java
public static void main(String[] args) {
Map<String, Object> map=new HashMap<>();
map.put("A","");
map.put("B",new ArrayList<>());
map.put("C",null);
map.put("D",123);
System.out.println(map.toString());
Map<String, Object> mapNew = removeEmpty(map);
System.out.println(mapNew.toString());
}
如: 对于 空的list ,以及 双引号的 字符串,都认为是空。
移除方法 :
java
private static Map<String, Object> removeEmpty(Map<String, ?> paramsMap) {
return paramsMap.entrySet().stream()
.filter(entry -> entry.getValue() != null)
.filter(entry -> !(entry.getValue() instanceof String) || StringUtils.isNotEmpty((String) entry.getValue()))
.filter(entry -> !(entry.getValue() instanceof List) || CollectionUtils.isNotEmpty((List<?>) entry.getValue()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
}
效果示例 :
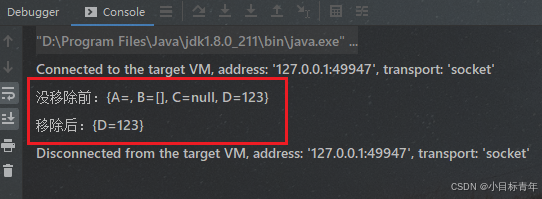