When your interviewer asks you to write "Hello World" using C, can you do as the following figure shows?
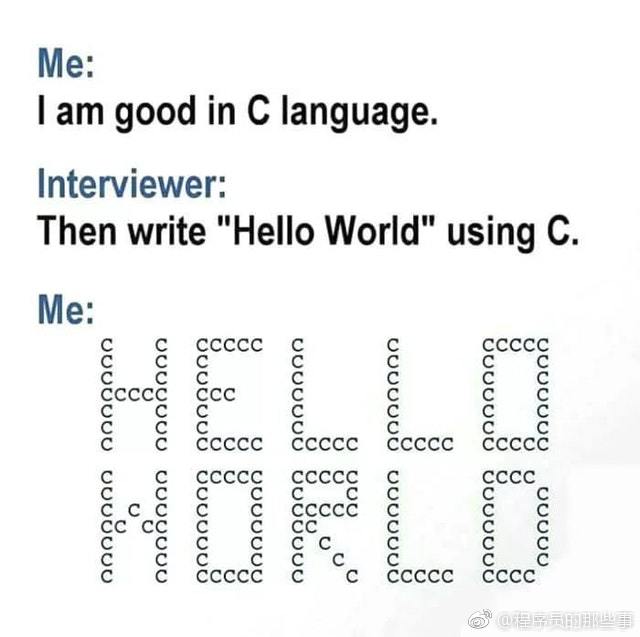
Input Specification:
Each input file contains one test case. For each case, the first part gives the 26 capital English letters A-Z, each in a 7×5 matrix of C
's and .
's. Then a sentence is given in a line, ended by a return. The sentence is formed by several words (no more than 10 continuous capital English letters each), and the words are separated by any characters other than capital English letters.
It is guaranteed that there is at least one word given.
Output Specification:
For each word, print the matrix form of each of its letters in a line, and the letters must be separated by exactly one column of space. There must be no extra space at the beginning or the end of the word.
Between two adjacent words, there must be a single empty line to separate them. There must be no extra line at the beginning or the end of the output.
Sample Input:
..C..
.C.C.
C...C
CCCCC
C...C
C...C
C...C
CCCC.
C...C
C...C
CCCC.
C...C
C...C
CCCC.
.CCC.
C...C
C....
C....
C....
C...C
.CCC.
CCCC.
C...C
C...C
C...C
C...C
C...C
CCCC.
CCCCC
C....
C....
CCCC.
C....
C....
CCCCC
CCCCC
C....
C....
CCCC.
C....
C....
C....
CCCC.
C...C
C....
C.CCC
C...C
C...C
CCCC.
C...C
C...C
C...C
CCCCC
C...C
C...C
C...C
CCCCC
..C..
..C..
..C..
..C..
..C..
CCCCC
CCCCC
....C
....C
....C
....C
C...C
.CCC.
C...C
C..C.
C.C..
CC...
C.C..
C..C.
C...C
C....
C....
C....
C....
C....
C....
CCCCC
C...C
C...C
CC.CC
C.C.C
C...C
C...C
C...C
C...C
C...C
CC..C
C.C.C
C..CC
C...C
C...C
.CCC.
C...C
C...C
C...C
C...C
C...C
.CCC.
CCCC.
C...C
C...C
CCCC.
C....
C....
C....
.CCC.
C...C
C...C
C...C
C.C.C
C..CC
.CCC.
CCCC.
C...C
CCCC.
CC...
C.C..
C..C.
C...C
.CCC.
C...C
C....
.CCC.
....C
C...C
.CCC.
CCCCC
..C..
..C..
..C..
..C..
..C..
..C..
C...C
C...C
C...C
C...C
C...C
C...C
.CCC.
C...C
C...C
C...C
C...C
C...C
.C.C.
..C..
C...C
C...C
C...C
C.C.C
CC.CC
C...C
C...C
C...C
C...C
.C.C.
..C..
.C.C.
C...C
C...C
C...C
C...C
.C.C.
..C..
..C..
..C..
..C..
CCCCC
....C
...C.
..C..
.C...
C....
CCCCC
HELLO~WORLD!
Sample Output:
C...C CCCCC C.... C.... .CCC.
