一、前言
需求: Qt开发Android程序过程中,点击按钮就打开一个PPT文件。
Qt在Windows上要打开PPT文件或者其他文件很容易。可以使用QDesktopServices
打开文件,非常方便。QDesktopServices
提供了静态接口调用系统级别的功能。
这里用的QDesktopServices
是 Qt 框架中的一个类,用于在跨平台应用程序中方便地访问和使用主机操作系统的桌面服务和功能。该类提供了一些静态方法,用于打开网址、文件和邮件客户端等系统默认应用程序。它的主要目的是让开发者能够轻松调用系统级别的功能,而不需要直接编写与操作系统相关的代码。
代码如下:
QUrl url = QUrl::fromLocalFile("storage/emulated/0/Download/UseHelp.ppt");
bool IsOK = QDesktopServices::openUrl(url);
下面是QDesktopServices
的帮助文档介绍。
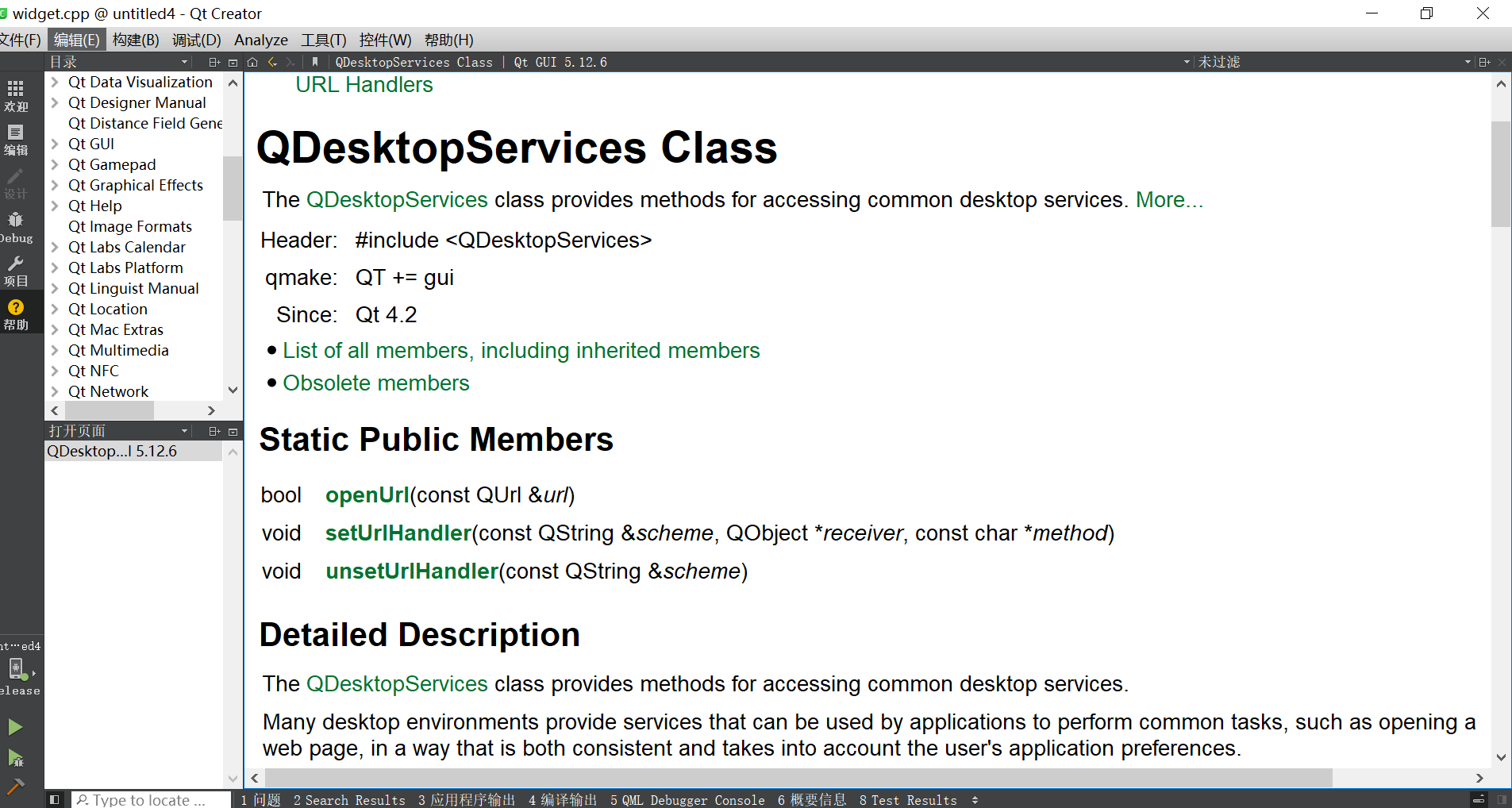
QDesktopServices
只适用于桌面应用程序。 如果在Linux系统下想要打开PPT文件,也可以采用QDesktopServices
来实现。
前提也是需要先安装LibreOffice
或OpenOffice
才可以。
在Qt的文档里也提供了openUrl
静态方法的使用说明。
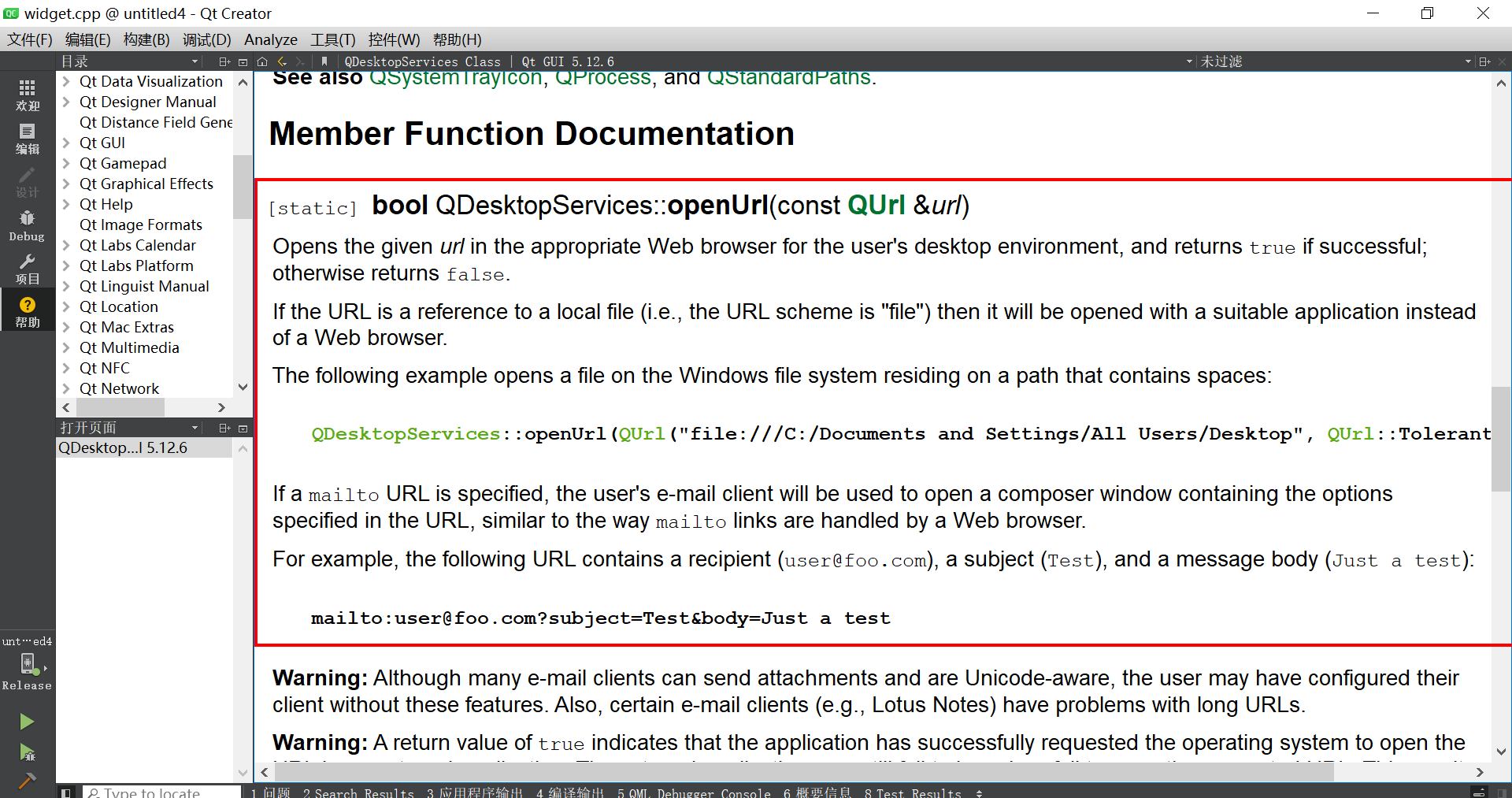
使用 QDesktopServices::openUrl
在Linux系统上打开PPT文件。
#include <QDesktopServices>
#include <QUrl>
#include <QDebug>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QString pptFilePath = "/path/to/your/presentation.ppt";
QUrl pptUrl = QUrl::fromLocalFile(pptFilePath);
if (!QDesktopServices::openUrl(pptUrl)) {
qWarning() << "Failed to open PPT file.";
}
return app.exec();
}
但是在Android移动平台上,这个办法就行不通了。 需要通过JNI接口与Android系统交互,才可以完成系统级别的操作。
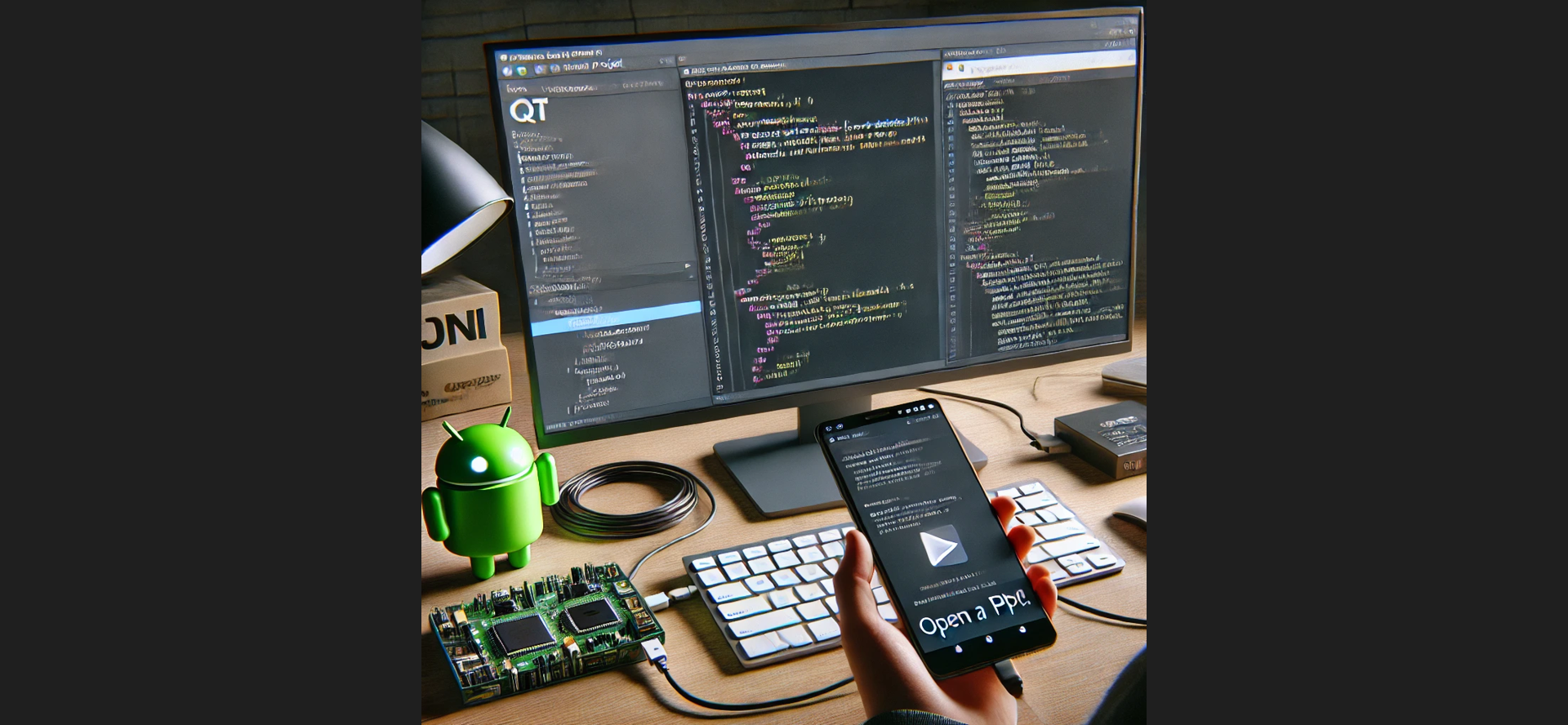
二、通过JNI与Android系统交互
在Qt for Android中,可以通过Qt提供的Java Native Interface (JNI)与Android系统交互,可以调用系统功能,打开PPT文件。
下面演示如何在Qt中使用C++代码,通过JNI调用Android的Intent来打开PPT文件。
2.1 操作步骤
-
在Qt项目中添加Android相关的权限和活动声明:
- 在
AndroidManifest.xml
文件中添加必要的权限和活动声明。
- 在
-
使用Qt的JNI接口调用Android Intent:
- 在C++代码中使用Qt的JNI接口来调用Android的Intent。
2.2 代码
(1)AndroidManifest.xml
在AndroidManifest.xml
文件中添加以下权限声明,确保应用有权读取文件:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.qtproject.example">
<application android:label="@string/app_name">
<activity android:name="org.qtproject.qt5.android.bindings.QtActivity"
android:configChanges="orientation|screenSize"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
