Android Xml LinearLayout
两个TextView
水平并排,宽度占比1:2
XML布局文件
Dart
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:gravity="center"
android:paddingTop="10dp"
android:paddingBottom="10dp"
android:textColor="@android:color/black"
android:background="@android:color/holo_orange_light"
android:text="占比1" />
<TextView
android:layout_width="wrap_content"
android:gravity="center"
android:paddingTop="10dp"
android:paddingBottom="10dp"
android:textColor="@android:color/black"
android:layout_height="wrap_content"
android:background="@android:color/holo_blue_light"
android:layout_weight="2.0"
android:text="占比2" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
预览效果
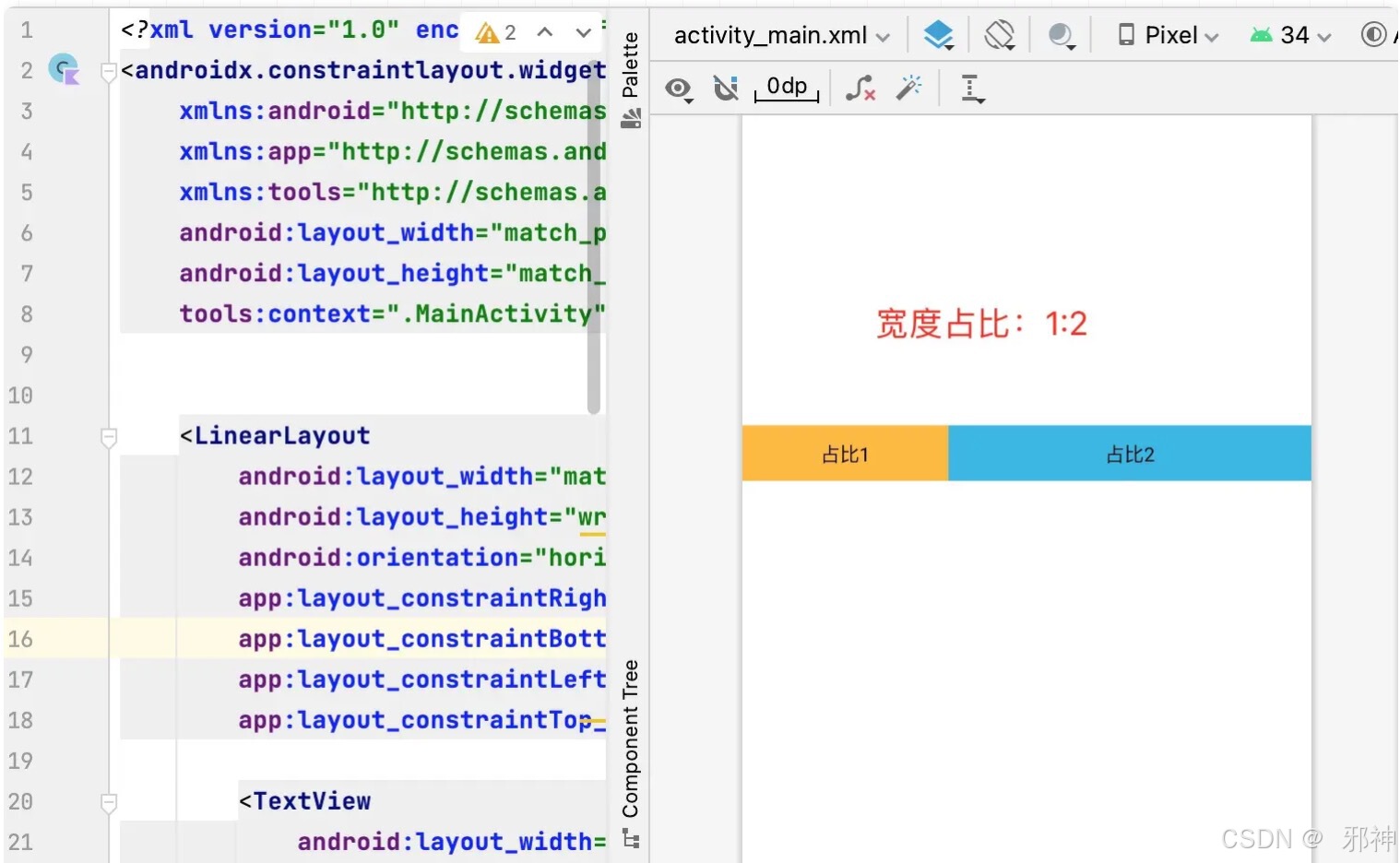
Android Compose 水平布局
导入依赖包
Dart
dependencies {
......
implementation ("androidx.activity:activity-compose:1.3.1")
implementation("androidx.compose.material:material:1.4.3")
implementation("androidx.compose.ui:ui-tooling:1.4.3")
}
启用Compose
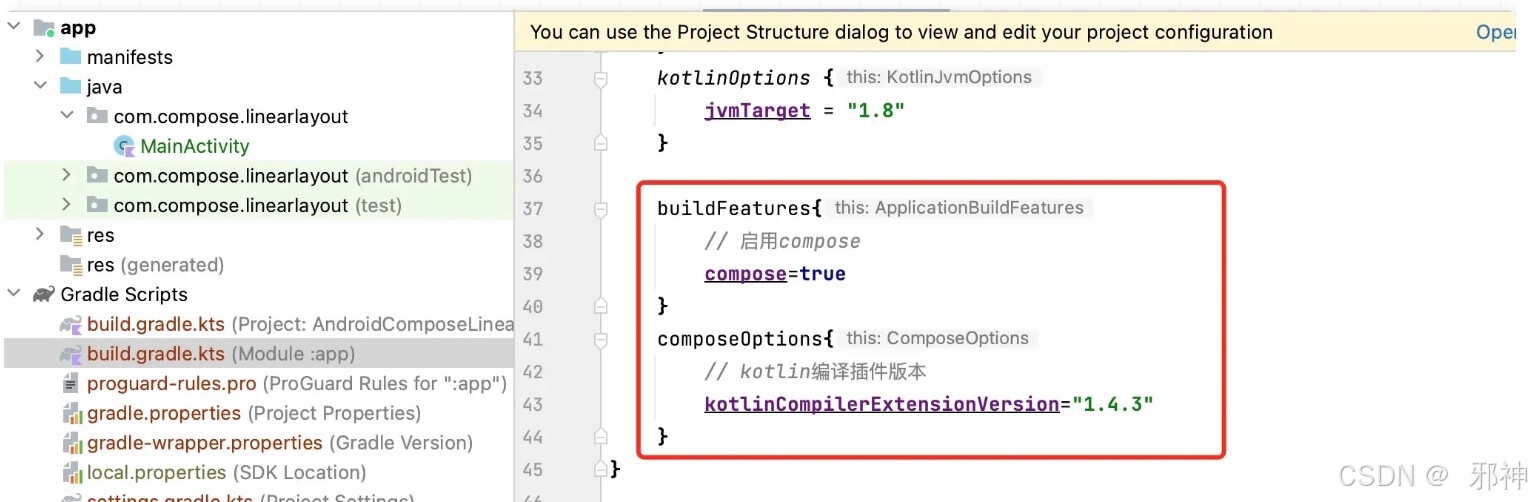
Row定义水平布局
两个Text
水平并排,宽度占比1:2
Dart
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.height
import androidx.compose.material.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
HorizontalLayoutLayout()
}
}
