查询记录:pageQueryOp中列表查询的group by
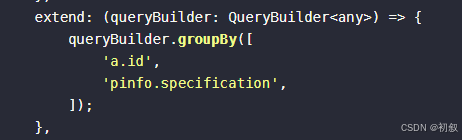
node ts controller代码如下
TypeScript
import { CoolController, BaseController } from '@cool-midway/core';
import { Inject, Post, Get, Param } from '@midwayjs/decorator';
import { ComparePricesPlanInfoEntity } from '../../entity/comparePricesPlanInfo';
import { InventoryOrderInfoService } from '../../service/inventoryOrderInfo';
import { PlanInfoEntity } from '../../entity/planInfo';
import { ConsumableDetailEntity } from '../../../consumable/entity/detail';
import { Context } from 'vm';
import { OrderEntity } from '../../entity/order';
import { ComparePricesEntity } from '../../entity/comparePrices';
import { ComparePricesInfoEntity } from '../../entity/comparePricesInfo';
import { VendorEntity } from '../../entity/vendor';
import { QueryBuilder } from 'typeorm';
/**
* 描述
*/
@CoolController({
api: ['add', 'delete', 'update', 'info', 'list', 'page'],
entity: OrderEntity,
service: InventoryOrderInfoService,
pageQueryOp: {
// keyWordLikeFields: ['vendorName'],
// 指定返回字段
select: [
//采购单信息
'a.id',
'a.state',//状态:待确认(0)、已发货(1)、已收货(2)
'a.inspectorName',//验收人员姓名
'a.acceptanceContent',//验收内容
'a.resultState',//验收结果状态:未验收(0)、通过(1)、不通过(2)
'a.reason',//通过/不通过原因
'a.createBy',//创建者
'a.createTime',//创建时间
'a.code',//订单编码
'a.title',//订单标题
'a.content',//订单内容
'a.remark as orderRemark',//订单备注
//比价
'cp.comparePricesInfoId',//比价信息ID
'cp.consumableDetailId',//耗材ID
//采用价格信息
'cpinfo.quotedPrice',//报价
'cpinfo.serviceContent',//服务内容
'cpinfo.deliveryTime',//货期
'cpinfo.isProposed',//拟用采纳
'cpinfo.vendorId',//供应商ID
// 'cpinfo.consumableDetailId',//耗材ID
'cpinfo.planInfoId',//计划明细表ID
'cpinfo.vcid',//供应商-耗材关联表ID
//耗材信息
'c.consumableNo',
'c.consumableName',
'c.brand',
'c.model',
'c.type',
'c.remark as consumableDetailRemark',
'c.status',
//供应商信息
'v.vendorCode',
'v.vendorName',
'v.vendorNick',
'v.vendorEn',
//采购计划数量
'SUM(pinfo.number) as sumNum',
//采购计划规格
'pinfo.specification',
//采购单信息
// 'a.id',
// 'a.planInfoId',
// 'a.comparePricesId',
// 'a.createTime',
// 'a.updateTime',
// //采购计划详情
// 'b.planId',
// 'b.consumableDetailId',
// 'b.specification',
// 'b.number',
// 'b.budget',
// 'b.deliveryTime',
// // 'b.createBy',
// // 'b.createTime',
],
// 联表查询
join: [
//ComparePricesEntity
{
entity: ComparePricesEntity,
alias: 'cp',
condition: 'a.comparePricesId = cp.id',
type: 'leftJoin',
},
//ComparePricesInfoEntity
{
entity: ComparePricesInfoEntity,
alias: 'cpinfo',
condition: 'cp.comparePricesInfoId = cpinfo.id',
type: 'leftJoin',
},
//ConsumableDetailEntity
{
entity: ConsumableDetailEntity,
alias: 'c',
condition: 'cp.consumableDetailId = c.id',
type: 'leftJoin',
},
//VendorEntity
{
entity: VendorEntity,
alias: 'v',
condition: 'cpinfo.vendorId = v.id',
type: 'leftJoin',
},
//ComparePricesPlanInfoEntity
{
entity: ComparePricesPlanInfoEntity,
alias: 'cppinfo',
condition: 'a.comparePricesId = cppinfo.comparePricesId',
type: 'leftJoin',
},
//PlanInfoEntity
{
entity: PlanInfoEntity,
alias: 'pinfo',
condition: 'cppinfo.planInfoId = pinfo.id',
type: 'leftJoin',
},
// {
// entity: OrderEntity,
// alias: 'o',
// condition: 'a.comparePricesId = o.comparePricesId',
// type: 'leftJoin',
// },
],
where: async (ctx: Context) => {
return [
// ['a.id not in (SELECT comparePricesId from pi_purchase_order)', {}],
// ['a.comparePricesId = :comparePricesId', { comparePricesId: ctx.request.body.comparePricesId }]
];
},
extend: (queryBuilder: QueryBuilder<any>) => {
queryBuilder.groupBy([
'a.id',
'pinfo.specification',
]);
},
addOrderBy: {
'a.createTime': 'asc',
},
},
})
export class InventoryOrderInfoController extends BaseController {
@Inject()
inventoryOrderInfoService: InventoryOrderInfoService;
}
具体的SQL执行语句:
sql
SELECT
a.id,
a.state,
a.inspectorName,
a.acceptanceContent,
a.resultState,
a.reason,
a.createBy,
a.createTime,
a.CODE,
a.title,
a.content,
a.remark AS orderRemark,
cp.comparePricesInfoId,
cp.consumableDetailId,
cpinfo.quotedPrice,
cpinfo.serviceContent,
cpinfo.deliveryTime,
cpinfo.isProposed,
cpinfo.vendorId,
cpinfo.planInfoId,
cpinfo.vcid,
c.consumableNo,
c.consumableName,
c.brand,
c.model,
c.type,
c.remark AS consumableDetailRemark,
c.STATUS,
v.vendorCode,
v.vendorName,
v.vendorNick,
v.vendorEn,
SUM( pinfo.number ) AS sumNum,
pinfo.specification
FROM
`pi_purchase_order` `a`
LEFT JOIN `pi_compare_prices` `cp` ON `a`.`comparePricesId` = `cp`.`id`
LEFT JOIN `pi_compare_prices_info` `cpinfo` ON `cp`.`comparePricesInfoId` = `cpinfo`.`id`
LEFT JOIN `consumable_detail` `c` ON `cp`.`consumableDetailId` = `c`.`id`
LEFT JOIN `pi_vendor` `v` ON `cpinfo`.`vendorId` = `v`.`id`
LEFT JOIN `pi_compare_prices_plan_info` `cppinfo` ON `a`.`comparePricesId` = `cppinfo`.`comparePricesId`
LEFT JOIN `pi_plan_info` `pinfo` ON `cppinfo`.`planInfoId` = `pinfo`.`id`
GROUP BY
`a`.`id`,
pinfo.specification
ORDER BY
`a`.`createTime` ASC,
`createTime` DESC