个人简介
👀个人主页: 前端杂货铺
🙋♂️学习方向: 主攻前端方向,正逐渐往全干发展
📃个人状态: 研发工程师,现效力于中国工业软件事业
🚀人生格言: 积跬步至千里,积小流成江海
🥇推荐学习:🍍前端面试宝典 🎨100个小功能 🍉Vue2 🍋Vue3 🍓Vue2/3项目实战 🥝Node.js实战 🍒Three.js
🌕个人推广:每篇文章最下方都有加入方式,旨在交流学习&资源分享,快加入进来吧
文章目录
-
- 前言
- [Ref 响应式(基本数据类型)](#Ref 响应式(基本数据类型))
- [Reactive 响应式(对象类型)](#Reactive 响应式(对象类型))
- [ref 对比 reactive](#ref 对比 reactive)
前言
重拾 Vue3,查缺补漏、巩固基础。
Ref 响应式(基本数据类型)
使用 ref 包裹,即可实现基本数据类型的响应式。
javascript
<template>
<div class="person">
<h2>{{ name }}</h2>
<h2>{{ age }}</h2>
<button @click="changeName">修改名字</button>
<button @click="changeAge">修改年龄</button>
<button @click="showTel">查看联系方式</button>
</div>
</template>
<script lang="ts" setup>
import { ref } from "vue";
let name = ref("zhangsan");
let age = ref(18);
let tel = 18588888888;
function changeName() {
name.value = "zs";
}
function changeAge() {
age.value += 1;
}
function showTel() {
alert(tel);
}
</script>
<style scoped>
.person {
background-color: skyblue;
box-shadow: 0 0 10px;
border-radius: 10px;
padding: 20px;
}
button {
margin: 0 5px;
}
</style>
Reactive 响应式(对象类型)
使用 reactive 包裹,即可实现基本数据类型的响应式。
javascript
<template>
<div class="person">
<h2>{{ car.brand }}: {{ car.price }}w</h2>
<button @click="changePrice">修改价格</button>
<ul v-for="item in person" :key="item.id">
<li>{{ item.name }}</li>
</ul>
<button @click="changeFirstName">修改第一个人的名字</button>
</div>
</template>
<script lang="ts" setup>
import { reactive } from "vue";
let car = reactive({ brand: "大奔", price: 80 });
let person = reactive([
{ id: 1, name: "zhangsan" },
{ id: 2, name: "lisi" },
{ id: 3, name: "zahuopu" },
]);
function changePrice() {
car.price += 10;
}
function changeFirstName() {
person[0].name = "hh";
}
</script>
<style scoped>
.person {
background-color: skyblue;
box-shadow: 0 0 10px;
border-radius: 10px;
padding: 20px;
}
button {
margin: 0 5px;
}
</style>
ref 对比 reactive
ref 创建的变量必须使用 .value
,reactive 重新分配一个新对象,会失去响应式(可以通过 Object.assign 去替换整体)。
使用 reactive 实现对象响应式。
javascript
<template>
<div class="person">
<h2>{{ car.brand }}: {{ car.price }}w</h2>
<button @click="changeCarInfo">修改汽车信息</button>
</div>
</template>
<script lang="ts" setup>
import { reactive } from "vue";
let car = reactive({ brand: "大奔", price: 80 });
function changeCarInfo() {
Object.assign(car, { brand: "小米", price: 29.98 });
}
</script>
使用 ref 实现对象的响应式。
javascript
<template>
<div class="person">
<h2>{{ car.brand }}: {{ car.price }}w</h2>
<button @click="changeCarInfo">修改汽车信息</button>
</div>
</template>
<script lang="ts" setup>
import { ref } from "vue";
let car = ref({ brand: "大奔", price: 80 });
function changeCarInfo() {
car.value = { brand: "小米", price: 29.98 };
}
</script>
参考资料:
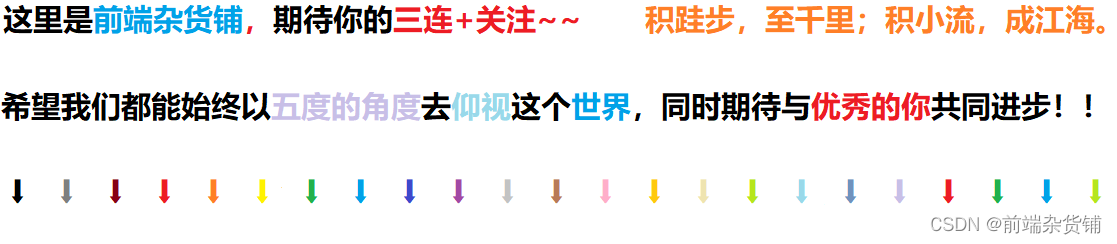