该工程为在保存时执行开发的功能,函数入口点ufput。其他还有新建、打开、另存等都可以加入开发的操作,具体看UF_EXIT下的介绍。
用户出口是一个可选特性,允许你在NX中某些预定义的位置(或出口)自动运行Open C API程序。如果你进入其中一个出口,NX会检查你是否定义了指向Open C API程序位置的指针。如果定义了指针,NX将运行Open C API程序。指针是一个环境变量。
注意:
一定要设置环境变量指向自己生成的DLL。例如:USER_FILE=E:\workspace\Infore\tcnx_project\application\tcnx_project.dll
// Mandatory UF Includes
#include <uf.h>
#include <uf_object_types.h>
#include <uf_draw.h>
#include <uf_part.h>
// Internal+External Includes
#include <NXOpen/Annotations.hxx>
#include <NXOpen/Assemblies_Component.hxx>
#include <NXOpen/Assemblies_ComponentAssembly.hxx>
#include <NXOpen/Body.hxx>
#include <NXOpen/BodyCollection.hxx>
#include <NXOpen/Face.hxx>
#include <NXOpen/Line.hxx>
#include <NXOpen/NXException.hxx>
#include <NXOpen/NXObject.hxx>
#include <NXOpen/Part.hxx>
#include <NXOpen/PartCollection.hxx>
#include <NXOpen/Session.hxx>
#include <NXOpen/PrintPDFBuilder.hxx>
#include <NXOpen/PlotManager.hxx>
#include <NXOpen/Drawings_DrawingSheet.hxx>
#include <NXOpen/NXObjectManager.hxx>
// Std C++ Includes
#include <iostream>
#include <sstream>
#include <vector>
#include <string>
#include <algorithm>
#include <tchar.h>
#include <atlconv.h>
#include <shellapi.h>
#include <windows.h>
#undef CreateDialog
#pragma comment(lib,"shell32.lib")
using namespace NXOpen;
using std::string;
using std::exception;
using std::stringstream;
using std::endl;
using std::cout;
using std::cerr;
//------------------------------------------------------------------------------
// Unload Handler
//------------------------------------------------------------------------------
extern "C" DllExport int ufusr_ask_unload()
{
return (int)NXOpen::Session::LibraryUnloadOptionImmediately;// 调试用
//return (int)NXOpen::Session::LibraryUnloadOptionAtTermination;// 程序发布用
//return (int)NXOpen::Session::LibraryUnloadOptionExplicitly;
}
int exportDwg2PDF(double &xDimension, double &yDimension, std::string &waterRemark, tag_t &sheetTAG, std::string &exportPath, bool appendStatus)
{
try{
if (xDimension < 200 || yDimension < 200 || sheetTAG == NULL_TAG || exportPath.empty() == true)
return -1;
NXOpen::Session *theSession = NXOpen::Session::GetSession();
NXOpen::Part *workPart(theSession->Parts()->Work());
NXOpen::Part *displayPart(theSession->Parts()->Display());
NXOpen::PrintPDFBuilder *printPDFBuilder1;
printPDFBuilder1 = workPart->PlotManager()->CreatePrintPdfbuilder();
printPDFBuilder1->SetScale(1.0);
printPDFBuilder1->SetSize(NXOpen::PrintPDFBuilder::SizeOptionScaleFactor);
printPDFBuilder1->SetOutputText(NXOpen::PrintPDFBuilder::OutputTextOptionPolylines);
printPDFBuilder1->SetXDimension(xDimension);
printPDFBuilder1->SetYDimension(yDimension);
printPDFBuilder1->SetColors(NXOpen::PrintPDFBuilder::ColorBlackOnWhite);
printPDFBuilder1->SetWidths(NXOpen::PrintPDFBuilder::WidthCustomThreeWidths);
printPDFBuilder1->SetRasterImages(true);
printPDFBuilder1->SetImageResolution(NXOpen::PrintPDFBuilder::ImageResolutionOptionHigh);
printPDFBuilder1->SetAddWatermark(true);
printPDFBuilder1->SetWatermark(waterRemark.c_str());
printPDFBuilder1->SetAppend(appendStatus);
std::vector<NXOpen::NXObject *> sheets1(1);
NXOpen::Drawings::DrawingSheet *drawingSheet1(dynamic_cast<NXOpen::Drawings::DrawingSheet *>(NXOpen::NXObjectManager::Get(sheetTAG)));
sheets1[0] = drawingSheet1;
printPDFBuilder1->SourceBuilder()->SetSheets(sheets1);
printPDFBuilder1->SetFilename(exportPath);
NXOpen::NXObject *nXObject1;
nXObject1 = printPDFBuilder1->Commit();
printPDFBuilder1->Destroy();
return 0;
}
catch (const exception& e2){
UI::GetUI()->NXMessageBox()->Show("Exception", NXOpen::NXMessageBox::DialogTypeError, e2.what());
throw;
}
}
int getSheetInfos()
{
// 获取显示部件及图纸信息
int _errCode = 0;
tag_t dispTAG = UF_PART_ask_display_part();
char part_fspec[MAX_FSPEC_BUFSIZE] = { 0 };
if (_errCode = UF_PART_ask_part_name(dispTAG, part_fspec) != 0) return _errCode;
std::string strPartName(part_fspec);
transform(strPartName.begin(), strPartName.end(), strPartName.begin(), ::tolower);
if (strPartName.find("dwg") == string::npos) return -1;
int num_draws = 0;
tag_t *drawTAGs = nullptr;
if (_errCode = UF_DRAW_ask_drawings(&num_draws, &drawTAGs) != 0)
return _errCode;
string export_path = strPartName.substr(0, strPartName.find_last_of("."));
for (int idx = 0; idx < num_draws; idx++){
// 导出PDF
UF_DRAW_info_t drawInfos;
_errCode = UF_DRAW_ask_drawing_info(drawTAGs[0], &drawInfos);
double xDimension = drawInfos.size.custom_size[0];
double yDimension = drawInfos.size.custom_size[1];
_errCode = exportDwg2PDF(xDimension, yDimension, string("huangym1\r\n2023-03-25"), drawTAGs[idx], export_path + ".pdf", false);
string tempStr(export_path + ".pdf");
// 打开PDF
USES_CONVERSION;
const WCHAR * cLineChar = A2W(tempStr.c_str());
SHELLEXECUTEINFO sei;
ZeroMemory(&sei, sizeof(SHELLEXECUTEINFO));//使用前最好清空
sei.cbSize = sizeof(SHELLEXECUTEINFO);//管理员权限执行cmd,最基本的使用与 ShellExecute 类似
sei.lpFile = cLineChar;
sei.nShow = SW_SHOW;
sei.lpVerb = _T("open");
BOOL bResult = ShellExecuteEx(&sei);
if (bResult)//执行成功
{
if (sei.hProcess)//指定 SEE_MASK_NOCLOSEPROCESS 并其成功执行,则 hProcess 将会返回执行成功的进程句柄
WaitForSingleObject(sei.hProcess, INFINITE);//等待执行完毕
}
}
if (drawTAGs){
UF_free(drawTAGs);
drawTAGs = nullptr;
}
return _errCode;
}
//========================
// 保存操作入口点函数
//========================
extern "C" DllExport void ufput()
{
try{
if (UF_initialize()) return;
getSheetInfos();
UF_terminate();
}
catch (const NXException& e1)
{
UI::GetUI()->NXMessageBox()->Show("NXException", NXOpen::NXMessageBox::DialogTypeError, e1.Message());
}
catch (const exception& e2)
{
UI::GetUI()->NXMessageBox()->Show("Exception", NXOpen::NXMessageBox::DialogTypeError, e2.what());
}
catch (...)
{
UI::GetUI()->NXMessageBox()->Show("Exception", NXOpen::NXMessageBox::DialogTypeError, "Unknown Exception.");
}
}
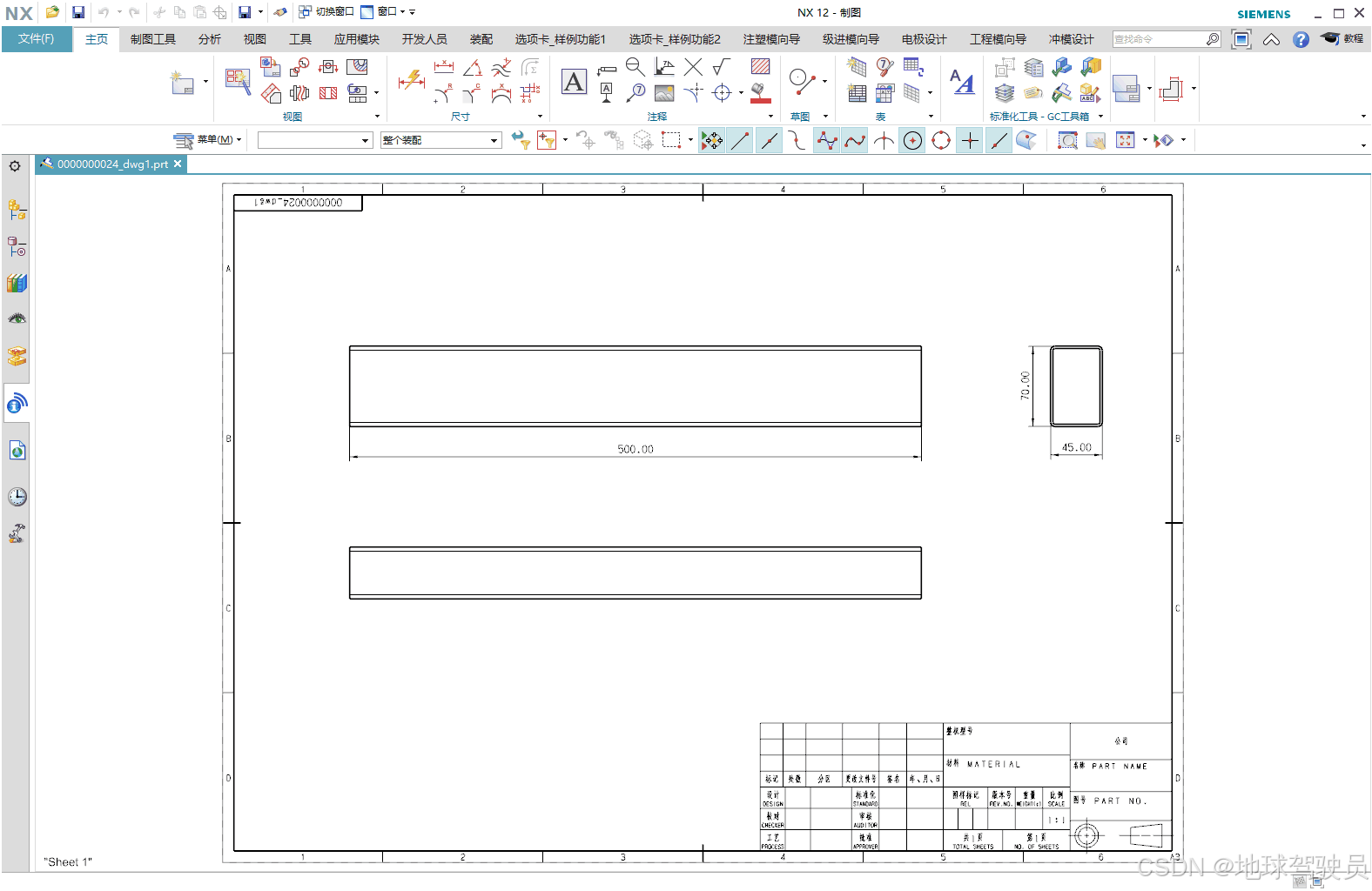