C...C C.... C.... C.... C...C
C...C C.... C.... C.... C...C
CCCCC CCCC. C.... C.... C...C
C...C C.... C.... C.... C...C
C...C C.... C.... C.... C...C
C...C CCCCC CCCCC CCCCC .CCC.
C...C .CCC. CCCC. C.... CCCC.
C...C C...C C...C C.... C...C
C...C C...C CCCC. C.... C...C
C.C.C C...C CC... C.... C...C
CC.CC C...C C.C.. C.... C...C
C...C C...C C..C. C.... C...C
C...C .CCC. C...C CCCCC CCCC.
题目大意:首先给出26个英文大写字母A-Z,每个字母用7×5的、由C和.组成的矩阵构成。最后一行给出一个句子,以回车结束。句子是由若干个单词(每个包含不超过10个连续的大写英文字母)组成的,单词间以任何非大写英文字母分隔。题目保证至少给出一个单词。对于每个单词,将其中每个字母用矩阵形式在一行中输出,字母间有一列空格分隔。单词的首尾不得有多余空格。相邻的两个单词间必须有一空行分隔。输出的首尾不得有多余空行。
分析:注意任何非大写英文字母分隔是指除了换行的其它任意字符,所以读入要用fgets或者gets。然后注意输出格式。模拟即可。
cpp
#include<algorithm>
#include <iostream>
#include <cstdlib>
#include <cstring>
#include <string>
#include <vector>
#include <cstdio>
#include <queue>
#include <stack>
#include <ctime>
#include <cmath>
#include <map>
#include <set>
#define INF 0xffffffff
#define db1(x) cout<<#x<<"="<<(x)<<endl
#define db2(x,y) cout<<#x<<"="<<(x)<<", "<<#y<<"="<<(y)<<endl
#define db3(x,y,z) cout<<#x<<"="<<(x)<<", "<<#y<<"="<<(y)<<", "<<#z<<"="<<(z)<<endl
#define db4(x,y,z,r) cout<<#x<<"="<<(x)<<", "<<#y<<"="<<(y)<<", "<<#z<<"="<<(z)<<", "<<#r<<"="<<(r)<<endl
#define db5(x,y,z,r,w) cout<<#x<<"="<<(x)<<", "<<#y<<"="<<(y)<<", "<<#z<<"="<<(z)<<", "<<#r<<"="<<(r)<<", "<<#w<<"="<<(w)<<endl
using namespace std;
int main(void)
{
#ifdef test
freopen("in.txt","r",stdin);
//freopen("in.txt","w",stdout);
clock_t start=clock();
#endif //test
char alp[30][10][10];
for(int i=0;i<26;++i)
for(int j=0;j<7;++j)
scanf("%s\n",alp[i][j]);
int f=0,flag=0;
char s[1000000];fgets(s,1000000,stdin);
int len=strlen(s);s[len-1]=0;len--;
// db1(s);
int i=0,l=0;
for(;s[i];++i)
if(s[i]>='A'&&s[i]<='Z')break;
for(l=i;i<len;++i)
{
if((s[i]<'A'||s[i]>'Z'))
{
// if(s[i]==0)break;
if(l==i)
{
l++;continue;
}
// db3(i,s[i],l);
if(flag)printf("\n");
// db2(l,i);
for(int j=0;j<7;++j)
{
int t=0;flag++;
for(int k=l;k<i;++k)
{
if(!t)printf("%s",alp[s[k]-'A'][j]),t++;
else printf(" %s",alp[s[k]-'A'][j]);
}
if(t)printf("\n");
}
l=i+1;
}
}
if(s[i-1]>='A'&&s[i-1]<='Z')
{
if(flag)printf("\n");
for(int j=0;j<7;++j)
{
int t=0;flag++;
for(int k=l;k<i;++k)
{
if(!t)printf("%s",alp[s[k]-'A'][j]),t++;
else printf(" %s",alp[s[k]-'A'][j]);
}
if(t)printf("\n");
}
}
#ifdef test
clockid_t end=clock();
double endtime=(double)(end-start)/CLOCKS_PER_SEC;
printf("\n\n\n\n\n");
cout<<"Total time:"<<endtime<<"s"<<endl; //s为单位
cout<<"Total time:"<<endtime*1000<<"ms"<<endl; //ms为单位
#endif //test
return 0;
}