</application>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
</manifest>
(2)Qt C++代码
在Qt C++代码中使用JNI接口调用Android的Intent来打开PPT文件。
#include <QCoreApplication>
#include <QAndroidJniObject>
#include <QtAndroid>
#include <QDebug>
void openPPTFile(const QString &filePath) {
QAndroidJniObject activity = QAndroidJniObject::callStaticObjectMethod("org/qtproject/qt5/android/QtNative", "activity", "()Landroid/app/Activity;");
if (activity.isValid()) {
QAndroidJniObject intent("android/content/Intent", "()V");
intent.callObjectMethod("setAction", "(Ljava/lang/String;)Landroid/content/Intent;", QAndroidJniObject::fromString("android.intent.action.VIEW").object<jstring>());
QAndroidJniObject uri = QAndroidJniObject::callStaticObjectMethod(
"android/net/Uri",
"parse",
"(Ljava/lang/String;)Landroid/net/Uri;",
QAndroidJniObject::fromString(filePath).object<jstring>());
intent.callObjectMethod("setDataAndType", "(Landroid/net/Uri;Ljava/lang/String;)Landroid/content/Intent;", uri.object<jobject>(), QAndroidJniObject::fromString("application/vnd.ms-powerpoint").object<jstring>());
intent.callObjectMethod("addFlags", "(I)Landroid/content/Intent;", 0x10000000); // Intent.FLAG_ACTIVITY_NEW_TASK
activity.callMethod<void>("startActivity", "(Landroid/content/Intent;)V", intent.object<jobject>());
} else {
qDebug() << "Failed to get the activity.";
}
}
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
QString pptFilePath = "/sdcard/Download/sample.pptx"; // 指定要打开的PPT文件路径
openPPTFile(pptFilePath);
return app.exec();
}
2.3 代码说明
-
openPPTFile
函数:该函数接受PPT文件的路径作为参数,并使用JNI接口调用Android的Intent来打开该文件。 -
QAndroidJniObject
:用于与Java对象和方法交互。 -
setAction
和setDataAndType
方法:设置Intent的操作和数据类型。 -
startActivity
方法:启动Intent以打开PPT文件。
2.4 编译和运行
需要先在Android设备上安装合适的PPT查看应用(比如:WPS),并且PPT文件路径正确。编译和运行Qt项目时,应用将通过系统默认的应用打开指定的PPT文件。