一、显示在右下角
1、timer.cpp
cpp
#include "timer.h"
#include "ui_timer.h"
#include <QStatusBar>
#include <QDateTime>
#include <QMenuBar>
Timer::Timer(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::Timer)
{
ui->setupUi(this);
// 创建状态栏
QStatusBar *statusBar = new QStatusBar(this);
this->setStatusBar(statusBar);
// 创建用于显示时间的标签
timeLabel = new QLabel(this);
statusBar->addPermanentWidget(timeLabel);
// 创建计时器,每秒更新一次
timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &Timer::updateTime);
timer->start(1000); // 设置每1000毫秒(1秒)触发一次
// 初始化时间显示
updateTime();
}
Timer::~Timer()
{
delete ui;
}
void Timer::updateTime()
{
timeLabel->setText(QDateTime::currentDateTime().toString("yyyy-MM-dd hh:mm:ss"));
}
2、timer.h
cpp
#ifndef TIMER_H
#define TIMER_H
#include <QMainWindow>
#include <QTimer>
#include <QLabel>
namespace Ui {
class Timer;
}
class Timer : public QMainWindow
{
Q_OBJECT
public:
explicit Timer(QWidget *parent = nullptr);
~Timer();
private slots:
void updateTime();
private:
Ui::Timer *ui;
QTimer *timer;
QLabel *timeLabel;
};
#endif
3、 演示效果
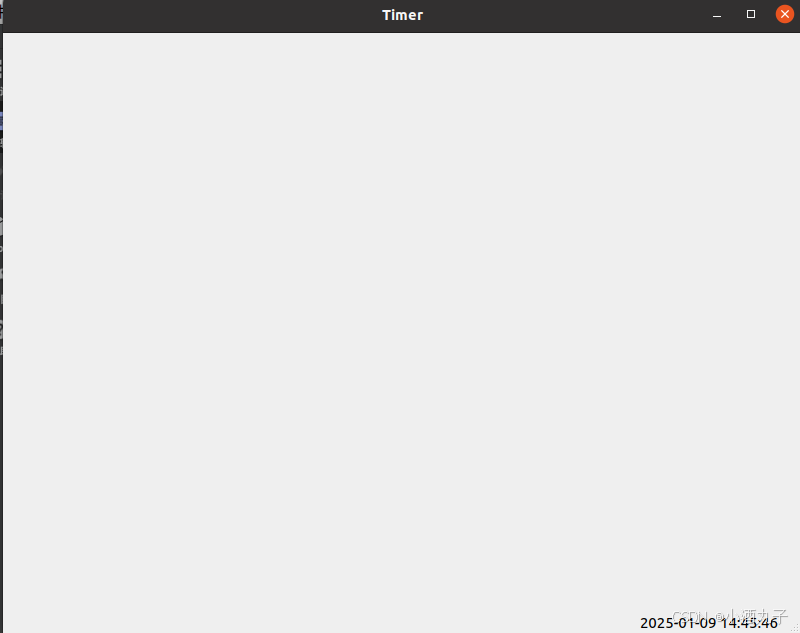
二、显示在左上角
1、timer.cpp
cpp
#include "timer.h"
#include "ui_timer.h"
#include <QMenuBar>
#include <QDateTime>
#include <QTimer>
Timer::Timer(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::Timer)
{
ui->setupUi(this);
// 创建菜单栏
menuBar = new QMenuBar(this);
setMenuBar(menuBar);
// 创建一个动作来显示时间
timeAction = new QAction(this);
timeAction->setText("00:00:00");
menuBar->addAction(timeAction);
// 创建计时器,每秒更新一次
timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, [this](){
timeAction->setText(QDateTime::currentDateTime().toString("yyyy-MM-dd hh:mm:ss"));
});
timer->start(1000); // 设置每1000毫秒(1秒)触发一次
}
Timer::~Timer()
{
delete ui;
}
2、timer.h
cpp
#ifndef TIMER_H
#define TIMER_H
#include <QMainWindow>
#include <QTimer>
#include <QMenuBar>
namespace Ui {
class Timer;
}
class Timer : public QMainWindow
{
Q_OBJECT
public:
explicit Timer(QWidget *parent = nullptr);
~Timer();
private:
Ui::Timer *ui;
QTimer *timer;
QMenuBar *menuBar;
QAction *timeAction; // 添加这个成员变量
};
#endif
3、运行效果
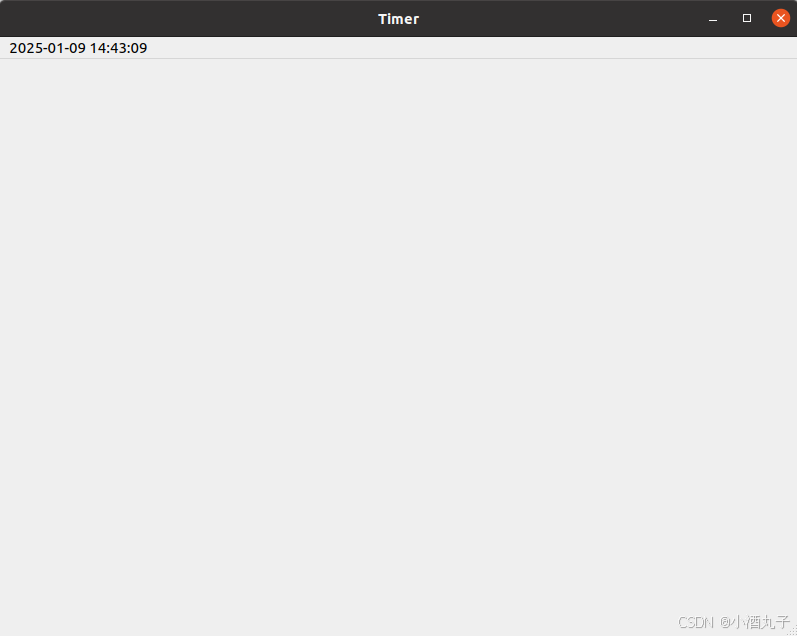