实训9 数据存储和访问
一、【实训目的】
1、 SharedPreferences存储数据;
2、 借助Java的I/O体系实现文件的存储,
3、使用Android内置的轻量级数据库SQLite存储数据;
二、【实训内容】
1、实现下图所示的界面,实现以下功能:
1)用SharedPreferences类,当点击"写入xml文件"按钮, 把"输入你想写入内容"控件中的数据写入到login.xml文件中;
2)用SharedPreferences类,当点击"读取内容"按钮, 把login.xml文件中的数据读到到一个TextView中,并显示内容;
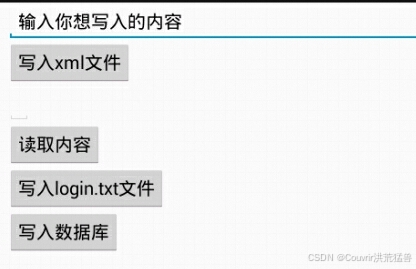
具体步骤:
MainActivity源文件:
java
package com.example.hahah;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import android.os.Bundle;
import android.app.Activity;
import android.content.Context;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
private Button write, read;
private EditText writeText, readText;
private String fileName = "context.txt";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
write = (Button) findViewById(R.id.btnwritexml);
read = (Button) findViewById(R.id.btnreadxml);
writeText = (EditText) findViewById(R.id.writecontent);
readText = (EditText) findViewById(R.id.readcontent);
write.setOnClickListener(new OnClickListener() {
public void onClick(View arg0) {
write(writeText.getText().toString());
}
});
read.setOnClickListener(new OnClickListener() {
public void onClick(View arg0) {
readText.setText(read());
}
});
}
public String read() {
StringBuilder sbBuilder = new StringBuilder("");
byte[] buffer = new byte[64];
int hasRead;
try {
FileInputStream fis = openFileInput(fileName);
while ((hasRead = fis.read(buffer)) != -1) {
sbBuilder.append(new String(buffer, 0, hasRead));
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return sbBuilder.toString();
}
public void write(String str) {
try {
FileOutputStream fos = openFileOutput(fileName, Context.MODE_APPEND);
PrintStream ps = new PrintStream(fos);
ps.print(str);
ps.close();
try {
fos.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} catch (FileNotFoundException ex) {
ex.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Activity_main源代码:
java
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:stretchColumns="2">
<TableRow>
<EditText
android:id="@+id/writecontent"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:hint="输入你想写入的内容" />
</TableRow>>
<TableRow>
<Button
android:id="@+id/btnwritexml"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="0"
android:text="写入xml文件" />
</TableRow>
<TableRow>
<EditText
android:id="@+id/readcontent"
android:layout_width="0dp"
android:layout_height="wrap_content"/>
</TableRow>>
<TableRow>
<Button
android:id="@+id/btnreadxml"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="0"
android:text="读取内容" />
</TableRow>
<TableRow>
<Button
android:id="@+id/btnwritetxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="0"
android:text="写入login.txt文件" />
</TableRow>
<TableRow>
<Button
android:id="@+id/btnwritesql"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="0"
android:text="写入数据库" />
</TableRow>
</TableLayout>
运行结果截图:
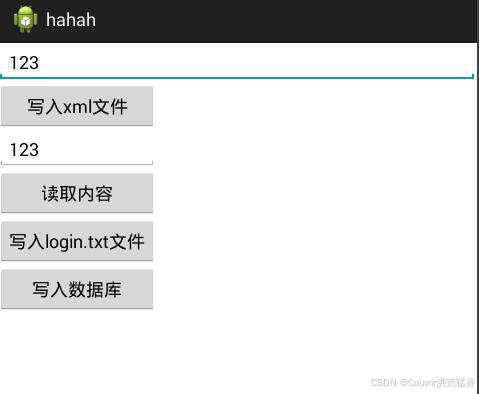
附注:该专栏是博主上学时的实训项目,可供访客练习与参考。代码质量不是很好,但能实现,仅供参考!