工程结构
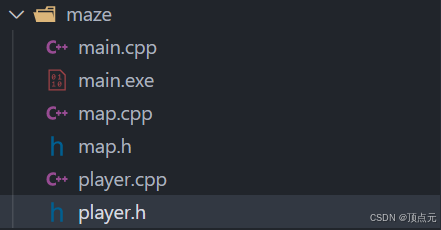
map
map.h
c
#ifndef __MAP_H
#define __MAP_H
#define WALL 0
#define ROAD 1
#define MAX_HEIGHT 30
#define MAX_WIDTH 30
typedef struct Point {
int x;
int y;
}Point_t;
class Map {
public:
Map();
Map(int*map, int row, int column, Point_t in);
~Map();
void draw(void);
void setRoadChar(char r);
char getRoadChar(void);
void setWallChar(char w);
bool checkWallOrNot(int x, int y);
int getHeight(void);
int getWidth(void);
private:
char road_;
char wall_;
int map_[MAX_HEIGHT][MAX_WIDTH];
int height_;
int width_;
//迷宫入口坐标
Point_t in_;
};
#endif /* __MAP_H */
map.cpp
cpp
#include "map.h"
#include <iostream>
using namespace std;
Map::Map() : wall_('$'), road_(' ') {
height_ = 5;
width_ = 10;
int default_map[5][10] = {WALL, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, ROAD,
ROAD, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, WALL,
WALL, WALL, WALL, ROAD, ROAD, ROAD, WALL, ROAD, WALL, ROAD,
WALL, ROAD, ROAD, ROAD, WALL, ROAD, ROAD, ROAD, WALL, WALL,
WALL, ROAD, WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL
};
for(int i = 0; i < 5; ++i) {
for(int j = 0; j < 10; ++j) {
map_[i][j] = default_map[i][j];
}
}
in_.x = 1;
in_.y = 4;
}
Map::Map(int* map, int height, int width, Point_t in) : wall_('$'),road_(' '),in_(in) {
height_ = height;
width_ = width;
if(height > MAX_HEIGHT || width > MAX_WIDTH) {
cout << " height or width more than limit!!!\n";
return;
}
for(int i = 0; i < height; ++i) {
for(int j = 0; j < width; ++j) {
map_[i][j] = *map;
map++;
}
}
}
Map::~Map() {
}
void Map::setWallChar(char w) {
wall_ = w;
}
void Map::setRoadChar(char r) {
road_ = r;
}
char Map::getRoadChar(void) {
return road_;
}
void Map::draw(void) {
for(int i = 0; i < height_; ++i) {
for(int j = 0; j < width_; ++j) {
map_[i][j] == WALL ? cout << wall_: cout << road_;
}
cout << endl;
}
}
bool Map::checkWallOrNot(int x, int y) {
return (map_[y][x] == WALL)? true: false;
}
int Map::getHeight(void) {
return height_;
}
int Map::getWidth(void) {
return width_;
}
player
player.h
cpp
#ifndef __PLAYER_H
#define __PLAYER_H
#include "map.h"
typedef enum {
UP,
DOWN,
LEFT,
RIGHT
}ForWardDirection_e;
class Player {
public:
Player(Map *map);
~Player();
void gotoXY(int x, int y);
void draw(void);
void goAhead(void);
void turnLeft(void);
void turnRight(void);
void start(void);
void setPlayerChar(char chr);
private:
char player_;
int prev_x_ = 1;
int prev_y_ = 4;
int curr_x_ = 1;
int curr_y_ = 4;
bool exit_flag_ = false;
ForWardDirection_e forward = UP;
int count = 0; //记录迷宫中行走的步数
Map *map_;
};
#endif /* __PLAYER_H */
player.cpp
cpp
#include "player.h"
#include <windows.h>
#include <iostream>
#include "map.h"
using namespace std;
Player::Player(Map *map): map_(map),player_('*') {
}
Player::~Player() {
}
void Player::gotoXY(int x, int y) {
COORD cd;
cd.X = x;
cd.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), cd);
}
void Player::setPlayerChar(char chr) {
player_ = chr;
}
void Player::draw(void) {
gotoXY(prev_x_, prev_y_);
cout << map_->getRoadChar();
gotoXY(curr_x_, curr_y_);
cout << player_;
Sleep(1000);
}
void Player::turnLeft(void) {
switch(forward) {
case UP:
forward = LEFT;
break;
case DOWN:
forward = RIGHT;
break;
case LEFT:
forward = DOWN;
break;
case RIGHT:
forward = UP;
break;
default:
break;
}
}
void Player::turnRight(void) {
switch(forward) {
case UP:
forward = RIGHT;
break;
case DOWN:
forward = LEFT;
break;
case LEFT:
forward = UP;
break;
case RIGHT:
forward = DOWN;
break;
default:
break;
}
}
void Player::goAhead(void) {
prev_x_ = curr_x_;
prev_y_ = curr_y_;
switch(forward) {
case UP:
curr_y_ -= 1;
break;
case DOWN:
curr_y_ += 1;
break;
case LEFT:
curr_x_ -= 1;
break;
case RIGHT:
curr_x_ += 1;
break;
default:
break;
}
count++;
}
void Player::start(void) {
while(!exit_flag_) {
int forward_x,forward_y; //前进位置
int right_x, right_y; //右侧位置
switch(forward) {
case UP:
forward_x = curr_x_;
forward_y = curr_y_ - 1;
right_x = curr_x_ + 1;
right_y = curr_y_;
break;
case DOWN:
forward_x = curr_x_;
forward_y = curr_y_+1;
right_x = curr_x_-1;
right_y = curr_y_;
break;
case LEFT:
forward_x = curr_x_-1;
forward_y = curr_y_;
right_x = curr_x_;
right_y = curr_y_-1;
break;
case RIGHT:
forward_x = curr_x_+1;
forward_y = curr_y_;
right_x = curr_x_;
right_y = curr_y_+1;
break;
default:
break;
}
if(map_ == NULL) {
cout << "map is NULL\n";
}
/*右侧是墙*/
if(map_->checkWallOrNot(right_x, right_y)) {
if(map_->checkWallOrNot(forward_x, forward_y)) {
turnLeft();
} else {
goAhead();
draw();
}
} else {
turnRight();
goAhead();
draw();
}
if(curr_y_ == 0) {
exit_flag_ = true;
}
}
/* 打印步数 */
gotoXY(0, map_->getHeight()+2);
cout << "total count: " << count << endl;
}
main
cpp
#include <iostream>
#include "player.h"
int main(int argc, char *argv[]) {
int default_map[5][10] = {ROAD, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, ROAD,
ROAD, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, WALL,
WALL, WALL, WALL, ROAD, ROAD, ROAD, WALL, ROAD, WALL, ROAD,
WALL, ROAD, ROAD, ROAD, WALL, ROAD, ROAD, ROAD, WALL, WALL,
WALL, ROAD, WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL
};
Point_t entry = {1, 4};
Map map(&default_map[0][0], 5, 10, entry);
map.setWallChar('|');
system("cls");
map.draw();
Player player(&map);
player.draw();
player.start();
return 0;
}
编译运行
编译指令
bash
g++ map.cpp player.cpp main.cpp -o main
运行效果: