前言
EXCEL管理数据
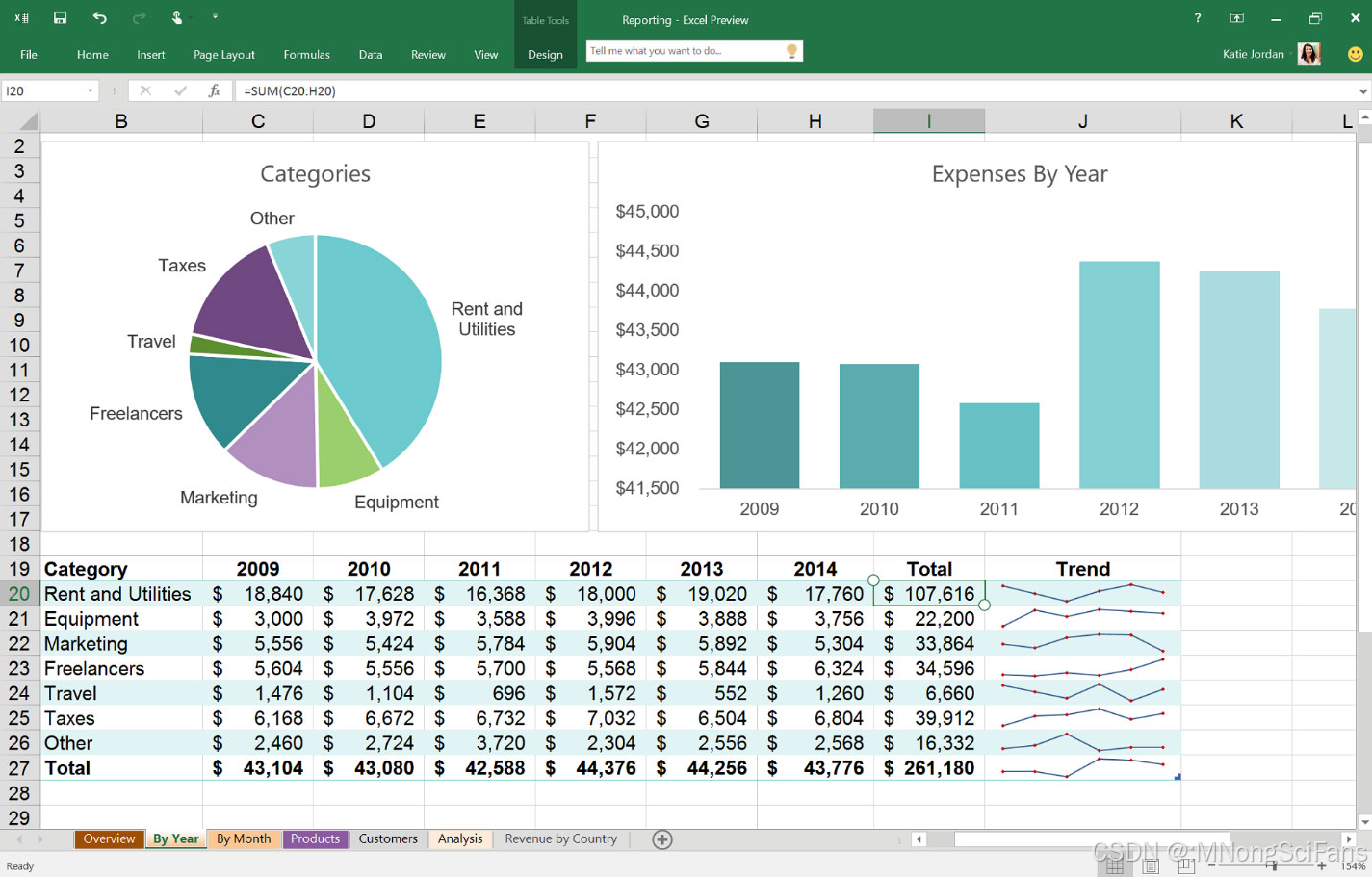
Bootstrap
Bootstrap 是一个流行的开源前端框架,它由 Twitter 的员工开发,用于快速开发响应式和移动设备优先的网页和应用程序。
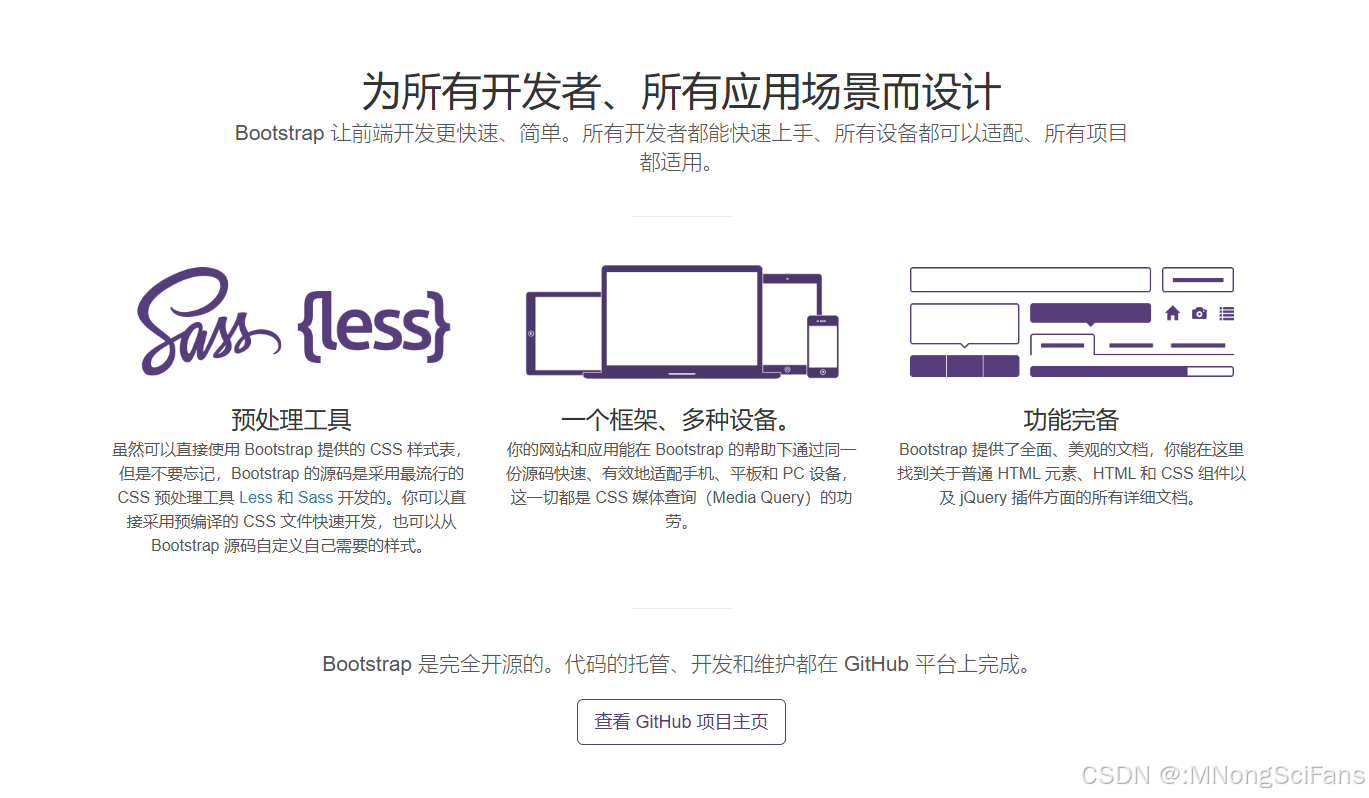
jQuery
jQuery 是一个快速、小巧且功能丰富的 JavaScript 库。它简化了 HTML 文档的遍历、事件处理、动画和 Ajax 交互,使得 Web 开发更加快捷。
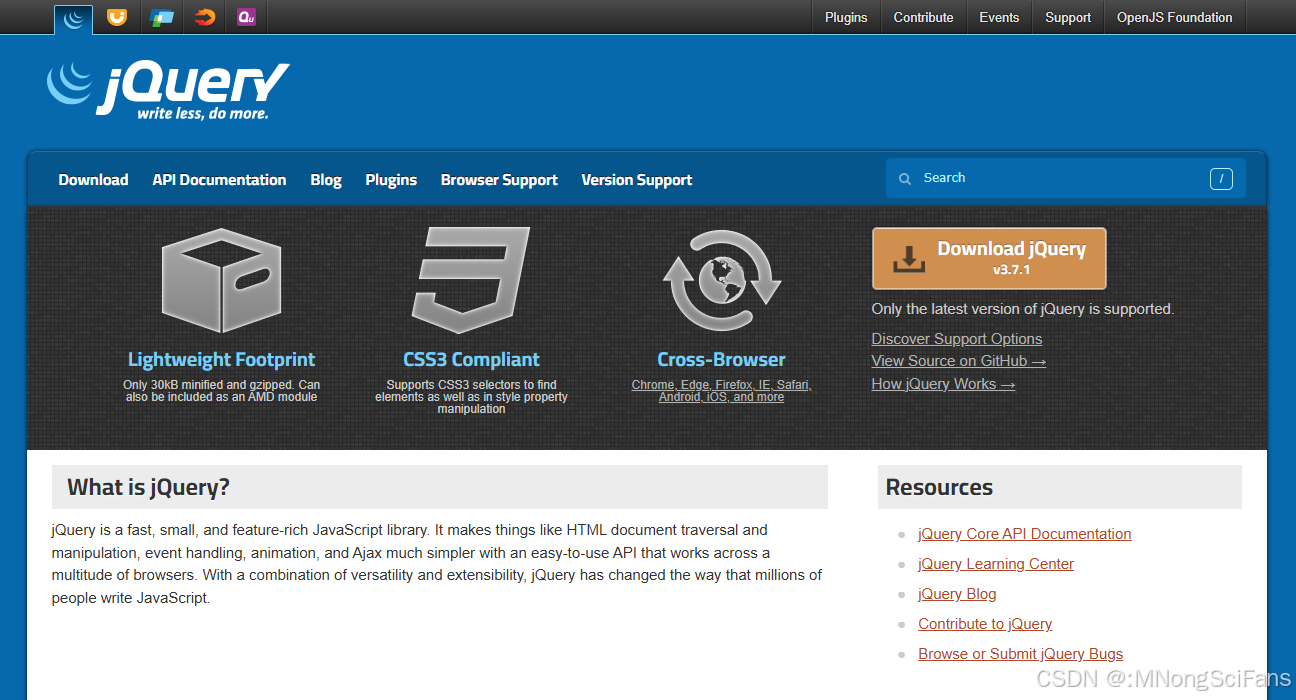
Bootstrap 和 jQuery 常常一起使用,jQuery 可以帮助开发者更容易地在 Bootstrap 构建的页面上实现复杂的交互效果。然而,随着现代前端框架(如 React、Vue 和 Angular)的兴起,jQuery 的使用逐渐减少,而 Bootstrap 也推出了专门为这些框架设计的版本,如 BootstrapVue 和 React-Bootstrap。
首先用table标签制作简单的表格
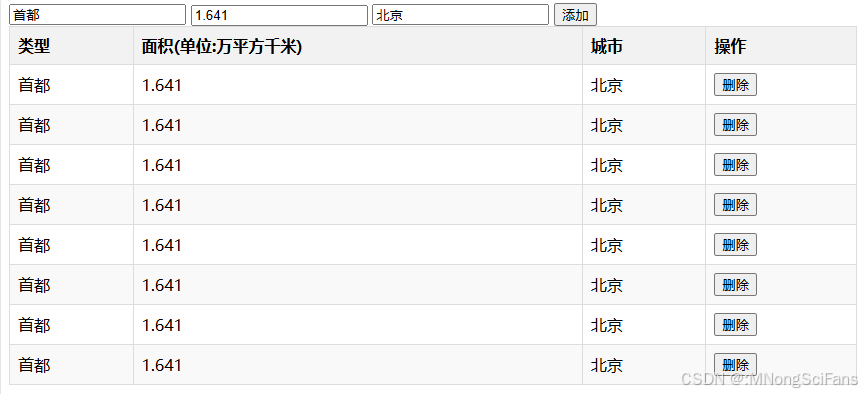
实现HTML\CSS\JS代码
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Web Table 实战示例</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
cursor: pointer;
}
tr:nth-child(even) {
background-color: #f9f9f9;
}
</style>
<script>
function sortTable(n) {
var table, rows, switching, i, x, y, shouldSwitch, dir, switchcount = 0;
table = document.getElementById("myTable");
switching = true;
// 设置排序方向,默认为升序
dir = "asc";
// 进行循环,直到没有更多需要交换的行
while (switching) {
switching = false;
rows = table.rows;
// 遍历所有行,除了第一行(表头)
for (i = 1; i < (rows.length - 1); i++) {
shouldSwitch = false;
// 获取当前行和下一行的指定列
x = rows[i].getElementsByTagName("TD")[n];
y = rows[i + 1].getElementsByTagName("TD")[n];
// 检查两个值,根据排序方向进行交换
if (dir == "asc") {
if (x.innerHTML.toLowerCase() > y.innerHTML.toLowerCase()) {
shouldSwitch = true;
break;
}
} else if (dir == "desc") {
if (x.innerHTML.toLowerCase() < y.innerHTML.toLowerCase()) {
shouldSwitch = true;
break;
}
}
}
if (shouldSwitch) {
// 如果找到需要交换的行,则交换它们,并标记交换了行
rows[i].parentNode.insertBefore(rows[i + 1], rows[i]);
switching = true;
// 每次交换后增加计数器
switchcount++;
} else {
// 如果没有交换,检查是否需要改变排序方向
if (switchcount == 0 && dir == "asc") {
dir = "desc";
switching = true;
}
}
}
}
function addRow() {
var type = document.getElementById("type").value;
var area = document.getElementById("area").value;
var city = document.getElementById("city").value;
var table = document.getElementById("myTable").getElementsByTagName('tbody')[0];
// 验证年龄是否为数字
if (isNaN(area) || area === "") {
alert("面积必须是数字!");
return;
}
// 验证城市是否为空
if (city === "") {
alert("城市不能为空!");
return;
}
// 创建新的行和单元格
var newRow = table.insertRow(table.rows.length);
var cell1 = newRow.insertCell(0);
var cell2 = newRow.insertCell(1);
var cell3 = newRow.insertCell(2);
var cell4 = newRow.insertCell(3);
// 添加数据到单元格
cell1.innerHTML = type;
cell2.innerHTML = area;
cell3.innerHTML = city;
cell4.innerHTML = '<button onclick="deleteRow(this)">删除</button>';
// 清空输入框
//document.getElementById("type").value = "";
//document.getElementById("area").value = "";
//document.getElementById("city").value = "";
}
function deleteRow(btn) {
var row = btn.parentNode.parentNode;
row.parentNode.removeChild(row);
}
</script>
</head>
<body>
<div>
<input value="首都" id="type"/>
<input value="1.641" id="area"/>
<input value="北京" id="city"/>
<button onclick="addRow()">添加</button>
</div>
<table id="myTable">
<thead>
<tr>
<th onclick="sortTable(0)">类型</th>
<th onclick="sortTable(1)">面积(单位:万平方千米)</th>
<th onclick="sortTable(2)">城市</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!--<tr>
<td id="type">首都</td>
<td id="area">1.641</td>
<td id="city">北京</td>
<td><button onclick="deleteRow(this)">删除</button></td>
</tr>-->
<!-- 更多行数据 -->
</tbody>
</table>
</body>
</html>
升级为bootstrap+jquery依赖的高级表格
html
<!DOCTYPE html>
<html lang="zh-CN" xmlns:th="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>水厂工作簿</title>
<style>
body {
padding: 0;
margin: 0;
font: normal 14px/1.42857 Tahoma;
}
.selector-for-some-widget {·
box-sizing: content-box;
}
@media print {
.container {
width: auto;
}
}
.itemArea{
list-style: none;
display: flex;
}
.bootstrap-table .fixed-table-container.fixed-height .table thead th {
background: #f5f7fa;
}
</style>
<!-- HTML5 Shiv 和 Respond.js 用于让 IE8 支持 HTML5元素和媒体查询 -->
<!-- 注意: 如果通过 file:// 引入 Respond.js 文件,则该文件无法起效果 -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
<!-- cdn引入样式和js库 -->
<link href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/css/bootstrap.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/bootstrap-table.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-icons/1.11.0/font/bootstrap-icons.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/font-awesome/6.4.2/css/all.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/font-awesome/6.4.2/css/fontawesome.min.css" rel="stylesheet" crossorigin="anonymous">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.7.1/jquery.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/js/bootstrap.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/js/bootstrap.bundle.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/bootstrap-table.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/locale/bootstrap-table-zh-CN.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/js/bootstrap-datepicker.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/locales/bootstrap-datepicker.zh-CN.min.js" crossorigin="anonymous"></script>
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/css/bootstrap-datepicker.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/css/bootstrap-datepicker3.min.css" rel="stylesheet" crossorigin="anonymous">
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/extensions/export/bootstrap-table-export.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/moment.js/2.29.4/moment.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/moment.js/2.29.4/locale/zh-cn.min.js" crossorigin="anonymous"></script>
</head>
<body>
</body>
</html>
水务业务场景概览
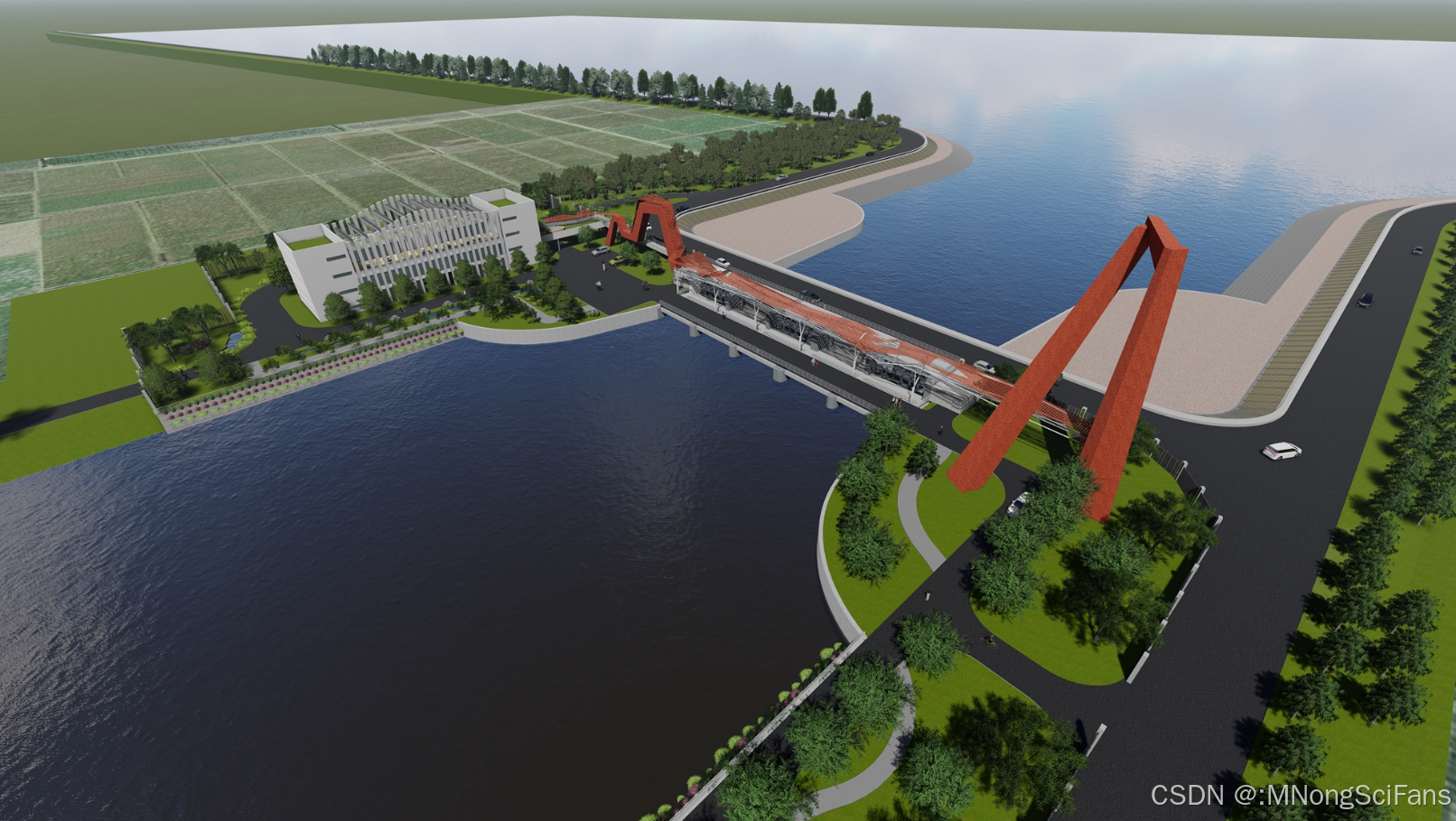
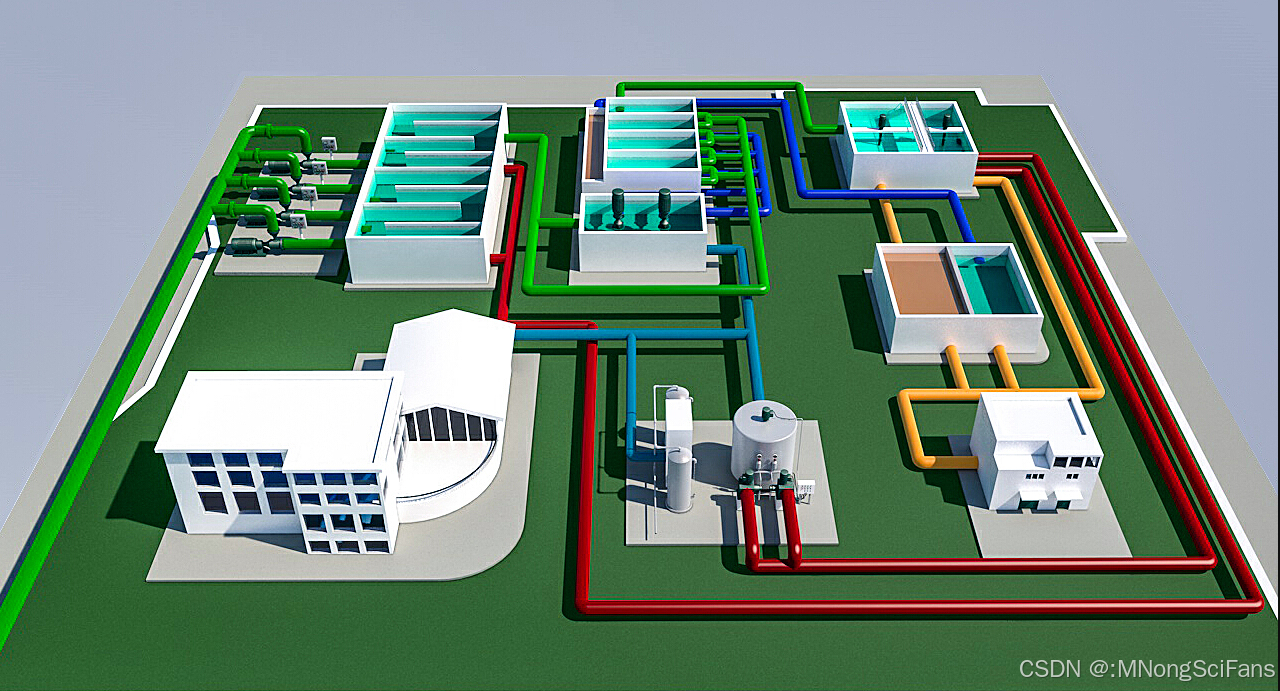
增加WEB界面
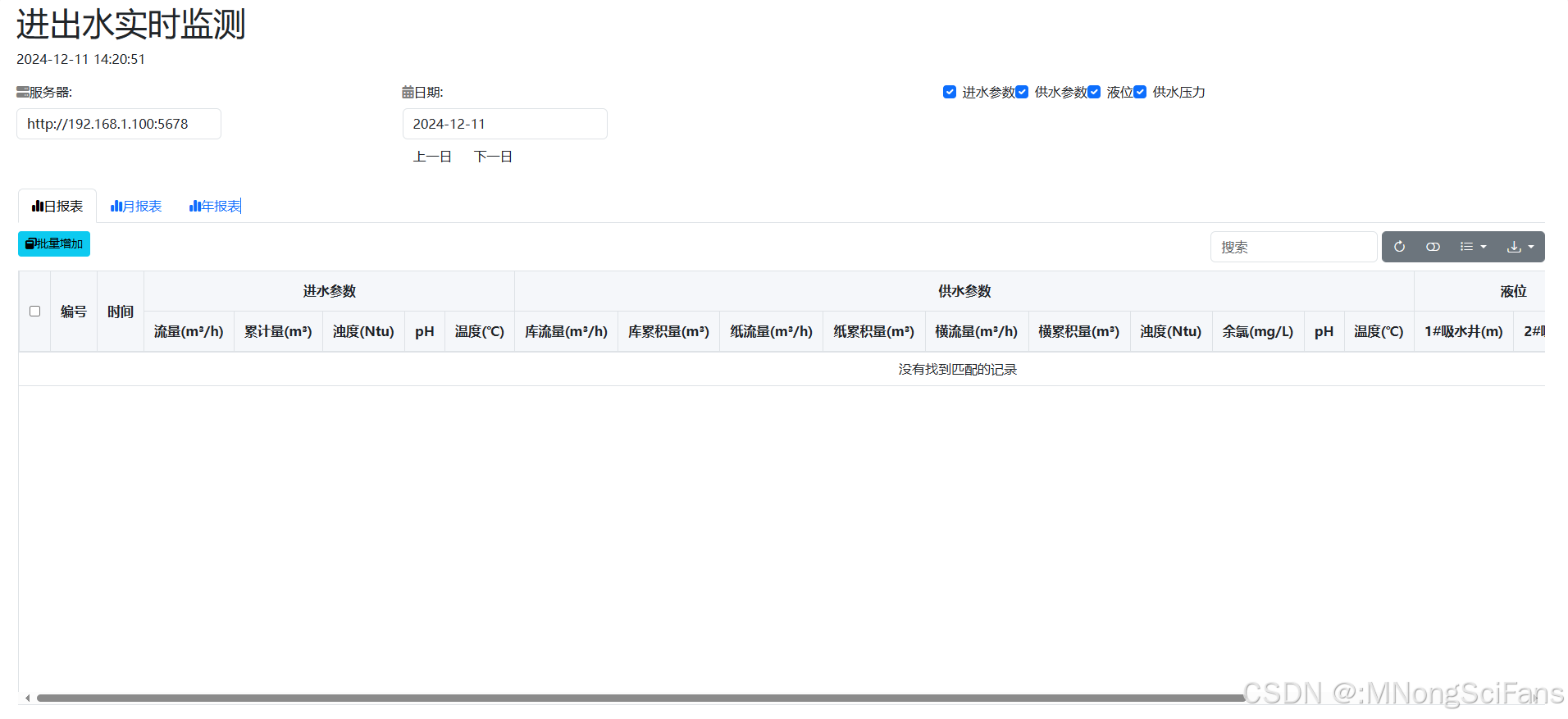
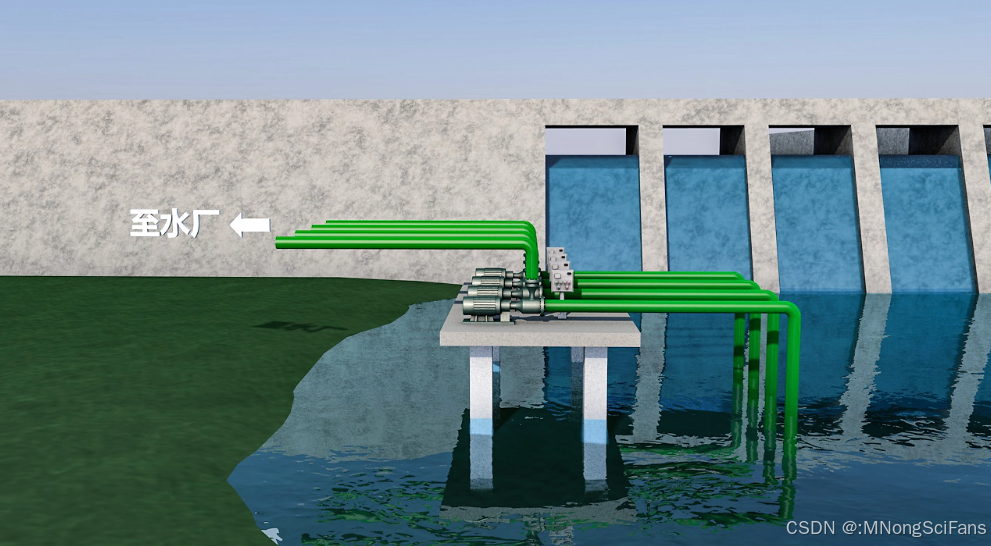
批量数据生成
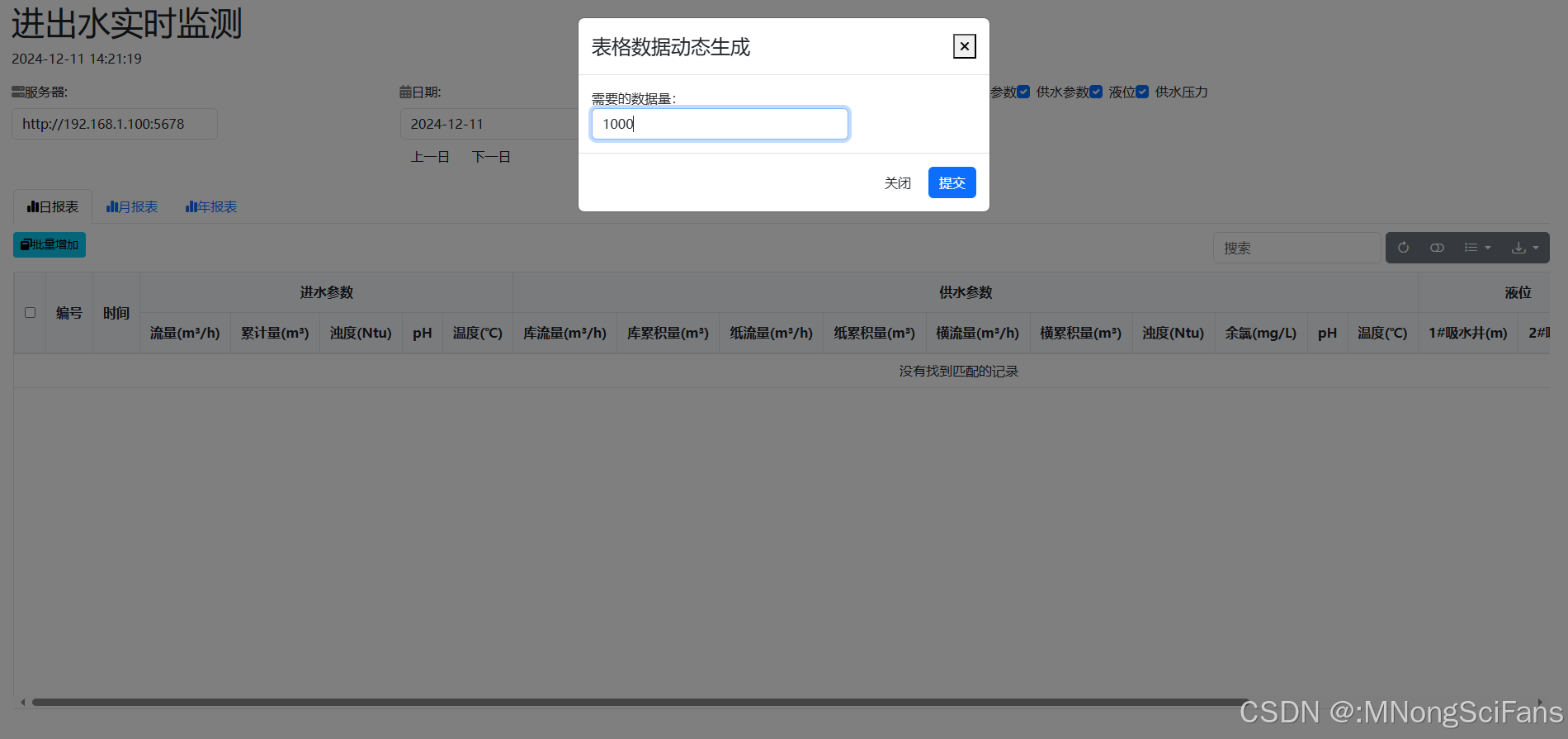
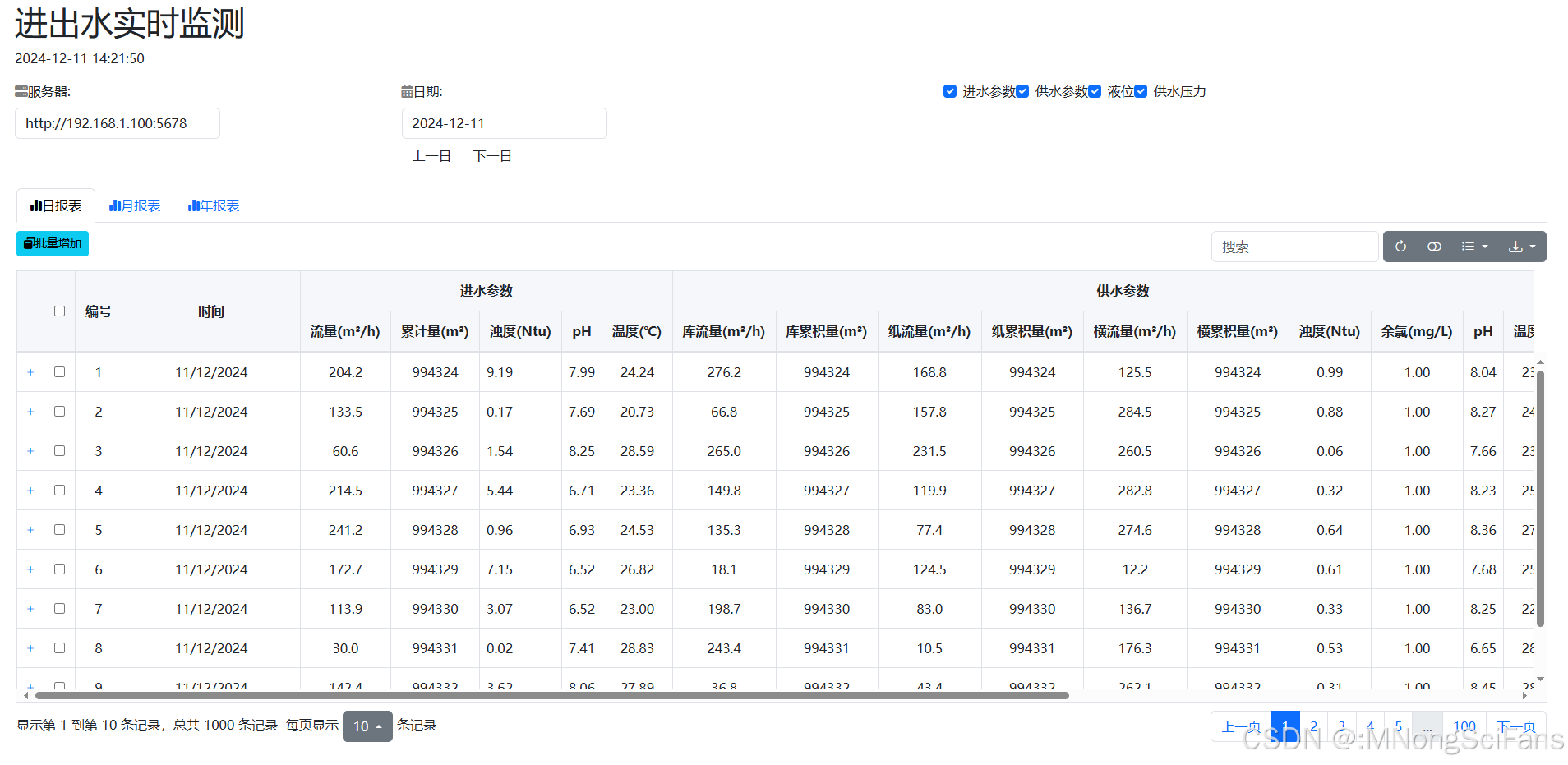
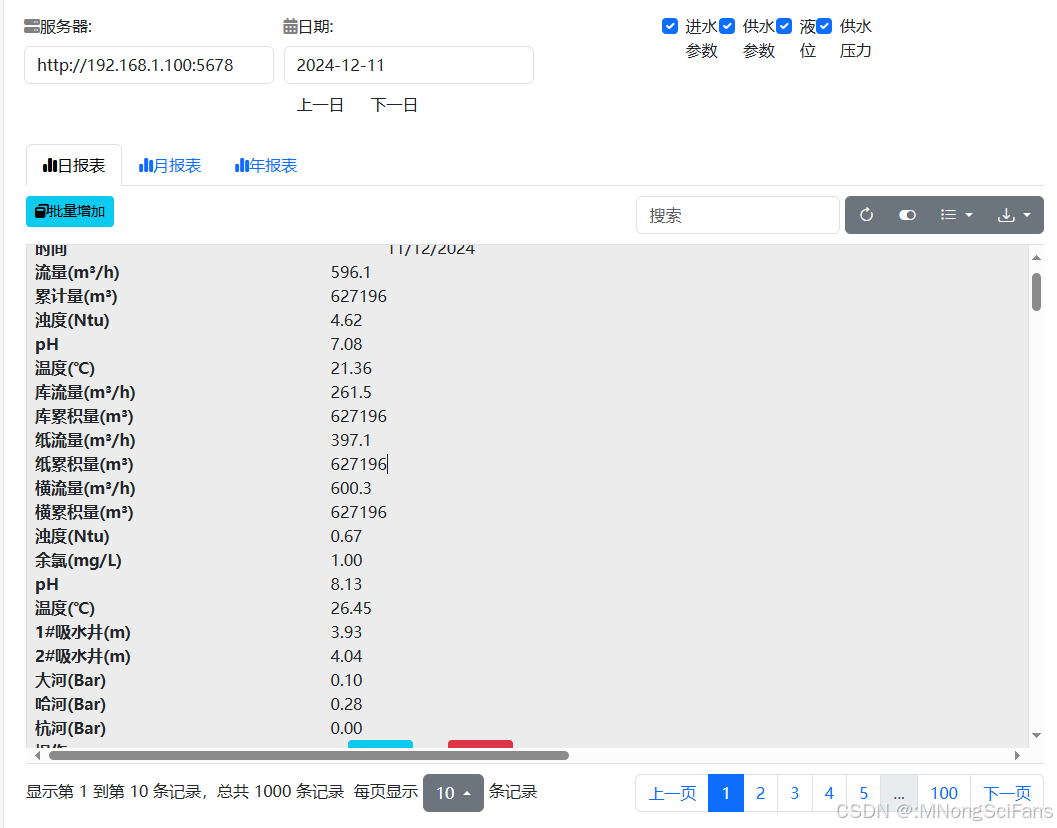
WEB界面代码
html
<div id="app" class="container" style="margin: 10px; height: calc(100vh - 20px);width: calc(100vw - 50px);">
<!-- Content here -->
<div class="wrapper wrapper-content" style="width: calc(100vw - 50px);">
<div class="row">
<div class="col-sm-12" style="padding: 0 10px;">
<h1>进出水实时监测</h1>
</div>
</div>
<div class="row">
<div class="col-sm-12" style="padding: 0 10px;">
<div><p id="time"></p></div>
<div id="dateSearch">
<div class="row g-3 needs-validation" novalidate>
<div class="col-md-3" >
<label for="ip-port" class="form-label"><i class="fa fa-server" aria-hidden="true" style="color:gray"></i>服务器: </label>
<input type="text" class="form-control" id="ip-port" name="ip-port" style="width:250px;" placeholder="服务IP:端口" value="http://192.168.1.100:5678" required>
<div class="valid-feedback"></div>
</div>
<div class="col-md-4">
<label for="startDate" class="form-label"><i class="fa fa-calendar-days" style="color:gray"></i>日期: </label>
<input type="text" class="form-control" id="startDate" name="startDate" style="width:250px;" placeholder="日期" value="" data-format="yyyy-MM-dd" required>
<div id="date-operate"></div>
<div class="valid-feedback"></div>
</div>
<div class="col-md-3">
<ul class="itemArea" >
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b01">
<input class="form-check-input" type="checkbox" checked="" data-code="B01" value="" id="b01">
进水参数
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b02">
<input class="form-check-input" type="checkbox" checked="" data-code="B02" value="" id="b02">
供水参数
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b03">
<input class="form-check-input" type="checkbox" checked="" data-code="B03" value="" id="b03">
液位
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b04">
<input class="form-check-input" type="checkbox" checked="" data-code="B04" value="" id="b04">
供水压力
</label>
</div>
</li>
</ul>
</div>
<div class="col-md-2" style="display: flex; justify-content: right;">
</div>
</div>
</div>
</div>
</div>
<div class="row" id="infoArea">
<div class="col-sm-12">
<ul class="nav nav-tabs" id="navList" role="tablist" style="margin: 20px 0 0 0;">
<li class="nav-item" data-name = "Tab1" onclick="getData('day')">
<a class="nav-link active" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-chart-simple"></i>日报表</a>
</li>
<li class="nav-item" data-name = "Tab2" onclick="getData('month')">
<a class="nav-link" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-chart-simple"></i>月报表</a>
</li>
<li class="nav-item" data-name = "Tab3" onclick="getData('year')">
<a class="nav-link" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-chart-simple"></i>年报表</a>
</li>
</ul>
<div class="tab-content" id="tabContent">
<div id="toolbar" class="btn-group">
<button class="btn btn-info btn-sm " data-bs-toggle="modal" data-bs-target="#myModal" >
<i class="fa fa-window-restore"></i>批量增加
</button>
</div>
<div id="reportTab" class="tab-pane fade show active" id="nav-home" role="tabpanel" aria-labelledby="nav-home-tab">
<table id="report-table"></table>
</div>
</div>
</div>
</div>
</div>
</div>
表格DOM挂载点
html
<div id="reportTab" class="tab-pane fade show active" id="nav-home" role="tabpanel" aria-labelledby="nav-home-tab">
<table id="report-table"></table>
</div>
批量生成数据的对话框
html
<!-- 模态框(Modal) -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h4 class="modal-title" id="myModalLabel">
表格数据动态生成
</h4>
<button type="button" class="close" data-bs-dismiss="modal" aria-hidden="true">
<span class="fa fa-close" aria-hidden="true"></span>
</button>
</div>
<div class="modal-body">
<div class="wrapper wrapper-content animated fadeInRight ibox-content">
<form class="form-horizontal m" id="form-madArea-add">
<div class="form-group">
<label class="col-sm-3 control-label " for="number">需要的数据量:</label>
<div class="col-sm-8">
<input class="form-control" type="text" id="number" name="number" value="1000">
</div>
</div>
<!--<div class="col-sm-12">
<div class="form-group">
<div class="pull-right">
<button id="button_preservation" type="button" class="btn btn-success">保存</button>
<button id="button_cancel" type="button" class="btn btn-warning">取消</button>
</div>
</div>
</div>-->
</form>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-bs-dismiss="modal">关闭</button>
<button type="button" class="btn btn-primary" onclick="submitFun()">提交</button>
</div>
</div>
<!-- /.modal-content -->
</div><!-- /.modal -->
</div>
界面数据逻辑的js代码
html
<script>
function formatDate(date) {
const day = date.getDate().toString().padStart(2, '0');
const month = (date.getMonth() + 1).toString().padStart(2, '0'); // 月份是从0开始的
const year = date.getFullYear();
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
const seconds = date.getSeconds().toString().padStart(2, '0');
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
}
let pid1
pid1 = setInterval(()=>{
// 使用示例
const now = new Date()
// 输出格式如: "2023-03-15 12:30:45"
document.getElementById('time').innerHTML = formatDate(now)
},0)
let myWorker
document.querySelector('#number').onchange = function() {
console.log('数据量:',document.querySelector('#number').value);
}
function startWorker() {
let message = {
num: document.querySelector('#number').value,
urls: ['./local.json', './local.json']
}
if (typeof Worker !== 'undefined') {
if(typeof(myWorker) == "undefined") {
// 支持Web worker 创建一个新的Web Worker
const blob = new Blob([document.getElementById('worker').textContent]);
const url = window.URL.createObjectURL(blob)
myWorker = new Worker(url);
}
// 定义一个函数来处理来自工作线程的消息
myWorker.onmessage = function(e) {
console.log('Message received from worker');
const res = e.data;
if(res.length==message.num){
tableData = res||[];
initTable(res);
}else{
console.log(res);
}
}
// 向worker发送消息以启动计算 启动请求
myWorker.postMessage(message);
} else {
// 不支持
console.log('Web Workers are not supported in this browser.');
console.error("抱歉,你的浏览器不支持 Web Workers...");
}
}
function stopWorker() {
if(typeof(myWorker) != "undefined") {
myWorker.terminate();
myWorker = undefined;
}
if(pid1){
clearInterval(pid1);
}
}
try {
//run(main)
}
catch(err) {
console.log(err)
}
finally {
console.log('finally done')
}
var queryParams = function (params) {
var param = {
pageIndex: Math.ceil(params.offset / params.limit) + 1,
pageSize: params.limit,
order: params.order,
ordername: params.sort,
time: $("#dateSearch .startDate").val(),
//startDateTime: $("#dateSearch .startDate").val(),
//endDateTime: $("#dateSearch .endDate").val(),
search: $("#dateSearch .imuserid").val()
};
//console.log(param)
return param;
}
//查询条件
function queryParams1(params){
params['project_id'] = $("select[name=project_id]").find("option:selected").val();
params['time_field'] = $("select[name=time_field]").find("option:selected").val();
params['start_time'] = $("input[name=start_time]").val();
params['end_time'] = $("input[name=end_time]").val();
params['user_name'] = $("input[name=user_name]").val();
params['telephone'] = $("input[name=telephone]").val();
params['room_confirm_number'] = $("input[name=room_confirm_number]").val();
params['lineson'] = $("select[name=lineson]").val();
params['invoice'] = $("select[name=invoice]").val();
return params;
}
var responseHandler = function (e) {
if (e.data && e.data.length > 0) {
return { "rows": e.data, "total": e.count };
}
else {
return { "rows": [], "total": 0 };
}
}
var uidHandle = function (value, row, index) {
var html = "<a href='#'>"+ res + "</a>";
return html;
}
var operateFormatter = function (value, row, index) {//赋予的参数
return [
'<div style="width: 200px;display: flex;justify-content: space-around;">',
'<button class="btn btn-info btn-sm rightSize detailBtn" type="button"><i class="fa fa-paste"></i> 详情</button>',
'<button class="btn btn-danger btn-sm rightSize packageBtn" type="button"><i class="fa fa-envelope"></i> 通知</button>',
'</div>'
].join('');
}
var rowStyle = function (row, index) {
var classes = ['success', 'info'];
if (index % 2 === 0) {//偶数行
return { classes: classes[0]};
} else {//奇数行
return {classes: classes[1]};
}
}
const tableName = "report-table";
var tableType = 'day'
function selectType(){
const date = $('#startDate').datepicker('getDate');
let time = ''
if(tableType=='day'){
time = date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate();
}else if(tableType=='month'){
time = date.getFullYear() + "-" + (date.getMonth() + 1);
}else if(tableType=='year'){
time = date.getFullYear();
}
return time
}
function reportName(){
let name = ''
let time = ''
const date = $('#startDate').datepicker('getDate');
if(tableType=='day'){
name = '日报表'
time = date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate();
}else if(tableType=='month'){
name = '月报表'
time = date.getFullYear() + "-" + (date.getMonth() + 1);
}else if(tableType=='year'){
name = '年报表'
time = date.getFullYear();
}
return name + '_' + time
}
function searchReport(){
buildTableColumns(); //根据因子选择状态创建表格属性
initTable();
//getServerData();
}
function getServerData(){
let timeformatted = selectType();
const serverUrl = $('#ip-port').val();
const url = serverUrl+'/api/SystemMonitor/GetReport?type='+tableType+'&time='+timeformatted;
const authorization = 'Bearer '+'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJVc2VySWQiOjE0MjMwNzA3MDkxMDU1MSwiVGVuYW50SWQiOjE0MjMwNzA3MDkxODc4MCwiQWNjb3VudCI6InN5c3RlbSIsIk5hbWUiOiLotoXnuqfnrqHnkIblkZgiLCJTdXBlckFkbWluIjoxLCJLZWxpU3VwZXJBZG1pbiI6MSwiT3JnSWQiOjE0MjMwNzA3MDkxMDUzOSwiT3JnTmFtZSI6IumbhuWbouWFrOWPuDk5OSIsImlhdCI6MTcwNDQ0MDM4NSwibmJmIjoxNzA0NDQwMzg1LCJleHAiOjE3MDcwMzIzODUsImlzcyI6IktlbGkiLCJhdWQiOiJLZWxpIn0.MHS1KUqzq8bKytYVH3W8pdjcr8EOVlFpxc-a5CaU64g'
$.ajax({
url: url,
method: 'get',
dataType: 'json',
//beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', authorization);
//},
//headers: {'Accept': 'application/json', 'Authorization': authorization},
contentType: "application/json,charset=utf-8",
success: (res)=> {
if(res.code==200){
tableData = res.data||[];
initTable();
}else{
alert(JSON.stringify(res.data))
}
return;
},
error: function(res) {
alert(JSON.stringify(res))
}
});
}
function typeView(type){
let viewMode = ''
switch(type){
case 'day':
viewMode = 'days'
break;
case 'month':
viewMode = 'months'
break;
case 'year':
viewMode = 'years'
break;
default:
break;
}
return viewMode
}
function viewModeTable(viewMode){
let tabType = ''
switch(viewMode){
case 'days':
tabType = 'day'
break;
case 'months':
tabType = 'month'
break;
case 'years':
tabType = 'year'
break;
default:
break;
}
return tabType
}
function getData(type){
tableType = type;
selectDateBtn(typeView(type));
searchReport();
}
function exportData(){
if (this.tableData && tableData.length) {
const timeformatted = selectType();
const serverUrl = $('#ip-port').val()
const url = serverUrl+'/api/SystemMonitor/ExportReport?type='+tableType+'&time='+timeformatted;
//const authorization = 'Bearer '+'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJVc2VySWQiOjE0MjMwNzA3MDkxMDU1MSwiVGVuYW50SWQiOjE0MjMwNzA3MDkxODc4MCwiQWNjb3VudCI6InN5c3RlbSIsIk5hbWUiOiLotoXnuqfnrqHnkIblkZgiLCJTdXBlckFkbWluIjoxLCJLZWxpU3VwZXJBZG1pbiI6MSwiT3JnSWQiOjE0MjMwNzA3MDkxMDUzOSwiT3JnTmFtZSI6IumbhuWbouWFrOWPuDk5OSIsImlhdCI6MTcwNDQ0MDM4NSwibmJmIjoxNzA0NDQwMzg1LCJleHAiOjE3MDcwMzIzODUsImlzcyI6IktlbGkiLCJhdWQiOiJLZWxpIn0.MHS1KUqzq8bKytYVH3W8pdjcr8EOVlFpxc-a5CaU64g'
$.ajax({
url: url,
method: 'get',
dataType: 'json',
//beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', authorization);
//},
//headers: {'Accept': 'application/json', 'Authorization': authorization},
contentType: "application/json,charset=utf-8",
success: (res)=> {
//console.log(res);
if(res.code==200){
if (res.data) {
let fileUrl = serverUrl + res.data;
let rename = reportName();
fileDownload(fileUrl, rename+'下载.html');
}
}
return;
},
error: function(res) {
console.log(res);
}
});
}
}
function fileDownload(content, filename) {
// 创建隐藏的可下载链接
var eleLink = document.createElement('a');
eleLink.download = filename;
eleLink.style.display = 'none';
// 字符内容转变成blob地址
//var blob = new Blob([content]);
//eleLink.href = URL.createObjectURL(blob);
eleLink.href = content;
// 触发点击
document.body.appendChild(eleLink);
eleLink.click();
// 然后移除
document.body.removeChild(eleLink);
};
var tableData = []
function initTable(){
$('#' + tableName).empty();
$('#' + tableName).bootstrapTable('destroy').bootstrapTable({
columns: columns, //动态拼接生成表格
data: tableData,
//data: data,
sortStable: true,
toolbar: "#toolbar", //一个jQuery 选择器,指明自定义的toolbar 例如:#toolbar, .toolbar.
uniqueId: "uid", //每一行的唯一标识,一般为主键列
height: document.body.clientHeight-300, //动态获取高度值,可以使表格自适应页面
cache: true, // 表格缓存
striped: true, // 隔行加亮 行间隔色
queryParamsType: "limit", //设置为"undefined",可以获取pageNumber,pageSize,searchText,sortName,sortOrder //设置为"limit",符合 RESTFul 格式的参数,可以获取limit, offset, search, sort, order
queryParams: queryParams,
sidePagination: "client", //分页方式:client客户端分页,server服务端分页(*)
sortable: true, //是否启用排序;意味着整个表格都会排序
sortName: 'uid', // 设置默认排序为 name
sortOrder: "asc", //排序方式
pagination: true, //是否显示分页(*)
search: true, //是否显示表格搜索,此搜索是客户端搜索,不会进服务端,所以,个人感觉意义不大
strictSearch: true,
showColumns: true, //是否显示所有的列
showRefresh: true, //是否显示刷新按钮
showToggle:true, //是否显示详细视图和列表视图
detailView: true, // 是否显示父子表
clickToSelect: true, //是否启用点击选中行
paginationLoop: true,
minimumCountColumns: 2, //最少允许的列数 clickToSelect: true, //是否启用点击选中行
pageNumber: 1, //初始化加载第一页,默认第一页
pageSize: 10, //每页的记录行数(*)
pageList: [10, 25, 50, 100], //可供选择的每页的行数(*)
paginationPreText: "上一页",
paginationNextText: "下一页",
paginationFirstText: "首页",
paginationLastText: "末页",
undefinedText: "...",
responseHandler: responseHandler,
showExport: true,
buttonsAlign: "right",//按钮位置
exportDataType: 'basic',//导出的数据类型,支持basic、all 、selected
exportOptions:{
ignoreColumn:[0], //导出数据忽略第一列
fileName:'报表', //导出数据的文件名
worksheetName:'sheet1',//表格工作区名称
tableName:'报表',
excelstyles:['background-color','color','font-size','font-weight']
},
rowStyle: rowStyle,
cellStyle:function(value,row,index){// value:单元格值,row:行对象,index:第几行
// 逻辑
return {css: {"background-color": 'rgb(11,22,33)'}};
},
onLoadSuccess: function (data) { //加载成功时执行
//console.log(data);
},
onLoadError: function (res) { //加载失败时执行
console.log(res);
},
onSort: function (a, b) { //点击排序时触发
$(".fixed-table-header").removeClass('hidden');
return a - b;
},
onExpandRow: function(index,row,$detail){
initSubTable(index,row,$detail)
}
});
$(".fixed-table-header").addClass('hidden');
//初始化子表 无限循环
const initSubTable = function(index,row,$detail){
let parentId = row.id
let cur_table = $detail.html('<table></table>').find('table')
$(cur_table).bootstrapTable({
data: [{
"mesh": 0,
"translation": [
-69.28118,
97.06617,
-31.3581028
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx001",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 1,
"translation": [
-384.306427,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx007"
},
{
"mesh": 2,
"translation": [
-384.306427,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx008"
},
{
"mesh": 3,
"translation": [
-265.6682,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx009"
},
{
"mesh": 4,
"translation": [
-265.6682,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx010"
},
{
"mesh": 5,
"translation": [
329.4485,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx011"
},
{
"mesh": 6,
"translation": [
329.4485,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx012"
},
{
"mesh": 7,
"translation": [
424.924927,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx013"
},
{
"mesh": 8,
"translation": [
424.924927,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx014"
},
{
"mesh": 9,
"translation": [
185.995361,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx015"
},
{
"mesh": 10,
"translation": [
185.995361,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx016"
},
{
"mesh": 11,
"translation": [
-180.629181,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx017"
},
{
"mesh": 12,
"translation": [
-180.629181,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx018"
},
{
"mesh": 13,
"translation": [
-95.59015,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx020"
},
{
"mesh": 14,
"translation": [
-95.59015,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx021"
},
{
"mesh": 15,
"translation": [
-10.5511055,
50.7831421,
178.388123
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx023"
},
{
"mesh": 16,
"translation": [
-10.5511055,
50.7831421,
153.324448
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx024"
},
{
"mesh": 17,
"translation": [
-5.850804,
97.06617,
195.659225
],
"name": "dmxx025",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 18,
"translation": [
-11.3755226,
52.31717,
23.8695
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx026",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 19,
"translation": [
20.13911,
104.698647,
-58.73637
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx027",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 20,
"translation": [
20.1939049,
104.698647,
-58.83061
],
"scale": [
703.122742,
1000,
27.22401
],
"name": "dmxx028",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 21,
"translation": [
20.13911,
109.331139,
-58.73637
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx029",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 22,
"translation": [
-357.0462,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx030"
},
{
"mesh": 23,
"translation": [
-353.402039,
20.8683167,
-105.386818
],
"rotation": [
0,
-8.742278e-8,
0,
1
],
"name": "dmxx034",
"scale": [
1,
1,
1
]
},
{
"mesh": 24,
"translation": [
14.6565981,
100.400383,
-78.23704
],
"scale": [
2000,
2000,
2000
],
"name": "dmxx040",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 25,
"translation": [
311.286682,
78.07455,
-23.8040924
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx041"
},
{
"mesh": 25,
"translation": [
311.286682,
78.07455,
-56.312088
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx044"
},
{
"mesh": 25,
"translation": [
311.286682,
78.07455,
-88.63709
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx047"
},
{
"mesh": 25,
"translation": [
311.286682,
78.07455,
-121.432076
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx050"
},
{
"mesh": 25,
"translation": [
312.5485,
78.07455,
-153.323837
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx053"
},
{
"mesh": 26,
"translation": [
307.3467,
102.492004,
-23.8041229
],
"scale": [
41.2218742,
1000,
849.2772
],
"name": "dmxx042",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 26,
"translation": [
307.3467,
102.492004,
-56.312088
],
"scale": [
41.2218742,
1000,
849.2772
],
"name": "dmxx045",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 26,
"translation": [
307.3467,
102.492004,
-88.63709
],
"scale": [
41.2218742,
1000,
849.2772
],
"name": "dmxx048",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 26,
"translation": [
307.3467,
102.492004,
-121.432091
],
"scale": [
41.2218742,
1000,
849.2772
],
"name": "dmxx051",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 26,
"translation": [
307.3467,
103.512192,
-153.323868
],
"scale": [
41.2218742,
1000,
849.2772
],
"name": "dmxx054",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 27,
"translation": [
311.178558,
108.826675,
-23.8226776
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx043",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 27,
"translation": [
311.178558,
108.826675,
-56.3306427
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx046",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 27,
"translation": [
311.178558,
108.826675,
-88.65565
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx049",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 27,
"translation": [
311.178558,
108.826675,
-121.450645
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx052",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 27,
"translation": [
312.440369,
109.846863,
-153.342422
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx055",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 28,
"translation": [
-263.4113,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx056"
},
{
"mesh": 29,
"translation": [
-173.5626,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx057"
},
{
"mesh": 30,
"translation": [
-67.6411057,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx058"
},
{
"mesh": 31,
"translation": [
38.2803955,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx059"
},
{
"mesh": 32,
"translation": [
144.20192,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx060"
},
{
"mesh": 33,
"translation": [
250.1234,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx061"
},
{
"mesh": 34,
"translation": [
362.969574,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx062"
},
{
"mesh": 35,
"translation": [
122.978882,
107.494537,
33.8315964
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx065"
},
{
"mesh": 35,
"translation": [
122.978882,
107.494537,
80.91254
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx067"
},
{
"mesh": 35,
"translation": [
122.978882,
107.494537,
127.993469
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx069"
},
{
"mesh": 35,
"translation": [
262.9095,
107.494537,
33.8315964
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx071"
},
{
"mesh": 35,
"translation": [
262.9095,
107.494537,
80.91254
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx073"
},
{
"mesh": 35,
"translation": [
262.9095,
107.494537,
127.993469
],
"rotation": [
0.500000238,
0.499999732,
-0.499999762,
0.500000238
],
"scale": [
102.549454,
102.549454,
102.549454
],
"name": "dmxx075"
},
{
"mesh": 36,
"translation": [
123.283409,
99.7992554,
33.8151779
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx066"
},
{
"mesh": 36,
"translation": [
123.283409,
99.7992554,
80.89612
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx068"
},
{
"mesh": 36,
"translation": [
123.283409,
99.7992554,
127.977051
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx070"
},
{
"mesh": 36,
"translation": [
263.21402,
99.7992554,
33.8151779
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx072"
},
{
"mesh": 36,
"translation": [
263.21402,
99.7992554,
80.89612
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx074"
},
{
"mesh": 36,
"translation": [
263.21402,
99.7992554,
127.977051
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx076"
},
{
"mesh": 37,
"translation": [
-354.3712,
21.3148766,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx078"
},
{
"mesh": 37,
"translation": [
-354.039642,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx080",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
-261.123566,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx084",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
-175.647079,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx087",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
-91.1806259,
103.6815,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx090",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
-6.7141614,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx093",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
335.155029,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx096",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
392.734375,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx099",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
424.99704,
24.196846,
234.26799
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx102",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 37,
"translation": [
-262.579529,
24.196846,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx105"
},
{
"mesh": 37,
"translation": [
-175.370026,
24.196846,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx108"
},
{
"mesh": 37,
"translation": [
-99.35006,
24.196846,
-351.338226
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx111"
},
{
"mesh": 37,
"translation": [
-17.0734463,
24.196846,
-351.338226
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
48.58575,
1178.64,
1000.99207
],
"name": "dmxx114"
},
{
"mesh": 38,
"translation": [
-354.211151,
29.43003,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx079"
},
{
"mesh": 38,
"translation": [
-354.199677,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx081",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
-261.2836,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx085",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
-175.807114,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx088",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
-91.34066,
111.796661,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx091",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
-6.87419558,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx094",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
334.995,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx097",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
392.574341,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx100",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
424.837,
32.312,
234.26799
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx103",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 38,
"translation": [
-262.419525,
32.312,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx106"
},
{
"mesh": 38,
"translation": [
-175.209976,
32.312,
-185.977631
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx109"
},
{
"mesh": 38,
"translation": [
-99.19002,
32.312,
-351.338226
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx112"
},
{
"mesh": 38,
"translation": [
-16.91341,
32.312,
-351.338226
],
"rotation": [
0,
-1,
0,
-7.54979e-8
],
"scale": [
1178.64,
1178.64,
1178.64
],
"name": "dmxx115"
},
{
"mesh": 39,
"translation": [
-354.291138,
67.79712,
235.0402
],
"rotation": [
0,
0,
8.742278e-8,
1
],
"name": "dmxx082",
"scale": [
1,
1,
1
]
},
{
"mesh": 40,
"translation": [
-13.5029039,
55.26396,
23.79452
],
"rotation": [
0.7071068,
0,
0,
0.7071067
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx116"
},
{
"mesh": 41,
"translation": [
-41.3215942,
0,
25.8910332
],
"name": "dmxx117",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 42,
"translation": [
575.1094,
4.56818771,
-394.632172
],
"rotation": [
-5.76011772e-8,
0.7071066,
5.76011381e-8,
0.707107067
],
"scale": [
39.9999962,
39.9999962,
39.9999962
],
"name": "dmxx118"
},
{
"mesh": 42,
"translation": [
-748.5892,
4.56818771,
-394.632172
],
"rotation": [
-5.76011772e-8,
0.7071066,
5.76011381e-8,
0.707107067
],
"scale": [
39.9999962,
39.9999962,
39.9999962
],
"name": "dmxx159"
},
{
"mesh": 43,
"translation": [
111.822212,
100.152115,
-180.306564
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx119",
"scale": [
1,
1,
1
]
},
{
"mesh": 44,
"translation": [
-58.72216,
101.010712,
-154.881439
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx122",
"scale": [
1,
1,
1
]
},
{
"mesh": 45,
"translation": [
282.5177,
101.010712,
-154.181885
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx123",
"scale": [
1,
1,
1
]
},
{
"mesh": 46,
"translation": [
111.876022,
101.010689,
-137.679047
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "huojia1",
"scale": [
1,
1,
1
]
},
{
"mesh": 47,
"translation": [
251.998413,
40.02563,
-153.323837
],
"rotation": [
0,
0,
0.7071069,
0.70710665
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx127"
},
{
"mesh": 47,
"translation": [
251.998413,
40.02563,
-121.256851
],
"rotation": [
0,
0,
0.7071069,
0.70710665
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx129"
},
{
"mesh": 47,
"translation": [
251.998413,
40.02563,
-88.64618
],
"rotation": [
0,
0,
0.7071069,
0.70710665
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx131"
},
{
"mesh": 47,
"translation": [
251.998413,
40.02563,
-56.3211746
],
"rotation": [
0,
0,
0.7071069,
0.70710665
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx133"
},
{
"mesh": 47,
"translation": [
251.998413,
40.02563,
-23.8131752
],
"rotation": [
0,
0,
0.7071069,
0.70710665
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx135"
},
{
"mesh": 48,
"translation": [
251.998413,
40.02563,
-153.323837
],
"rotation": [
0,
0,
0.70710665,
0.7071069
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx128"
},
{
"mesh": 48,
"translation": [
251.998413,
40.02563,
-121.256851
],
"rotation": [
0,
0,
0.70710665,
0.7071069
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx130"
},
{
"mesh": 48,
"translation": [
251.998413,
40.02563,
-88.64618
],
"rotation": [
0,
0,
0.70710665,
0.7071069
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx132"
},
{
"mesh": 48,
"translation": [
251.998413,
40.02563,
-56.3211746
],
"rotation": [
0,
0,
0.70710665,
0.7071069
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx134"
},
{
"mesh": 48,
"translation": [
251.998413,
40.02563,
-23.8131752
],
"rotation": [
0,
0,
0.70710665,
0.7071069
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx136"
},
{
"mesh": 49,
"translation": [
111.897774,
101.010689,
-104.808395
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "huojia2",
"scale": [
1,
1,
1
]
},
{
"mesh": 50,
"translation": [
111.876022,
101.010689,
-72.813
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "huojia3",
"scale": [
1,
1,
1
]
},
{
"mesh": 51,
"translation": [
111.761848,
92.45464,
-24.595459
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx145",
"scale": [
1,
1,
1
]
},
{
"mesh": 52,
"translation": [
-58.7439079,
101.010712,
-7.71492624
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx147",
"scale": [
1,
1,
1
]
},
{
"mesh": 53,
"translation": [
111.800461,
100.152115,
2.51851177
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx148",
"scale": [
1,
1,
1
]
},
{
"mesh": 54,
"translation": [
111.897758,
101.010689,
-39.97574
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "huojia4",
"scale": [
1,
1,
1
]
},
{
"mesh": 55,
"translation": [
282.495941,
101.010712,
-8.314145
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx151",
"scale": [
1,
1,
1
]
},
{
"mesh": 56,
"translation": [
111.381035,
82.89289,
-23.9728642
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "dmxx152",
"scale": [
1,
1,
1
]
},
{
"children": [
113,
114
],
"mesh": 57,
"translation": [
-262.176,
24.9275455,
314.977051
],
"rotation": [
0,
-0.7071068,
0,
0.7071067
],
"name": "dmxx153",
"scale": [
1,
1,
1
]
},
{
"mesh": 58,
"translation": [
27.1406269,
-17.60384,
-0.0261875018
],
"rotation": [
0,
0,
0.8660247,
0.500001252
],
"name": "dmxx154",
"scale": [
1,
1,
1
]
},
{
"mesh": 59,
"translation": [
-12.2425632,
-15.750452,
-0.0001875
],
"rotation": [
0,
0,
0.8660247,
0.500001252
],
"name": "dmxx155",
"scale": [
1,
1,
1
]
},
{
"children": [
116,
117
],
"mesh": 57,
"translation": [
-617.656738,
25.039875,
-446.740631
],
"rotation": [
0,
-1.78813934e-7,
0,
1
],
"name": "dmxx316",
"scale": [
1,
1,
1
]
},
{
"mesh": 64,
"translation": [
26.968,
-17.60384,
-0.01053125
],
"rotation": [
1.25059278e-13,
1.78813934e-7,
6.993822e-7,
1
],
"name": "dmxx389",
"scale": [
1,
1,
1
]
},
{
"mesh": 65,
"translation": [
-12.4151878,
-15.750452,
0.0154687511
],
"rotation": [
1.25059278e-13,
1.78813934e-7,
6.993822e-7,
1
],
"name": "dmxx390",
"scale": [
1,
1,
1
]
},
{
"mesh": 60,
"translation": [
337.334229,
103.957069,
-9.232151
],
"scale": [
0.742666364,
0.631332636,
0.742666364
],
"name": "dmxx156",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 61,
"translation": [
741.881531,
32.5010338,
-384.892059
],
"name": "dmxx185",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 62,
"translation": [
-581.1957,
14.7027626,
-503.6794
],
"name": "dmxx278",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 63,
"translation": [
-546.6353,
27.7788963,
-482.8991
],
"rotation": [
0,
-0.7071068,
0,
0.7071067
],
"scale": [
1.00000012,
1.00000012,
1.00000012
],
"name": "dmxx279"
},
{
"mesh": 66,
"translation": [
-503.148071,
53.3803864,
-585.816467
],
"name": "dmxx317",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"children": [
124,
132
],
"translation": [
-334.901855,
26.5603485,
10.5192423
],
"rotation": [
0,
-1,
0,
-1.94707184e-7
],
"scale": [
18.1919937,
18.1919937,
18.1919937
],
"name": "dmxx318"
},
{
"children": [
125
],
"translation": [
-0.0171035156,
-1.02223742,
-0.07051178
],
"rotation": [
0,
0.3132618,
0,
0.9496668
],
"scale": [
33.9823341,
33.9823341,
33.9823341
],
"name": "dmxx319"
},
{
"children": [
126
],
"translation": [
-0.0164908767,
-0.0000109090806,
0.00318090827
],
"rotation": [
-0.5000001,
0.5,
-0.499999881,
-0.5
],
"scale": [
1.00000012,
1,
0.9999999
],
"name": "dmxx320"
},
{
"children": [
127
],
"translation": [
0,
0,
-0.0155947218
],
"rotation": [
-0.630633831,
1.32566393e-8,
3.63701531e-8,
0.7760806
],
"scale": [
0.99999994,
1,
1.00000012
],
"name": "dmxx321"
},
{
"children": [
128
],
"translation": [
-0.00710638473,
-3.81469745e-9,
-0.00970999151
],
"rotation": [
-1.02388341e-7,
-0.6306851,
0.7760389,
1.80895938e-7
],
"scale": [
0.99999994,
0.9999999,
0.9999999
],
"name": "dmxx322"
},
{
"children": [
129
],
"translation": [
-3.051758e-8,
0,
-0.0285357609
],
"rotation": [
2.21543868e-8,
-0.151268512,
0.9884927,
-1.9608531e-7
],
"scale": [
1.00000012,
1.00000012,
1.00000012
],
"name": "dmxx323"
},
{
"children": [
130
],
"translation": [
0.005835236,
0,
-0.006521393
],
"rotation": [
-0.595496356,
3.296687e-8,
9.654272e-9,
0.803358
],
"scale": [
1,
0.99999994,
0.99999994
],
"name": "dmxx324"
},
{
"children": [
131
],
"translation": [
0,
3.051758e-8,
-0.0252421275
],
"rotation": [
0.0264743622,
-2.25363728e-8,
3.8032983e-8,
0.9996495
],
"scale": [
0.99999994,
0.9999999,
1
],
"name": "dmxx325"
},
{
"children": [],
"translation": [
0,
0,
-0.006470673
],
"rotation": [
-0.0222050343,
1.05348985e-9,
-4.08145482e-8,
0.9997535
],
"scale": [
1.00000012,
1,
0.999999762
],
"name": "dmxx326"
},
{
"skin": 0,
"mesh": 67,
"translation": [
-0.0171035156,
-1.02223742,
-0.07051178
],
"rotation": [
0,
0.3132618,
0,
0.9496668
],
"scale": [
33.9823341,
33.9823341,
33.9823341
],
"name": "dmxx328"
},
{
"mesh": 68,
"translation": [
-2.87668753,
2.284103,
12.0431881
],
"name": "dmxx329",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 69,
"translation": [
-352.263153,
103.957069,
-241.219147
],
"scale": [
0.742666364,
0.631332636,
0.742666364
],
"name": "dmxx386",
"rotation": [
0,
0,
0,
1
]
},
{
"mesh": 70,
"translation": [
42.0269547,
144.5176,
23.8153057
],
"name": "dmxx387",
"rotation": [
0,
0,
0,
1
],
"scale": [
1,
1,
1
]
},
{
"mesh": 71,
"translation": [
-244.126038,
7.963813,
11.8019915
],
"rotation": [
0,
-0.949666858,
0,
0.313261658
],
"scale": [
618.2065,
618.2064,
618.2065
],
"name": "dmxx362"
},
{
"mesh": 71,
"translation": [
-158.024063,
7.963813,
11.8019915
],
"rotation": [
0,
-0.949666858,
0,
0.313261658
],
"scale": [
618.2065,
618.2064,
618.2065
],
"name": "dmxx388"
},
{
"mesh": 72,
"translation": [
-326.640259,
6.26502466,
6.236844
],
"rotation": [
0,
0.7071066,
0,
0.707106948
],
"scale": [
39.9999962,
39.9999962,
39.9999962
],
"name": "adad1v001"
},
{
"mesh": 73,
"translation": [
-236.36116,
6.26502466,
6.236844
],
"rotation": [
0,
0.7071066,
0,
0.707106948
],
"scale": [
39.9999962,
39.9999962,
39.9999962
],
"name": "adad1v002"
},
{
"mesh": 73,
"translation": [
-150.3011,
6.26502466,
6.236844
],
"rotation": [
0,
0.7071066,
0,
0.707106948
],
"scale": [
39.9999962,
39.9999962,
39.9999962
],
"name": "adad1v003"
},
{
"mesh": 74,
"translation": [
346.052948,
50.7831421,
13.3077393
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx391"
},
{
"mesh": 75,
"translation": [
424.924927,
50.7831421,
13.3077393
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx393"
},
{
"mesh": 76,
"translation": [
346.052948,
50.7831421,
-40.48396
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx395"
},
{
"mesh": 77,
"translation": [
424.924927,
50.7831421,
-40.48396
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx397"
},
{
"mesh": 78,
"translation": [
346.052948,
50.7831421,
-94.2396
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx399"
},
{
"mesh": 79,
"translation": [
424.924927,
50.7831421,
-94.2396
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx401"
},
{
"mesh": 80,
"translation": [
346.052948,
50.7831421,
-149.506744
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx403"
},
{
"mesh": 81,
"translation": [
424.924927,
50.7831421,
-149.506744
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx405"
},
{
"mesh": 82,
"translation": [
423.3623,
50.7831421,
-241.902939
],
"rotation": [
0.5,
-0.5,
0.50000006,
0.49999994
],
"scale": [
1000,
1000,
1000
],
"name": "dmxx407"
},
{
"mesh": 83,
"translation": [
111.381035,
82.89289,
-153.671509
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "对象005",
"scale": [
1,
1,
1
]
},
{
"mesh": 84,
"translation": [
111.761848,
93.180336,
-154.2941
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "对象006",
"scale": [
1,
1,
1
]
},
{
"mesh": 85,
"translation": [
282.5177,
101.010712,
-24.48324
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "对象009",
"scale": [
1,
1,
1
]
},
{
"mesh": 86,
"translation": [
-58.72216,
101.010712,
-25.1828022
],
"rotation": [
0,
8.742278e-8,
0,
1
],
"name": "对象010",
"scale": [
1,
1,
1
]
}
],
columns:[
{
field: 'uid',
title: '编号',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
//formatter: uidHandle,//自定义方法设置uid跳转链接
formatter: function (value, row, index) {
return index+1;
},
width: 30
},
{
field: 'name',
title: 'name',
width: 100
},
{
field: 'translation',
title: 'translation',
width: 100
},
{
field: 'scale',
title: 'scale',
width: 100
},
{
field: 'rotation',
title: 'rotation',
width: 100
}],
uniqueId: "uid", //每一行的唯一标识,一般为主键列
//无限循环取子表,直到子表里面没有记录
onExpandRow: function(index,row,$detail){
