NIO2
从 Java 7 在之前的NIO基础上,它提供了异步 IO 操作、文件系统访问增强等诸多功能
路径(Path)接口
Path 接口代表了文件系统的路径。它可以用来定位一个文件或目录。
提供了多种方法来解析、转换和查询路径信息。Paths 类提供了一些静态方法用于获取 Path 。
java
Path path1 = Paths.get("/home/user/documents");
Path path2 = Paths.get("file.txt");
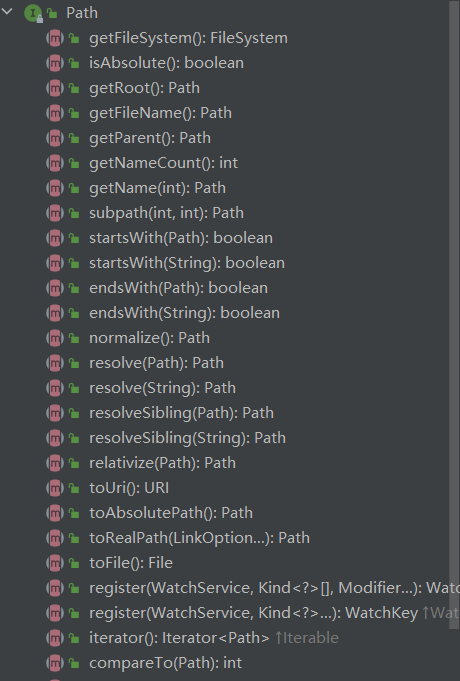
Files
Files 类提供了大量的静态方法来执行文件操作,比如 读取、写入、复制、删除、移动
java
Path sourcePath = Paths.get("source.txt");
Path targetPath = Paths.get("target.txt");
Files.copy(sourcePath, targetPath);
异步 IO
相对而言这是最重要的功能,异步 IO 操作使得在处理大量 IO 任务时可以充分利用系统资源,NIO2 主要增加了 文件 和 Socket异步IO支持。
- AsynchronousFileChannel
允许异步地从文件读取数据或向文件写入数据。
适用于需要高并发性能且不希望线程被I/O操作阻塞的情况。
- AsynchronousSocketChannel 和 AsynchronousServerSocketChannel:
分别对应客户端和服务端的异步套接字通信,支持非阻塞模式下的连接建立、数据发送与接收。
Future 和 CompletionHandler
异步IO 主要通过 CompletionHandler 接口或 Future 对象来处理完成事件。
java
Path path = Paths.get("data.txt");
AsynchronousFileChannel fileChannel =
AsynchronousFileChannel.open(path, StandardOpenOption.WRITE);
ByteBuffer buffer = ByteBuffer.allocate(1024);
long position = 0;
buffer.put("test future".getBytes());
buffer.flip();
Future<Integer> operation = fileChannel.write(buffer, position);
...
java
Path path = Paths.get("data.txt");
hronousFileChannel fileChannel =
AsynchronousFileChannel.open(path, StandardOpenOption.WRITE);
ByteBuffer buffer = ByteBuffer.allocate(1024);
long position = 0;
buffer.put("test CimpletionHandler".getBytes());
buffer.flip();
fileChannel.write(buffer, position, buffer, new CompletionHandler<Integer, ByteBuffer>() {
@Override
public void completed(Integer result, ByteBuffer attachment) {
System.out.println("bytes written: " + result);
}
@Override
public void failed(Throwable exc, ByteBuffer attachment) {
exc.printStackTrace();
}
});