1. 准备
编程语言:Java
JDK版本:17
Maven版本:3.6.1
2. 开始
声明:本次只测试Java的Selenium自动化功能
本次示例过程:打开谷歌游览器,进入目标网址,找到网页的输入框元素,输入指定内容,点击提交按钮,成功后关闭网页。
2.1. 目录结构和内容
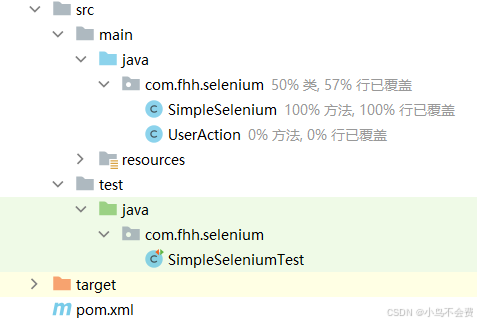
pom.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.fhh.selenium</groupId>
<artifactId>demo</artifactId>
<version>1.0.0</version>
<name>SeleniumDemo</name>
<description>Selenium demo</description>
<url/>
<licenses>
<license/>
</licenses>
<developers>
<developer/>
</developers>
<scm>
<connection/>
<developerConnection/>
<tag/>
<url/>
</scm>
<properties>
<java.version>17</java.version>
<selenium.version>4.23.1</selenium.version>
<webdrivermanager.version>5.9.2</webdrivermanager.version>
</properties>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>${selenium.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/io.github.bonigarcia/webdrivermanager -->
<!-- https://github.com/bonigarcia/webdrivermanager-->
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>${webdrivermanager.version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
SimpleSelenium.java
java
/**
* Copyright (C) 2024-2024, FHH. All rights reserved.
*/
package com.fhh.selenium;
import lombok.AllArgsConstructor;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import java.util.function.Consumer;
/**
* SimpleSelenium
*
* @apiNote <a href="https://www.selenium.dev/zh-cn/documentation/overview/">文档详情</a>
* @since 1.0.0
*/
@AllArgsConstructor
public class SimpleSelenium {
private final WebDriver driver;
public SimpleSelenium openWeb() {
driver.get("https://www.selenium.dev/selenium/web/web-form.html");
return this;
}
public SimpleSelenium getWebTitle(Consumer<? super String> action) {
action.accept(driver.getTitle());
return this;
}
public SimpleSelenium fillForm() {
WebElement textBox = driver.findElement(By.name("my-text"));
// 设置textBox的值为"Test selenium"
textBox.clear();
textBox.sendKeys("Test selenium");
return this;
}
public SimpleSelenium submit() {
WebElement submitButton = driver.findElement(By.cssSelector("button"));
submitButton.click();
return this;
}
public SimpleSelenium getMessage(Consumer<? super String> action) {
WebElement message = driver.findElement(By.id("message"));
action.accept(message.getText());
return this;
}
public void quit() {
driver.quit();
}
}
UserAction.java
封装用户操作的示例
java
/**
* Copyright (C) 2024-2024, FHH. All rights reserved.
*/
package com.fhh.selenium;
import lombok.AllArgsConstructor;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
/**
* 封装一些用户的必要动作
*
* @since 1.0.0
*/
@AllArgsConstructor
public class UserAction {
private final WebDriver driver;
/**
* 登录用户
*
* @param username 用户名
* @param password 密码
* @return this
*/
public UserAction login(String username, String password) {
WebElement loginField = driver.findElement(By.id("username"));
loginField.clear();
loginField.sendKeys(username);
// Fill out the password field. The locator we're using is "By.id", and we should
// have it defined elsewhere in the class.
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.clear();
passwordField.sendKeys(password);
// Click the login button, which happens to have the id "submit".
driver.findElement(By.id("submit")).click();
return this;
}
/**
* 注销登录
*
* @return this
*/
public UserAction logOut() {
WebElement logOutButton = driver.findElement(By.id("log-out"));
logOutButton.click();
return this;
}
}
SimpleSeleniumTest.java
java
/**
* Copyright (C) 2024-2024, FHH. All rights reserved.
*/
package com.fhh.selenium;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.time.Duration;
import static org.junit.jupiter.api.Assertions.assertEquals;
class SimpleSeleniumTest {
WebDriver driver;
@BeforeEach
public void setup() {
driver = new ChromeDriver();
}
@Test
public void simpleTest() {
// 1. 打开网页
driver.manage().timeouts().implicitlyWait(Duration.ofMillis(500));
driver.get("https://www.selenium.dev/selenium/web/web-form.html");
// 2. 获取网页标题,并断言为Web form
String title = driver.getTitle();
assertEquals("Web form", title);
// 3. 找到网页上name属性为"my-text"的元素textBox
WebElement textBox = driver.findElement(By.name("my-text"));
// 3.1 设置textBox的值为Selenium
textBox.sendKeys("Test selenium");
// sleep(2000);
// 4. 点击提交元素
WebElement submitButton = driver.findElement(By.cssSelector("button"));
submitButton.click();
// sleep(2000);
// 5. 断言页面名称为"Web form - target page"
assertEquals("Web form - target page", driver.getTitle());
// sleep(2000);
// 6. 找到id为"message"的消息,断言为"Received!"
WebElement message = driver.findElement(By.id("message"));
assertEquals("Received!", message.getText());
}
@Test
public void simpleSeleniumTest() {
new SimpleSelenium(driver)
.openWeb()
.getWebTitle(title -> assertEquals("Web form", title))
.fillForm()
.submit()
.getWebTitle(title -> assertEquals("Web form - target page", title))
.getMessage(message -> assertEquals("Received!", message))
.quit();
}
@AfterEach
public void teardown() {
driver.quit();
}
public void sleep(long timeMillis) {
try {
Thread.sleep(timeMillis);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
3. 效果
运行测试后,会先等待1~5秒(具体看机器性能),打开一个谷歌游览器,在输入网址,进入网页,并找到网页中的元素,输入内容后,点击提交按钮,网页会自动跳转到新的页面。结束
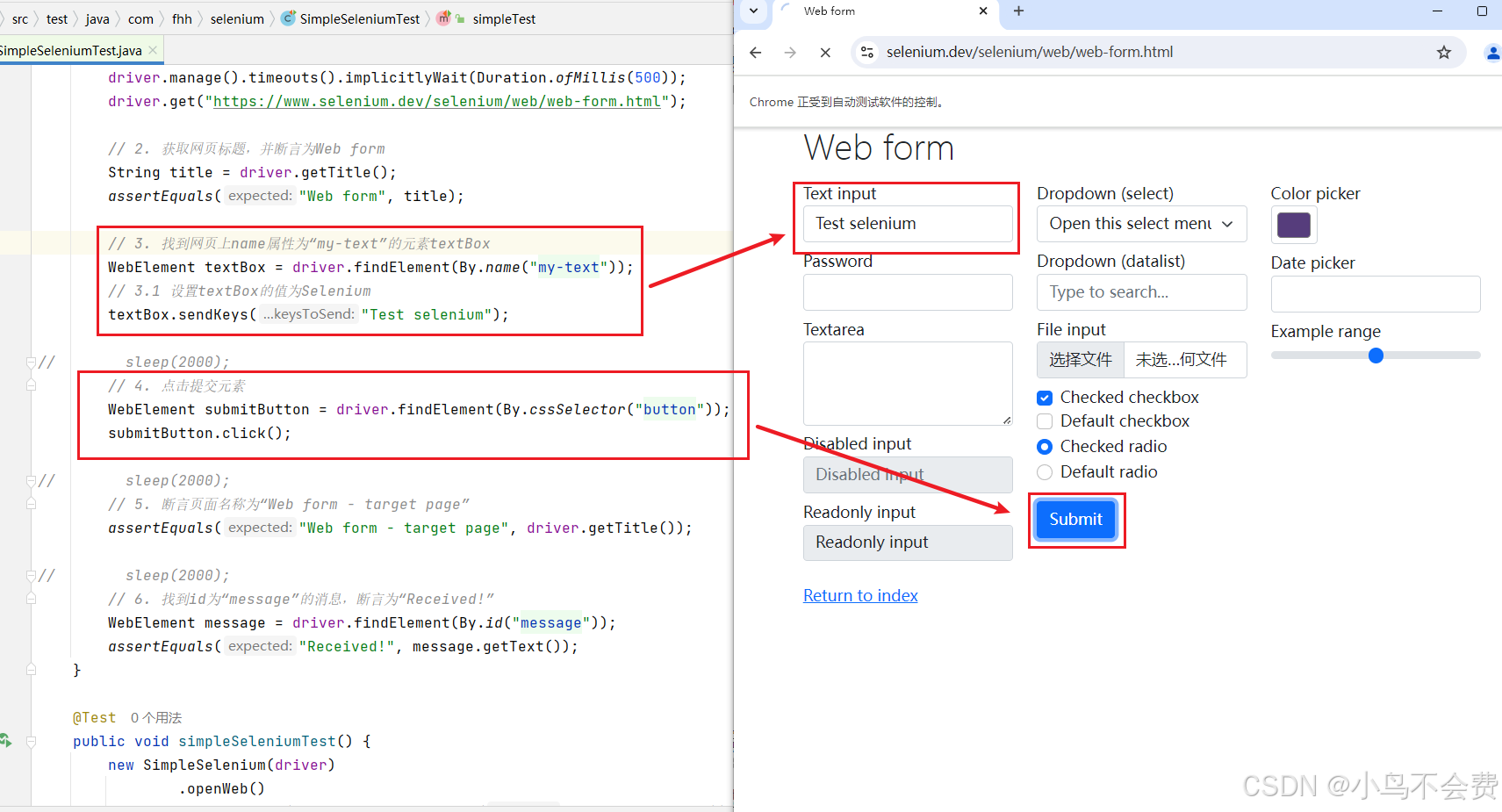
测试用例结果
如果对你有用,就点个赞吧~多谢