1. 图元添加
1.1 添加直线
csharp
// 添加直线
[CommandMethod(nameof(Test_AddLine1))]
public void Test_AddLine1()
{
using var tr = new DBTrans();
Line line1 = new Line(new Point3d(0, 0, 0), new Point3d(50, 50, 0));
Line line2 = new Line(new Point3d(50, 50, 0), new Point3d(0, 50, 0));
Line line3 = new Line(new Point3d(50, 50, 0), new Point3d(50, 0, 0));
line1.ColorIndex = 1;
line2.ColorIndex = 1;
line3.ColorIndex = 1;
tr.CurrentSpace.AddEntity(line1,line2,line3);
}
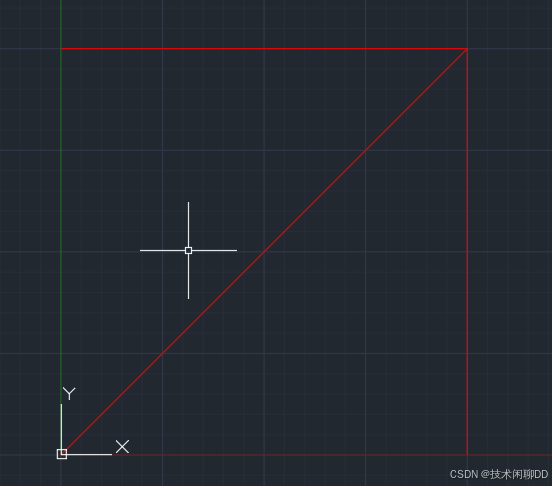
1.2 画弧
csharp
[CommandMethod(nameof(Test_Drawarc))]
public void Test_Drawarc() {
using DBTrans tr = new();
Arc arc1 = ArcEx.CreateArcSCE(new Point3d(2, 0, 0), new Point3d(0, 0, 0), new Point3d(0, 2, 0));// 起点,圆心,终点
Arc arc2 = ArcEx.CreateArc(new Point3d(4, 0, 0), new Point3d(0, 0, 0), Math.PI / 2); // 起点,圆心,弧度
Arc arc3 = ArcEx.CreateArc(new Point3d(1, 0, 0), new Point3d(0, 0, 0), new Point3d(0, 1, 0)); // 起点,圆上一点,终点
tr.CurrentSpace.AddEntity(arc1, arc2, arc3);
}
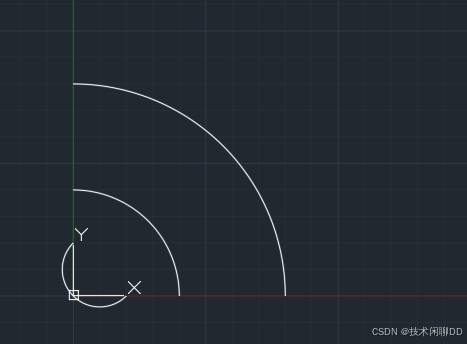
1.3 画圆
csharp
[CommandMethod(nameof(Test_DrawCircle))]
public void Test_DrawCircle()
{
using DBTrans tr = new();
var circle1 = CircleEx.CreateCircle(new Point3d(0, 0, 0), new Point3d(1, 0, 0));// 两点画圆(直径上的两个点)
var circle2 = CircleEx.CreateCircle(new Point3d(-2, 0, 0), new Point3d(2, 0, 0), new Point3d(0, 2, 0));// 三点画圆,成功
var circle3 = CircleEx.CreateCircle(new Point3d(-2, 0, 0), new Point3d(0, 0, 0), new Point3d(2, 0, 0));// 三点画圆,失败
tr.CurrentSpace.AddEntity(circle1, circle2!);
if (circle3 is not null)
tr.CurrentSpace.AddEntity(circle3);
else
Env.Printl("三点画圆失败");
}
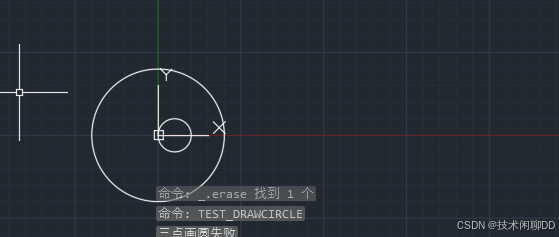
1.4 多段线的绘制
csharp
[CommandMethod(nameof(Test_AddPolyline3))]
