十三、文章分类页面 - [element-plus 表格]
Git仓库:https://gitee.com/msyycn/vue3-hei-ma.git
基本架子 - PageContainer
功能需求说明:
- 基本架子-PageContainer封装
- 文章分类渲染 & loading处理
- 文章分类添加编辑[element-plus弹层]
- 文章分类删除
- 基本结构样式,用到了
el-card
组件
jsx
<template>
<el-card class="page-container">
<template #header>
<div class="header">
<span>文章分类</span>
<div class="extra">
<el-button type="primary">添加分类</el-button>
</div>
</div>
</template>
...
</el-card>
</template>
<style lang="scss" scoped>
.page-container {
min-height: 100%;
box-sizing: border-box;
.header {
display: flex;
align-items: center;
justify-content: space-between;
}
}
</style>
- 考虑到多个页面复用,封装成组件
props
定制标题(父传子)- 默认插槽
default
定制内容主体 - 具名插槽
extra
定制头部右侧额外的按钮
jsx
<script setup>
defineProps({
title: {
required: true,
type: String
}
})
</script>
<template>
<el-card class="page-container">
<template #header>
<div class="header">
<span>{{ title }}</span>
<div class="extra">
<slot name="extra"></slot>
</div>
</div>
</template>
<slot></slot>
</el-card>
</template>
<style lang="scss" scoped>
.page-container {
min-height: 100%;
box-sizing: border-box;
.header {
display: flex;
align-items: center;
justify-content: space-between;
}
}
</style>
- 页面中直接使用测试 ( unplugin-vue-components 会自动注册)
- 文章分类测试:
jsx
<template>
<page-container title="文章分类">
<template #extra>
<el-button type="primary"> 添加分类 </el-button>
</template>
主体部分
</page-container>
</template>
- 文章管理测试:
jsx
<template>
<page-container title="文章管理">
<template #extra>
<el-button type="primary">发布文章</el-button>
</template>
主体部分
</page-container>
</template>
视图预览
文章分类渲染
封装API - 请求获取表格数据
- 新建
api/article.js
封装获取频道列表的接口
jsx
import request from '@/utils/request'
export const artGetChannelsService = () => request.get('/my/cate/list')
- 页面中调用接口,获取数据存储
jsx
const channelList = ref([])
const getChannelList = async () => {
const res = await artGetChannelsService()
channelList.value = res.data.data
}
ArticleChannel.vue
vue
<script setup>
import { artGetChannelsService } from '@/api/article'
import {ref} from 'vue'
const channelList = ref([]) // 文章分类列表
// 获取文章分类列表
const getChannelList = async () => {
const res = await artGetChannelsService()
channelList.value = res.data.data
console.log('文章分类列表',channelList.value);
}
// 调用获取文章分类列表
getChannelList()
</script>
<template>
<div>
<!-- 按需引入 -->
<page-container title="文章分类">
<!-- 右侧按钮 - 添加文章 - 具名插槽 -->
<template #extra>
<el-button>添加分类</el-button>
</template>
主体部分--表格
</page-container>
</div>
</template>
<style lang="scss" scoped>
</style>
视图预览
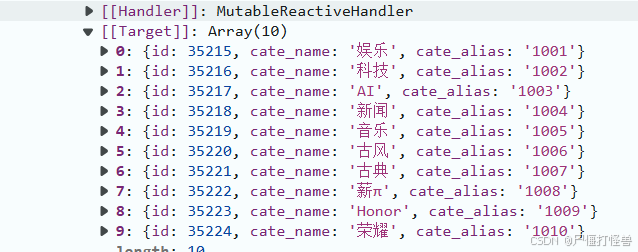
el-table 表格动态渲染
jsx
<el-table :data="channelList" style="width: 100%">
<el-table-column label="序号" width="100" type="index"> </el-table-column>
<el-table-column label="分类名称" prop="cate_name"></el-table-column>
<el-table-column label="分类别名" prop="cate_alias"></el-table-column>
<el-table-column label="操作" width="100">
<template #default="{ row }">
<el-button
:icon="Edit"
circle
plain
type="primary"
@click="onEditChannel(row)"
></el-button>
<el-button
:icon="Delete"
