目录:
1、发起网络请求的两种方式
第一种使用httpRequest发送http的请求:
1.1、在进行网络请求前,您需要在module.json5文件中申明网络访问权限
typescript
{
"module" : {
"requestPermissions":[
{
"name": "ohos.permission.INTERNET"
}
]
}
}
1.2、GET 请求
typescript
//导入http模块
import http from '@ohos.net.http';
//创建httpRequest对象
let httpRequest = http.createHttp();
//订阅请求头(可选)
httpRequest.on('headersReceive', (header) => {
console.info('header: ' + JSON.stringify(header));
});
//发送请求
let url= "https://EXAMPLE_URL?param1=v1¶m2=v2";
let promise = httpRequest.request(
// 请求url地址
url,
{
// 请求方式
method: http.RequestMethod.GET,
// 可选,默认为60s
connectTimeout: 60000,
// 可选,默认为60s
readTimeout: 60000,
// 开发者根据自身业务需要添加header字段
header: {
'Content-Type': 'application/json'
}
});
1.3、POST请求
typescript
//导入http模块
import http from '@ohos.net.http';
//创建httpRequest对象
let httpRequest = http.createHttp();
//订阅请求头(可选)
httpRequest.on('headersReceive', (header) => {
console.info('header: ' + JSON.stringify(header));
});
//发送请求
let url = "https://EXAMPLE_URL";
let promise = httpRequest.request(
// 请求url地址
url,
{
// 请求方式
method: http.RequestMethod.POST,
// 请求的额外数据。
extraData: {
"param1": "value1",
"param2": "value2",
},
// 可选,默认为60s
connectTimeout: 60000,
// 可选,默认为60s
readTimeout: 60000,
// 开发者根据自身业务需要添加header字段
header: {
'Content-Type': 'application/json'
}
});
1.4、处理响应的结果
typescript
promise.then((data) => {
if (data.responseCode === http.ResponseCode.OK) {
console.info('Result:' + data.result);
console.info('code:' + data.responseCode);
}
}).catch((err) => {
console.info('error:' + JSON.stringify(err));
});
第二种使用axios发送http的请求:
1.1、在进行网络请求前,您需要在module.json5文件中申明网络访问权限
typescript
{
"module" : {
"requestPermissions":[
{
"name": "ohos.permission.INTERNET"
}
]
}
}
1.2、安装axios
typescript
ohpm install @ohos/axios
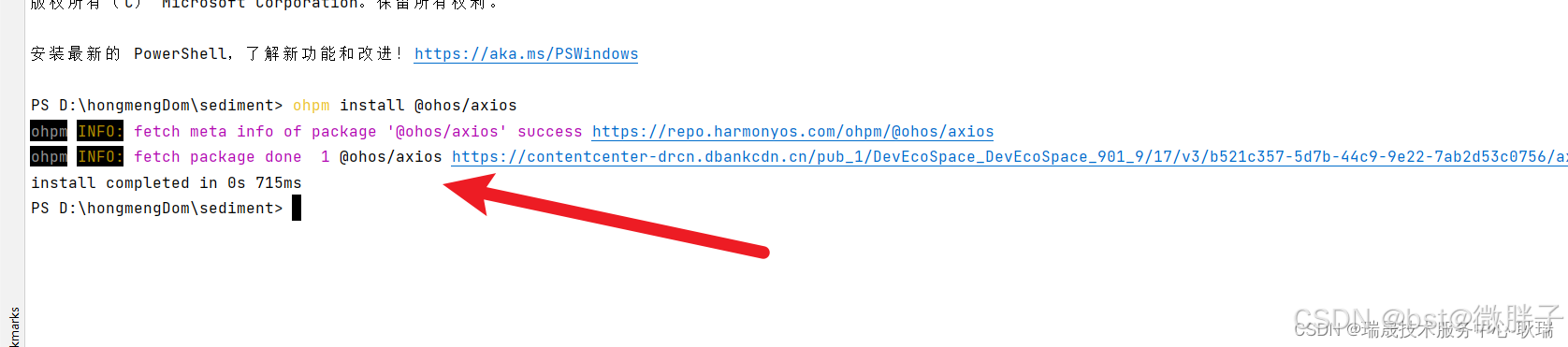
1.3、使用axios发送请求
typescript
import axios from "@ohos/axios";
@Entry
@Component
struct Dom {
aboutToAppear() {
axios.get(
"http://localhost/books",
).then(res =>{
let data:string = JSON.stringify(res);
console.log(data);
}).catch(err=> {
console.log("请求失败");
})
}
build() {
Column({space: 30}) {
}
.width('100%')
.height('100%')
}
}
2、异步的调用
typescript
// 假设你有一个网络请求的函数,例如使用fetch API
function fetchData(url) {
return new Promise((resolve, reject) => {
fetch(url).then(response => {
if (response.ok) {
response.json().then(data => resolve(data)).catch(error => reject(error));
} else {
reject(new Error('Network response was not ok.'));
}
}).catch(error => reject(error));
});
}
// 使用Promise进行异步请求
fetchData('https://your-api.com/data').then(data => {
// 处理响应数据
console.log(data);
}).catch(error => {
// 处理错误
console.error(error);
});
3、Promise.all()的用法
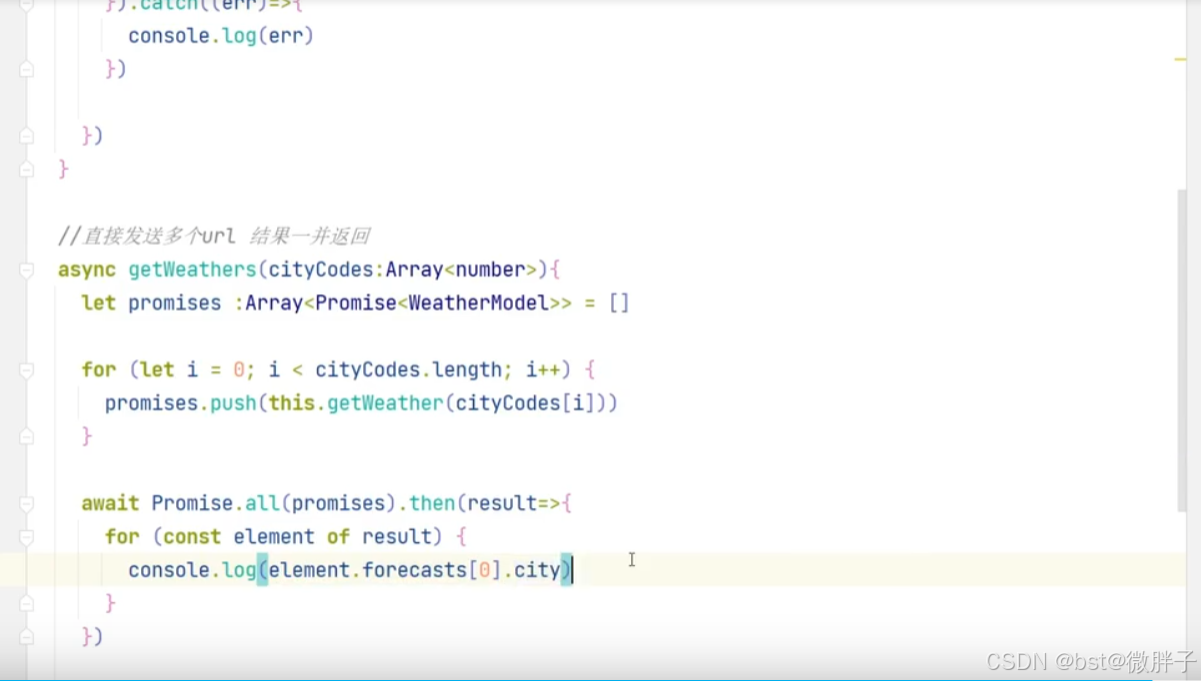
这里示例this.getWeather返回一个promise后,然后被push到数组promises中,Promise.all()方法处理数组promises,一次处理多个promise函数,后端返回的数据,前端也要通过相同的数据结构去接收。