public void Test_AddPolyline3()
{
using var tr = new DBTrans();
var pts = new List<Point3d>
{
new(0, 0, 0),
new(0, 1, 0),
new(1, 1, 0),
new(1, 0, 0)
};
var pline = pts.CreatePolyline();
tr.CurrentSpace.AddEntity(pline);
var pline1 = pts.CreatePolyline(p =>
{
p.Closed = true;
p.ConstantWidth = 0.2;
p.ColorIndex = 1;
});
tr.CurrentSpace.AddEntity(pline1);
}
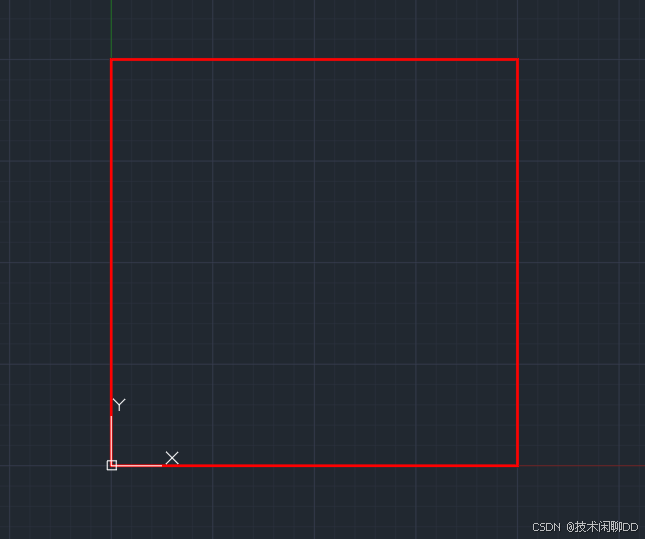
2. 图元操作
2.1 遍历图元名字
csharp
[CommandMethod(nameof(iterateEntity))]
public void iterateEntity()
{
using DBTrans tr = new DBTrans();
tr.CurrentSpace.ForEach(id =>
{
var ent = id.GetObject<Entity>();
//如果要修改图元时var entity1 = id.GetObject<Entity>(OpenMode.ForWrite);
ent?.GetRXClass().DxfName.Print();
Env.Print("\n ");
Env.Print("\nDXF 名称: " + ent?.GetRXClass().Name);
//AcDbLine,AcDbPolyline,AcDbText
Env.Print("\n图元名称: " + ent?.GetType().Name);
//Line,Polyline,DBText
Env.Print("\nObjectID: " + ent?.ToString());
Env.Print("\nHandle: " + ent?.Handle.ToString());
if (ent is Line acdbLine)//如果ent是直线,转换为直线变量cdbLine
{
Env.Print("\nacDbLinet长度为: ");
//var txt = acdbLine.GetProperty("Length");//取得直度长度属性
var txt = acdbLine.Length; // 这么写就可以了
txt.Print();
}
Env.Print("\n********************\n");
});
}
[CommandMethod(nameof(Test_PrintDxfname))]
public void Test_PrintDxfname()
{
using var tr = new DBTrans();
tr.CurrentSpace.ForEach(id => {
id.GetObject<Entity>()?.GetRXClass().DxfName.Print();
});
}
2.2 移动
源码
用法
csharp
ent.Move(pt1, pt2);
2.3 旋转
源码
csharp
ent.Rotation(new(100, 0, 0), Math.PI / 2);
2.4 镜像
源码:
用法
csharp
// 沿两点组成的线镜像
ent.Mirror(pt1, pt2);
// 沿面镜像
var plane = new Plane(Point3d.Origin, Vector3d.ZAxis);
ent.Mirror(plane);
// 沿对称点镜像
ent.Mirror(pt);
2.5 缩放
源码
用法
csharp
ent.Scale(scaleCenter,scaleValue);