaeumer.vscode-eslint"
},
"[javascript]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "dbaeumer.vscode-eslint"
}
}
4. 配置ESLint
在项目根目录创建 .eslintrc
.eslintrc
代码最后注释掉的 overrides 数组为对 Vue 组件及命名规范
建议打开并使用
javascript
{
"root": true,
"extends": [
"plugin:vue/vue3-recommended",
"plugin:vue-scoped-css/base",
"plugin:prettier/recommended",
"plugin:vue/vue3-essential",
"eslint:recommended",
"@vue/eslint-config-prettier/skip-formatting"
],
"env": {
"browser": true, // 浏览器全局变量
"node": true, // Node.js全局变量和作用域
"jest": true, // Jest全局变量
"es6": true // 启用ES6的特性
},
"globals": {
"defineProps": "readonly", // 将defineProps定义为全局只读
"defineEmits": "readonly" // 将defineEmits定义为全局只读
},
"plugins": [
"vue", // 使用Vue插件
"simple-import-sort" // 使用simple-import-sort插件进行导入排序
],
"parserOptions": {
"sourceType": "module", // 指定来源的类型,是模块
"ecmaFeatures": {
"jsx": true // 启用JSX
}
},
"settings": {
"import/extensions": [".js", ".jsx"] // 为导入语句指定文件扩展名
},
"rules": {
"no-console": "off", // 关闭禁止console规则
"no-continue": "off", // 关闭禁止continue语句规则
"no-restricted-syntax": "off", // 关闭限制特定语法规则
"no-plusplus": "off", // 允许使用++操作符
"no-param-reassign": "off", // 关闭禁止对函数参数重新赋值规则
"no-shadow": "off", // 关闭变量声明覆盖外层作用域变量规则
"guard-for-in": "off", // 关闭需要在for-in循环中有if语句的规则
"import/extensions": "off", // 关闭导入文件需包含文件后缀规则
"import/no-unresolved": "off", // 关闭导入路径错误检查
"import/no-extraneous-dependencies": "off", // 关闭禁止未列在package.json的依赖导入规则
"import/prefer-default-export": "off", // 关闭优先使用默认导出的规则
"import/first": "off", // 关闭所有导入语句之前不能有执行代码规则
"no-unused-vars": [
"error", // 启用未使用变量错误提示
{
"argsIgnorePattern": "^_", // 忽略以下划线开头的参数
"varsIgnorePattern": "^_" // 忽略以下划线开头的变量
}
],
"no-use-before-define": "off", // 关闭禁止在声明之前使用变量或函数规则
"class-methods-use-this": "off", // 关闭类方法必须使用this规则
"simple-import-sort/imports": "error", // 对导入语句进行排序
"simple-import-sort/exports": "error" // 对导出语句进行排序
}
// "overrides": [
// {
// "files": ["*.vue"],
// "rules": {
// "vue/component-name-in-template-casing": [2, "kebab-case"], // 组件名必须是kebab-case
// "vue/require-default-prop": 0, // 关闭属性必须有默认值规则
// "vue/multi-word-component-names": 0, // 关闭组件名必须为多单词规则
// "vue/no-reserved-props": 0, // 关闭禁止使用保留字作为组件属性规则
// "vue/no-v-html": 0, // 关闭禁止使用v-html指令规则
// "vue-scoped-css/enforce-style-type": ["error", { "allows": ["scoped"] }] // 强制使用scoped样式
// }
// }
// ]
}
5. 配置 Prettier
在项目根目录创建 .prettierrc.js
.prettierrc.js
javascript
export default {
// 一行最多 120 字符..
printWidth: 120,
// 使用 2 个空格缩进
tabWidth: 2,
// 不使用缩进符,而使用空格
useTabs: false,
// 行尾需要有分号
semi: true,
// 使用单引号
singleQuote: true,
// 对象的 key 仅在必要时用引号
quoteProps: 'as-needed',
// jsx 不使用单引号,而使用双引号
jsxSingleQuote: false,
// 末尾需要有逗号
trailingComma: 'all',
// 大括号内的首尾需要空格
bracketSpacing: true,
// jsx 标签的反尖括号需要换行
jsxBracketSameLine: false,
// 箭头函数,只有一个参数的时候,也需要括号
arrowParens: 'always',
// 每个文件格式化的范围是文件的全部内容
rangeStart: 0,
rangeEnd: Infinity,
// 不需要写文件开头的 @prettier
requirePragma: false,
// 不需要自动在文件开头插入 @prettier
insertPragma: false,
// 使用默认的折行标准
proseWrap: 'preserve',
// 根据显示样式决定 html 要不要折行
htmlWhitespaceSensitivity: 'css',
// vue 文件中的 script 和 style 内不用缩进
vueIndentScriptAndStyle: false,
// 换行符使用 lf
endOfLine: 'lf',
};
至此 所有配置结束
但需要注意的是 此时您的项目可能会各种红线
执行如下命令即可对所有文件执行格式化操作
javascript
npm run lint --fix