之前整理过一篇 openfeign的快速使用。openfeign简化服务间调用-笔记-CSDN博客
今天补充一下一些个性化配置。
一 日志:
默认的openfeign不输出日志,想看日志需要单独配置下。
日志级别
java
public static enum Level {
NONE,
BASIC,
HEADERS,
FULL;
private Level() {
}
}
日志级别有以上枚举,测试选个FULL。
- NONE: 不记录任何日志,是 OpenFeign 默认日志级别(性能最佳,适用于生产环境)。
- BASIC: 仅记录请求方法、URL、响应状态码、执行时间(适用于生产环境追踪问题)。
- HEADERS: 在记录 BASIC 级别的基础上,记录请求和响应的 header 头部信息。
- FULL: 记录请求和响应的 header、body 和元数据(适用于开发和测试环境定位问题)
配置
新建一个配置文件,注意使用注解@Configuration是全局配置
java
package org.tuling.tlmalluser.config;
import feign.Logger;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
//@Configuration 使用@Configuration是全局配置
public class FeignConfig {
@Bean
public Logger.Level feignLoggerLevel(){
return Logger.Level.FULL;
}
}
feign调用方法configuration增加刚才的配置
java
package org.tuling.tlmalluser.feign;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.tuling.tlmallcommon.Result;
import org.tuling.tlmalluser.config.FeignConfig;
@FeignClient(name = "tlmall-order01",configuration = FeignConfig.class)
public interface OrderServiceFeignClient {
@GetMapping(value = "/order/getOrder")
Result<?> getorder(@RequestParam("userId") String userId);
}
测试代码:
java
@RestController
@RequestMapping("/user")
@Slf4j
public class UserController {
private Logger log = LoggerFactory.getLogger(getClass());
// @Autowired
// private RestTemplate restTemplate;
@Autowired
private OrderServiceFeignClient orderService;
@RequestMapping(value = "/getOrder")
public Result<?> getOrderByUserId(@RequestParam("userId") String userId) {
log.info("根据userId:"+userId+"查询订单信息");
// 方式1:restTemplate调用,url写死
//String url = "http://localhost:8060/order/getOrder?userId="+userId;
//方式2:利用@LoadBalanced,restTemplate需要添加@LoadBalanced注解
// String url = "http://tlmall-order01/order/getOrder?userId="+userId;
// Result result = restTemplate.getForObject(url,Result.class);
//方式3:openfeign
Result result = orderService.getorder(userId);
return result;
}
}
调整下日志级别:feign默认是debug,修改application.yml
logging:
level:
org.tuling.tlmalluser.feign: debug
调用:
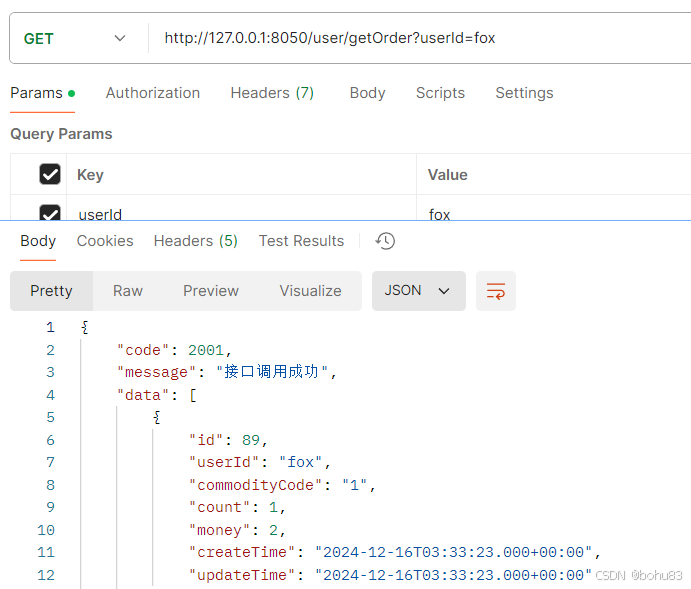
看日志输出
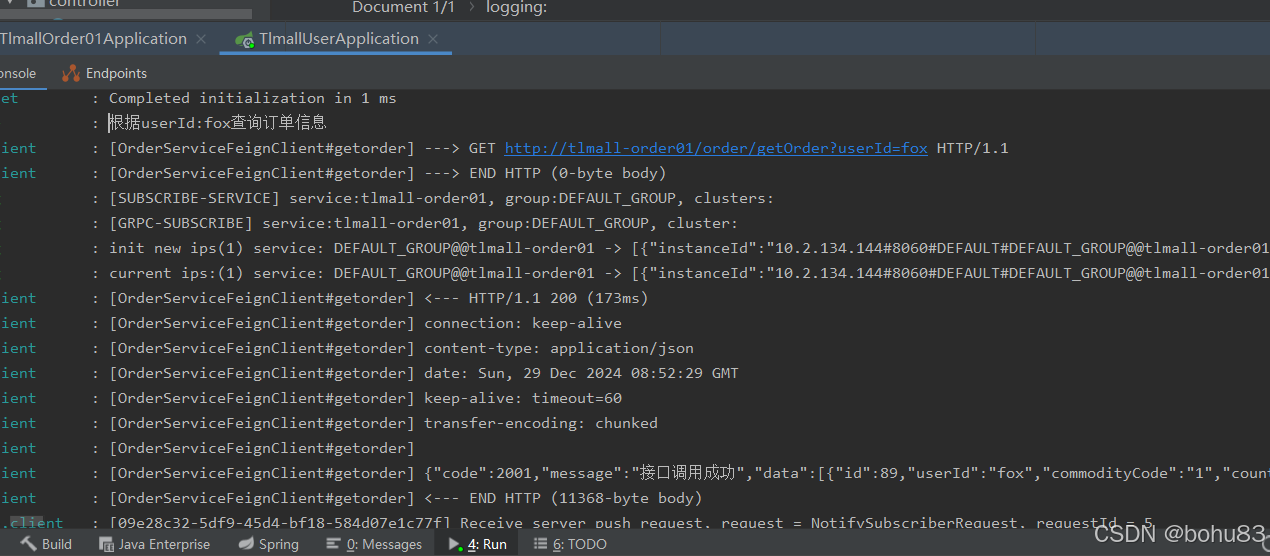
也可以配置文件实现
二 超时时间
官网介绍
1.3. Timeout Handling
We can configure timeouts on both the default and the named client. OpenFeign works with two timeout parameters:
connectTimeout
prevents blocking the caller due to the long server processing time.