initSubTable(index,row,$detail)
}
})
}
}
/***************************************
* 生成从minNum到maxNum的随机数。
* 如果指定decimalNum个数,则生成指定小数位数的随机数
* 如果不指定任何参数,则生成0-1之间的随机数。
*
* @minNum:[数据类型是Integer]生成的随机数的最小值(minNum和maxNum可以调换位置)
* @maxNum:[数据类型是Integer]生成的随机数的最大值
* @decimalNum:[数据类型是Integer]如果生成的是带有小数的随机数,则指定随机数的小数点后的位数
*
****************************************/
function randomNum(maxNum, minNum, decimalNum) {
var max = 0, min = 0;
minNum <= maxNum ? (min = minNum, max = maxNum) : (min = maxNum, max = minNum);
switch (arguments.length) {
case 1:
return Math.floor(Math.random() * (max + 1));
break;
case 2:
return Math.floor(Math.random() * (max - min + 1) + min);
break;
case 3:
return (Math.random() * (max - min) + min).toFixed(decimalNum);
break;
default:
return Math.random();
break;
}
}
function refresh(){
$('#' + tableName).bootstrapTable('refresh');
}
function DoOnMsoNumberFormat(cell, row, col) {
var result = "";
if (row > 0 && col == 0)
result = "\\@";
return result;
}
var columns=[]
function buildTableColumns() {
columns = []
var itemCode=[], //所选择因子的code
itemName=[]; // 所选择因子的text
$.each($(".itemArea li input[type='checkbox']"),function () {
if($(this).is(":checked")){
itemCode.push($(this).data("code"));
itemName.push($(this).parents("label").text());
}
});
var initColumns = []; //公共固定列
var itemColumns = []; //动态因子列
initColumns=[
{
rowspan: 2, //合并两行
align: 'center',
align: "center",
halign: "center",
valign: "middle",
checkbox: true
},
{
field: 'uid',
title: '编号',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
//formatter: uidHandle,//自定义方法设置uid跳转链接
formatter: function (value, row, index) {
return index+1;
},
rowspan: 2, //合并两行
width: 30
}, {
field: 'time',
title: '时间',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
width: 200,
rowspan: 2, //合并两行
formatter: function (value, row, index) {//赋予的参数
return ['<div style="width: 200px;display: flex;justify-content: space-around;">'+value+'</div>'].join('');
},
sortable:false //本列不可以排序
}];
if(itemCode.length>0){
//根据选择因子绘制动态表头
for (var i = 0; i < itemCode.length; i++) {
switch(itemCode[i]){
case 'B01':
initColumns.push (
{
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 5
}
);
itemColumns.push({
field: 'value0',
title: '流量(m³/h)',
align: 'center'
}, {
field: 'value1',
title: '累计量(m³)',
align: 'center',
//sortable: true,
//clickToSelect: false,
sortName: "age",
order:"asc"
}, {
field: 'value2',
title: '浊度(Ntu)',
align: 'left',
halign:'center' //设置表头列居中对齐
}, {
field: 'value3',
title: 'pH',
align: 'center'
}, {
field: 'value4',
title: '温度(℃)',
align: 'center'
});
break;
case 'B02':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 10
});
itemColumns.push({
field: 'value5',
title: '库流量(m³/h)',
align: 'center'
}, {
field: 'value6',
title: '库累积量(m³)',
align: 'center'
}, {
field: 'value7',
title: '纸流量(m³/h)',
align: 'center'
}, {
field: 'value8',
title: '纸累积量(m³)',
align: 'center'
}, {
field: 'value9',
title: '横流量(m³/h)',
align: 'center'
}, {
field: 'value10',
title: '横累积量(m³)',
align: 'center'
}, {
field: 'value11',
title: '浊度(Ntu)',
align: 'center'
}, {
field: 'value12',
title: '余氯(mg/L)',
align: 'center'
}, {
field: 'value13',
title: 'pH',
align: 'center'
}, {
field: 'value14',
title: '温度(℃)',
align: 'center'
});
break;
case 'B03':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 2
});
itemColumns.push({
field: 'value15',
title: '1#吸水井(m)',
align: 'center'
}, {
field: 'value16',
title: '2#吸水井(m)',
align: 'center'
});
break;
case 'B04':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 3
});
itemColumns.push({
field: 'value17',
title: '大河(Bar)',
align: 'center'
}, {
field: 'value18',
title: '哈河(Bar)',
align: 'center'
}, {
field: 'value19',
title: '杭河(Bar)',
align: 'center'
});
break;
default:
break;
}
}
initColumns.push ({
field: 'operate',
title: '操作',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
rowspan: 2, //合并两行
width: 200,
formatter: operateFormatter //自定义方法,添加操作按钮
});
}
columns.push(initColumns, itemColumns); //合并表格内容
}
$(function(){
var hash = window.location.hash;
hash && $('ul.nav a[href="'+hash+'"]').tab('show');
$("#navList a").click(function(e){
e.preventDefault()
$(this).tab('show');
});
var nua = navigator.userAgent
var isAndroid = (nua.indexOf('Mozilla/5.0') > -1 && nua.indexOf('Android ') > -1 && nua.indexOf('AppleWebKit') > -1 && nua.indexOf('Chrome') === -1)
if (isAndroid) {
$('select.form-control').removeClass('form-control').css('width', '100%')
}
init()
});
// Example starter JavaScript for disabling form submissions if there are invalid fields
$(() => {
'use strict'
// Fetch all the forms we want to apply custom Bootstrap validation styles to
const forms = document.querySelectorAll('.needs-validation')
// Loop over them and prevent submission
Array.from(forms).forEach(form => {
form.addEventListener('submit', event => {
if (!form.checkValidity()) {
event.preventDefault()
event.stopPropagation()
}
form.classList.add('was-validated')
}, false)
})
});
function dateFormat(viewMode){
let format= ''
switch(viewMode){
case 'days':
format = 'yyyy-mm-dd'
break;
case 'months':
format = 'yyyy-mm'
break;
case 'years':
format = 'yyyy'
break;
default:
break;
}
return format
}
function dateFormatCap(viewMode){
let format= ''
switch(viewMode){
case 'days':
format = 'YYYY-MM-DD'
break;
case 'months':
format = 'YYYY-MM'
break;
case 'years':
format = 'YYYY'
break;
default:
break;
}
return format
}
function addDate(viewMode='days',num){
let temp = today
today = moment(temp).add(parseInt(num), viewMode).format(dateFormatCap(viewMode))
$("#startDate").datepicker("destroy");
initDate(viewMode)
getData(viewModeTable(viewMode))
}
function subtractDate(viewMode='days',num){
let temp = today
today = moment(temp).subtract(parseInt(num), viewMode).format(dateFormatCap(viewMode))
$("#startDate").datepicker("destroy");
initDate(viewMode)
getData(viewModeTable(viewMode))
}
function selectDateBtn(viewMode='days'){
let str = ''
if(viewMode=='days'){
str = '日'
}else if(viewMode=='months'){
str = '月'
}else if(viewMode=='years'){
str = '年'
}
let htmlDiv = '<button class="btn btn-default" onclick='+'subtractDate("'+viewMode+'",1)'+' style="height:40px;">上一'+str+'</button>'+
'<button class="btn btn-default" onclick='+'addDate("'+viewMode+'",1)'+' style="height:40px;">下一'+str+'</button>'
$('#date-operate').html(htmlDiv)
$("#startDate").datepicker("destroy");
initDate(viewMode)
}
let today = new Date();
function initDate(viewMode='days'){
//'days' 'months' 'years'
//初始化日期
var ops = {
todayHighlight:true, //设置当天日期高亮
language: 'zh-CN', //语言
autoclose: false, //选择后自动关闭
clearBtn: true,//清除按钮
format: dateFormat(viewMode),//日期格式
todayBtn: true,//设置今天按钮显示
minViewMode: viewMode,
maxViewMode: viewMode
};
$("#startDate").datepicker(ops);
$("#startDate").datepicker("update", today);
}
function init(){
initDate();
// 获取动态因子数据
getItemData();
// 监听因子点击事件
$(".itemArea li label").click( ()=> {
getItemData();
});
}
function getItemData() {
getData('day');
}
function add(){
var mockDatas=[];
let liuliang = randomNum(0,1000)
let leijiliuliang = randomNum(100000,1000000)
for(let i = 0;i< 1;i++){
let temp = {
uid: i,
time: new Date().toLocaleDateString('en-GB'),
value0: ((liuliang+i)*Math.random()).toFixed(1),
value1: leijiliuliang+i,
value2: randomNum(0,10,2),
value3: randomNum(6.5,8.5,2),
value4: randomNum(20,30,2),
value5: ((liuliang+i)*Math.random()).toFixed(1),
value6: leijiliuliang+i,
value7: ((liuliang+i)*Math.random()).toFixed(1),
value8: leijiliuliang+i,
value9: ((liuliang+i)*Math.random()).toFixed(1),
value10: leijiliuliang+i,
value11: randomNum(0,1,2),
value12: randomNum(1,1,2),
value13: randomNum(6.5,8.5,2),
value14: randomNum(20,30,2),
value15: randomNum(3,5,2),
value16: randomNum(3,5,2),
value17: randomNum(0,1,2),
value18: randomNum(0,1,2),
value19: randomNum(0,1,2),
value20: randomNum(1,100),
value21: randomNum(1,100),
}
mockDatas.push(temp)
}
// 添加记录
//$('#btn_add').click(function() {
// 使用bootstrap-table的编辑器API
$('#' + tableName).bootstrapTable('insertRow', mockDatas[0] );
//});
}
function edit(){
// 编辑记录
//$('#btn_edit').click(function() {
var rows = $('#' + tableName).bootstrapTable('getSelections');
if (rows.length != 1) {
alert('Please select one row to edit!');
return;
}
// 启用编辑模式
$('#' + tableName).bootstrapTable('editRow', rows[0]);
//});
}
function remove(){
// 删除记录
//$('#btn_delete').click(function() {
var rows = $('#' + tableName).bootstrapTable('getSelections');
if (rows.length == 0) {
alert('No rows selected');
return;
}
var tid;// 声明一个tid
// $("#table").bootstrapTable('getSelections');为bootstrapTable自带的,所以说一定要使用bootstrapTable显示表格,#table:为table的id
var rows = $('#' + tableName).bootstrapTable('getSelections');
if (rows.length == 0) {// rows 主要是为了判断是否选中,下面的else内容才是主要
alert("请先选择要删除的记录!");
return;
}
if ((rows.length >= 2)) {
alert("请先选择一条记录!");
return;
} else {
$(rows).each(function () {// 通过获得别选中的来进行遍历
tid = this.tid;// cid为获得到的整条数据中的一列
});
}
//})
}
function removeBatch(){
var tids;
// $("#table").bootstrapTable('getSelections');为bootstrapTable自带的,所以说一定要使用bootstrapTable显示表格,#table:为table的id
var rows = $('#' + tableName).bootstrapTable('getSelections');
if (rows.length == 0) {// rows 主要是为了判断是否选中,下面的else内容才是主要
alert("请至少选择一条要删除的记录!");
return;
} else {
var arrays = new Array();// 声明一个数组
$(rows).each(function () {// 通过获得别选中的来进行遍历
arrays.push(this.tid);// cid为获得到的整条数据中的一列
});
tids = arrays.join(','); // 获得要删除的id
}
/*$.ajax({
type: "post",//方法类型
dataType: "json",//预期服务器返回的数据类型
url: ctx + 'system/madareanumberview/removeBatch?tids=' + tids,//url
success: function (result) {
if (result.success) {
alert("删除成功");
} else {
alert(result.message);
}
}
})*/
}
function submitFun(e){
console.log('提交表单')
$('#myModal').modal('hide')
startWorker()
}
function fullscreen() {
var element = document.getElementById('reportTab');
if (element.requestFullscreen) {
element.requestFullscreen();
} else if (element.mozRequestFullScreen) { /* Firefox */
element.mozRequestFullScreen();
} else if (element.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
element.webkitRequestFullscreen();
} else if (element.msRequestFullscreen) { /* IE/Edge */
element.msRequestFullscreen();
}
}
document.addEventListener('fullscreenchange', function() {
if (!document.fullscreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('mozfullscreenchange', function() {
if (!document.mozFullScreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('webkitfullscreenchange', function() {
if (!document.webkitFullscreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('msfullscreenchange', function() {
if (!document.msFullscreenElement) {
// 退出全屏时的代码
}
}, false);
</script>
使用Web Worker执行复杂或耗时的模拟数据异步生成任务
html
<script type="lslsls" id="worker">
// worker.js代码写入html中
// 在worker.js里面 定义一个函数来执行计算
function compute(num) {
let sum = 0;
for (let i = 0; i < num; i++) {
sum += i;
}
return sum;
}
function randomNum(maxNum, minNum, decimalNum) {
let max = 0, min = 0;
minNum <= maxNum ? (min = minNum, max = maxNum) : (min = maxNum, max = minNum);
switch (arguments.length) {
case 1:
return Math.floor(Math.random() * (max + 1));
break;
case 2:
return Math.floor(Math.random() * (max - min + 1) + min);
break;
case 3:
return (Math.random() * (max - min) + min).toFixed(decimalNum);
break;
default:
return Math.random();
break;
}
}
function addMockData(n){
let mockDatas=[];
const liuliang = randomNum(0,1000)
const leijiliuliang = randomNum(100000,1000000)
for(let i = 0;i< n;i++){
let temp = {
uid: i,
time: new Date().toLocaleDateString('en-GB'),
value0: ((liuliang+i)*Math.random()).toFixed(1),
value1: leijiliuliang+i,
value2: randomNum(0,10,2),
value3: randomNum(6.5,8.5,2),
value4: randomNum(20,30,2),
value5: ((liuliang+i)*Math.random()).toFixed(1),
value6: leijiliuliang+i,
value7: ((liuliang+i)*Math.random()).toFixed(1),
value8: leijiliuliang+i,
value9: ((liuliang+i)*Math.random()).toFixed(1),
value10: leijiliuliang+i,
value11: randomNum(0,1,2),
value12: randomNum(1,1,2),
value13: randomNum(6.5,8.5,2),
value14: randomNum(20,30,2),
value15: randomNum(3,5,2),
value16: randomNum(3,5,2),
value17: randomNum(0,1,2),
value18: randomNum(0,1,2),
value19: randomNum(0,1,2),
value20: randomNum(1,100),
value21: randomNum(1,100),
}
mockDatas.push(temp)
}
return mockDatas
}
// 定义一个函数来处理来自主线程的消息
//add event listener to handle the different message
onmessage = async function(e) {
console.log("Worker: Message received from main script");
const num = e.data.num;
const res1 = compute(num);
postMessage(res1);
const res2 = addMockData(num);
postMessage(res2);
const urls = e.data.urls;
const results = await Promise.all(urls.map(request));
postMessage(results);
if (e.data.action === 'start') {
// 使用setTimeout来异步执行一些计算
setTimeout(() => {
// 将结果发送回主线程
postMessage({ action: 'result', data: res1 });
}, 0);
}
};
// 在worker.js里面:
// 定义一个函数来处理网络请求。
function request(url) {
return fetch(url).then(response => response.json());
}
// 创建消息队列来累积消息
const messageQueue = [];
// 创建一个将消息添加到队列的函数
function addToQueue(message) {
messageQueue.push(message);
// 检查队列是否已达到阈值大小。
if (messageQueue.length >= 10) {
// 如果是,将批处理消息发送到主线程。
postMessage(messageQueue);
// 清除消息队列。
messageQueue.length = 0;
}
}
// 向队列中添加消息
addToQueue({type: 'log', message: 'Hello, world!'});
for(let i = 0; i< 9;i++){
// 向队列中添加另一条消息
addToQueue({type: 'info', message: 'An info '+i+Math.random()+' occurred.'});
}
function doSomeComputation(data){
return data
}
function processItem(data){
return data
}
</script>
升级为布草仓库进销存管理
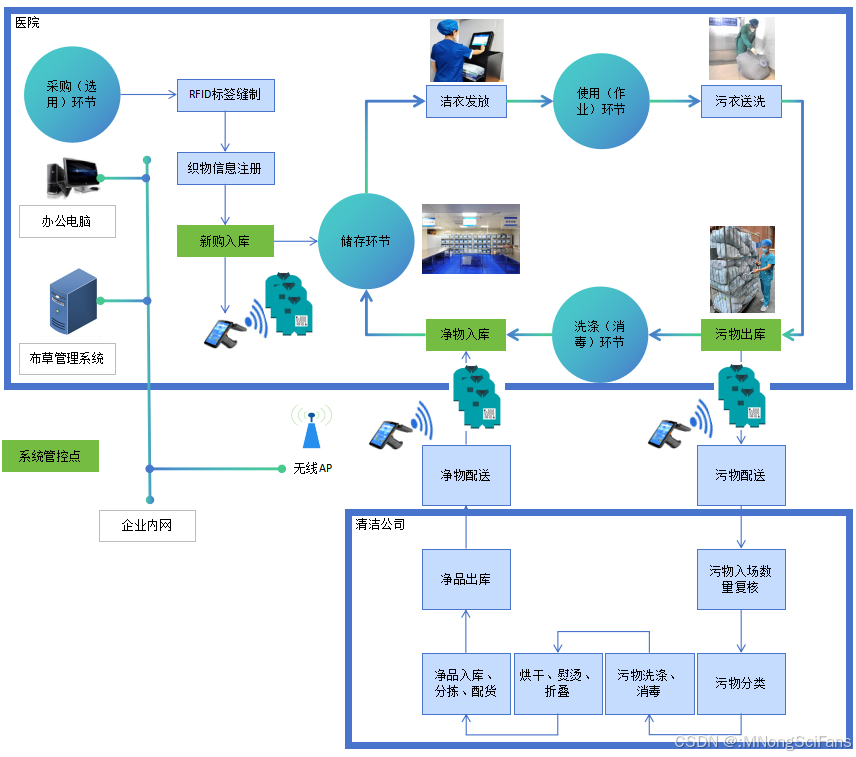
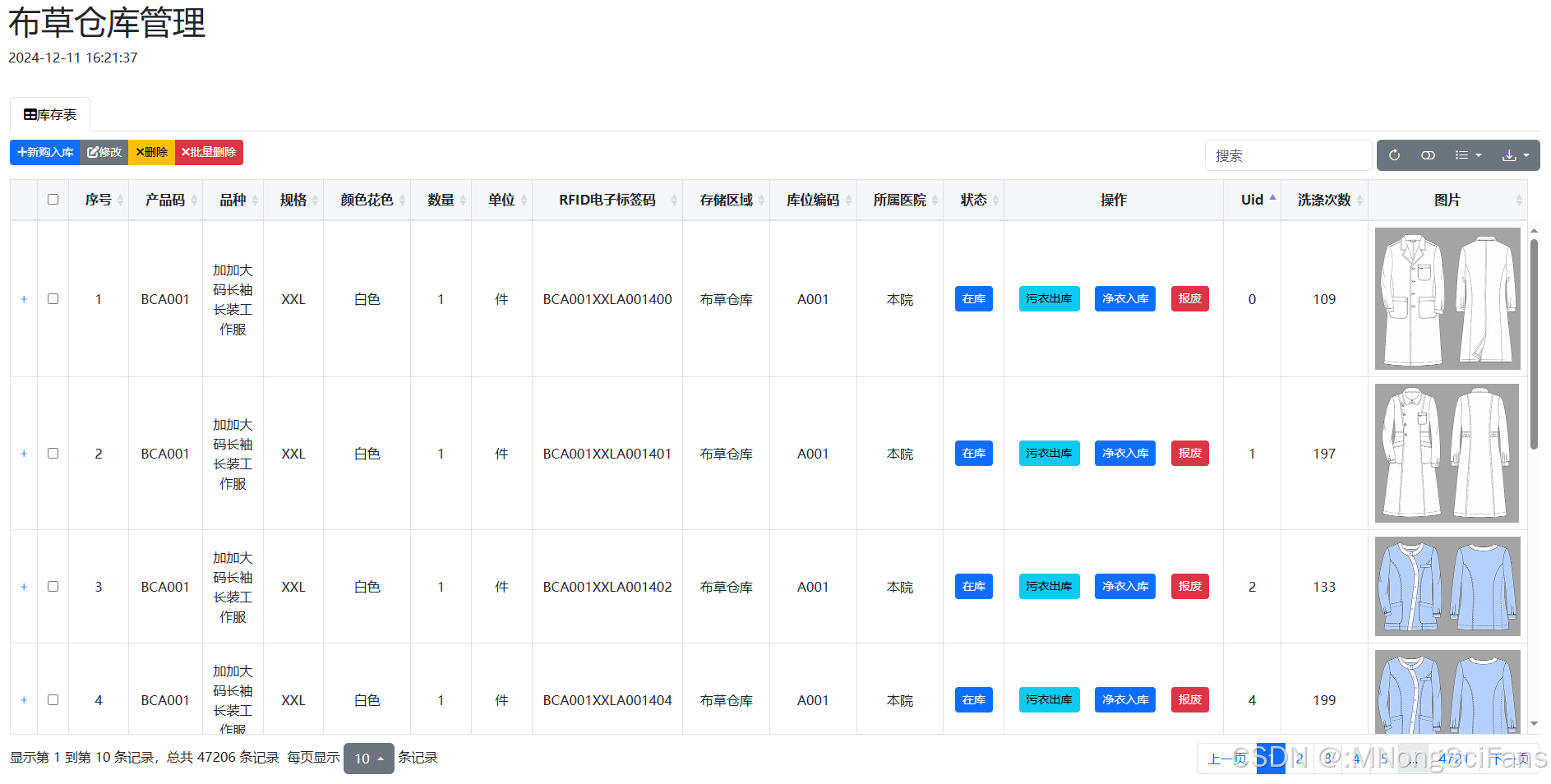
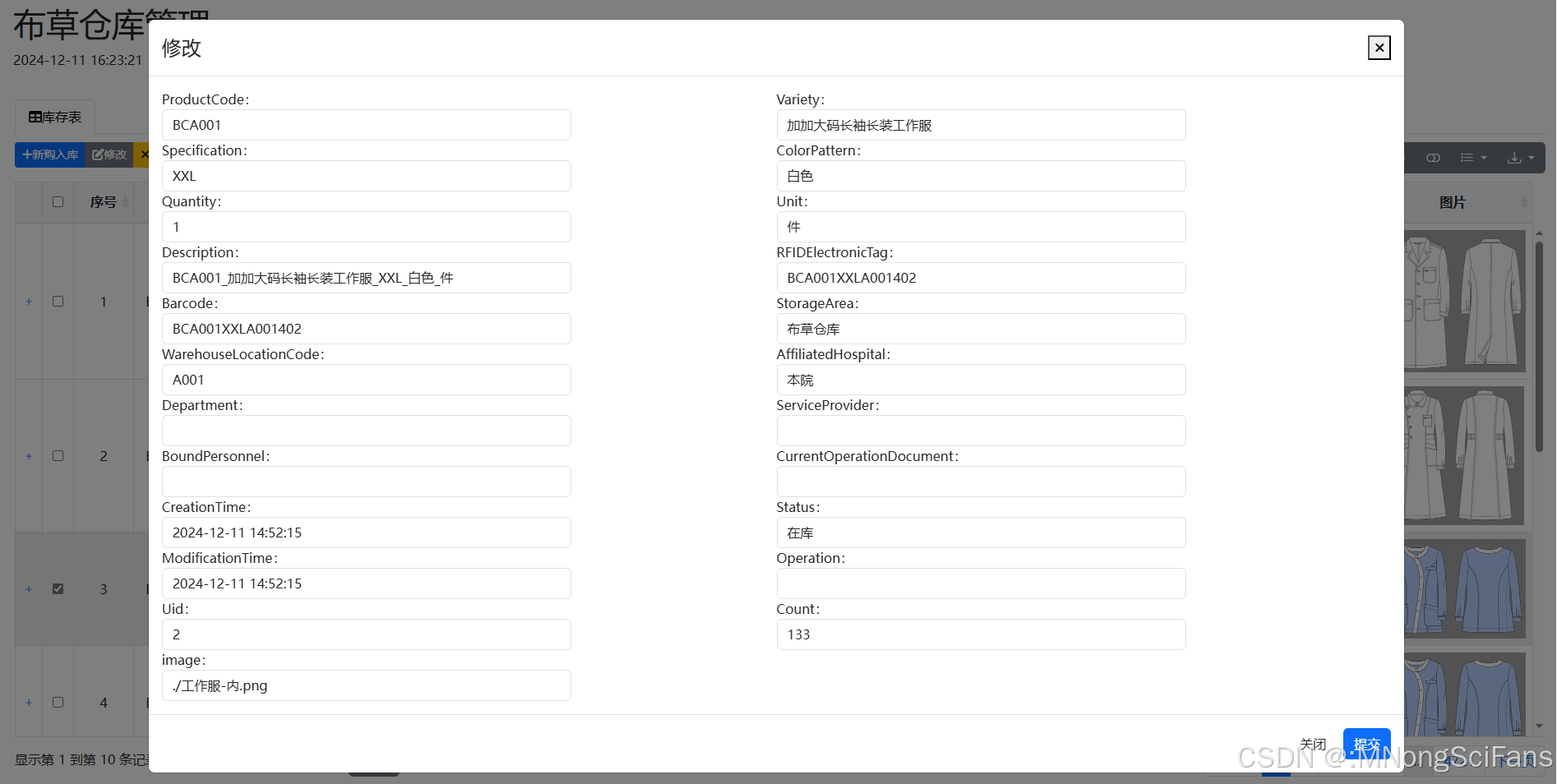
html
<!DOCTYPE html>
<html lang="zh-CN" xmlns:th="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>布草仓库管理</title>
<style>
body {
padding: 0;
margin: 0;
font: normal 14px/1.42857 Tahoma;
}
.selector-for-some-widget {·
box-sizing: content-box;
}
@media print {
.container {
width: auto;
}
}
.itemArea{
list-style: none;
display: flex;
}
.bootstrap-table .fixed-table-container.fixed-height .table thead th {
background: #f5f7fa;
}
</style>
<!-- HTML5 Shiv 和 Respond.js 用于让 IE8 支持 HTML5元素和媒体查询 -->
<!-- 注意: 如果通过 file:// 引入 Respond.js 文件,则该文件无法起效果 -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
<!-- cdn引入样式和js库 -->
<link href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/css/bootstrap.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/bootstrap-table.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-icons/1.11.0/font/bootstrap-icons.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/font-awesome/6.4.2/css/all.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/font-awesome/6.4.2/css/fontawesome.min.css" rel="stylesheet" crossorigin="anonymous">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.7.1/jquery.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/js/bootstrap.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/5.3.1/js/bootstrap.bundle.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/bootstrap-table.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/locale/bootstrap-table-zh-CN.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/js/bootstrap-datepicker.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/locales/bootstrap-datepicker.zh-CN.min.js" crossorigin="anonymous"></script>
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/css/bootstrap-datepicker.min.css" rel="stylesheet" crossorigin="anonymous">
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-datepicker/1.10.0/css/bootstrap-datepicker3.min.css" rel="stylesheet" crossorigin="anonymous">
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.22.1/extensions/export/bootstrap-table-export.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/moment.js/2.29.4/moment.min.js" crossorigin="anonymous"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/moment.js/2.29.4/locale/zh-cn.min.js" crossorigin="anonymous"></script>
</head>
<body>
<div id="app" class="container" style="margin: 10px; height: calc(100vh - 20px);width: calc(100vw - 50px);">
<!-- Content here -->
<div class="wrapper wrapper-content" style="width: calc(100vw - 50px);">
<div class="row">
<div class="col-sm-12" style="padding: 0 10px;">
<h1>布草仓库管理</h1>
<div><p id="time"></p></div>
</div>
</div>
<div class="row">
<div class="col-sm-12" style="padding: 0 10px;display: none">
<div id="dateSearch">
<div class="row g-3 needs-validation" novalidate>
<div class="col-md-3" >
<label for="ip-port" class="form-label"><i class="fa fa-server" aria-hidden="true" style="color:gray"></i>服务器: </label>
<input type="text" class="form-control" id="ip-port" name="ip-port" style="width:250px;" placeholder="服务IP:端口" value="http://192.168.1.100:5678" required>
<div class="valid-feedback"></div>
</div>
<div class="col-md-4">
<label for="startDate" class="form-label"><i class="fa fa-calendar-days" style="color:gray"></i>日期: </label>
<input type="text" class="form-control" id="startDate" name="startDate" style="width:250px;" placeholder="日期" value="" data-format="yyyy-MM-dd" required>
<div id="date-operate"></div>
<div class="valid-feedback"></div>
</div>
<div class="col-md-3">
<ul class="itemArea" >
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b01">
<input class="form-check-input" type="checkbox" checked="" data-code="B01" value="" id="b01">
进水参数
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b02">
<input class="form-check-input" type="checkbox" checked="" data-code="B02" value="" id="b02">
供水参数
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b03">
<input class="form-check-input" type="checkbox" checked="" data-code="B03" value="" id="b03">
液位
</label>
</div>
</li>
<li role="menuitem" class="checkItem">
<div class="form-check">
<label class="form-check-label" for="b04">
<input class="form-check-input" type="checkbox" checked="" data-code="B04" value="" id="b04">
供水压力
</label>
</div>
</li>
</ul>
</div>
<div class="col-md-2" style="display: flex; justify-content: right;">
<button class="btn btn-primary search" onclick="searchReport()" style="height:40px;margin:0 10px 0 0;"> <i class="fa fa-search"></i>搜索</button>
<button class="btn btn-success" onclick="exportData()" style="height:40px;"> <i class="fa fa-cloud-download" aria-hidden="true"></i>导出</button>
</div>
</div>
</div>
</div>
</div>
<div class="row" id="infoArea">
<div class="col-sm-12">
<ul class="nav nav-tabs" id="navList" role="tablist" style="margin: 20px 0 0 0;">
<li class="nav-item" data-name = "Tab1" onclick="getData('day')">
<a class="nav-link active" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-table"></i>库存表</a>
</li>
<!--<li class="nav-item" data-name = "Tab2" onclick="getData('month')">
<a class="nav-link" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-chart-simple"></i>月报表</a>
</li>
<li class="nav-item" data-name = "Tab3" onclick="getData('year')">
<a class="nav-link" data-toggle="tab" href="#reportTab" role="tab" aria-current="page" aria-controls="tab" aria-selected="true"><i class="fa fa-chart-simple"></i>年报表</a>
</li>-->
</ul>
<div class="tab-content" id="tabContent">
<div id="toolbar" class="btn-group">
<button id="btn_add" type="button" onclick="add()" class="btn btn-primary btn-sm rightSize">
<span class="fa fa-plus" aria-hidden="true"></span>新购入库
</button>
<button id="btn_edit" type="button" onclick="edit()" class="btn btn-secondary btn-sm rightSize">
<span class="fa fa-edit" aria-hidden="true"></span>修改
</button>
<button id="btn_delete" type="button" onclick="remove()" class="btn btn-warning btn-sm rightSize">
<span class="fa fa-remove" aria-hidden="true"></span>删除
</button>
<button id="btn_delete_batch" class="btn btn-danger btn-sm btn-del " onclick="removeBatch()">
<i class="fa fa-remove"></i>批量删除
</button>
<!--
<button class="btn btn-info btn-sm " data-bs-toggle="modal" data-bs-target="#myModal" >
<i class="fa fa-window-restore"></i>弹窗
</button>-->
<!--<button class="btn btn-primary btn-sm" onclick="fullscreen()">
<i class="fa fa-expand-arrows-alt"></i>全屏
</button>-->
<!--<button class="btn btn-success btn-sm" onclick="btnTask()">
<i class="fa fa-user-times"></i>分时任务
</button>-->
</div>
<div id="reportTab" class="tab-pane fade show active" id="nav-home" role="tabpanel" aria-labelledby="nav-home-tab">
<table id="report-table"></table>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- 模态框(Modal) -->
<div class="modal fade" id="myModal1" tabindex="-1" role="dialog" aria-labelledby="myModal1Label" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h4 class="modal-title" id="myModal1Label">
表格数据动态生成
</h4>
<button type="button" class="close" data-bs-dismiss="modal" aria-hidden="true">
<span class="fa fa-close" aria-hidden="true"></span>
</button>
</div>
<div class="modal-body">
<div class="wrapper wrapper-content animated fadeInRight ibox-content">
<form class="form-horizontal m" id="form-madArea-add">
<!--<div class="form-group">
<label class="col-sm-3 control-label ">代码:</label>
<div class="col-sm-8">
<input class="form-control" type="text" name="tcode" id="tcode"/>
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">名称:</label>
<div class="col-sm-8">
<input class="form-control" type="text" name="tname" id="tname">
</div>
</div>
<div class="form-group">
<label class="col-sm-3 control-label">备注:</label>
<div class="col-sm-8">
<input class="form-control" type="text" name="remark" id="remark">
</div>
</div>-->
<div class="form-group">
<label class="col-sm-3 control-label " for="number">需要的数据量:</label>
<div class="col-sm-8">
<input class="form-control" type="text" id="number" name="number" value="1000">
</div>
</div>
<!--<div class="col-sm-12">
<div class="form-group">
<div class="pull-right">
<button id="button_preservation" type="button" class="btn btn-success">保存</button>
<button id="button_cancel" type="button" class="btn btn-warning">取消</button>
</div>
</div>
</div>-->
</form>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-bs-dismiss="modal">关闭</button>
<button type="button" class="btn btn-primary" onclick="submitFun()">提交</button>
</div>
</div>
<!-- /.modal-content -->
</div><!-- /.modal -->
</div>
<!-- 模态框(Modal) -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog" style="max-width: fit-content;">
<div class="modal-content" style="width: 80vw;">
<div class="modal-header">
<h4 class="modal-title" id="myModalLabel">
修改
</h4>
<button type="button" class="close" data-bs-dismiss="modal" aria-hidden="true">
<span class="fa fa-close" aria-hidden="true"></span>
</button>
</div>
<div class="modal-body">
<div class="wrapper wrapper-content animated fadeInRight ibox-content">
<form class="form-horizontal m" id="editerModal" style="display: flex;flex-wrap: wrap;">
<!--<div class="col-sm-12">
<div class="form-group">
<div class="pull-right">
<button id="button_preservation" type="button" class="btn btn-success">保存</button>
<button id="button_cancel" type="button" class="btn btn-warning">取消</button>
</div>
</div>
</div>-->
</form>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-bs-dismiss="modal">关闭</button>
<button type="button" class="btn btn-primary" onclick="saveEdit()">提交</button>
</div>
</div>
<!-- /.modal-content -->
</div><!-- /.modal -->
</div>
<script type="lslsls" id="worker">
// worker.js代码写入html中
// 在worker.js里面 定义一个函数来执行计算
function compute(num) {
let sum = 0;
for (let i = 0; i < num; i++) {
sum += i;
}
return sum;
}
function randomNum(maxNum, minNum, decimalNum) {
let max = 0, min = 0;
minNum <= maxNum ? (min = minNum, max = maxNum) : (min = maxNum, max = minNum);
switch (arguments.length) {
case 1:
return Math.floor(Math.random() * (max + 1));
break;
case 2:
return Math.floor(Math.random() * (max - min + 1) + min);
break;
case 3:
return (Math.random() * (max - min) + min).toFixed(decimalNum);
break;
default:
return Math.random();
break;
}
}
function addMockData(n){
let mockDatas=[];
const liuliang = randomNum(0,1000)
const leijiliuliang = randomNum(100000,1000000)
for(let i = 0;i< n;i++){
let temp = {
uid: i,
time: new Date().toLocaleDateString('en-GB'),
value0: ((liuliang+i)*Math.random()).toFixed(1),
value1: leijiliuliang+i,
value2: randomNum(0,10,2),
value3: randomNum(6.5,8.5,2),
value4: randomNum(20,30,2),
value5: ((liuliang+i)*Math.random()).toFixed(1),
value6: leijiliuliang+i,
value7: ((liuliang+i)*Math.random()).toFixed(1),
value8: leijiliuliang+i,
value9: ((liuliang+i)*Math.random()).toFixed(1),
value10: leijiliuliang+i,
value11: randomNum(0,1,2),
value12: randomNum(1,1,2),
value13: randomNum(6.5,8.5,2),
value14: randomNum(20,30,2),
value15: randomNum(3,5,2),
value16: randomNum(3,5,2),
value17: randomNum(0,1,2),
value18: randomNum(0,1,2),
value19: randomNum(0,1,2),
value20: randomNum(1,100),
value21: randomNum(1,100),
}
mockDatas.push(temp)
}
return mockDatas
}
// 定义一个函数来处理来自主线程的消息
//add event listener to handle the different message
onmessage = async function(e) {
console.log("Worker: Message received from main script");
const num = e.data.num;
const res1 = compute(num);
postMessage(res1);
const res2 = addMockData(num);
postMessage(res2);
const urls = e.data.urls;
const results = await Promise.all(urls.map(request));
postMessage(results);
if (e.data.action === 'start') {
// 使用setTimeout来异步执行一些计算
setTimeout(() => {
// 将结果发送回主线程
postMessage({ action: 'result', data: res1 });
}, 0);
}
};
// 在worker.js里面:
// 定义一个函数来处理网络请求。
function request(url) {
return fetch(url).then(response => response.json());
}
// 创建消息队列来累积消息
const messageQueue = [];
// 创建一个将消息添加到队列的函数
function addToQueue(message) {
messageQueue.push(message);
// 检查队列是否已达到阈值大小。
if (messageQueue.length >= 10) {
// 如果是,将批处理消息发送到主线程。
postMessage(messageQueue);
// 清除消息队列。
messageQueue.length = 0;
}
}
// 向队列中添加消息
addToQueue({type: 'log', message: 'Hello, world!'});
for(let i = 0; i< 9;i++){
// 向队列中添加另一条消息
addToQueue({type: 'info', message: 'An info '+i+Math.random()+' occurred.'});
}
function doSomeComputation(data){
return data
}
function processItem(data){
return data
}
</script>
<script>
function formatDate(date) {
const day = date.getDate().toString().padStart(2, '0');
const month = (date.getMonth() + 1).toString().padStart(2, '0'); // 月份是从0开始的
const year = date.getFullYear();
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
const seconds = date.getSeconds().toString().padStart(2, '0');
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
}
let pid1
pid1 = setInterval(()=>{
// 使用示例
const now = new Date()
// 输出格式如: "2023-03-15 12:30:45"
document.getElementById('time').innerHTML = formatDate(now)
},0)
let myWorker
document.querySelector('#number').onchange = function() {
console.log('数据量:',document.querySelector('#number').value);
}
function startWorker() {
let message = {
num: document.querySelector('#number').value,
urls: ['http://192.168.88.220:3333/local.json', 'http://192.168.88.220:3333/local.json']
}
if (typeof Worker !== 'undefined') {
if(typeof(myWorker) == "undefined") {
// 支持Web worker 创建一个新的Web Worker
const blob = new Blob([document.getElementById('worker').textContent]);
const url = window.URL.createObjectURL(blob)
myWorker = new Worker(url);
}
// 定义一个函数来处理来自工作线程的消息
myWorker.onmessage = function(e) {
console.log('Message received from worker');
const res = e.data;
if(res.length==message.num){
tableData = res||[];
initTable(res);
}else{
console.log(res);
}
}
// 向worker发送消息以启动计算 启动请求
myWorker.postMessage(message);
} else {
// 不支持
console.log('Web Workers are not supported in this browser.');
console.error("抱歉,你的浏览器不支持 Web Workers...");
}
}
function stopWorker() {
if(typeof(myWorker) != "undefined") {
myWorker.terminate();
myWorker = undefined;
}
if(pid1){
clearInterval(pid1);
}
}
try {
//run(main)
}
catch(err) {
console.log(err)
}
finally {
console.log('finally done')
}
var queryParams = function (params) {
var param = {
pageIndex: Math.ceil(params.offset / params.limit) + 1,
pageSize: params.limit,
order: params.order,
ordername: params.sort,
time: $("#dateSearch .startDate").val(),
//startDateTime: $("#dateSearch .startDate").val(),
//endDateTime: $("#dateSearch .endDate").val(),
search: $("#dateSearch .imuserid").val()
};
//console.log(param)
return param;
}
//查询条件
function queryParams1(params){
params['project_id'] = $("select[name=project_id]").find("option:selected").val();
params['time_field'] = $("select[name=time_field]").find("option:selected").val();
params['start_time'] = $("input[name=start_time]").val();
params['end_time'] = $("input[name=end_time]").val();
params['user_name'] = $("input[name=user_name]").val();
params['telephone'] = $("input[name=telephone]").val();
params['room_confirm_number'] = $("input[name=room_confirm_number]").val();
params['lineson'] = $("select[name=lineson]").val();
params['invoice'] = $("select[name=invoice]").val();
return params;
}
var responseHandler = function (e) {
if (e.data && e.data.length > 0) {
return { "rows": e.data, "total": e.count };
}
else {
return { "rows": [], "total": 0 };
}
}
var uidHandle = function (value, row, index) {
var html = "<a href='#'>"+ res + "</a>";
return html;
}
var operateFormatterStatus = function (value, row, index) {//赋予的参数
let htmlDoc = ''
if(value == '出库'){
htmlDoc = `<button class="btn btn-info btn-sm rightSize detailBtn" type="button" >${value}</button>`
}else if(value == '在库'){
htmlDoc = `<button class="btn btn-primary btn-sm rightSize detailBtn" type="button" >${value}</button>`
}else if(value == '报废'){
htmlDoc = `<button class="btn btn-danger btn-sm rightSize detailBtn" type="button" >${value}</button>`
}
return [htmlDoc].join('');
}
var operateFormatter = function (value, row, index) {//赋予的参数
return [
`<div style="width: 250px;display: flex;justify-content: space-around;">
<button class="btn btn-info btn-sm rightSize detailBtn" type="button" onclick="changeLinenStatus('${index}','出库')"><i class="fa fa-out"></i>污衣出库</button>
<button class="btn btn-primary btn-sm rightSize detailBtn" type="button" onclick="changeLinenStatus('${index}','在库')"><i class="fa fa-in"></i>净衣入库</button>
<button class="btn btn-danger btn-sm rightSize packageBtn" type="button" onclick="changeLinenStatus('${index}','报废')"><i class="fa fa-delete"></i>报废</button>
</div>`
].join('');
}
var imageFormatter = function (value, row, index) {//赋予的参数
return [
'<div style="display: flex;justify-content: start;">',
'<img style="max-width: 300px;" src="'+value+'"/>',
'</div>'
].join('');
}
var rowStyle = function (row, index) {
var classes = ['success', 'info'];
if (index % 2 === 0) {//偶数行
return { classes: classes[0]};
} else {//奇数行
return {classes: classes[1]};
}
}
const tableName = "report-table";
var tableType = 'day'
function selectType(){
const date = $('#startDate').datepicker('getDate');
let time = ''
if(tableType=='day'){
time = date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate();
}else if(tableType=='month'){
time = date.getFullYear() + "-" + (date.getMonth() + 1);
}else if(tableType=='year'){
time = date.getFullYear();
}
return time
}
function reportName(){
let name = ''
let time = ''
const date = $('#startDate').datepicker('getDate');
if(tableType=='day'){
name = '日报表'
time = date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate();
}else if(tableType=='month'){
name = '月报表'
time = date.getFullYear() + "-" + (date.getMonth() + 1);
}else if(tableType=='year'){
name = '年报表'
time = date.getFullYear();
}
return name + '_' + time
}
function searchReport(){
buildTableColumns(); //根据因子选择状态创建表格属性
initTable();
//getServerData();
}
function getServerData(){
let timeformatted = selectType();
const serverUrl = $('#ip-port').val();
const url = serverUrl+'/api/SystemMonitor/GetReport?type='+tableType+'&time='+timeformatted;
const authorization = 'Bearer '+'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJVc2VySWQiOjE0MjMwNzA3MDkxMDU1MSwiVGVuYW50SWQiOjE0MjMwNzA3MDkxODc4MCwiQWNjb3VudCI6InN5c3RlbSIsIk5hbWUiOiLotoXnuqfnrqHnkIblkZgiLCJTdXBlckFkbWluIjoxLCJLZWxpU3VwZXJBZG1pbiI6MSwiT3JnSWQiOjE0MjMwNzA3MDkxMDUzOSwiT3JnTmFtZSI6IumbhuWbouWFrOWPuDk5OSIsImlhdCI6MTcwNDQ0MDM4NSwibmJmIjoxNzA0NDQwMzg1LCJleHAiOjE3MDcwMzIzODUsImlzcyI6IktlbGkiLCJhdWQiOiJLZWxpIn0.MHS1KUqzq8bKytYVH3W8pdjcr8EOVlFpxc-a5CaU64g'
$.ajax({
url: url,
method: 'get',
dataType: 'json',
//beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', authorization);
//},
//headers: {'Accept': 'application/json', 'Authorization': authorization},
contentType: "application/json,charset=utf-8",
success: (res)=> {
if(res.code==200){
tableData = res.data||[];
initTable();
}else{
alert(JSON.stringify(res.data))
}
return;
},
error: function(res) {
alert(JSON.stringify(res))
}
});
}
function typeView(type){
let viewMode = ''
switch(type){
case 'day':
viewMode = 'days'
break;
case 'month':
viewMode = 'months'
break;
case 'year':
viewMode = 'years'
break;
default:
break;
}
return viewMode
}
function viewModeTable(viewMode){
let tabType = ''
switch(viewMode){
case 'days':
tabType = 'day'
break;
case 'months':
tabType = 'month'
break;
case 'years':
tabType = 'year'
break;
default:
break;
}
return tabType
}
function getData(type){
tableType = type;
selectDateBtn(typeView(type));
searchReport();
}
function exportData(){
if (this.tableData && tableData.length) {
const timeformatted = selectType();
const serverUrl = $('#ip-port').val()
const url = serverUrl+'/api/SystemMonitor/ExportReport?type='+tableType+'&time='+timeformatted;
//const authorization = 'Bearer '+'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJVc2VySWQiOjE0MjMwNzA3MDkxMDU1MSwiVGVuYW50SWQiOjE0MjMwNzA3MDkxODc4MCwiQWNjb3VudCI6InN5c3RlbSIsIk5hbWUiOiLotoXnuqfnrqHnkIblkZgiLCJTdXBlckFkbWluIjoxLCJLZWxpU3VwZXJBZG1pbiI6MSwiT3JnSWQiOjE0MjMwNzA3MDkxMDUzOSwiT3JnTmFtZSI6IumbhuWbouWFrOWPuDk5OSIsImlhdCI6MTcwNDQ0MDM4NSwibmJmIjoxNzA0NDQwMzg1LCJleHAiOjE3MDcwMzIzODUsImlzcyI6IktlbGkiLCJhdWQiOiJLZWxpIn0.MHS1KUqzq8bKytYVH3W8pdjcr8EOVlFpxc-a5CaU64g'
$.ajax({
url: url,
method: 'get',
dataType: 'json',
//beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', authorization);
//},
//headers: {'Accept': 'application/json', 'Authorization': authorization},
contentType: "application/json,charset=utf-8",
success: (res)=> {
//console.log(res);
if(res.code==200){
if (res.data) {
let fileUrl = serverUrl + res.data;
let rename = reportName();
fileDownload(fileUrl, rename+'下载.html');
}
}
return;
},
error: function(res) {
console.log(res);
}
});
}
}
function fileDownload(content, filename) {
// 创建隐藏的可下载链接
var eleLink = document.createElement('a');
eleLink.download = filename;
eleLink.style.display = 'none';
// 字符内容转变成blob地址
//var blob = new Blob([content]);
//eleLink.href = URL.createObjectURL(blob);
eleLink.href = content;
// 触发点击
document.body.appendChild(eleLink);
eleLink.click();
// 然后移除
document.body.removeChild(eleLink);
};
const bucaoData = [
{"ProductCode":"BCA001","Variety":"加加大码长袖长装工作服","Specification":"XXL","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA001_加加大码长袖长装工作服_XXL_白色_件","RFIDElectronicTag":"BCA001XXLA00140","Barcode":"BCA001XXLA00140","StorageArea":"布草仓库","WarehouseLocationCode":"A001","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA002","Variety":"加大码长袖长装工作服","Specification":"XL","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA002_加大码长袖长装工作服_XL_白色_件","RFIDElectronicTag":"BCA002XLA00260","Barcode":"BCA002XLA00260","StorageArea":"布草仓库","WarehouseLocationCode":"A002","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA003","Variety":"大码长袖长装工作服","Specification":"L","ColorPattern":"白色","Quantity":100,"Unit":"件","Description":"BCA003_大码长袖长装工作服_L_白色_件","RFIDElectronicTag":"BCA003LA003100","Barcode":"BCA003LA003100","StorageArea":"布草仓库","WarehouseLocationCode":"A003","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA004","Variety":"中码长袖长装工作服","Specification":"M","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA004_中码长袖长装工作服_M_白色_件","RFIDElectronicTag":"BCA004MA00460","Barcode":"BCA004MA00460","StorageArea":"布草仓库","WarehouseLocationCode":"A004","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA005","Variety":"小码长袖长装工作服","Specification":"S","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA005_小码长袖长装工作服_S_白色_件","RFIDElectronicTag":"BCA005SA00540","Barcode":"BCA005SA00540","StorageArea":"布草仓库","WarehouseLocationCode":"A005","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA006","Variety":"加加大码短袖长装工作服","Specification":"XXL","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA006_加加大码短袖长装工作服_XXL_白色_件","RFIDElectronicTag":"BCA006XXLA00640","Barcode":"BCA006XXLA00640","StorageArea":"布草仓库","WarehouseLocationCode":"A006","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA007","Variety":"加大码短袖长装工作服","Specification":"XL","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA007_加大码短袖长装工作服_XL_白色_件","RFIDElectronicTag":"BCA007XLA00760","Barcode":"BCA007XLA00760","StorageArea":"布草仓库","WarehouseLocationCode":"A007","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA008","Variety":"大码短袖长装工作服","Specification":"L","ColorPattern":"白色","Quantity":100,"Unit":"件","Description":"BCA008_大码短袖长装工作服_L_白色_件","RFIDElectronicTag":"BCA008LA008100","Barcode":"BCA008LA008100","StorageArea":"布草仓库","WarehouseLocationCode":"A008","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA009","Variety":"中码短袖长装工作服","Specification":"M","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA009_中码短袖长装工作服_M_白色_件","RFIDElectronicTag":"BCA009MA00960","Barcode":"BCA009MA00960","StorageArea":"布草仓库","WarehouseLocationCode":"A009","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA010","Variety":"小码短袖长装工作服","Specification":"S","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA010_小码短袖长装工作服_S_白色_件","RFIDElectronicTag":"BCA010SA01040","Barcode":"BCA010SA01040","StorageArea":"布草仓库","WarehouseLocationCode":"A010","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA011","Variety":"加加大码长袖短装工作服","Specification":"XXL","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA011_加加大码长袖短装工作服_XXL_白色_件","RFIDElectronicTag":"BCA011XXLA01140","Barcode":"BCA011XXLA01140","StorageArea":"布草仓库","WarehouseLocationCode":"A011","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA012","Variety":"加大码长袖短装工作服","Specification":"XL","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA012_加大码长袖短装工作服_XL_白色_件","RFIDElectronicTag":"BCA012XLA01260","Barcode":"BCA012XLA01260","StorageArea":"布草仓库","WarehouseLocationCode":"A012","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA013","Variety":"大码长袖短装工作服","Specification":"L","ColorPattern":"白色","Quantity":100,"Unit":"件","Description":"BCA013_大码长袖短装工作服_L_白色_件","RFIDElectronicTag":"BCA013LA013100","Barcode":"BCA013LA013100","StorageArea":"布草仓库","WarehouseLocationCode":"A013","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA014","Variety":"中码长袖短装工作服","Specification":"M","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA014_中码长袖短装工作服_M_白色_件","RFIDElectronicTag":"BCA014MA01460","Barcode":"BCA014MA01460","StorageArea":"布草仓库","WarehouseLocationCode":"A014","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA015","Variety":"小码长袖短装工作服","Specification":"S","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA015_小码长袖短装工作服_S_白色_件","RFIDElectronicTag":"BCA015SA01540","Barcode":"BCA015SA01540","StorageArea":"布草仓库","WarehouseLocationCode":"A015","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA016","Variety":"加加大码短袖短装工作服","Specification":"XXL","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA016_加加大码短袖短装工作服_XXL_白色_件","RFIDElectronicTag":"BCA016XXLA01640","Barcode":"BCA016XXLA01640","StorageArea":"布草仓库","WarehouseLocationCode":"A016","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA017","Variety":"加大码短袖短装工作服","Specification":"XL","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA017_加大码短袖短装工作服_XL_白色_件","RFIDElectronicTag":"BCA017XLA01760","Barcode":"BCA017XLA01760","StorageArea":"布草仓库","WarehouseLocationCode":"A017","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA018","Variety":"大码短袖短装工作服","Specification":"L","ColorPattern":"白色","Quantity":100,"Unit":"件","Description":"BCA018_大码短袖短装工作服_L_白色_件","RFIDElectronicTag":"BCA018LA018100","Barcode":"BCA018LA018100","StorageArea":"布草仓库","WarehouseLocationCode":"A018","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA019","Variety":"中码短袖短装工作服","Specification":"M","ColorPattern":"白色","Quantity":60,"Unit":"件","Description":"BCA019_中码短袖短装工作服_M_白色_件","RFIDElectronicTag":"BCA019MA01960","Barcode":"BCA019MA01960","StorageArea":"布草仓库","WarehouseLocationCode":"A019","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA020","Variety":"小码短袖短装工作服","Specification":"S","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA020_小码短袖短装工作服_S_白色_件","RFIDElectronicTag":"BCA020SA02040","Barcode":"BCA020SA02040","StorageArea":"布草仓库","WarehouseLocationCode":"A020","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA021","Variety":"加加大码长袖长装孕妇服","Specification":"XXL","ColorPattern":"白色","Quantity":10,"Unit":"件","Description":"BCA021_加加大码长袖长装孕妇服_XXL_白色_件","RFIDElectronicTag":"BCA021XXLA02110","Barcode":"BCA021XXLA02110","StorageArea":"布草仓库","WarehouseLocationCode":"A021","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA022","Variety":"加大码长袖长装孕妇服","Specification":"XL","ColorPattern":"白色","Quantity":20,"Unit":"件","Description":"BCA022_加大码长袖长装孕妇服_XL_白色_件","RFIDElectronicTag":"BCA022XLA02220","Barcode":"BCA022XLA02220","StorageArea":"布草仓库","WarehouseLocationCode":"A022","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA023","Variety":"大码长袖长装孕妇服","Specification":"L","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA023_大码长袖长装孕妇服_L_白色_件","RFIDElectronicTag":"BCA023LA02340","Barcode":"BCA023LA02340","StorageArea":"布草仓库","WarehouseLocationCode":"A023","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA024","Variety":"中码长袖长装孕妇服","Specification":"M","ColorPattern":"白色","Quantity":20,"Unit":"件","Description":"BCA024_中码长袖长装孕妇服_M_白色_件","RFIDElectronicTag":"BCA024MA02420","Barcode":"BCA024MA02420","StorageArea":"布草仓库","WarehouseLocationCode":"A024","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA025","Variety":"小码长袖长装孕妇服","Specification":"S","ColorPattern":"白色","Quantity":10,"Unit":"件","Description":"BCA025_小码长袖长装孕妇服_S_白色_件","RFIDElectronicTag":"BCA025SA02510","Barcode":"BCA025SA02510","StorageArea":"布草仓库","WarehouseLocationCode":"A025","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA026","Variety":"加加大码短袖长装孕妇服","Specification":"XXL","ColorPattern":"白色","Quantity":10,"Unit":"件","Description":"BCA026_加加大码短袖长装孕妇服_XXL_白色_件","RFIDElectronicTag":"BCA026XXLA02610","Barcode":"BCA026XXLA02610","StorageArea":"布草仓库","WarehouseLocationCode":"A026","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA027","Variety":"加大码短袖长装孕妇服","Specification":"XL","ColorPattern":"白色","Quantity":20,"Unit":"件","Description":"BCA027_加大码短袖长装孕妇服_XL_白色_件","RFIDElectronicTag":"BCA027XLA02720","Barcode":"BCA027XLA02720","StorageArea":"布草仓库","WarehouseLocationCode":"A027","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA028","Variety":"大码短袖长装孕妇服","Specification":"L","ColorPattern":"白色","Quantity":40,"Unit":"件","Description":"BCA028_大码短袖长装孕妇服_L_白色_件","RFIDElectronicTag":"BCA028LA02840","Barcode":"BCA028LA02840","StorageArea":"布草仓库","WarehouseLocationCode":"A028","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA029","Variety":"中码短袖长装孕妇服","Specification":"M","ColorPattern":"白色","Quantity":20,"Unit":"件","Description":"BCA029_中码短袖长装孕妇服_M_白色_件","RFIDElectronicTag":"BCA029MA02920","Barcode":"BCA029MA02920","StorageArea":"布草仓库","WarehouseLocationCode":"A029","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA030","Variety":"小码短袖长装孕妇服","Specification":"S","ColorPattern":"白色","Quantity":10,"Unit":"件","Description":"BCA030_小码短袖长装孕妇服_S_白色_件","RFIDElectronicTag":"BCA030SA03010","Barcode":"BCA030SA03010","StorageArea":"布草仓库","WarehouseLocationCode":"A030","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA031","Variety":"加加大码护士长裤","Specification":"XXL","ColorPattern":"紫色","Quantity":40,"Unit":"件","Description":"BCA031_加加大码护士长裤_XXL_紫色_件","RFIDElectronicTag":"BCA031XXLA03140","Barcode":"BCA031XXLA03140","StorageArea":"布草仓库","WarehouseLocationCode":"A031","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA032","Variety":"加大码护士长裤","Specification":"XL","ColorPattern":"紫色","Quantity":60,"Unit":"件","Description":"BCA032_加大码护士长裤_XL_紫色_件","RFIDElectronicTag":"BCA032XLA03260","Barcode":"BCA032XLA03260","StorageArea":"布草仓库","WarehouseLocationCode":"A032","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA033","Variety":"大码护士长裤","Specification":"L","ColorPattern":"紫色","Quantity":100,"Unit":"件","Description":"BCA033_大码护士长裤_L_紫色_件","RFIDElectronicTag":"BCA033LA033100","Barcode":"BCA033LA033100","StorageArea":"布草仓库","WarehouseLocationCode":"A033","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA034","Variety":"中码护士长裤","Specification":"M","ColorPattern":"紫色","Quantity":60,"Unit":"件","Description":"BCA034_中码护士长裤_M_紫色_件","RFIDElectronicTag":"BCA034MA03460","Barcode":"BCA034MA03460","StorageArea":"布草仓库","WarehouseLocationCode":"A034","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA035","Variety":"小码护士长裤","Specification":"S","ColorPattern":"紫色","Quantity":40,"Unit":"件","Description":"BCA035_小码护士长裤_S_紫色_件","RFIDElectronicTag":"BCA035SA03540","Barcode":"BCA035SA03540","StorageArea":"布草仓库","WarehouseLocationCode":"A035","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA036","Variety":"加加大码护士短裤","Specification":"XXL","ColorPattern":"紫色","Quantity":10,"Unit":"件","Description":"BCA036_加加大码护士短裤_XXL_紫色_件","RFIDElectronicTag":"BCA036XXLA03610","Barcode":"BCA036XXLA03610","StorageArea":"布草仓库","WarehouseLocationCode":"A036","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA037","Variety":"加大码护士短裤","Specification":"XL","ColorPattern":"紫色","Quantity":20,"Unit":"件","Description":"BCA037_加大码护士短裤_XL_紫色_件","RFIDElectronicTag":"BCA037XLA03720","Barcode":"BCA037XLA03720","StorageArea":"布草仓库","WarehouseLocationCode":"A037","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA038","Variety":"大码护士短裤","Specification":"L","ColorPattern":"紫色","Quantity":40,"Unit":"件","Description":"BCA038_大码护士短裤_L_紫色_件","RFIDElectronicTag":"BCA038LA03840","Barcode":"BCA038LA03840","StorageArea":"布草仓库","WarehouseLocationCode":"A038","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA039","Variety":"中码护士短裤","Specification":"M","ColorPattern":"紫色","Quantity":20,"Unit":"件","Description":"BCA039_中码护士短裤_M_紫色_件","RFIDElectronicTag":"BCA039MA03920","Barcode":"BCA039MA03920","StorageArea":"布草仓库","WarehouseLocationCode":"A039","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA040","Variety":"小码护士短裤","Specification":"S","ColorPattern":"紫色","Quantity":10,"Unit":"件","Description":"BCA040_小码护士短裤_S_紫色_件","RFIDElectronicTag":"BCA040SA04010","Barcode":"BCA040SA04010","StorageArea":"布草仓库","WarehouseLocationCode":"A040","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA041","Variety":"加加大码长袖洗手衣","Specification":"XXL","ColorPattern":"兰色","Quantity":800,"Unit":"件","Description":"BCA041_加加大码长袖洗手衣_XXL_兰色_件","RFIDElectronicTag":"BCA041XXLA041800","Barcode":"BCA041XXLA041800","StorageArea":"布草仓库","WarehouseLocationCode":"A041","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA042","Variety":"加大码长袖洗手衣","Specification":"XL","ColorPattern":"兰色","Quantity":1200,"Unit":"件","Description":"BCA042_加大码长袖洗手衣_XL_兰色_件","RFIDElectronicTag":"BCA042XLA0421200","Barcode":"BCA042XLA0421200","StorageArea":"布草仓库","WarehouseLocationCode":"A042","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA043","Variety":"大码长袖洗手衣","Specification":"L","ColorPattern":"兰色","Quantity":2000,"Unit":"件","Description":"BCA043_大码长袖洗手衣_L_兰色_件","RFIDElectronicTag":"BCA043LA0432000","Barcode":"BCA043LA0432000","StorageArea":"布草仓库","WarehouseLocationCode":"A043","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA044","Variety":"中码长袖洗手衣","Specification":"M","ColorPattern":"兰色","Quantity":1200,"Unit":"件","Description":"BCA044_中码长袖洗手衣_M_兰色_件","RFIDElectronicTag":"BCA044MA0441200","Barcode":"BCA044MA0441200","StorageArea":"布草仓库","WarehouseLocationCode":"A044","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA045","Variety":"小码长袖洗手衣","Specification":"S","ColorPattern":"兰色","Quantity":800,"Unit":"件","Description":"BCA045_小码长袖洗手衣_S_兰色_件","RFIDElectronicTag":"BCA045SA045800","Barcode":"BCA045SA045800","StorageArea":"布草仓库","WarehouseLocationCode":"A045","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA046","Variety":"加加大码短袖洗手衣","Specification":"XXL","ColorPattern":"兰色","Quantity":1000,"Unit":"件","Description":"BCA046_加加大码短袖洗手衣_XXL_兰色_件","RFIDElectronicTag":"BCA046XXLA0461000","Barcode":"BCA046XXLA0461000","StorageArea":"布草仓库","WarehouseLocationCode":"A046","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA047","Variety":"加大码短袖洗手衣","Specification":"XL","ColorPattern":"兰色","Quantity":2500,"Unit":"件","Description":"BCA047_加大码短袖洗手衣_XL_兰色_件","RFIDElectronicTag":"BCA047XLA0472500","Barcode":"BCA047XLA0472500","StorageArea":"布草仓库","WarehouseLocationCode":"A047","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA048","Variety":"大码短袖洗手衣","Specification":"L","ColorPattern":"兰色","Quantity":3000,"Unit":"件","Description":"BCA048_大码短袖洗手衣_L_兰色_件","RFIDElectronicTag":"BCA048LA0483000","Barcode":"BCA048LA0483000","StorageArea":"布草仓库","WarehouseLocationCode":"A048","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA049","Variety":"中码短袖洗手衣","Specification":"M","ColorPattern":"兰色","Quantity":2500,"Unit":"件","Description":"BCA049_中码短袖洗手衣_M_兰色_件","RFIDElectronicTag":"BCA049MA0492500","Barcode":"BCA049MA0492500","StorageArea":"布草仓库","WarehouseLocationCode":"A049","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA050","Variety":"小码短袖洗手衣","Specification":"S","ColorPattern":"兰色","Quantity":1000,"Unit":"件","Description":"BCA050_小码短袖洗手衣_S_兰色_件","RFIDElectronicTag":"BCA050SA0501000","Barcode":"BCA050SA0501000","StorageArea":"布草仓库","WarehouseLocationCode":"A050","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA051","Variety":"加加大码洗手裤","Specification":"XXL","ColorPattern":"兰色","Quantity":1000,"Unit":"件","Description":"BCA051_加加大码洗手裤_XXL_兰色_件","RFIDElectronicTag":"BCA051XXLA0511000","Barcode":"BCA051XXLA0511000","StorageArea":"布草仓库","WarehouseLocationCode":"A051","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA052","Variety":"加大码洗手裤","Specification":"XL","ColorPattern":"兰色","Quantity":2500,"Unit":"件","Description":"BCA052_加大码洗手裤_XL_兰色_件","RFIDElectronicTag":"BCA052XLA0522500","Barcode":"BCA052XLA0522500","StorageArea":"布草仓库","WarehouseLocationCode":"A052","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA053","Variety":"大码洗手裤","Specification":"L","ColorPattern":"兰色","Quantity":3000,"Unit":"件","Description":"BCA053_大码洗手裤_L_兰色_件","RFIDElectronicTag":"BCA053LA0533000","Barcode":"BCA053LA0533000","StorageArea":"布草仓库","WarehouseLocationCode":"A053","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA054","Variety":"中码洗手裤","Specification":"M","ColorPattern":"兰色","Quantity":2500,"Unit":"件","Description":"BCA054_中码洗手裤_M_兰色_件","RFIDElectronicTag":"BCA054MA0542500","Barcode":"BCA054MA0542500","StorageArea":"布草仓库","WarehouseLocationCode":"A054","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA055","Variety":"小码洗手裤","Specification":"S","ColorPattern":"兰色","Quantity":1000,"Unit":"件","Description":"BCA055_小码洗手裤_S_兰色_件","RFIDElectronicTag":"BCA055SA0551000","Barcode":"BCA055SA0551000","StorageArea":"布草仓库","WarehouseLocationCode":"A055","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA056","Variety":"加加大码手术衣","Specification":"XXL","ColorPattern":"绿色","Quantity":200,"Unit":"件","Description":"BCA056_加加大码手术衣_XXL_绿色_件","RFIDElectronicTag":"BCA056XXLA056200","Barcode":"BCA056XXLA056200","StorageArea":"布草仓库","WarehouseLocationCode":"A056","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA057","Variety":"加大码手术衣","Specification":"XL","ColorPattern":"绿色","Quantity":300,"Unit":"件","Description":"BCA057_加大码手术衣_XL_绿色_件","RFIDElectronicTag":"BCA057XLA057300","Barcode":"BCA057XLA057300","StorageArea":"布草仓库","WarehouseLocationCode":"A057","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA058","Variety":"大码手术衣","Specification":"L","ColorPattern":"绿色","Quantity":500,"Unit":"件","Description":"BCA058_大码手术衣_L_绿色_件","RFIDElectronicTag":"BCA058LA058500","Barcode":"BCA058LA058500","StorageArea":"布草仓库","WarehouseLocationCode":"A058","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA059","Variety":"中码手术衣","Specification":"M","ColorPattern":"绿色","Quantity":300,"Unit":"件","Description":"BCA059_中码手术衣_M_绿色_件","RFIDElectronicTag":"BCA059MA059300","Barcode":"BCA059MA059300","StorageArea":"布草仓库","WarehouseLocationCode":"A059","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA060","Variety":"小码手术衣","Specification":"S","ColorPattern":"绿色","Quantity":200,"Unit":"件","Description":"BCA060_小码手术衣_S_绿色_件","RFIDElectronicTag":"BCA060SA060200","Barcode":"BCA060SA060200","StorageArea":"布草仓库","WarehouseLocationCode":"A060","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA061","Variety":"加加大码隔离衣","Specification":"XXL","ColorPattern":"兰色","Quantity":50,"Unit":"件","Description":"BCA061_加加大码隔离衣_XXL_兰色_件","RFIDElectronicTag":"BCA061XXLA06150","Barcode":"BCA061XXLA06150","StorageArea":"布草仓库","WarehouseLocationCode":"A061","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA062","Variety":"加大码隔离衣","Specification":"XL","ColorPattern":"兰色","Quantity":60,"Unit":"件","Description":"BCA062_加大码隔离衣_XL_兰色_件","RFIDElectronicTag":"BCA062XLA06260","Barcode":"BCA062XLA06260","StorageArea":"布草仓库","WarehouseLocationCode":"A062","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA063","Variety":"大码隔离衣","Specification":"L","ColorPattern":"兰色","Quantity":80,"Unit":"件","Description":"BCA063_大码隔离衣_L_兰色_件","RFIDElectronicTag":"BCA063LA06380","Barcode":"BCA063LA06380","StorageArea":"布草仓库","WarehouseLocationCode":"A063","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA064","Variety":"中码隔离衣","Specification":"M","ColorPattern":"兰色","Quantity":60,"Unit":"件","Description":"BCA064_中码隔离衣_M_兰色_件","RFIDElectronicTag":"BCA064MA06460","Barcode":"BCA064MA06460","StorageArea":"布草仓库","WarehouseLocationCode":"A064","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA065","Variety":"小码隔离衣","Specification":"S","ColorPattern":"兰色","Quantity":50,"Unit":"件","Description":"BCA065_小码隔离衣_S_兰色_件","RFIDElectronicTag":"BCA065SA06550","Barcode":"BCA065SA06550","StorageArea":"布草仓库","WarehouseLocationCode":"A065","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA066","Variety":"加加大码成人病人长袖衫","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":500,"Unit":"件","Description":"BCA066_加加大码成人病人长袖衫_XXL_蓝色格子条纹_件","RFIDElectronicTag":"BCA066XXLA066500","Barcode":"BCA066XXLA066500","StorageArea":"布草仓库","WarehouseLocationCode":"A066","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA067","Variety":"加大码成人病人长袖衫","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":600,"Unit":"件","Description":"BCA067_加大码成人病人长袖衫_XL_蓝色格子条纹_件","RFIDElectronicTag":"BCA067XLA067600","Barcode":"BCA067XLA067600","StorageArea":"布草仓库","WarehouseLocationCode":"A067","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA068","Variety":"大码成人病人长袖衫","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":800,"Unit":"件","Description":"BCA068_大码成人病人长袖衫_L_蓝色格子条纹_件","RFIDElectronicTag":"BCA068LA068800","Barcode":"BCA068LA068800","StorageArea":"布草仓库","WarehouseLocationCode":"A068","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA069","Variety":"中码成人病人长袖衫","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":600,"Unit":"件","Description":"BCA069_中码成人病人长袖衫_M_蓝色格子条纹_件","RFIDElectronicTag":"BCA069MA069600","Barcode":"BCA069MA069600","StorageArea":"布草仓库","WarehouseLocationCode":"A069","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA070","Variety":"小码成人病人长袖衫","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":500,"Unit":"件","Description":"BCA070_小码成人病人长袖衫_S_蓝色格子条纹_件","RFIDElectronicTag":"BCA070SA070500","Barcode":"BCA070SA070500","StorageArea":"布草仓库","WarehouseLocationCode":"A070","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA071","Variety":"加加大码成人病人长裤","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":500,"Unit":"条","Description":"BCA071_加加大码成人病人长裤_XXL_蓝色格子条纹_条","RFIDElectronicTag":"BCA071XXLA071500","Barcode":"BCA071XXLA071500","StorageArea":"布草仓库","WarehouseLocationCode":"A071","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA072","Variety":"加大码成人病人长裤","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":600,"Unit":"条","Description":"BCA072_加大码成人病人长裤_XL_蓝色格子条纹_条","RFIDElectronicTag":"BCA072XLA072600","Barcode":"BCA072XLA072600","StorageArea":"布草仓库","WarehouseLocationCode":"A072","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA073","Variety":"大码成人病人长裤","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":800,"Unit":"条","Description":"BCA073_大码成人病人长裤_L_蓝色格子条纹_条","RFIDElectronicTag":"BCA073LA073800","Barcode":"BCA073LA073800","StorageArea":"布草仓库","WarehouseLocationCode":"A073","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA074","Variety":"中码成人病人长裤","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":600,"Unit":"条","Description":"BCA074_中码成人病人长裤_M_蓝色格子条纹_条","RFIDElectronicTag":"BCA074MA074600","Barcode":"BCA074MA074600","StorageArea":"布草仓库","WarehouseLocationCode":"A074","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA075","Variety":"小码成人病人长裤","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":500,"Unit":"条","Description":"BCA075_小码成人病人长裤_S_蓝色格子条纹_条","RFIDElectronicTag":"BCA075SA075500","Barcode":"BCA075SA075500","StorageArea":"布草仓库","WarehouseLocationCode":"A075","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA076","Variety":"加加大码骨科单开边绑带病人长袖衫","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA076_加加大码骨科单开边绑带病人长袖衫_XXL_蓝色格子条纹_件","RFIDElectronicTag":"BCA076XXLA07630","Barcode":"BCA076XXLA07630","StorageArea":"布草仓库","WarehouseLocationCode":"A076","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA077","Variety":"加大码骨科单开边绑带病人长袖衫","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA077_加大码骨科单开边绑带病人长袖衫_XL_蓝色格子条纹_件","RFIDElectronicTag":"BCA077XLA07740","Barcode":"BCA077XLA07740","StorageArea":"布草仓库","WarehouseLocationCode":"A077","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA078","Variety":"大码骨科单开边绑带病人长袖衫","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"件","Description":"BCA078_大码骨科单开边绑带病人长袖衫_L_蓝色格子条纹_件","RFIDElectronicTag":"BCA078LA07860","Barcode":"BCA078LA07860","StorageArea":"布草仓库","WarehouseLocationCode":"A078","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA079","Variety":"中码骨科单开边绑带病人长袖衫","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA079_中码骨科单开边绑带病人长袖衫_M_蓝色格子条纹_件","RFIDElectronicTag":"BCA079MA07940","Barcode":"BCA079MA07940","StorageArea":"布草仓库","WarehouseLocationCode":"A079","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA080","Variety":"小码骨科单开边绑带病人长袖衫","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA080_小码骨科单开边绑带病人长袖衫_S_蓝色格子条纹_件","RFIDElectronicTag":"BCA080SA08030","Barcode":"BCA080SA08030","StorageArea":"布草仓库","WarehouseLocationCode":"A080","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA081","Variety":"加加大码骨科单开边绑带病人长裤","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA081_加加大码骨科单开边绑带病人长裤_XXL_蓝色格子条纹_条","RFIDElectronicTag":"BCA081XXLA08130","Barcode":"BCA081XXLA08130","StorageArea":"布草仓库","WarehouseLocationCode":"A081","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA082","Variety":"加大码骨科单开边绑带病人长裤","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA082_加大码骨科单开边绑带病人长裤_XL_蓝色格子条纹_条","RFIDElectronicTag":"BCA082XLA08240","Barcode":"BCA082XLA08240","StorageArea":"布草仓库","WarehouseLocationCode":"A082","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA083","Variety":"大码骨科单开边绑带病人长裤","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"条","Description":"BCA083_大码骨科单开边绑带病人长裤_L_蓝色格子条纹_条","RFIDElectronicTag":"BCA083LA08360","Barcode":"BCA083LA08360","StorageArea":"布草仓库","WarehouseLocationCode":"A083","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA084","Variety":"中码骨科单开边绑带病人长裤","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA084_中码骨科单开边绑带病人长裤_M_蓝色格子条纹_条","RFIDElectronicTag":"BCA084MA08440","Barcode":"BCA084MA08440","StorageArea":"布草仓库","WarehouseLocationCode":"A084","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA085","Variety":"小码骨科单开边绑带病人长裤","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA085_小码骨科单开边绑带病人长裤_S_蓝色格子条纹_条","RFIDElectronicTag":"BCA085SA08530","Barcode":"BCA085SA08530","StorageArea":"布草仓库","WarehouseLocationCode":"A085","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA086","Variety":"加加大码骨科双开边绑带病人长袖衫","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA086_加加大码骨科双开边绑带病人长袖衫_XXL_蓝色格子条纹_件","RFIDElectronicTag":"BCA086XXLA08630","Barcode":"BCA086XXLA08630","StorageArea":"布草仓库","WarehouseLocationCode":"A086","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA087","Variety":"加大码骨科双开边绑带病人长袖衫","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA087_加大码骨科双开边绑带病人长袖衫_XL_蓝色格子条纹_件","RFIDElectronicTag":"BCA087XLA08740","Barcode":"BCA087XLA08740","StorageArea":"布草仓库","WarehouseLocationCode":"A087","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA088","Variety":"大码骨科双开边绑带病人长袖衫","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"件","Description":"BCA088_大码骨科双开边绑带病人长袖衫_L_蓝色格子条纹_件","RFIDElectronicTag":"BCA088LA08860","Barcode":"BCA088LA08860","StorageArea":"布草仓库","WarehouseLocationCode":"A088","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA089","Variety":"中码骨科双开边绑带病人长袖衫","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA089_中码骨科双开边绑带病人长袖衫_M_蓝色格子条纹_件","RFIDElectronicTag":"BCA089MA08940","Barcode":"BCA089MA08940","StorageArea":"布草仓库","WarehouseLocationCode":"A089","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA090","Variety":"小码骨科双开边绑带病人长袖衫","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA090_小码骨科双开边绑带病人长袖衫_S_蓝色格子条纹_件","RFIDElectronicTag":"BCA090SA09030","Barcode":"BCA090SA09030","StorageArea":"布草仓库","WarehouseLocationCode":"A090","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA091","Variety":"加加大码骨科双开边绑带病人长裤","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA091_加加大码骨科双开边绑带病人长裤_XXL_蓝色格子条纹_条","RFIDElectronicTag":"BCA091XXLA09130","Barcode":"BCA091XXLA09130","StorageArea":"布草仓库","WarehouseLocationCode":"A091","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA092","Variety":"加大码骨科双开边绑带病人长裤","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA092_加大码骨科双开边绑带病人长裤_XL_蓝色格子条纹_条","RFIDElectronicTag":"BCA092XLA09240","Barcode":"BCA092XLA09240","StorageArea":"布草仓库","WarehouseLocationCode":"A092","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA093","Variety":"大码骨科双开边绑带病人长裤","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"条","Description":"BCA093_大码骨科双开边绑带病人长裤_L_蓝色格子条纹_条","RFIDElectronicTag":"BCA093LA09360","Barcode":"BCA093LA09360","StorageArea":"布草仓库","WarehouseLocationCode":"A093","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA094","Variety":"中码骨科双开边绑带病人长裤","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA094_中码骨科双开边绑带病人长裤_M_蓝色格子条纹_条","RFIDElectronicTag":"BCA094MA09440","Barcode":"BCA094MA09440","StorageArea":"布草仓库","WarehouseLocationCode":"A094","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA095","Variety":"小码骨科双开边绑带病人长裤","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA095_小码骨科双开边绑带病人长裤_S_蓝色格子条纹_条","RFIDElectronicTag":"BCA095SA09530","Barcode":"BCA095SA09530","StorageArea":"布草仓库","WarehouseLocationCode":"A095","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA096","Variety":"加加大码孕产妇病人长袖衫","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA096_加加大码孕产妇病人长袖衫_XXL_蓝色格子条纹_件","RFIDElectronicTag":"BCA096XXLA09640","Barcode":"BCA096XXLA09640","StorageArea":"布草仓库","WarehouseLocationCode":"A096","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA097","Variety":"加大码孕产妇病人长袖衫","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"件","Description":"BCA097_加大码孕产妇病人长袖衫_XL_蓝色格子条纹_件","RFIDElectronicTag":"BCA097XLA09760","Barcode":"BCA097XLA09760","StorageArea":"布草仓库","WarehouseLocationCode":"A097","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA098","Variety":"大码孕产妇病人长袖衫","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":100,"Unit":"件","Description":"BCA098_大码孕产妇病人长袖衫_L_蓝色格子条纹_件","RFIDElectronicTag":"BCA098LA098100","Barcode":"BCA098LA098100","StorageArea":"布草仓库","WarehouseLocationCode":"A098","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA099","Variety":"中码孕产妇病人长袖衫","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"件","Description":"BCA099_中码孕产妇病人长袖衫_M_蓝色格子条纹_件","RFIDElectronicTag":"BCA099MA09960","Barcode":"BCA099MA09960","StorageArea":"布草仓库","WarehouseLocationCode":"A099","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA100","Variety":"小码孕产妇病人长袖衫","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA100_小码孕产妇病人长袖衫_S_蓝色格子条纹_件","RFIDElectronicTag":"BCA100SA10040","Barcode":"BCA100SA10040","StorageArea":"布草仓库","WarehouseLocationCode":"A100","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA101","Variety":"加加大码孕产妇病人长裤","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA101_加加大码孕产妇病人长裤_XXL_蓝色格子条纹_条","RFIDElectronicTag":"BCA101XXLA10140","Barcode":"BCA101XXLA10140","StorageArea":"布草仓库","WarehouseLocationCode":"A101","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA102","Variety":"加大码孕产妇病人长裤","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"条","Description":"BCA102_加大码孕产妇病人长裤_XL_蓝色格子条纹_条","RFIDElectronicTag":"BCA102XLA10260","Barcode":"BCA102XLA10260","StorageArea":"布草仓库","WarehouseLocationCode":"A102","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA103","Variety":"大码孕产妇病人长裤","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":100,"Unit":"条","Description":"BCA103_大码孕产妇病人长裤_L_蓝色格子条纹_条","RFIDElectronicTag":"BCA103LA103100","Barcode":"BCA103LA103100","StorageArea":"布草仓库","WarehouseLocationCode":"A103","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA104","Variety":"中码孕产妇病人长裤","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"条","Description":"BCA104_中码孕产妇病人长裤_M_蓝色格子条纹_条","RFIDElectronicTag":"BCA104MA10460","Barcode":"BCA104MA10460","StorageArea":"布草仓库","WarehouseLocationCode":"A104","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA105","Variety":"小码孕产妇病人长裤","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA105_小码孕产妇病人长裤_S_蓝色格子条纹_条","RFIDElectronicTag":"BCA105SA10540","Barcode":"BCA105SA10540","StorageArea":"布草仓库","WarehouseLocationCode":"A105","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA106","Variety":"加加大码特诊成人病人长袖衫","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA106_加加大码特诊成人病人长袖衫_XXL_蓝色格子条纹_件","RFIDElectronicTag":"BCA106XXLA10630","Barcode":"BCA106XXLA10630","StorageArea":"布草仓库","WarehouseLocationCode":"A106","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA107","Variety":"加大码特诊成人病人长袖衫","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA107_加大码特诊成人病人长袖衫_XL_蓝色格子条纹_件","RFIDElectronicTag":"BCA107XLA10740","Barcode":"BCA107XLA10740","StorageArea":"布草仓库","WarehouseLocationCode":"A107","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA108","Variety":"大码特诊成人病人长袖衫","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"件","Description":"BCA108_大码特诊成人病人长袖衫_L_蓝色格子条纹_件","RFIDElectronicTag":"BCA108LA10860","Barcode":"BCA108LA10860","StorageArea":"布草仓库","WarehouseLocationCode":"A108","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA109","Variety":"中码特诊成人病人长袖衫","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"件","Description":"BCA109_中码特诊成人病人长袖衫_M_蓝色格子条纹_件","RFIDElectronicTag":"BCA109MA10940","Barcode":"BCA109MA10940","StorageArea":"布草仓库","WarehouseLocationCode":"A109","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA110","Variety":"小码特诊成人病人长袖衫","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"件","Description":"BCA110_小码特诊成人病人长袖衫_S_蓝色格子条纹_件","RFIDElectronicTag":"BCA110SA11030","Barcode":"BCA110SA11030","StorageArea":"布草仓库","WarehouseLocationCode":"A110","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA111","Variety":"加加大码特诊成人病人长裤","Specification":"XXL","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA111_加加大码特诊成人病人长裤_XXL_蓝色格子条纹_条","RFIDElectronicTag":"BCA111XXLA11130","Barcode":"BCA111XXLA11130","StorageArea":"布草仓库","WarehouseLocationCode":"A111","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA112","Variety":"加大码特诊成人病人长裤","Specification":"XL","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA112_加大码特诊成人病人长裤_XL_蓝色格子条纹_条","RFIDElectronicTag":"BCA112XLA11240","Barcode":"BCA112XLA11240","StorageArea":"布草仓库","WarehouseLocationCode":"A112","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA113","Variety":"大码特诊成人病人长裤","Specification":"L","ColorPattern":"蓝色格子条纹","Quantity":60,"Unit":"条","Description":"BCA113_大码特诊成人病人长裤_L_蓝色格子条纹_条","RFIDElectronicTag":"BCA113LA11360","Barcode":"BCA113LA11360","StorageArea":"布草仓库","WarehouseLocationCode":"A113","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA114","Variety":"中码特诊成人病人长裤","Specification":"M","ColorPattern":"蓝色格子条纹","Quantity":40,"Unit":"条","Description":"BCA114_中码特诊成人病人长裤_M_蓝色格子条纹_条","RFIDElectronicTag":"BCA114MA11440","Barcode":"BCA114MA11440","StorageArea":"布草仓库","WarehouseLocationCode":"A114","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA115","Variety":"小码特诊成人病人长裤","Specification":"S","ColorPattern":"蓝色格子条纹","Quantity":30,"Unit":"条","Description":"BCA115_小码特诊成人病人长裤_S_蓝色格子条纹_条","RFIDElectronicTag":"BCA115SA11530","Barcode":"BCA115SA11530","StorageArea":"布草仓库","WarehouseLocationCode":"A115","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA116","Variety":"加大码儿科病人长袖衫(12-14岁)","Specification":"XL","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA116_加大码儿科病人长袖衫(12-14岁)_XL_花色_件","RFIDElectronicTag":"BCA116XLA11630","Barcode":"BCA116XLA11630","StorageArea":"布草仓库","WarehouseLocationCode":"A116","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA117","Variety":"大码儿科病人长袖衫(9-11岁)","Specification":"L","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA117_大码儿科病人长袖衫(9-11岁)_L_花色_件","RFIDElectronicTag":"BCA117LA11730","Barcode":"BCA117LA11730","StorageArea":"布草仓库","WarehouseLocationCode":"A117","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA118","Variety":"中码儿科病人长袖衫(6-8岁)","Specification":"M","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA118_中码儿科病人长袖衫(6-8岁)_M_花色_件","RFIDElectronicTag":"BCA118MA11830","Barcode":"BCA118MA11830","StorageArea":"布草仓库","WarehouseLocationCode":"A118","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA119","Variety":"小码儿科病人长袖衫(3-5岁)","Specification":"S","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA119_小码儿科病人长袖衫(3-5岁)_S_花色_件","RFIDElectronicTag":"BCA119SA11930","Barcode":"BCA119SA11930","StorageArea":"布草仓库","WarehouseLocationCode":"A119","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA120","Variety":"加小码儿科病人长袖衫(1-2岁)","Specification":"XS","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA120_加小码儿科病人长袖衫(1-2岁)_XS_花色_件","RFIDElectronicTag":"BCA120XSA12030","Barcode":"BCA120XSA12030","StorageArea":"布草仓库","WarehouseLocationCode":"A120","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA121","Variety":"大码儿科病人双袖开纽长袖服","Specification":"L","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA121_大码儿科病人双袖开纽长袖服_L_花色_件","RFIDElectronicTag":"BCA121LA12130","Barcode":"BCA121LA12130","StorageArea":"布草仓库","WarehouseLocationCode":"A121","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA122","Variety":"中码儿科病人双袖开纽长袖服","Specification":"M","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA122_中码儿科病人双袖开纽长袖服_M_花色_件","RFIDElectronicTag":"BCA122MA12230","Barcode":"BCA122MA12230","StorageArea":"布草仓库","WarehouseLocationCode":"A122","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA123","Variety":"小码儿科病人双袖开纽长袖服","Specification":"S","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA123_小码儿科病人双袖开纽长袖服_S_花色_件","RFIDElectronicTag":"BCA123SA12330","Barcode":"BCA123SA12330","StorageArea":"布草仓库","WarehouseLocationCode":"A123","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA124","Variety":"加大码儿科病人长裤(12-14岁)","Specification":"XL","ColorPattern":"花色","Quantity":30,"Unit":"条","Description":"BCA124_加大码儿科病人长裤(12-14岁)_XL_花色_条","RFIDElectronicTag":"BCA124XLA12430","Barcode":"BCA124XLA12430","StorageArea":"布草仓库","WarehouseLocationCode":"A124","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA125","Variety":"大码儿科病人长裤(9-11岁)","Specification":"L","ColorPattern":"花色","Quantity":30,"Unit":"条","Description":"BCA125_大码儿科病人长裤(9-11岁)_L_花色_条","RFIDElectronicTag":"BCA125LA12530","Barcode":"BCA125LA12530","StorageArea":"布草仓库","WarehouseLocationCode":"A125","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA126","Variety":"中码儿科病人长裤(6-8岁)","Specification":"M","ColorPattern":"花色","Quantity":30,"Unit":"条","Description":"BCA126_中码儿科病人长裤(6-8岁)_M_花色_条","RFIDElectronicTag":"BCA126MA12630","Barcode":"BCA126MA12630","StorageArea":"布草仓库","WarehouseLocationCode":"A126","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA127","Variety":"小码儿科病人长裤(3-5岁)","Specification":"S","ColorPattern":"花色","Quantity":30,"Unit":"条","Description":"BCA127_小码儿科病人长裤(3-5岁)_S_花色_条","RFIDElectronicTag":"BCA127SA12730","Barcode":"BCA127SA12730","StorageArea":"布草仓库","WarehouseLocationCode":"A127","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA128","Variety":"加小码儿科病人长裤(1-2岁)","Specification":"XS","ColorPattern":"花色","Quantity":30,"Unit":"条","Description":"BCA128_加小码儿科病人长裤(1-2岁)_XS_花色_条","RFIDElectronicTag":"BCA128XSA12830","Barcode":"BCA128XSA12830","StorageArea":"布草仓库","WarehouseLocationCode":"A128","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA129","Variety":"婴儿衫","Specification":"-","ColorPattern":"花色","Quantity":100,"Unit":"件","Description":"BCA129_婴儿衫_-_花色_件","RFIDElectronicTag":"BCA129-A129100","Barcode":"BCA129-A129100","StorageArea":"布草仓库","WarehouseLocationCode":"A129","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA130","Variety":"双袖开纽婴儿衫","Specification":"-","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA130_双袖开纽婴儿衫_-_花色_件","RFIDElectronicTag":"BCA130-A13030","Barcode":"BCA130-A13030","StorageArea":"布草仓库","WarehouseLocationCode":"A130","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA131","Variety":"病人睡袍","Specification":"-","ColorPattern":"蓝色格子条纹","Quantity":150,"Unit":"件","Description":"BCA131_病人睡袍_-_蓝色格子条纹_件","RFIDElectronicTag":"BCA131-A131150","Barcode":"BCA131-A131150","StorageArea":"布草仓库","WarehouseLocationCode":"A131","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA132","Variety":"婴儿衫","Specification":"-","ColorPattern":"花色","Quantity":200,"Unit":"件","Description":"BCA132_婴儿衫_-_花色_件","RFIDElectronicTag":"BCA132-A132200","Barcode":"BCA132-A132200","StorageArea":"布草仓库","WarehouseLocationCode":"A132","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA133","Variety":"双层婴儿夹棉袍","Specification":"-","ColorPattern":"花色","Quantity":200,"Unit":"件","Description":"BCA133_双层婴儿夹棉袍_-_花色_件","RFIDElectronicTag":"BCA133-A133200","Barcode":"BCA133-A133200","StorageArea":"布草仓库","WarehouseLocationCode":"A133","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA134","Variety":"双层婴儿背心","Specification":"-","ColorPattern":"花色","Quantity":30,"Unit":"件","Description":"BCA134_双层婴儿背心_-_花色_件","RFIDElectronicTag":"BCA134-A13430","Barcode":"BCA134-A13430","StorageArea":"布草仓库","WarehouseLocationCode":"A134","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA135","Variety":"婴儿服","Specification":"-","ColorPattern":"花色","Quantity":100,"Unit":"件","Description":"BCA135_婴儿服_-_花色_件","RFIDElectronicTag":"BCA135-A135100","Barcode":"BCA135-A135100","StorageArea":"布草仓库","WarehouseLocationCode":"A135","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA136","Variety":"婴儿和尚袍","Specification":"-","ColorPattern":"花色","Quantity":100,"Unit":"件","Description":"BCA136_婴儿和尚袍_-_花色_件","RFIDElectronicTag":"BCA136-A136100","Barcode":"BCA136-A136100","StorageArea":"布草仓库","WarehouseLocationCode":"A136","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA137","Variety":"夹棉背心","Specification":"-","ColorPattern":"白色","Quantity":50,"Unit":"件","Description":"BCA137_夹棉背心_-_白色_件","RFIDElectronicTag":"BCA137-A13750","Barcode":"BCA137-A13750","StorageArea":"布草仓库","WarehouseLocationCode":"A137","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA138","Variety":"婴儿被袋(长110*宽100cm双层)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA138_婴儿被袋(长110*宽100cm双层)_-_白色_张","RFIDElectronicTag":"BCA138-A138100","Barcode":"BCA138-A138100","StorageArea":"布草仓库","WarehouseLocationCode":"A138","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA139","Variety":"三层床单(长200*宽80cm)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA139_三层床单(长200*宽80cm)_-_白色_张","RFIDElectronicTag":"BCA139-A139100","Barcode":"BCA139-A139100","StorageArea":"布草仓库","WarehouseLocationCode":"A139","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA140","Variety":"婴儿被袋(长90*宽90cm双层)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA140_婴儿被袋(长90*宽90cm双层)_-_白色_张","RFIDElectronicTag":"BCA140-A140100","Barcode":"BCA140-A140100","StorageArea":"布草仓库","WarehouseLocationCode":"A140","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA141","Variety":"婴儿床罩(长70*宽45*高35cm)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA141_婴儿床罩(长70*宽45*高35cm)_-_白色_张","RFIDElectronicTag":"BCA141-A141100","Barcode":"BCA141-A141100","StorageArea":"布草仓库","WarehouseLocationCode":"A141","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA142","Variety":"婴儿床单(长90*宽60cm单层)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA142_婴儿床单(长90*宽60cm单层)_-_白色_张","RFIDElectronicTag":"BCA142-A142100","Barcode":"BCA142-A142100","StorageArea":"布草仓库","WarehouseLocationCode":"A142","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA143","Variety":"婴儿枕袋(长40*25cm双层)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA143_婴儿枕袋(长40*25cm双层)_-_白色_张","RFIDElectronicTag":"BCA143-A143100","Barcode":"BCA143-A143100","StorageArea":"布草仓库","WarehouseLocationCode":"A143","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA144","Variety":"妇检床床套(长95*宽55cm))","Specification":"-","ColorPattern":"白色","Quantity":20,"Unit":"张","Description":"BCA144_妇检床床套(长95*宽55cm))_-_白色_张","RFIDElectronicTag":"BCA144-A14420","Barcode":"BCA144-A14420","StorageArea":"布草仓库","WarehouseLocationCode":"A144","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA145","Variety":"床罩(200*86*25cm)","Specification":"-","ColorPattern":"白色","Quantity":200,"Unit":"张","Description":"BCA145_床罩(200*86*25cm)_-_白色_张","RFIDElectronicTag":"BCA145-A145200","Barcode":"BCA145-A145200","StorageArea":"布草仓库","WarehouseLocationCode":"A145","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA146","Variety":"内镜床罩(200*70*18cm)","Specification":"-","ColorPattern":"白色","Quantity":50,"Unit":"张","Description":"BCA146_内镜床罩(200*70*18cm)_-_白色_张","RFIDElectronicTag":"BCA146-A14650","Barcode":"BCA146-A14650","StorageArea":"布草仓库","WarehouseLocationCode":"A146","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA147","Variety":"中单(长165*宽125CM)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA147_中单(长165*宽125CM)_-_白色_张","RFIDElectronicTag":"BCA147-A147100","Barcode":"BCA147-A147100","StorageArea":"布草仓库","WarehouseLocationCode":"A147","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA148","Variety":"中单(240*90cm)","Specification":"-","ColorPattern":"白色","Quantity":100,"Unit":"张","Description":"BCA148_中单(240*90cm)_-_白色_张","RFIDElectronicTag":"BCA148-A148100","Barcode":"BCA148-A148100","StorageArea":"布草仓库","WarehouseLocationCode":"A148","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA149","Variety":"枕袋","Specification":"-","ColorPattern":"白色","Quantity":1500,"Unit":"张","Description":"BCA149_枕袋_-_白色_张","RFIDElectronicTag":"BCA149-A1491500","Barcode":"BCA149-A1491500","StorageArea":"布草仓库","WarehouseLocationCode":"A149","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA150","Variety":"被袋","Specification":"-","ColorPattern":"白色","Quantity":1500,"Unit":"张","Description":"BCA150_被袋_-_白色_张","RFIDElectronicTag":"BCA150-A1501500","Barcode":"BCA150-A1501500","StorageArea":"布草仓库","WarehouseLocationCode":"A150","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA151","Variety":"床单","Specification":"-","ColorPattern":"白色","Quantity":1500,"Unit":"张","Description":"BCA151_床单_-_白色_张","RFIDElectronicTag":"BCA151-A1511500","Barcode":"BCA151-A1511500","StorageArea":"布草仓库","WarehouseLocationCode":"A151","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA152","Variety":"捆红边枕袋","Specification":"-","ColorPattern":"白色","Quantity":300,"Unit":"张","Description":"BCA152_捆红边枕袋_-_白色_张","RFIDElectronicTag":"BCA152-A152300","Barcode":"BCA152-A152300","StorageArea":"布草仓库","WarehouseLocationCode":"A152","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA153","Variety":"捆红边床罩","Specification":"-","ColorPattern":"白色","Quantity":300,"Unit":"张","Description":"BCA153_捆红边床罩_-_白色_张","RFIDElectronicTag":"BCA153-A153300","Barcode":"BCA153-A153300","StorageArea":"布草仓库","WarehouseLocationCode":"A153","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA154","Variety":"重症三层床单","Specification":"-","ColorPattern":"白色","Quantity":300,"Unit":"张","Description":"BCA154_重症三层床单_-_白色_张","RFIDElectronicTag":"BCA154-A154300","Barcode":"BCA154-A154300","StorageArea":"布草仓库","WarehouseLocationCode":"A154","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA155","Variety":"工衣袋(宽110*高125cm)","Specification":"-","ColorPattern":"白色","Quantity":500,"Unit":"个","Description":"BCA155_工衣袋(宽110*高125cm)_-_白色_个","RFIDElectronicTag":"BCA155-A155500","Barcode":"BCA155-A155500","StorageArea":"布草仓库","WarehouseLocationCode":"A155","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA156","Variety":"腹带((长85*宽20cm))","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"条","Description":"BCA156_腹带((长85*宽20cm))_-_白色_条","RFIDElectronicTag":"BCA156-A15630","Barcode":"BCA156-A15630","StorageArea":"布草仓库","WarehouseLocationCode":"A156","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA157","Variety":"披肩((长70*宽60cm)","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"个","Description":"BCA157_披肩((长70*宽60cm)_-_白色_个","RFIDElectronicTag":"BCA157-A15730","Barcode":"BCA157-A15730","StorageArea":"布草仓库","WarehouseLocationCode":"A157","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA158","Variety":"冰袋套(长48*宽15cm)","Specification":"-","ColorPattern":"白色","Quantity":200,"Unit":"个","Description":"BCA158_冰袋套(长48*宽15cm)_-_白色_个","RFIDElectronicTag":"BCA158-A158200","Barcode":"BCA158-A158200","StorageArea":"布草仓库","WarehouseLocationCode":"A158","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA159","Variety":"袖套(长36*宽19cm)","Specification":"-","ColorPattern":"白色","Quantity":20,"Unit":"双","Description":"BCA159_袖套(长36*宽19cm)_-_白色_双","RFIDElectronicTag":"BCA159-A15920","Barcode":"BCA159-A15920","StorageArea":"布草仓库","WarehouseLocationCode":"A159","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA160","Variety":"婴儿手镯","Specification":"-","ColorPattern":"白色","Quantity":50,"Unit":"个","Description":"BCA160_婴儿手镯_-_白色_个","RFIDElectronicTag":"BCA160-A16050","Barcode":"BCA160-A16050","StorageArea":"布草仓库","WarehouseLocationCode":"A160","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA161","Variety":"湿化瓶套(长24*宽22cm)","Specification":"-","ColorPattern":"白色","Quantity":10,"Unit":"个","Description":"BCA161_湿化瓶套(长24*宽22cm)_-_白色_个","RFIDElectronicTag":"BCA161-A16110","Barcode":"BCA161-A16110","StorageArea":"布草仓库","WarehouseLocationCode":"A161","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA162","Variety":"氧气筒套(长128*宽41cm)","Specification":"-","ColorPattern":"白色","Quantity":10,"Unit":"个","Description":"BCA162_氧气筒套(长128*宽41cm)_-_白色_个","RFIDElectronicTag":"BCA162-A16210","Barcode":"BCA162-A16210","StorageArea":"布草仓库","WarehouseLocationCode":"A162","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA163","Variety":"多层氧气袋(长21*宽18cm四层))","Specification":"-","ColorPattern":"白色","Quantity":10,"Unit":"个","Description":"BCA163_多层氧气袋(长21*宽18cm四层))_-_白色_个","RFIDElectronicTag":"BCA163-A16310","Barcode":"BCA163-A16310","StorageArea":"布草仓库","WarehouseLocationCode":"A163","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA164","Variety":"口腔科椅套(长120cm*宽60cm)","Specification":"-","ColorPattern":"白色","Quantity":10,"Unit":"套","Description":"BCA164_口腔科椅套(长120cm*宽60cm)_-_白色_套","RFIDElectronicTag":"BCA164-A16410","Barcode":"BCA164-A16410","StorageArea":"布草仓库","WarehouseLocationCode":"A164","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA165","Variety":"耳鼻咽喉科椅套(长105*宽50cm)","Specification":"-","ColorPattern":"白色","Quantity":10,"Unit":"套","Description":"BCA165_耳鼻咽喉科椅套(长105*宽50cm)_-_白色_套","RFIDElectronicTag":"BCA165-A16510","Barcode":"BCA165-A16510","StorageArea":"布草仓库","WarehouseLocationCode":"A165","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA166","Variety":"可水洗枕芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重900g","Variety":"-","Specification":"白色","ColorPattern":1500,"Quantity":"个","Unit":"BCA166_可水洗枕芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重900g_-_白色_个","Variety":"BCA166-A1661500","Specification":"BCA166-A1661500","ColorPattern":"布草仓库","Quantity":"A166","Unit":"","Description":"","RFIDElectronicTag":"","Barcode":"","StorageArea":"","WarehouseLocationCode":"","AffiliatedHospital":"","Department":"","ServiceProvider":""},
{"ProductCode":"BCA167","Variety":"可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重1500g","Variety":"-","Specification":"白色","ColorPattern":1500,"Quantity":"张","Unit":"BCA167_可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重1500g_-_白色_张","Variety":"BCA167-A1671500","Specification":"BCA167-A1671500","ColorPattern":"布草仓库","Quantity":"A167","Unit":"","Description":"","RFIDElectronicTag":"","Barcode":"","StorageArea":"","WarehouseLocationCode":"","AffiliatedHospital":"","Department":"","ServiceProvider":""},
{"ProductCode":"BCA168","Variety":"可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重2000g","Variety":"-","Specification":"白色","ColorPattern":1500,"Quantity":"张","Unit":"BCA168_可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重2000g_-_白色_张","Variety":"BCA168-A1681500","Specification":"BCA168-A1681500","ColorPattern":"布草仓库","Quantity":"A168","Unit":"","Description":"","RFIDElectronicTag":"","Barcode":"","StorageArea":"","WarehouseLocationCode":"","AffiliatedHospital":"","Department":"","ServiceProvider":""},
{"ProductCode":"BCA169","Variety":"可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重2500g","Variety":"-","Specification":"白色","ColorPattern":1500,"Quantity":"张","Unit":"BCA169_可水洗被芯面料C40S*C40S/135*95/防羽布"},
{"ProductCode":"填充100%聚酯纤维(赛羽绒)克重2500g_-_白色_张","Variety":"BCA169-A1691500","Specification":"BCA169-A1691500","ColorPattern":"布草仓库","Quantity":"A169","Unit":"","Description":"","RFIDElectronicTag":"","Barcode":"","StorageArea":"","WarehouseLocationCode":"","AffiliatedHospital":"","Department":"","ServiceProvider":""},
{"ProductCode":"BCA170","Variety":"33*30cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA170_33*30cm双层包布_-_白色_张","RFIDElectronicTag":"BCA170-A17030","Barcode":"BCA170-A17030","StorageArea":"布草仓库","WarehouseLocationCode":"A170","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA171","Variety":"55*52cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA171_55*52cm双层包布_-_白色_张","RFIDElectronicTag":"BCA171-A17130","Barcode":"BCA171-A17130","StorageArea":"布草仓库","WarehouseLocationCode":"A171","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA172","Variety":"66*62cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA172_66*62cm双层包布_-_白色_张","RFIDElectronicTag":"BCA172-A17230","Barcode":"BCA172-A17230","StorageArea":"布草仓库","WarehouseLocationCode":"A172","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA173","Variety":"75*75cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA173_75*75cm双层包布_-_白色_张","RFIDElectronicTag":"BCA173-A17330","Barcode":"BCA173-A17330","StorageArea":"布草仓库","WarehouseLocationCode":"A173","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA174","Variety":"97*94cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA174_97*94cm双层包布_-_白色_张","RFIDElectronicTag":"BCA174-A17430","Barcode":"BCA174-A17430","StorageArea":"布草仓库","WarehouseLocationCode":"A174","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA175","Variety":"140*118cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA175_140*118cm双层包布_-_白色_张","RFIDElectronicTag":"BCA175-A17530","Barcode":"BCA175-A17530","StorageArea":"布草仓库","WarehouseLocationCode":"A175","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA176","Variety":"92*55cm单层托布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA176_92*55cm单层托布_-_白色_张","RFIDElectronicTag":"BCA176-A17630","Barcode":"BCA176-A17630","StorageArea":"布草仓库","WarehouseLocationCode":"A176","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA177","Variety":"70*45cm单层治疗巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA177_70*45cm单层治疗巾_-_白色_张","RFIDElectronicTag":"BCA177-A17730","Barcode":"BCA177-A17730","StorageArea":"布草仓库","WarehouseLocationCode":"A177","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA178","Variety":"90*80cm单层治疗巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA178_90*80cm单层治疗巾_-_白色_张","RFIDElectronicTag":"BCA178-A17830","Barcode":"BCA178-A17830","StorageArea":"布草仓库","WarehouseLocationCode":"A178","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA179","Variety":"70*45cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA179_70*45cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA179-A17930","Barcode":"BCA179-A17930","StorageArea":"布草仓库","WarehouseLocationCode":"A179","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA180","Variety":"97*94cm双层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA180_97*94cm双层孔巾_-_白色_张","RFIDElectronicTag":"BCA180-A18030","Barcode":"BCA180-A18030","StorageArea":"布草仓库","WarehouseLocationCode":"A180","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA181","Variety":"90*75cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA181_90*75cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA181-A18130","Barcode":"BCA181-A18130","StorageArea":"布草仓库","WarehouseLocationCode":"A181","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA182","Variety":"95*83cm双层治疗巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA182_95*83cm双层治疗巾_-_白色_张","RFIDElectronicTag":"BCA182-A18230","Barcode":"BCA182-A18230","StorageArea":"布草仓库","WarehouseLocationCode":"A182","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA183","Variety":"125*105cm双层治疗巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA183_125*105cm双层治疗巾_-_白色_张","RFIDElectronicTag":"BCA183-A18330","Barcode":"BCA183-A18330","StorageArea":"布草仓库","WarehouseLocationCode":"A183","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA184","Variety":"210*145cm双层夹单","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA184_210*145cm双层夹单_-_白色_张","RFIDElectronicTag":"BCA184-A18430","Barcode":"BCA184-A18430","StorageArea":"布草仓库","WarehouseLocationCode":"A184","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA185","Variety":"127*97cm双层夹单","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA185_127*97cm双层夹单_-_白色_张","RFIDElectronicTag":"BCA185-A18530","Barcode":"BCA185-A18530","StorageArea":"布草仓库","WarehouseLocationCode":"A185","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA186","Variety":"260*90cm单层中单","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA186_260*90cm单层中单_-_白色_张","RFIDElectronicTag":"BCA186-A18630","Barcode":"BCA186-A18630","StorageArea":"布草仓库","WarehouseLocationCode":"A186","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA187","Variety":"270*95cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA187_270*95cm双层包布_-_白色_张","RFIDElectronicTag":"BCA187-A18730","Barcode":"BCA187-A18730","StorageArea":"布草仓库","WarehouseLocationCode":"A187","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA188","Variety":"220*112cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA188_220*112cm双层包布_-_白色_张","RFIDElectronicTag":"BCA188-A18830","Barcode":"BCA188-A18830","StorageArea":"布草仓库","WarehouseLocationCode":"A188","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA189","Variety":"225*150cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA189_225*150cm双层包布_-_白色_张","RFIDElectronicTag":"BCA189-A18930","Barcode":"BCA189-A18930","StorageArea":"布草仓库","WarehouseLocationCode":"A189","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA190","Variety":"190*170cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA190_190*170cm双层包布_-_白色_张","RFIDElectronicTag":"BCA190-A19030","Barcode":"BCA190-A19030","StorageArea":"布草仓库","WarehouseLocationCode":"A190","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA191","Variety":"145*135cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA191_145*135cm双层包布_-_白色_张","RFIDElectronicTag":"BCA191-A19130","Barcode":"BCA191-A19130","StorageArea":"布草仓库","WarehouseLocationCode":"A191","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA192","Variety":"130*100双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA192_130*100双层包布_-_白色_张","RFIDElectronicTag":"BCA192-A19230","Barcode":"BCA192-A19230","StorageArea":"布草仓库","WarehouseLocationCode":"A192","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA193","Variety":"97*94cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA193_97*94cm双层包布_-_白色_张","RFIDElectronicTag":"BCA193-A19330","Barcode":"BCA193-A19330","StorageArea":"布草仓库","WarehouseLocationCode":"A193","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA194","Variety":"55*52cm双层包布","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA194_55*52cm双层包布_-_白色_张","RFIDElectronicTag":"BCA194-A19430","Barcode":"BCA194-A19430","StorageArea":"布草仓库","WarehouseLocationCode":"A194","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA195","Variety":"350*225cm双层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA195_350*225cm双层孔巾_-_白色_张","RFIDElectronicTag":"BCA195-A19530","Barcode":"BCA195-A19530","StorageArea":"布草仓库","WarehouseLocationCode":"A195","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA196","Variety":"305*220cm双层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA196_305*220cm双层孔巾_-_白色_张","RFIDElectronicTag":"BCA196-A19630","Barcode":"BCA196-A19630","StorageArea":"布草仓库","WarehouseLocationCode":"A196","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA197","Variety":"148*95cm双层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA197_148*95cm双层孔巾_-_白色_张","RFIDElectronicTag":"BCA197-A19730","Barcode":"BCA197-A19730","StorageArea":"布草仓库","WarehouseLocationCode":"A197","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA198","Variety":"220*210cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA198_220*210cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA198-A19830","Barcode":"BCA198-A19830","StorageArea":"布草仓库","WarehouseLocationCode":"A198","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA199","Variety":"230*130cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA199_230*130cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA199-A19930","Barcode":"BCA199-A19930","StorageArea":"布草仓库","WarehouseLocationCode":"A199","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA200","Variety":"97*74cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA200_97*74cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA200-A20030","Barcode":"BCA200-A20030","StorageArea":"布草仓库","WarehouseLocationCode":"A200","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA201","Variety":"110*77cm单层治疗巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA201_110*77cm单层治疗巾_-_白色_张","RFIDElectronicTag":"BCA201-A20130","Barcode":"BCA201-A20130","StorageArea":"布草仓库","WarehouseLocationCode":"A201","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA202","Variety":"200*125cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA202_200*125cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA202-A20230","Barcode":"BCA202-A20230","StorageArea":"布草仓库","WarehouseLocationCode":"A202","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA203","Variety":"165*90cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA203_165*90cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA203-A20330","Barcode":"BCA203-A20330","StorageArea":"布草仓库","WarehouseLocationCode":"A203","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA204","Variety":"110*97cm单层孔巾","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA204_110*97cm单层孔巾_-_白色_张","RFIDElectronicTag":"BCA204-A20430","Barcode":"BCA204-A20430","StorageArea":"布草仓库","WarehouseLocationCode":"A204","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA205","Variety":"110*16cm布套","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA205_110*16cm布套_-_白色_张","RFIDElectronicTag":"BCA205-A20530","Barcode":"BCA205-A20530","StorageArea":"布草仓库","WarehouseLocationCode":"A205","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
{"ProductCode":"BCA206","Variety":"53*25cm袖套","Specification":"-","ColorPattern":"白色","Quantity":30,"Unit":"张","Description":"BCA206_53*25cm袖套_-_白色_张","RFIDElectronicTag":"BCA206-A20630","Barcode":"BCA206-A20630","StorageArea":"布草仓库","WarehouseLocationCode":"A206","AffiliatedHospital":"","Department":"","ServiceProvider":"","BoundPersonnel":"","CurrentOperationDocument":"","CreationTime":"","Status":"","ModificationTime":"","Operation":""},
]
let tempBucaoData = []
let uidnum = 0
bucaoData.map((item)=>{
let len = item.Quantity
const epc = item.RFIDElectronicTag
for(let i = 0;i<len;i++){
let tempItem = {...item}
tempItem.Uid = uidnum
uidnum++
tempItem.Quantity = 1
let count = Math.floor(Math.random()*210-1)+1
//Math.floor(Math.random() * 100) + 1;
//new Date().getTime() % 100) + 1
tempItem.Count = count
tempItem.Status = count>200?'报废':'在库' //在库 1 使用 2 送洗 3 报废 4
tempItem.RFIDElectronicTag = epc+i
tempItem.Barcode = tempItem.RFIDElectronicTag
tempItem.AffiliatedHospital = '本院'
const now = new Date()
// 输出格式如: "2023-03-15 12:30:45"
tempItem.CreationTime = formatDate(now)
tempItem.ModificationTime = formatDate(now)
if(i==0){
tempItem.image = "./工作服-男.png"
}else if(i==1){
tempItem.image = "./工作服-女.png"
}else if(i%2){
tempItem.image = "./工作服-分.png"
}else if(i%3){
tempItem.image = "./工作服-内.png"
}else if(i%5){
tempItem.image = "./手术服.png"
}else if(i%7){
tempItem.image = "./病服.png"
}else if(i%11){
tempItem.image = "./病服1.png"
}else if(i%13){
tempItem.image = "./病服2.png"
}else if(i%17){
tempItem.image = "./病服3.png"
}else{
tempItem.image = "./病床.png"
}
tempBucaoData.push(tempItem)
tempItem = null
}
})
//console.log(JSON.stringify(tempBucaoData))
let bucaoColumns=[
{
//rowspan: 2, //合并两行
align: "center",
halign: "center",
valign: "middle",
checkbox: true
},
{
field: 'index',
title: '序号',
align: "center",
halign: "center",
valign: "middle",
sortable: true,
//formatter: uidHandle,//自定义方法设置uid跳转链接
formatter: function (value, row, index) {
return index+1;
},
//rowspan: 2, //合并两行
width: 30
}
]
//console.log(JSON.stringify(tempBucaoData))
let cols = Object.keys(tempBucaoData[0])
cols.map((item)=>{
if(item == 'Operation'){
bucaoColumns.push({
field: item,
title: '操作',
align: "center",
halign: "center",
valign: "middle",
//rowspan: 2, //合并两行
//width: 400,
formatter: operateFormatter //自定义方法,添加操作按钮
})
}else if(item != 'Department'&&
item != 'ServiceProvider'&&
item != 'BoundPersonnel'&&
item != 'CurrentOperationDocument'&&
item != 'Description' &&
item != 'Barcode' &&
item != 'ModificationTime' &&
item != 'CreationTime' && item!="image"){
let tempData = {
field: item,
title: item=='ProductCode'?"产品码":
item=='Variety'?"品种":
item=='Specification'?"规格":
item=='ColorPattern'?"颜色花色":
item=='Quantity'?"数量":
item=='Unit'?"单位":
item=='Description'?"描述":
item=='RFIDElectronicTag'?"RFID电子标签码":
item=='Barcode'?"条码":
item=='StorageArea'?"存储区域":
item=='WarehouseLocationCode'?"库位编码":
item=='AffiliatedHospital'?"所属医院":
item=='Status'?"状态":
item=='ModificationTime'?"修改时间":
item=='Count'?"洗涤次数":
item,
align: "center",
halign: "center",
valign: "middle",
sortable: true,
//rowspan: 2, //合并两行
width: "300px",
editable: true,
//formatter: operateFormatter //自定义方法,添加操作按钮
}
if(item=='Status'){
tempData = {
field: item,
title: "状态",
align: "center",
halign: "center",
valign: "middle",
sortable: true,
//rowspan: 2, //合并两行
width: "300px",
editable: true,
formatter: operateFormatterStatus //自定义方法,添加操作按钮
}
}
bucaoColumns.push(tempData)
}else if(item=="image"){
bucaoColumns.push({
field: item,
title: '图片',
align: "center",
halign: "center",
valign: "middle",
sortable: true,
//rowspan: 2, //合并两行
width: "300px",
formatter: imageFormatter //自定义方法,添加操作按钮
})
}
})
var tableData = []
$(window).on('resize', function() {
$('#' + tableName).bootstrapTable('resetView');
});
function initTable(){
$('#' + tableName).empty();
$('#' + tableName).bootstrapTable('destroy').bootstrapTable({
//columns: columns, //动态拼接生成表格
//data: tableData,
columns: bucaoColumns,
data: tempBucaoData,
//data: [],
sortStable: true,
toolbar: "#toolbar", //一个jQuery 选择器,指明自定义的toolbar 例如:#toolbar, .toolbar.
