直接上代码:
cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace ProcessItems
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
// 启用拖放
treeViewItems.AllowDrop = true;
// 拖放进入事件
treeViewItems.DragEnter += new DragEventHandler(treeView_DragEnter);
// 拖放事件
treeViewItems.DragDrop += new DragEventHandler(treeView_DragDrop);
}
// 允许拖放的文件类型
private void treeView_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop))
{
e.Effect = DragDropEffects.Copy; // 或者其他效果
}
else
{
e.Effect = DragDropEffects.None;
}
}
// 处理拖放事件
private void treeView_DragDrop(object sender, DragEventArgs e)
{
string[] files = (string[])e.Data.GetData(DataFormats.FileDrop);
foreach (string file in files)
{
// 检查文件是否存在
if (/*File.Exists(file) || */Directory.Exists(file))
{
// 在这里添加文件到TreeView的逻辑
treeViewItems.Nodes.Add(new TreeNode(file));
}
}
}
private void buttonOK_Click(object sender, EventArgs e)
{
var items = GetAllTreeViewItems(treeViewItems);
foreach (var item in items)
{
MoveSingleSubdirectory(item);
}
}
// 递归函数,用于获取TreeView中的所有节点文本
private static void GetTreeViewItems(TreeNodeCollection nodes, List<string> itemList)
{
foreach (TreeNode node in nodes)
{
itemList.Add(node.Text); // 添加当前节点的文本到列表中
if (node.Nodes.Count > 0)
{
// 如果当前节点有子节点,则递归调用此函数来获取子节点的文本
GetTreeViewItems(node.Nodes, itemList);
}
}
}
// 公共方法,供外部调用以获取TreeView中的所有项
public static List<string> GetAllTreeViewItems(TreeView treeView)
{
List<string> itemList = new List<string>();
GetTreeViewItems(treeView.Nodes, itemList);
return itemList;
}
static void MoveSingleSubdirectory(string dirPath)
{
try
{
// 检查指定的目录是否存在
if (!Directory.Exists(dirPath))
{
Console.WriteLine($"目录 '{dirPath}' 不存在。");
return;
}
// 获取该目录中的所有子目录
string[] subDirs = Directory.GetDirectories(dirPath);
// 如果子目录数量不等于1,直接返回
if (subDirs.Length != 1)
{
Console.WriteLine($"目录 '{dirPath}' 中的子目录数量不是1。");
return;
}
// 获取唯一的子目录路径
string subDirPath = subDirs[0];
// 获取上一级目录路径
string parentDirPath = Directory.GetParent(dirPath).FullName;
// 获取当前目录的名字
string subDirName = new DirectoryInfo(dirPath).Name;
// 目标路径初始为上一级目录加上子目录的名字
string destPath = Path.Combine(parentDirPath, subDirName);
// 检查是否存在同名目录,如果存在则添加数字进行重命名
int counter = 1;
while (Directory.Exists(destPath))
{
destPath = Path.Combine(parentDirPath, $"{subDirName}_{counter}");
counter++;
}
// 移动子目录到上一级目录
Directory.Move(subDirPath, destPath);
//删除空目录
DeleteIfEmpty(parentDirPath);
Console.WriteLine($"子目录已成功移动到: {destPath}");
}
catch (Exception ex)
{
Console.WriteLine($"操作过程中出现错误: {ex.Message}");
}
}
public static bool DeleteIfEmpty(string directoryPath)
{
try
{
// 检查目录是否存在
if (!Directory.Exists(directoryPath))
{
Console.WriteLine($"目录 '{directoryPath}' 不存在。");
return false;
}
// 获取目录中的所有文件和子目录
string[] files = Directory.GetFiles(directoryPath);
string[] subDirs = Directory.GetDirectories(directoryPath);
// 如果目录中没有文件也没有子目录,则认为是空目录
if (files.Length == 0 && subDirs.Length == 0)
{
// 删除空目录
Directory.Delete(directoryPath);
Console.WriteLine($"空目录 '{directoryPath}' 已被删除。");
return true;
}
else
{
Console.WriteLine($"目录 '{directoryPath}' 不是空目录。");
return false;
}
}
catch (Exception ex)
{
// 捕获并处理可能出现的异常
Console.WriteLine($"操作过程中出现错误: {ex.Message}");
return false;
}
}
}
}
实现效果,可以用于整理特殊结构的目录。
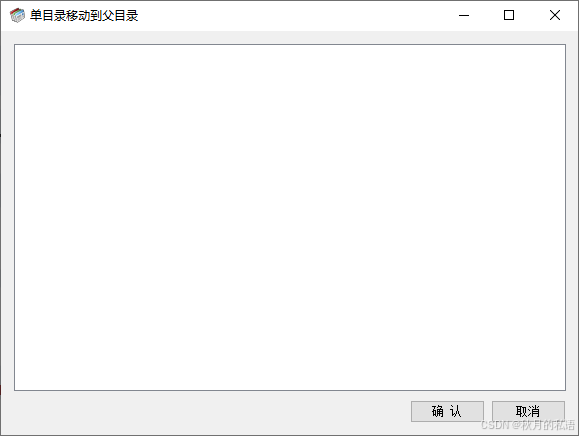