在App内实现直接预览pdf文件,而不是通过调用第三方软件,如WPS office等打开pdf。
主要思路:通过PhotoView将pdf读取为图片流进行展示。
一、首先,获取对本地文件读取的权限
在AndrooidManifest.xml中声明权限,以及页面中动态获取权限。
二、在build.gradle中引入需要的包
XML
implementation 'com.github.chrisbanes:PhotoView:2.0.0'
三、在项目库地址中添加
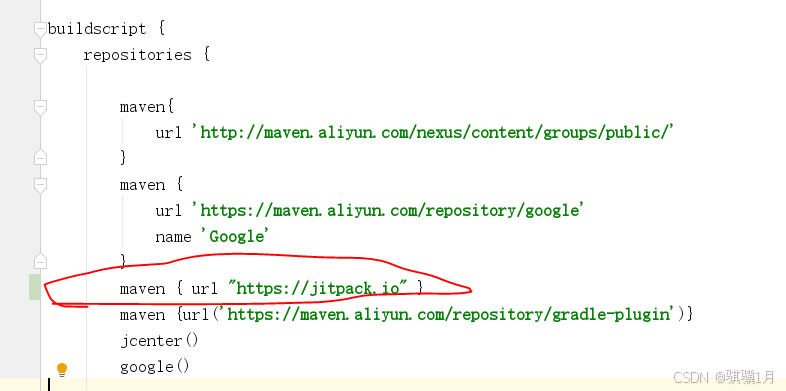
以及:
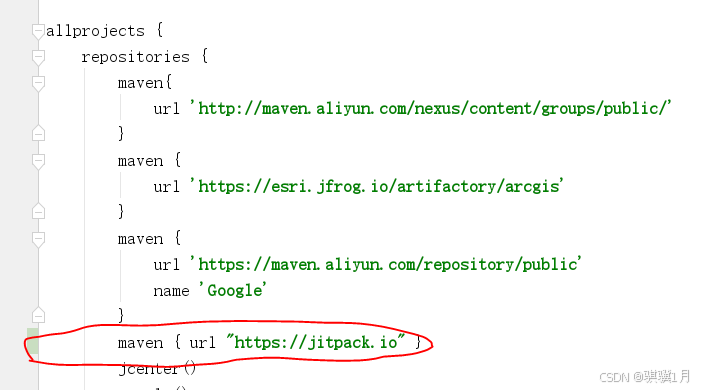
四、添加页面布局文件
添加一个页面布局文件:activity_pdf.xml
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.v4.view.ViewPager
android:id="@+id/vp_pdf"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</android.support.v4.view.ViewPager>
</LinearLayout >
添加一个适配器布局:item_pdf.xml
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<com.github.chrisbanes.photoview.PhotoView
android:id="@+id/iv_pdf"
android:layout_width="match_parent"
android:layout_height="match_parent">
</com.github.chrisbanes.photoview.PhotoView>
</LinearLayout>
五、打开和关闭的实现代码
java
public class PdfActivity extends AppCompatActivity {
LayoutInflater mInflater;
PdfRenderer mRenderer;
ViewPager vpPdf;
ParcelFileDescriptor mDescriptor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_pdf);
init();
}
public void init() {
mInflater = LayoutInflater.from(this);
vpPdf =findViewById(R.id.vp_pdf);
try {
openRender();
} catch (IOException e) {
e.printStackTrace();
}
}
@TargetApi(Build.VERSION_CODES.LOLLIPOP)
private void openRender() throws IOException {
File file = new File(DataHelper.GetHelpPdfPath());
//初始化PdfRender
mDescriptor = ParcelFileDescriptor.open(file, ParcelFileDescriptor.MODE_READ_ONLY);
if (mDescriptor != null) {
mRenderer = new PdfRenderer(mDescriptor);
}
//初始化ViewPager的适配器并绑定
MyAdapter adapter = new MyAdapter();
vpPdf.setAdapter(adapter);
}
class MyAdapter extends PagerAdapter {
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
@Override
public int getCount() {
return mRenderer.getPageCount();
}
@Override
public boolean isViewFromObject(View view, Object object) {
return view==object;
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
@Override
public Object instantiateItem(ViewGroup container, int position) {
View view = mInflater.inflate(R.layout.item_pdf, null);
PhotoView pvPdf = view.findViewById(R.id.iv_pdf);
pvPdf.setEnabled(true);
if (getCount() <= position) {
return view;
}
PdfRenderer.Page currentPage = mRenderer.openPage(position);
Bitmap bitmap = Bitmap.createBitmap(1080, 1760, Bitmap.Config.ARGB_8888);
currentPage.render(bitmap, null, null, PdfRenderer.Page.RENDER_MODE_FOR_DISPLAY);
pvPdf.setImageBitmap(bitmap);
//关闭当前Page对象
currentPage.close();
container.addView(view);
return view;
}
@Override
public void destroyItem(ViewGroup container, int position, Object object) {
//销毁需要销毁的视图
container.removeView((View) object);
}
}
//关闭pdf
@Override
protected void onDestroy() {
super.onDestroy();
try{
closeRenderer();
}
catch (Exception ex){
ex.printStackTrace();
}
}
//关闭pdf
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
private void closeRenderer() throws IOException {
if (mRenderer != null){
mRenderer.close();
}
if (mDescriptor != null){
mDescriptor.close();
}
}
}