1. 为什么要写写单例模式基类
用面向对象的思想避免代码冗余(多余、重复)
2. 实现不继承MonoBehaviour的单例模式基类
单例模式的基类
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/// <summary>
/// 单例模式基类,主要目的是避免代码的冗余,方便实现单例模式的类
/// </summary>
/// <typeparam name="T"></typeparam>
//where约束T必须是class,还有有一个公共的无参构造函数
public class BaseManager<T> where T : class,new()
{
public static T instance;
// 属性的方式
public static T Instance
{
get
{
if(instance == null)
{
instance = new T();
}
return instance;
}
}
// 方法的方式
public static T GetInstance()
{
if(instance == null)
{
instance = new T();
}
return instance;
}
}
当其他类需要实现单例时候,直接继承基类
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TestMgr : BaseManager<TestMgr>
{
public void TestLog()
{
Debug.Log("Test");
}
}
好处就是可以避免代码冗余,不用重复定义单例,需要单例直接继承就行
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TestMgr2 : BaseManager<TestMgr2>
{
public void TestLog()
{
Debug.Log("test2");
}
}
测试
csharp
public class Main : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
TestMgr.Instance.TestLog();
TestMgr2.Instance.TestLog();
}
// Update is called once per frame
void Update()
{
}
}
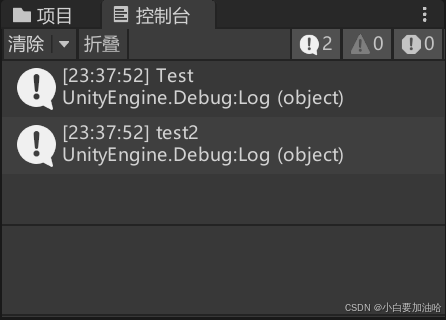
3. 潜在的安全问题
1.构造函数问题:构造函数可在外部调用 可能会破坏唯一性
比如csharp TestMgr t = new TestMgr();
不能new一个单例模式的对象,可以通过反射解决
2.多线程问题:当多个线程同时访问管理器时,可能会出现共享资源的安全访问问题