uniqueId: "Uid", //每一行的唯一标识,一般为主键列
height: document.body.clientHeight-150, //动态获取高度值,可以使表格自适应页面
cache: true, // 表格缓存
striped: true, // 隔行加亮 行间隔色
queryParamsType: "limit", //设置为"undefined",可以获取pageNumber,pageSize,searchText,sortName,sortOrder //设置为"limit",符合 RESTFul 格式的参数,可以获取limit, offset, search, sort, order
queryParams: queryParams,
sidePagination: "client", //分页方式:client客户端分页,server服务端分页(*)
sortable: true, //是否启用排序;意味着整个表格都会排序
sortName: 'Uid', // 设置默认排序为 name
sortOrder: "asc", //排序方式
pagination: true, //是否显示分页(*)
search: true, //是否显示表格搜索,此搜索是客户端搜索,不会进服务端,所以,个人感觉意义不大
strictSearch: true,
showColumns: true, //是否显示所有的列
showRefresh: true, //是否显示刷新按钮
showToggle:true, //是否显示详细视图和列表视图
detailView: true, // 是否显示父子表
clickToSelect: true, //是否启用点击选中行
paginationLoop: true,
minimumCountColumns: 2, //最少允许的列数 clickToSelect: true, //是否启用点击选中行
pageNumber: 1, //初始化加载第一页,默认第一页
pageSize: 10, //每页的记录行数(*)
pageList: [10, 25, 50, 100], //可供选择的每页的行数(*)
paginationPreText: "上一页",
paginationNextText: "下一页",
paginationFirstText: "首页",
paginationLastText: "末页",
undefinedText: "...",
responseHandler: responseHandler,
showExport: true,
buttonsAlign: "right",//按钮位置
resizable: true,
exportDataType: 'all',//导出的数据类型,支持basic、all 、selected
exportOptions:{
ignoreColumn:[0], //导出数据忽略第一列
fileName:'报表', //导出数据的文件名
worksheetName:'sheet1',//表格工作区名称
tableName:'报表',
excelstyles:['background-color','color','font-size','font-weight']
},
rowStyle: rowStyle,
cellStyle:function(value,row,index){// value:单元格值,row:行对象,index:第几行
// 逻辑
return {css: {"background-color": 'rgb(11,22,33)'}};
},
onLoadSuccess: function (data) { //加载成功时执行
//console.log(data);
},
onLoadError: function (res) { //加载失败时执行
console.log(res);
},
onSort: function (a, b) { //点击排序时触发
$(".fixed-table-header").removeClass('hidden');
return a - b;
},
onExpandRow: function(index,row,$detail){
initSubTable(index,row,$detail)
},
editable: true, // 启用编辑功能
onEditableSave: function(field, row, oldValue, $el) {
// 保存编辑后的值
console.log('Edited:', field, row, oldValue, $el);
}
});
$(".fixed-table-header").addClass('hidden');
//初始化子表 无限循环
const initSubTable = function(index,row,$detail){
let parentId = row.id
let cur_table = $detail.html('<table></table>').find('table')
let len = row.Count
let tData = []
let currentDate = new Date(); // 获取当前日期
if(len != 0){
for(let i = 1;i<len+1;i++){
let previousDate = formatDate(new Date(currentDate.getTime() - (len-i)*2*24*60*60*1000)); // 将当前日期减去一天的毫秒数
let tCountObj = {
"mesh": i,
"translation": [
(-69.28118*i).toFixed(1),
(97.06617*i).toFixed(1),
(-31.3581028*i).toFixed(1)
],
"scale": [
1,
1,
1
],
"Description": i>100?"第"+i+"次清洗,超过洗涤次数上限,需要申请报废!":"第"+i+"次清洗",
"date": previousDate,
"LaundryFactory": "医用织物洗涤工厂",
"operate": "作业员",
"rotation": [
i,
-i,
(i/3).toFixed(1),
1
]
}
tData.push(tCountObj)
}
}
$(cur_table).bootstrapTable({
data: tData,
columns:[
{
field: 'Uid',
title: '编号',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
//formatter: uidHandle,//自定义方法设置uid跳转链接
formatter: function (value, row, index) {
return index+1;
},
width: 30
},
{
field: 'Description',
title: '描述',
width: 100
},
{
field: 'LaundryFactory',
title: '洗涤工厂',
width: 100
},
{
field: 'operate',
title: '操作员',
width: 100
},
{
field: 'date',
title: '修改日期',
width: 100
},
/*
{
field: 'translation',
title: 'translation',
width: 100
},
{
field: 'scale',
title: 'scale',
width: 100
},
{
field: 'rotation',
title: 'rotation',
width: 100
}*/
],
uniqueId: "Uid", //每一行的唯一标识,一般为主键列
//无限循环取子表,直到子表里面没有记录
onExpandRow: function(index,row,$detail){
initSubTable(index,row,$detail)
}
})
}
//执行分时任务
btnTask()
}
/***************************************
* 生成从minNum到maxNum的随机数。
* 如果指定decimalNum个数,则生成指定小数位数的随机数
* 如果不指定任何参数,则生成0-1之间的随机数。
*
* @minNum:[数据类型是Integer]生成的随机数的最小值(minNum和maxNum可以调换位置)
* @maxNum:[数据类型是Integer]生成的随机数的最大值
* @decimalNum:[数据类型是Integer]如果生成的是带有小数的随机数,则指定随机数的小数点后的位数
*
****************************************/
function randomNum(maxNum, minNum, decimalNum) {
var max = 0, min = 0;
minNum <= maxNum ? (min = minNum, max = maxNum) : (min = maxNum, max = minNum);
switch (arguments.length) {
case 1:
return Math.floor(Math.random() * (max + 1));
break;
case 2:
return Math.floor(Math.random() * (max - min + 1) + min);
break;
case 3:
return (Math.random() * (max - min) + min).toFixed(decimalNum);
break;
default:
return Math.random();
break;
}
}
function refresh(){
$('#' + tableName).bootstrapTable('refresh');
}
function DoOnMsoNumberFormat(cell, row, col) {
var result = "";
if (row > 0 && col == 0)
result = "\\@";
return result;
}
var columns=[]
function buildTableColumns() {
columns = []
var itemCode=[], //所选择因子的code
itemName=[]; // 所选择因子的text
$.each($(".itemArea li input[type='checkbox']"),function () {
if($(this).is(":checked")){
itemCode.push($(this).data("code"));
itemName.push($(this).parents("label").text());
}
});
var initColumns = []; //公共固定列
var itemColumns = []; //动态因子列
initColumns=[
{
rowspan: 2, //合并两行
align: 'center',
align: "center",
halign: "center",
valign: "middle",
checkbox: true
},
{
field: 'Uid',
title: '编号',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
//formatter: uidHandle,//自定义方法设置uid跳转链接
formatter: function (value, row, index) {
return index+1;
},
rowspan: 2, //合并两行
width: 30
}, {
field: 'time',
title: '时间',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
width: 200,
rowspan: 2, //合并两行
formatter: function (value, row, index) {//赋予的参数
return ['<div style="width: 200px;display: flex;justify-content: space-around;">'+value+'</div>'].join('');
},
sortable:false //本列不可以排序
}];
if(itemCode.length>0){
//根据选择因子绘制动态表头
for (var i = 0; i < itemCode.length; i++) {
switch(itemCode[i]){
case 'B01':
initColumns.push (
{
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 5
}
);
itemColumns.push({
field: 'value0',
title: '流量(m³/h)',
align: 'center'
}, {
field: 'value1',
title: '累计量(m³)',
align: 'center',
//sortable: true,
//clickToSelect: false,
sortName: "age",
order:"asc"
}, {
field: 'value2',
title: '浊度(Ntu)',
align: 'left',
halign:'center' //设置表头列居中对齐
}, {
field: 'value3',
title: 'pH',
align: 'center'
}, {
field: 'value4',
title: '温度(℃)',
align: 'center'
});
break;
case 'B02':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 10
});
itemColumns.push({
field: 'value5',
title: '库流量(m³/h)',
align: 'center'
}, {
field: 'value6',
title: '库累积量(m³)',
align: 'center'
}, {
field: 'value7',
title: '纸流量(m³/h)',
align: 'center'
}, {
field: 'value8',
title: '纸累积量(m³)',
align: 'center'
}, {
field: 'value9',
title: '横流量(m³/h)',
align: 'center'
}, {
field: 'value10',
title: '横累积量(m³)',
align: 'center'
}, {
field: 'value11',
title: '浊度(Ntu)',
align: 'center'
}, {
field: 'value12',
title: '余氯(mg/L)',
align: 'center'
}, {
field: 'value13',
title: 'pH',
align: 'center'
}, {
field: 'value14',
title: '温度(℃)',
align: 'center'
});
break;
case 'B03':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 2
});
itemColumns.push({
field: 'value15',
title: '1#吸水井(m)',
align: 'center'
}, {
field: 'value16',
title: '2#吸水井(m)',
align: 'center'
});
break;
case 'B04':
initColumns.push ({
field: "",
title: itemName[i] ,
//width: 80,
align: "right",
halign: "center",
colspan: 3
});
itemColumns.push({
field: 'value17',
title: '大河(Bar)',
align: 'center'
}, {
field: 'value18',
title: '哈河(Bar)',
align: 'center'
}, {
field: 'value19',
title: '杭河(Bar)',
align: 'center'
});
break;
default:
break;
}
}
initColumns.push ({
field: 'operate',
title: '操作',
align: 'center',
align: "center",
halign: "center",
valign: "middle",
rowspan: 2, //合并两行
width: 200,
formatter: operateFormatter//自定义方法,添加操作按钮
});
}
columns.push(initColumns, itemColumns); //合并表格内容
}
$(function(){
var hash = window.location.hash;
hash && $('ul.nav a[href="'+hash+'"]').tab('show');
$("#navList a").click(function(e){
e.preventDefault()
$(this).tab('show');
});
var nua = navigator.userAgent
var isAndroid = (nua.indexOf('Mozilla/5.0') > -1 && nua.indexOf('Android ') > -1 && nua.indexOf('AppleWebKit') > -1 && nua.indexOf('Chrome') === -1)
if (isAndroid) {
$('select.form-control').removeClass('form-control').css('width', '100%')
}
init()
});
// Example starter JavaScript for disabling form submissions if there are invalid fields
$(() => {
'use strict'
// Fetch all the forms we want to apply custom Bootstrap validation styles to
const forms = document.querySelectorAll('.needs-validation')
// Loop over them and prevent submission
Array.from(forms).forEach(form => {
form.addEventListener('submit', event => {
if (!form.checkValidity()) {
event.preventDefault()
event.stopPropagation()
}
form.classList.add('was-validated')
}, false)
})
});
function dateFormat(viewMode){
let format= ''
switch(viewMode){
case 'days':
format = 'yyyy-mm-dd'
break;
case 'months':
format = 'yyyy-mm'
break;
case 'years':
format = 'yyyy'
break;
default:
break;
}
return format
}
function dateFormatCap(viewMode){
let format= ''
switch(viewMode){
case 'days':
format = 'YYYY-MM-DD'
break;
case 'months':
format = 'YYYY-MM'
break;
case 'years':
format = 'YYYY'
break;
default:
break;
}
return format
}
function addDate(viewMode='days',num){
let temp = today
today = moment(temp).add(parseInt(num), viewMode).format(dateFormatCap(viewMode))
$("#startDate").datepicker("destroy");
initDate(viewMode)
getData(viewModeTable(viewMode))
}
function subtractDate(viewMode='days',num){
let temp = today
today = moment(temp).subtract(parseInt(num), viewMode).format(dateFormatCap(viewMode))
$("#startDate").datepicker("destroy");
initDate(viewMode)
getData(viewModeTable(viewMode))
}
function selectDateBtn(viewMode='days'){
let str = ''
if(viewMode=='days'){
str = '日'
}else if(viewMode=='months'){
str = '月'
}else if(viewMode=='years'){
str = '年'
}
let htmlDiv = '<button class="btn btn-default" onclick='+'subtractDate("'+viewMode+'",1)'+' style="height:40px;">上一'+str+'</button>'+
'<button class="btn btn-default" onclick='+'addDate("'+viewMode+'",1)'+' style="height:40px;">下一'+str+'</button>'
$('#date-operate').html(htmlDiv)
$("#startDate").datepicker("destroy");
initDate(viewMode)
}
let today = new Date();
function initDate(viewMode='days'){
//'days' 'months' 'years'
//初始化日期
var ops = {
todayHighlight:true, //设置当天日期高亮
language: 'zh-CN', //语言
autoclose: false, //选择后自动关闭
clearBtn: true,//清除按钮
format: dateFormat(viewMode),//日期格式
todayBtn: true,//设置今天按钮显示
minViewMode: viewMode,
maxViewMode: viewMode
};
$("#startDate").datepicker(ops);
$("#startDate").datepicker("update", today);
}
function init(){
initDate();
// 获取动态因子数据
getItemData();
// 监听因子点击事件
$(".itemArea li label").click( ()=> {
getItemData();
});
}
function getItemData() {
getData('day');
}
function add(){
var mockDatas=[];
let liuliang = randomNum(0,1000)
let leijiliuliang = randomNum(100000,1000000)
for(let i = 0;i< 1;i++){
let temp = {
uid: i,
time: new Date().toLocaleDateString('en-GB'),
value0: ((liuliang+i)*Math.random()).toFixed(1),
value1: leijiliuliang+i,
value2: randomNum(0,10,2),
value3: randomNum(6.5,8.5,2),
value4: randomNum(20,30,2),
value5: ((liuliang+i)*Math.random()).toFixed(1),
value6: leijiliuliang+i,
value7: ((liuliang+i)*Math.random()).toFixed(1),
value8: leijiliuliang+i,
value9: ((liuliang+i)*Math.random()).toFixed(1),
value10: leijiliuliang+i,
value11: randomNum(0,1,2),
value12: randomNum(1,1,2),
value13: randomNum(6.5,8.5,2),
value14: randomNum(20,30,2),
value15: randomNum(3,5,2),
value16: randomNum(3,5,2),
value17: randomNum(0,1,2),
value18: randomNum(0,1,2),
value19: randomNum(0,1,2),
value20: randomNum(1,100),
value21: randomNum(1,100),
}
mockDatas.push(temp)
}
// 添加记录
//$('#btn_add').click(function() {
// 使用bootstrap-table的编辑器API
//$('#' + tableName).bootstrapTable('append', mockDatas[0] );
$('#' + tableName).bootstrapTable('prepend', mockDatas[0]);
//});
}
let selectedRows = null
function edit(){
// 编辑记录
//$('#btn_edit').click(function() {
selectedRows = $('#' + tableName).bootstrapTable('getSelections');
if (selectedRows.length == 0) {
alert('Please select one row to edit!');
return;
}
if ((selectedRows.length >= 2)) {
alert("只能选择一条记录!");
return;
} else {
// 启用编辑模式
// 使用jQuery来打开模态框
$('#myModal').modal('show');
// 假设表格配置了uniqueId属性,且每行数据有一个唯一的id字段
let uniqueId = 'Uid'; // 这应该与表格配置的uniqueId属性相匹配
let rowIndex = ''
$.each(selectedRows, function(index, row) {
// 使用uniqueId找到行索引
rowIndex = row[uniqueId];
let htmlDoc = ''
Object.keys(row).map((key)=>{
if(!['0','_position'].includes(key)){
htmlDoc += `<div class="col-sm-6"><div class="form-group"><label class="col-sm-3 control-label">${key}:</label>
<div class="col-sm-8"><input class="form-control" type="text" name='${key}' id='${key}' value='${row[key]}' >
</div></div></div>`
}
})
document.querySelector('#editerModal').innerHTML = htmlDoc
});
//$('#' + tableName).bootstrapTable('updateRow', {
// index: rowIndex, // 行索引
// row: tempBucaoData[rowIndex+1] // 新的行数据
//});
}
//});
}
function saveEdit(){
// 假设表格配置了uniqueId属性,且每行数据有一个唯一的id字段
let uniqueId = 'Uid'; // 这应该与表格配置的uniqueId属性相匹配
let rowIndex = ''
let newData = {}
$.each(selectedRows, function(index, row) {
// 使用uniqueId找到行索引
rowIndex = row[uniqueId];
newData = row;
Object.keys(row).map((key)=>{
if(!['0','_position'].includes(key)){
// 获取id为"myInputId"的input元素
let inputElement = document.getElementById(key);
// 获取这个input元素的值
let inputValue = inputElement.value;
newData[key] = inputValue;
}
})
});
$('#' + tableName).bootstrapTable('updateRow', {
index: rowIndex, // 行索引
row: newData // 新的行数据
});
// 使用jQuery来关闭模态框
$('#myModal').modal('hide');
document.querySelector('#editerModal').innerHTML = ''
selectedRows = null
}
function changeLinenStatus(index,data){
let temp = tempBucaoData[index]
temp.Status = data
$('#' + tableName).bootstrapTable('updateRow', {
index: index, // 行索引
row: temp // 新的行数据
});
}
function remove(){
// 删除记录
//$('#btn_delete').click(function() {
let selectedRows = $('#' + tableName).bootstrapTable('getSelections');
if (selectedRows.length == 0) {
alert('Please select one row to edit!');
return;
} else if ((selectedRows.length >= 2)) {
alert("只能选择一条记录进行删除操作!");
return;
} else {
// 启用编辑模式
// 假设表格配置了uniqueId属性,且每行数据有一个唯一的id字段
let uniqueId = 'Uid'; // 这应该与表格配置的uniqueId属性相匹配
let rowIndex = ''
$.each(selectedRows, function(index, row) {
// 使用uniqueId找到行索引
rowIndex = row[uniqueId];
});
$('#' + tableName).bootstrapTable('remove', {
field: 'Uid',
values: [rowIndex]
});
// 删除所有行
//$('#' + tableName).bootstrapTable('removeAll');
}
//})
}
function removeBatch(){
let selectedRows = $('#' + tableName).bootstrapTable('getSelections');
if (selectedRows.length == 0) {
alert('Please select one row to edit!');
return;
} else {
// 启用编辑模式
// 假设表格配置了uniqueId属性,且每行数据有一个唯一的id字段
let uniqueId = 'Uid'; // 这应该与表格配置的uniqueId属性相匹配
let rowIndex = []
$.each(selectedRows, function(index, row) {
// 使用uniqueId找到行索引
rowIndex.push(row[uniqueId]);
});
$('#' + tableName).bootstrapTable('remove', {
field: 'Uid',
values: rowIndex
});
// 删除所有行
//$('#' + tableName).bootstrapTable('removeAll');
}
/*let tids;
let rows = $('#' + tableName).bootstrapTable('getSelections');
if (rows.length == 0) {// rows 主要是为了判断是否选中,下面的else内容才是主要
alert("请至少选择一条要删除的记录!");
return;
} else {
let arrays = new Array();// 声明一个数组
$(rows).each(function () {// 通过获得别选中的来进行遍历
arrays.push(this.uid);// uid为获得到的整条数据中的一列
});
tids = arrays.join(','); // 获得要删除的id
}*/
/*$.ajax({
type: "post",//方法类型
dataType: "json",//预期服务器返回的数据类型
url: ctx + 'system/madareanumberview/removeBatch?tids=' + tids,//url
success: function (result) {
if (result.success) {
alert("删除成功");
} else {
alert(result.message);
}
}
})*/
}
function submitFun(e){
console.log('提交表单')
$('#myModal').modal('hide')
startWorker()
}
function fullscreen() {
var element = document.getElementById('reportTab');
if (element.requestFullscreen) {
element.requestFullscreen();
} else if (element.mozRequestFullScreen) { /* Firefox */
element.mozRequestFullScreen();
} else if (element.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
element.webkitRequestFullscreen();
} else if (element.msRequestFullscreen) { /* IE/Edge */
element.msRequestFullscreen();
}
}
document.addEventListener('fullscreenchange', function() {
if (!document.fullscreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('mozfullscreenchange', function() {
if (!document.mozFullScreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('webkitfullscreenchange', function() {
if (!document.webkitFullscreenElement) {
// 退出全屏时的代码
}
}, false);
document.addEventListener('msfullscreenchange', function() {
if (!document.msFullscreenElement) {
// 退出全屏时的代码
}
}, false);
//高阶函数 分时执行 封装举例
//const btn = document.querySelector('#btnTask');
//生成 长度100000 数组
//sconst datas = new Array(100000).fill(0).map((_, i)=>i);
function btnTask() {
/*for(const i of datas){
const div = document.createElement('div');
div.innerText = i;
document.body.appendChild(div);
}*/
const taskHandler = (_, i)=>{
//const div = document.createElement('div');
//div.innerText = i;
//document.body.appendChild(div);
//console.log('执行分时任务',tempBucaoData[i]);
//在调用 load 方法之前,你可以保存当前表格的状态,例如当前页码、每页显示的行数、排序字段和排序顺序。
//let currentPage = $('#' + tableName).bootstrapTable('getOptions').pageNumber;
//let pageSize = $('#' + tableName).bootstrapTable('getOptions').pageSize;
//let sortName = $('#' + tableName).bootstrapTable('getOptions').sortName;
//let sortOrder = $('#' + tableName).bootstrapTable('getOptions').sortOrder;
//尾部插入新数据
//$('#' + tableName).bootstrapTable('append', tempBucaoData[i]);
//头部插入新数据
//$('#' + tableName).bootstrapTable('prepend', tempBucaoData[i]);
//$('#' + tableName).bootstrapTable('load', tempBucaoData[i]);
//$('#' + tableName).bootstrapTable('selectPage', currentPage);
//$('#' + tableName).bootstrapTable('refreshOptions', {
// pageSize: pageSize,
// sortName: sortName,
// sortOrder: sortOrder
//});
//如果你使用的是服务器端分页、排序和搜索,那么在调用 load 方法后,你可能需要重新发起请求到服务器,以获取正确的数据集,同时传递相应的分页、排序和搜索参数
//$('#' + tableName).bootstrapTable('load', newDataArray, { silent: true });
//设置 silent 选项为 true 在 load 方法中,这样它就不会触发 load 事件,但这不会保留状态,只是防止事件触发。
//$('#' + tableName).bootstrapTable('updateRow', {
// index: rowIndex, // 行索引
// row: newRowData // 新的行数据
//});
//$('#' + tableName).bootstrapTable('remove', {
// field: 'id', // 根据哪个字段来删除
// values: [value1, value2] // 要删除的值数组
//});
//使用 refresh 方法而不是 load 方法,它会刷新当前数据,而不是替换它们,从而可能保留状态。
//$('#' + tableName).bootstrapTable('refresh');
//为了更好地管理表格状态,你可能需要编写额外的逻辑来确保在数据更新后,用户界面的一致性和预期的行为。
};
const scheduler = (task)=>{
setTimeout(()=>{
const now = performance.now()
task(()=>performance.now()-now<=10)
},1000)
//requestIdleCallback((idle)=>{
// task(()=>idle.timeRemaining())
//})
}
//performChunk(datas, taskHandler, scheduler)
//通用函数,保证易用性和灵活性
//browserPerformChunk(datas, taskHandler)
browserPerformChunk(tempBucaoData, taskHandler)
}
function performChunk(datas, taskHandler, scheduler){
//参数归一化
if(typeof datas === 'number'){
datas = {
length: datas
}
}
let i = 0;
function _run(){
if(i>=datas.length){
return
}
//一个渲染帧中,空闲时开启分片执行
scheduler((goOn)=>{
while(goOn()&& i<datas.length){
taskHandler(datas[i],i)
i++;
}
//此次分片完成
_run();
})
/*requestIdleCallback(()=>{
while(idle.timeRemaining()>0&& i<datas.length){
taskHandler(datas[i],i)
i++;
}
//此次分片完成
_run();
})*/
}
_run()
}
function browserPerformChunk(datas, taskHandler){
const scheduler = (task)=>{
//requestAnimationFrame()
requestIdleCallback((idle)=>{
task(()=>idle.timeRemaining())
})
}
//performChunk(datas, taskHandler, scheduler)
performChunk(datas, taskHandler, scheduler)
}
</script>
</body>
</html>
参见: