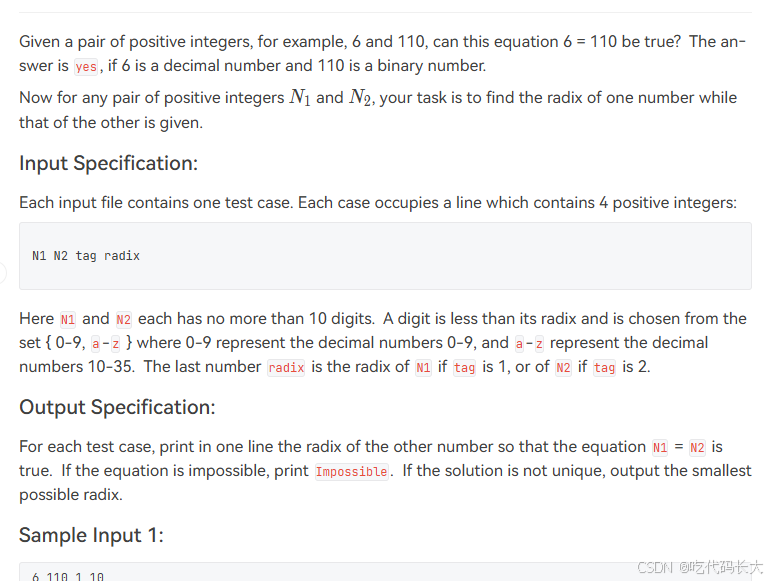
BigInteger:
java
import java.util.Scanner;
import java.math.BigInteger;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String N1 = in.next();
String N2 = in.next();
int tag = in.nextInt();
long radix = in.nextLong(); // 初始进制
BigInteger tgt = BigInteger.ZERO;
if (tag == 1) {
tgt = convert10(N1, radix);
} else {
tgt = convert10(N2, radix);
}
String process = (tag == 1) ? N2 : N1;
BigInteger res = BigInteger.valueOf(-1);
long maxDigit = getRange(process) + 1; // 可能的最小基数
BigInteger l = BigInteger.valueOf(maxDigit);
BigInteger r = tgt.max(BigInteger.valueOf(maxDigit));
while (l.compareTo(r) <= 0) { // 左闭右闭区间
BigInteger mid = l.add(r).divide(BigInteger.TWO);
BigInteger cur = convert10(process, mid.longValue());
if (cur.equals(tgt)) {
res = mid;
break;
} else if (cur.compareTo(tgt) > 0) {
r = mid.subtract(BigInteger.ONE); // cur 超过 tgt 或发生溢出,缩小右边界
} else {
l = mid.add(BigInteger.ONE); // cur 小于 tgt,增加左边界
}
}
if (res.equals(BigInteger.valueOf(-1))) {
System.out.println("Impossible");
} else {
System.out.println(res);
}
}
// 将字符串 s 按照 radix 进制转换为 10 进制数
public static BigInteger convert10(String s, long radix) {
BigInteger res = BigInteger.ZERO;
BigInteger base = BigInteger.ONE;
BigInteger bigRadix = BigInteger.valueOf(radix);
for (int i = s.length() - 1; i >= 0; i--) {
char c = s.charAt(i);
BigInteger value;
if (Character.isDigit(c)) {
value = BigInteger.valueOf(c - '0');
} else {
value = BigInteger.valueOf(c - 'a' + 10);
}
res = res.add(value.multiply(base));
base = base.multiply(bigRadix);
}
return res;
}
// 获取字符串中最大的字符所代表的数值,用于确定最小的可能进制
public static long getRange(String s) {
long maxDigit = -1;
for (char c : s.toCharArray()) {
if (Character.isDigit(c)) {
maxDigit = Math.max(maxDigit, c - '0'); // 数字字符
} else if (Character.isLetter(c)) {
maxDigit = Math.max(maxDigit, c - 'a' + 10); // 字母字符
}
}
return maxDigit;
}
}
long:
java
import java.util.Scanner;
import java.math.BigInteger;
public class Main{
public static void main(String[] args){
Scanner in = new Scanner(System.in);
String N1 = in.next();
String N2 = in.next();
int tag = in.nextInt();
long radix = (long) in.nextInt();
long tgt = -1;
if(tag == 1){
tgt = convert10(N1, radix);
}else{
tgt = convert10(N2, radix);
}
String process = tag == 1 ? N2 : N1;
long res = -1;
long l = getRange(process) + 1;
long r = Math.max(tgt, l);
while(l <= r){//左闭右闭
long mid = (l+r) >> 1;
long cur = convert10(process, mid);
if(cur == tgt){
res = mid;
break;
}else if(cur == -1 || cur > tgt){
r = mid - 1;
}else{
l = mid + 1;
}
}
if(res == -1){
System.out.println("Impossible");
}else{
System.out.println(res);
}
}
public static long convert10(String s, long radix){
char[] ch = s.toCharArray();
long res = 0;
long base = 1;
for(int i = ch.length-1; i >= 0; i--){
if(0 <= ch[i]-'0' && ch[i]-'0' <= 9){
res += base * (ch[i]-'0');
}else if(0 <= ch[i]-'a' && ch[i]-'a' <= 25){
res += base * (ch[i]-'a'+10);
}
base *= radix;
if(res < 0 || base < 0){
return -1;
}
}
return res;
}
public static long getRange(String s) {
char[] ch = s.toCharArray();
long maxDigit = -1;
for (char c : ch) {
if (Character.isDigit(c)) {
maxDigit = Math.max(maxDigit, c - '0'); // 处理数字
} else if (Character.isLetter(c)) {
maxDigit = Math.max(maxDigit, c - 'a' + 10); // 处理字母
}
}
return maxDigit;
}
}