circle
plain
type="danger"
@click="onDelChannel(row)"
></el-button>
</template>
</el-table-column>
<template #empty>
<el-empty description="没有数据" />
</template>
</el-table>
const onEditChannel = (row) => {
console.log(row)
}
const onDelChannel = (row) => {
console.log(row)
}
预览视图
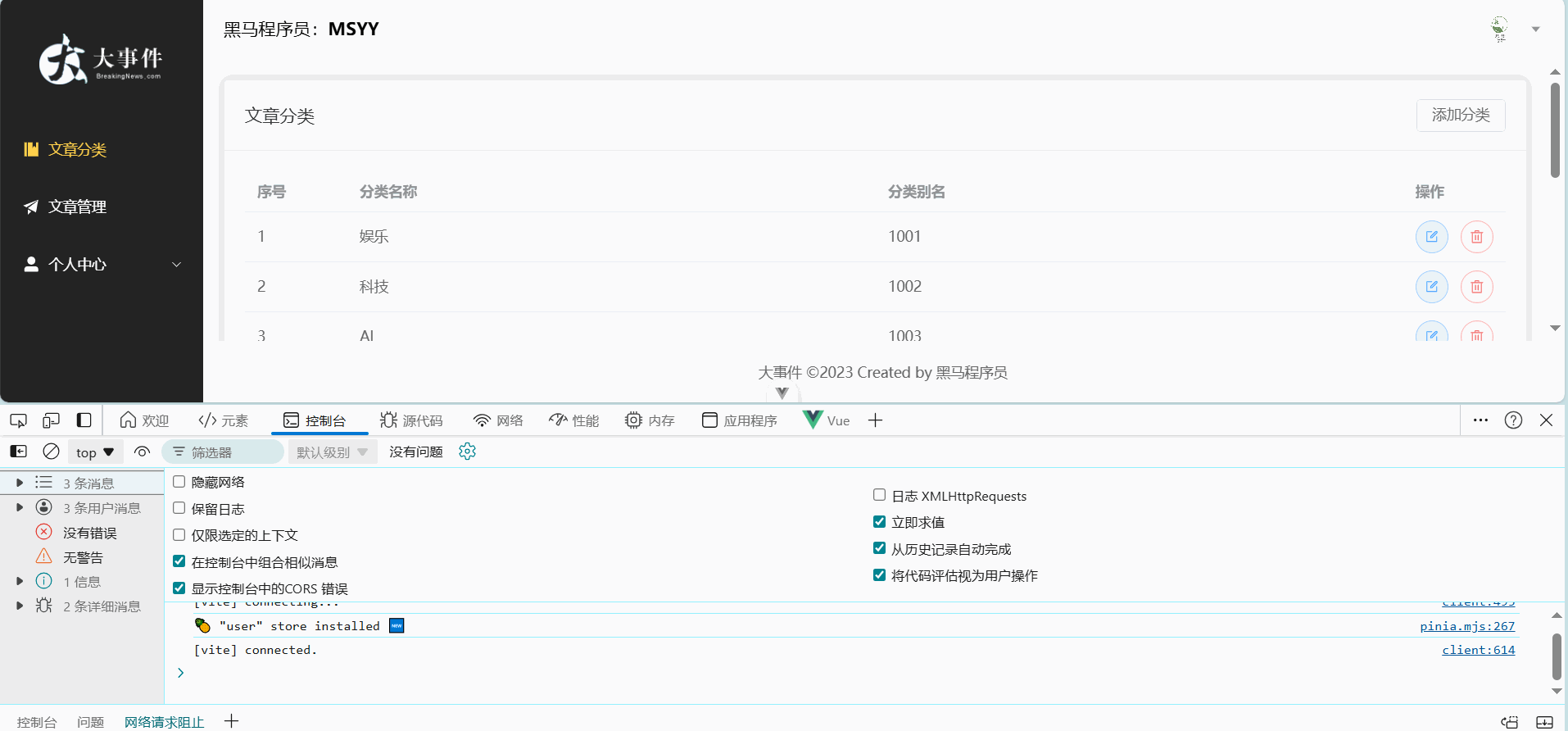
el-table 表格 loading 效果
- 定义变量,v-loading绑定
jsx
const loading = ref(false)
<el-table v-loading="loading">
- 发送请求前开启,请求结束关闭
jsx
const getChannelList = async () => {
loading.value = true
const res = await artGetChannelsService()
channelList.value = res.data.data
loading.value = false
}
ArticleChannel.vue
vue
<script setup>
import { artGetChannelsService } from '@/api/article'
import { Delete,Edit } from '@element-plus/icons-vue'
import {ref} from 'vue'
const channelList = ref([]) // 文章分类列表
const loading = ref(false) //加载状态
// 获取文章分类列表
const getChannelList = async () => {
// 发请求之前先将loading设置为true
loading.value = true
// 调用接口
const res = await artGetChannelsService()
channelList.value = res.data.data
// 发完请求,关闭loading
loading.value = false
// console.log('文章分类列表',channelList.value);
}
// 调用获取文章分类列表
getChannelList()
// 编辑文章分类
const onEditChannel =(row,$index) =>{
console.log(row,$index)
}
// 删除文章分类
const onDelChannel =(row,$index)=>{
console.log(row,$index)
}
</script>
<template>
<div>
<!-- 按需引入 -->
<page-container title="文章分类">
<!-- 右侧按钮 - 添加文章 - 具名插槽 -->
<template #extra>
<el-button>添加分类</el-button>
</template>
<!-- 主体部分--表格 -->
<el-table :data="channelList" style="width: 100%" v-loading="loading">
<el-table-column label="序号" type="index" width="100" ></el-table-column>
<el-table-column label="分类名称" prop="cate_name" ></el-table-column>
<el-table-column label="分类别名" prop="cate_alias" ></el-table-column>
<el-table-column label="操作" width="100">
<template #default="{row,$index}">
<el-button @click="onEditChannel(row,$index)" plain :icon="Edit" circle type="primary" ></el-button>
<el-button @click="onDelChannel(row,$index)" plain :icon="Delete" circle type="danger" ></el-button>
</template>
</el-table-column>
</el-table>
</page-container>
</div>
</template>
<style lang="scss" scoped>
</style>
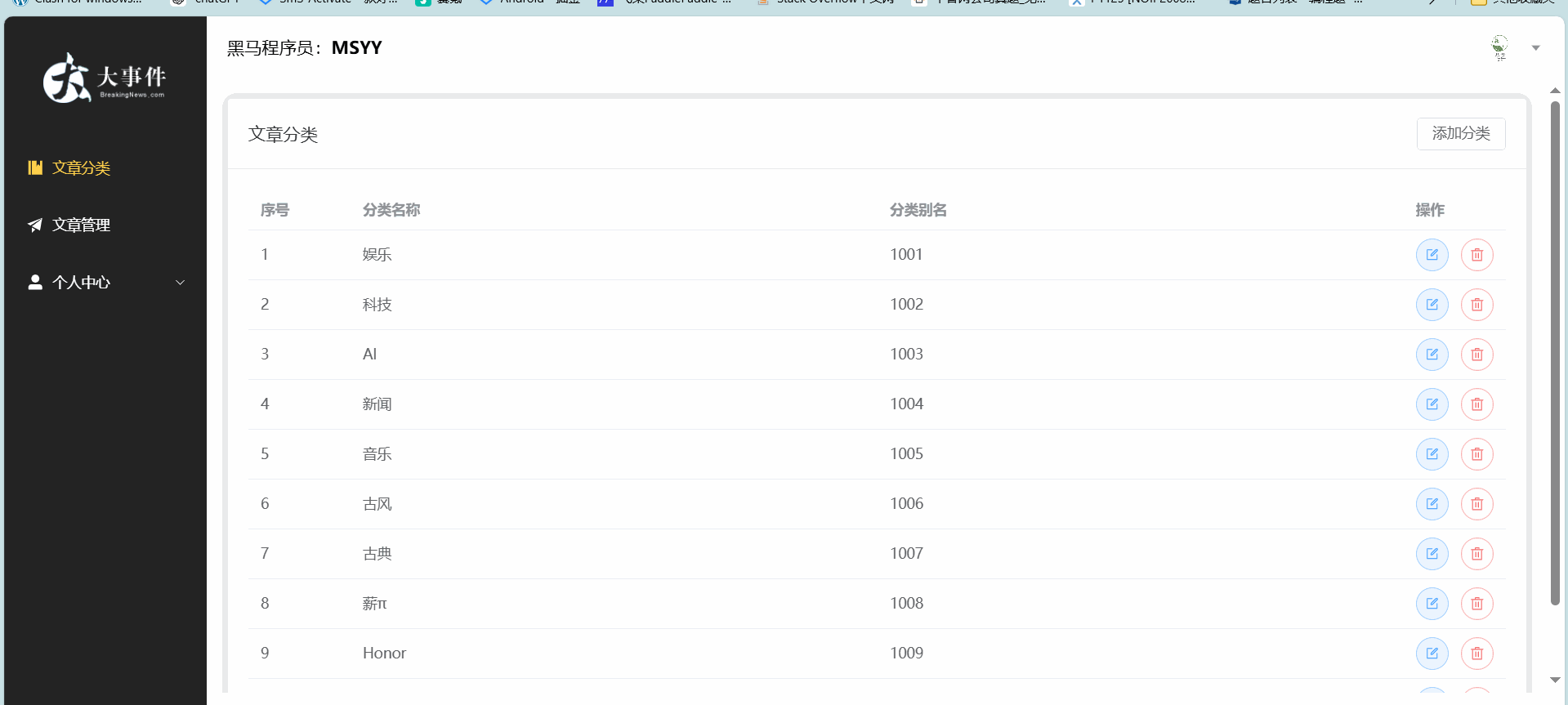
请求到的数组为空时
vue
channelList.value = []

添加element-plus插槽:
vue
<!-- 主体部分--表格 -->
<el-table :data="channelList" style="width: 100%" v-loading="loading">
<el-table-column label="序号" type="index" width="100" ></el-table-column>
<el-table-column label="分类名称" prop="cate_name" ></el-table-column>
<el-table-column label="分类别名" prop="cate_alias" ></el-table-column>
<el-table-column label="操作" width="100">
<template #default="{row,$index}">
<el-button @click="onEditChannel(row,$index)" plain :icon="Edit" circle type="primary" ></el-button>
<el-button @click="onDelChannel(row,$index)" plain :icon="Delete" circle type="danger" ></el-button>
</template>
</el-table-column>
<!-- 没有数据 -->
<template #empty>
<el-empty description="暂无数据"></el-empty>
</template>
</el-table>
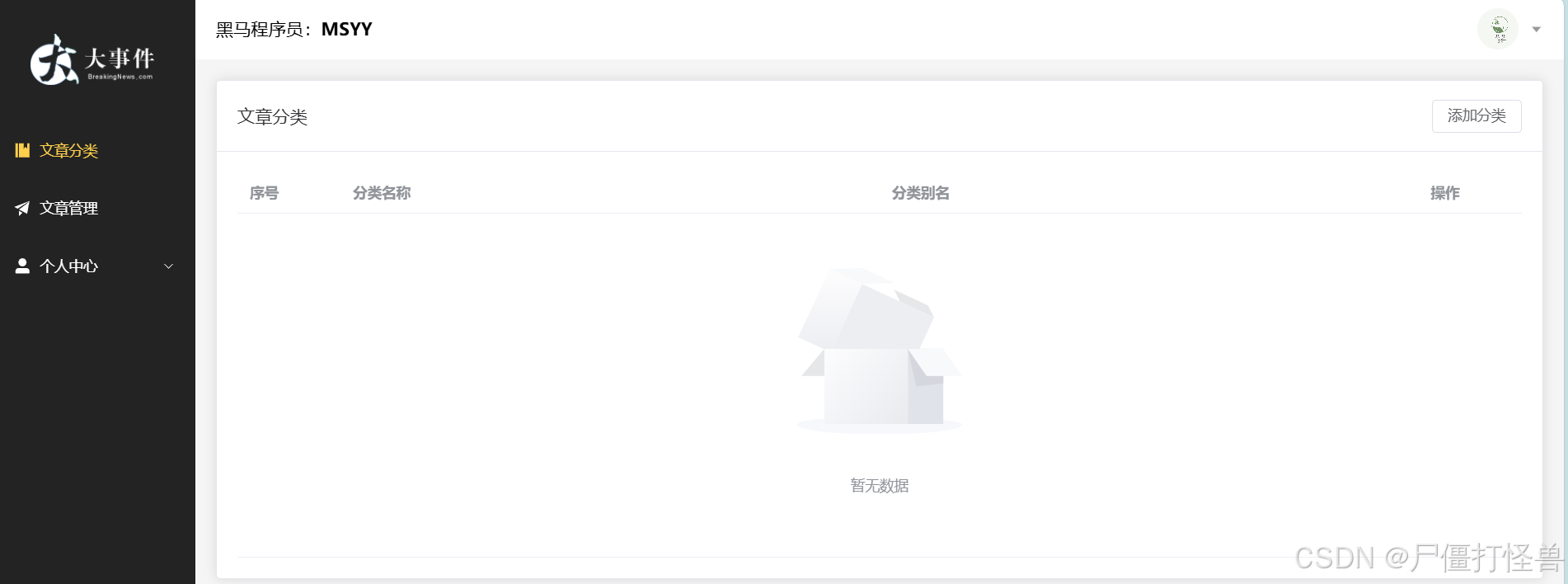
下期见!