@Preview
@Composable
fun HorizontalLayoutLayout() {
Row(
) {
Box(
modifier = Modifier
.weight(1.0f)
.height(60.dp)
.background(color = Color.Red),
contentAlignment = Alignment.Center
) {
Text(
text = "占比1",
color = Color.Black,
fontSize = 12.sp,
)
}
Box(
modifier = Modifier
.weight(2.0f)
.height(60.dp)
.background(color = Color.Yellow),
contentAlignment = Alignment.Center
) {
Text(
text = "占比2",
color = Color.Black,
fontSize = 12.sp,
)
}
}
}
}
预览效果
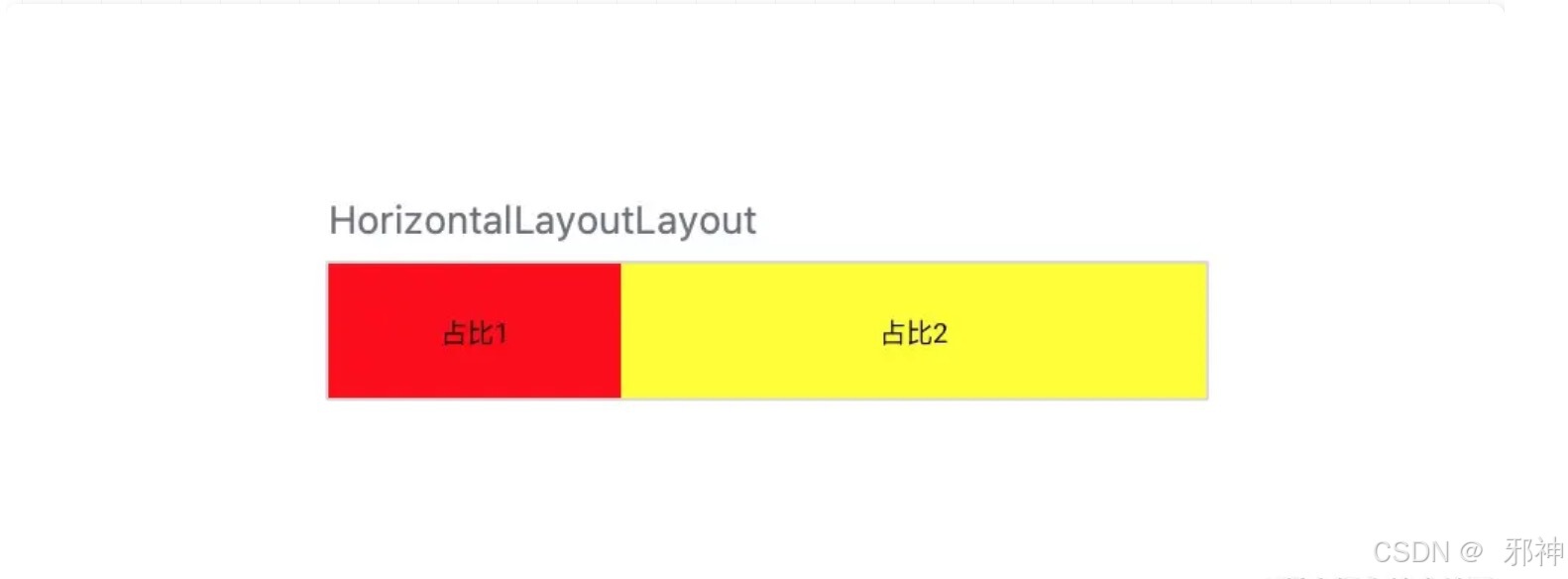
Flutter 水平布局
两个Text
水平并排,宽度占比1:2
Dart
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Row(
children: [
Expanded(
flex: 1,
child: Container(
height: 60.0,
alignment: Alignment.center,
color: Colors.red,
child: const Text(
'占比1',
),
),
),
Expanded(
flex: 2,
child: Container(
height: 60.0,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text(
'占比2',
),
),
)
],
)),
);
}
}
