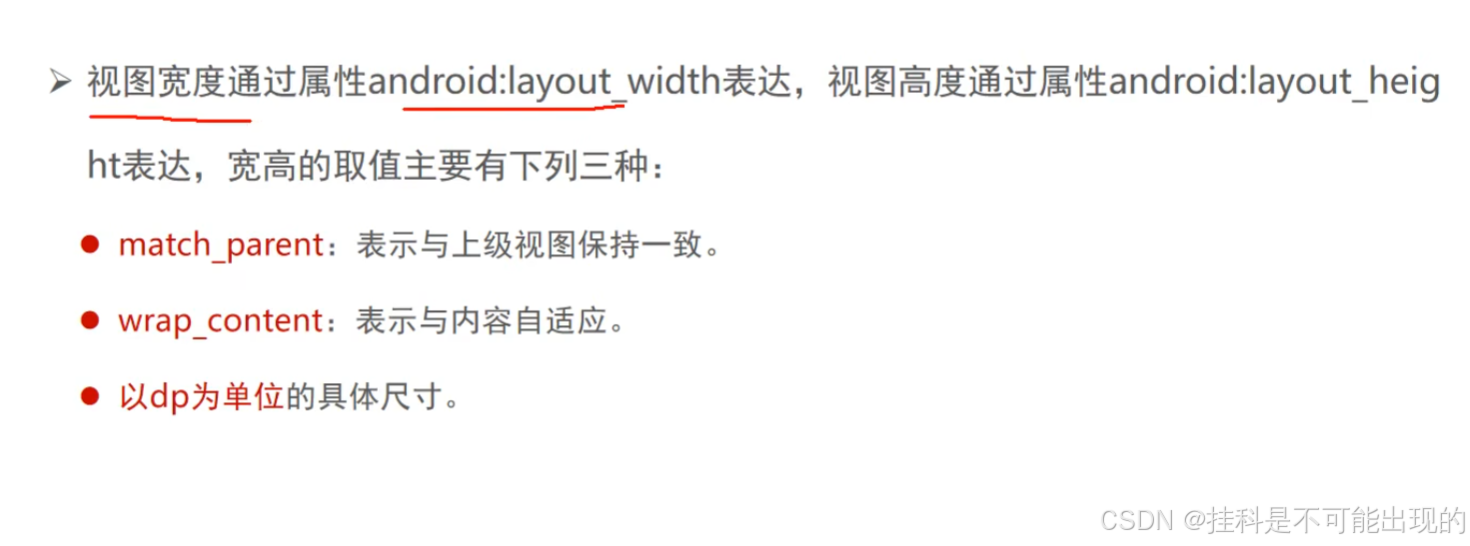
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MyApplication">
<activity
android:name=".ViewBorderActivity"
android:exported="false" />
<activity
android:name=".TextSizeActivity"
android:exported="true" />
</application>
</manifest>
activity_view_border.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="视图宽高采用wrap_content定义"
android:textColor="#000000"
android:textSize="17sp" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="视图宽高采用match_parent定义"
android:textColor="#000000"
android:textSize="17sp" />
<TextView
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="视图宽度采用固定大小"
android:textColor="#000000"
android:textSize="17sp" />
<TextView
android:id="@+id/tv_code"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="通过代码指定视图宽度"
android:textColor="#000000"
android:textSize="17sp" />
</LinearLayout>
ViewBorderActivity.java
package com.example.chapter03;
import android.os.Bundle;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
import com.example.chapter03.util.Utils;
public class ViewBorderActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_view_border);
TextView tv_code = findViewById(R.id.tv_code);
//获取tv_code的布局参数(含宽度和高度)
ViewGroup.LayoutParams params = tv_code.getLayoutParams();
//修改布局参数中的宽度数值,注意默认px单位,需要吧dp数值转成px数值
params.width = Utils.dip2px(this,300);
//设置tv_code的布局参数
tv_code.setLayoutParams(params);
}
}
Utils.java
package com.example.chapter03.util;
import android.content.Context;
public class Utils {
public static int dip2px(Context context, float dpValue){
//获取当前手机的像素密度(1个dp对应几个px)
float scale =context.getResources().getDisplayMetrics().density;
return (int)(dpValue*scale + 0.5f);
}
}