文章目录
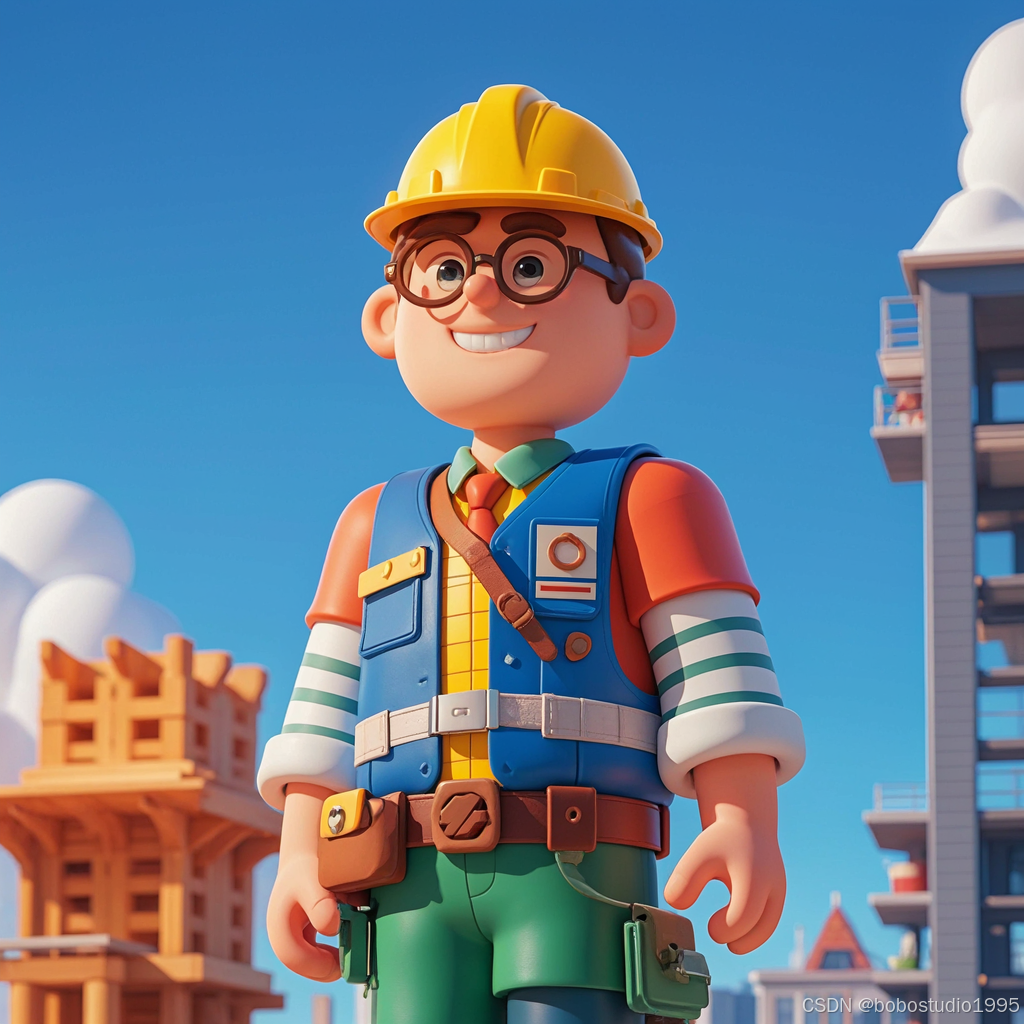
建造者模式:打造你的梦想之屋
假设你想要一栋完美的房子,里面有舒适的卧室、明亮的客厅、现代化的厨房,还有一个漂亮的花园。如果你自己来建,可能会手忙脚乱,不知从何下手。这就像是在代码中创建一个复杂的对象,如果所有细节都塞进一个超长的构造函数里,或者散布在代码的各个角落,那简直就是一场噩梦! 建造者模式就像是一位神奇的建筑大师,能够按照你的心意,一步步打造出你梦想中的房子。
建造者的秘密
建造者模式就像是聘请了一位专业的建筑师。他会把建房子的过程分解成一系列简单的步骤,比如打地基、砌墙、安装屋顶等。这样,你就不用亲自处理所有繁琐的细节了。
建造者有什么利与害?
建造者模式就像搭积木一样,可以一块一块慢慢来,不急于一时,用同样的建造步骤,可以盖出不同风格的房子,建筑师专注于建造,你只需要告诉他你想要什么样的房子就行。缺点就是需要雇佣更多的工人(增加更多的类),使得整个工程看起来更复杂。
如何使用建造者搭建各种房子
建房涉及角色
-
建筑师(Builder):就像一份建房合同,列出了建造房子需要的所有步骤
-
不同风格的建筑师,例如哥特式建筑师,罗马建筑师(GothicBuilder,RomanBuilder):每种风格的建造方法都不同
-
工程总监(Director):负责协调整个建造过程,确保房子按照正确的顺序建造
建房步骤
-
建筑师负责设计建造房子的步骤
-
邀请不同建造风格的建筑师
-
工程总监指挥不同风格的建筑师建造房子
-
不同风格的房子建造完成
选择合适的建造者,你就能轻松打造出心目中的完美之屋!
代码实现案例
typescript
// 建筑师
interface Builder {
// 重置房子
reset(): void;
// 建造墙
buildWall(): void;
// 建造门
buildDoor(): void;
// 建造窗户
buildWindow(): void;
// 建造屋顶
buildRoof(): void;
// 建造花园
buildGarden(): void;
// 得到房子
getHouse(): void;
}
// 哥特式建筑师
class GothicBuilder implements Builder {
// 重置房子
reset(): void {
console.log("Resetting Gothic House");
}
// 建造哥特式墙
buildWall(): void {
console.log("Building Gothic Wall");
}
// 建造哥特式门
buildDoor(): void {
console.log("Building Gothic Door");
}
// 建造哥特式窗
buildWindow(): void {
console.log("Building Gothic Window");
}
// 建造哥特式屋顶
buildRoof(): void {
console.log("Building Gothic Roof");
}
// 建造哥特式花园
buildGarden(): void {
console.log("Building Gothic Garden");
}
// 获取哥特式房子
getHouse(): void {
console.log("Gothic House");
}
}
// 罗马建筑师
class RomanBuilder implements Builder {
// 重置房子
reset(): void {
console.log("Resetting Roman House");
}
// 建造罗马墙
buildWall(): void {
console.log("Building Roman Wall");
}
// 建造罗马门
buildDoor(): void {
console.log("Building Roman Door");
}
// 建造罗马窗
buildWindow(): void {
console.log("Building Roman Window");
}
// 建造罗马屋顶
buildRoof(): void {
console.log("Building Roman Roof");
}
// 建造罗马花园
buildGarden(): void {
console.log("Building Roman Garden");
}
// 获取罗马房子
getHouse(): void {
console.log("Roman House");
}
}
// 工程总监
class Director {
private builder: Builder | null = null;
// 设置建筑师风格
setBuilder(builder: Builder): void {
this.builder = builder;
}
// 建造房子
make(builder: Builder): void {
if (this.builder) {
this.builder.reset();
this.builder.buildWall();
this.builder.buildDoor();
this.builder.buildWindow();
this.builder.buildRoof();
this.builder.buildGarden();
}
}
}
// 工程总监
const director = new Director();
// 哥特式建筑风格
const gothicBuilder = new GothicBuilder();
// 罗马建筑风格
const romanBuilder = new RomanBuilder();
// 哥特式房子
director.setBuilder(gothicBuilder);
director.make(gothicBuilder); // 输出 Building Gothic Wall Building Gothic Door Building Gothic Window Building Gothic Roof Building Gothic Garden
gothicBuilder.getHouse(); // 输出 Gothic House
// 罗马房子
director.setBuilder(romanBuilder);
director.make(romanBuilder); // 输出 Resetting Roman House Building Roman Wall Building Roman Door Building Roman Window Building Roman Roof Building Roman Garden
romanBuilder.getHouse(); // 输出 Roman House
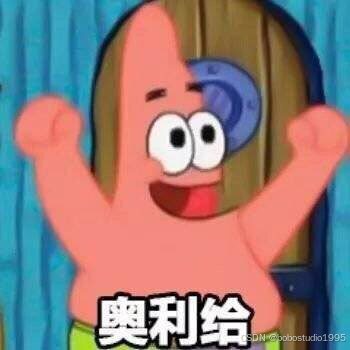
建造者模式的主要优点
-
封装性好,建造者模式将复杂对象的构建过程封装起来,客户端不需要了解具体的构建细节。
-
扩展性强,增加新的具体建造者,容易扩展新的产品类型。
-
控制细节,能够控制产品的构建过程,从而保证产品的质量。
-
易于使用,用清晰的方式来创建复杂对象,客户端代码更加简洁明。
建造者模式的主要缺点
-
需要创建多个具体建造者风格类,这样会增加系统的复杂度。
-
建造者模式需要客户端代码与具体建造者风格类进行交互,这增加了系统的耦合度。
建造者模式的适用场景
-
遇到创建复杂对象时,使用建造者模式来简化对象的创建过程。
-
需要创建的对象具有多个部分组成时,使用建造者模式来创建对象。
总结
建造者模式是一种创建型设计模式,将复杂对象的构建过程分解为多个简单步骤,代码更加简洁明了,它封装性好、扩展性强、易于控制细节,但也需要创建多个具体建造者类、增加系统的复杂度和耦合度。合理使用建造者模式,可以提高代码的可维护性和可扩展性。
喜欢的话就点个赞 ❤️,关注一下吧,有问题也欢迎讨论指教。感谢大家!!!
下期预告:TypeScript 设计模式之【单例】