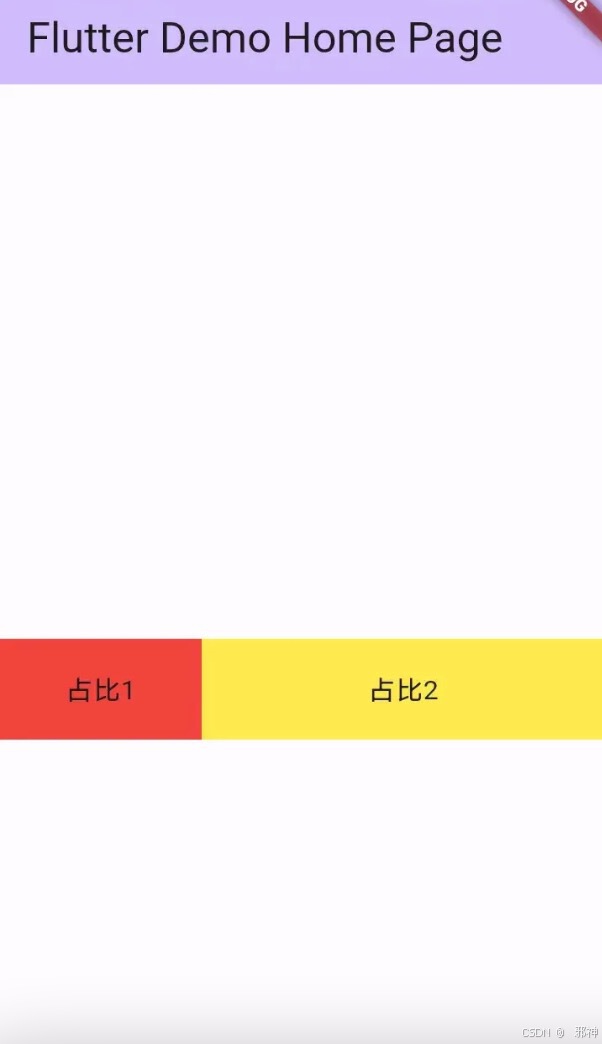
IOS Object-c 水平布局
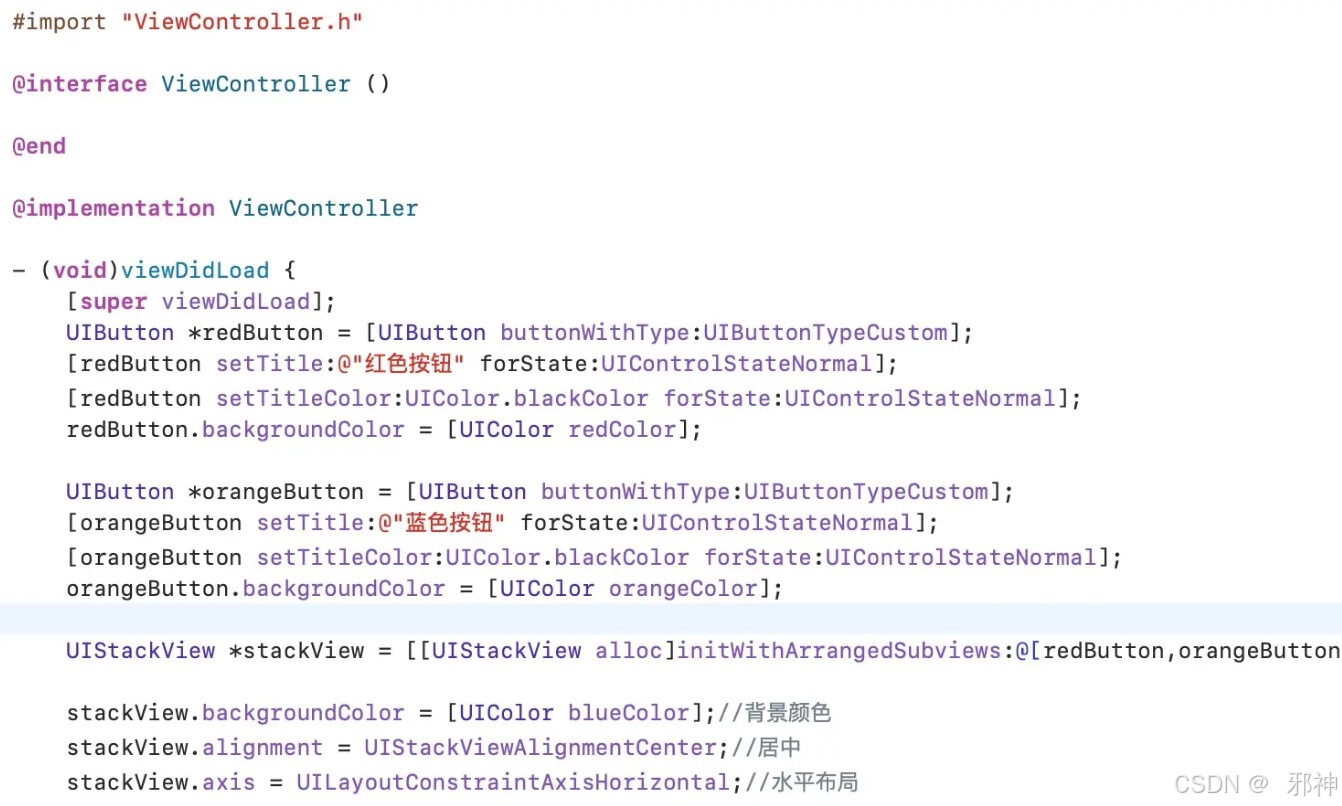
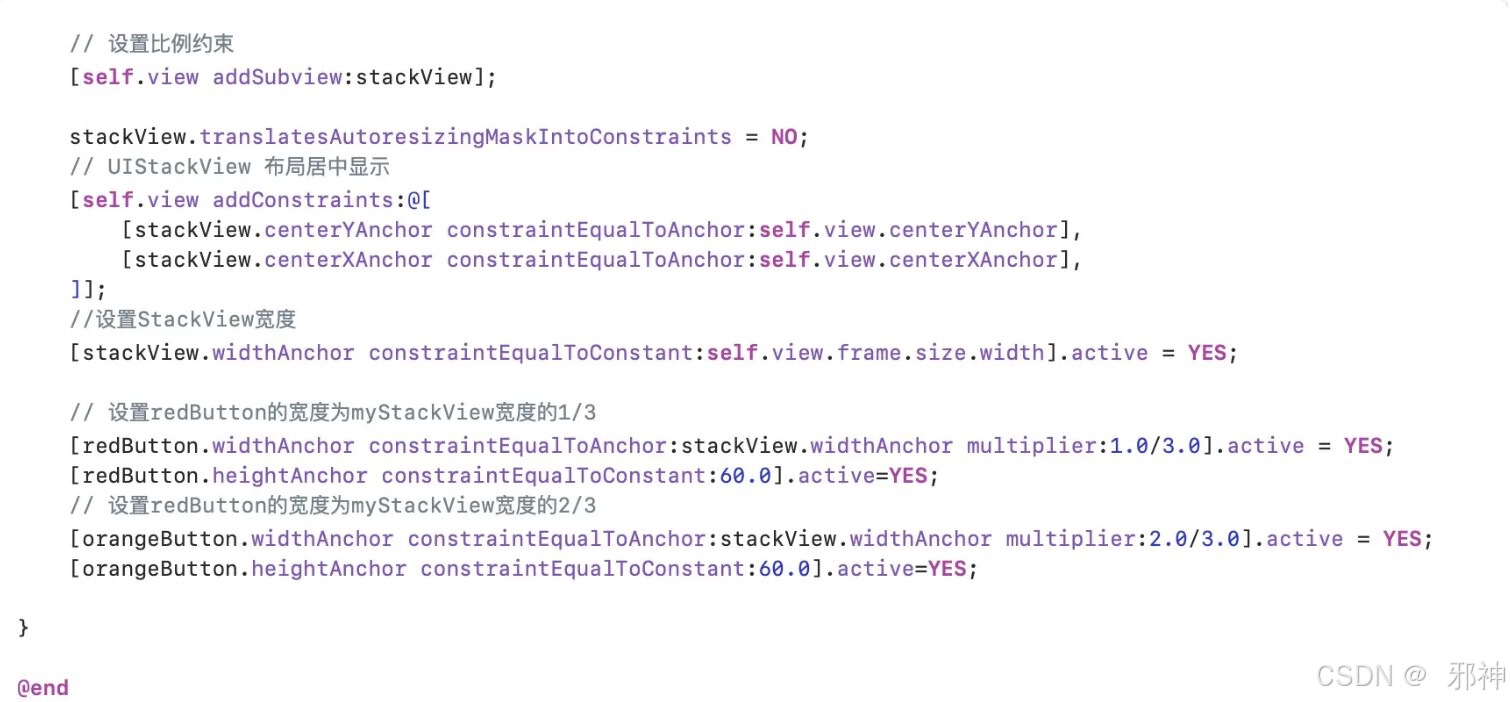
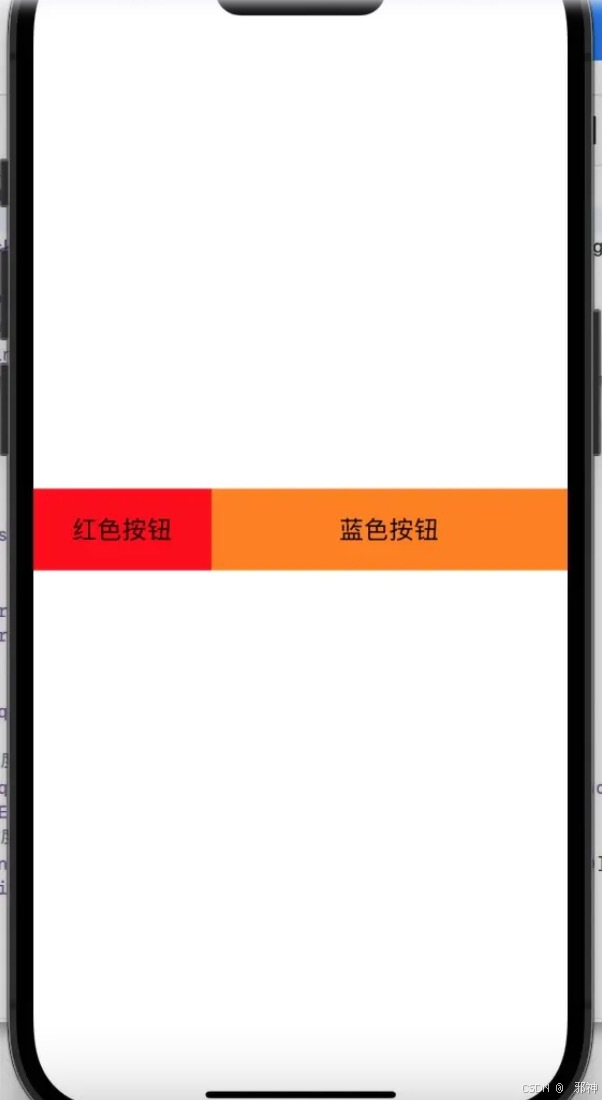
IOS Swift 水平布局
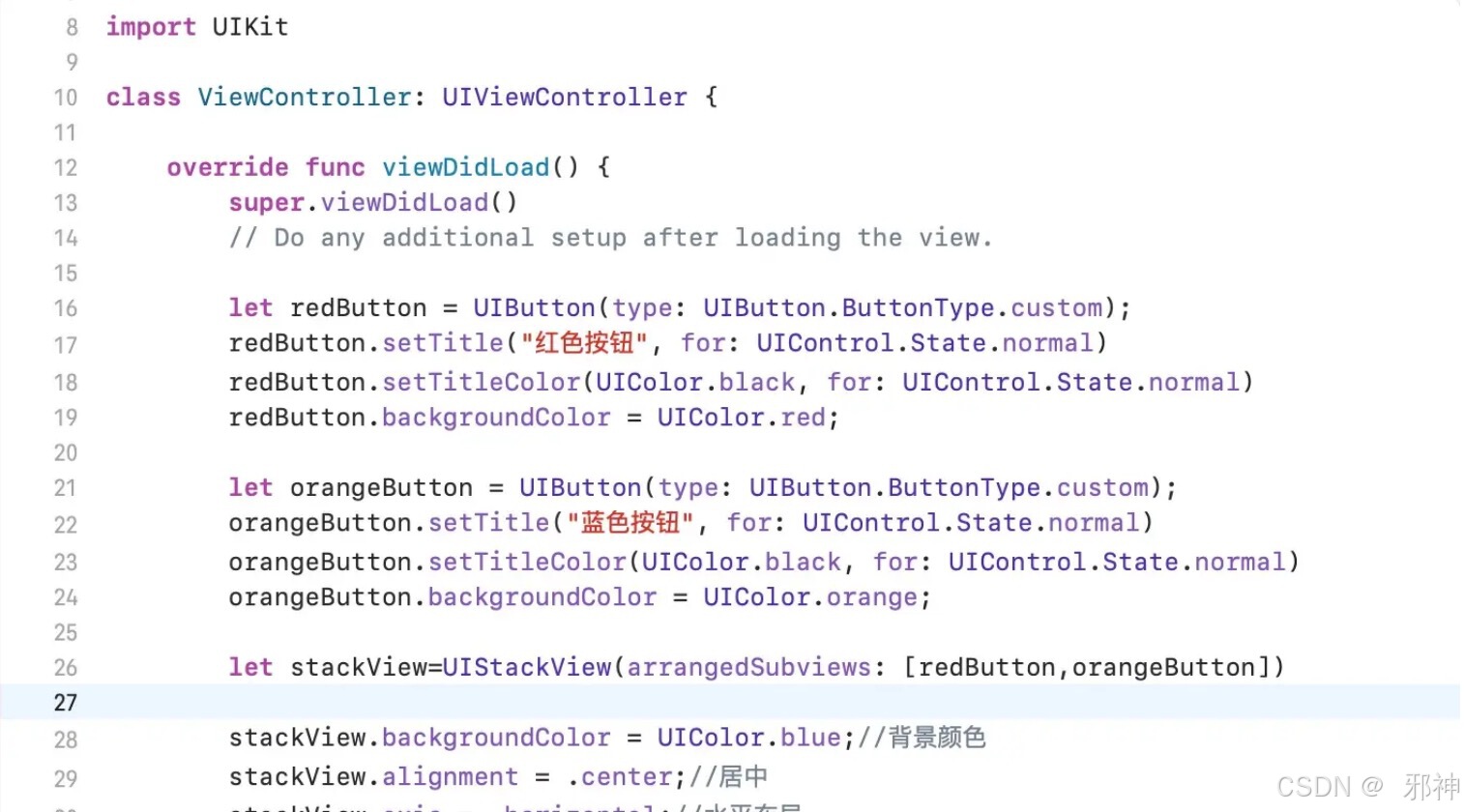
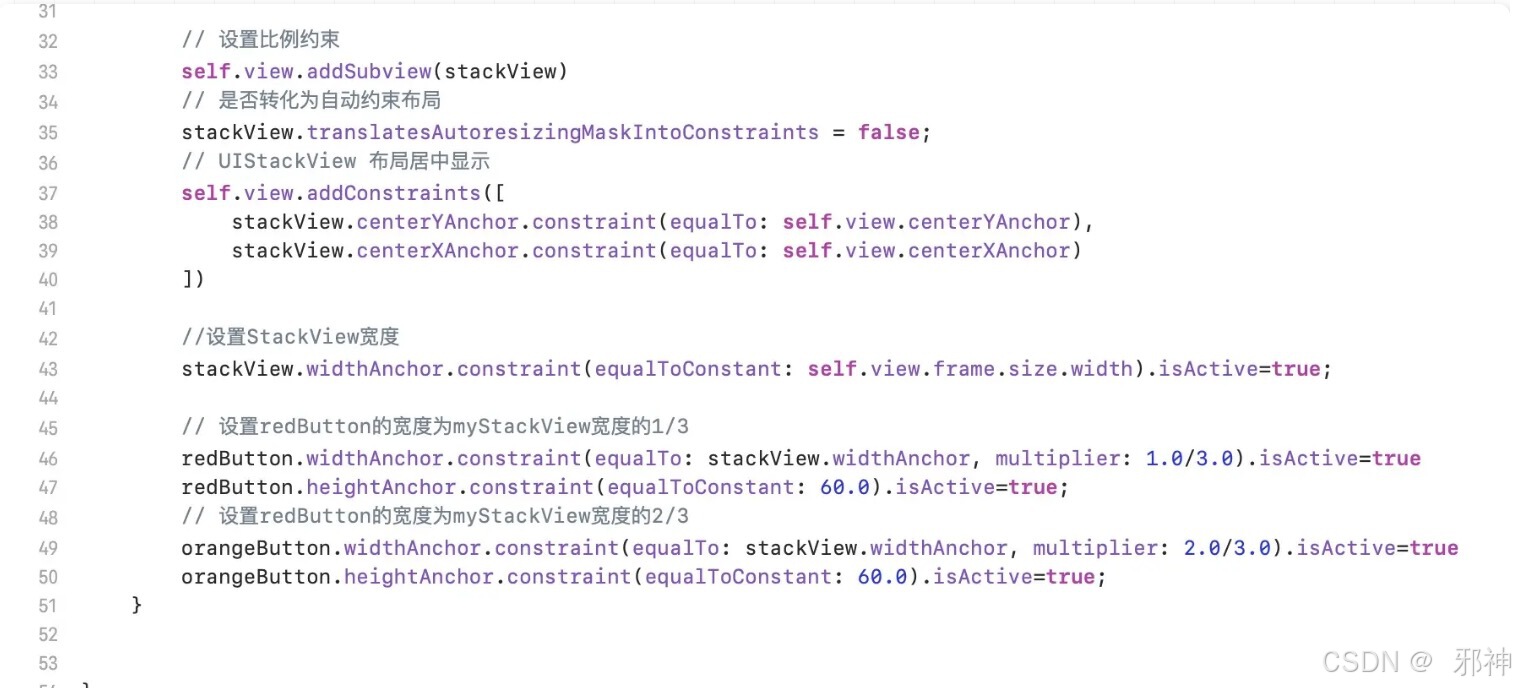
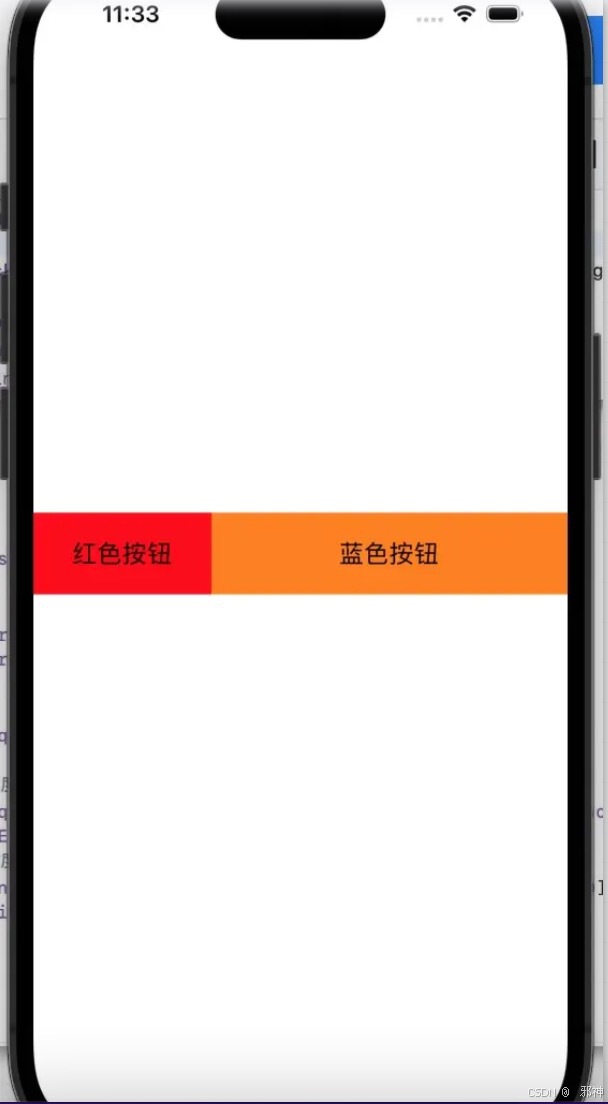
鸿蒙 水平布局
Dart
@Entry
@Component
struct Index {
@State text1: string = '占比1'
@State text2: string = '占比2'
build() {
Flex({