readTimeout
is applied from the time of connection establishment and is triggered when returning the response takes too long.
代码配置
通过 Options 可以配置连接超时时间和读取超时时间。
java
package org.tuling.tlmalluser.config;
import feign.Logger;
import feign.Request;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
//@Configuration 使用@Configuration是全局配置
public class FeignConfig {
@Bean
public Logger.Level feignLoggerLevel(){
return Logger.Level.FULL;
}
/**
* 超时时间配置
*/
@Bean
public Request.Options options(){
return new Request.Options(2000,1000);
}
}
order 测试类增加耗时
Thread.sleep(3000);
重启order模块,user 模块。调用测试:
java.net.SocketTimeoutException: Read timed out
at java.base/sun.nio.ch.NioSocketImpl.timedRead(NioSocketImpl.java:288) ~[na:na]
at java.base/sun.nio.ch.NioSocketImpl.implRead(NioSocketImpl.java:314) ~[na:na]
配置文件
java
spring:
application:
name: tlmall-user
cloud:
nacos:
config:
server-addr: 127.0.0.1:8848
username: bohu
password: 123456
openfeign:
client:
config:
default:
# 日志级别
loggerLevel: basic
# 超时设置 1.5 秒超时
connectTimeout: 1500
readTimeout: 1500
注意,我测试用的版本是springboot 3, 网上之前老版本的不生效。
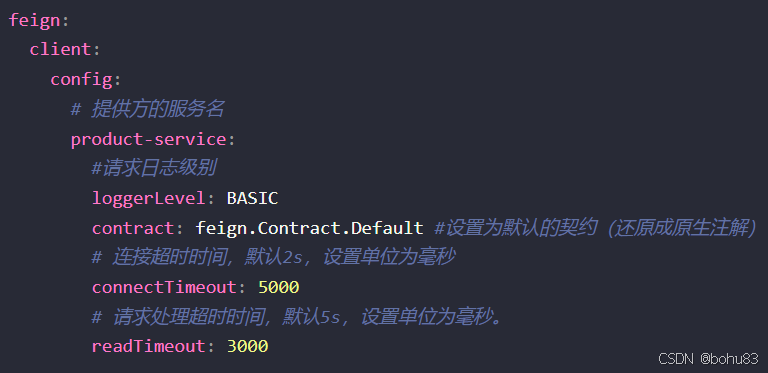
原码在org.springframework.cloud.openfeign.FeignClientProperties
java
@ConfigurationProperties("spring.cloud.openfeign.client")
public class FeignClientProperties {
private boolean defaultToProperties = true;
private String defaultConfig = "default";
private Map<String, FeignClientProperties.FeignClientConfiguration> config = new HashMap();
private boolean decodeSlash = true;
public FeignClientProperties() {
}
三 自定义拦截器
java
public class CustomFeignInterceptor implements RequestInterceptor {
Logger logger = LoggerFactory.getLogger(this.getClass());
@Override
public void apply(RequestTemplate requestTemplate) {
String testInfo = UUID.randomUUID().toString();
requestTemplate.header("test_info", testInfo);
logger.info("feign拦截器,info:{}",testInfo);
}
}
java
openfeign:
client:
config:
default:
# 日志级别
loggerLevel: basic
# 超时设置 1.5 秒超时
connectTimeout: 5000
readTimeout: 5000
request-interceptors: org.tuling.tlmalluser.interceptor.CustomFeignInterceptor
调用下接口,能看到拦截器日志。拦截器还是要结合具体业务来看。
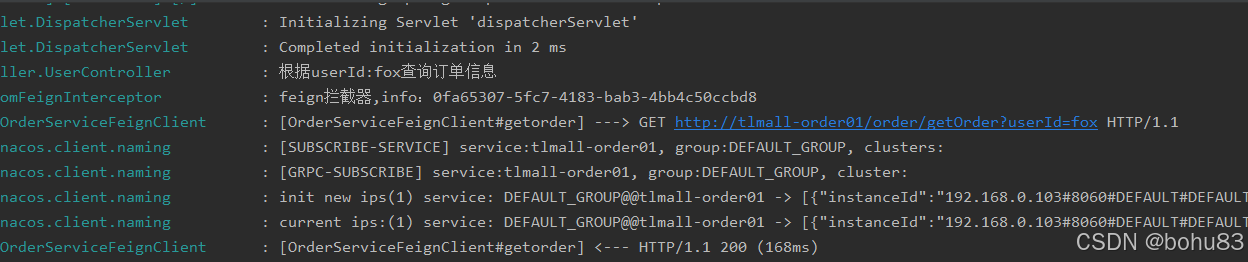