环信单群聊 UIKit 是基于环信即时通讯云 IM SDK 开发的一款即时通讯 UI 组件库,提供各种组件实现会话列表、聊天界面、联系人列表及后续界面等功能,帮助开发者根据实际业务需求快速搭建包含 UI 界面的即时通讯应用。
本文教大家使用环信 uniapp UIKit 快速实现一个IM即时聊天应用
基本项目结构
└── ChatUIKit
├── assets // UIKit 资源文件
├── components // UIKit 通用组件
├── const // UIKit 常量
├── locales // UIKit 国际化
├── modules // UIKit 页面组件
│ ├── Chat // 聊天功能模块
│ ├── ChatNew // 发起新会话模块
│ ├── ContactAdd // 添加联系人模块
│ ├── ContactList // 联系人列表模块
│ ├── ContactRequestList // 联系人好友请求列表模块
│ ├── ContactSearchList // 联系人搜索列表模块
│ ├── Conversation // 会话列表模块
│ ├── ConversationSearchList // 会话搜索列表模块
│ ├── GroupCreate // 创建群组模块
│ ├── GroupList // 群组列表模块
│ ├── VideoPreview // 视频消息预览模块
├── store // UIKit store
│ ├── appUser.ts // UIKit用户属性store
│ ├── chat.ts // IM连接状态和事件处理
│ ├── config.ts // UIKit Config
│ ├── conn.ts // 管理 SDK 实例
│ ├── contact.ts // 联系人相关 store
│ ├── conversation.ts // 会话相关 store
│ ├── group.ts // 群组相关 store
│ ├── message.ts // 消息相关 store
├── styles // UIKit 通用样式
├── types // UIKit 类型定义
├── utils // UIKit 通用工具函数
├── configTypes.ts // UIKit 配置类型定义
├── index.ts // UIKit 入口文件
├── log.ts // UIKit 日志类
├── sdk.ts // UIKit IM SDK 类型
功能介绍
单群聊 UIKit 中业务相关的 UI 组件在 ChatUIKit/modules
目录下
单群聊 UIKit 主要功能:
聊天页面
ChatUIKit/modules/Chat
提供所有聊天视图的容器。
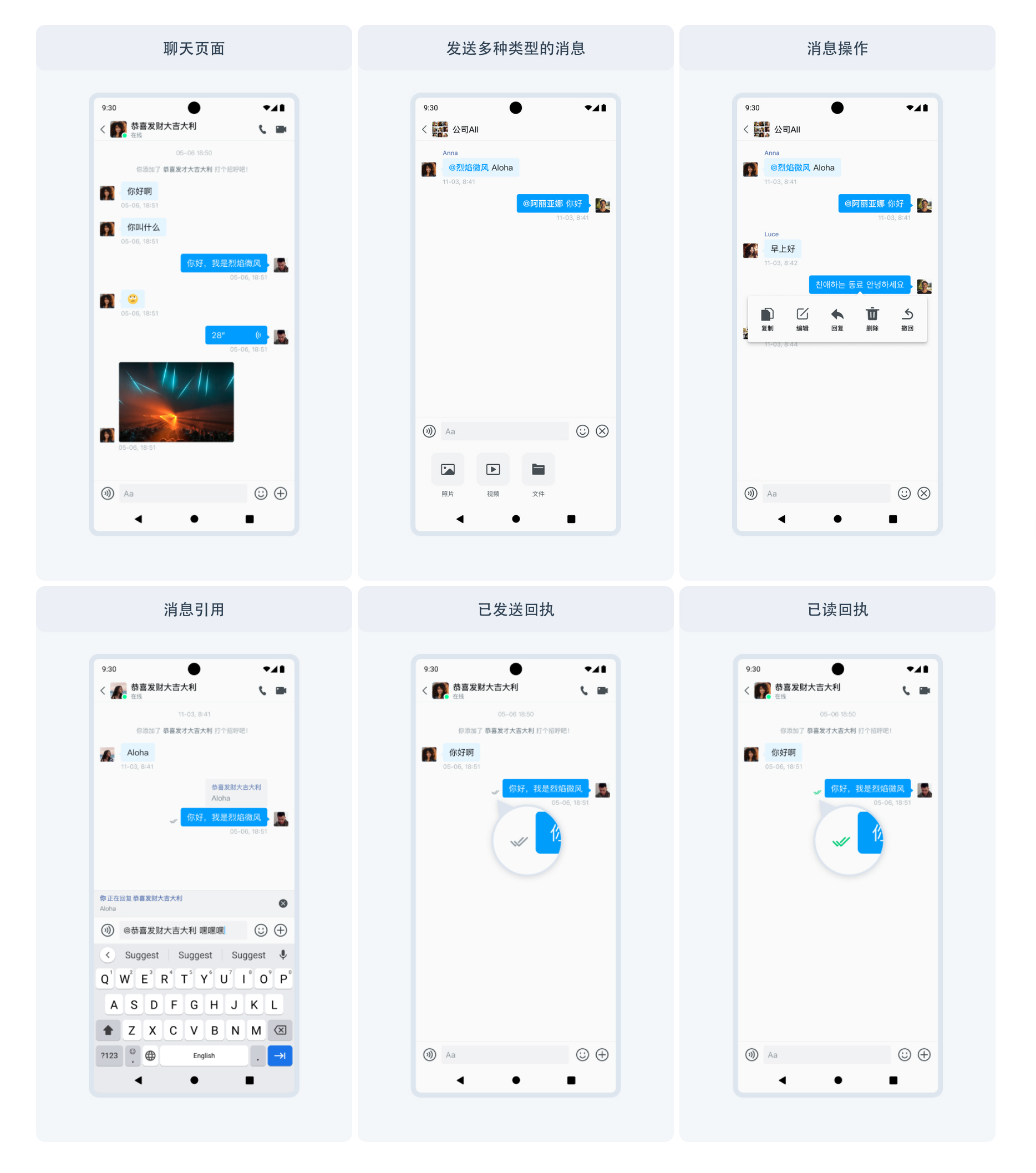
快速实现一个IM即时聊天
支持平台(vue3)
- Android
- iOS
- 微信小程序
- H5
前提条件
开始前,请确保你的开发环境满足以下条件:
- HBuilderX 最新版
- Vue3
- sass:sass-loader 10.1.1 及之前版本
- node:12.13.0 - 17.0.0,推荐 LTS 版本 16.17.0
- npm:版本请与 Node.js 版本匹配
项目集成
1、创建 uni-app Vue3 项目,详情参考 uni-app 项目创建。
2、下载 UIKit 源码
源码地址:https://github.com/easemob/easemob-uikit-uniapp
UIKit 中依赖的静态资源(
ChatUIKit/assets
)放置在环信服务器中,存在访问频率限制,建议你将静态资源放置在你的业务服务器上,然后修改ChatUIKit/const/index.ts
文件中的ASSETS_URL
为你的资源服务器地址。
javascript
# 克隆 UIKit
git clone https://github.com/easemob/easemob-uikit-uniapp.git
# 在你的 uni-app 项目根目录下执行以下命令,拷贝组件文件
mkdir -p ./ChatUIKit
# macOS
mv ${组件项目路径}/ChatUIKit/* ./ChatUIKit
# windows
move ${组件项目路径}/ChatUIKit/* .\ChatUIKit
3、添加依赖
环信即时通讯 IM Web SDK 4.10.0 及以上。
在项目根目录下执行以下命令,添加依赖:
javascript
npm init -y
npm i [email protected] [email protected] [email protected] --save
4、引入 ChatUIKit 初始化并设置通用样式。
在你的项目的App.vue
文件中引入 ChatUIKit
组件,并进行初始化。
要查看服务器域名配置,请点击这里 。
javascript
<script lang="ts">
import { ChatUIKit } from "./ChatUIKit";
import websdk from "easemob-websdk/uniApp/Easemob-chat";
import { EasemobChatStatic } from "easemob-websdk/Easemob-chat";
// 创建 IM 实例
const chat = new (websdk as unknown as EasemobChatStatic).connection({
appKey: '', // 应用的 App Key
isHttpDNS: false,
url: '', // 环信 websocket URL
apiUrl: '', // 环信 Restful API URL
delivery: true // 是否开启消息已送达回执
});
// 初始化 ChatUIKit
ChatUIKit.init({
chat, // 传入 IM 实例
config: {
theme: {
// 头像形状:圆形(circle)和方形(square)
avatarShape: "square"
},
isDebug: true // 是否开启调试模式
}
});
uni.$UIKit = ChatUIKit;
// 登录环信即时通讯 IM
const login = () => {
uni.$UIKit.chatStore.login({
user: "", // 用户 ID
accessToken: "" // 用户 Token
}).then(() => {
// 登录成功后,跳转会话列表页面
uni.navigateTo({
url: '/ChatUIKit/modules/Conversation/index'
})
});
}
// 退出登录
const logout = () => {
uni.$UIKit.chatStore.logout();
}
export default {
onLaunch: function () {
// 应用启动时,调用登录方法
login();
console.log("App Launch");
},
onShow: function () {
console.log("App Show");
// 在 onShow 中调用 ChatUIKit.onShow() 方法,主动监测 IM 连接状态
ChatUIKit.onShow();
},
onHide: function () {
console.log("App Hide");
}
};
</script>
<style>
/* 通用样式 */
html,body,page {
height: 100%;
width: 100%;
}
</style>
5、配置路由。
在你项目的 pages.json
文件中更新 pages
路由:
javascript
{
"pages": [
{
"path": "ChatUIKit/modules/Conversation/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/Chat/index",
"style": {
"navigationStyle": "custom",
// #ifdef MP-WEIXIN
"disableScroll": true,
// #endif
"app-plus": {
"bounce": "none",
"softinputNavBar": "none"
}
}
},
{
"path": "ChatUIKit/modules/ChatNew/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/GroupList/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/ConversationSearchList/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/VideoPreview/index",
"style": {
"navigationBarTitleText": "Video Preview",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/ContactList/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/ContactAdd/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/ContactRequestList/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/ContactSearchList/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
},
{
"path": "ChatUIKit/modules/GroupCreate/index",
"style": {
"navigationStyle": "custom",
"app-plus": {
"bounce": "none"
}
}
}
]
}
6、运行 Demo。
在 uni-app IDE 中,运行 Demo:
大功告成!~
参考文档: