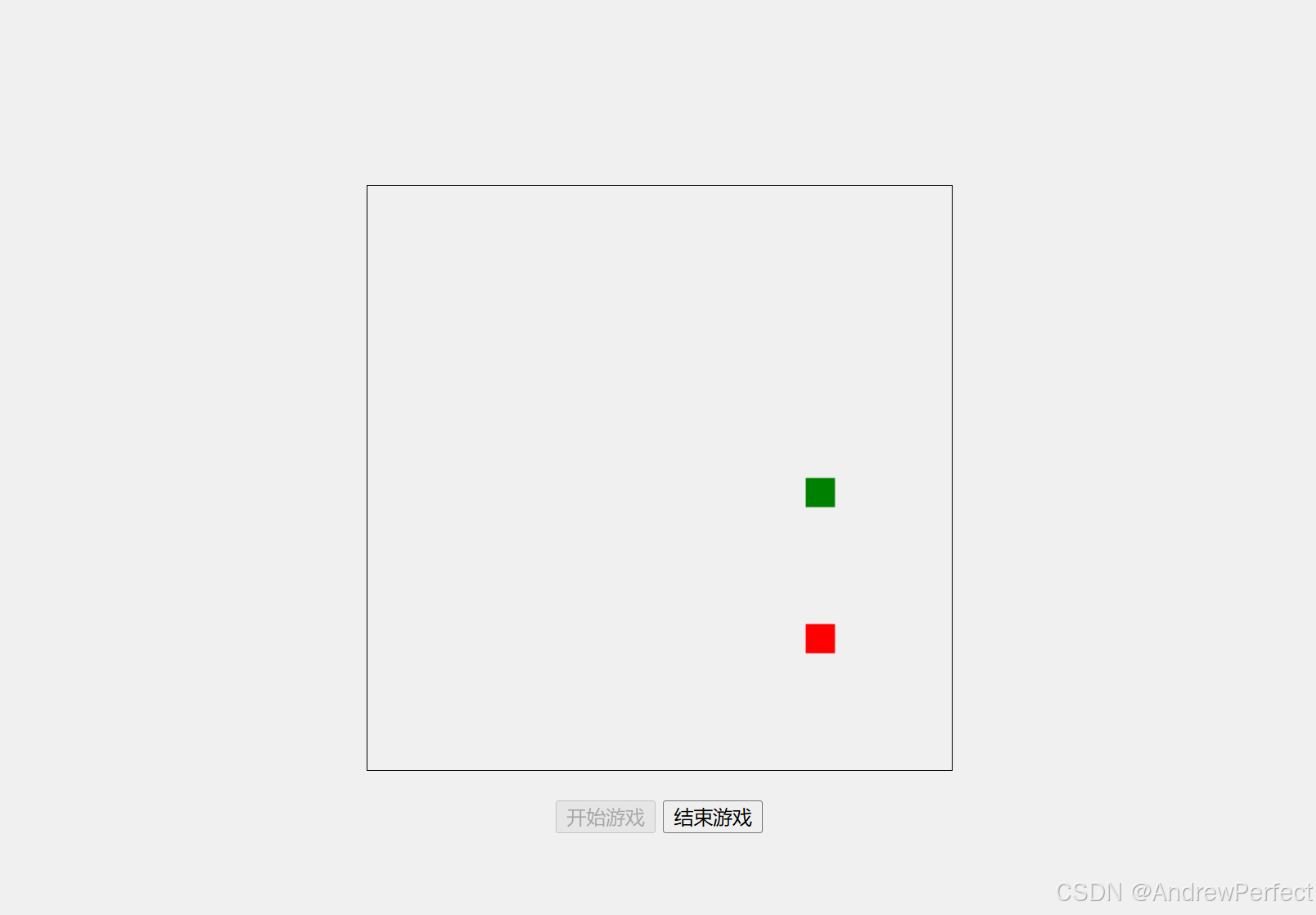
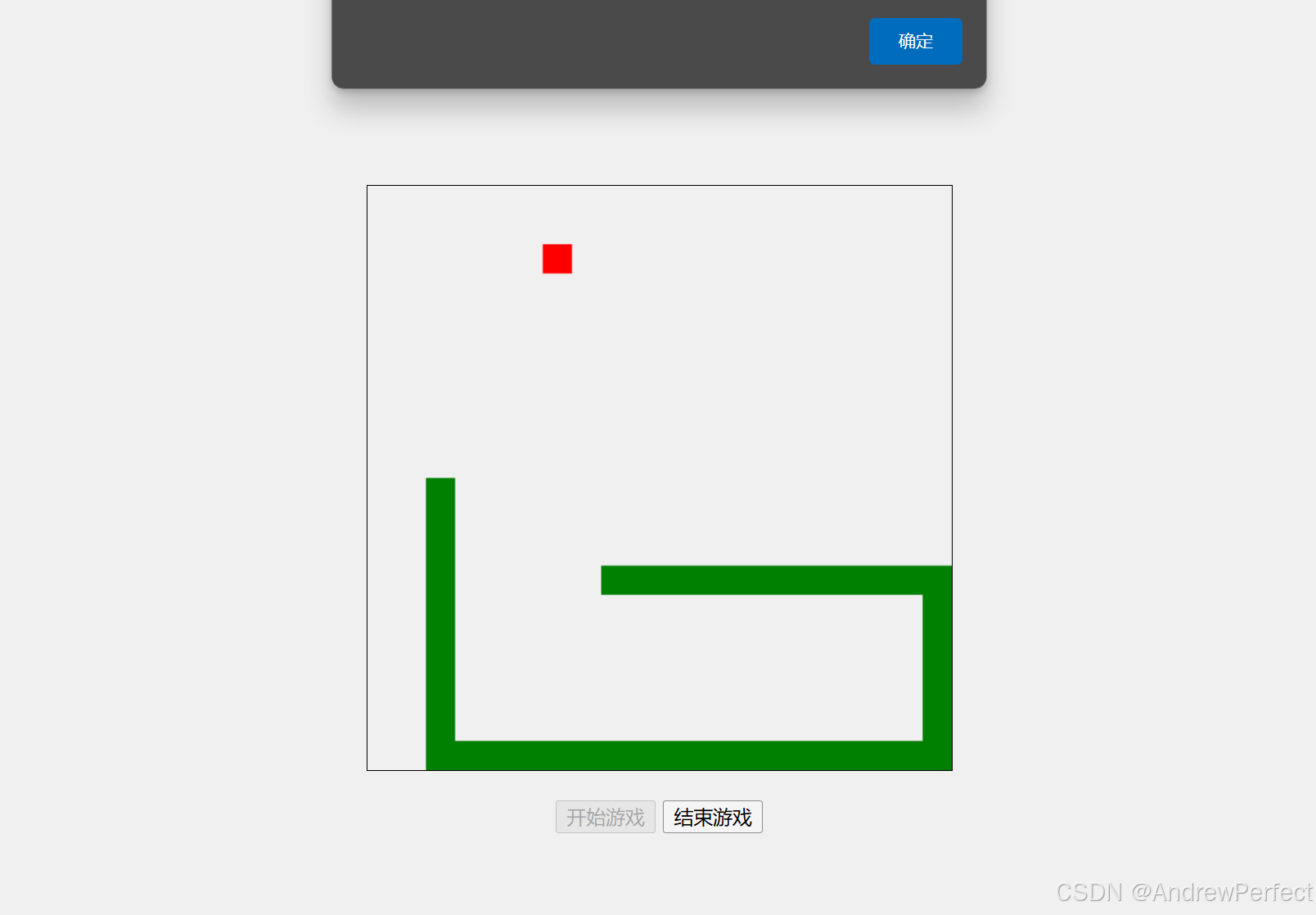
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>贪吃蛇小游戏</title>
<style>
body {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
margin: 0;
}
#gameCanvas {
border: 1px solid black;
}
.buttons {
margin-top: 20px;
}
</style>
</head>
<body>
<canvas id="gameCanvas" width="400" height="400"></canvas>
<div class="buttons">
<button id="startButton">开始游戏</button>
<button id="endButton" disabled>结束游戏</button>
</div>
<script>
// 获取画布和上下文
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const boxSize = 20;
let snake = [{x: 10, y: 10}];
let food = {x: 15, y: 15};
let dx = boxSize;
let dy = 0;
let gameLoop;
// 绘制游戏画面
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 移动蛇
let head = {x: snake[0].x + dx / boxSize, y: snake[0].y + dy / boxSize};
// 检查是否撞墙
if (head.x < 0 || head.x >= canvas.width / boxSize || head.y < 0 || head.y >= canvas.height / boxSize) {
endGame();
return;
}
// 检查是否撞到自己
if (snake.slice(1).some(segment => segment.x === head.x && segment.y === head.y)) {
endGame();
return;
}
snake.unshift(head);
// 检查是否吃到食物
if (head.x === food.x && head.y === food.y) {
food = {x: Math.floor(Math.random() * (canvas.width / boxSize)), y: Math.floor(Math.random() * (canvas.height / boxSize))};
} else {
snake.pop();
}
// 画蛇
snake.forEach(segment => {
ctx.fillStyle = 'green';
ctx.fillRect(segment.x * boxSize, segment.y * boxSize, boxSize, boxSize);
});
// 画食物
ctx.fillStyle = 'red';
ctx.fillRect(food.x * boxSize, food.y * boxSize, boxSize, boxSize);
}
// 开始游戏
function startGame() {
snake = [{x: 10, y: 10}];
food = {x: 15, y: 15};
dx = boxSize;
dy = 0;
document.getElementById('startButton').disabled = true;
document.getElementById('endButton').disabled = false;
gameLoop = setInterval(draw, 200); // 增加时间间隔以降低速度
}
// 结束游戏
function endGame() {
clearInterval(gameLoop);
alert('游戏结束');
document.getElementById('startButton').disabled = false;
document.getElementById('endButton').disabled = true;
}
document.getElementById('startButton').addEventListener('click', startGame);
document.getElementById('endButton').addEventListener('click', endGame);
document.addEventListener('keydown', (e) => {
if (e.key === 'ArrowUp' && dy === 0) {
dx = 0;
dy = -boxSize;
} else if (e.key === 'ArrowDown' && dy === 0) {
dx = 0;
dy = boxSize;
} else if (e.key === 'ArrowLeft' && dx === 0) {
dx = -boxSize;
dy = 0;
} else if (e.key === 'ArrowRight' && dx === 0) {
dx = boxSize;
dy = 0;
}
});
</script>
</body>
</html>
(AI教授的,算是自娱自乐吧。这代码还挺有意思的。)