注意:要引入jquery
可以直接使用弹框播放iframe
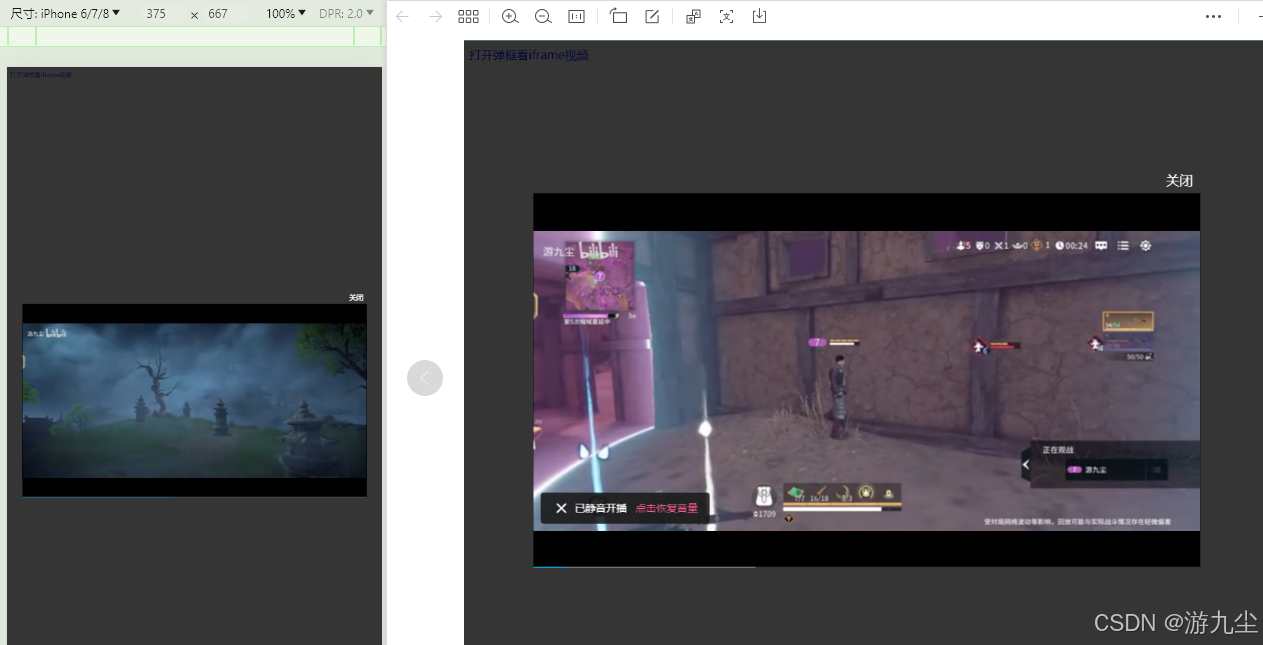
一、创建 index.html
html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
.modal {
/* 默认隐藏 */
display: none;
position: fixed;
z-index: 99999;
left: 0;
top: 0;
width: 100%;
height: 100%;
background-color: rgba(3, 3, 3, .8);
}
.close-btn {
/* 关闭按钮 */
position: absolute;
z-index: 100;
right: 10px;
top: -30px;
font-size: 18px;
color: #fff;
text-align: center;
cursor: pointer;
}
/* 弹窗内容 */
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 13px 9px;
width: 100%; /* 这个要先去掉,手机端时在加上 */
display: flex;
justify-content: center;
align-items: center;
}
.iframe{
position: relative;
padding-bottom: 56.25%;
height: 0;
overflow: hidden;
}
.iframe iframe {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.iframe-width{
/* PC */
position: absolute; left:50%; transform: translateX(-50%);
width: 900px;
}
@media (max-width: 900px){
/* 移动 */
.iframe-width{
/* position: absolute; top:50%; left:0; transform: translateY(-50%);
margin: unset; */
width: 100%;
}
}
</style>
</head>
<body>
<div onclick="videoIframe()" style="color: blue;">打开弹框看iframe视频</div>
<div id="myModal" class="modal">
<div class="modal-content">
<div id="iframeFuDiv" class="iframe-width">
<span class="close-btn">关闭</span>
<div class="modal-content-div">
</div>
</div>
</div>
</div>
<script src="jquery-2.2.0.min.js"></script>
<script src="index.js"></script>
</body>
</html>
二、创建 index.js
javascript
window.onload = function(){
// 打开弹框( class="open-btn" )
$(document).delegate('.open-btn','click',function(e){
document.getElementById("myModal").style.display = "block";
})
// 关闭弹框( class="close-btn" )
$(document).delegate('.close-btn','click',function(e){
document.getElementById("myModal").style.display = "none";
$(".modal-content-div").html('') // 关闭后清除视频
})
// 点击空白区域关闭弹框
const myModal = document.getElementById('myModal');
myModal.addEventListener('click', function(event) {
if (event.target === myModal) {
myModal.style.display = 'none'
$(".modal-content-div").html('') // 关闭后清除视频
}
});
}
// iframe视频弹框播放
function videoIframe(){
let videoUrl = '//player.bilibili.com/player.html?isOutside=true&aid=113331050385027&bvid=BV144CDYzESY&cid=26355240647&p=1'
let html = `
<iframe id="videoIframe" height="506" width="900" src="${videoUrl}" frameborder=0 allowfullscreen></iframe>
`
$(".modal-content-div").html(html)
// 这个需要加上
$('iframe').wrap('<div class="iframe"></div>')
document.getElementById("myModal").style.display = "block"
}