QFile 是 Qt 框架中用于文件操作的类,提供了丰富的功能来处理文件的读写、复制、删除、重命名等操作。以下是一些常见的 QFile 操作示例,包括打开文件、读取文件、写入文件、复制文件、删除文件等。
QFile 提供了丰富的文件操作功能,包括打开、读取、写入、复制、删除、重命名和获取文件信息等。通过这些操作,开发者可以方便地处理文件,满足各种应用需求。
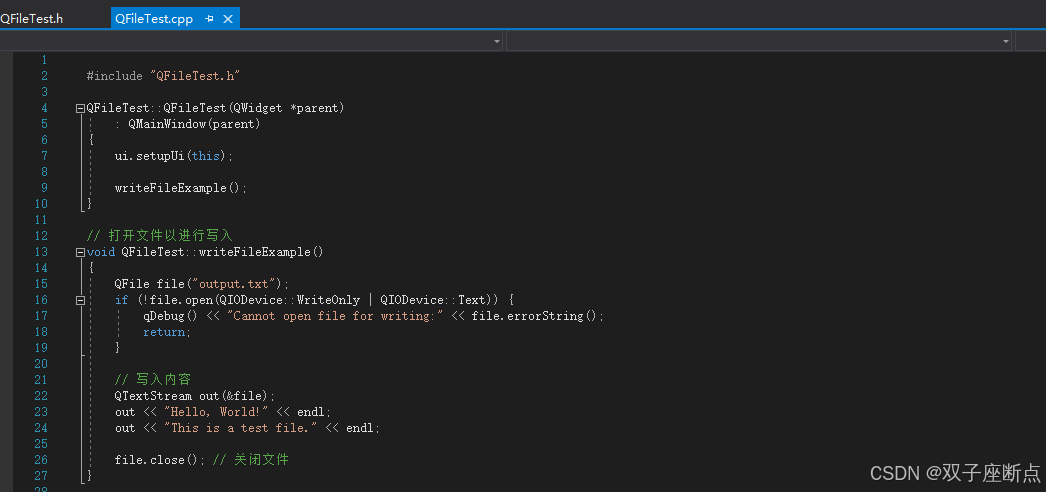
++转载请附上文章出处与本文链接。++
QFile 使用详解目录
[1 写文件](#1 写文件)
[2 读文件](#2 读文件)
[3 追加内容](#3 追加内容)
[4 复制文件](#4 复制文件)
[5 删除文件](#5 删除文件)
[6 重命名文件](#6 重命名文件)
[7 检查文件](#7 检查文件)
[8 获取文件信息](#8 获取文件信息)
9选择文件
[10 专栏文章](#10 专栏文章)
1 写文件
可以使用 QFile 将数据写入文件。
cpp
void QFileTest::writeFileExample()
{
QFile file("output.txt");
if (!file.open(QIODevice::WriteOnly | QIODevice::Text)) {
qDebug() << "Cannot open file for writing:" << file.errorString();
return;
}
// 写入内容
QTextStream out(&file);
out << "Hello, World!" << endl;
out << "This is a test file." << endl;
file.close(); // 关闭文件
}
2 读文件
可以使用 QFile 获取数据文件。
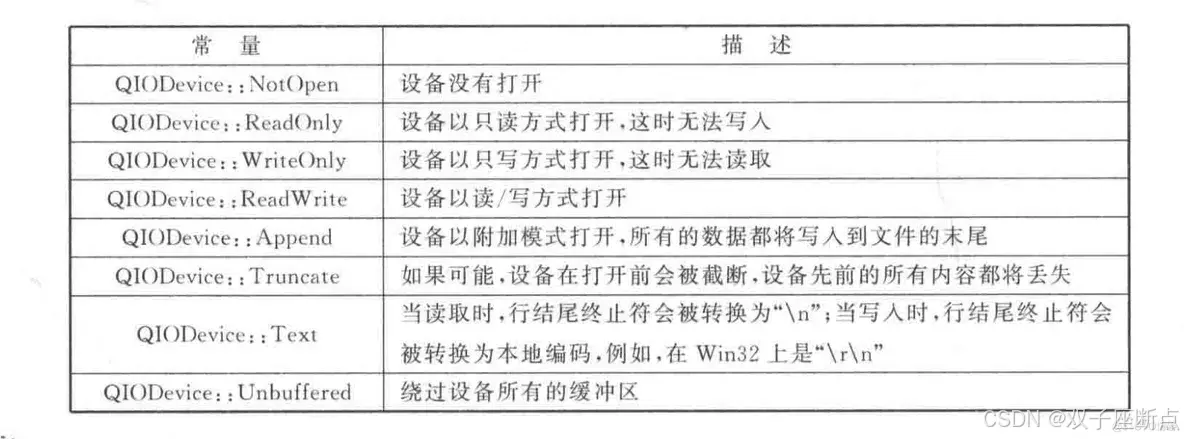
cpp
void QFileTest::openFileExample()
{
QFile file("example.txt");
if (!file.open(QIODevice::ReadOnly | QIODevice::Text))
{
qDebug() << "Cannot open file for reading:" << file.errorString();
return;
}
// 读取文件内容
QTextStream in(&file);
while (!in.atEnd())
{
QString line = in.readLine();
qDebug() << line;
}
file.close(); // 关闭文件
}
3 追加内容
可以将数据追加到现有文件的末尾。
cpp
// 打开文件以进行追加
void QFileTest::appendToFileExample()
{
QFile file("output.txt");
if (!file.open(QIODevice::Append | QIODevice::Text))
{
qDebug() << "Cannot open file for appending:" << file.errorString();
return;
}
// 追加内容
QTextStream out(&file);
out << "Appending this line to the file." << endl;
file.close(); // 关闭文件
}
4 复制文件
使用 QFile::copy() 方法可以复制文件
cpp
void QFileTest::copyFileExample()
{
QString sourceFile = "output.txt";
QString destFile = "copy_of_output.txt";
if (QFile::copy(sourceFile, destFile))
{
qDebug() << "File copied successfully.";
}
else {
qDebug() << "Failed to copy file:";//<< QFile::errorString();
}
}
5 删除文件
使用 QFile::remove() 方法可以删除文件。
cpp
void QFileTest::deleteFileExample()
{
QFile file("output.txt");
if (file.remove())
{
qDebug() << "File deleted successfully.";
}
else {
qDebug() << "Failed to delete file:" << file.errorString();
}
}
6 重命名文件
使用 QFile::rename() 方法可以重命名文件。
cpp
void QFileTest::renameFileExample()
{
QString oldName = "copy_of_output.txt";
QString newName = "renamed_output.txt";
if (QFile::rename(oldName, newName))
{
qDebug() << "File renamed successfully.";
}
else {
qDebug() << "Failed to rename file:";// << QFile::errorString();
}
}
7 检查文件
可以使用 QFile::exists() 方法检查文件是否存在。
cpp
void QFileTest::checkFileExistsExample()
{
QString fileName = "output.txt";
if (QFile::exists(fileName))
{
qDebug() << "File exists.";
}
else {
qDebug() << "File does not exist.";
}
}
8 获取文件信息
使用 QFileInfo 类可以获取文件的详细信息。
cpp
void QFileTest::fileInfoExample()
{
QFile file("output.txt");
QFileInfo fileInfo(file);
if (fileInfo.exists())
{
qDebug() << "File Name:" << fileInfo.fileName();
qDebug() << "File Size:" << fileInfo.size() << "bytes";
qDebug() << "File Path:" << fileInfo.absoluteFilePath();
qDebug() << "Last Modified:" << fileInfo.lastModified();
}
else {
qDebug() << "File does not exist.";
}
}
9 文件选择
cpp
QString folder = QFileDialog::getExistingDirectory(this, "选择文件夹", QString(),
QFileDialog::ShowDirsOnly | QFileDialog::DontResolveSymlinks);
if (!folder.isEmpty())
{
qDebug() << folder;
}
else
{
return;
}
//选择文件 QFileDialog::getOpenFileName() 方法
10 专栏文章
QT基本类使用详解系列文章包括以下内容: