目录
[1. 安装pytest和Selenium](#1. 安装pytest和Selenium)
[2. 编写测试用例](#2. 编写测试用例)
[3. 运行测试](#3. 运行测试)
[1. 编写测试类](#1. 编写测试类)
[2. 运行测试](#2. 运行测试)
[1. 使用Page Object模式](#1. 使用Page Object模式)
[2. 合理利用等待机制](#2. 合理利用等待机制)
[3. 跨浏览器测试](#3. 跨浏览器测试)
[4. 编写清晰的测试报告](#4. 编写清晰的测试报告)
引言
随着软件开发的日益复杂,自动化测试在软件开发周期中的重要性愈发凸显。自动化测试不仅提高了测试效率,还确保了软件质量的一致性和可重复性。Selenium作为Web自动化测试领域的佼佼者,以其强大的跨浏览器支持和灵活的API设计,在自动化测试领域占据了重要地位。本文将详细探讨如何将Selenium集成到两种常见的Python测试框架------pytest和unittest中,并通过丰富的案例和代码示例,帮助新手朋友快速上手。
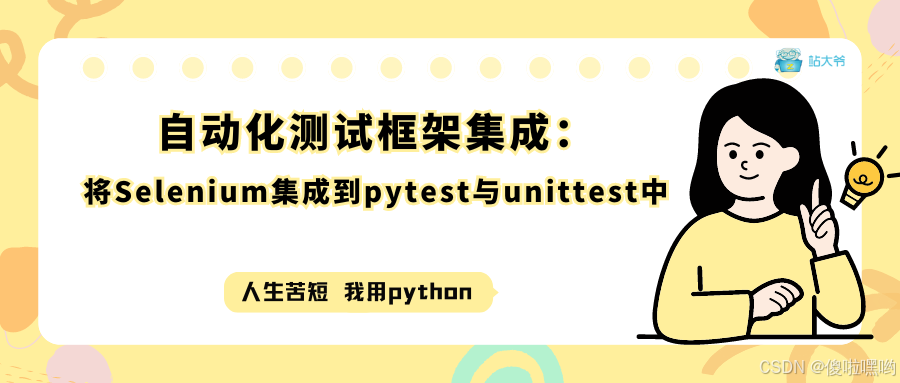
一、Selenium简介
Selenium是一个用于Web应用程序自动化测试的工具集,它直接运行在浏览器中,模拟用户的操作行为,如点击、输入、导航等。Selenium支持多种浏览器和平台,包括Chrome、Firefox、Edge、Safari等,且支持多种编程语言,如Java、Python、C#等。Selenium主要由几个部分组成:
- Selenium IDE:一个用于记录、编辑和调试测试用例的图形界面工具。
- Selenium WebDriver:提供了一套API,允许开发者编写代码来控制浏览器。
- Selenium Grid:支持分布式测试用例执行,可以将测试用例分布到不同的测试机器上执行。
- Selenium WebDriver API 概览
- Selenium WebDriver API提供了丰富的功能,用于定位页面元素、模拟用户输入、处理弹窗、执行JavaScript代码等。
以下是一些常用的API示例:
python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
# 初始化WebDriver
driver = webdriver.Chrome()
# 打开网页
driver.get("http://www.example.com")
# 定位元素并输入文本
search_box = driver.find_element(By.ID, "search-box")
search_box.send_keys("Selenium")
# 模拟点击搜索按钮
search_button = driver.find_element(By.ID, "search-button")
search_button.click()
# 执行JavaScript代码
driver.execute_script("alert('Hello, Selenium!');")
# 关闭浏览器
driver.quit()
二、Selenium与pytest的集成
pytest是Python的一个非常流行的测试框架,以其简单、灵活和可扩展性著称。将Selenium集成到pytest中,可以使Web自动化测试更加高效和灵活。
1. 安装pytest和Selenium
首先,需要确保安装了pytest和Selenium。可以通过pip安装:
bash
pip install pytest selenium
2. 编写测试用例
使用pytest编写Selenium测试用例时,通常会在测试文件中定义多个测试函数,每个函数代表一个测试用例。每个测试函数都会接收一个fixture作为参数,该fixture负责初始化WebDriver并管理其生命周期。
示例:百度搜索测试
python
# test_baidu.py
import pytest
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# 定义WebDriver fixture
@pytest.fixture(scope="function")
def driver():
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("--headless") # 无头模式运行
driver = webdriver.Chrome(options=chrome_options)
yield driver
driver.quit()
def test_baidu_search(driver):
driver.get("https://www.baidu.com")
search_box = driver.find_element(By.ID, "kw")
search_box.send_keys("Selenium")
search_button = driver.find_element(By.ID, "su")
search_button.click()
# 等待搜索结果加载
WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "result-op"))
)
# 验证搜索结果
assert "Selenium" in driver.title
# 运行测试
# pytest test_baidu.py
3. 运行测试
使用pytest命令运行测试文件,pytest会自动发现并执行所有以test_开头的函数。
bash
pytest test_baidu.py
三、Selenium与unittest的集成
unittest是Python自带的单元测试框架,虽然不如pytest灵活,但在一些项目中仍然被广泛使用。将Selenium集成到unittest中,可以实现Web自动化测试的基本需求。
1. 编写测试类
在unittest中,测试通常以测试类的形式组织,每个测试方法都以test_开头。测试类需要继承自unittest.TestCase。
示例:百度搜索测试
python
# test_baidu_unittest.py
import unittest
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
class TestBaidu(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_baidu_search(self):
self.driver.get("https://www.baidu.com")
search_box = self.driver.find_element(By.ID, "kw")
search_box.send_keys("Selenium")
search_button = self.driver.find_element(By.ID, "su")
search_button.click()
# 等待搜索结果加载
WebDriverWait(self.driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "result-op"))
)
# 验证搜索结果
self.assertIn("Selenium", self.driver.title)
def tearDown(self):
self.driver.quit()
if __name__ == '__main__':
unittest.main()
2. 运行测试
使用unittest自带的unittest.main()函数运行测试。
bash
python test_baidu_unittest.py
四、Selenium自动化测试的最佳实践
1. 使用Page Object模式
Page Object模式是一种将页面上的元素和操作封装成一个类的设计模式。这样做的好处是可以减少代码重复,提高测试的可维护性。例如,可以将百度首页的搜索功能封装成一个类,然后在多个测试用例中重复使用。
python
# baidu_page.py
from selenium.webdriver.common.by import By
class BaiduPage:
def __init__(self, driver):
self.driver = driver
def search(self, keyword):
search_box = self.driver.find_element(By.ID, "kw")
search_box.send_keys(keyword)
search_button = self.driver.find_element(By.ID, "su")
search_button.click()
# test_baidu_with_page_object.py
import unittest
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from baidu_page import BaiduPage
class TestBaiduWithPageObject(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.baidu_page = BaiduPage(self.driver)
def test_baidu_search(self):
self.baidu_page.search("Selenium")
WebDriverWait(self.driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "result-op"))
)
self.assertIn("Selenium", self.driver.title)
def tearDown(self):
self.driver.quit()
if __name__ == '__main__':
unittest.main()
2. 合理利用等待机制
在Web自动化测试中,经常需要等待页面元素加载完成后再进行操作。Selenium提供了显示等待(Explicit Wait)和隐式等待(Implicit Wait)两种机制。显示等待可以根据具体的条件等待元素,更加灵活;隐式等待则是在整个WebDriver的生命周期内设置等待时间,较为简单但不够灵活。
3. 跨浏览器测试
Selenium支持多种浏览器,可以通过修改WebDriver的初始化代码来实现跨浏览器测试。例如,通过修改webdriver.Chrome()为webdriver.Firefox(),即可在Firefox浏览器中运行测试。
4. 编写清晰的测试报告
测试报告是测试结果的重要展示形式。pytest和unittest都支持生成测试报告,但有时候可能需要更详细的HTML报告。可以使用HTMLTestRunner(一个第三方库)来生成HTML格式的测试报告。
bash
pip install HTMLTestRunner
然后,在unittest的测试脚本中添加生成HTML报告的代码。
五、总结
Selenium作为Web自动化测试的强大工具,与pytest和unittest等测试框架的集成,可以极大地提升Web应用的测试效率和测试质量。通过合理的测试设计、Page Object模式的应用、等待机制的使用以及跨浏览器测试,可以构建出稳定、可靠的自动化测试体系。希望本文的介绍和示例能够帮助新手朋友快速入门Selenium自动化测试,并在实际项目中灵活运用。