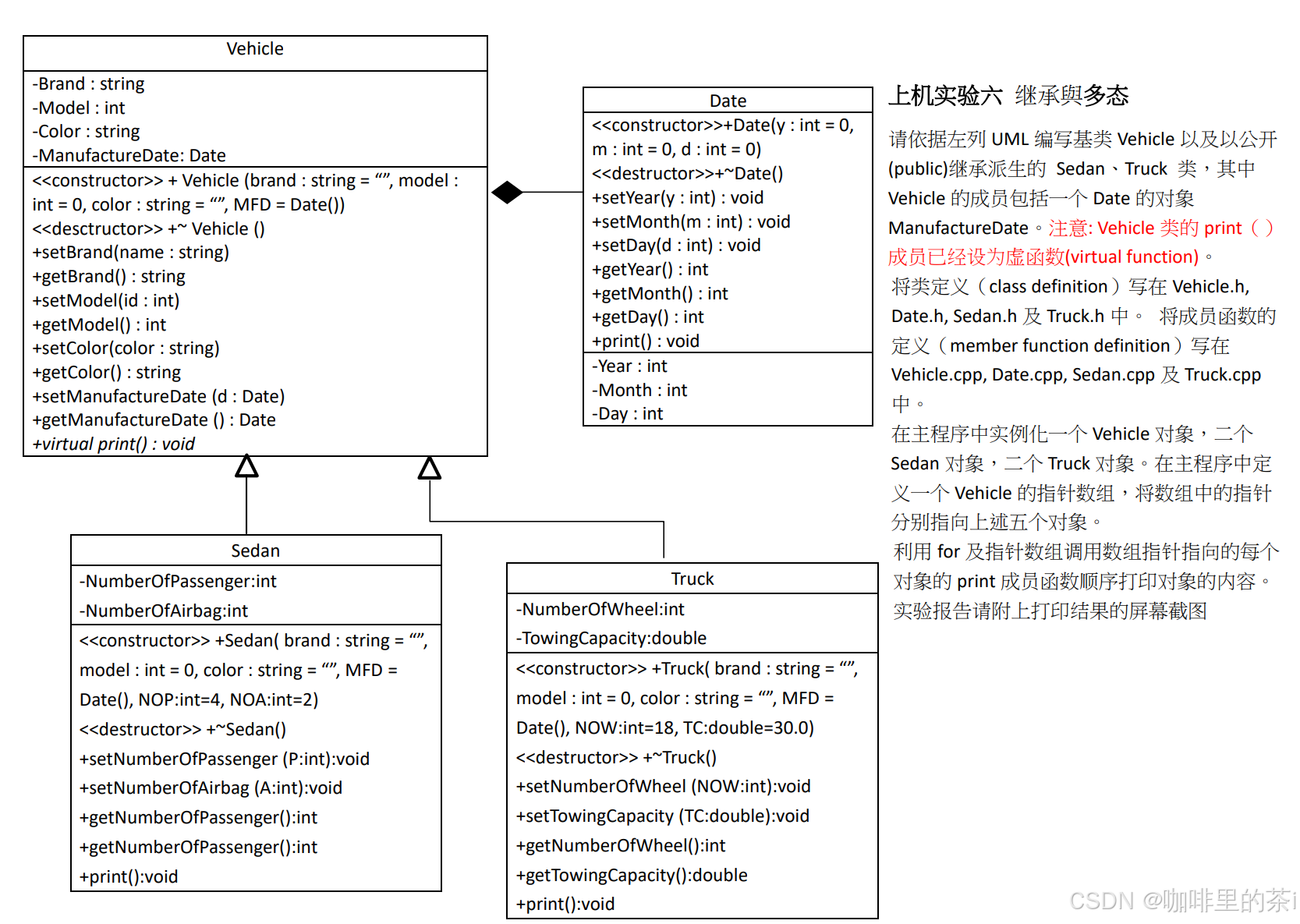
main.cpp
cpp
#include <iostream>
#include "Vehicle.h"
#include "Sedan.h"
#include "Truck.h"
using namespace std;
int main()
{
Vehicle vehicle("Generic", 100, "Gray", Date(2005, 6, 6));
Sedan sedan1("Toyota", 200, "Red", Date(2020, 1, 15), 5, 4);
Sedan sedan2("Honda", 201, "Blue", Date(2021, 5, 20), 4, 6);
Truck truck1("Ford", 300, "Black", Date(2019, 10, 5), 18, 40.0);
Truck truck2("Chevy", 301, "Silver", Date(2022, 3, 10), 20, 50.0);
Vehicle* vehicles[] = {&vehicle, &sedan1, &sedan2, &truck1, &truck2};
for (const auto& vehiclePtr : vehicles)
{
vehiclePtr->print();
}
return 0;
}
Date.h
cpp
#ifndef DATE_H
#define DATE_H
#include <iostream>
using namespace std;
class Date
{
friend class Vehicle;
public:
Date();
Date(int y, int m, int d);
~Date();
void setYear(int y);
void setMonth(int m);
void setDay(int d);
int getYear();
int getMonth();
int getDay();
void print();
private:
int Year;
int Month;
int Day;
};
#endif // DATE_H
Date.cpp
cpp
#include "Date.h"
#include <iostream>
using namespace std;
Date::Date()
{
Year = 0;
Month = 0;
Day = 0;
}
Date::Date(int year, int month, int day)
{
setYear(year);
setMonth(month);
setDay(day);
}
Date::~Date()
{
//cout << "object Destructor is called" << endl;
}
void Date::setYear(int year)
{
Year = year;
}
void Date::setMonth(int month)
{
Month = month;
}
void Date::setDay(int day)
{
Day = day;
}
int Date::getYear()
{
return Year;
}
int Date::getMonth()
{
return Month;
}
int Date::getDay()
{
return Day;
}
void Date::print()
{
cout << endl;
cout << "Year :" << getYear() << endl;
cout << "Month :" << getMonth() << endl;
cout << "Day :" << getDay() << endl;
}
Sedan.h
cpp
#ifndef SEDAN_H
#define SEDAN_H
#include <iostream>
#include "Vehicle.h"
using namespace std;
class Sedan :public Vehicle
{
public:
Sedan();
Sedan(string brand, int model, string color, Date MFD, int NOP, int NOA);
~Sedan();
void setNumberOfPassenger(int P);
int getNumberOfPassenger();
void setNumberOfAirbag(int A);
int getNumberOfAirbag();
void print();
private:
int NumberOfPassenger;
int NumberOfAirbag;
};
#endif // SEDAN_H
Sedan.cpp
cpp
#include "Sedan.h"
#include <iostream>
using namespace std;
Sedan::Sedan()
{
NumberOfPassenger = 0;
NumberOfAirbag = 0;
}
Sedan::Sedan(string brand, int model, string color, Date MFD, int NOP, int NOA)
{
setBrand(brand);
setModel(model);
setColor(color);
setManufactureDate(MFD);
setNumberOfPassenger(NOP);
setNumberOfAirbag(NOA);
}
Sedan::~Sedan()
{
}
void Sedan::setNumberOfPassenger(int P)
{
NumberOfPassenger = P;
}
int Sedan::getNumberOfPassenger()
{
return NumberOfPassenger;
}
void Sedan::setNumberOfAirbag(int A)
{
NumberOfAirbag = A;
}
int Sedan::getNumberOfAirbag()
{
return NumberOfAirbag;
}
void Sedan::print()
{
Vehicle::print();
cout << "Number of Passengers: " << NumberOfPassenger << ", Number of Airbags: " << NumberOfAirbag << endl;
}
Truck.h
cpp
#ifndef TRUCK_H
#define TRUCK_H
#include "Vehicle.h"
using namespace std;
class Truck : public Vehicle
{
public:
Truck();
Truck(string brand, int model, string color, Date MFD, int NOW, double TC);
~Truck();
void setNumberOfWheel(int NOW);
int getNumberOfWheel();
void setTowingCapacity(double TC);
double getTowingCapacity();
void print();
private:
int NumberOfWheel;
double TowingCapacity;
};
#endif // TRUCK_H
Truck.cpp
cpp
#include "Truck.h"
#include <iostream>
using namespace std;
Truck::Truck()
{
NumberOfWheel = 0;
TowingCapacity = 0;
}
Truck::Truck(string brand, int model, string color, Date MFD, int NOW, double TC)
{
setBrand(brand);
setModel(model);
setColor(color);
setManufactureDate(MFD);
setNumberOfWheel(NOW);
setTowingCapacity(TC);
}
Truck::~Truck()
{
}
void Truck::setNumberOfWheel(int NOW)
{
NumberOfWheel = NOW;
}
int Truck::getNumberOfWheel()
{
return NumberOfWheel;
}
void Truck::setTowingCapacity(double TC)
{
TowingCapacity = TC;
}
double Truck::getTowingCapacity()
{
return TowingCapacity;
}
void Truck::print()
{
Vehicle::print();
cout << "Number of Wheels: " << NumberOfWheel << ", Towing Capacity: " << TowingCapacity << " tons" << endl;
}
Vehicle.h
cpp
#ifndef VEHICLE_H
#define VEHICLE_H
#include <iostream>
#include "Date.h"
using namespace std;
class Vehicle
{
public:
Vehicle();
Vehicle(string brand, int model, string color, Date MFD);
~Vehicle();
void setBrand(string brand);
string getBrand();
void setModel(int id);
int getModel();
void setColor(string color);
string getColor();
void setManufactureDate(Date d);
Date getManufactureDate();
virtual void print();
private:
string Brand;
int Model;
string Color;
Date ManufactureDate;
};
#endif // VEHICLE_H
Vehicle.cpp
cpp
#include "Vehicle.h"
#include <iostream>
using namespace std;
Vehicle::Vehicle()
{
Brand = "XXX";
Model = 10086;
Color = "Black";
}
Vehicle::Vehicle(string brand, int model, string color, Date MFD)
{
setBrand(brand);
setModel(model);
setColor(color);
setManufactureDate(MFD);
}
Vehicle::~Vehicle()
{
}
void Vehicle::setBrand(string brand)
{
Brand = brand;
}
string Vehicle::getBrand()
{
return Brand;
}
void Vehicle::setModel(int id)
{
Model = id;
}
int Vehicle::getModel()
{
return Model;
}
void Vehicle::setColor(string color)
{
Color = color;
}
string Vehicle::getColor()
{
return Color;
}
void Vehicle::setManufactureDate(Date d)
{
ManufactureDate = d;
}
Date Vehicle::getManufactureDate()
{
return ManufactureDate;
}
void Vehicle::print()
{
cout << "Brand: " << Brand << ", Model: " << Model << ", Color: " << Color << ", ManufactureDate: ";
ManufactureDate.print();
cout << endl;
}
Result:
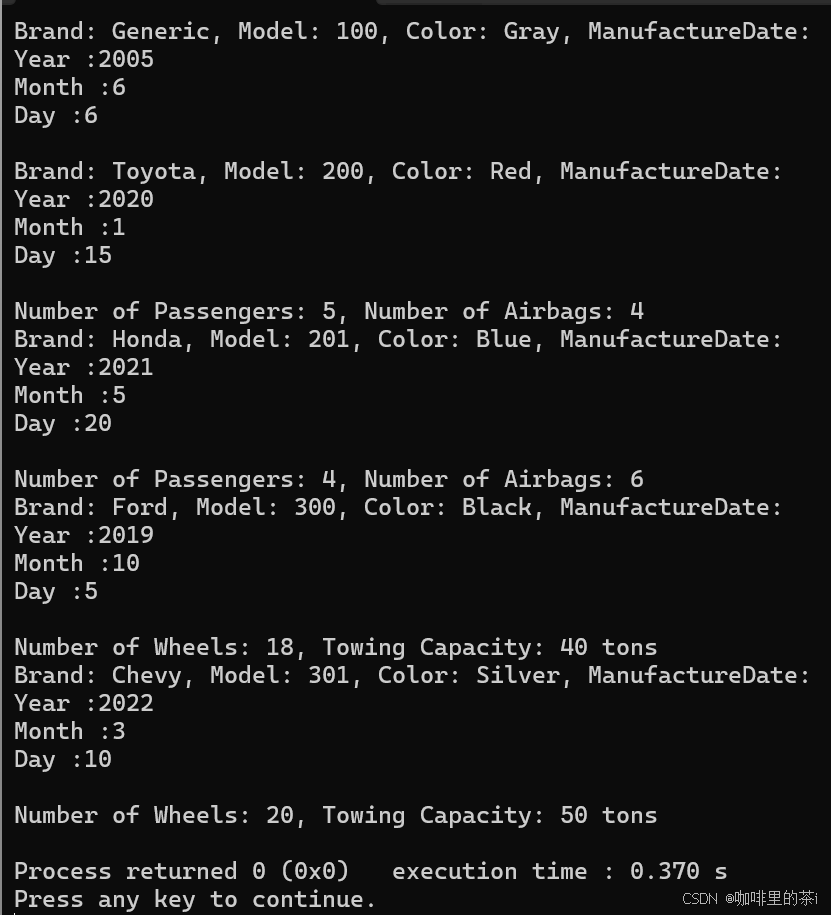