alignItems: ItemAlign.Center, //居中
}) {
Text(this.text1)
.width(100 / 3 + '%') // 占比1/3
.height(50)
.fontColor(Color.White)
.backgroundColor(Color.Blue)
.textAlign(TextAlign.Center) //文本居中
Text(this.text2)
.width(100 / 3 * 2 + '%') // 占比2/3
.height(50)
.fontColor(Color.Black)
.backgroundColor(Color.Yellow)
.textAlign(TextAlign.Center) //文本居中
}
.width('100%')
.height('100%')
}
}
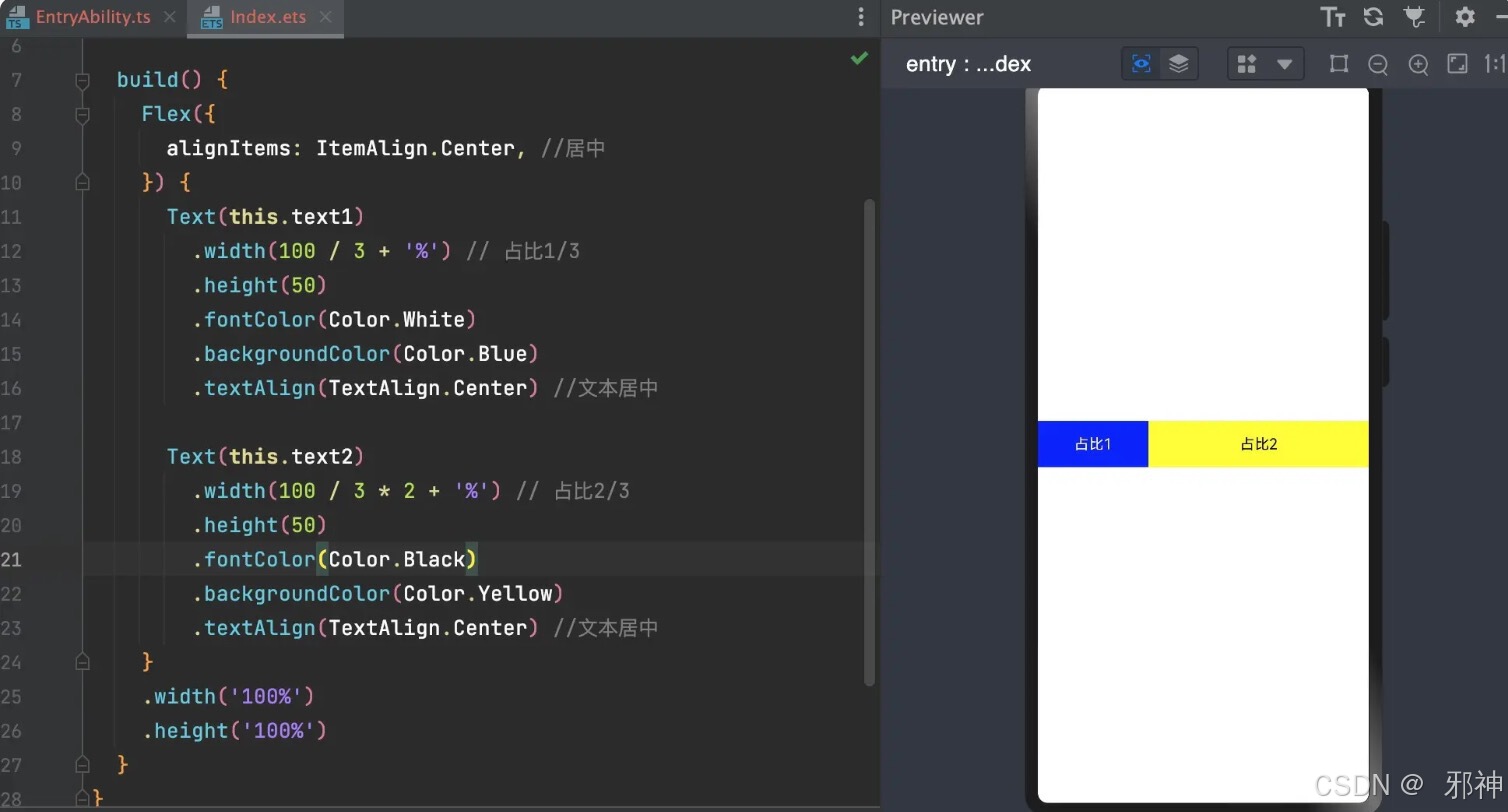
ReactNative 水平布局
Dart
import App from './App';
import {name as appName} from './app.json';
import React, { Component } from 'react';
import { AppRegistry, View,StyleSheet,Text} from 'react-native';
class RowApp extends Component {
render() {
return (
// 尝试把`flexDirection`改为`column`看看
<View style={{flex: 1, flexDirection: 'row', alignItems: 'center',}}>
<View style={styles.view1} >
<Text>占比1</Text>
</View>
<View style={styles.view2} >
<Text>占比2</Text>
</View>
</View>
);
}
};
//具体演示
const styles = StyleSheet.create({
view1:{
flex:1, //占比1
height: 60,
backgroundColor: 'red',
alignItems: 'center',//水平居中
justifyContent: 'center',//垂直居中
},
view2:{
flex:2, //占比2
height: 60,
backgroundColor: 'orange',
alignItems: 'center',//水平居中
justifyContent: 'center',//垂直居中
},
});
//注册
AppRegistry.registerComponent(appName, () => RowApp);
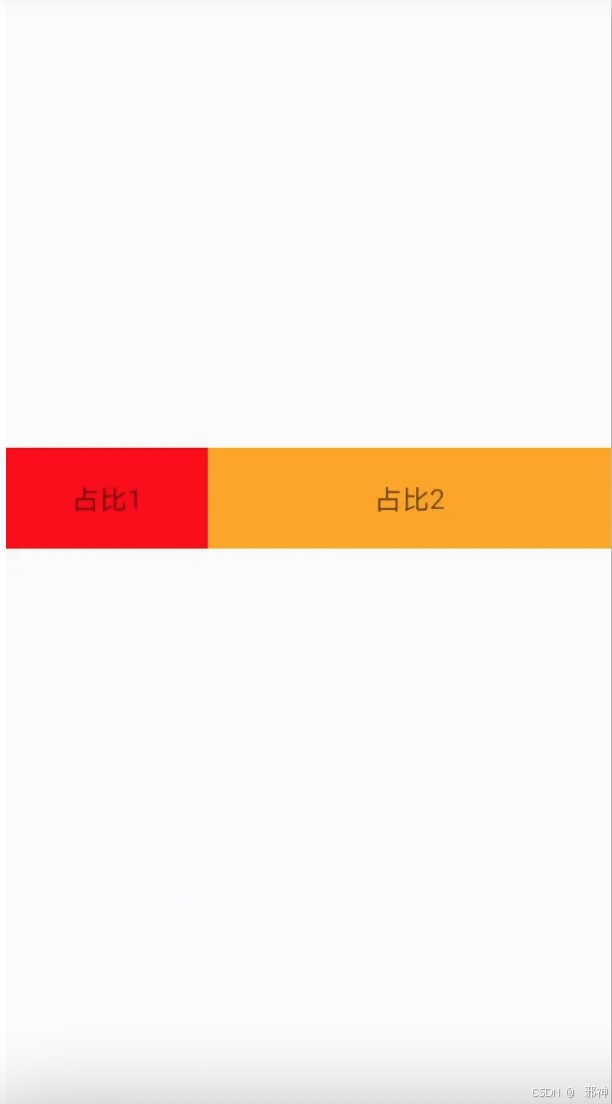
案例
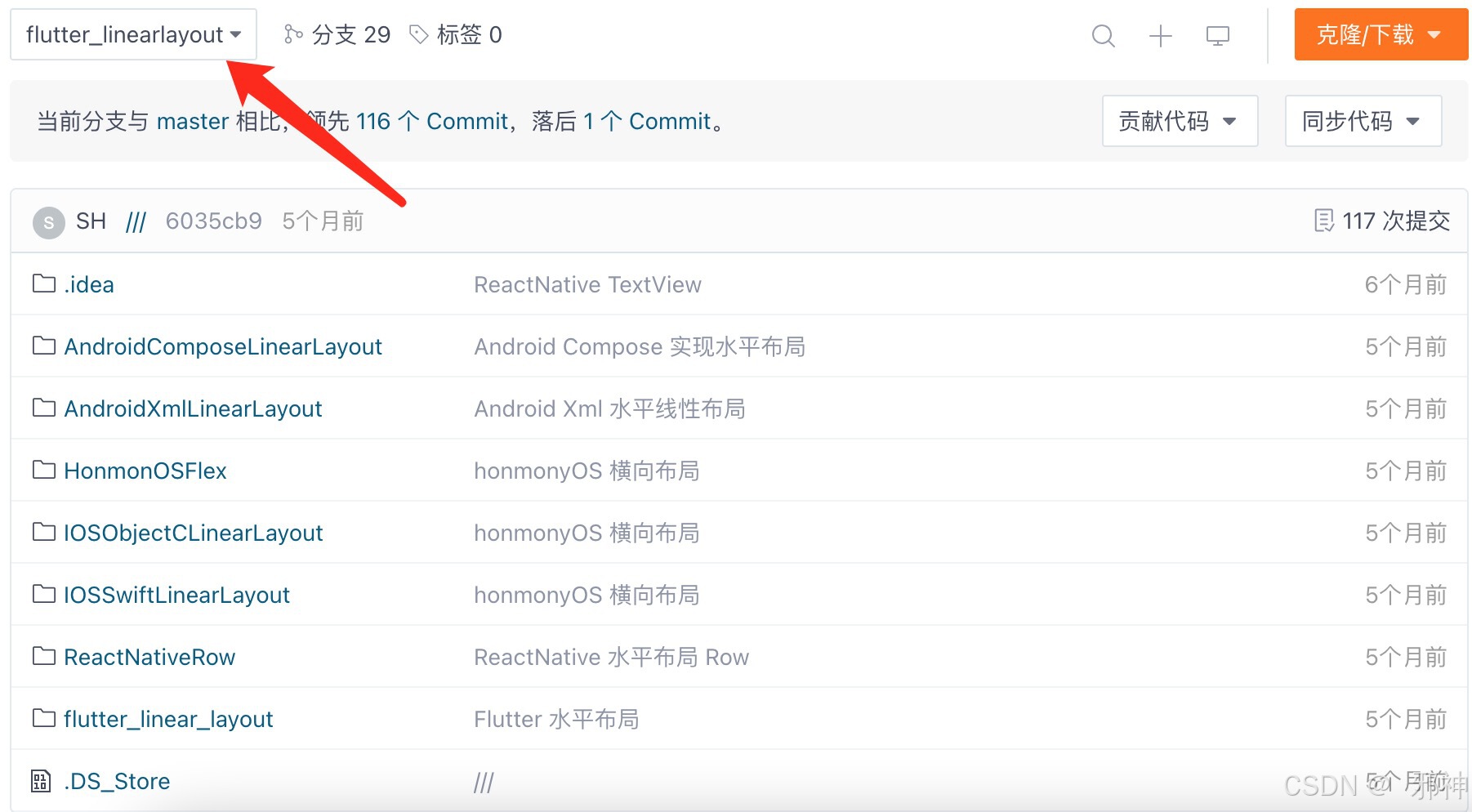